Visual C++ Runtime is a collection of libraries and components that are necessary for running applications developed with Microsoft Visual C++, allowing them to execute properly on Windows systems.
Here’s a simple code snippet that demonstrates the inclusion of a standard library in a Visual C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Visual C++
Visual C++ is a powerful programming environment developed by Microsoft as part of the Visual Studio suite. It is designed for C++ programming and offers developers a richly integrated environment where they can create, debug, and manage their applications efficiently.
Emerging in the early days of Windows application development, Visual C++ has grown to be a crucial tool, providing features like code editing, debugging, and project management. Its capabilities extend beyond standard C++ programming, integrating with various Microsoft technologies that allow for the creation of robust applications, including desktop software, web applications, and even games.
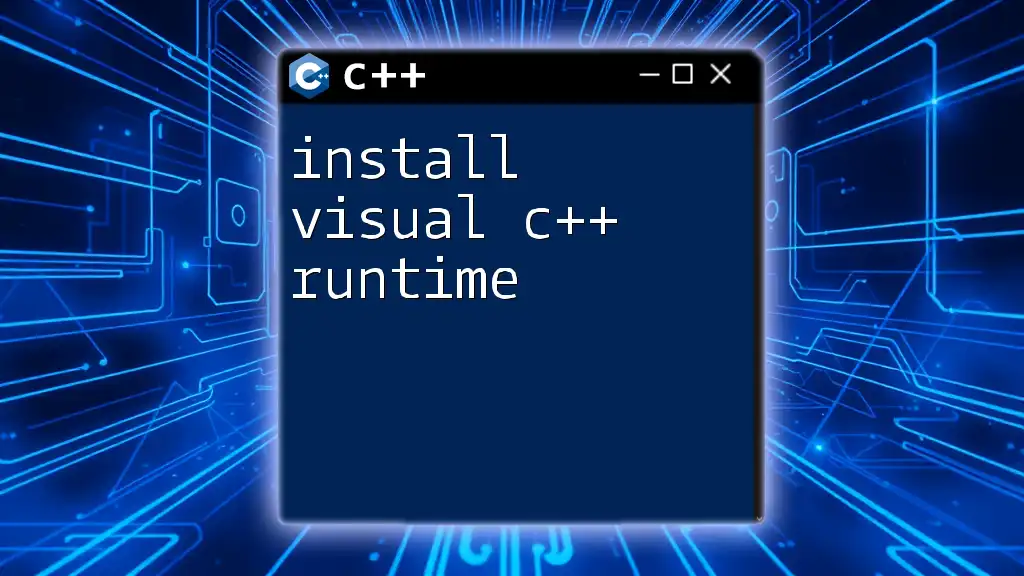
What is Visual C++ Runtime?
When discussing Visual C++ Runtime, we refer to a set of libraries and components essential for running applications built with Visual C++.
The Visual C++ Runtime consists of runtime libraries that provide various functions needed by the executable files produced by Visual C++. These libraries facilitate functions like memory management, input/output processing, and error handling, ensuring that applications run smoothly across different systems.
In essence, the Visual C++ Runtime bridges the compiled application code and the operating system, providing the necessary tools that the application needs to run without issues.
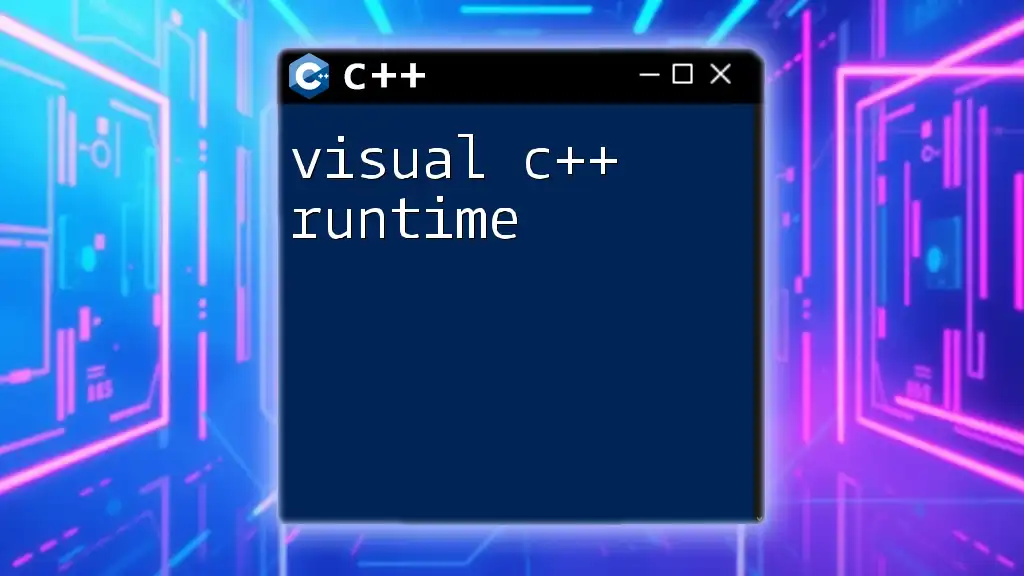
Components of Visual C++ Runtime
Core Components
The Visual C++ Runtime is primarily made up of several core components:
-
C Runtime Library (CRT): This library offers fundamental routines for programming in C and C++, including functions for string manipulation, input/output, and file operations.
-
Standard Template Library (STL): STL consists of template classes and functions that allow for the efficient management of collections of data and algorithms. This library is integral to modern C++ programming and empowers developers to create more complex data structures and algorithms easily.
Supporting Libraries
Additionally, there are supporting libraries that extend the functionality of Visual C++ applications:
-
MFC (Microsoft Foundation Class) Framework: MFC provides a set of classes that simplify the process of building Windows applications. It offers features for creating user interfaces, managing documents, and handling messages in the Windows environment.
-
ATL (Active Template Library): ATL is a set of template-based C++ programming classes designed for developing lightweight COM components and ActiveX controls. It focuses on performance and scalability, making it ideal for server-side applications.
Core Libraries Explained
C Runtime Library (CRT)
The CRT is fundamental for applications developed in C or C++. It provides essential functionalities such as memory management, string handling, and file operations.
For example, simple I/O operations can be performed using the CRT like this:
#include <cstdio>
int main() {
printf("Hello, World!\n");
return 0;
}
This code snippet showcases how the `printf` function from the CRT can be used to output text to the console, a fundamental operation for any C++ application.
Standard Template Library (STL)
The STL introduces a range of powerful tools for managing collections of data. For instance, vectors are dynamic arrays provided by STL that allow for easy manipulation of data.
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
This example shows how to declare a vector, initialize it with elements, and iterate over its contents, reflecting the simplicity and efficiency that STL brings to C++ programming.
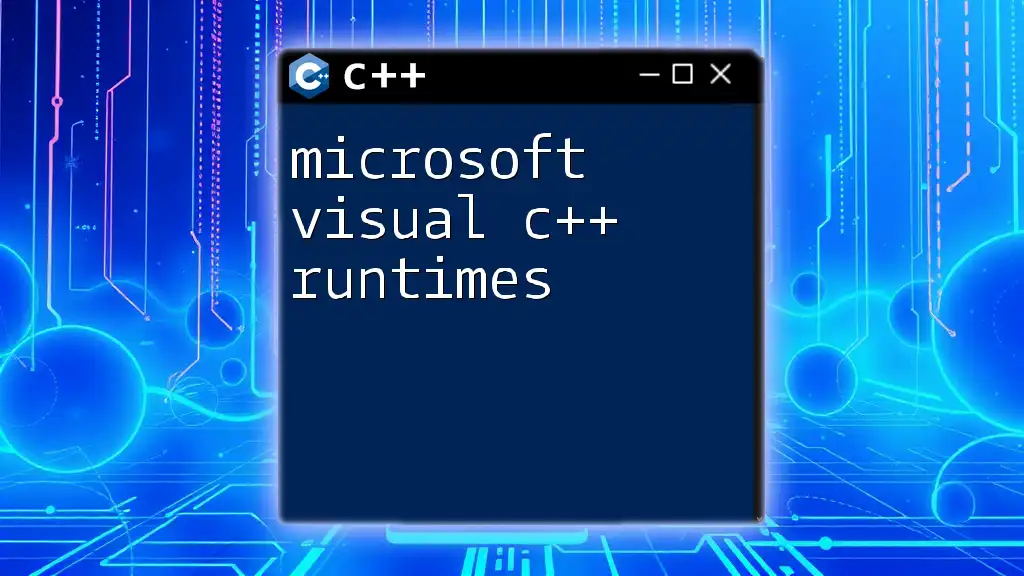
Importance of Visual C++ Runtime
Visual C++ Runtime plays a crucial role in ensuring that applications created using Visual C++ function as intended regardless of the environment they are executed in.
Compatibility Across Applications
One of the primary purposes of the runtime is to maintain compatibility across various applications. When a developer updates their software, the runtime ensures that these updates are implemented correctly without needing to rebuild the entire application. If components are missing, it can lead to runtime errors—issues that disrupt the user experience and are often tied to missing libraries or outdated runtime installations.
Performance Optimization
The runtime also significantly contributes to the performance of applications. By managing resources efficiently during runtime, it allows applications to run faster and with less overhead. This optimization is vital for high-performance computing applications where resource management is critical.
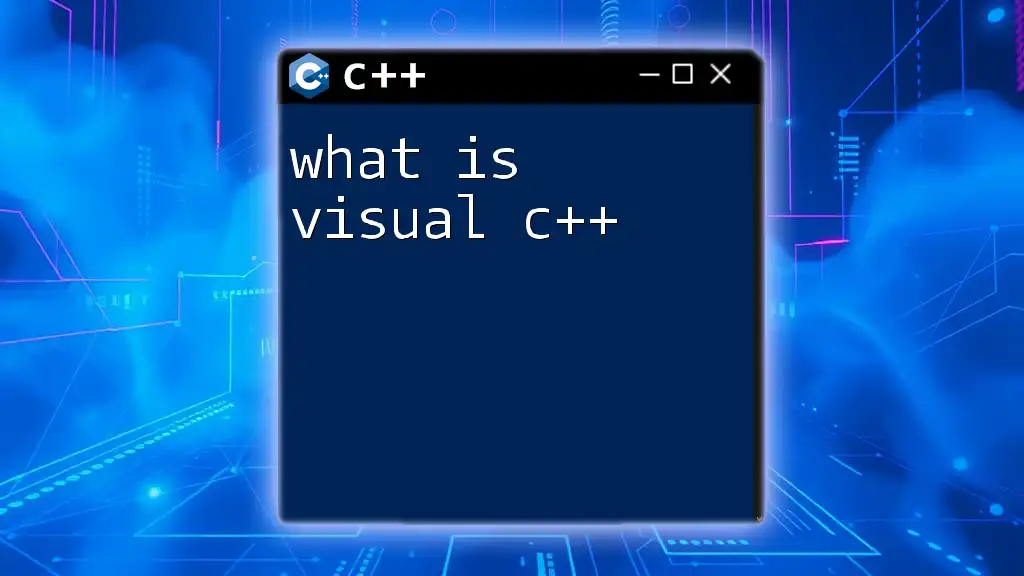
Common Errors Related to Visual C++ Runtime
Runtime Errors
Working with Visual C++ Runtime can sometimes lead to runtime errors. Common issues include missing DLLs or mismatches between the application and the installed runtime version. These errors can manifest as application crashes, unexpected behavior, or system compatibility problems.
Debugging Visual C++ Runtime Errors
Debugging is an essential skill for developers working with Visual C++. Tools integrated within Visual Studio can assist developers in identifying and resolving these runtime errors. The process typically involves using breakpoints, watches, and call stack inspections to locate issues within the code.
For example, if an application crashes due to a missing DLL, developers can use Dependency Walker or Visual Studio's built-in tools to analyze dependencies and pinpoint the exact missing components needed for the application to function correctly.
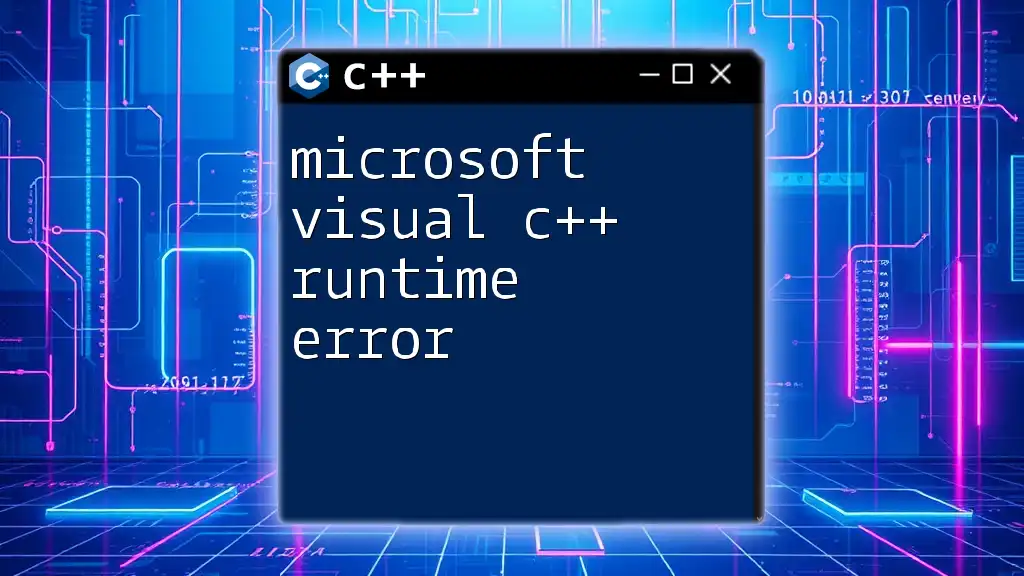
Installing Visual C++ Runtime
Installing the Visual C++ Runtime is often a straightforward process, but it's crucial for ensuring that applications run correctly on Windows systems.
How to Install Visual C++ Redistributable
The Visual C++ Redistributable is a package that contains the required runtime components. Installation typically involves downloading the appropriate version from the Microsoft website and running the setup. It is essential to select the correct version for your operating system (32-bit vs 64-bit), as applications built with Visual C++ may target different architectures.
Updating the Runtime
Keeping the Visual C++ Runtime updated is vital for security and performance. Regular updates often contain bug fixes, optimizations, and security patches that improve application performance and stability. Users should regularly check for updates on Microsoft’s download page to ensure they are using the latest runtime version.
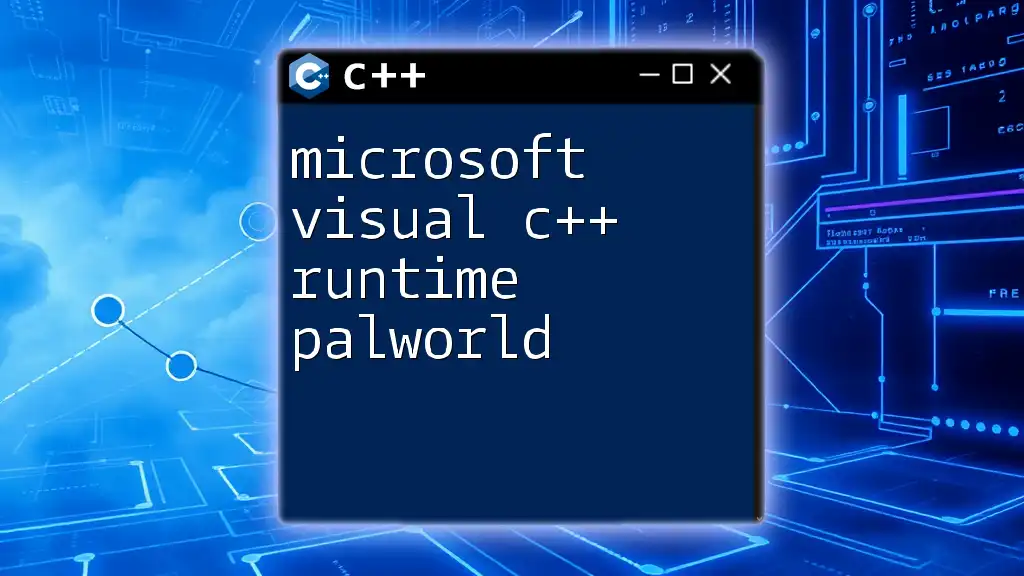
Best Practices for Using Visual C++ Runtime
Development Considerations
When developing applications with Visual C++, it is essential to choose the correct runtime configurations. This means deciding between static and dynamic linking, depending on the specific needs of your application. Static linking embeds the necessary libraries within the executable, while dynamic linking relies on the runtime installation available on the host machine.
Performance Best Practices
To maximize the benefits of Visual C++ Runtime, developers should apply performance best practices. This includes optimizing code for speed and memory usage, avoiding memory leaks, and minimizing the overhead caused by unnecessary runtime checks.
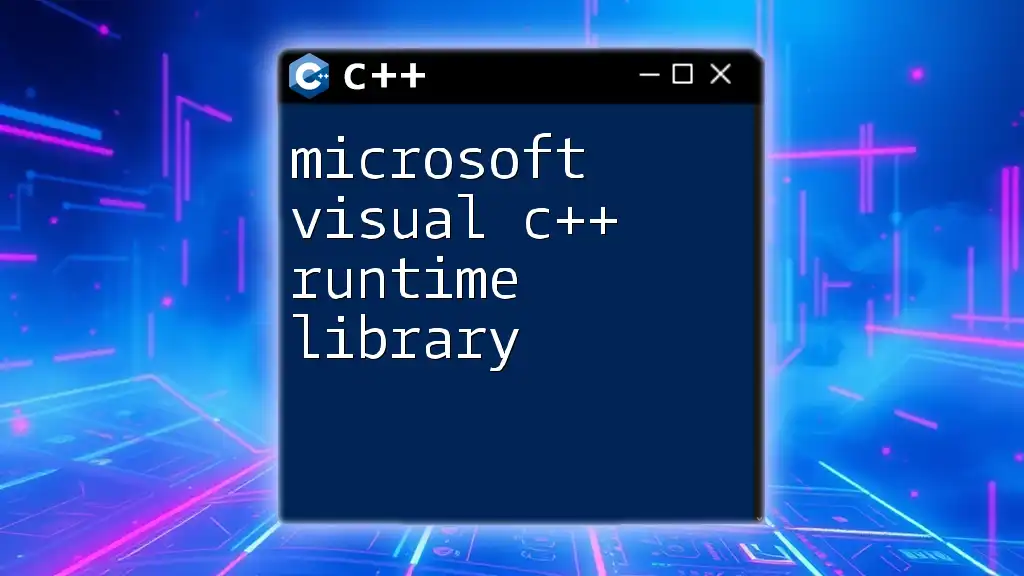
Conclusion
In summary, Visual C++ Runtime is a foundational aspect of C++ application development that ensures compatibility and performance. Understanding the components, installation, and common issues surrounding it is crucial for any developer aiming to build efficient C++ applications. Armed with this knowledge, programmers can harness the full potential of Visual C++ and create applications that run seamlessly across various Windows environments.
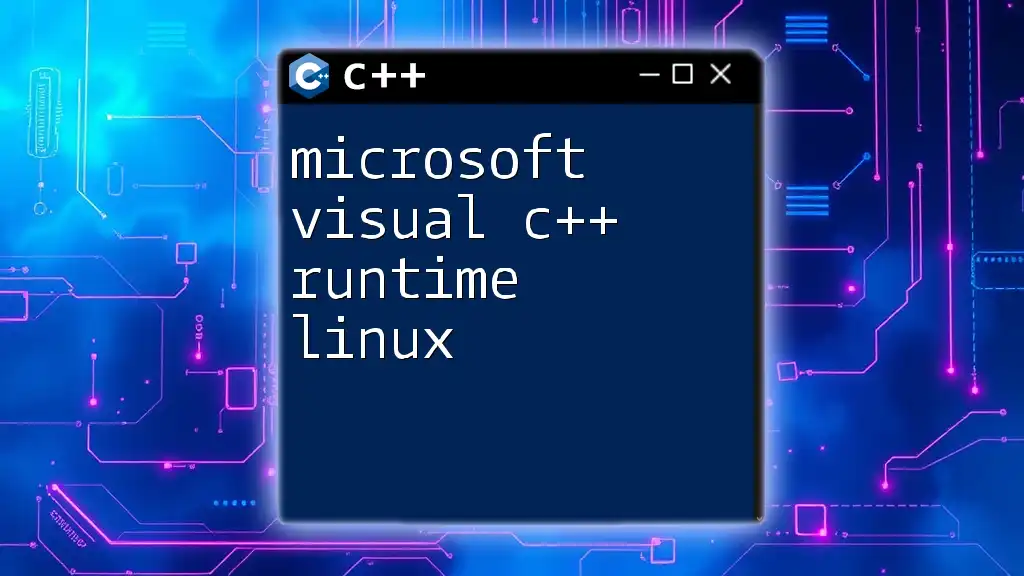
Additional Resources
To further explore Visual C++ Runtime, developers can refer to official documentation provided by Microsoft, books dedicated to C++ programming, or online courses that cover advanced topics in Visual C++. Engaging with these resources can deepen understanding and skill in utilizing Visual C++ to its fullest extent.