C++ runtime refers to the period during which a C++ program is executing, and it encompasses the management of memory, execution flow, and the dynamic features of the C++ language.
Here’s a simple code snippet demonstrating a basic C++ program that outputs text during its runtime:
#include <iostream>
int main() {
std::cout << "Hello, C++ Runtime!" << std::endl;
return 0;
}
Understanding C++ Runtime
What is C++ Runtime?
C++ runtime refers to the period when a C++ program is executed, and it encompasses the dynamic aspects of that execution. This phase is vital because it differs significantly from compile-time, where the code is translated into machine language. The runtime environment allows the program to operate and manage memory, handle exceptions, and realize the functionalities defined in the source code.
The Role of Runtime in C++
In C++, the runtime acts as a bridge between the compiled code and the operating system. It plays a crucial role in managing the execution process. Unlike compile-time checks, which validate the syntax and semantics of code before execution, the runtime environment deals with actions that occur while the program is running.
Understanding the distinction between compile-time and runtime helps developers write more efficient and functional code, recognizing where potential issues may arise.
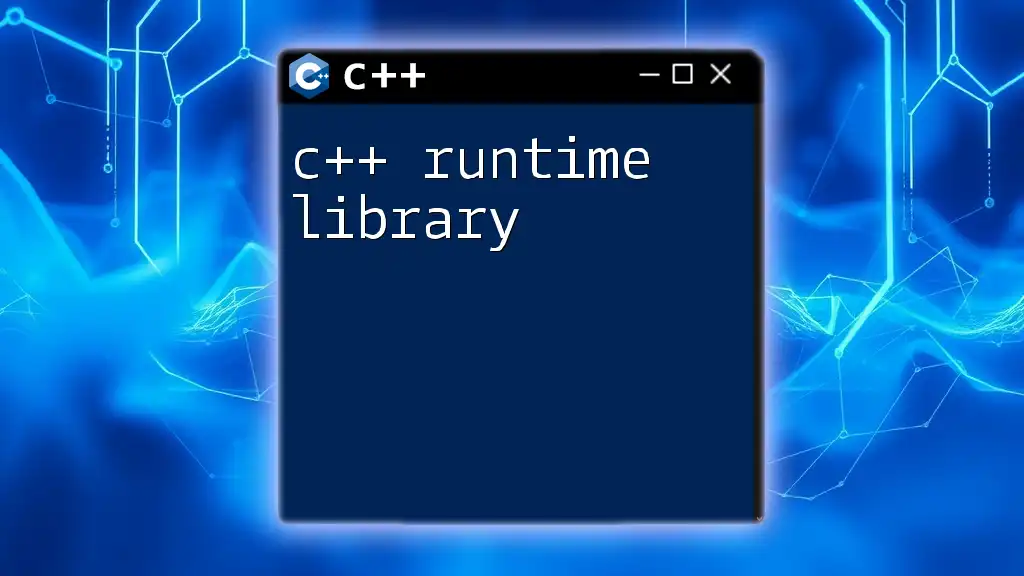
Components of C++ Runtime
Runtime Libraries
Runtime libraries are a collection of routines that a C++ program can utilize during its execution. They provide essential functions that simplify programming tasks, such as input/output operations, memory management, and data structures.
Some commonly used libraries in C++ include:
- `libstdc++`: Primarily used with GCC (GNU Compiler Collection).
- `libc++`: Trend toward modern C++ features, often used with LLVM/Clang.
Here's a simple example showing how to use the Standard Library's `vector` class to manage a dynamic array:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
for (int num : vec) {
std::cout << num << " ";
}
return 0;
}
In this example, `vector` is part of the C++ Standard Library, which the runtime makes available to manage data more efficiently.
Memory Management
One of the critical aspects of C++ runtime is memory management. The runtime environment handles both the allocation and deallocation of memory as the program executes.
C++ offers two types of memory allocation: stack and heap. Stack memory is automatically managed, meaning an object goes out of scope when it is no longer needed, whereas heap memory is dynamically allocated and requires manual management.
For instance, consider the following code snippet demonstrating dynamic memory allocation:
int* arr = new int[10]; // Allocation of an array of 10 integers
delete[] arr; // Deallocation of the previously allocated memory
Here, the use of `new` allocates memory on the heap, while `delete` frees that memory. This explicit management is essential to avoid memory leaks during runtime.
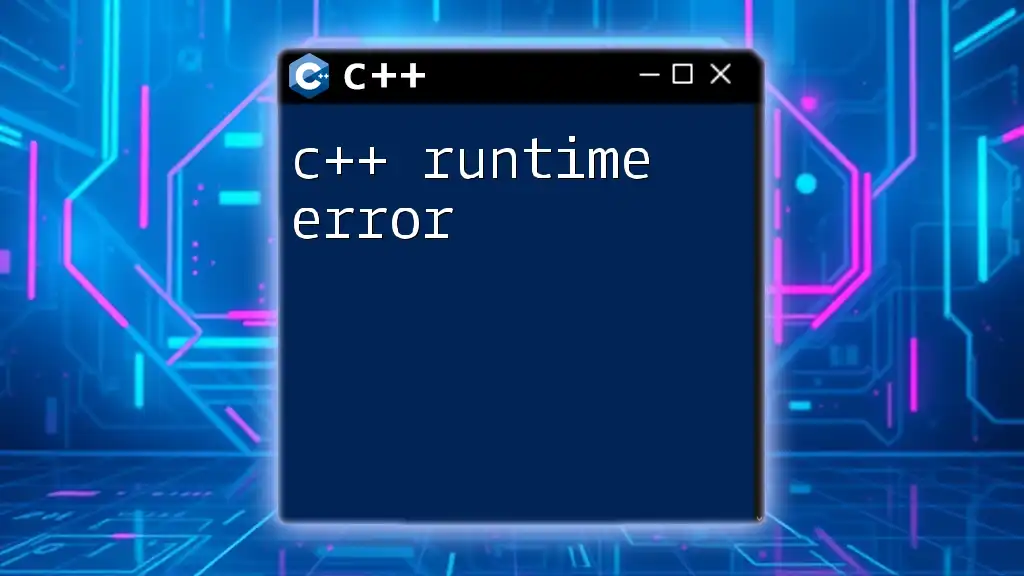
Key Concepts in C++ Runtime
Object Lifetime and Scope
The concept of object lifetime refers to the duration an object remains in existence during program execution. It's crucial to understand how objects behave between stack and heap allocation.
Objects created in stack memory are automatically destroyed when they go out of scope. Conversely, objects in heap memory remain until they are explicitly deleted.
For example:
void createObject() {
MyClass obj; // object allocated on the stack
} // 'obj' is destroyed here
void createDynamicObject() {
MyClass* obj = new MyClass(); // object allocated on the heap
delete obj; // explicit destruction needed
}
Understanding these distinctions can help you avoid runtime errors and memory leaks.
Exception Handling during Runtime
C++ offers robust exception handling mechanisms to gracefully deal with errors occurring during runtime. Using `try`, `catch`, and `throw`, developers can anticipate potential failures in their applications.
Here’s how you might handle exceptions in C++:
try {
throw std::runtime_error("Error occurred!");
} catch (const std::runtime_error& e) {
std::cerr << e.what() << std::endl; // Output the error message
}
In this code, if an error occurs within the `try` block, control is transferred to the corresponding `catch` block, allowing for a clean way to address runtime exceptions.
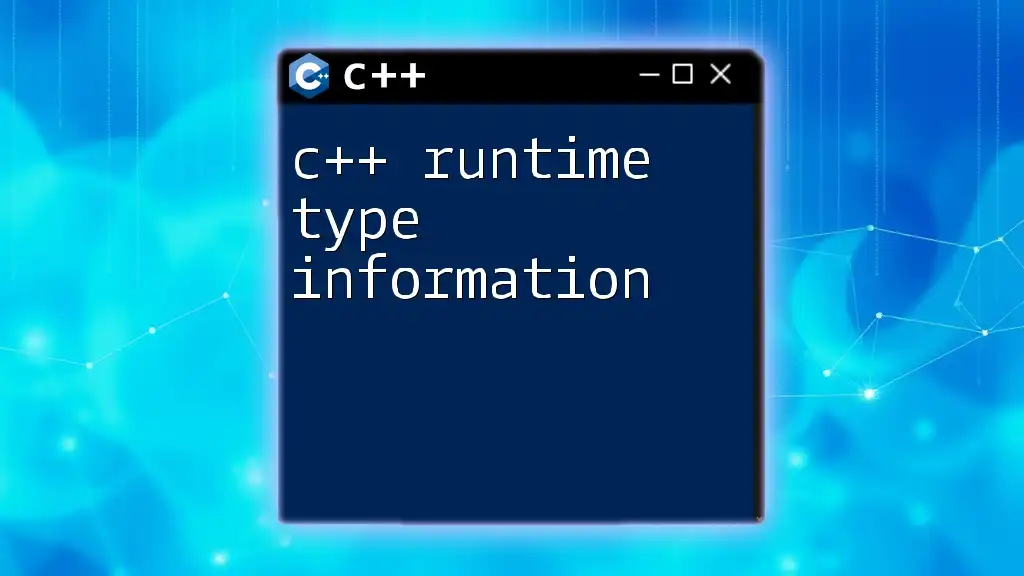
C++ Runtime in Practice
Performance Considerations
The performance of a C++ application can be heavily influenced by how runtime elements are managed. Efficient memory usage and reduced overhead during runtime can lead to faster execution. Here are some tips to enhance runtime efficiency:
- Use stack allocation whenever possible for temporary objects.
- Minimize dynamic memory allocation within frequently called functions.
- Employ smart pointers (like `std::unique_ptr` and `std::shared_ptr`) to automate memory management.
Debugging and Profiling
Debugging is another essential aspect of managing C++ runtime. Utilizing tools designed for profiling can help identify bottlenecks and inefficiencies. Some widely-used profilers in the C++ ecosystem include `gprof`, `valgrind`, and Visual Studio’s built-in tools.
For instance, to profile an application using `gprof`, you would compile your program with the `-pg` flag:
g++ -pg my_program.cpp -o my_program
This command enables profiling, and upon executing the program, a `gmon.out` file will be generated for further analysis.
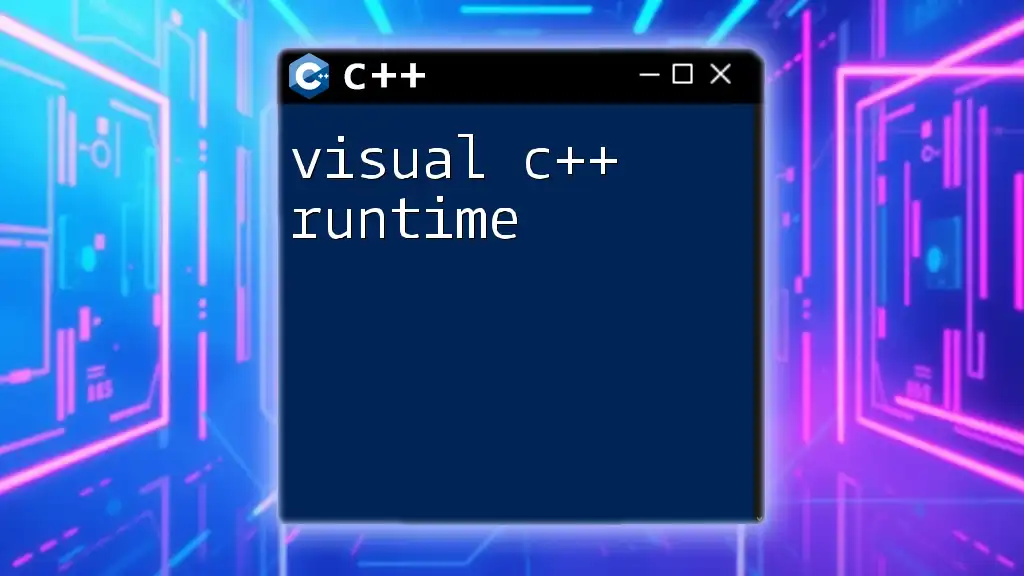
Real-World Applications of C++ Runtime
Game Development
C++ is a prevalent language in game development due to its performance efficiency and direct interaction with hardware. The C++ runtime environment is critical in game engines like Unreal Engine and Unity, where real-time processing and memory management are vital.
System Software
C++ also plays a significant role in developing system software like operating systems, device drivers, and real-time systems. The C++ runtime is instrumental in providing robust performance and resource management necessary in these applications.
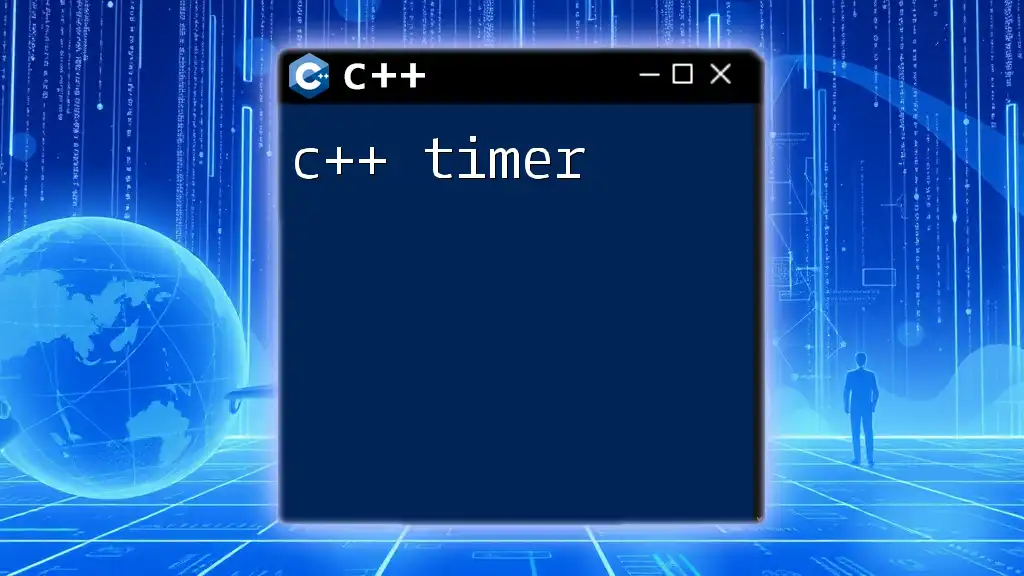
Conclusion
Understanding C++ runtime is crucial for enhancing programming skills and building efficient software. By mastering memory management, exception handling, and performance optimization, developers can create robust applications. For those looking to delve further into the world of C++, exploring comprehensive resources can provide more insights and practical knowledge. Engaging with community forums, documentation, and specialized courses is also highly recommended to enhance your understanding of C++ commands and runtime intricacies.