SIMD (Single Instruction, Multiple Data) in C++ allows you to perform the same operation on multiple data points simultaneously, leveraging vectorization for enhanced performance.
Here's a simple code snippet demonstrating SIMD using the AVX intrinsics for vector addition:
#include <immintrin.h>
#include <iostream>
int main() {
__m256 a = _mm256_set1_ps(1.0f); // Set all elements to 1.0
__m256 b = _mm256_set1_ps(2.0f); // Set all elements to 2.0
__m256 result = _mm256_add_ps(a, b); // Perform SIMD addition
float* res = (float*)&result;
for(int i = 0; i < 8; i++) {
std::cout << res[i] << " "; // Output the result
}
return 0;
}
What is SIMD?
SIMD stands for Single Instruction, Multiple Data, a parallel processing architecture that allows a single operation to be applied simultaneously across multiple data points. This capability is crucial in modern computing, where performance and efficiency are paramount. In contrast to other computing methods, such as MIMD (Multiple Instruction, Multiple Data), SIMD focuses on executing the same instruction on multiple pieces of data at once, leading to significant performance improvements in data-heavy applications.
The adoption of SIMD techniques allows developers to tap into the power of modern CPUs and GPUs efficiently, offering enhanced performance in various domains, including gaming, graphics processing, and scientific computing.
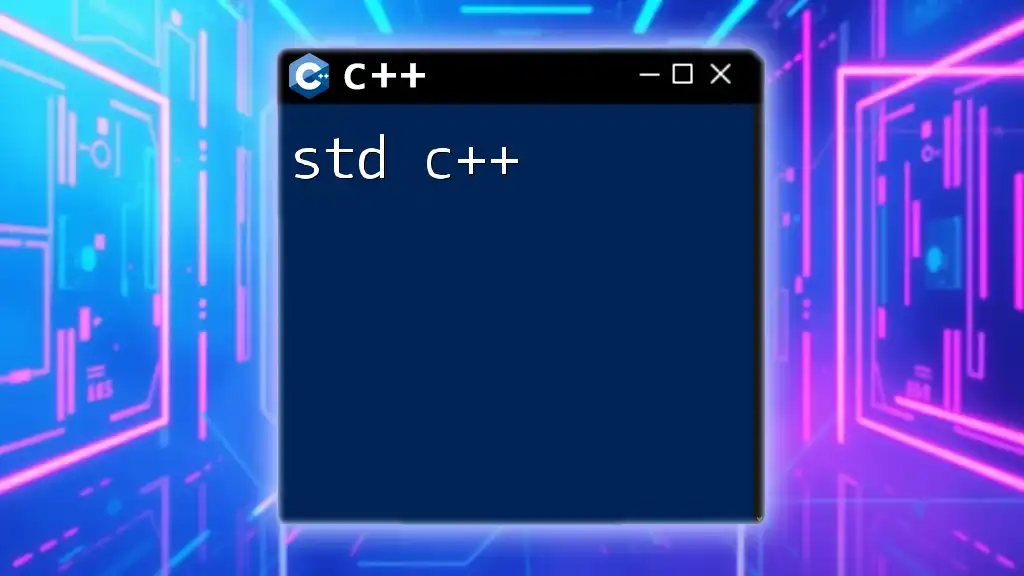
Benefits of Using SIMD in C++
The implementation of SIMD in C++ can lead to several substantial benefits, including:
- Performance improvements: SIMD enables the execution of vectorized code, resulting in faster processing times, especially for large data sets.
- Efficient resource utilization: By leveraging hardware capabilities, SIMD reduces the computational load and optimizes the use of available resources.
- Versatile applications: SIMD is widely applicable across various fields, including machine learning, signal processing, and rendering in graphics.
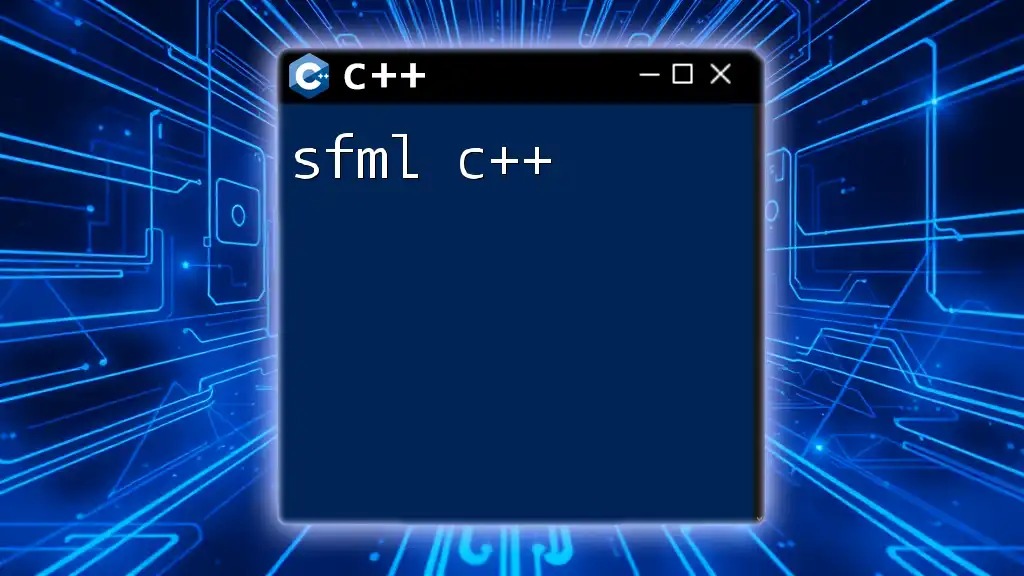
Understanding the Basics of SIMD
How SIMD Works
The core principle behind SIMD lies in its ability to process multiple data elements simultaneously using the same instruction. This design takes advantage of parallelism, significantly increasing throughput for data-intensive tasks.
Vector operations are central to SIMD. Instead of performing operations on individual data points, SIMD handles vectors (or arrays) of data, executing the same operation across an entire set in a single instruction. This approach allows for more efficient execution compared to scalar processing of each individual data point.
SIMD Architectures
Several SIMD architectures are widely recognized in both CPU and GPU designs. Key SIMD extensions to be aware of in C++ include:
- SSE (Streaming SIMD Extensions): Introduced by Intel, SSE supports various data types and provides a range of instructions.
- AVX (Advanced Vector Extensions): An enhancement over SSE, AVX allows for wider SIMD processing, utilizing 256-bit registers.
- AVX2 and AVX512: Further extensions that provide even more advanced capabilities and performance improvements.
When working with SIMD operations, being aware of the specific hardware architecture is crucial, as it will determine the supported instruction sets and overall performance.
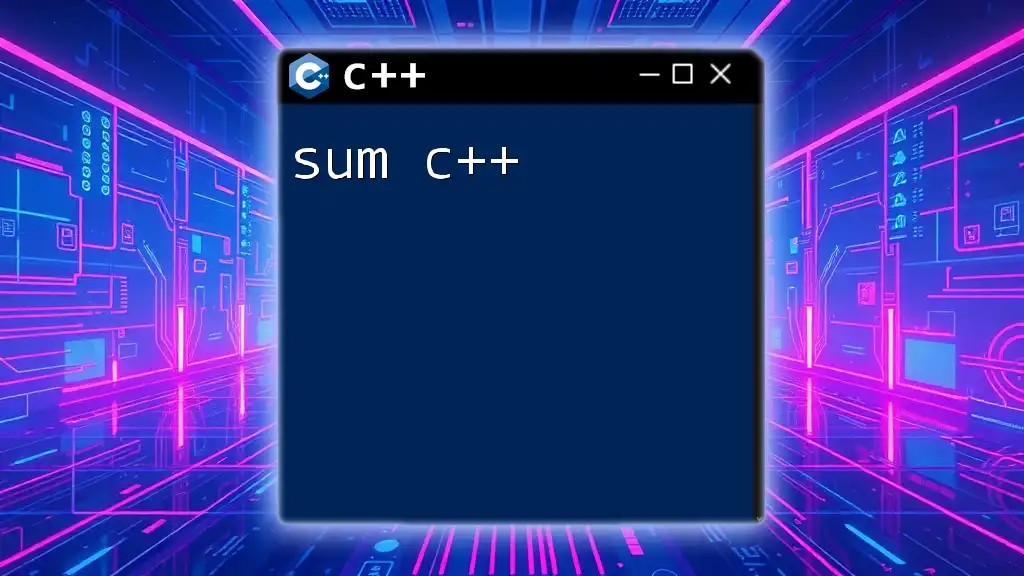
Getting Started with SIMD in C++
Enabling SIMD in Your C++ Project
To enable SIMD capabilities in a C++ project, it’s essential to ensure that your compiler supports the desired extensions. Most modern compilers, such as GCC and Clang, include flags to enable SIMD optimizations. For example, using `-march=native` allows the compiler to use instructions optimized for the current architecture.
Including the appropriate libraries is also necessary; typically, header files like `<xmmintrin.h>` or `<immintrin.h>` are included to access the available SIMD operations.
Basic SIMD Operations in C++
To illustrate how SIMD can be leveraged in C++, let's explore a simple yet powerful example: vector addition. Here is a code snippet that demonstrates how to perform vector addition using SIMD:
#include <immintrin.h>
void addVectors(const float* a, const float* b, float* c, int n) {
for (int i = 0; i < n; i += 4) {
__m128 vecA = _mm_loadu_ps(&a[i]);
__m128 vecB = _mm_loadu_ps(&b[i]);
__m128 vecC = _mm_add_ps(vecA, vecB);
_mm_storeu_ps(&c[i], vecC);
}
}
In this snippet, the `_mm_loadu_ps` function is used to load four single-precision floating-point values, and `_mm_add_ps` performs the addition. The result is then stored in the output vector `c`.
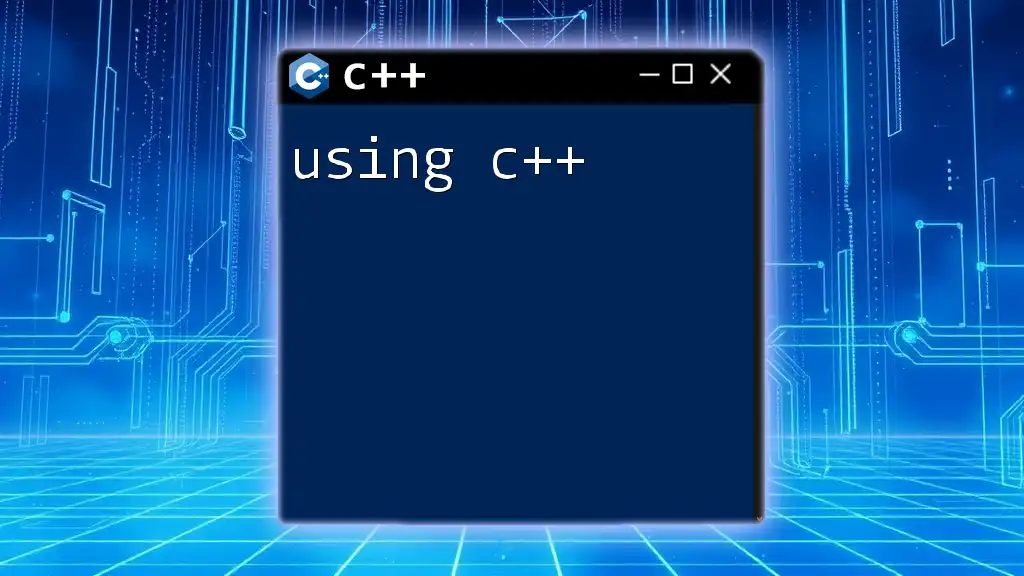
Advanced SIMD Techniques
Optimizing Performance with SIMD
To maximize the performance when using SIMD, aligning data in memory is crucial. Aligned data allows the CPU to access memory more efficiently. Techniques like loop unrolling can also be employed; by manually expanding loop iterations, the compiler can better optimize the workload, enhancing SIMD performance.
Common SIMD Libraries and Frameworks
Several powerful libraries are available to facilitate SIMD programming:
- Intel Intrinsics: A low-level library allowing direct access to SIMD instructions for Intel processors.
- SIMD Everywhere: A standardized approach to SIMD that promotes portability across various hardware architectures.
When choosing a library, consider factors like compatibility with your target hardware and the level of abstraction you're comfortable with.
Implementing Advanced SIMD Operations
Beyond basic operations, SIMD can efficiently handle complex arithmetic, such as multiplication. Here’s a code example that performs SIMD-based vector multiplication:
void multiplyVectors(const float* a, const float* b, float* c, int n) {
for (int i = 0; i < n; i += 4) {
__m128 vecA = _mm_loadu_ps(&a[i]);
__m128 vecB = _mm_loadu_ps(&b[i]);
__m128 vecC = _mm_mul_ps(vecA, vecB);
_mm_storeu_ps(&c[i], vecC);
}
}
In this example, the `_mm_mul_ps` function efficiently computes the element-wise product of two vectors.
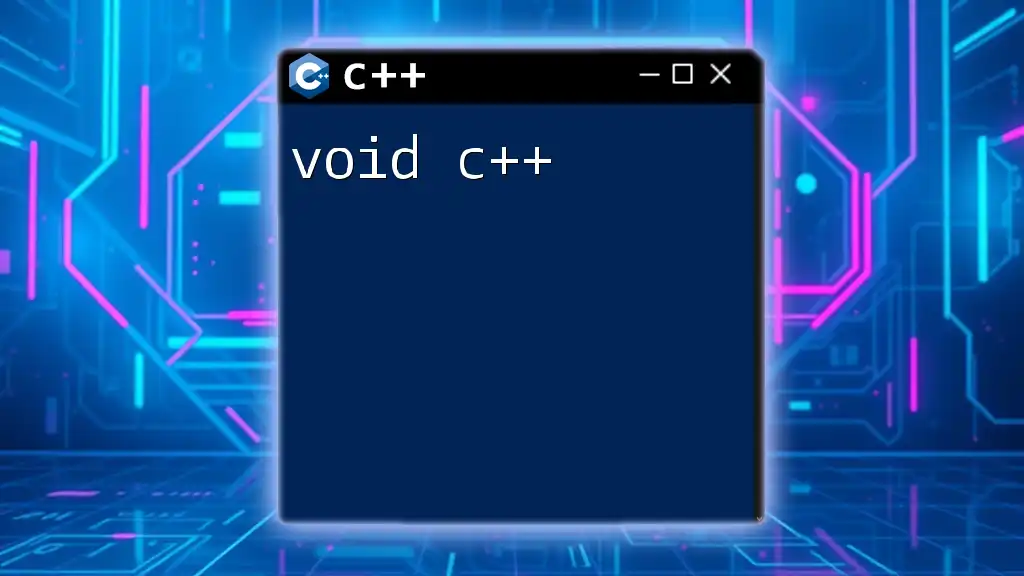
SIMD in Real-World Applications
Case Study: Gaming Performance Enhancements
In gaming, performance is vital for creating immersive experiences. SIMD techniques are frequently used in game engines to manage large quantities of data, such as textures, animations, and physics calculations. By applying SIMD operations, developers can achieve substantial performance gains, allowing for smoother gameplay and enhanced graphics.
Case Study: Image Processing
Another compelling application of SIMD is in image processing. By leveraging parallelism, developers can apply transformations, filters, and manipulations to entire images rapidly. Here’s an example of using SIMD for grayscale image conversion:
void grayscaleImage(const uint8_t* src, uint8_t* dst, int width, int height) {
for (int i = 0; i < width * height; i += 4) {
__m128i pixel = _mm_loadu_si128((__m128i*)&src[i * 3]);
__m128i r = _mm_shuffle_epi8(pixel, _mm_setr_epi8(0, 0, 0, 0, 1, 1, 1, 1, 2, 2, 2, 2, 3, 3, 3, 3));
__m128i g = _mm_shuffle_epi8(pixel, _mm_setr_epi8(0, 0, 0, 0, 4, 4, 4, 4, 8, 8, 8, 8, 12, 12, 12, 12));
__m128i b = _mm_shuffle_epi8(pixel, _mm_setr_epi8(0, 0, 0, 0, 2, 2, 2, 2, 6, 6, 6, 6, 10, 10, 10, 10));
__m128i gray = _mm_add_epi8(r, _mm_add_epi8(g, b));
gray = _mm_srli_epi16(gray, 2); // Average
_mm_storeu_si128((__m128i*)&dst[i], gray);
}
}
This code processes pixels in parallel, achieving efficient grayscale conversion by manipulating multiple values simultaneously.
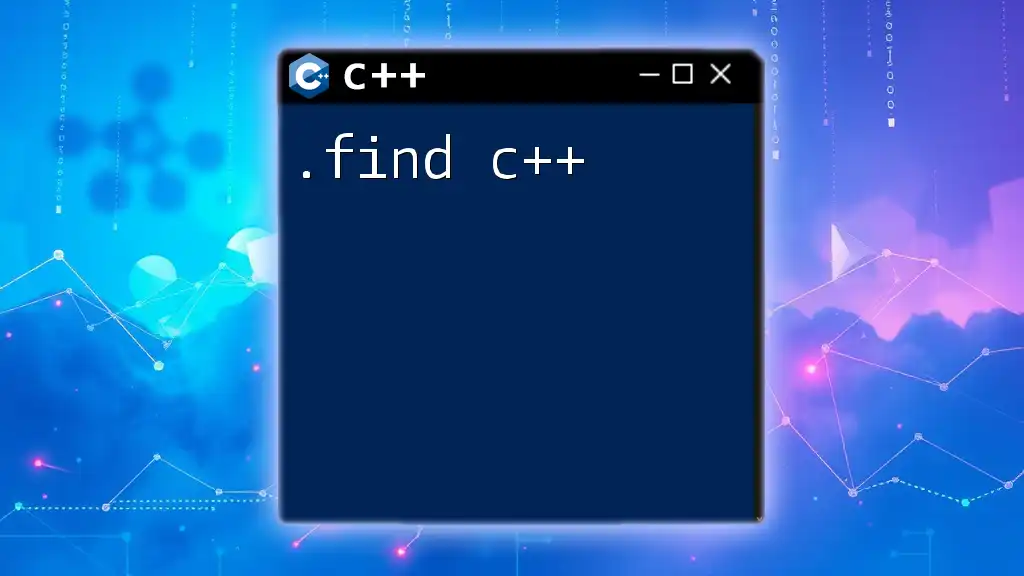
Best Practices for SIMD in C++
Writing Maintainable SIMD Code
When working with SIMD, it’s essential to prioritize code maintainability. Adopting naming conventions and structuring code logically help improve readability. Implementing reusable functions and avoiding deep nesting of SIMD operations can also enhance understanding for future development.
Debugging SIMD Performance Issues
To identify performance bottlenecks when using SIMD, developers can leverage profiling tools to analyze the execution time of different operations. Identifying and addressing inefficient data movement or misaligned data can lead to meaningful performance gains.
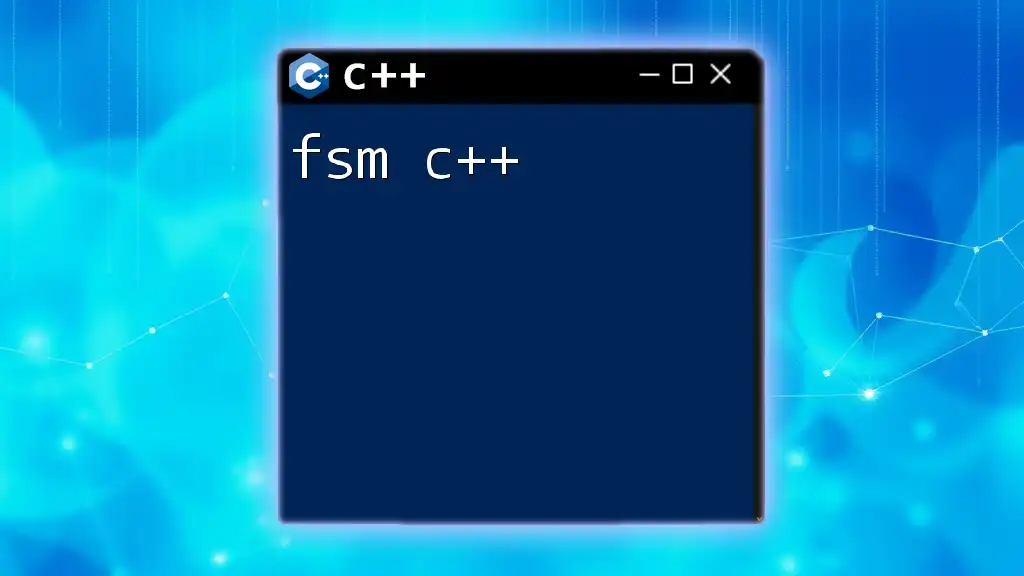
Conclusion
As the landscape of computing continues to evolve, SIMD remains a pivotal technique for enhancing the performance of C++ applications. Being abreast of emerging trends and technologies within SIMD will allow developers to harness its full potential, ensuring efficient and robust applications across diverse domains.
Additional Resources
For those looking to delve deeper into SIMD in C++, consider exploring recommended readings, online courses, and community forums. Engaging with the developer community will foster growth and deepen your understanding of this powerful programming paradigm.