The Microsoft Visual C++ Redistributable 2012 is a package that installs runtime components required to run applications built with Visual C++ 2012, ensuring that essential C++ libraries are available on the user's system.
Here’s a code snippet demonstrating how to check if the Visual C++ Redistributable 2012 is installed on a Windows machine using C++:
#include <windows.h>
#include <iostream>
bool IsVC2012RedistributableInstalled() {
HKEY hKey;
if (RegOpenKeyEx(HKEY_LOCAL_MACHINE,
TEXT("SOFTWARE\\Microsoft\\VisualStudio\\11.0\\VC\\Runtimes\\x86"),
0, KEY_READ, &hKey) == ERROR_SUCCESS) {
RegCloseKey(hKey);
return true; // Installed
}
return false; // Not installed
}
int main() {
if (IsVC2012RedistributableInstalled()) {
std::cout << "Microsoft Visual C++ Redistributable 2012 is installed." << std::endl;
} else {
std::cout << "Microsoft Visual C++ Redistributable 2012 is not installed." << std::endl;
}
return 0;
}
Understanding the Microsoft Visual C++ Redistributable
What is the Microsoft C++ 2012 Redistributable?
The Microsoft Visual C++ Redistributable 2012 is a set of runtime components that are necessary for running applications developed with Microsoft C++ 2012. It enables developers to use libraries that enhance performance and functionality within their software.
These components are crucial for application consistency across various systems, as they allow applications to use common libraries packaged with the Redistributable, avoiding redundancy and ensuring compatibility. In essence, the redistributable serves as a bridge between your application and the underlying Windows operating system, thus simplifying software installation and deployment.
Why Do You Need Microsoft C++ Redistributable 2012?
When an application is built using Microsoft C++ 2012, it often relies on specific libraries provided by the Microsoft C++ 2012 Redistributable. If the redistributable is missing from a user’s system, errors will occur when attempting to run these applications. Common issues may include:
- Application crashes upon startup.
- Error messages indicating that specific DLL files (like `msvcr110.dll`) are missing.
By ensuring that the Visual C++ Redistributable 2012 is installed, you eliminate these common problems, allowing your software to run smoothly on any compatible machine.
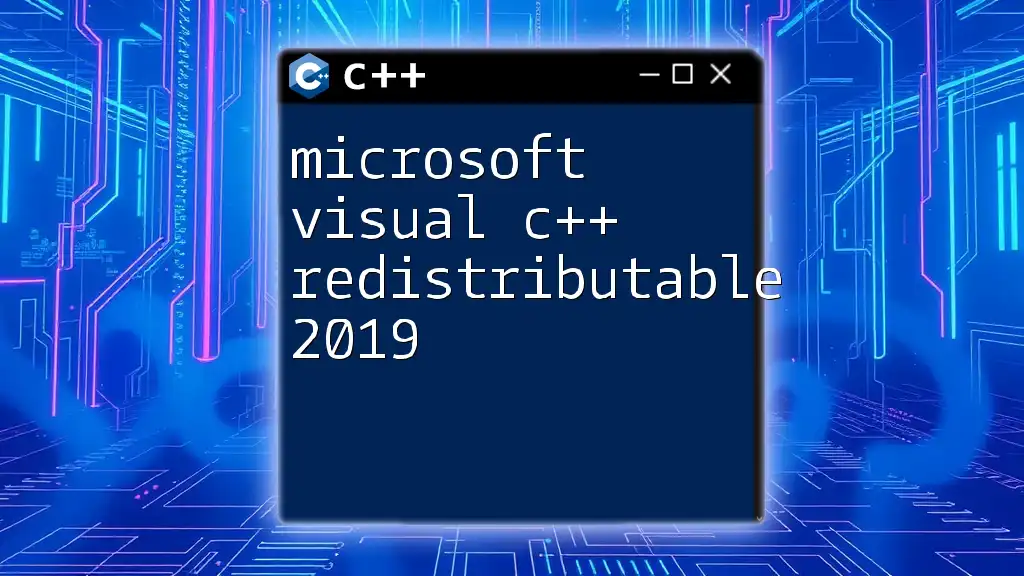
Installation of Microsoft Visual C++ Redistributable 2012
System Requirements
Before installing the Microsoft Visual C++ Redistributable 2012, it's important to verify that your system meets the following requirements:
- Operating Systems: Windows 7 (Service Pack 1) or later, Windows Server 2008 R2, Windows Server 2012, or Windows 8.
- Processor: 1 GHz or faster with x86 or x64 architecture.
- Memory: At least 512 MB of RAM (1 GB or more is recommended).
How to Download the Microsoft 2012 Visual C++ Redistributable
To download the Redistributable, follow these steps:
- Visit the official Microsoft Download Center.
- Search for Microsoft Visual C++ 2012 Redistributable.
- Select the appropriate version: x86 (for 32-bit applications) or x64 (for 64-bit applications).
- Click on the download link and follow the prompts to obtain the installer.
Installing the Redistributable Package
Once downloaded, follow these steps to install:
- Locate the downloaded installer file and double-click it to run.
- Accept terms and conditions when prompted.
- Follow the on-screen instructions to complete the installation.
Common installation errors may arise due to pre-existing software conflicts or missing system updates. If installation fails, ensure that your Windows OS is fully updated and temporarily disable any conflicting applications such as antivirus software during installation.
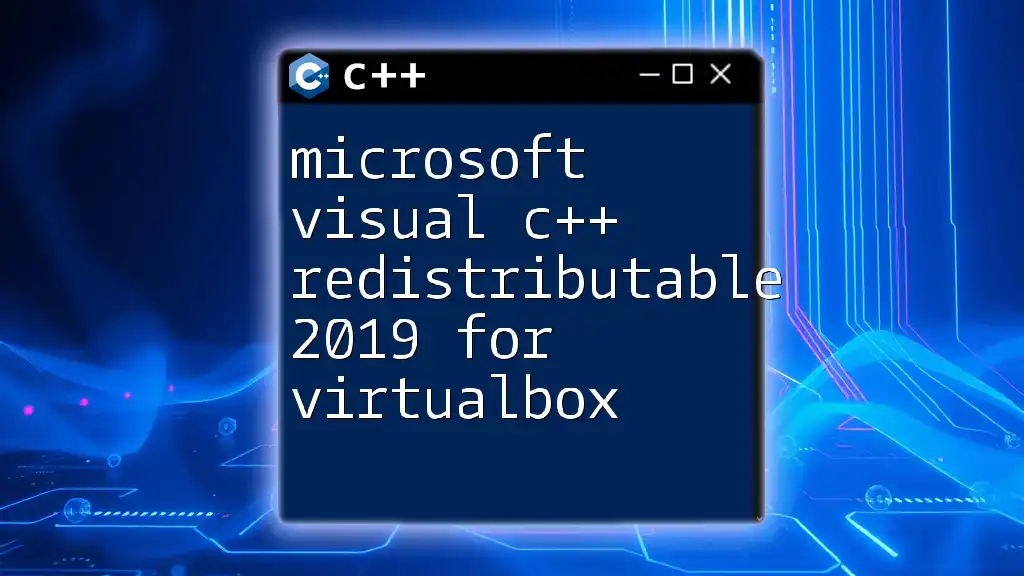
Common Issues and Troubleshooting
Identifying Missing Redistributable Errors
When users face missing component errors, it often manifests through messages indicating that specific DLL files are not found. For example, an error message may read:
The program can't start because msvcr110.dll is missing from your computer. Try reinstalling the program to fix this problem.
This signifies that the application relies on components from the Microsoft Visual C++ Redistributable 2012 that are not present on the system. The best course of action in this case is to download and install the redistributable package.
Troubleshooting Installation Problems
If you encounter issues during installation, consider the following solutions:
- Run as Administrator: Right-click the installer and select "Run as administrator" to ensure proper permissions.
- Check Windows Update: Ensure your operating system is up to date, as missing updates might impact the installation process.
- Uninstall Previous Versions: If a prior version of the C++ Redistributable is conflicting, uninstall it through the Control Panel before proceeding to install version 2012.
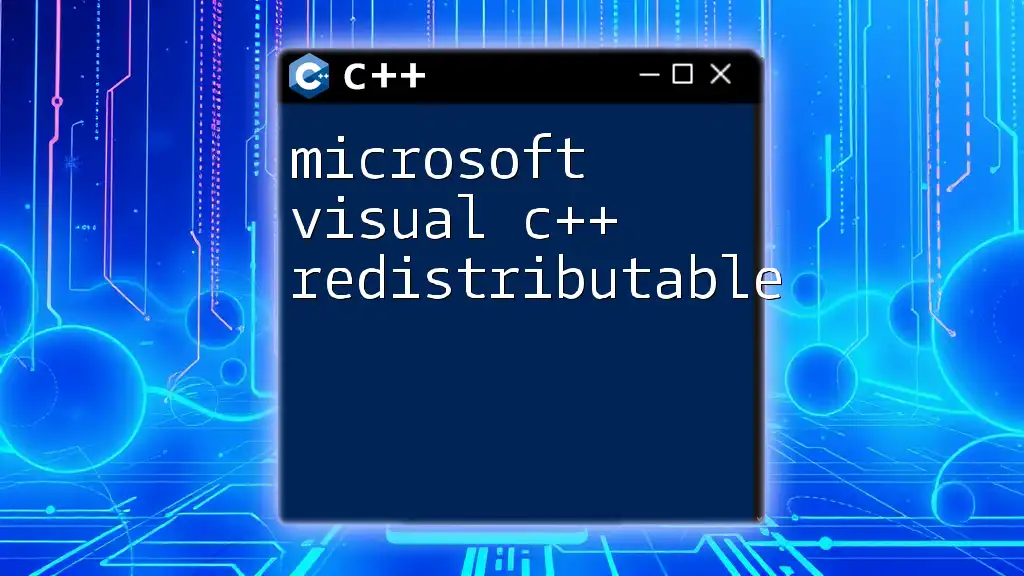
Using Microsoft Visual C++ Redistributable 2012 in Your Projects
Setting Up Your Development Environment
To utilize the Microsoft C++ redistributable 2012 in your projects, configure your Integrated Development Environment (IDE) properly. If you’re using Microsoft Visual Studio, you can add redistributable dependencies in the project settings.
For instance, to link your project:
- Open your project in Visual Studio.
- Navigate to the Project Properties.
- Go to Configuration Properties > C/C++ > Code Generation.
- Under Runtime Library, select the appropriate runtime for your project (e.g., Multi-threaded DLL (/MD)) that corresponds to the Redistributable.
Best Practices for Developers
When developing applications that utilize the Microsoft Visual C++ Redistributable 2012, consider the following best practices:
- Clearly document which version of the redistributable is required for your application.
- Include the Redistributable installer in your software package. This can simplify the installation for users who may not have the necessary components.
- Always test your application in an environment that mimics the end-user's environment to catch potential redistributable issues early in the development process.
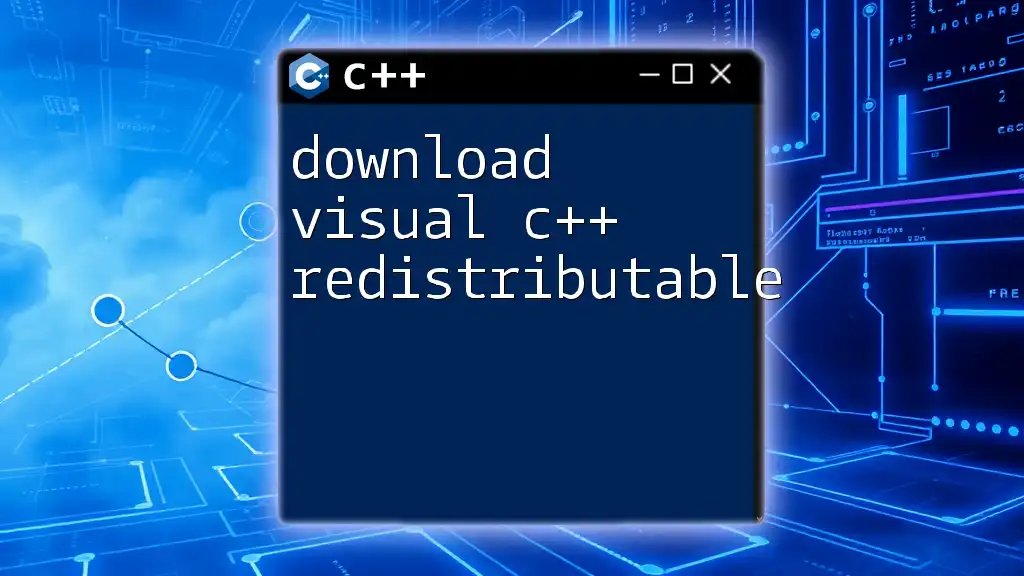
Conclusion
The Microsoft Visual C++ Redistributable 2012 is a vital component for running applications developed with Microsoft C++. Ensuring that users have the appropriate redistributable installed not only enhances application performance but also prevents frustrating errors. By understanding the importance of the redistributable, following proper installation procedures, and adhering to best practices in software development, you can deliver a seamless experience for your users, significantly reducing support requests and improving satisfaction ratings.
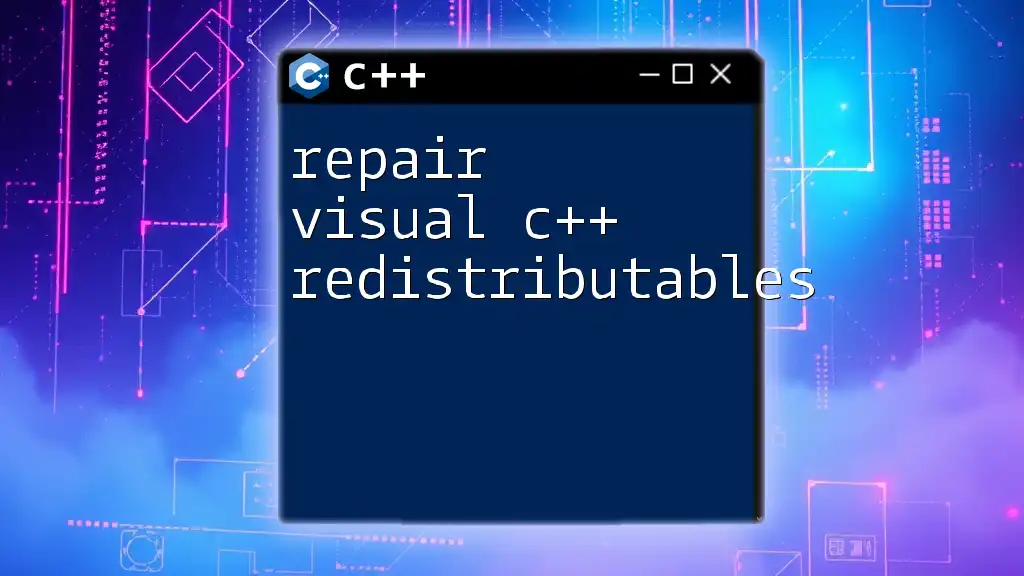
Additional Resources
For further information, consult:
- The official [Microsoft Documentation](https://docs.microsoft.com/en-us/cpp/standard-library/visual-cpp-redistributable-packages?view=msvc-160) for Visual C++ Redistributables.
- Online forums and community discussions regarding troubleshooting and advanced usage of Visual C++ Redistributables.
- Various coding tutorials and courses targeted at mastering Visual C++ development.
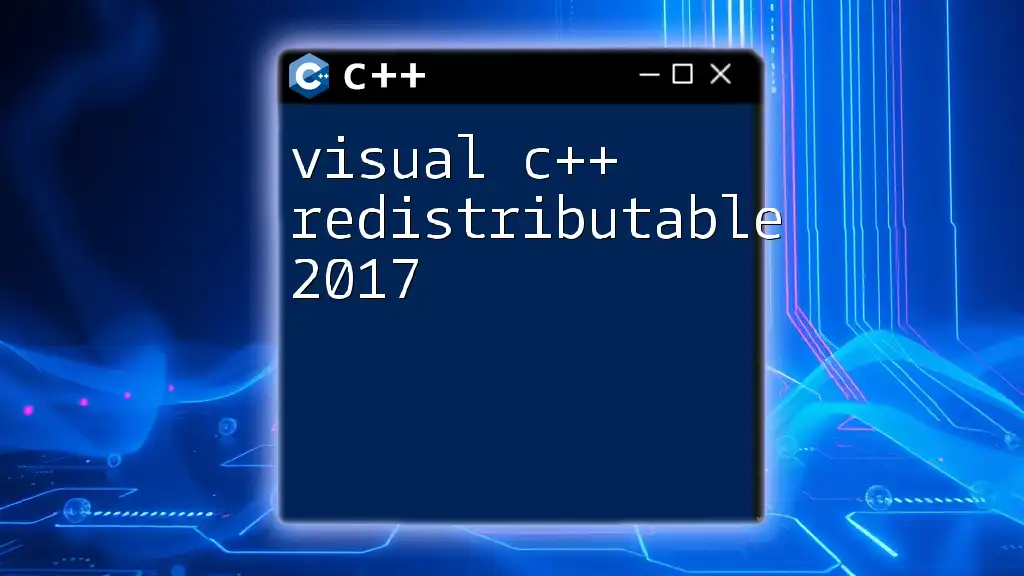
Call to Action
Stay updated with tips and tricks on utilizing C++ commands effectively by subscribing to our platform. Share your thoughts or any questions you have about the Microsoft C++ Redistributable 2012 and join a community passionate about programming!