The latest supported Visual C++ Redistributable downloads are essential for ensuring that applications developed with Microsoft Visual Studio run efficiently on your system by providing the necessary runtime components.
Here's an example code snippet for checking if the redistributable is installed in C++:
#include <iostream>
#include <windows.h>
bool IsRedistributableInstalled() {
HKEY hKey;
if (RegOpenKeyEx(HKEY_LOCAL_MACHINE,
TEXT("SOFTWARE\\Microsoft\\VisualStudio\\14.0\\VC\\Runtimes\\x86"),
0, KEY_READ, &hKey) == ERROR_SUCCESS) {
RegCloseKey(hKey);
return true; // Redistributable is installed
}
return false; // Not installed
}
int main() {
if (IsRedistributableInstalled()) {
std::cout << "Visual C++ Redistributable is installed." << std::endl;
} else {
std::cout << "Visual C++ Redistributable is not installed." << std::endl;
}
return 0;
}
Understanding Visual C++ Redistributables
What Are Visual C++ Redistributables?
Visual C++ Redistributables are packages that contain runtime components needed to run applications developed with Visual C++. They include libraries and necessary files that ensure consistent functionality across different devices and versions of Microsoft Windows. Unlike the Visual C++ Integrated Development Environment (IDE), which is used for developing applications, redistributables are meant for users who install software that depends on certain C++ libraries.
Why Do You Need Them?
In many cases, installed applications rely on specific versions of the Visual C++ runtime. Without the appropriate redistributables, you might encounter errors or the application might fail to run altogether. This is particularly relevant for software that has been developed with earlier or particular versions of Visual C++, as these applications may be looking for specific library versions that are not already present on the user's system.
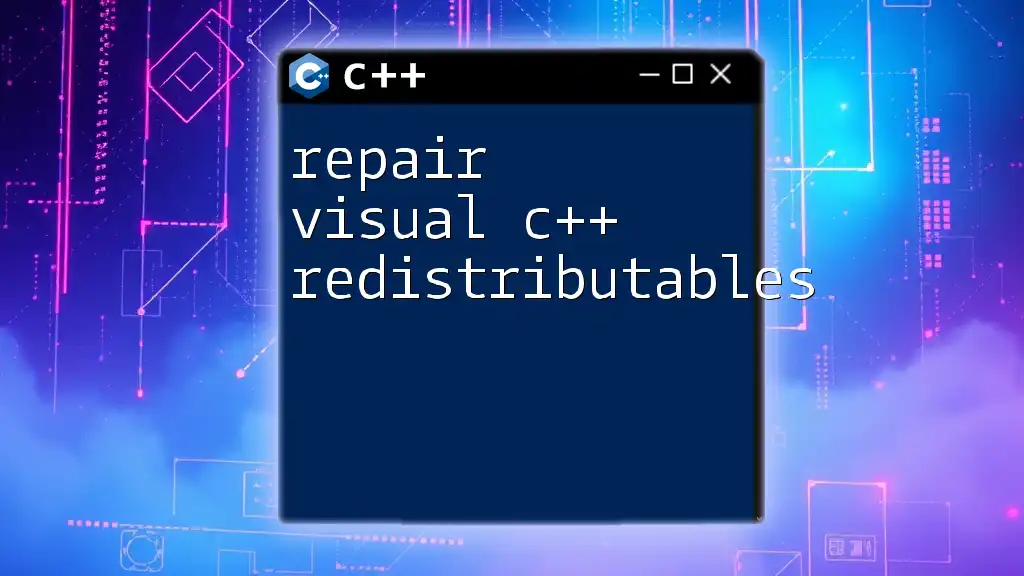
Latest Supported Visual C++ Redistributable Downloads
What Are the Latest Versions?
The latest visual C++ redistributables include releases that are compatible with Visual Studio 2015, 2017, 2019, and 2022. Each of these includes critical updates and fixes.
- Visual C++ 2015 Redistributable: Used by applications developed with Visual Studio 2015.
- Visual C++ 2017 Redistributable: Aimed at users with applications made with Visual Studio 2017.
- Visual C++ 2019 Redistributable: For software built with Visual Studio 2019.
- Visual C++ 2022 Redistributable: The most recent version supporting applications built with Visual Studio 2022.
Key Features of Each Version
Visual C++ 2015 Redistributable
This version introduced modern C++ features and improvements, particularly with runtime security and stability. This version is crucial for older applications that still rely on it for stability.
Visual C++ 2017 Redistributable
Key improvements include additional security measures, bug fixes, and better performance optimization compared to its predecessor. This version supports applications that require a more efficient runtime environment.
Visual C++ 2019 Redistributable
The 2019 version brought enhancements for the C++ standard libraries and optimization for new hardware designs. It also tackled many bugs and included a more robust support system for asynchronous programming.
Visual C++ 2022 Redistributable
As the latest iteration, this version combines all the improvements from prior releases while focusing on adding modern C++20 features. It provides enhanced performance and security optimizations that every developer should leverage.
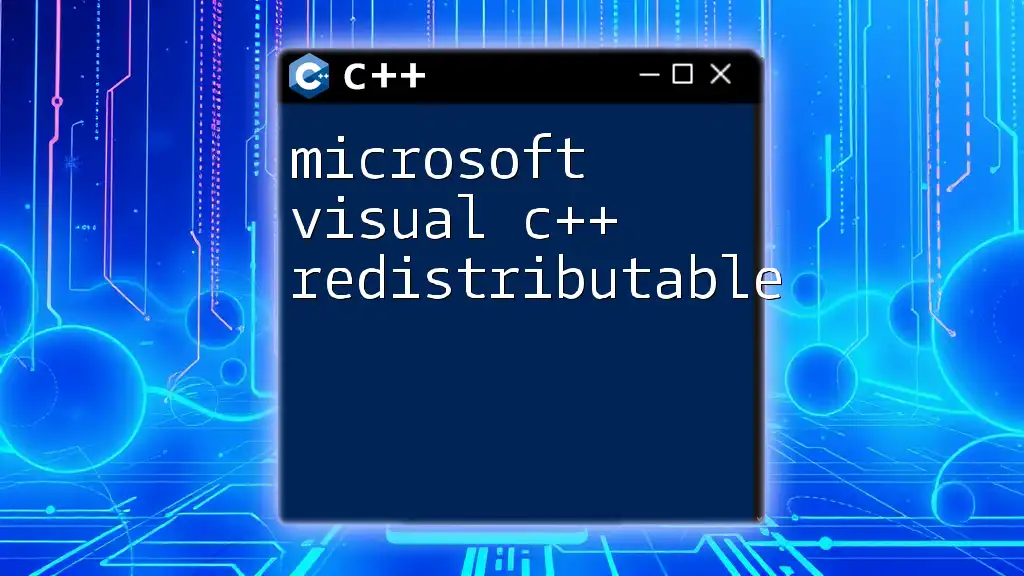
How to Download and Install the Redistributables
Step-by-Step Guide to Downloading
To download the latest supported Visual C++ redistributable packages, visit the official Microsoft website. Ensure you are downloading the correct version for your application. The official site often hosts the most up-to-date versions, ensuring your software will run smoothly.
Installation Process
- Run the downloaded installer: Just double-click on the downloaded file to launch the installation wizard.
- Follow the on-screen instructions: Accept the license agreement, choose your installation folder, and click install.
- Wait for the installation to complete: This generally takes a few minutes depending on your system specifications.
Verifying Installation
To check whether the redistributables have been installed correctly:
- Press `Win + R`, type `appwiz.cpl`, and hit Enter. This opens the "Programs and Features" window.
- Look for "Microsoft Visual C++ Redistributable" in the list. You should see all installed versions here.
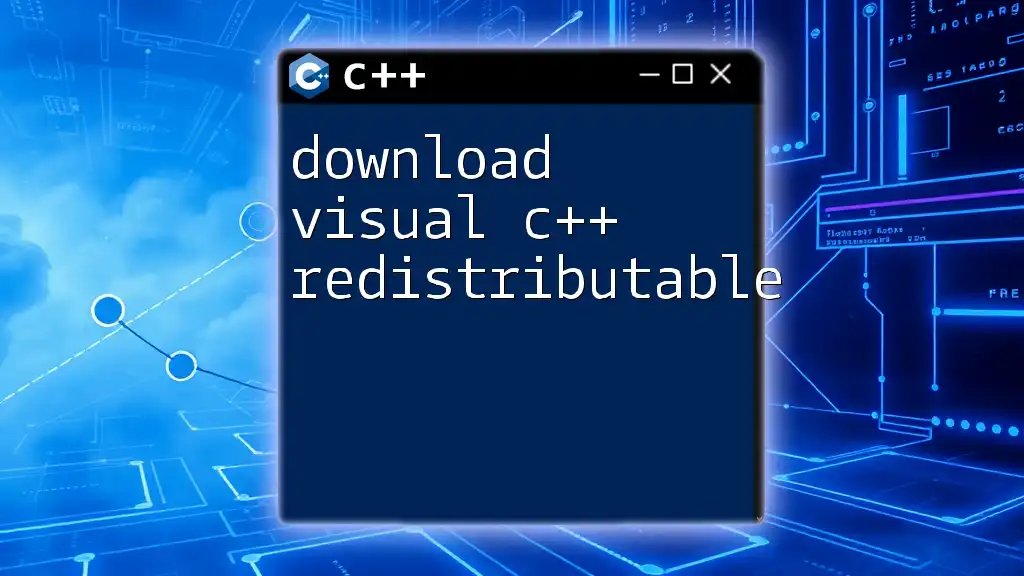
Common Issues and Troubleshooting
Common Errors Related to Missing Redistributables
You may encounter error messages such as:
- "This application failed to start because it could not find the MSVCRXXXX.dll."
- "The program can't start because MSVCPXXXX.dll is missing."
These indicate that the application is dependent on a specific redistributable version that isn't installed on your system.
How to Resolve Installation Issues
If you face problems during installation, try the following:
- Ensure you have the right version for your application.
- Close all instances of the application you are trying to run.
- Restart your computer and attempt re-installing the redistributable.
For more help, Microsoft provides support documentation that addresses specific issues.
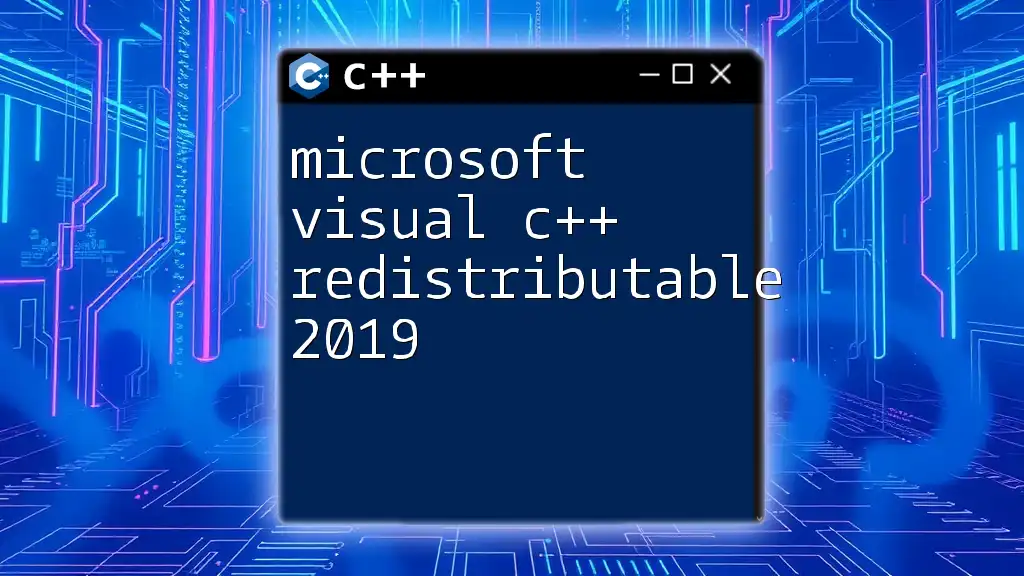
Code Examples and Use Cases
Integrating Redistributables with C++ Projects
When developing a C++ application, it’s essential to ensure that your project settings include the correct redistributable dependencies, especially when distributing the application.
Here’s an example of a basic console application that requires the Visual C++ runtime:
#include <iostream>
#include <windows.h>
int main() {
// Basic program that requires Visual C++ runtime
std::cout << "Hello, Visual C++ Redistributable!" << std::endl;
return 0;
}
This simple program outputs a message to the console. If the appropriate version of the Visual C++ Redistributable is not installed on the user’s machine, they might receive an error when they try to run this application.
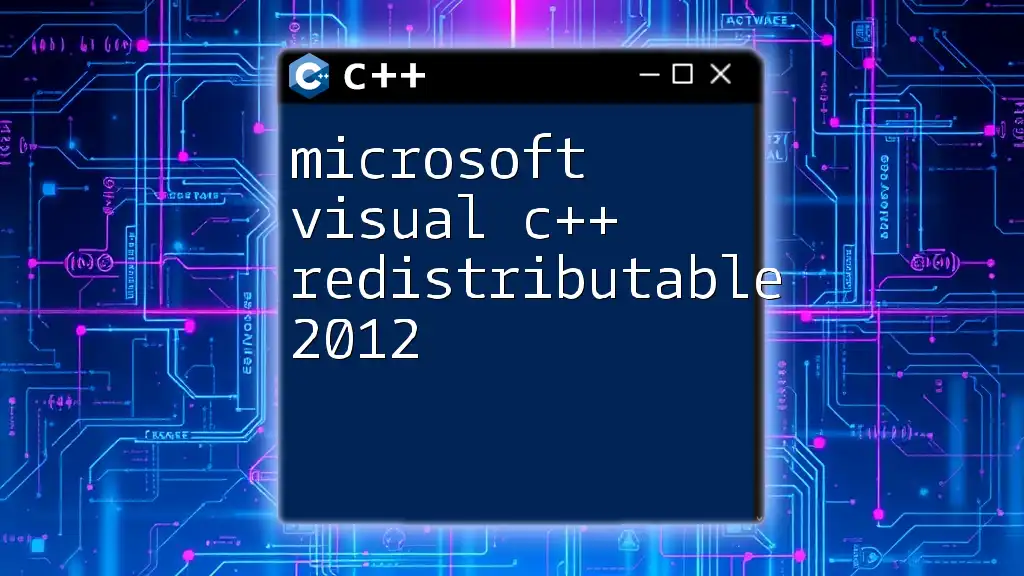
Best Practices for Developers
Ensuring Compatibility
Always reference the specific versions of the redistributables required for your application. This ensures that users won’t face issues related to missing libraries.
Regular Updates
Frequent updates can prevent potential running issues and provide performance enhancements. Monitor official releases and communicate any updates clearly to your users.
Distribution Considerations
When packaging applications for distribution, consider bundling the necessary redistributables or including a clear installation guide. This will minimize the risk of runtime errors and ensure a smoother user experience.
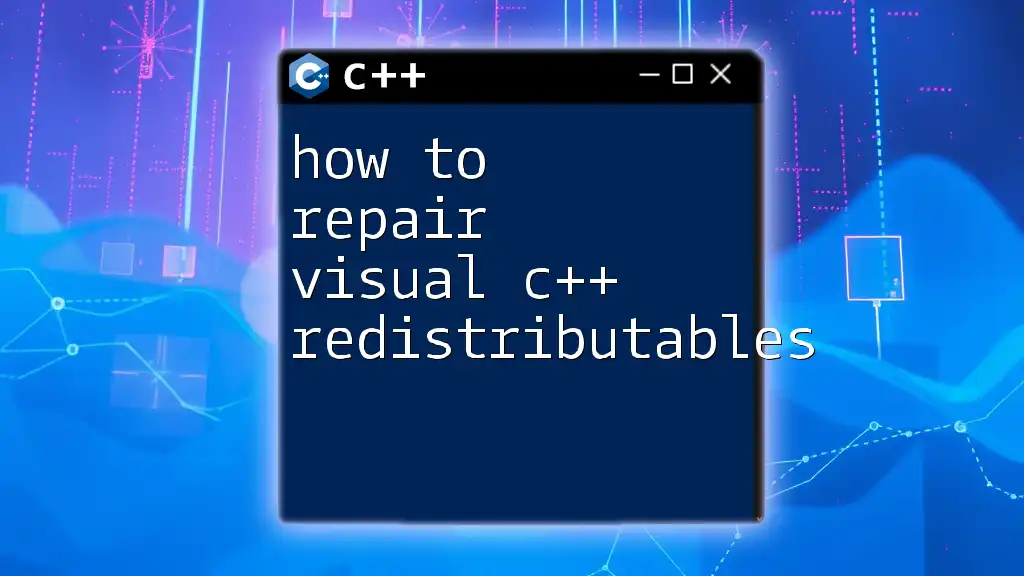
Conclusion
Visual C++ Redistributables are integral for running applications developed using different versions of C++. Staying updated with the latest supported Visual C++ redistributable downloads not only ensures compatibility but also enhances performance and security. By understanding their necessity and following best installation practices, developers can provide a better experience for their users. Always encourage users to keep their environments updated and check for dependencies critical to their applications.
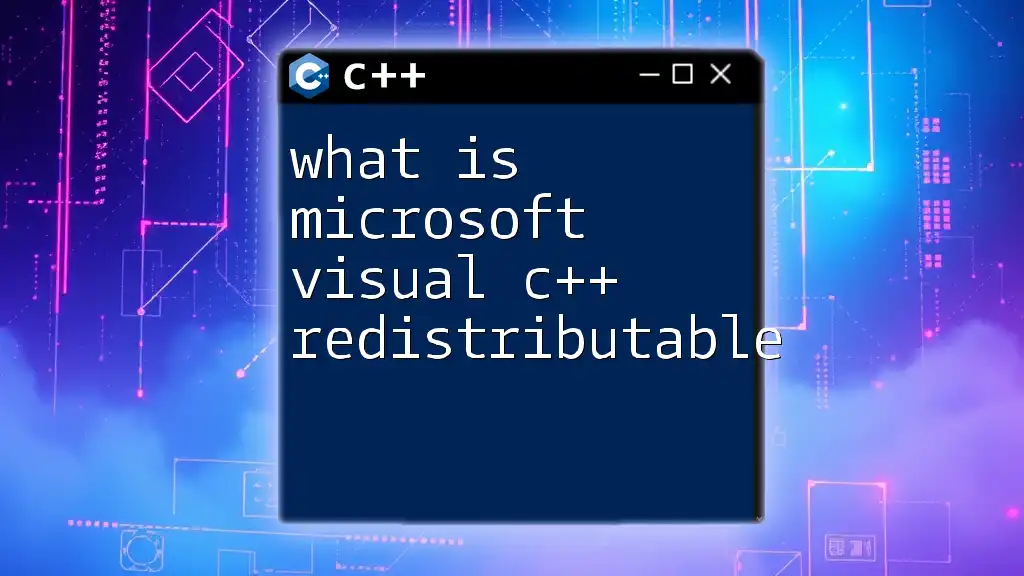
Additional Resources
- Official Microsoft Documentation
- Further Reading on Visual C++ Programming
- Links to Community Forums and Help Sites
This comprehensive guide should serve as a valuable resource for understanding and implementing the latest supported Visual C++ redistributable downloads.