To utilize C++ applications effectively, downloading the Visual C++ Redistributable is essential, as it ensures that the necessary runtime components are available on your system.
Here’s a basic snippet demonstrating how you might check for the installation in a C++ program:
#include <iostream>
#include <windows.h>
int main() {
if (IsDebuggerPresent()) {
std::cout << "Visual C++ Redistributable is installed." << std::endl;
} else {
std::cout << "Visual C++ Redistributable is NOT installed." << std::endl;
}
return 0;
}
Understanding Visual C++ Redistributables
What are Redistributables?
Redistributables are essential packages that developers create to facilitate the distribution of software applications. They contain components required by programs developed with Visual C++, allowing these applications to run smoothly on end-user systems without bundling the libraries directly within each application. Essentially, redistributables provide the necessary runtime components that allow applications to leverage specific functionalities of the C++ standard libraries.
Components of Visual C++ Redistributable
The Visual C++ Redistributable packages typically include critical runtime libraries, such as the MSVC (Microsoft Visual C++) runtime, that are needed for C++ applications to function. These components handle functionalities like memory management, exception handling, and more tailored functionalities that various applications depend on.
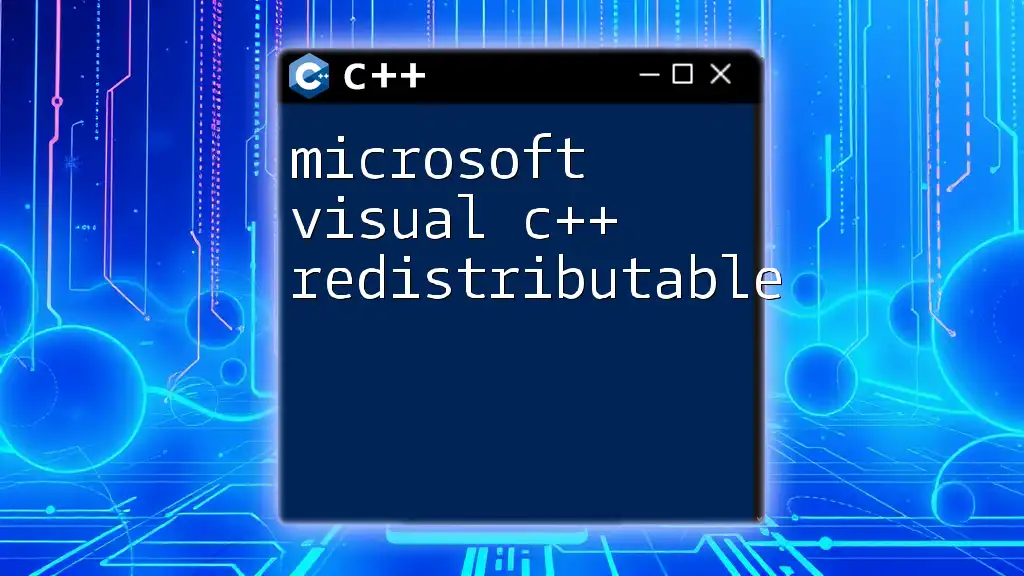
Types of Visual C++ Redistributables
Different Versions of Visual C++ Redistributable
Microsoft has released multiple versions of the Visual C++ Redistributable over the years, correlating with different releases of Visual Studio. Here’s a brief overview of those versions:
- Visual C++ 2005
- Visual C++ 2008
- Visual C++ 2010
- Visual C++ 2012
- Visual C++ 2013
- Visual C++ 2015
- Visual C++ 2017
- Visual C++ 2019
- Visual C++ 2022
Each version of the redistributable is generally backward compatible, meaning applications built to use older versions can typically still run on systems with newer redistributables installed. However, the reverse is not true; applications requiring a specific older version may encounter issues if that version is not present.
Choosing the Right Version for Your Application
Selecting the correct version of the Visual C++ Redistributable is crucial for the application's success. Always check the application's documentation or contact the developer to find out which version is needed. Compatibility between system architecture (x86 vs. x64) and the specific redistributable version required must be considered before making any downloads.
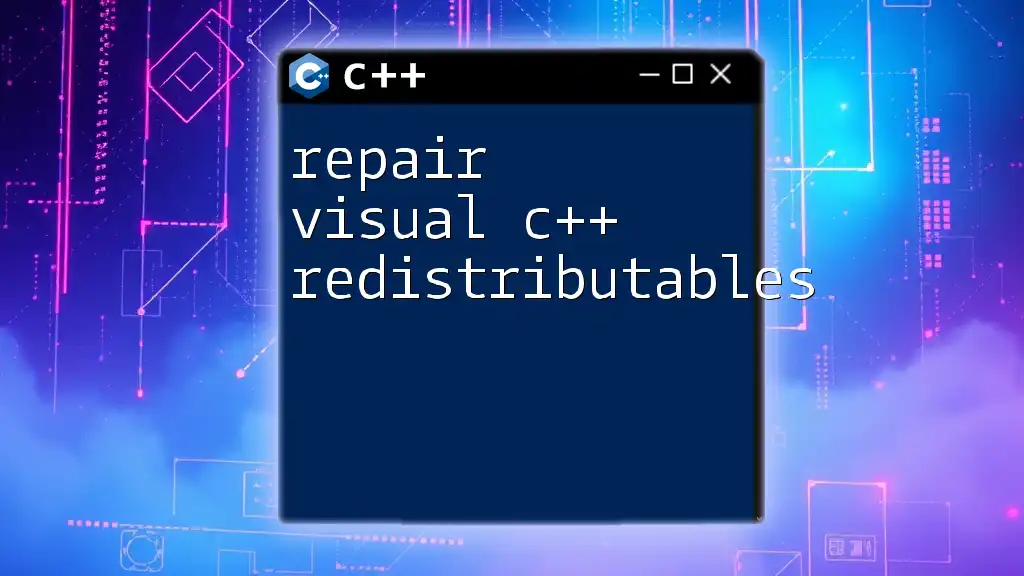
Where to Download Visual C++ Redistributables
Official Microsoft Download Links
To ensure system security and integrity, it is highly recommended to download the Visual C++ Redistributables from official sources. Microsoft provides the redistributables on its official website. Below are some links to popular versions:
- [Visual C++ Redistributable 2015-2022](https://learn.microsoft.com/en-us/cpp/windows/latest/release/visual-cpp-redistributable-installation?view=msvc-160)
- [Visual C++ 2013 Redistributable](https://www.microsoft.com/en-us/download/details.aspx?id=40784)
- [Visual C++ Redistributable 2010](https://www.microsoft.com/en-us/download/details.aspx?id=5555)
Alternatives and Additional Sources
While downloading from official sources is safest, some alternative repositories can provide these libraries. Be cautious here—only use reputable sites to avoid introducing malware or unreliable versions.
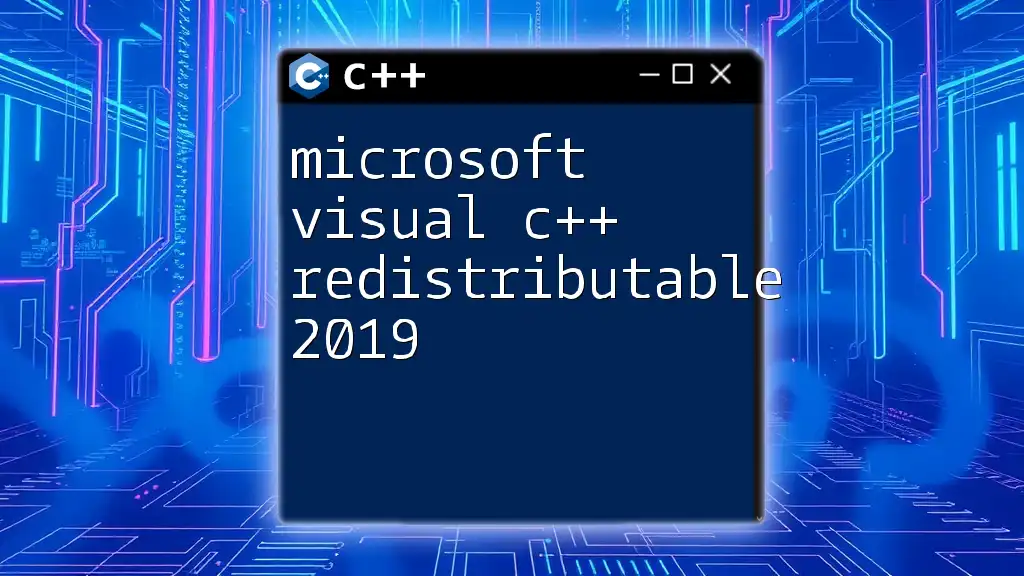
How to Install Visual C++ Redistributable
System Requirements
Before installation, it’s important to check that the target operating system is compatible with the Visual C++ Redistributable you wish to install. Ensure your system meets minimum hardware specifications as well.
Step-by-Step Installation Guide
- Downloading the Installer: Navigate to the link for the appropriate version, and click the download button.
- Running the Installer: After the download completes, locate the installer file and double-click to run it. Follow the on-screen prompts. You may be presented with options like "Install" or "Repair" if an earlier version is already installed.
- Common Installation Options and Settings: Be sure to read each prompt carefully. Some installers offer options for specific components; make sure to include everything necessary for your application.
Verifying Successful Installation
After installation, it's good practice to confirm that the redistributable has been installed correctly. You can check this by:
- Going to “Programs and Features” in the Control Panel, where you should see the Visual C++ Redistributable listed.
- Using a command line verification method:
dotnet --info
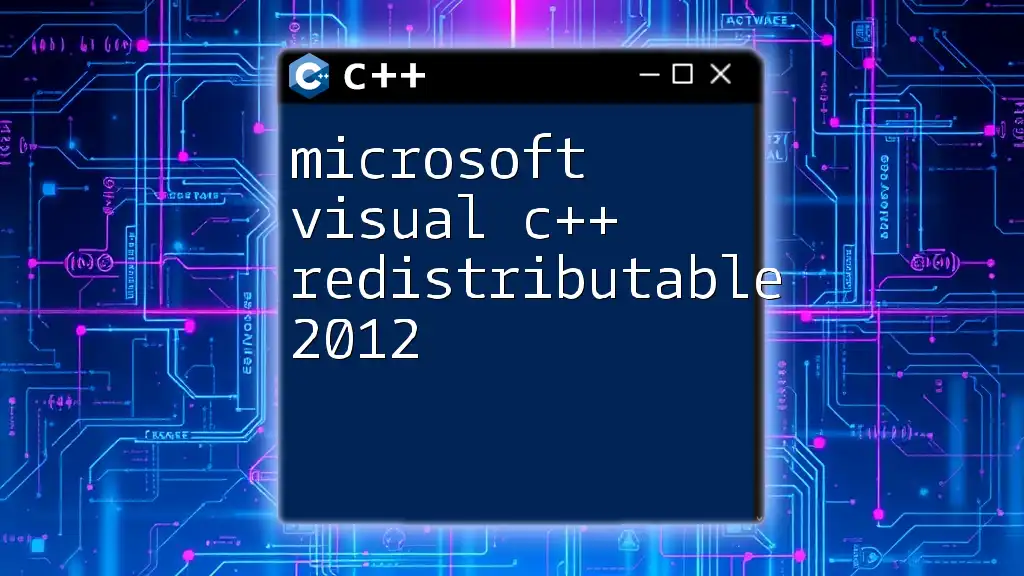
Troubleshooting Installation Issues
Common Installation Problems
During the installation process, you might encounter common issues such as error messages indicating missing prerequisites or compatibility problems. These messages often provide guidance on what is wrong. Checking Microsoft’s documentation or forums may provide solutions for specific errors.
Repairing Existing Installations
If an installation is not functioning as expected, you can often repair it through the Control Panel:
- Navigate to “Programs and Features.”
- Find the version of Visual C++ Redistributable you want to repair.
- Right-click and select “Repair.” The installer will launch and guide you through a repair process.
Reinstall the redistributable as a last resort if issues persist.
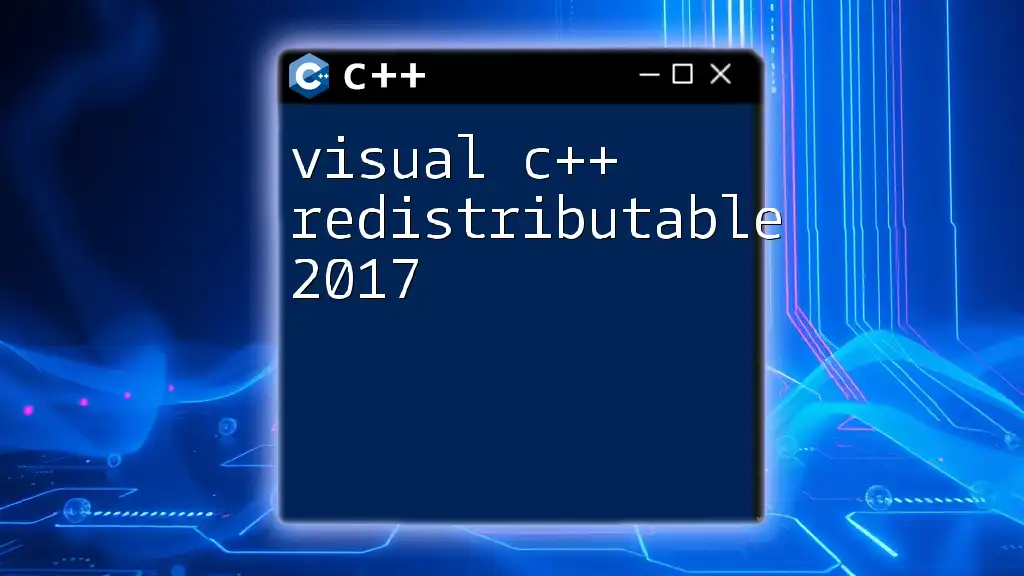
Updating Visual C++ Redistributables
Importance of Keeping Redistributables Updated
Keeping redistributables updated is vital for maintaining software performance and security. Updates can include critical patches that fix vulnerabilities, potentially enhancing overall system stability.
How to Check for Updates
Check for updates through Windows Update, which often includes updates for Visual C++ Redistributables. Manual downloads can be done through the official Microsoft download pages as needed.
Updating Procedures
To keep your Visual C++ Redistributables updated, you can do the following:
- Use Windows Update: Ensure that your system is set to automatically install updates. This includes updates for Microsoft software.
- Manual Download and Install: Periodically check the Microsoft download pages for newer versions and install them as needed.
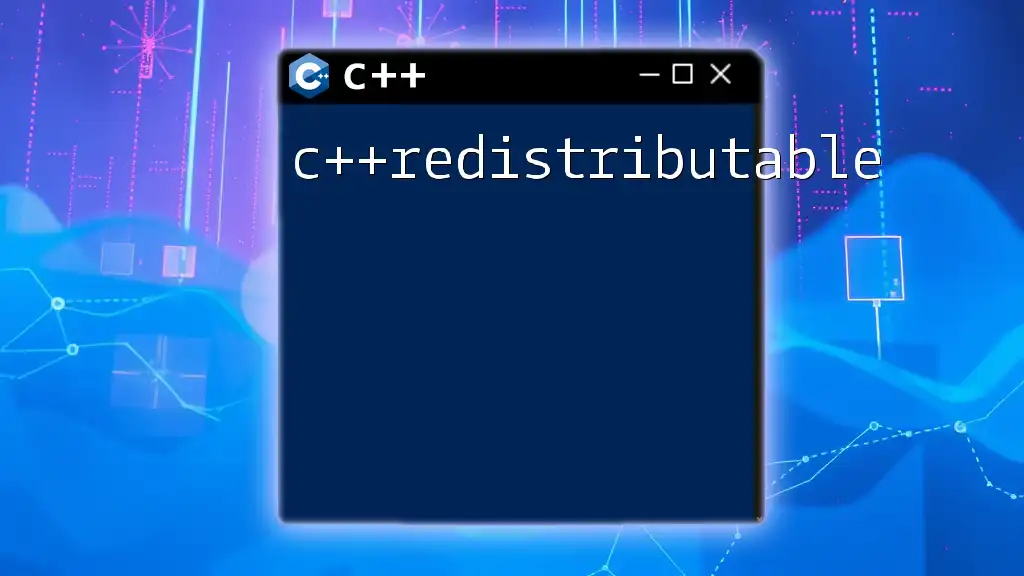
Code Snippets and Practical Usage
Using APIs that Require Visual C++ Redistributables
Many developers rely on APIs that require Visual C++ Redistributables to function correctly. Below is an example of simple C++ code that can only run if the appropriate runtime components are installed.
#include <iostream>
void exampleFunction() {
std::cout << "This is a Visual C++ example." << std::endl;
}
In this example, the program outputs a simple message to the console. If the necessary redistributable is missing, the application may throw runtime errors, making the correct installation paramount.
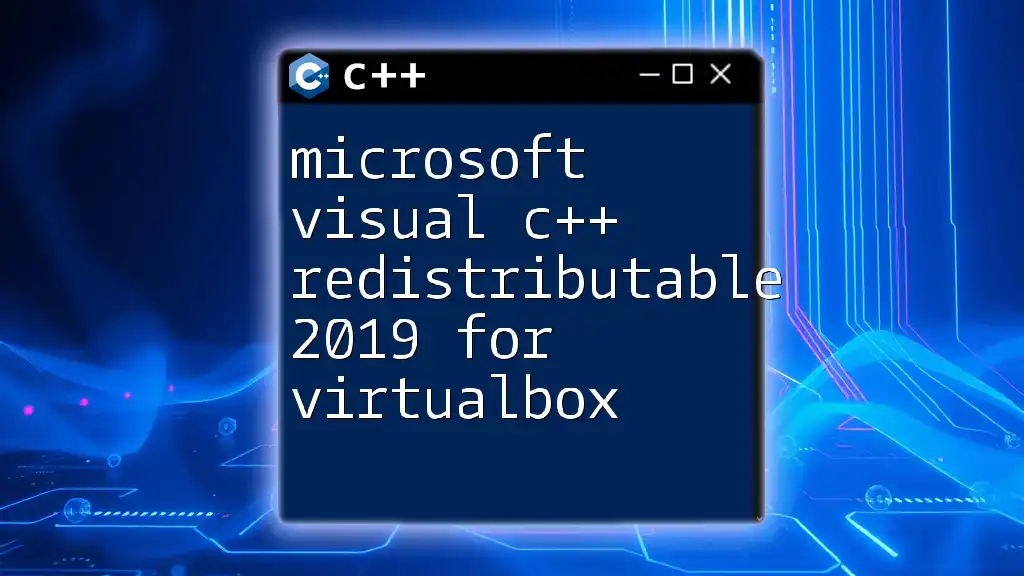
Conclusion
Understanding the importance and utility of Visual C++ Redistributables is vital for both developers and end-users. Knowing how to download and install the correct version can save you from encountering issues that disrupt normal functionality. Continuous learning about updates and troubleshooting techniques will ensure that your applications run smoothly and securely.
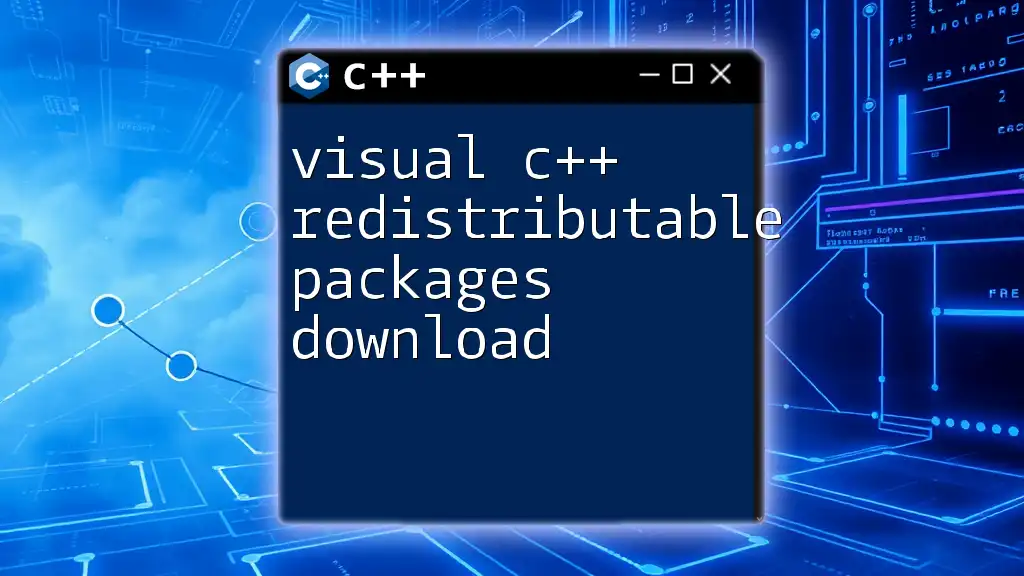
Additional Resources
As you dive deeper into Visual C++ development and redistributables, consider visiting official documentation and joining forums where you can share your experiences and ask questions. Whether you opt for books or online courses, numerous options are available to enhance your C++ knowledge. By understanding these components, you can better equip yourself for a successful programming career.