The Visual C++ 2017 Redistributable is a package that installs the runtime components required to run applications developed with Visual C++ on a user's machine, ensuring that all necessary libraries are available.
Here's a simple code snippet to demonstrate how to check for the Visual C++ Redistributable installation using a C++ command:
#include <iostream>
#include <windows.h>
int main() {
HKEY hKey;
if (RegOpenKeyEx(HKEY_LOCAL_MACHINE,
TEXT("SOFTWARE\\Microsoft\\VisualStudio\\14.0\\VC\\Runtimes\\x86"),
0, KEY_READ, &hKey) == ERROR_SUCCESS) {
std::cout << "Visual C++ 2017 Redistributable is installed." << std::endl;
RegCloseKey(hKey);
} else {
std::cout << "Visual C++ 2017 Redistributable is not installed." << std::endl;
}
return 0;
}
Understanding Visual C++ Redistributables
What is a Redistributable?
A redistributable is a package containing a collection of runtime components that are necessary for running applications developed using specific development environments, such as Visual C++. These packages allow developers to deploy their applications without having to include the hundreds of files that might be required. Instead, the redistributables ensure that necessary libraries are available on the target machine.
The Role of Visual C++ in Modern Software Development
Visual C++ serves as a powerful IDE (Integrated Development Environment) for building applications on Microsoft platforms. Due to its ability to interface with low-level APIs and its compatibility with multiple programming languages, it is invaluable for developing high-performance software. Applications ranging from multimedia to gaming engines often rely on Visual C++. Many popular applications, such as Adobe products and various game engines, leverage the capabilities of Visual C++ to deliver seamless user experiences.
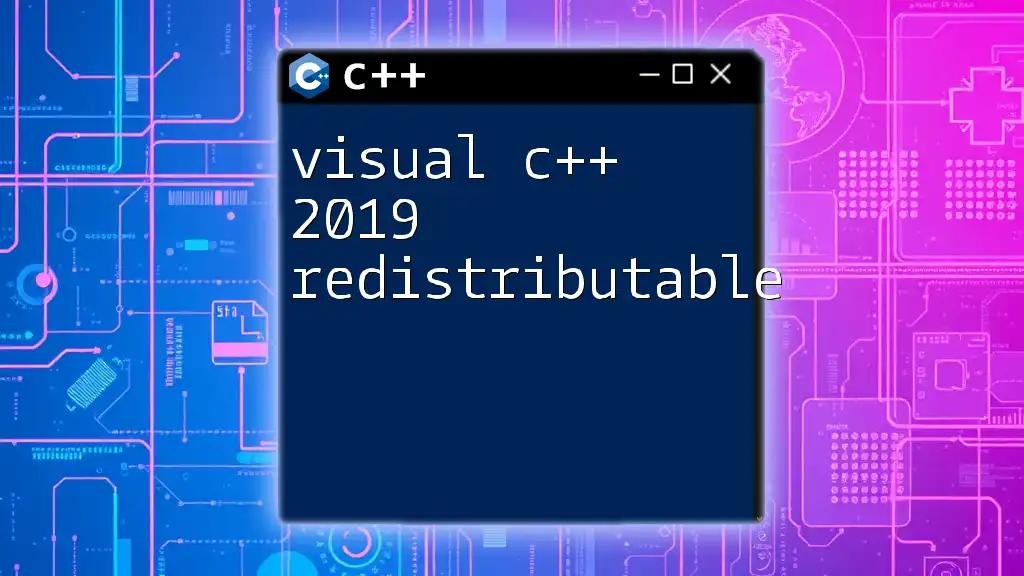
Visual C++ 2017 Redistributable: Key Features
Compatibility
The Visual C++ 2017 Redistributable is designed to work across various operating systems, specifically Windows 7 and later versions, and supports both x86 (32-bit) and x64 (64-bit) architectures. This ensures that applications built with Visual C++ can run on most user machines, regardless of their setup.
Runtime Components Included
The Visual C++ 2017 Redistributable includes essential components such as the MSVCR (Microsoft Visual C++ Runtime) and MSVCP (Microsoft Visual C++ Standard Library) libraries. Each of these components plays a critical role:
- MSVCR (Microsoft Visual C++ Runtime): Provides functions for memory management, file handling, and other core functionalities.
- MSVCP (Microsoft Visual C++ Standard Library): Supplies standard templates and utilities, essential for developing C++ applications.
For example, consider a simple C++ application requiring these components to function:
#include <iostream>
int main() {
std::cout << "Hello, Visual C++ 2017!" << std::endl;
return 0;
}
In this situation, the runtime libraries provided by the Visual C++ 2017 Redistributable ensure that standard input and output functions work correctly on the end-user's system.
Versioning
When working with redistributables, understanding versioning is crucial. The Visual C++ 2017 Redistributable is part of a larger family of redistributables, each built for specific versions of Visual Studio. Developers need to ensure that they are using the correct version corresponding to the libraries their application was built with.
To check which versions are installed on Windows, use the following command in the Command Prompt:
dir /b "%windir%\System32\vcruntime*.dll"
This will display a list of the installed Visual C++ runtime files, making it easier for developers to verify compatibility.
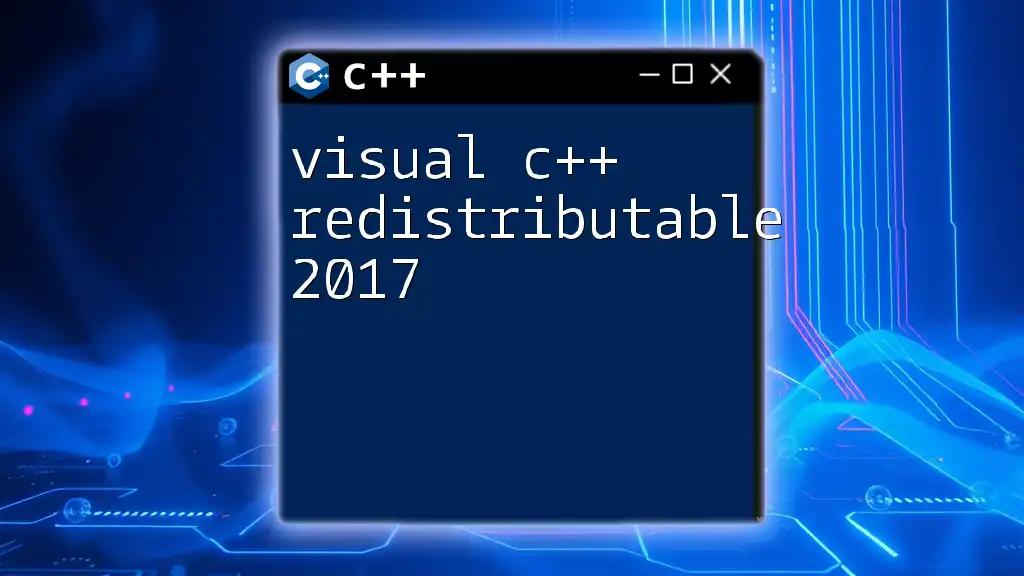
Installation of Visual C++ 2017 Redistributable
Downloading the Redistributable
You can find the Visual C++ 2017 Redistributable on Microsoft’s official website. It's crucial to download the correct version that aligns with your application’s architecture—either x86 or x64. Selecting the wrong version can lead to compatibility issues.
Installation Process
The installation process is straightforward. Here's a step-by-step guide:
- Once you've downloaded the installer, double-click on it to initiate the setup process.
- Follow the on-screen instructions, accepting the license agreement.
- Choose the installation location if prompted (typically, the default location is recommended).
- Wait for the installation to complete; it generally takes just a few seconds.
If you encounter any errors during installation, it may be due to missing Windows updates or insufficient permissions. Ensure your system is up-to-date and that you have administrative rights to install software.
Verifying Installation
After installation, it is important to verify that the redistributable has been installed correctly. Use the same Command Prompt technique mentioned earlier to check if the necessary files are in place. The presence of `vcruntime*.dll` files in your `System32` directory indicates a successful installation.
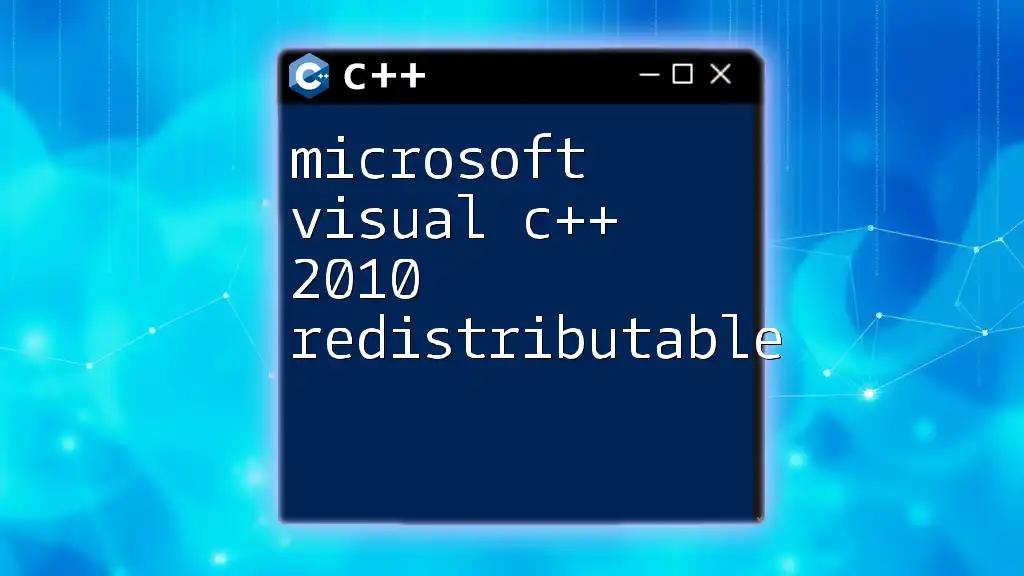
Common Issues and Troubleshooting
Missing MSVCR*.DLL Errors
One common issue that users encounter is the missing MSVCR.DLL error*. This usually occurs when the application requires a specific version of the MSVCR DLL that is not present on the user's system.
To resolve this issue, you can:
- Ensure that the correct version of the Visual C++ 2017 Redistributable is installed.
- Reinstall the redistributable if necessary.
- Check for any application-specific dependencies that may require additional runtime libraries.
Conflicts with Different Redistributable Versions
Working with multiple redistributable versions can lead to conflicts. It's not uncommon for applications to require specific versions of the Visual C++ Redistributable. In such cases, ensure that:
- You install all necessary versions (both x86 and x64 if your application supports them).
- You manage the installation of multiple versions to prevent conflicts.
If a conflict arises, it can often be resolved by modifying or reinstalling the conflicting version. You can remove a conflicting redistributable using the Control Panel's uninstall feature.
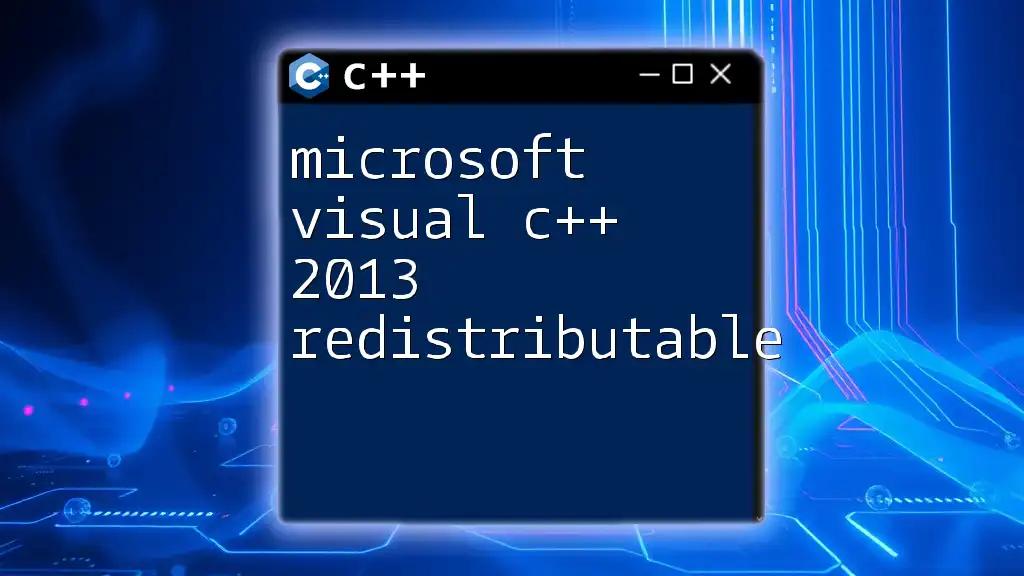
Best Practices for Developers
Packaging Applications with Visual C++ Redistributable
When packaging applications for distribution, it’s essential to include the Visual C++ 2017 Redistributable as part of the installation process. Many installers, like NSIS or WiX, allow you to bundle redistributables smoothly, ensuring that end-users always have the necessary libraries to run your application.
Future-Proofing Your Applications
To future-proof your applications, keep track of updates and new versions of the Visual C++ Redistributable. Regular updates to the redistributable can fix bugs and enhance performance, benefiting your application significantly. Keeping your runtime libraries current ensures that your software remains compatible with the latest operating systems and security standards.

Conclusion
In summary, the Visual C++ 2017 Redistributable is a fundamental requirement for running applications built with Visual Studio 2017. Understanding its role, features, installation process, and best practices can significantly ease the development and deployment of C++ applications. By effectively managing redistributable versions and following the outlined strategies, developers can deliver reliable software while minimizing compatibility issues.
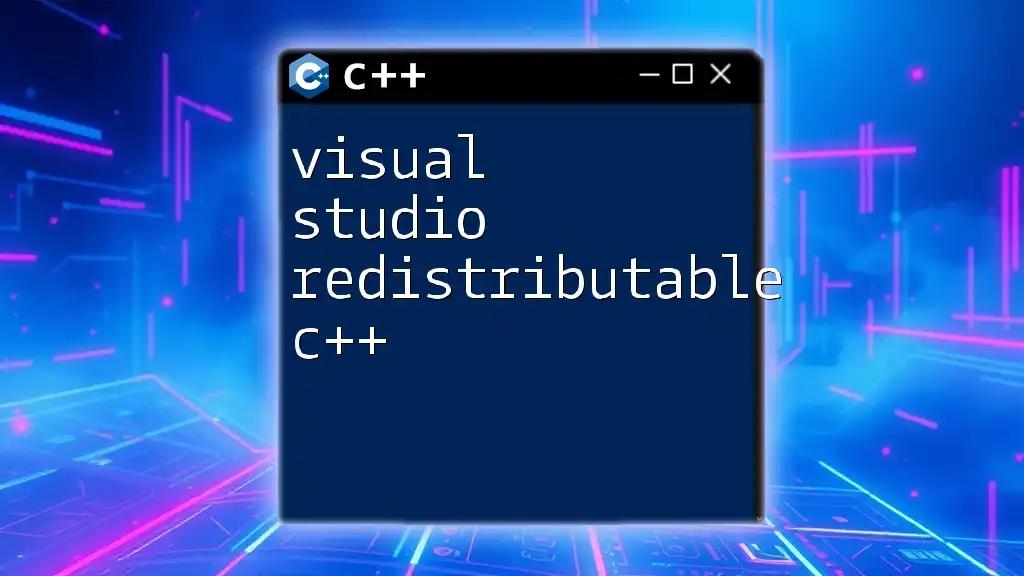
Additional Resources
For further reading and guidance, refer to the official Microsoft documentation on Visual C++ redistributables and explore community forums for additional support and insights.