The Visual C++ Redistributable 2017 is a package of runtime components that are essential for running applications developed with Visual C++ 2017, ensuring that all necessary libraries are available on the system.
Here’s a basic code snippet demonstrating how to check if the Visual C++ Redistributable is installed using a C++ script:
#include <iostream>
#include <Windows.h>
int main() {
// Check if the Visual C++ Redistributable 2017 is installed
HKEY hKey;
if (RegOpenKeyEx(HKEY_LOCAL_MACHINE,
TEXT("SOFTWARE\\Microsoft\\VisualStudio\\15.0\\VC\\Runtimes\\x86"),
0, KEY_READ, &hKey) == ERROR_SUCCESS) {
std::cout << "Visual C++ Redistributable 2017 is installed." << std::endl;
RegCloseKey(hKey);
} else {
std::cout << "Visual C++ Redistributable 2017 is not installed." << std::endl;
}
return 0;
}
Understanding Visual C++ Redistributable
What is Visual C++ Redistributable?
Visual C++ Redistributables are packages that install runtime components necessary for running applications developed with Visual C++. They allow software created with particular versions of Visual C++ to run on machines that do not have Visual C++ installed directly. Unlike static linking where all necessary libraries are bundled within the application, dynamic linking relies on the availability of these redistributable packages, which reduces the application size but increases dependency management complexity.
The importance of redistributable packages in application development cannot be overstated. They ensure that users can run applications seamlessly without having to install a full development environment. This becomes crucial especially when distributing applications to end users who might not be tech-savvy or familiar with development tools.
Key Features of Visual C++ Redistributable 2017
Visual C++ Redistributable 2017 includes several enhancements that cater to modern application needs. Some of the notable features include:
-
Support for New Language Standards: Visual C++ Redistributable 2017 supports advances in C++ standards, including C++11, C++14, and C++17, which introduce numerous language enhancements that improve code efficiency and clarity.
-
Compatibility: This version is designed to work with various Windows operating systems, making it an essential tool for software developers targeting multiple user environments.
-
Performance Improvements: Introducing several optimizations, this redistributable aims to offer better runtime performance, which is critical for resource-intensive applications.

Installing Visual C++ Redistributable 2017
System Requirements
Before installation, make sure your system meets the minimum requirements:
- Operating System: Windows 7, 8, 8.1, or 10 (x86 and x64 compatible).
- Memory: Sufficient free memory based on the application requirements.
You can check system compatibility by navigating to the System section under Control Panel.
Installation Process
To install Visual C++ Redistributable 2017, follow these steps:
-
Download: You can download the redistributable packages from the official Microsoft website. Be sure to select the correct version based on your application architecture: x86 for 32-bit systems or x64 for 64-bit systems.
-
Run the Installer: After downloading, launch the setup file.
-
Complete the Installation: Follow the on-screen instructions. It’s usually just a matter of clicking "Next" and agreeing to the terms. After installation, it’s wise to verify by checking "Programs and Features" in the Control Panel.
Common Installation Issues
At times, users may encounter installation errors. Here’s how to tackle some common problems:
-
Missing Components: If you receive an error about missing components during installation, ensure that all previous versions of Visual C++ Redistributables are either installed or updated.
-
Failed Installations: If the installation fails, try running the installer as an administrator or disable any antivirus software temporarily. Rebooting your system before re-attempting the installation may also help.

Using Visual C++ Redistributable 2017
Configuring Projects in Visual Studio
Once the redistributable is installed, you can start linking with your Visual Studio projects. Here’s a quick code illustration that demonstrates basic project configuration:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, Visual C++ Redistributable 2017!" << endl;
return 0;
}
In Visual Studio, ensure that your project settings include the appropriate libraries. Go to Project Properties > C/C++ > Code Generation, and confirm that the appropriate runtime library is selected (e.g., Multi-threaded DLL).
Deploying Applications with Redistributables
When deploying applications, it’s crucial to include the correct Visual C++ Redistributable version in your application’s setup. Whether you are using InstallShield, WiX, or any other installer framework, make sure that the redistributable is part of the install package to avoid run-time errors on users' machines.

Common Issues Encountered
Runtime Errors
One of the most common issues developers face is runtime errors stemming from the absence of the Visual C++ Redistributable. These errors can mislead users into thinking there’s something wrong with the application itself. Here is an example of handling such scenarios:
#include <iostream>
#include <stdexcept>
using namespace std;
int main() {
try {
throw runtime_error("Visual C++ Redistributable not found!");
} catch (const runtime_error& e) {
cout << e.what() << endl;
}
return 0;
}
This code snippet demonstrates how to effectively catch runtime errors and provide users with meaningful feedback regarding missing dependencies.
Debugging Tips
To debug issues associated with missing or corrupted redistributables, consider using dependency walkers or tools like Process Monitor. These tools can help identify missing components by logging errors associated with your application’s execution.
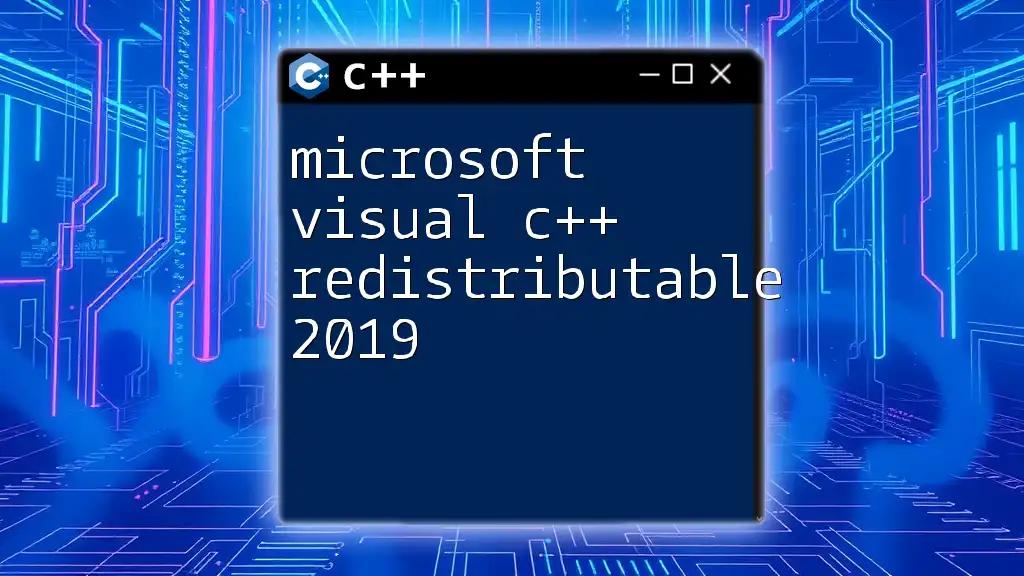
Updating Visual C++ Redistributable 2017
Importance of Keeping Up-to-Date
Regularly updating the Visual C++ Redistributable is essential for maintaining application stability and security. Updates often include critical security patches and bug fixes, which can prevent vulnerabilities in your application.
Steps to Update
To keep your installation current, you can check for updates via the Windows Update feature or manually download the latest version from the Microsoft website. Just follow the same installation steps, and the installer will replace older versions, if necessary.
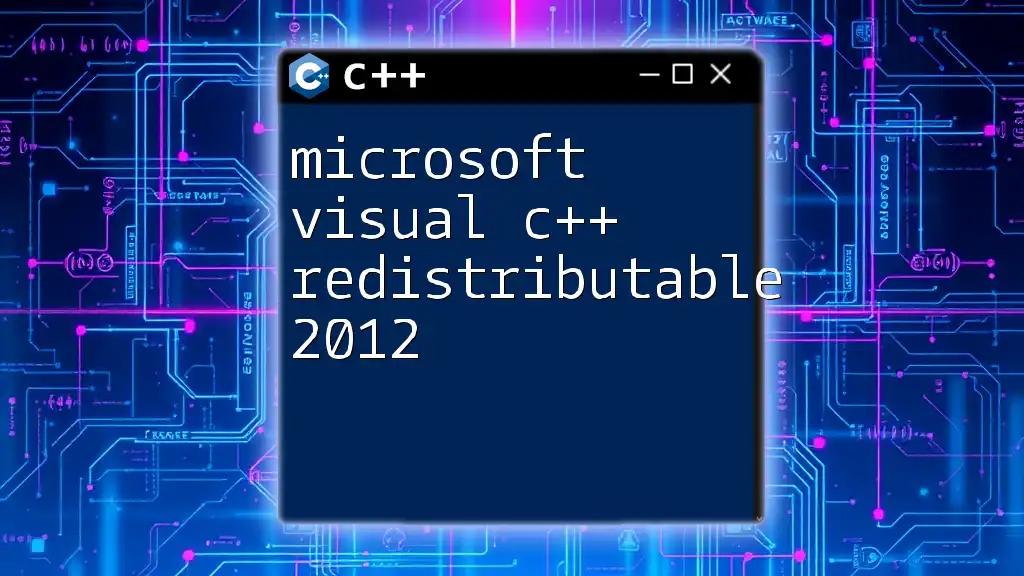
Best Practices
Managing Different Versions of Redistributables
For developers who manage multiple projects, it’s important to keep track of the different versions of Visual C++ Redistributables required. This can help prevent conflicts and ensure that your applications run smoothly on user machines. It’s advisable to document the requirements for each project.
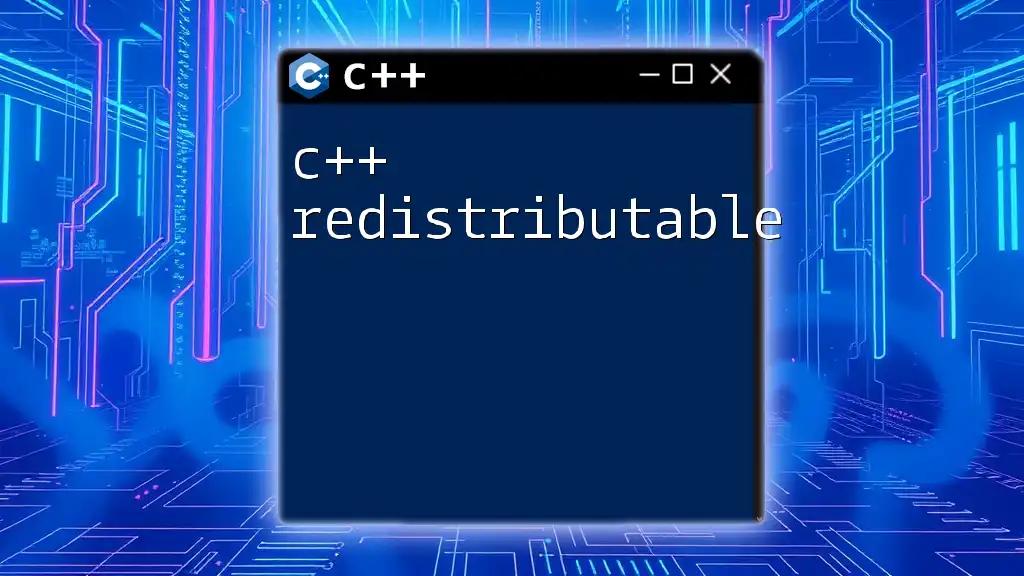
Conclusion
Incorporating Visual C++ Redistributable 2017 into your development workflow is vital for creating reliable applications that run smoothly across various systems. Following the guidelines laid out in this guide can help you avoid common pitfalls and ensure users have a seamless experience with your software.
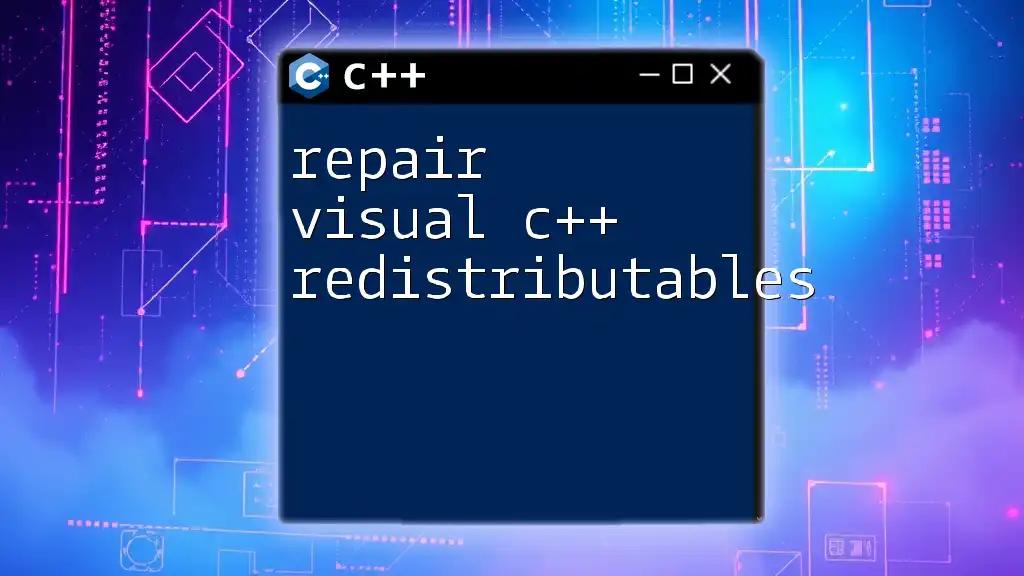
Frequently Asked Questions (FAQs)
What is the difference between VC++ Redistributable 2017 and other versions?
Visual C++ Redistributable 2017 comes with updates and security enhancements specific to the C++ language features introduced in that version. It may not provide backward compatibility to older libraries, hence the differences.
Can I install multiple versions of Visual C++ Redistributable on the same machine?
Yes, multiple versions can coexist on the same machine. It’s common for applications to rely on different versions based on their development environment.
How do I know if my application requires the Visual C++ Redistributable?
If your application was developed with Visual C++, it will typically require the corresponding redistributable package. Always consult the application's documentation or error messages for clues.
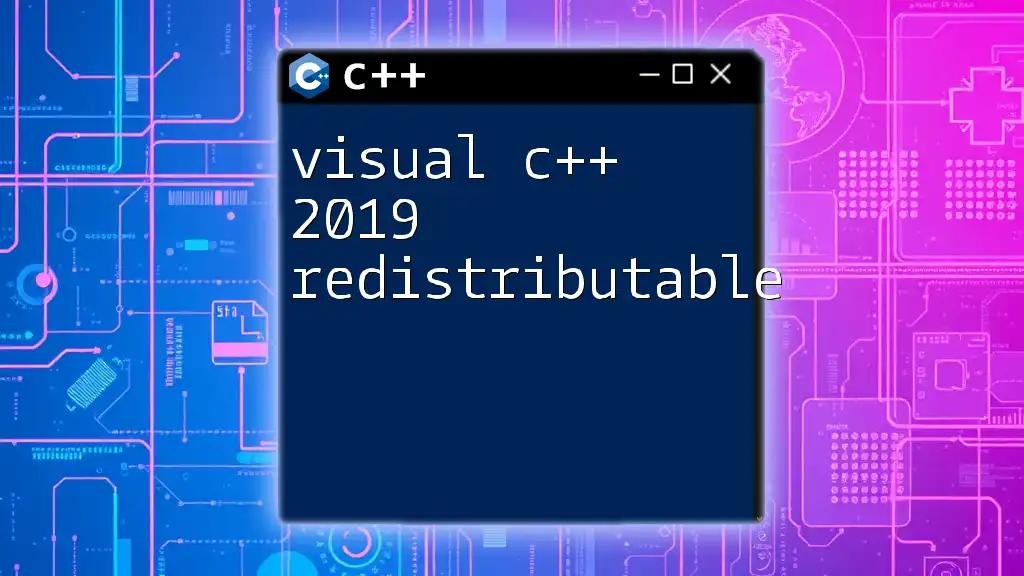
Additional Resources
For further information and assistance, you can consult official Microsoft documentation, video tutorials that visually guide you through the installation process, and various forums where you can engage with a community of developers facing similar challenges.