A C++ timer can be implemented using the `<chrono>` library to measure the duration of code execution efficiently. Here's an example code snippet to illustrate this:
#include <iostream>
#include <chrono>
int main() {
auto start = std::chrono::high_resolution_clock::now();
// Your code to be timed here
for (volatile int i = 0; i < 1000000; ++i);
auto end = std::chrono::high_resolution_clock::now();
std::chrono::duration<double> duration = end - start;
std::cout << "Execution time: " << duration.count() << " seconds\n";
return 0;
}
Understanding Timing Mechanisms in C++
A C++ timer is essential for measuring the passage of time in your applications. It allows you to record elapsed time, manage execution duration, and create dynamic functionalities based on time intervals. Understanding the timing mechanisms available in C++ is crucial for leveraging these capabilities effectively.
In C++, time measurement can be achieved through different methods. Depending on your project's requirements, you might choose between high-resolution timers, which provide very precise measurements, and standard timers for simpler time recording. High-resolution timers are especially useful in applications such as gaming or scientific computing, where millisecond-level accuracy is necessary.
The C++ Standard Library and Timing
The C++ Standard Library provides robust support for timing through the `<chrono>` library. This library is designed to simplify timer implementation and offers various tools for working with time-based tasks. With `<chrono>`, you can measure intervals, timestamps, and run timers effectively.
The three main components of `<chrono>` are:
- Duration: Represents a time interval.
- Time Point: Represents a specific point in time.
- Clock: Offers the current time point using various clock types.
Using `<chrono>`, you can create precise timers without much hassle, which is a significant advantage over manual timing techniques.
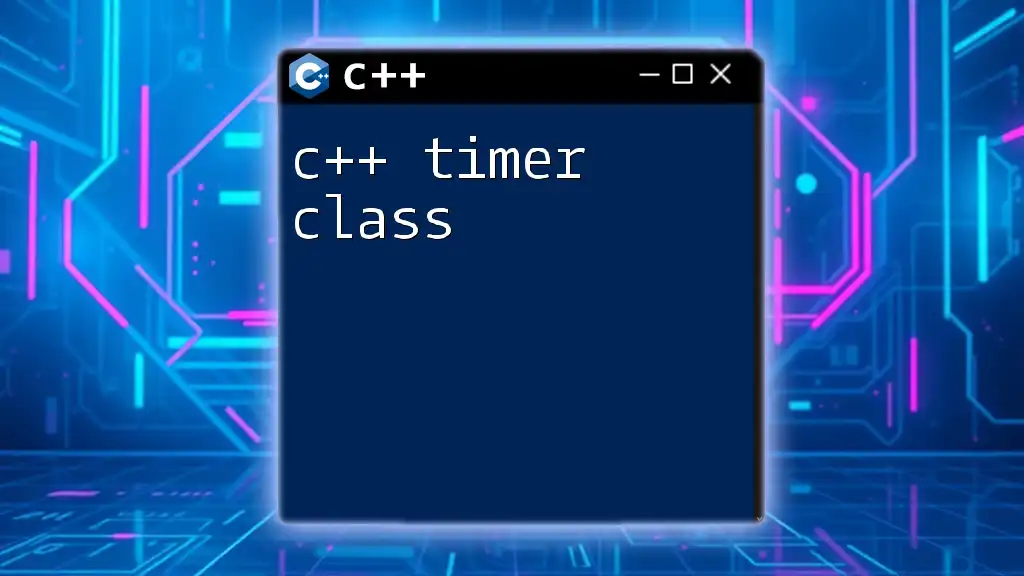
Implementing a Basic Timer in C++
To get started with a C++ timer, you can create a simple timer function using the features of `std::chrono`. Below is a demonstration of how to measure the time taken by a task.
#include <iostream>
#include <chrono>
void simple_timer() {
auto start = std::chrono::high_resolution_clock::now();
// Simulated workload
for (int i = 0; i < 1e7; ++i);
auto end = std::chrono::high_resolution_clock::now();
std::chrono::duration<double> elapsed = end - start;
std::cout << "Elapsed time: " << elapsed.count() << " seconds." << std::endl;
}
Explanation of the Code Snippet
- Start Measurement: The timer begins by capturing the current time using `std::chrono::high_resolution_clock::now()`.
- Simulated Workload: Here, we are simply executing a loop that performs a trivial task to simulate a workload.
- End Measurement: Once the workload is complete, the end time is recorded.
- Calculate Elapsed Time: We then calculate the elapsed time by subtracting the start time from the end time, and the result is printed.
This simple timer implementation is effective for profiling small sections of code and provides you with basic insight into performance characteristics.
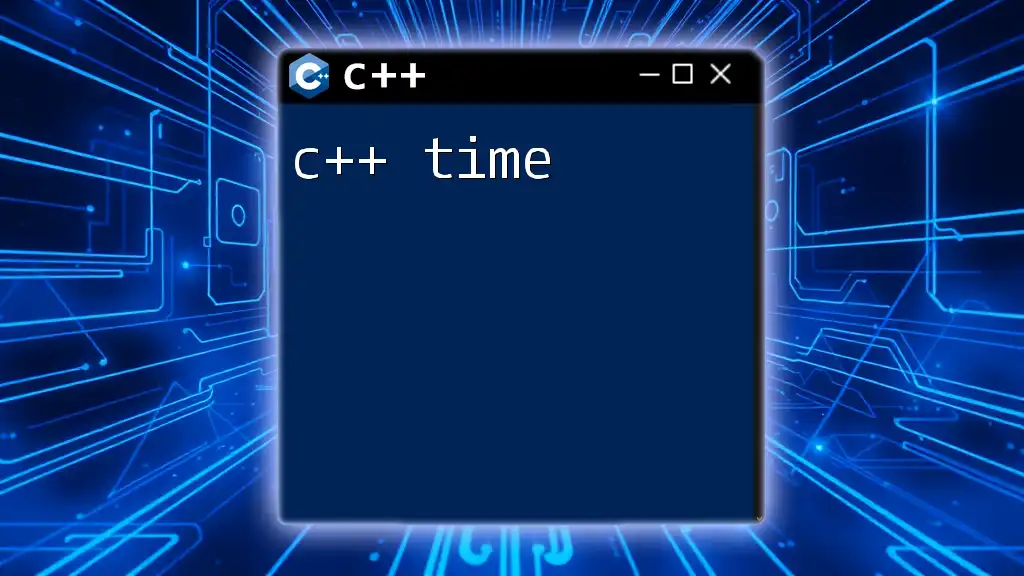
Advanced Timer Functionality
Creating Repeating Timers with Looping
In many applications, you may want to create a timer that runs periodically. A repeating timer can be achieved easily with a loop. Below is an example:
#include <iostream>
#include <chrono>
#include <thread>
void repeating_timer(int duration_sec) {
for (int i = 0; i < 10; ++i) {
auto start = std::chrono::high_resolution_clock::now();
// Simulated workload
for (int j = 0; j < 1e6; ++j);
auto end = std::chrono::high_resolution_clock::now();
std::chrono::duration<double> elapsed = end - start;
std::cout << "Elapsed time: " << elapsed.count() << " seconds. Iteration: " << i + 1 << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(duration_sec));
}
}
Explanation of the Code Snippet
- Loop through iterations: The function allows the user to specify the duration of the wait (in seconds). It performs a series of iterations.
- Measure Each Iteration: For each iteration, the elapsed time is measured like before, followed by a pause (using `std::this_thread::sleep_for`) to wait for the specified duration.
- Output: It outputs the elapsed time along with the iteration number.
Using Timers for Performance Measurement
A C++ timer is not only useful for regular applications but plays a vital role in performance measurement. Understanding how long various sections of code take to execute can help you identify bottlenecks and optimize your code accordingly. By wrapping critical parts of your code with timers, you gain insights into which operations are time-consuming and can refactor those for better performance.
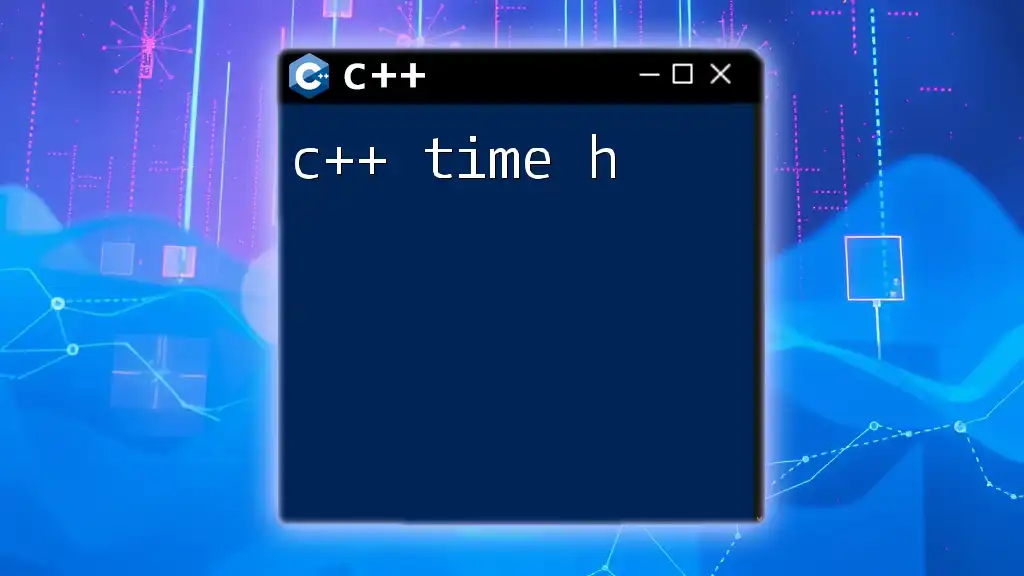
Timer Classes in C++
Building a Custom Timer Class
Creating a custom timer class can encapsulate timing functionalities and enhance code readability. Here’s a simple implementation:
#include <chrono>
#include <iostream>
class Timer {
public:
Timer() : start_time_point(std::chrono::high_resolution_clock::now()) {}
void reset() {
start_time_point = std::chrono::high_resolution_clock::now();
}
double elapsed() const {
auto end_time_point = std::chrono::high_resolution_clock::now();
return std::chrono::duration<double>(end_time_point - start_time_point).count();
}
private:
std::chrono::high_resolution_clock::time_point start_time_point;
};
Explanation and Usage of the Timer Class
- Initialization: The constructor captures the current time upon the creation of a Timer object.
- Reset Function: The `reset()` function allows users to reset the timer back to the current time.
- Elapsed Time Calculation: The `elapsed()` function calculates and returns the total time since the timer was created or last reset.
Using a timer class streamlines the process of timing various operations and can be reused across different parts of your application, promoting clean coding practices.
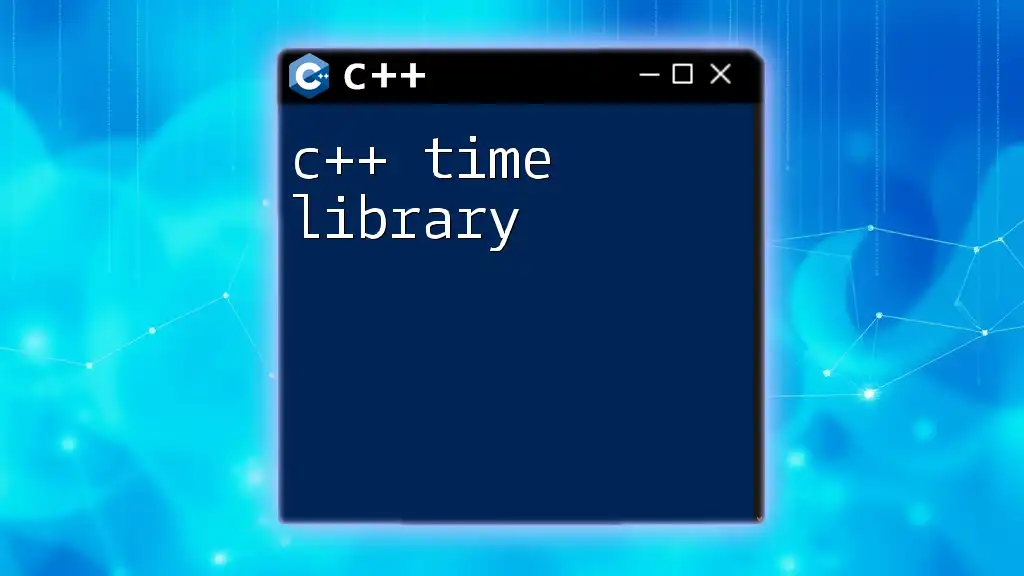
Practical Examples of Timer Use Cases
Game Development
In game development, timers can manage time-based events such as animations, cooldowns for actions, or creating delays in gameplay. For example, you can use a timer to determine how long a player has to wait before they can perform another action.
Real-Time Systems
In real-time systems, such as regulatory control systems, a timer can monitor the execution time of critical tasks to ensure they meet strict timing constraints. For instance, you might use a timer to control the frequency of sensor readings or updates to the user interface.
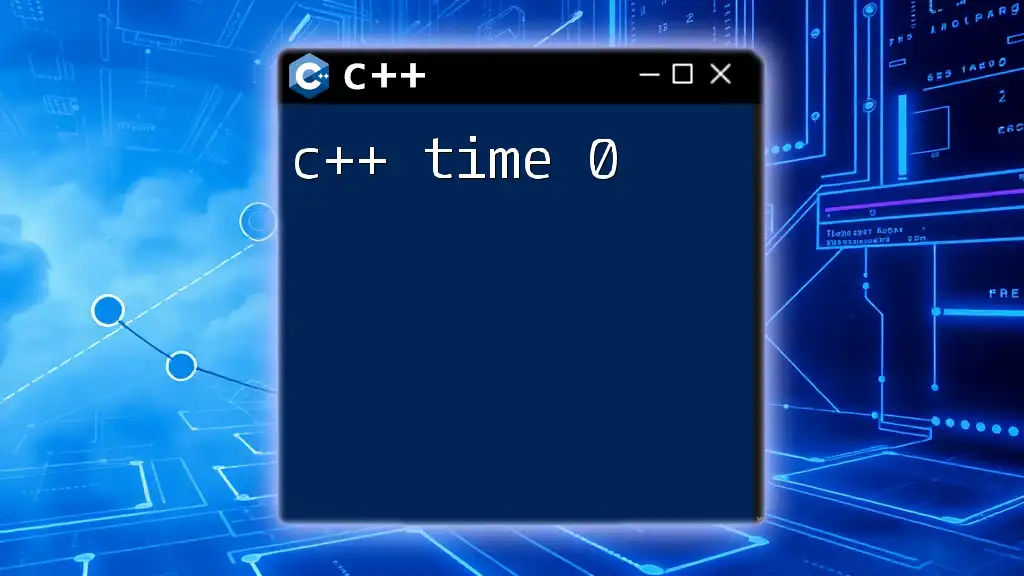
Best Practices for Using Timers in C++
- Use High-Resolution Timers: Whenever precise timing is required, opt for high-resolution clocks provided by `<chrono>`.
- Avoid Excessive Output: When measuring performance, too much console output can skew your timing results. Limit output to necessary information.
- Profile Code in Different Scenarios: Timing results can vary based on CPU load, so profile in different conditions to get meaningful insights.
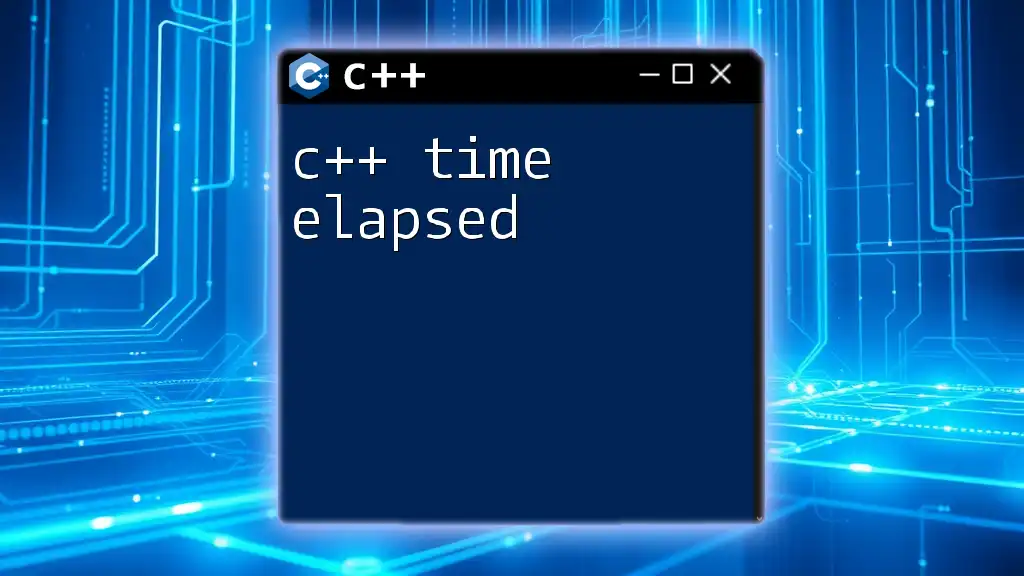
Conclusion
Timers are a powerful tool in C++ programming, providing developers with significant capabilities to measure performance and execute time-sensitive tasks. By utilizing the features offered by `<chrono>`, you can manage time effectively in various applications.
Exploring C++ timers opens doors to optimizing your code, improving responsiveness, and enhancing user experience. With careful application, a C++ timer can play a significant role in elevating your programming projects.
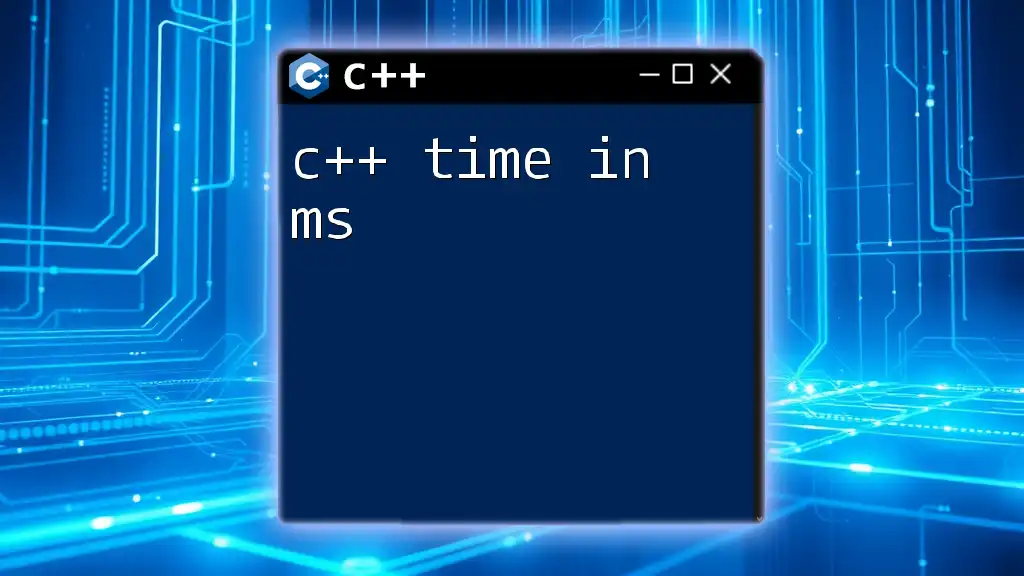
Additional Resources
For further exploration on timers in C++, consider the following resources:
- Books on C++ programming and the Standard Library
- Online tutorials focused on performance measurement and optimization
- Additional articles that cover related topics in-depth