The `std::numeric_limits` template in C++ provides a standardized way to access the properties of fundamental data types, such as their minimum and maximum values.
Here's a code snippet that demonstrates how to use `std::numeric_limits` to find the minimum and maximum values of an `int` type:
#include <iostream>
#include <limits>
int main() {
std::cout << "The minimum value of int: " << std::numeric_limits<int>::min() << std::endl;
std::cout << "The maximum value of int: " << std::numeric_limits<int>::max() << std::endl;
return 0;
}
Understanding the Basics of `numeric_limits`
What are Numeric Limits?
In C++, `numeric_limits` is a template class included in the `<limits>` header that provides a standardized way to access information about numerical types. It allows programmers to determine the properties and characteristics of the various numerical types available in the language, enabling safer and more effective coding practices. The numeric types include both integral types, such as `int` and `long`, and floating-point types, including `float` and `double`.
The `<limits>` Header
To use `numeric_limits`, you need to include the `<limits>` header file within your program. This file provides the framework needed to work with the numeric properties without the need to implement custom logic repeatedly. Including this header is essential as it establishes the foundation for any numeric operations that require defining limits.
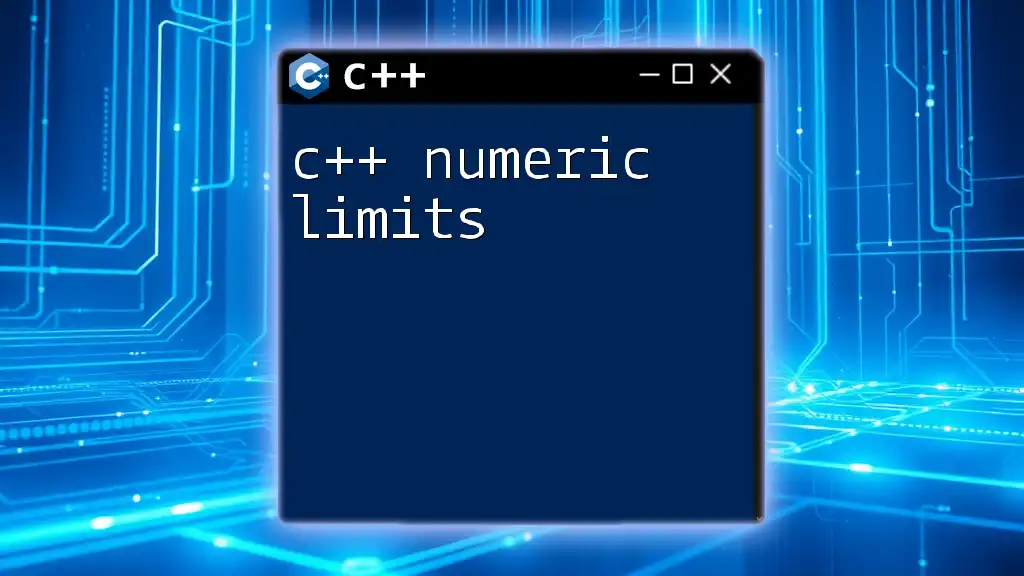
Properties of Numeric Types
Overview of Common Numeric Types
C++ provides various numeric types, mainly categorized into two groups:
- Integral Types: These types include `int`, `short`, `long`, and their unsigned variants, which represent whole numbers without fractions.
- Floating-Point Types: This group encompasses `float`, `double`, and `long double`, which represent numbers with fractions, offering a broader range of values through decimal points.
Key Properties Obtained from `numeric_limits`
C++ allows developers to extract various properties of numeric types, which can be vital for effective programming. Here are some key properties provided by `numeric_limits`:
- Minimum and Maximum Values: `numeric_limits` provides the minimum and maximum values for a given numeric type, which helps prevent overflow and underflow in mathematical operations. This can be demonstrated with the following code snippet:
#include <iostream>
#include <limits>
int main() {
std::cout << "Max int: " << std::numeric_limits<int>::max() << std::endl;
std::cout << "Min int: " << std::numeric_limits<int>::min() << std::endl;
return 0;
}
- Precision: For floating-point types, precision is a crucial metric that helps maintain the integrity of calculations. The number of digits that can be represented is expressed through `digits10`. Here’s how you can retrieve the precision:
#include <iostream>
#include <limits>
int main() {
std::cout << "Precision of double: " << std::numeric_limits<double>::digits10 << std::endl;
return 0;
}
- Is Signed / Is Integer: Using `numeric_limits`, one can easily check whether a specific type is signed or integer. This is important for understanding how values are stored and how they may behave during operations:
#include <iostream>
#include <limits>
int main() {
std::cout << "Is int signed? " << std::numeric_limits<int>::is_signed << std::endl;
return 0;
}
Special Values
In addition to characteristics of numeric types, `numeric_limits` provides access to special values, such as positive and negative infinity, as well as `NaN` (Not a Number). These special values can be crucial, especially when dealing with calculations involving division by zero or undefined values. Accessing these values can be done as follows:
#include <iostream>
#include <limits>
int main() {
std::cout << "Positive infinity for double: " << std::numeric_limits<double>::infinity() << std::endl;
std::cout << "NaN for double: " << std::numeric_limits<double>::quiet_NaN() << std::endl;
return 0;
}
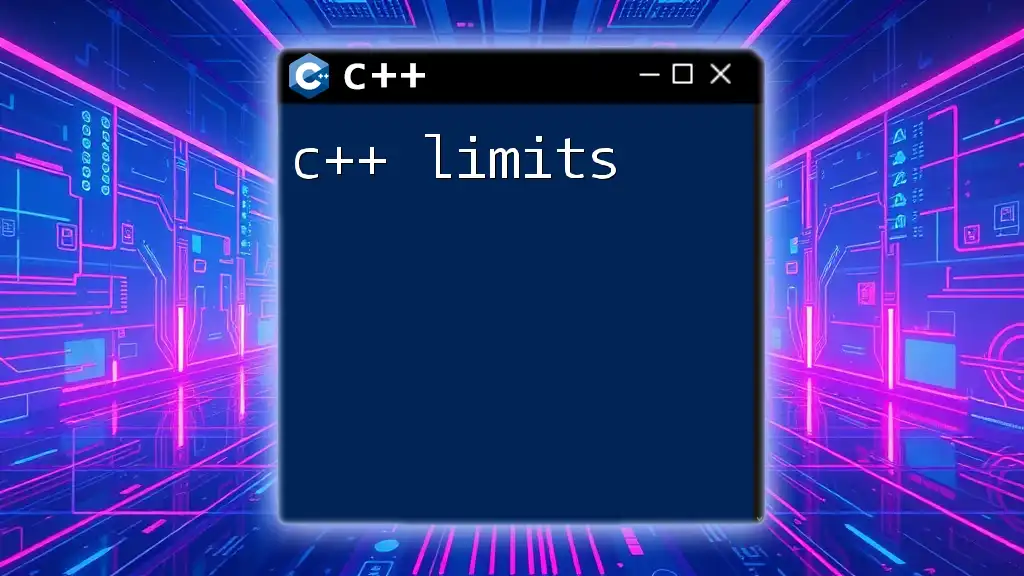
Practical Uses of `numeric_limits`
Handling Edge Cases
One of the most important roles of `numeric_limits` is in the careful handling of edge cases in a program. It provides pre-defined constants that can be used to avoid overflow or underflow during arithmetic operations. For instance, before performing an addition operation where the result might exceed the maximum value, you could check if the operation is safe:
#include <iostream>
#include <limits>
int main() {
int a = std::numeric_limits<int>::max();
int b = 1;
if (a + b > std::numeric_limits<int>::max()) {
std::cout << "Overflow will occur!" << std::endl;
} else {
std::cout << "Result: " << a + b << std::endl;
}
return 0;
}
Type Safety and Generic Programming
`numeric_limits` also plays a fundamental role in C++ template programming, allowing for type safety across numerous types. When templates are utilized, knowing the limits can ensure that the code remains robust, regardless of the data type being used. The following example illustrates how `numeric_limits` can be employed in a template function:
#include <iostream>
#include <limits>
template <typename T>
void printLimits() {
std::cout << "Min: " << std::numeric_limits<T>::min()
<< ", Max: " << std::numeric_limits<T>::max() << std::endl;
}
int main() {
printLimits<int>();
printLimits<double>();
return 0;
}
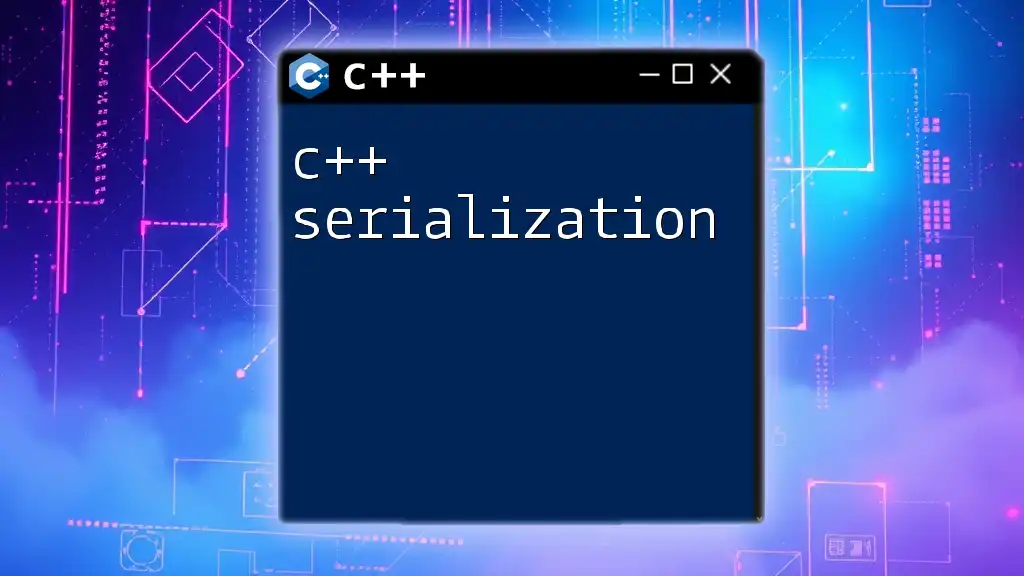
Performance Considerations
Efficiency of Using `numeric_limits`
Using `numeric_limits` efficiently can enhance the performance of a C++ application. It centralizes the logic to access limits and properties, preventing the need for repetitive calculations. This can lead to cleaner and more maintainable code.
When to Avoid Frequent Checks
Even though `numeric_limits` provides essential properties, it is crucial to avoid excessive checks in performance-critical code. It's often best to store results in variables when the limits will be accessed multiple times within a loop or similar construct. This prevents redundant calls and improves performance.
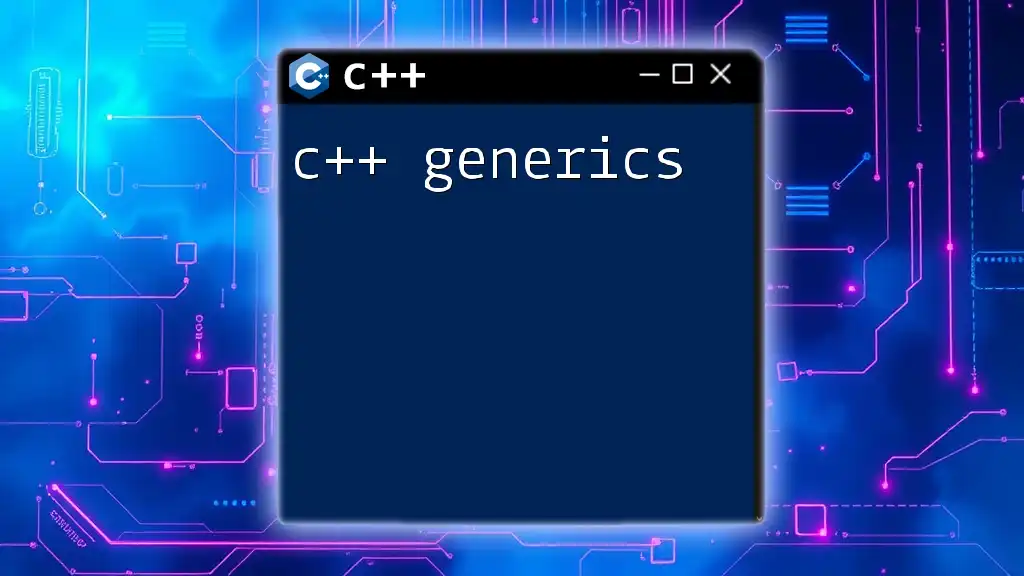
Summary
In conclusion, understanding and utilizing C++ `numeric_limits` is vital for any C++ developer. It not only helps in grasping the fundamental properties of numeric types but also ensures that mathematical operations are performed safely and meaningfully. By incorporating `numeric_limits` into coding practices, one can write efficient, safe, and robust C++ applications that adhere to best practices.
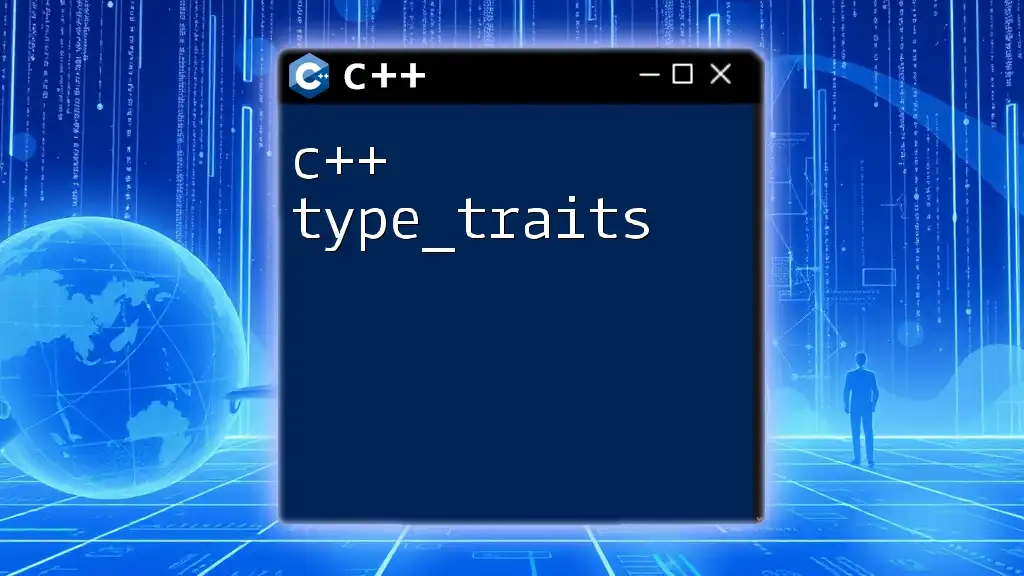
Further Resources
Books and Online References
To gain a deeper insight into C++ and its numeric limits, consider exploring noteworthy texts such as “The C++ Programming Language” by Bjarne Stroustrup and various online resources available on official documentation and trusted programming sites.
Community and Forums
Engaging with communities such as Stack Overflow, Reddit's r/cpp, and other C++ forums can facilitate discussions that can further enrich your understanding of `numeric_limits` and its application in real-world projects. Joining these platforms is an excellent way to connect with other programmers, share knowledge, and solve C++-related challenges together.
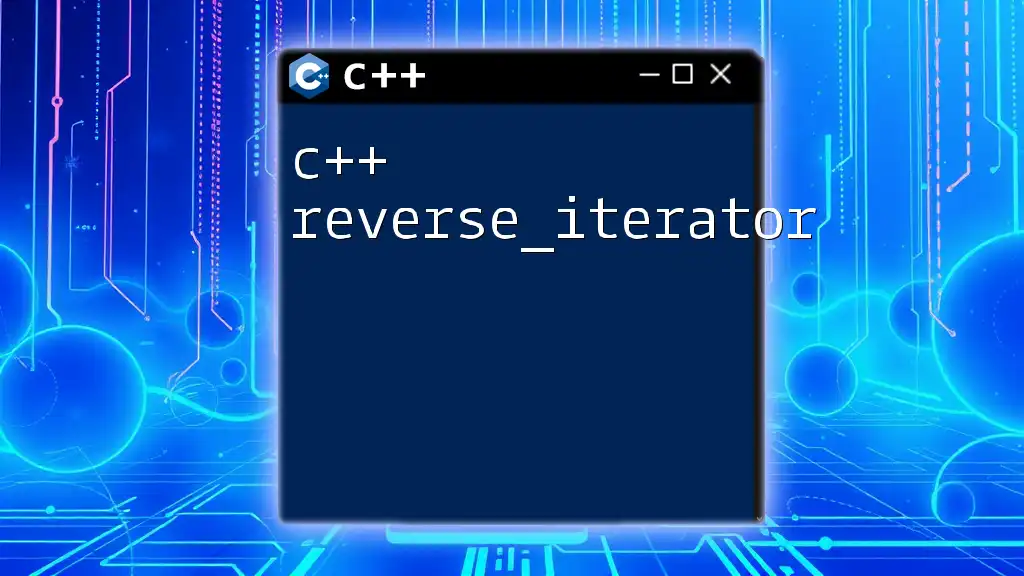
Conclusion
Embracing `numeric_limits` within your C++ programming toolkit opens doors to more efficient, precise, and error-free coding. It empowers developers to handle numerical data confidently, reinforcing the importance of understanding the intrinsic properties of the data they work with. Dive into `numeric_limits` and explore its features to maximize your effectiveness as a C++ developer!