In C++, the `time(0)` function from the `<ctime>` library returns the current time in seconds since the Unix epoch (January 1, 1970), which can be used for various time-related calculations.
Here’s a simple code snippet demonstrating its usage:
#include <iostream>
#include <ctime>
int main() {
time_t currentTime = time(0);
std::cout << "Current time in seconds since the Unix epoch: " << currentTime << std::endl;
return 0;
}
Overview of C++ Time Handling
Understanding Time in Programming
Time plays a crucial role in almost every application, from managing events to performance measurement. In programming, understanding time is essential for creating efficient and effective software. It is not just about knowing the current time, but also how to manipulate time-related data to meet specific needs.
Real-world examples of time applications include logging events, scheduling tasks, and measuring the duration of operations. Without a solid grasp of time handling, applications could malfunction, resulting in inefficiencies or errors.
Why C++ Time 0 Matters
The concept of "Time 0" signifies a reference point in time used for calculations. In programming contexts, this often equates to the lowest possible point in time—an essential concept for ensuring accurate time measurement and event scheduling.
Understanding C++ Time 0 is vital for applications that require precise timing and scheduling. For instance, if a system resets, its operations often reference "Time 0" to measure how much time has elapsed since that point.
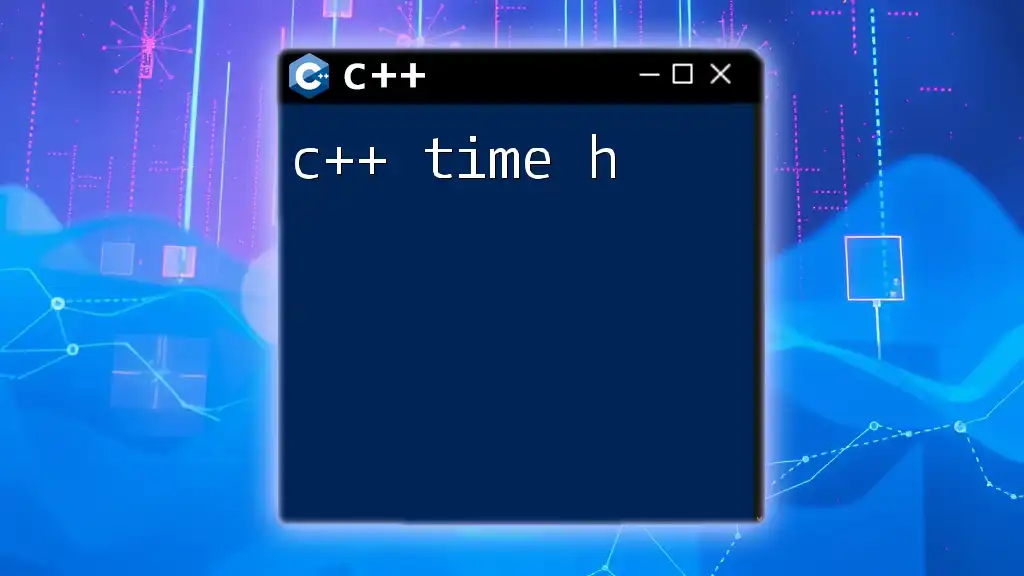
The C++ Standard Library for Time Management
Introduction to `<chrono>` Library
The `<chrono>` library in C++ provides a powerful system to perform time-related operations. It comprises three key components: Durations, Time Points, and Clocks. The library allows programmers to measure elapsed time, create delays, and manage time intervals—providing a high level of precision and control.
Basic Types of Time Representations
Time Points
Time points represent a moment in time relative to a clock. They can be obtained using various clock types found in the `<chrono>` library.
Here’s an example of obtaining the current time:
#include <chrono>
using namespace std::chrono;
auto now = steady_clock::now();
In this snippet, `steady_clock::now()` gives the current time that remains constant throughout the program's execution.
Durations
Durations represent time intervals, allowing developers to specify time lengths in various units such as seconds, milliseconds, and microseconds.
For instance, to define a duration of 500 milliseconds, you can use the following code:
auto duration = milliseconds(500); // 500 milliseconds
Understanding how to work with durations is fundamental when dealing with timing and scheduling in applications.
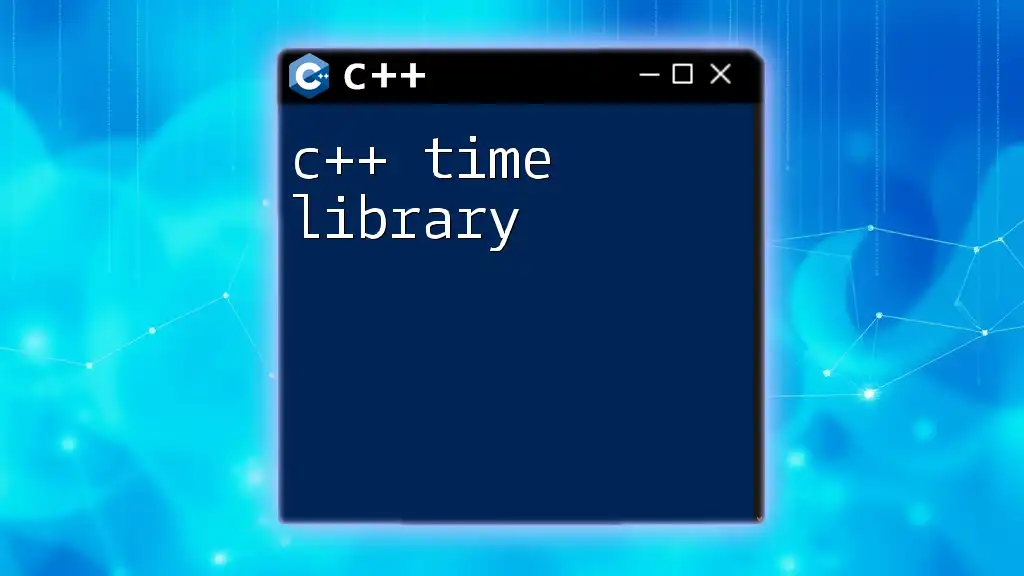
Working with Time 0 in C++
Setting Time to Zero
Setting a time or duration to zero is essential for establishing a clear baseline in programming. In C++, this can be easily accomplished by either initializing variables with zero values or using functions designed for that purpose.
You can achieve this by using the `duration::zero()` method:
#include <chrono>
#include <iostream>
int main() {
auto zeroDuration = std::chrono::duration<double>::zero();
std::cout << "Zero Duration: " << zeroDuration.count() << " seconds\n";
return 0;
}
In the above code snippet, `std::chrono::duration<double>::zero()` initializes a zero-duration, which can be critical in many timing operations.
Time Calculations Involving Time 0
Adding and Subtracting Time
Using Time 0 as a base reference allows for precise calculations when adding or subtracting time intervals. For example, if you want to add a duration of one second to a Time 0 duration, you can do so like this:
auto oneSecond = seconds(1);
auto newTime = zeroDuration + oneSecond;
std::cout << "New Duration: " << newTime.count() << " seconds\n";
Here, we create a new duration which effectively shifts the base reference from Time 0 by one second.
Comparing Time Points
Time comparisons become straightforward when you regard Time 0 as your reference point. This comparison can help in conditions where you need to determine if a certain operation occurs after a baseline time.
For instance, you can compare a current time with Time 0 like this:
if (now > steady_clock::time_point::min()) {
std::cout << "Current time is past Time 0.\n";
}
This statement checks whether the current time exceeds the minimum time point defined by the clock.
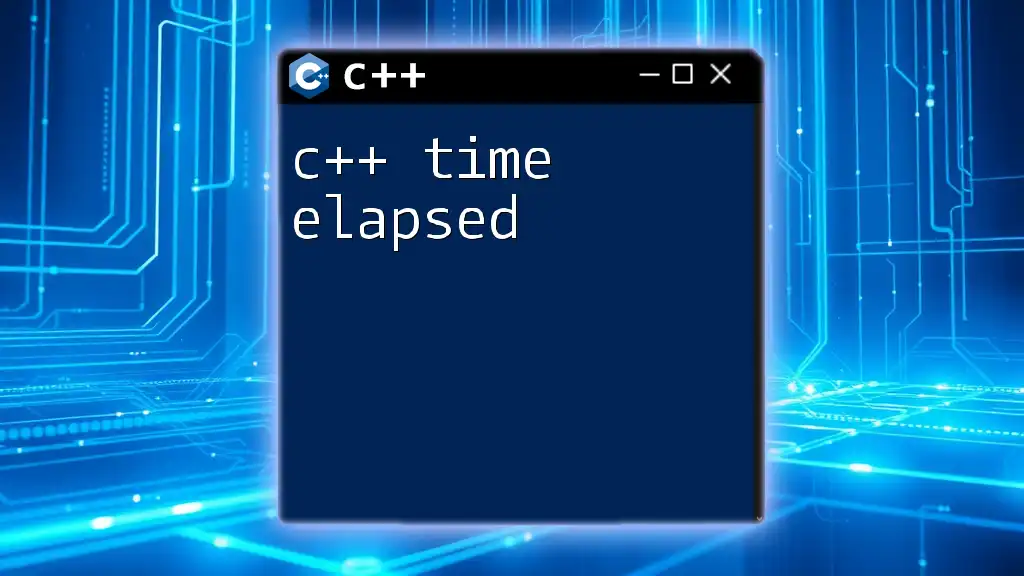
Practical Applications of Time 0
Event Scheduling
Time 0 is particularly useful in event scheduling where actions depend on a precise timing framework. By using Time 0 as a reference, developers can schedule tasks to trigger at defined intervals.
Here’s a simplified example:
auto scheduledTime = steady_clock::now() + seconds(10); // Schedule for 10 seconds later
if (steady_clock::now() >= scheduledTime) {
std::cout << "Executing scheduled task.\n";
}
This code snippet schedules an event to execute after 10 seconds, demonstrating how Time 0 can serve as a reliable base for scheduling.
Performance Measurement
Performance measurement is another vital application of Time 0. By evaluating the time taken to execute code blocks, developers can optimize their applications.
Consider the following example, which measures how long it takes for a function to execute:
auto start = steady_clock::now();
// Function to measure
auto end = steady_clock::now();
auto duration = duration_cast<milliseconds>(end - start);
std::cout << "Execution Time: " << duration.count() << " ms\n";
In this scenario, you measure the execution time of a function by capturing the time before and after its execution. The elapsed time gives developers valuable insights into performance.
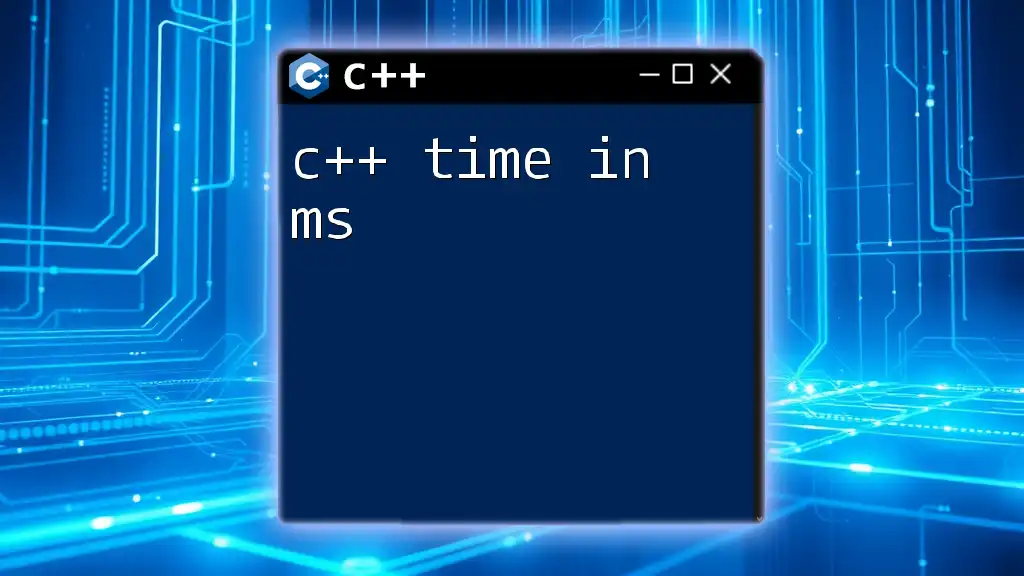
Common Pitfalls and Best Practices
Common Mistakes with Time 0
While working with time, some common mistakes arise, particularly in the initialization of durations and time points. Often, developers mistakenly assume default values represent a valid point in time, leading to unexpected behavior.
Time zone issues can also complicate time management. Always ensure that the clocks and durations used are consistent throughout your application to avoid errors.
Best Practices for Robust Time Management
To avoid pitfalls, it’s wise to adopt best practices. Employ the `<chrono>` library effectively by understanding its various components and using them appropriately. Here are some tips:
- Always initialize your time variables to a defined state, ideally to Time 0 for clarity.
- Use `duration_cast<T>()` to ensure proper type matching when performing time calculations.
- Document any assumptions about time handling in code to ensure clarity for future developers.
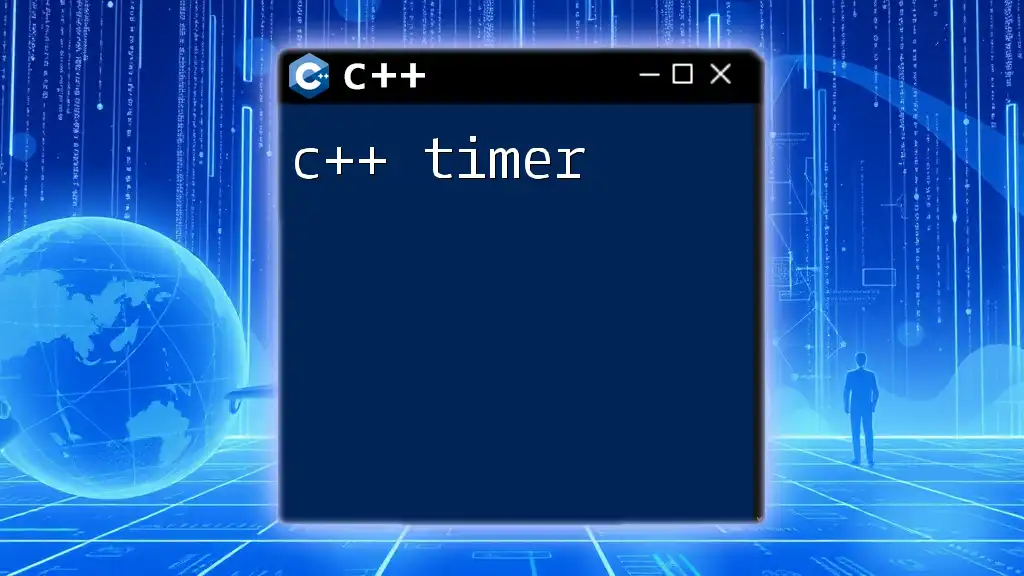
Conclusion
Recap of Key Points
In summary, understanding C++ Time 0 is crucial for accurate time management in applications. The ability to set and manipulate time points and durations gives developers the tools necessary to create efficient, reliable applications.
Further Reading and Resources
To expand your knowledge and skills in C++ time management, consider consulting advanced resources, such as recommended programming books and official documentation. Engaging with community forums and tutorials can also provide valuable insights into best practices and new techniques.
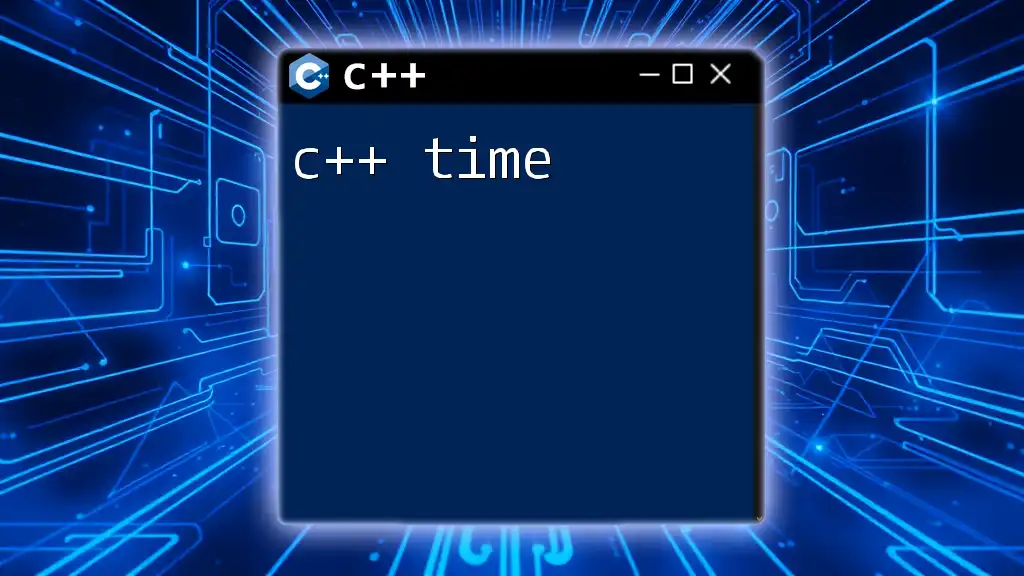
Call to Action
Now that you have a foundational understanding of C++ time management and the importance of Time 0, it’s time to apply this knowledge. Dive into your projects and experiment with time functions in C++. Join our community to share your tips and learn more about mastering C++ programming!