SFML (Simple and Fast Multimedia Library) is a versatile multimedia library for C++ that simplifies the process of creating graphics, audio, and windowing applications, allowing for quick game and application development.
Here's a basic example of setting up a window using SFML in C++:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "SFML Window");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.display();
}
return 0;
}
What is SFML?
The Simple and Fast Multimedia Library (SFML) is a powerful and easy-to-use multimedia library designed for developers working with C++. It provides a comprehensive suite of features ideal for building games and other multimedia applications. SFML is particularly attractive for beginners due to its clean and intuitive API and its portability across various operating systems, including Windows, macOS, and Linux.
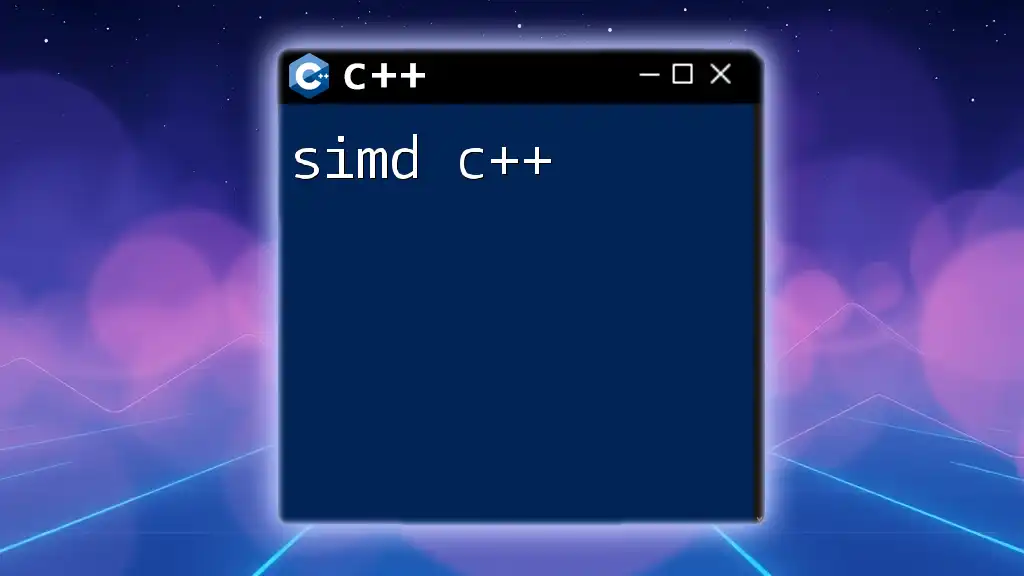
Why Use SFML?
Choosing SFML as your multimedia library has several advantages:
- Cross-Platform Capabilities: Write your code once and run it across multiple platforms without worrying about compilation issues.
- Simple Interface: It offers a straightforward and user-friendly interface, making it easier for newcomers to pick up.
- Extensive Features: SFML includes functionalities for graphics, audio, network communication, and system interaction, allowing developers to create rich applications.
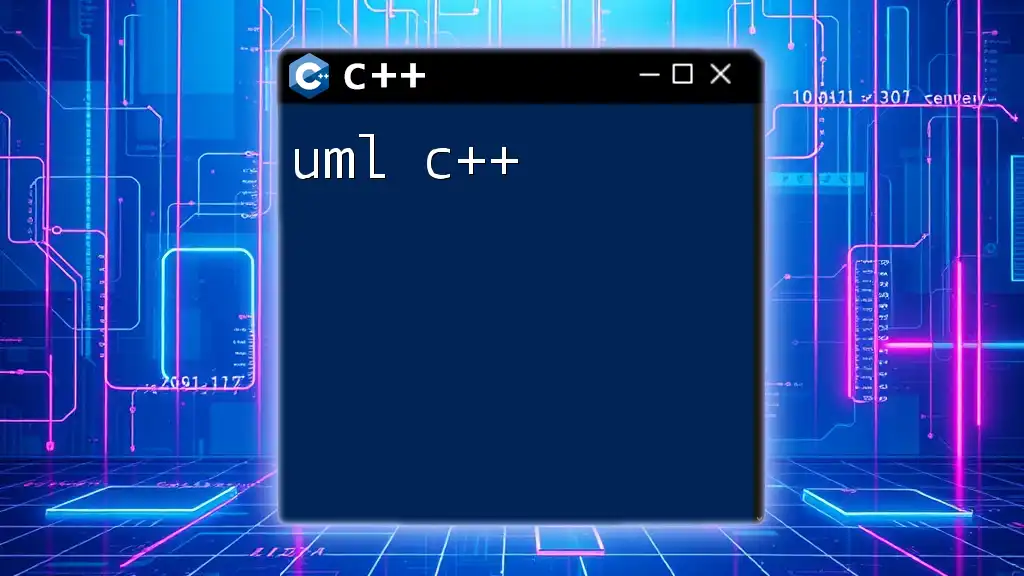
Setting Up SFML
Prerequisites
Before you can dive into developing applications with SFML C++, you need to ensure you have certain prerequisites:
- A compatible C++ compiler (GCC, Clang, Visual Studio).
- A build system such as CMake or your preferred IDE set up and ready for use.
Installing SFML
Installing SFML will vary depending on your operating system. For instance:
-
Windows: You can use the Package Manager vcpkg for a seamless installation:
vcpkg install sfml
-
macOS: Use Homebrew:
brew install sfml
-
Linux distributions can usually install SFML via their respective package managers. For Ubuntu, you would execute:
sudo apt-get install libsfml-dev
Setting Up Your Development Environment
Once SFML is installed, configuring your development environment is the next step. This includes:
- Adjusting your IDE or editor settings to include the SFML headers and linking against its libraries.
- For example, in Visual Studio, you can set the additional include directories under Project Properties → C/C++ → General → Additional Include Directories.
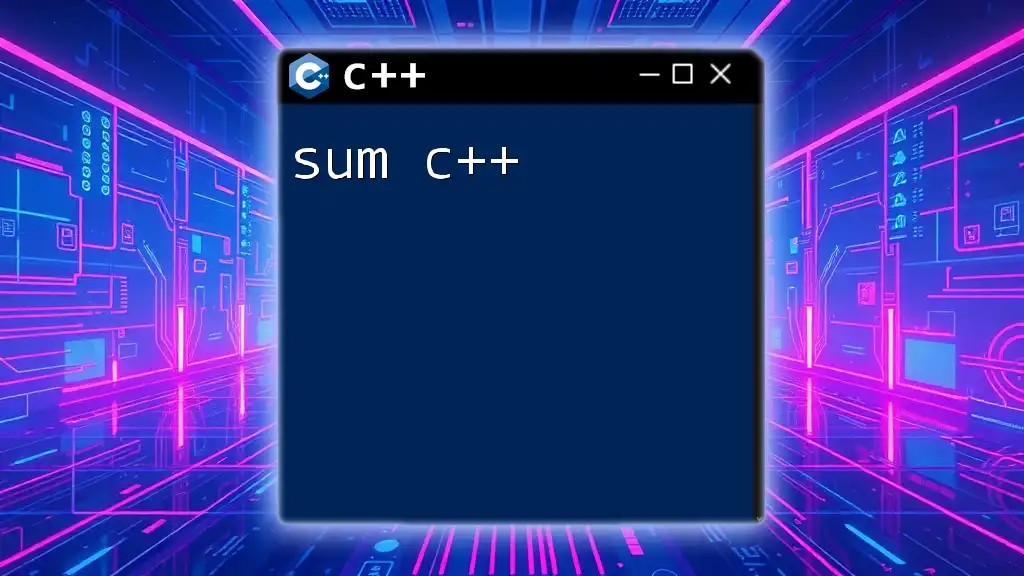
Core Concepts of SFML
Window Management
Creating a Window is often the first step in any SFML application. The `sf::RenderWindow` class makes this task straightforward. Here’s a simple code snippet that demonstrates creating a window:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "SFML Window");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
// Drawing code will go here
window.display();
}
return 0;
}
The above code creates a window of size 800x600 pixels, where you can handle events (like closing the window) and perform rendering tasks.
Drawing in SFML
SFML provides various ways to draw shapes and textures.
Shapes and Textures
To draw shapes, you can use classes like `sf::CircleShape` or `sf::RectangleShape`. For example, creating and drawing a circle is as simple as:
sf::CircleShape circle(50); // Radius of the circle
circle.setFillColor(sf::Color::Green);
window.draw(circle);
Importing and Displaying Textures
To load images and display them, you can use `sf::Texture` and `sf::Sprite`. Below is an example of how to do this:
sf::Texture texture;
if (!texture.loadFromFile("image.png"))
return -1;
sf::Sprite sprite;
sprite.setTexture(texture);
window.draw(sprite);
By applying textures to sprites, you can create visually appealing graphics in your application.
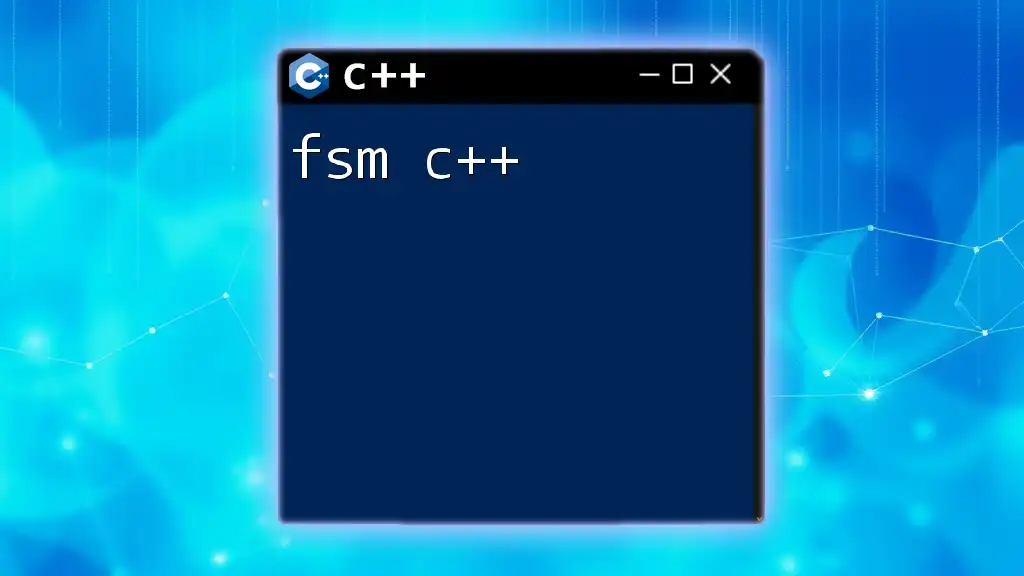
Handling User Input
Keyboard Input
Capturing keyboard input is an essential part of developing interactive applications. SFML provides the `sf::Keyboard` class to check for key presses. Here’s a simple example:
if (sf::Keyboard::isKeyPressed(sf::Keyboard::Escape))
window.close();
This code checks if the Escape key has been pressed, which triggers the closure of the window.
Mouse Input
Handling mouse input is just as critical. The `sf::Mouse` class allows you to detect mouse buttons and positions. Here’s a way to check for mouse clicks:
if (sf::Mouse::isButtonPressed(sf::Mouse::Left)) {
sf::Vector2i position = sf::Mouse::getPosition(window);
// You can now use the position variable
}
This enables you to respond to user actions effectively and create engaging interfaces.
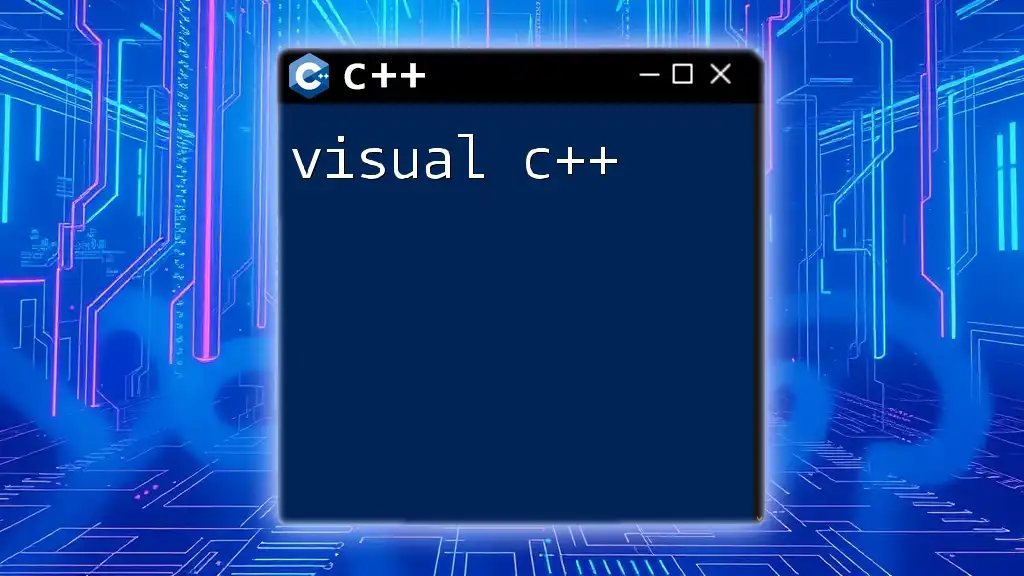
Implementing Animation
Basic Animation Techniques
Animation can enhance user experience significantly. In SFML, the simplest way to implement animation is through frame-based animation. You can update object positions within your game loop. Here’s an illustrative example:
float x = 0;
while (window.isOpen()) {
// Update logic to animate object
x += 0.1; // Move to the right
// Update position of your shape/sprite here.
}
By incrementally updating the position, you can create smooth animations.
Frame Rate and Timing
Managing frame rates is crucial for consistent animations. You can achieve this using `sf::Clock` to track elapsed time:
sf::Clock clock;
float deltaTime = clock.restart().asSeconds();
The `deltaTime` variable can be used to ensure consistent movement speeds across different hardware.
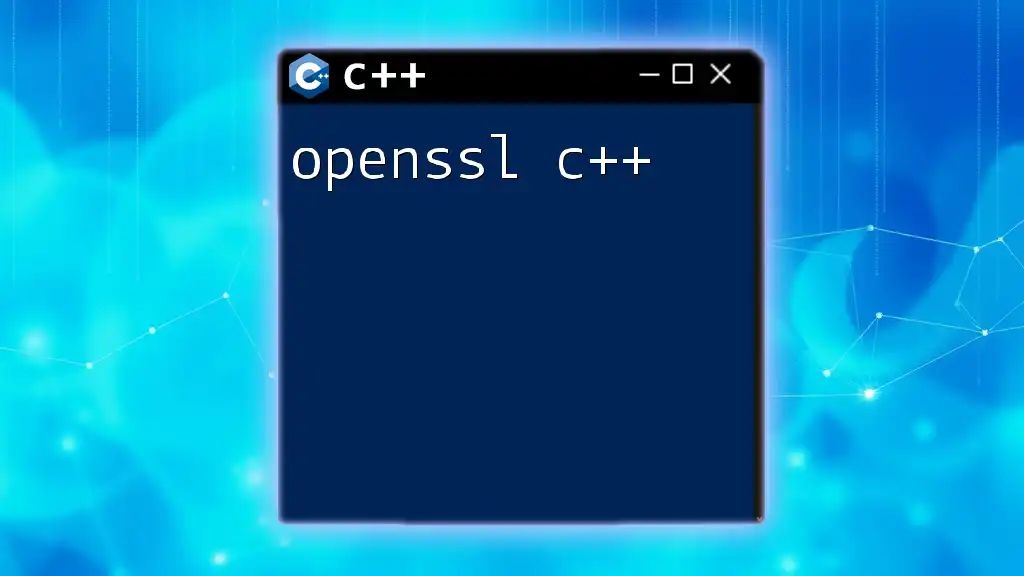
Working with Audio
Audio Management in SFML
Sound is critical in multimedia applications. SFML provides `sf::SoundBuffer` and `sf::Sound` classes to handle audio. Here’s how you can play a sound file:
sf::SoundBuffer buffer;
buffer.loadFromFile("sound.wav");
sf::Sound sound;
sound.setBuffer(buffer);
sound.play();
Implementing audio will significantly elevate your application’s immersion.
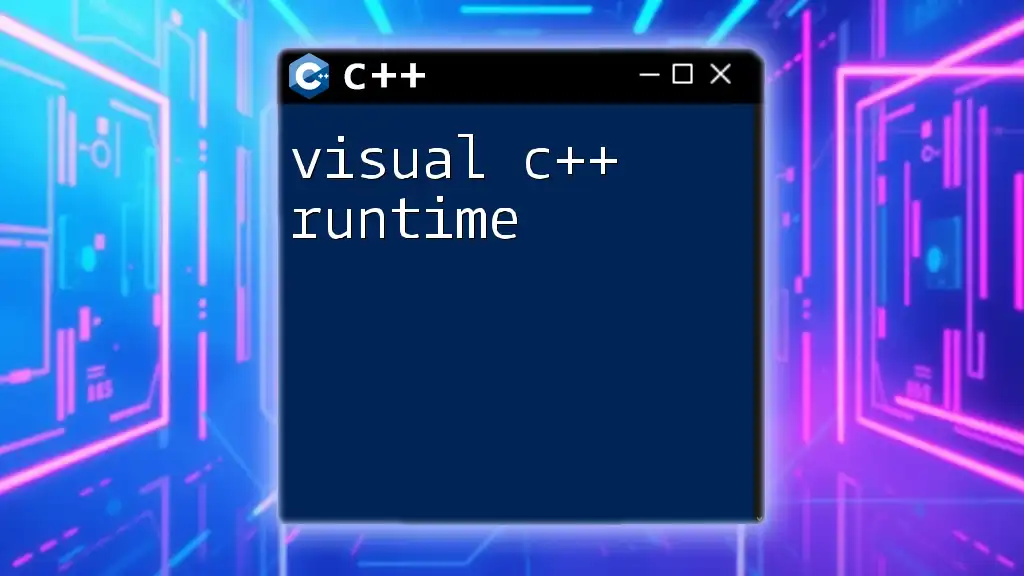
Building a Simple Game Example
Game Structure Overview
Developing games in SFML generally relies on a simple game loop structure that handles initialization, event processing, updating logic, and rendering.
Code Walkthrough
Consider a straightforward example of a player moving a shape around the screen. Here’s how you can implement it:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "Simple Game");
sf::CircleShape player(25); // Player shape
player.setFillColor(sf::Color::Red);
player.setPosition(400, 300); // Start position
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
if (sf::Keyboard::isKeyPressed(sf::Keyboard::Left))
player.move(-0.1f, 0);
if (sf::Keyboard::isKeyPressed(sf::Keyboard::Right))
player.move(0.1f, 0);
if (sf::Keyboard::isKeyPressed(sf::Keyboard::Up))
player.move(0, -0.1f);
if (sf::Keyboard::isKeyPressed(sf::Keyboard::Down))
player.move(0, 0.1f);
window.clear();
window.draw(player);
window.display();
}
return 0;
}
This sample code allows the player to move a red circle around the screen using the arrow keys, demonstrating basic interaction and movement.
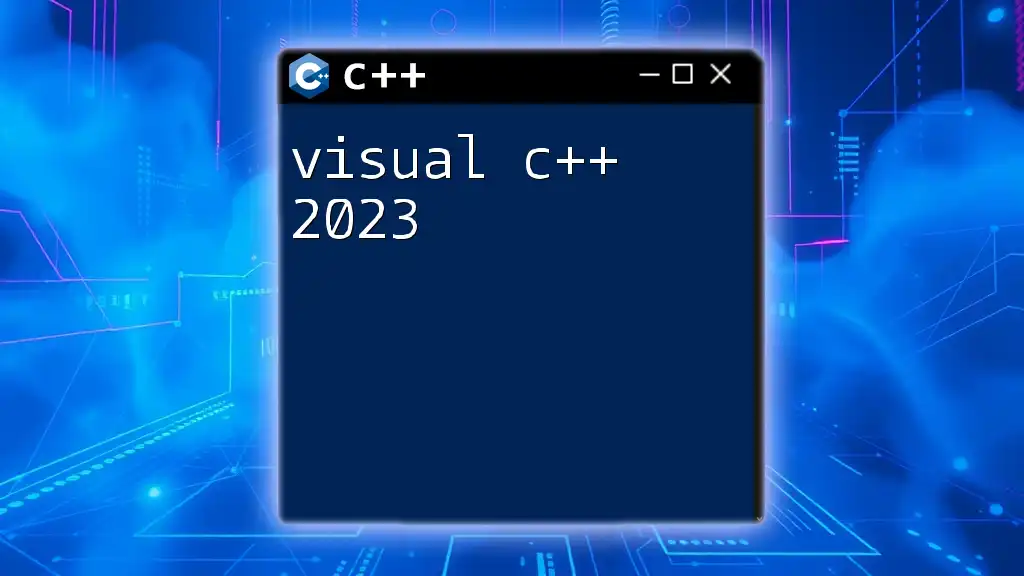
Troubleshooting Common Issues
Common Errors and Solutions
As you work with SFML, you may encounter various issues, particularly linkage errors or missing libraries. Ensure that your IDE project settings are configured properly to link against the appropriate SFML libraries.
Best Practices
Maintaining clean and organized code is vital. Structure your directories for assets, separate your code into modules, and ensure you manage your resources efficiently to avoid memory leaks.
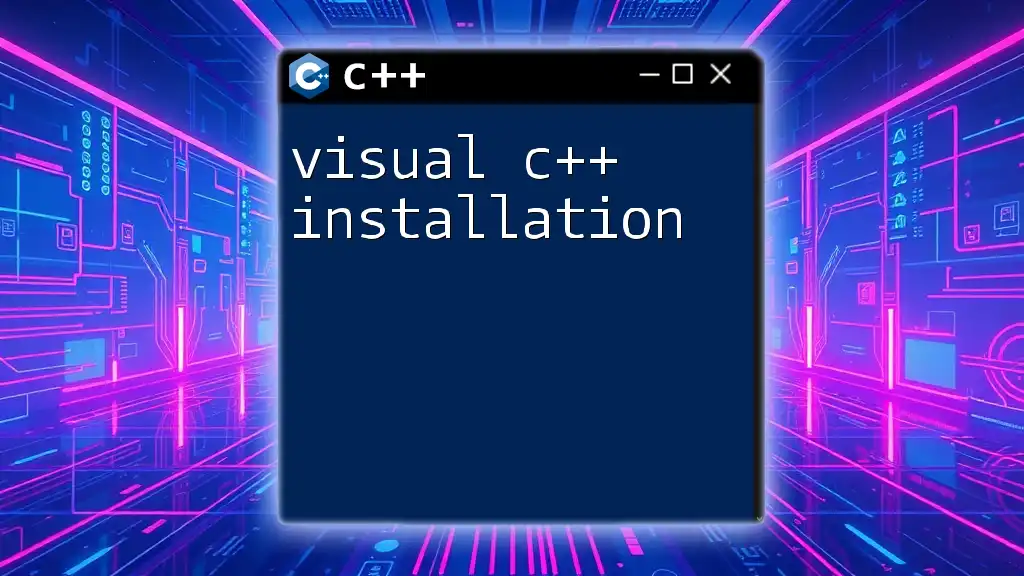
Conclusion
In summary, SFML offers a robust framework for developing multimedia applications with C++. Its user-friendly API, coupled with rich functionalities, makes it an ideal choice for both beginners and experienced developers alike. Using SFML, you can create a wide range of applications—from simple games to complex multimedia platforms. As you gain comfort with the basics, you can dive deeper into more advanced features, such as networking or custom shaders, to enhance your projects even further. Happy coding!