Visual C++ 6.0 is an integrated development environment (IDE) from Microsoft that allows developers to create Windows applications using the C++ programming language.
Here's a simple example of a "Hello, World!" program in Visual C++ 6.0:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Installation and Setup
System Requirements
Before diving into Visual C++ 6.0, it’s crucial to ensure that your system meets the necessary requirements to run this software efficiently. The minimum system requirements typically include:
- Processor: Pentium or compatible processor
- RAM: 16 MB (32 MB recommended)
- Hard Disk: 150 MB of free disk space
- Operating System: Windows 95/98/NT/2000
For optimal performance, it is recommended to have:
- Processor: Pentium II or higher
- RAM: 64 MB or more
- Hard Disk: More than 300 MB of free space
Downloading Visual C++ 6.0
You can obtain Visual C++ 6.0 legally through various online platforms that may still offer old software versions, such as eBay or trusted software archives. Always ensure that you are downloading from a reputable source to avoid malware or security issues.
Installation Process
To install Visual C++ 6.0, follow these steps:
- Locate the setup file you downloaded.
- Run the setup executable and follow the installation prompts.
- Select the installation type: You may opt for typical installation or custom installation, depending on your needs.
- Choose the installation directory where the software will be installed. The default location is usually fine.
- Complete the installation process and restart your computer if prompted.
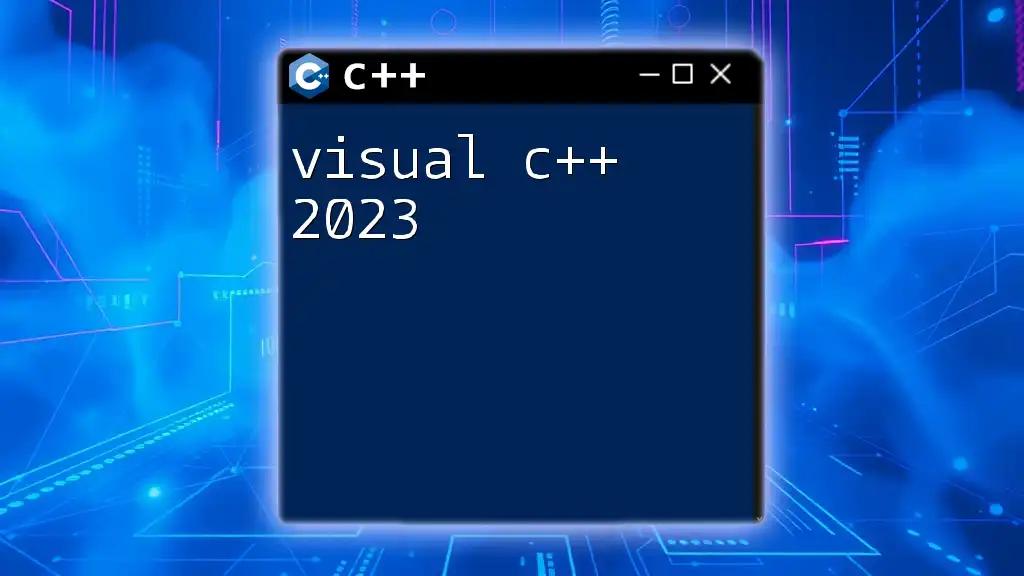
Understanding the IDE (Integrated Development Environment)
Overview of the Visual C++ IDE
The Visual C++ IDE is a powerful interface designed to facilitate C++ programming. It includes several key components that aid in development:
- Menu Bar: Access various commands and tools.
- Toolbars: Quick access buttons for common tasks (e.g., compile, run).
- Workspace: Organize projects and files for easy navigation.
Project Creation and Management
Creating a new project in Visual C++ 6.0 is simple. Start the IDE, then navigate to `File > New > Project`. You will be presented with options for different project types, including Win32 Console Applications and MFC Applications. Select your desired project type and follow the prompts to name your project and define its directory.
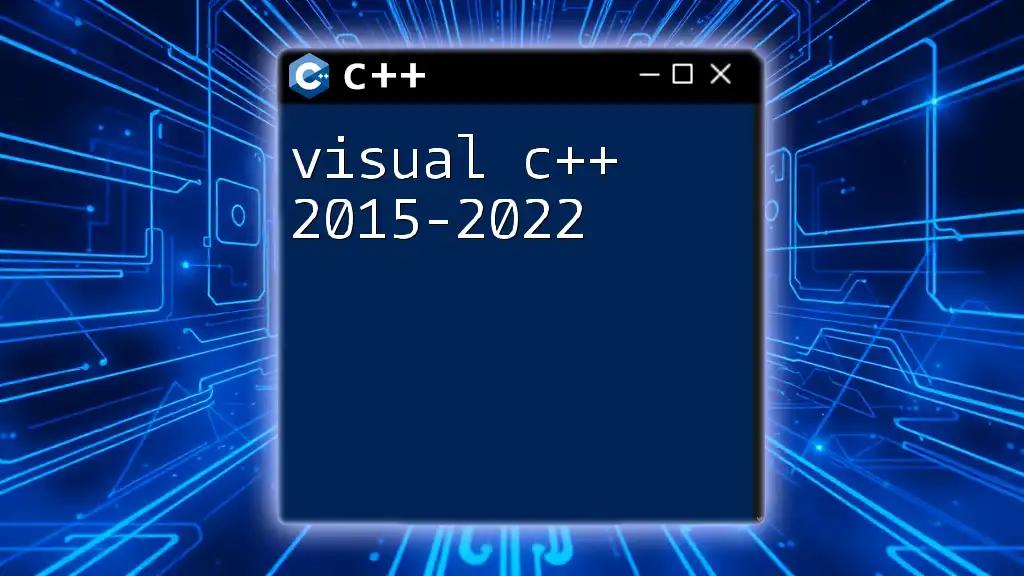
Basic Features of Visual C++ 6.0
Code Editor Functionality
The code editor in Visual C++ 6.0 comes equipped with several features that streamline the development process. These include:
- Syntax Highlighting: Different colors for keywords, comments, and variables enhance readability.
- Auto-Completion: The IDE can suggest completions for variable names and functions, significantly speeding up the coding process.
Debugging Tools
Debugging is crucial for identifying and resolving issues within your code. Visual C++ provides an integrated debugging environment that allows you to:
- Set Breakpoints: Click in the margin next to a line of code to pause execution.
- Inspect Variables: View the current values of variables and expressions during runtime.
- Step Through Code: Execute your program line by line to observe behavior.
Build Process
To compile and link your programs in Visual C++ 6.0, select `Build > Build Solution`. Understanding the difference between debug and release configurations is essential. In debug mode, additional information is included in the executable to aid debugging, while release mode creates a more optimized version for distribution.
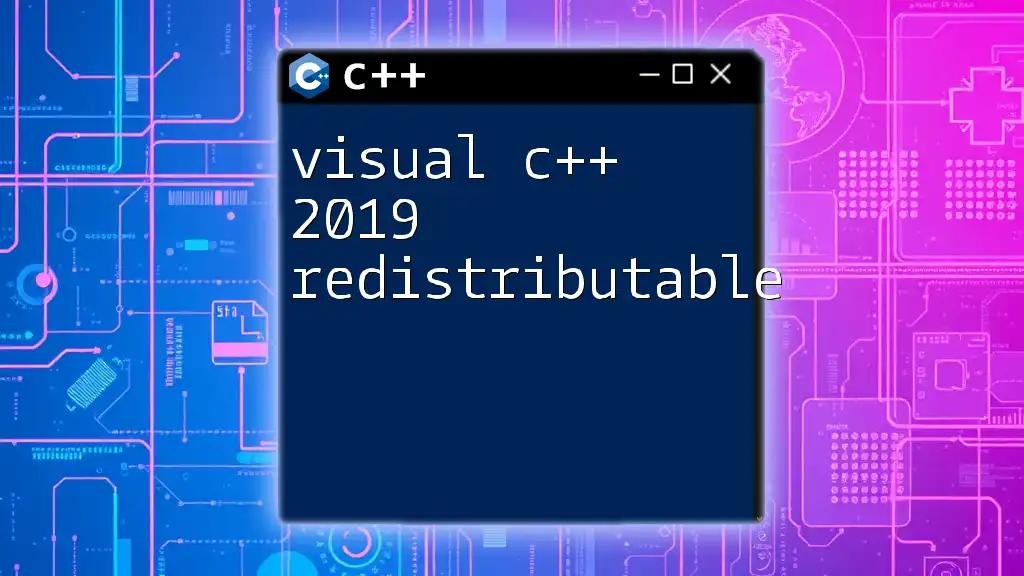
Writing Your First Program in Visual C++ 6.0
Creating a Simple "Hello, World!" Application
Let's create a simple "Hello, World!" application to familiarize yourself with the environment. Follow these steps:
- Start Visual C++ 6.0 and create a new Win32 Console Application project.
- Name your project and choose a directory.
- Replace the default code with the following:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Explanation of the Code
- The `#include <iostream>` directive is necessary for input and output operations.
- `using namespace std;` allows you to use standard library names without the `std::` prefix.
- The `main()` function serves as the entry point for any C++ program.
- `cout` is used to display output to the console.
Compiling and Running the Program
To compile your program, navigate to `Build > Build Solution` or press `Ctrl + F7`. Once compilation is successful, run the program by selecting `Debug > Start Without Debugging` (or press `Ctrl + F5`). The console will display "Hello, World!"
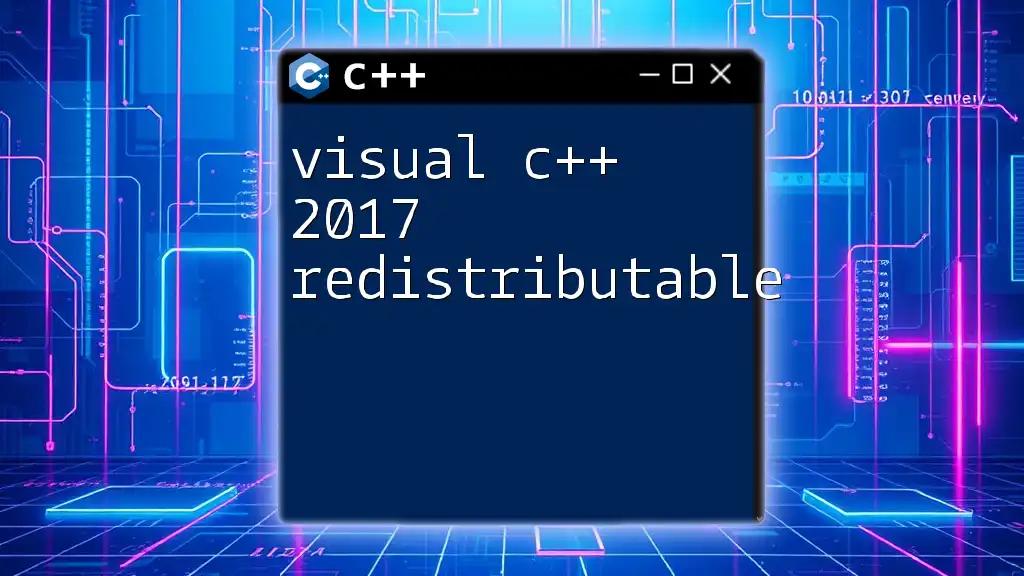
Advanced Features of Visual C++ 6.0
Object-Oriented Programming in Visual C++
Visual C++ 6.0 supports Object-Oriented Programming (OOP), which is fundamental for efficient software development. Key OOP concepts include:
- Classes: The blueprint for creating objects.
- Objects: Instances of classes that hold state and behavior.
- Inheritance: Allows creating new classes based on existing ones.
- Polymorphism: Enables one interface to be used for different underlying forms (data types).
Code Example
Consider the following code that demonstrates OOP concepts:
class Animal {
public:
virtual void makeSound() {
cout << "Animal sound" << endl;
}
};
class Dog : public Animal {
public:
void makeSound() override {
cout << "Woof!" << endl;
}
};
In this example, `Animal` is a base class with a virtual method `makeSound()`. The `Dog` class, which inherits from `Animal`, overrides this method to provide specific functionality.
MFC (Microsoft Foundation Classes)
MFC is a set of C++ class libraries that simplify Windows application programming. It provides tools for developing graphical user interfaces with ease. To create your first MFC application:
- Create a new project and choose "MFC Application."
- Follow the prompts to set up your project.
- The MFC Wizard will generate a basic application structure that you can modify.
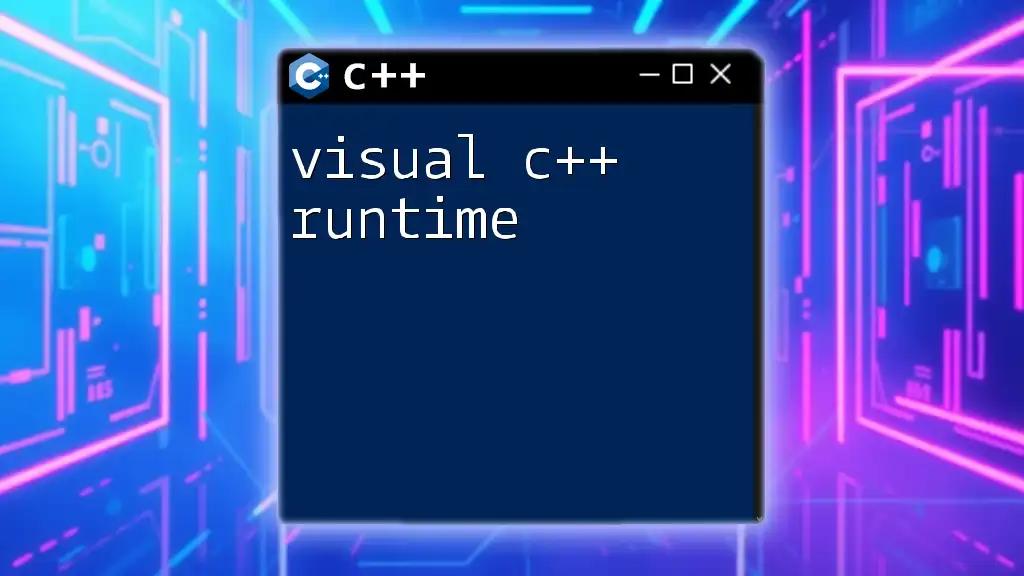
Best Practices for Developing in Visual C++ 6.0
Code Organization
Code organization is crucial for maintainability. Always aim for a modular design, separating your code into logical units/classes. This approach makes your code easier to read, test, and debug.
Commenting and Documentation
Effective commenting improves code understandability. Use comments to explain complex logic or to mark important sections of code. Additionally, maintain documentation that outlines your project's structure and functionality.
Version Control
Utilizing version control systems like Git is essential, especially for larger projects or collaborative efforts. Version control allows tracking changes, reverting to previous versions, and collaborative contributions without conflicts.
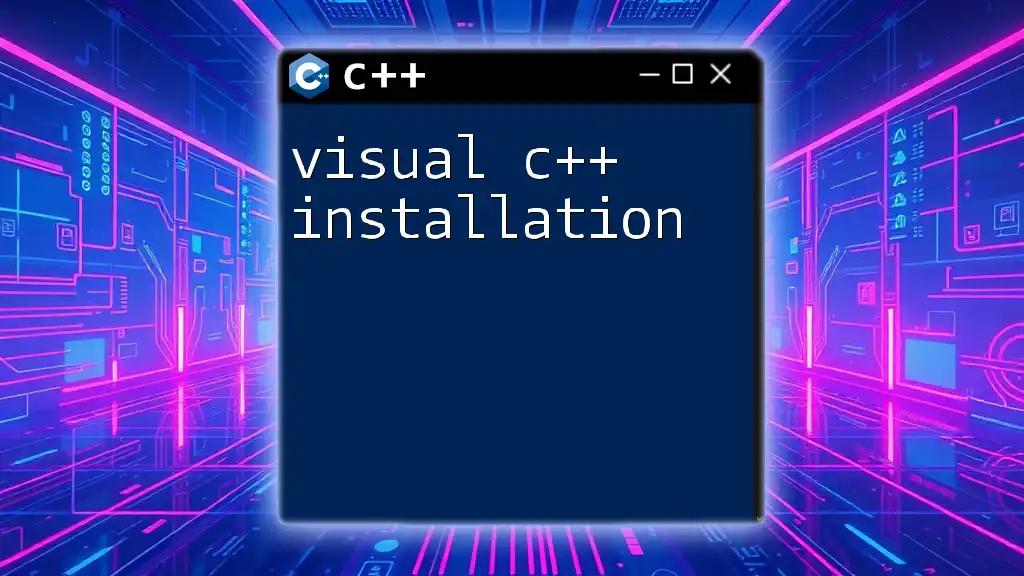
Troubleshooting Common Issues
Installation Problems
Installation issues are sometimes encountered. Common solutions include:
- Checking system requirements to ensure compatibility.
- Running the installer as an administrator to avoid permission issues.
- Ensuring that no conflicting software is running during installation.
Debugging Common Programming Errors
As you write code, encountering errors is common, especially syntax and logical errors. Use the debugging tools in Visual C++ to help identify issues, such as:
- Compiler error messages that describe the problem.
- Examining variable states during debugging to understand unexpected behavior.
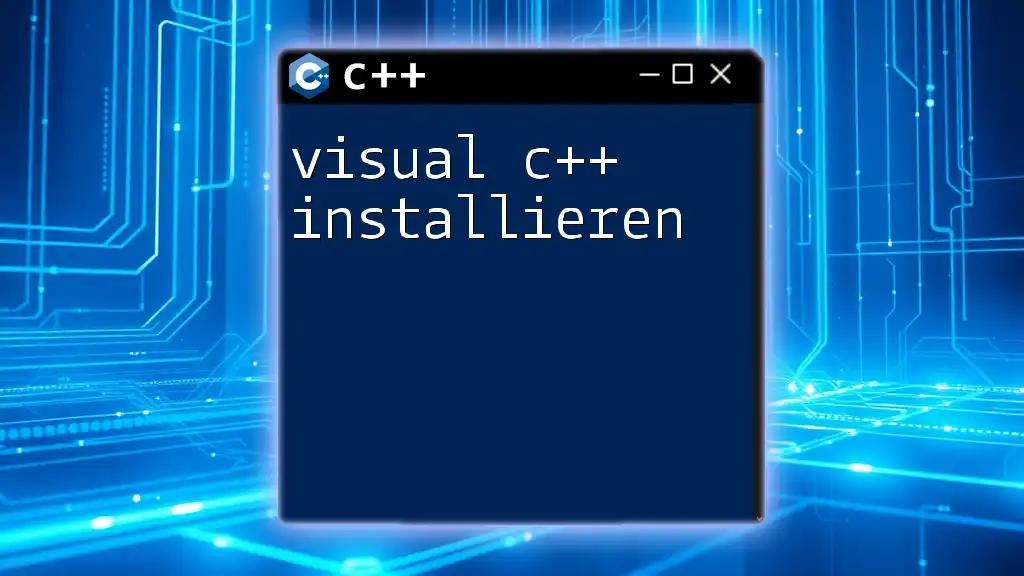
Conclusion
Visual C++ 6.0 remains a valuable asset for developers interested in mastering C++. Its rich feature set and integration with Windows applications provide a solid foundation for software development. Engaging with this IDE will empower you to build robust applications while exploring advanced programming paradigms.
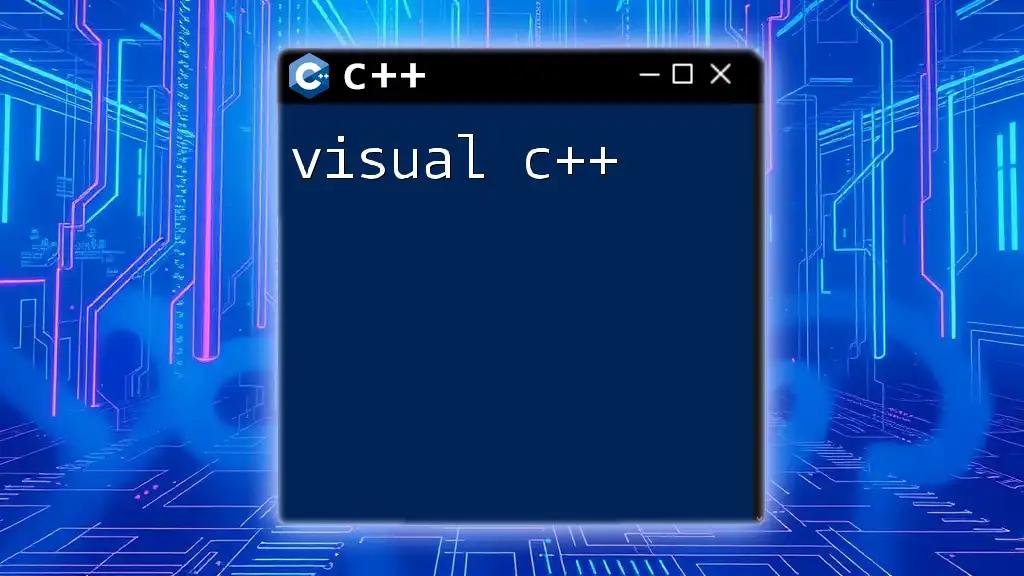
Additional Resources
Forums and Community Support
Consider joining online programming communities, such as Stack Overflow or GitHub, where you can seek guidance from experienced developers as you navigate your learning journey.
Further Reading and Tutorials
To advance your knowledge, explore books and online courses focused on Visual C++ 6.0. These resources offer insights into advanced features and techniques, ensuring you develop a comprehensive understanding of the language.
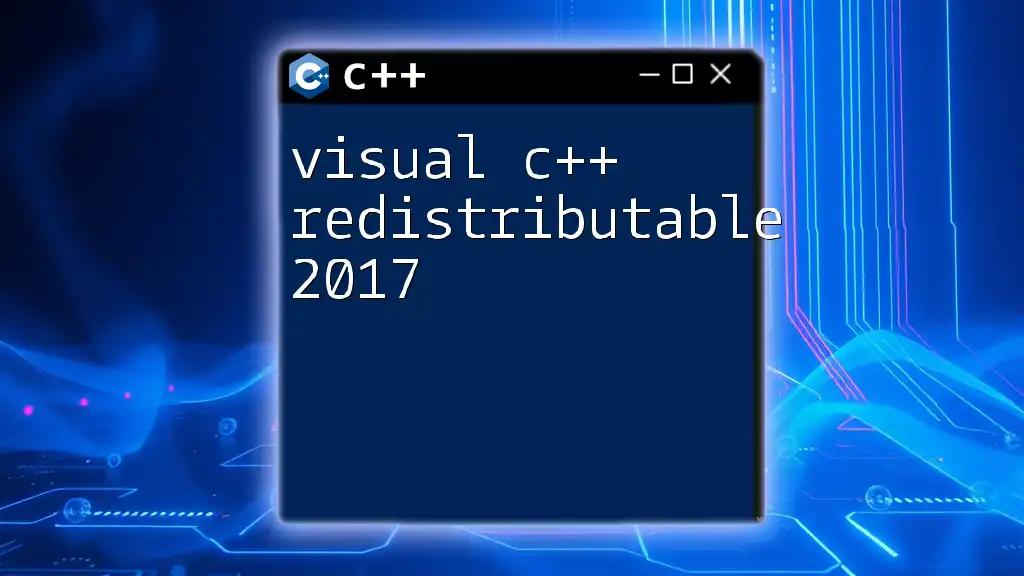
Call to Action
Embark on your journey with Visual C++ 6.0 today! Explore the wealth of resources available and practice building your own applications to deepen your understanding. Join our courses or access our materials to accelerate your learning experience!