The Microsoft Visual C++ Runtime Library provides essential functions and classes that allow C++ applications developed with Visual Studio to run efficiently on Windows by facilitating memory management, input/output operations, and exception handling.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Runtime Libraries
Definition of a Runtime Library
A runtime library is a collection of software functions and routines that a program can call at runtime, providing essential support during the execution of the program. This library plays a critical role in a programming environment by allowing developers to utilize pre-written functionalities, thus enhancing productivity and reducing the need to write repetitive code.
How Runtime Libraries Function
When a program is executed, it often requires a variety of functions to perform tasks such as memory allocation, string manipulation, or file operations. The runtime library establishes a layer between the application and the operating system, facilitating the execution of these functions. For example, when a program needs to open a file, it makes a call to the runtime library, which then handles the interaction with the operating system to fulfill this request.
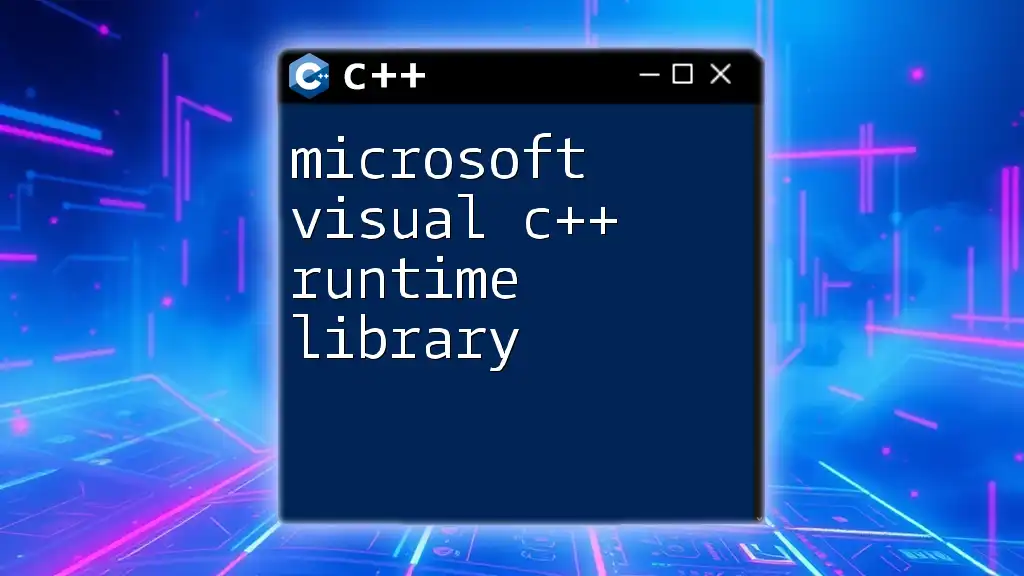
What is Microsoft Visual C++ Runtime Library?
Overview
The Microsoft Visual C++ (MSVC) Runtime Library is a crucial component of the Microsoft development environment, specifically designed for C and C++ programming languages. It provides a comprehensive set of libraries that programmers can utilize to create efficient and high-performance applications. Historically, this library has evolved alongside the Microsoft Windows operating system, maintaining a consistent support structure for developers.
Key Components
-
CRT (C Runtime): The CRT is the backbone of the MSVC runtime library. It offers a variety of robust functions needed for standard C programming. From memory management with functions like `malloc` and `free` to file operations (`fopen`, `fclose`), the CRT simplifies commonly used tasks, ensuring developers can build applications effectively.
-
MFC (Microsoft Foundation Classes): MFC is an object-oriented framework that simplifies the development of Windows applications by providing a comprehensive set of functions for working with graphical user interfaces. It offers support for creating windows, managing controls, and handling events, which are essential for application development. For example, a simple MFC application can be set up like this:
#include <afxwin.h> // MFC core and standard components class CMyApp : public CWinApp { public: virtual BOOL InitInstance() { CFrameWnd* pFrame = new CFrameWnd(); pFrame->Create(NULL, _T("My Application")); pFrame->ShowWindow(SW_SHOW); m_pMainWnd = pFrame; return TRUE; } }; CMyApp theApp; // Entry point for the application
-
C++ Standard Library: The MSVC runtime incorporates features from the C++ Standard Library, which includes important components such as containers (like vectors and maps), algorithms, and input/output capabilities. This integration allows developers to use modern C++ practices while still relying on the powerful capabilities of MSVC.
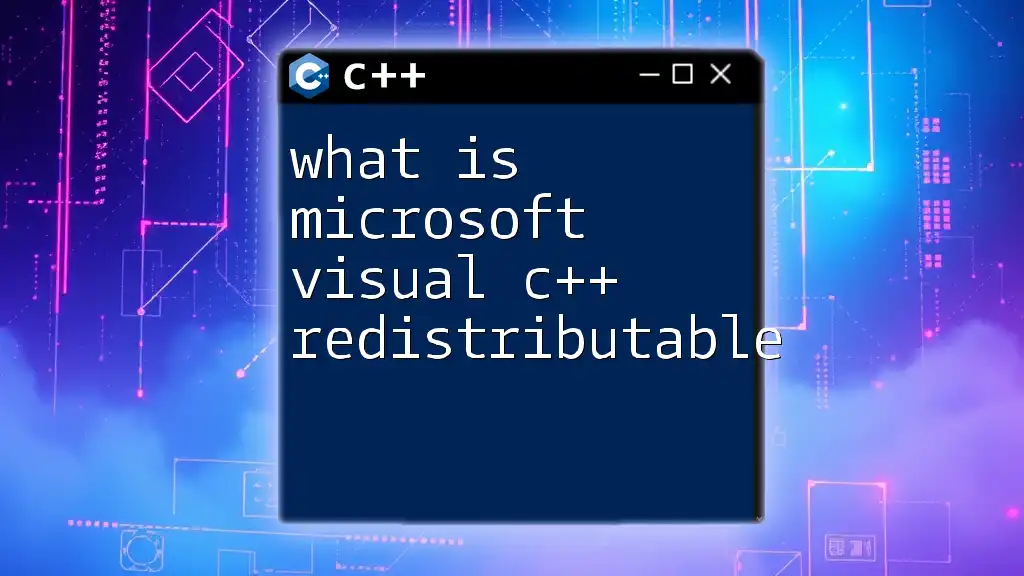
Importance of the Runtime Library
Facilitating Application Development
The Microsoft Visual C++ Runtime Library significantly simplifies application development. By providing standardized tools and libraries, developers can focus on building innovative features without reinventing fundamental functionalities. This means less time spent on error-prone, low-level code, leading to faster development cycles and more robust applications.
Ensuring Compatibility
One of the runtime library's primary roles is to ensure that applications can operate smoothly across different Windows versions. Compatible applications function correctly whether they are deployed on Windows 10, Windows 8, or earlier versions, thanks to the runtime library's careful handling of underlying changes in the OS. This compatibility is crucial for businesses that rely on legacy applications while adopting new technologies.
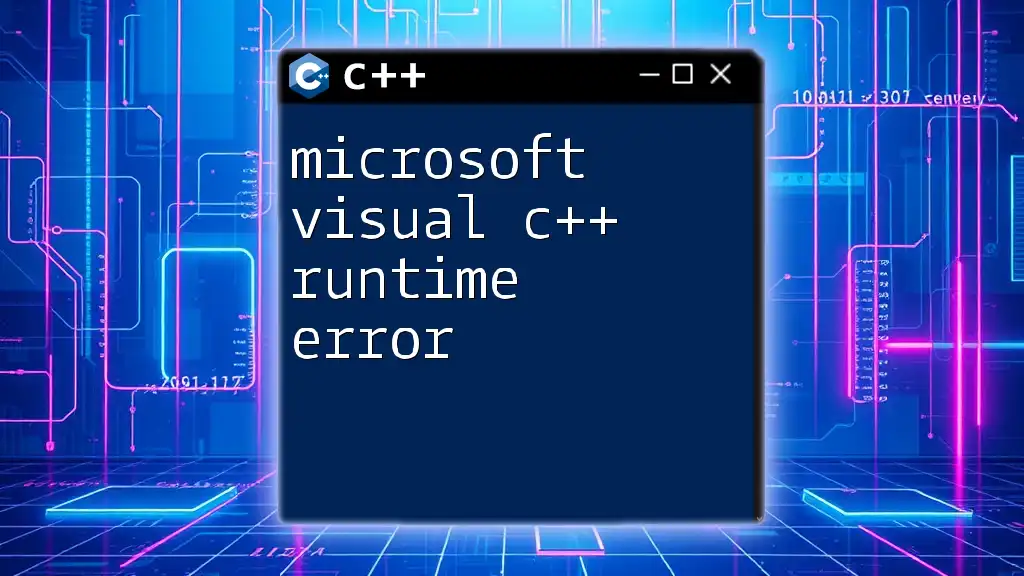
Common Uses of Microsoft Visual C++ Runtime Library
Windows Application Development
In the realm of Windows application development, the Microsoft Visual C++ Runtime Library serves as an indispensable toolkit. By leveraging its built-in functionalities, developers can create high-performance applications tailored to the Windows environment. A simple example of a Windows application would be:
#include <iostream>
#include <windows.h>
int main() {
MessageBox(NULL, "Hello, World!", "Hello Dialog", MB_OK);
return 0;
}
This snippet uses the Windows API to present a message box, demonstrating how MSVC can streamline Windows-based programming.
Game Development
Game developers also benefit greatly from the Microsoft Visual C++ Runtime Library. Given the resource-intensive nature of games, developers can rely on the efficiency of the CRT for memory management and performance optimization. By using the runtime library, they can avoid common pitfalls such as memory leaks, thus enhancing overall game stability and player experience.
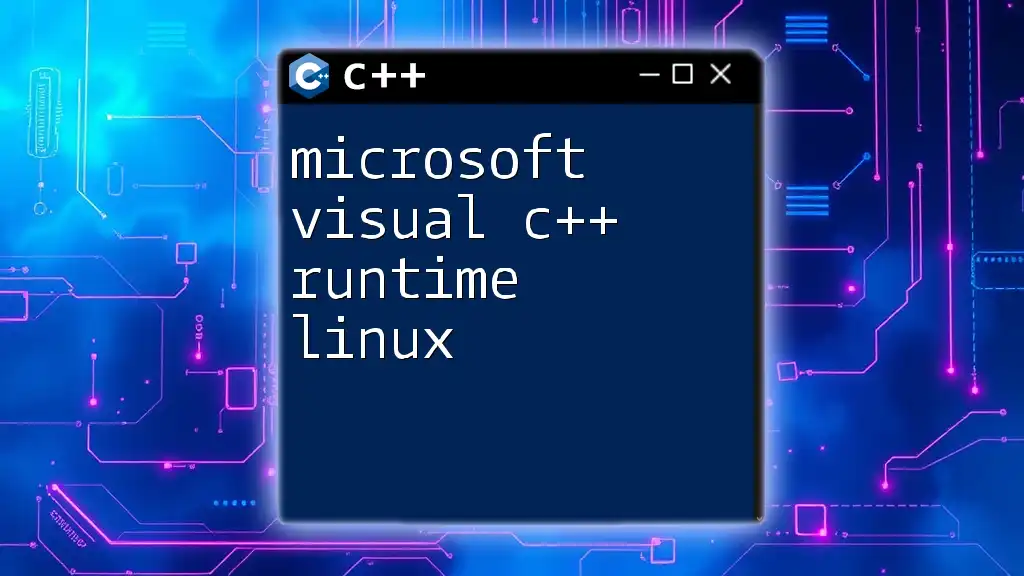
Installing Microsoft Visual C++ Runtime Library
Why Installation is Necessary
Installing the runtime library is essential for running many third-party applications that depend on specific versions of the MSVC libraries. If an application tries to use functions that aren't available on your machine, it can lead to errors and crashes.
Installation Process
To install the Microsoft Visual C++ Runtime Library:
- Download: Go to the official Microsoft website and navigate to the Visual Studio downloads section.
- Choose Version: Select the appropriate version of the runtime library that matches the needs of the application you want to run.
- Run Installer: Launch the installer and follow the prompts to complete the installation.
- Restart (if necessary): Some installations may require you to restart your computer.
Troubleshooting Common Installation Issues:
- Ensure that you are downloading the correct version (x86 vs. x64) based on your system architecture.
- Check for existing installations of the runtime to avoid conflicts.
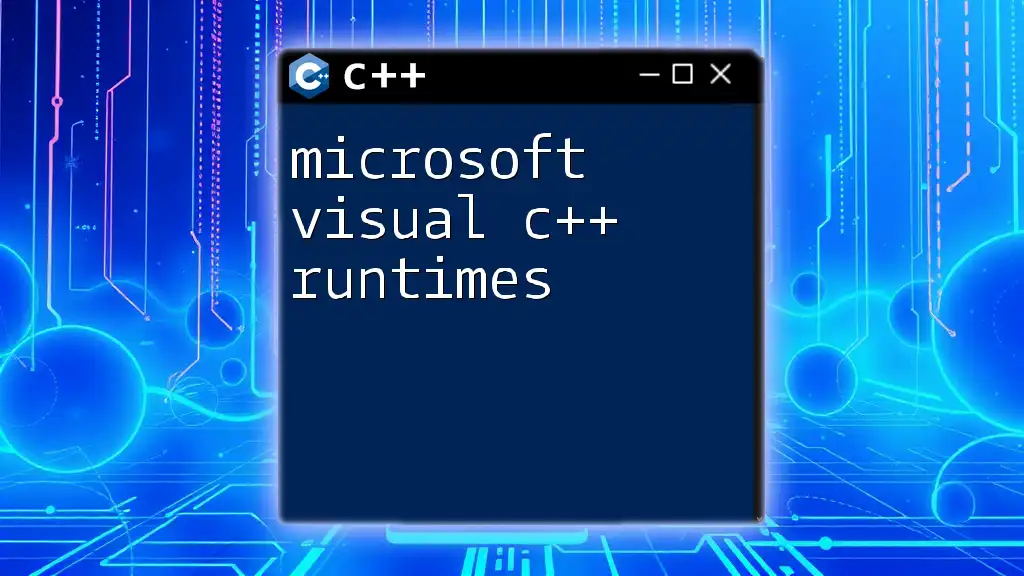
Common Errors Related to MSVC Runtime Library
Identifying Runtime Errors
Common runtime errors related to the Microsoft Visual C++ Runtime Library usually manifest as error messages indicating that certain DLL files are missing or incompatible. For instance, users may encounter messages like "msvcrxxx.dll is missing." These errors can prevent applications from loading, causing frustration for both developers and end-users.
Solving Runtime Issues
To address runtime issues effectively:
- Reinstall the Microsoft Visual C++ Runtime Library. This often resolves DLL-related problems.
- Use Dependency Walker: This tool allows you to analyze which DLLs an application depends on and identify missing files.
- Update Your Windows: Sometimes, updating your Windows installation resolves compatibility issues or missing system components.
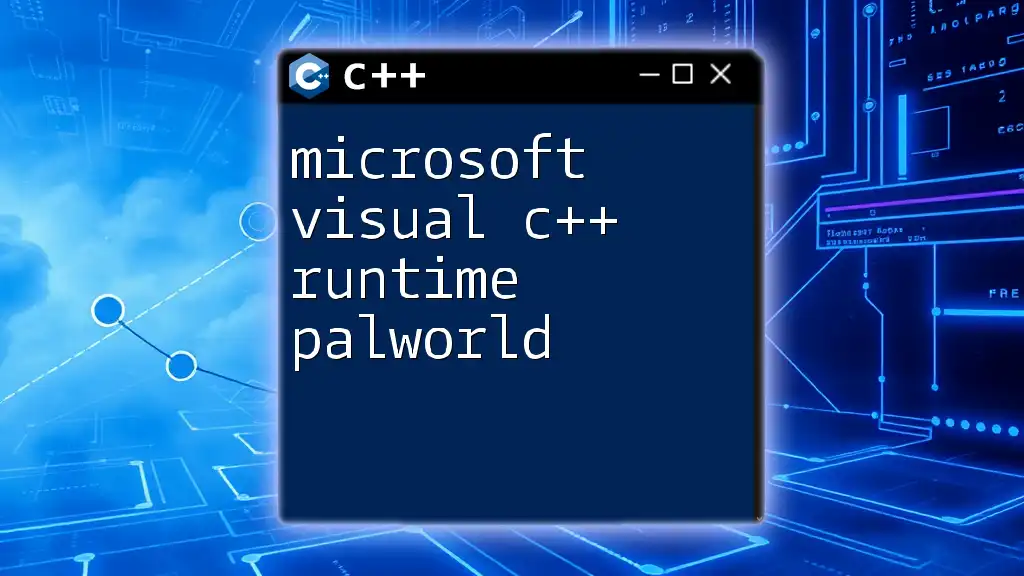
Conclusion
The Microsoft Visual C++ Runtime Library is a pivotal resource for developers, providing essential tools and functionalities that streamline the development process and ensure compatibility across various Windows platforms. By understanding its components and functionality, programmers can greatly enhance their productivity and reduce the likelihood of common runtime errors. Embracing this library is a crucial step toward mastering application development in the C and C++ environments.
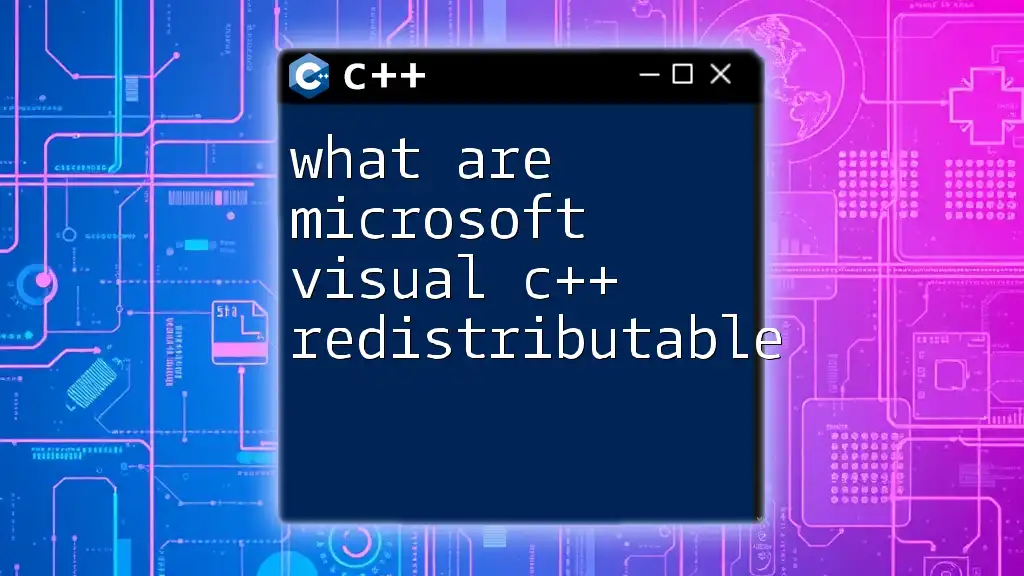
Additional Resources
For those eager to delve deeper into learning and mastering the Microsoft Visual C++ environment, consider exploring:
- The official Microsoft documentation for [Microsoft Visual C++](https://docs.microsoft.com/en-us/cpp/?view=msvc-160)
- Recommended books on C++ programming and the Visual Studio development environment
- Online courses and tutorials focused on C++ and MSVC development techniques.