The C++ `<chrono>` library provides a way to work with time durations, clocks, and time points, enabling precise measurement and manipulation of time intervals. Here's a simple example demonstrating how to measure the execution time of a code block:
#include <iostream>
#include <chrono>
int main() {
auto start = std::chrono::high_resolution_clock::now();
// Code block to measure
for (volatile int i = 0; i < 1000000; ++i);
auto end = std::chrono::high_resolution_clock::now();
std::chrono::duration<double> duration = end - start;
std::cout << "Execution time: " << duration.count() << " seconds" << std::endl;
return 0;
}
Understanding the C++ Time Library Components
Overview of Time Representations
The C++ time library introduces crucial concepts like time points, durations, and clocks.
- Time Point: Represents a specific point in time. A time point is often a duration since the epoch (usually January 1, 1970).
- Duration: Represents a time span; how much time has elapsed between two time points.
- Clock: Provides utilities to measure time, including different kinds of clocks, like system clock and steady clock.
Knowing these foundational concepts will allow developers to work effectively with timings in their applications.
Key Classes in the Time Library
The core components of the C++ time library are encapsulated in several key classes, each serving specific purposes.
std::chrono::duration
`std::chrono::duration` represents a time duration and offers a way to work with time intervals in a type-safe manner. It can represent various time units, such as seconds, milliseconds, microseconds, etc.
Here’s an example showcasing how to create and manipulate duration:
#include <iostream>
#include <chrono>
int main() {
std::chrono::duration<int> seconds(10); // 10 seconds
std::chrono::duration<double, std::ratio<60>> minutes = seconds / 60; // Convert seconds to minutes
std::cout << "Duration in seconds: " << seconds.count() << std::endl;
std::cout << "Duration in minutes: " << minutes.count() << std::endl;
return 0;
}
In this example, we create a duration of 10 seconds and convert it into minutes, using type-safe ratio values.
std::chrono::time_point
`std::chrono::time_point` is utilized to represent specific moments in time. This class allows you to create, manipulate, and query the time.
Here’s a code snippet demonstrating how to create a time point and access its properties:
#include <iostream>
#include <chrono>
int main() {
std::chrono::time_point<std::chrono::system_clock> now = std::chrono::system_clock::now();
std::chrono::system_clock::duration d = now.time_since_epoch();
std::cout << "Current time since epoch: " << d.count() << " nanoseconds" << std::endl;
return 0;
}
In this example, we create a time point representing the current time and print the number of nanoseconds since the epoch.
std::chrono::clock
The C++ time library provides different types of clocks, each suitable for various applications. The most common clocks are:
- System Clock: Measures the current time, which can be affected by system time adjustments.
- Steady Clock: Guarantees that the time never goes backward, making it suitable for measuring intervals.
- High-Resolution Clock: Offers the highest precision available on the system.
Here’s how you can measure time intervals using clocks:
#include <iostream>
#include <chrono>
#include <thread>
int main() {
auto start = std::chrono::high_resolution_clock::now();
std::this_thread::sleep_for(std::chrono::seconds(1)); // Simulate work
auto end = std::chrono::high_resolution_clock::now();
auto duration = std::chrono::duration_cast<std::chrono::milliseconds>(end - start);
std::cout << "Execution time: " << duration.count() << " milliseconds" << std::endl;
return 0;
}
In this code, we measure the time taken for a simulated 1-second delay and display the execution time in milliseconds.
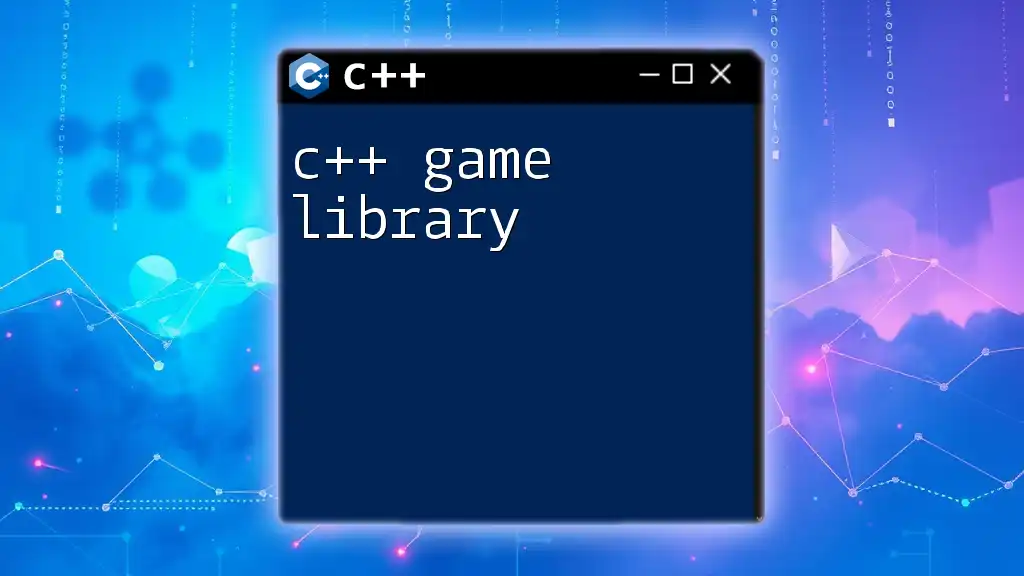
Practical Uses of the C++ Time Library
Measuring Execution Time
In performance-critical applications, measuring execution time is crucial. The C++ time library allows you to capture the duration of code execution accurately.
Here’s an example that measures the time taken by a function:
#include <iostream>
#include <chrono>
void performTask() {
std::this_thread::sleep_for(std::chrono::seconds(2)); // Simulate work
}
int main() {
auto start = std::chrono::high_resolution_clock::now();
performTask(); // Function whose execution time is to be measured
auto end = std::chrono::high_resolution_clock::now();
auto duration = std::chrono::duration_cast<std::chrono::seconds>(end - start);
std::cout << "Task took: " << duration.count() << " seconds" << std::endl;
return 0;
}
This snippet illustrates how developers can use the C++ time library to track the duration of code execution effectively.
Time Manipulation and Formatting
Adding and Subtracting Durations
Manipulating time points by adding or subtracting durations is a common requirement. Here’s how to do that:
#include <iostream>
#include <chrono>
int main() {
auto now = std::chrono::system_clock::now();
auto future = now + std::chrono::hours(2); // Add 2 hours
auto future_time = std::chrono::system_clock::to_time_t(future);
std::cout << "Future time (2 hours later): " << std::ctime(&future_time);
return 0;
}
This code adds 2 hours to the current time and prints the resulting future time.
Formatting Time as Strings
While the C++ time library doesn’t directly provide formatting utilities, you can combine it with the `<iomanip>` library to format time into a human-readable format.
Here's an example:
#include <iostream>
#include <chrono>
#include <iomanip>
#include <ctime>
int main() {
auto now = std::chrono::system_clock::now();
std::time_t now_time = std::chrono::system_clock::to_time_t(now);
std::cout << "Current date and time: " << std::ctime(&now_time);
return 0;
}
In this snippet, we convert the current time point into a standard time format and print it.
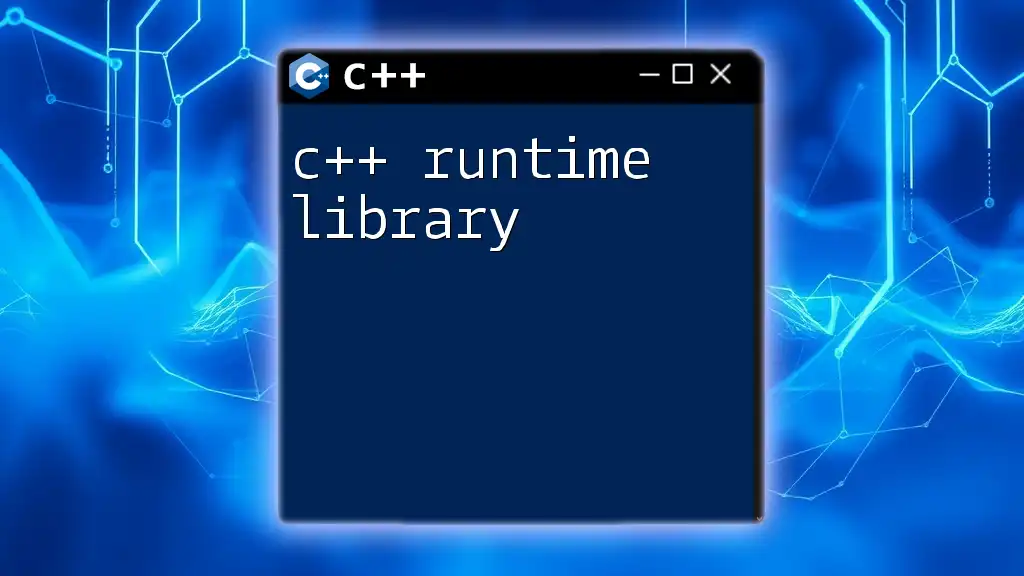
Advanced Features of the Time Library
Handling Time Zones
Handling time zones is essential for applications that cross geographical boundaries. While the C++ time library does not directly manage time zones, you may rely on third-party libraries, such as date.h by Howard Hinnant or `tz` library, to complement your time functionalities.
Working with Clock Precision
Understanding clock precision is vital when doing performance measurements. The C++ time library enables developers to choose between different clocks:
#include <iostream>
#include <chrono>
int main() {
auto start = std::chrono::steady_clock::now();
// Some processing...
auto end = std::chrono::steady_clock::now();
auto elapsed = std::chrono::duration_cast<std::chrono::microseconds>(end - start).count();
std::cout << "Elapsed time: " << elapsed << " microseconds" << std::endl;
return 0;
}
In this code, we utilize the steady clock for precise interval measurements, ensuring accurate results.
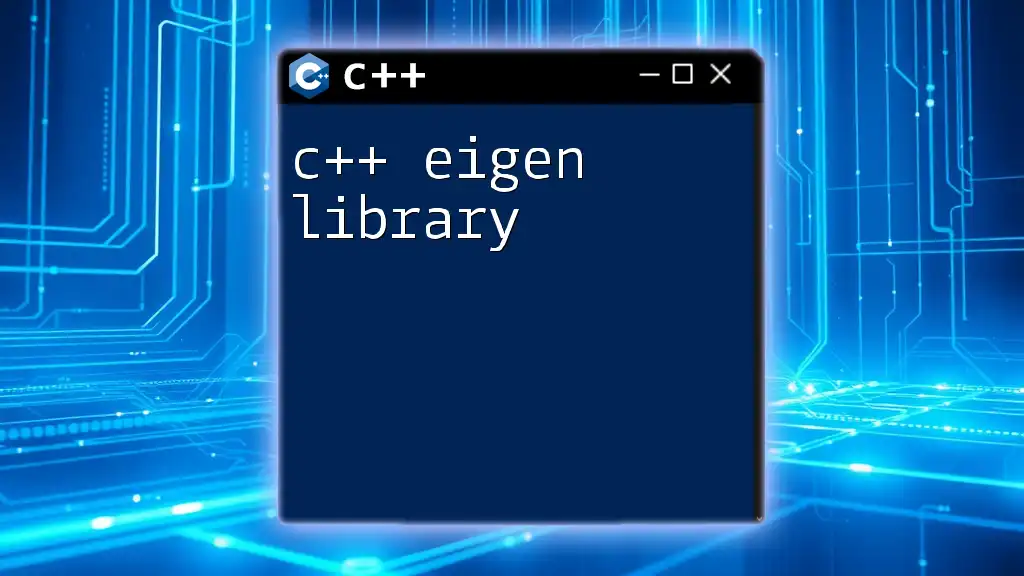
Common Pitfalls and Best Practices
Common Misconceptions
A common issue when working with the C++ time library is equating different clock types. It’s vital to recognize that while the system clock can be affected by manual time adjustments, the steady clock is designed not to.
Performance Considerations
Using the library efficiently can ensure smooth performance in applications. For instance, measure execution time before and after a block of code to determine if optimizations are necessary. Avoid frequent calls to the clock in tight loops if precision isn't a requirement—this can slow down performance. Here’s a code comparison:
auto start = std::chrono::high_resolution_clock::now();
// Intensive computation here
auto end = std::chrono::high_resolution_clock::now();
This approach yields a performance benchmark without losing accuracy.
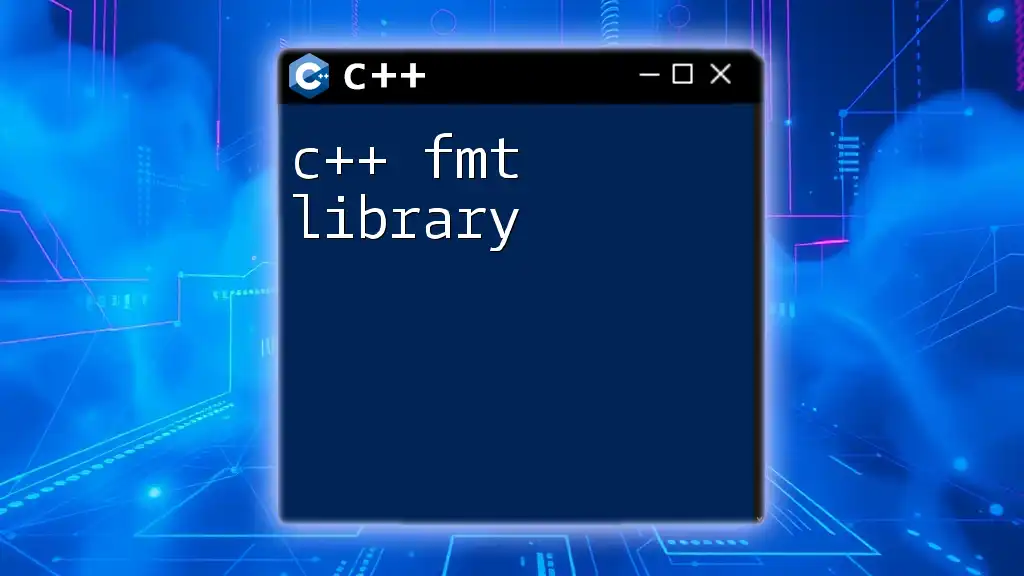
Conclusion
The C++ time library is a powerful toolset for managing time and duration in a program. By understanding its various components and functionalities, you can measure performance, manipulate time effectively, and produce user-friendly outputs. The importance of experimenting and practicing these techniques cannot be overstated—enhancing your proficiency with the C++ time library will undoubtedly elevate your programming skills.
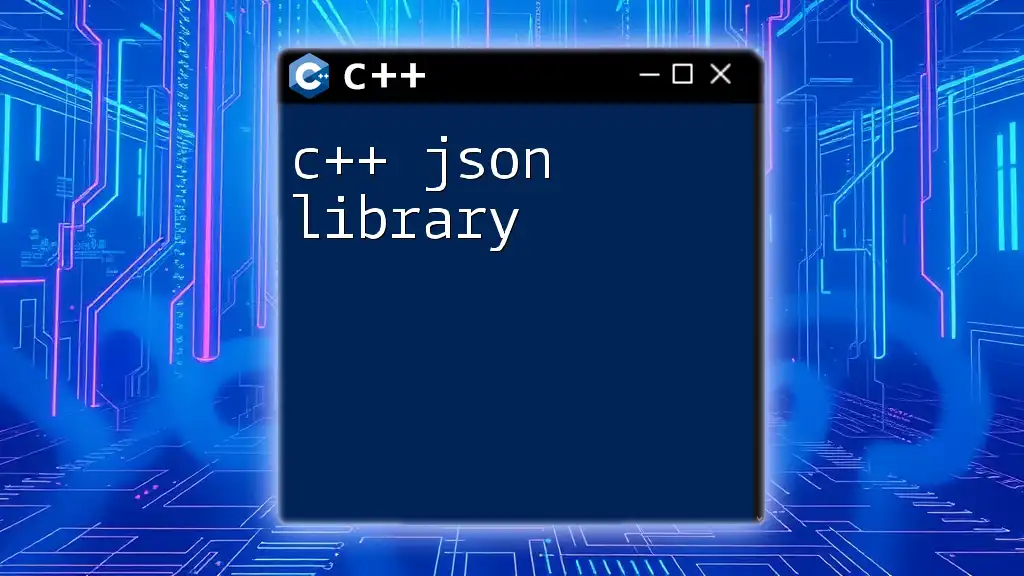
Additional Resources
For those eager to dive deeper into the world of C++, consider exploring comprehensive documentation and community resources. Some excellent references include the official C++ standard documentation, effective C++ books, and online courses that offer structured learning paths. Engaging with developer communities can also provide valuable insights and answers to your questions.