C++ `type_traits` is a header that provides template classes and functions which allow you to query or modify the properties of types at compile time, enabling safer and more efficient generic programming.
#include <iostream>
#include <type_traits>
template <typename T>
void checkType() {
if (std::is_integral<T>::value) {
std::cout << "T is an integral type." << std::endl;
} else {
std::cout << "T is not an integral type." << std::endl;
}
}
int main() {
checkType<int>(); // T is an integral type.
checkType<double>(); // T is not an integral type.
return 0;
}
What are Type Traits?
Definition
In C++, type traits are template structures that provide information about types at compile time. This information can be used for type manipulation, allowing developers to write more robust and type-safe code. By leveraging type traits, programmers can create more generic and reusable code components.
History
Type traits emerged in the C++ Standard Library beginning with C++98, but they underwent significant advancements with the introduction of C++11. The updated features and enhancements give developers the tools to implement more sophisticated type manipulations, paving the way for modern template programming.
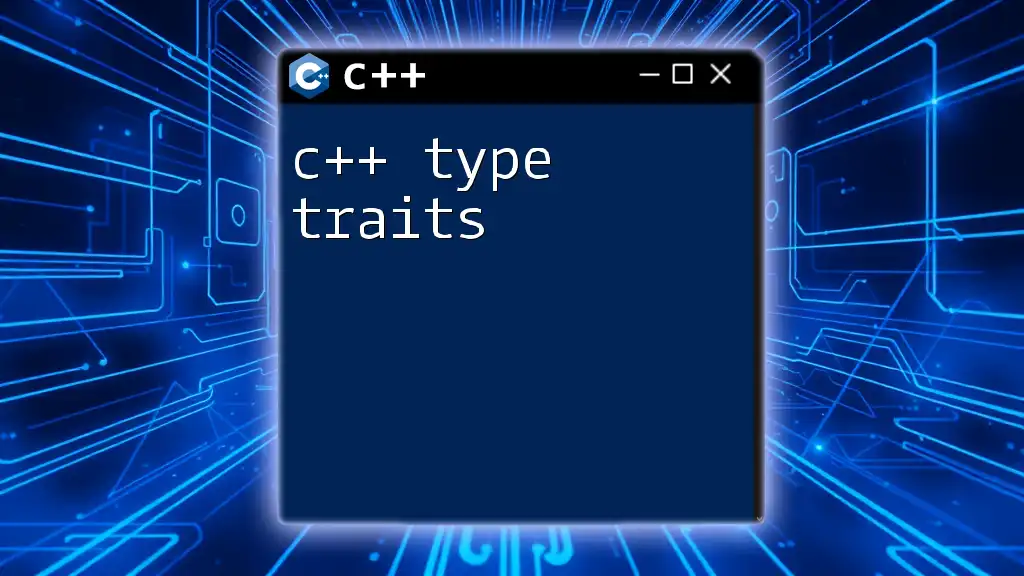
The `<type_traits>` Header
Overview
To utilize type traits, you need to include the `<type_traits>` header. This essential header file encapsulates various type traits that help determine certain properties about types, allowing for complex type detection and manipulation tasks that are designed to enhance code portability and safety.
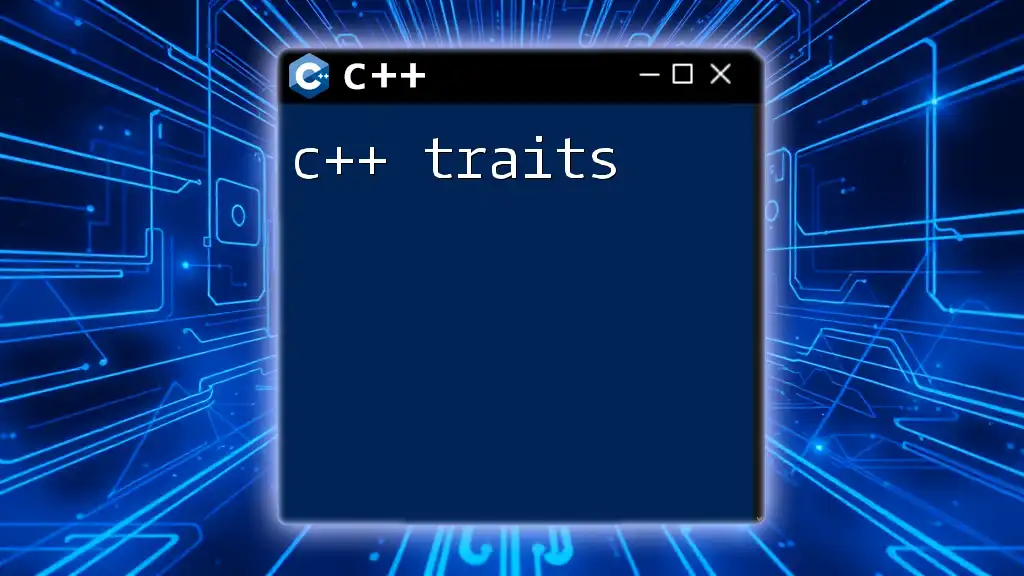
Fundamental Type Traits
is_void
The `is_void` trait checks if a type is `void`. This is particularly useful when creating templates or generic functions that should behave differently based on whether a type is void or not.
Example:
#include <type_traits>
static_assert(is_void<void>::value, "void should be a void type");
static_assert(!is_void<int>::value, "int should not be a void type");
is_integral
The `is_integral` trait determines if a type is an integral type (e.g., `int`, `char`, `long`). This can be helpful in template specialization and overload resolution, allowing you to create functions that only accept integral types.
Example:
static_assert(is_integral<int>::value, "int should be an integral type");
static_assert(!is_integral<float>::value, "float should not be an integral type");
is_floating_point
Similarly, the `is_floating_point` trait verifies if a type is a floating-point type (e.g., `float`, `double`). This type trait is instrumental when you want to differentiate functions that handle floating-point numbers.
Example:
static_assert(is_floating_point<float>::value, "float should be a floating-point type");
static_assert(!is_floating_point<int>::value, "int should not be a floating-point type");
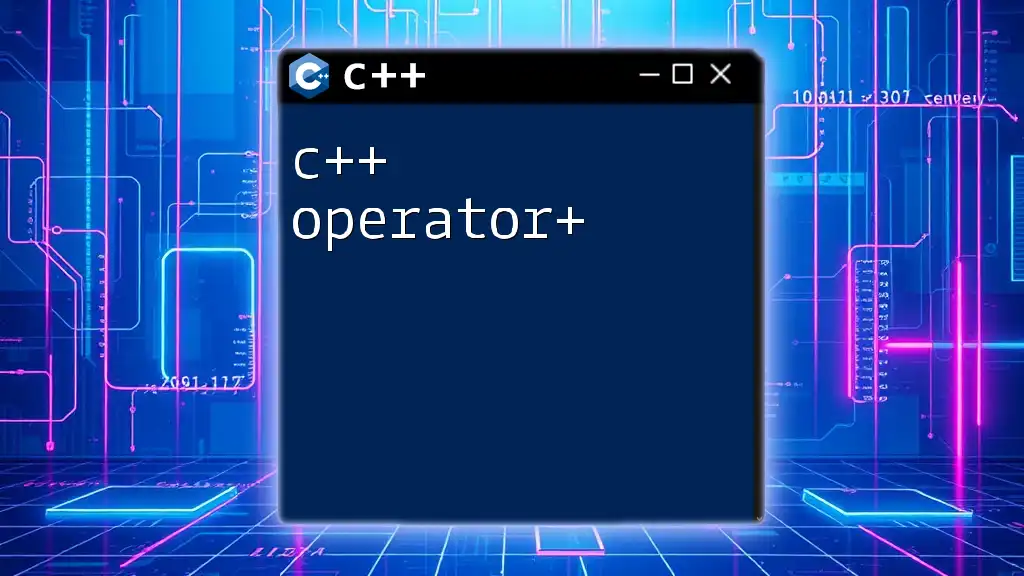
Composite Type Traits
is_same
The `is_same` trait checks if two types are identical. This can be particularly valuable in template programming, allowing for decisions based on exact type matches.
Example:
static_assert(is_same<int, int>::value, "int and int should be the same type");
static_assert(!is_same<int, float>::value, "int and float should not be the same type");
is_base_of
The `is_base_of` trait helps determine whether one type is a base class of another. This is useful in scenarios where you want to create functions that should operate only on derived classes of a specific base class.
Example:
class Base {};
class Derived : public Base {};
static_assert(is_base_of<Base, Derived>::value, "Derived should be derived from Base");
static_assert(!is_base_of<Derived, Base>::value, "Base should not be derived from Derived");
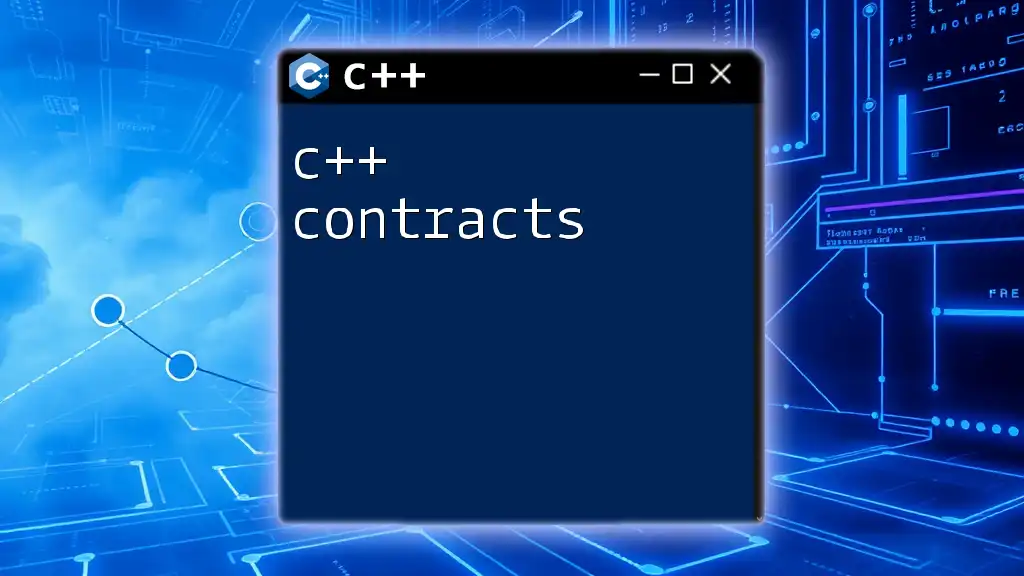
Utilizing Type Traits in Template Metaprogramming
Enabling Conditional Compilation
Type traits enable a sophisticated mechanism known as SFINAE (Substitution Failure Is Not An Error). This can be used to defer template instantiation until the exact type is known, allowing for safer and more adaptable code.
An example of utilizing `std::enable_if` to conditionally compile functions based on type traits is illustrated below:
#include <type_traits>
template<typename T>
typename std::enable_if<is_integral<T>::value, T>::type add(T a, T b) {
return a + b;
}
// This will fail to compile because 'float' is not an integral type
// template<typename T>
// typename std::enable_if<is_integral<T>::value, T>::type add(T a, T b) {
// return a + b;
// }
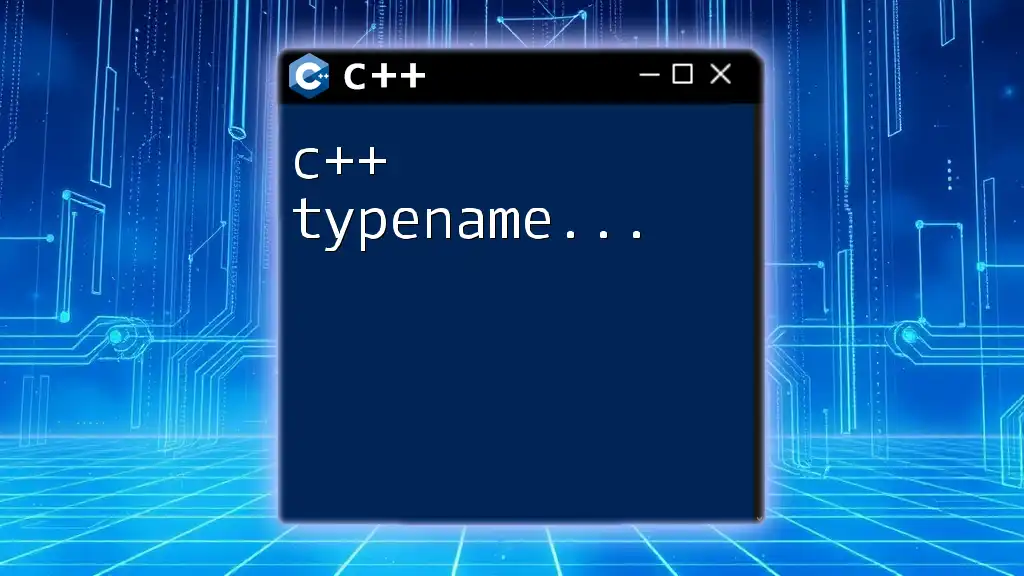
Custom Type Traits
Creating Your Own Type Traits
In addition to the numerous predefined type traits, you can also create your own custom type traits. Custom traits allow programmers to extend their functionality to meet specific needs.
Example: Here’s how you can create a simple custom type trait to check whether a given type is `Foo`.
template<typename T>
struct is_foo {
static const bool value = false;
};
class Foo {};
template<>
struct is_foo<Foo> {
static const bool value = true;
};
// Testing the custom type trait
static_assert(is_foo<Foo>::value, "Foo should be considered a Foo type");
static_assert(!is_foo<int>::value, "int should not be considered a Foo type");
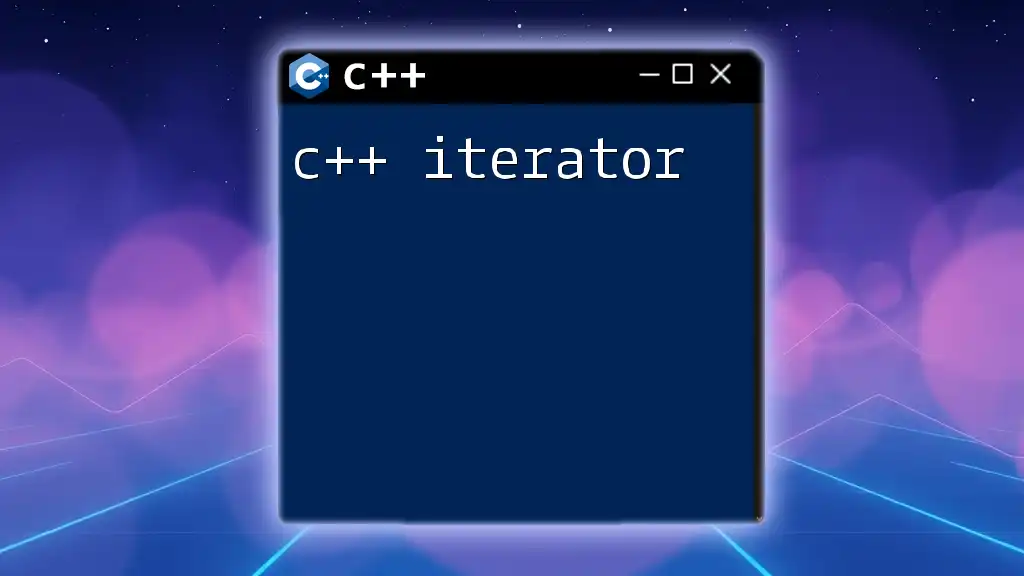
Practical Applications of Type Traits
Type Checking in Templates
Type traits significantly streamline type checking in templates. By employing these traits, you ensure that only appropriate types are processed, thus reducing runtime errors and enhancing code quality.
Case Study: Using traits for a generic algorithm can improve both performance and readability. For instance, if you have a sort function, you can enforce that it only accepts integral or floating-point types.
Optimizing Code Performance
Using type traits can aid in optimizing code performance through conditional compilation. You can use `std::conditional` to create efficient pathways through templates.
Example:
#include <type_traits>
template<typename T>
typename std::conditional<is_integral<T>::value, int, double>::type myFunction(T t) {
return t; // Different implementation based on type T
}
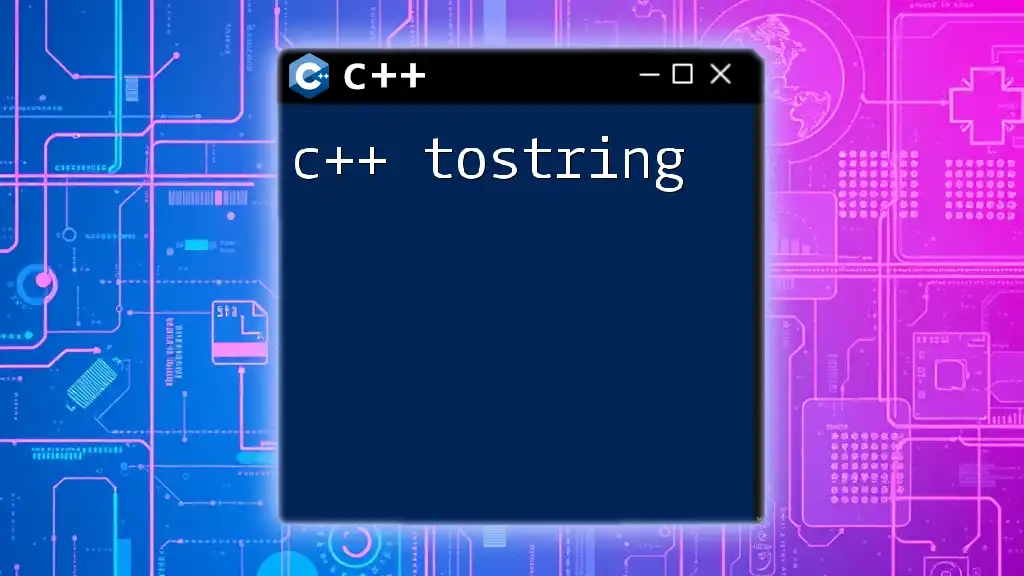
Best Practices When Using Type Traits
Keep Traits Concise
When using type traits, it is essential to maintain clarity and brevity in your trait definitions. This not only enhances code readability but also minimizes the potential for errors.
Compatibility and Forward-Compatibility
To ensure your code remains compatible across different versions of C++, it's crucial to utilize type traits judiciously. Be mindful of using traits that might not be available in older standards, as this will save future headaches when maintaining and upgrading your code.
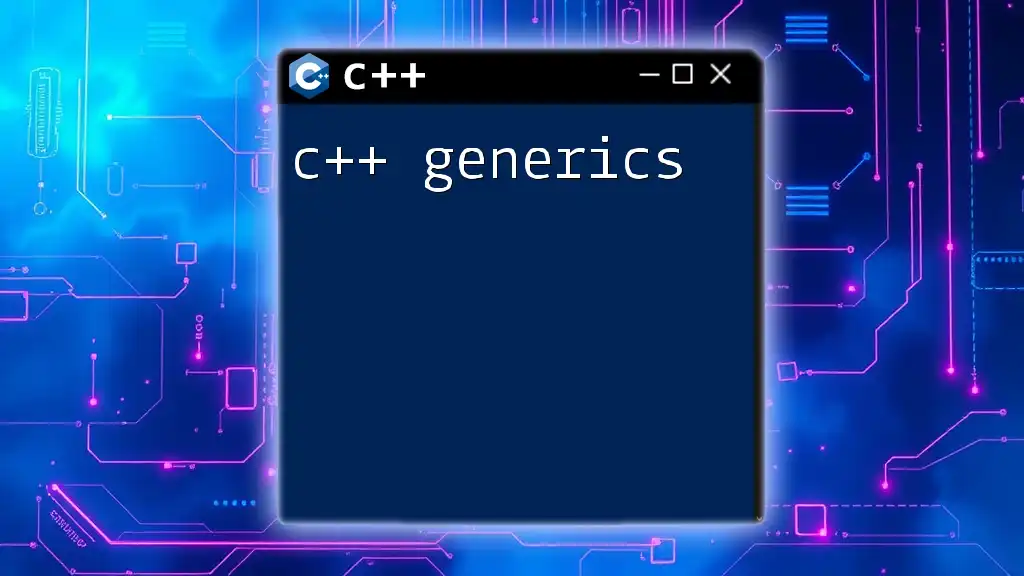
Conclusion
Understanding and applying C++ type_traits is essential for any modern C++ programmer. Through compile-time checks and type manipulation abilities, you can write more generic, safe, and efficient code. Delve deeper into the `<type_traits>` functionalities, and empower yourself to write clean, type-safe code that stands the test of time.
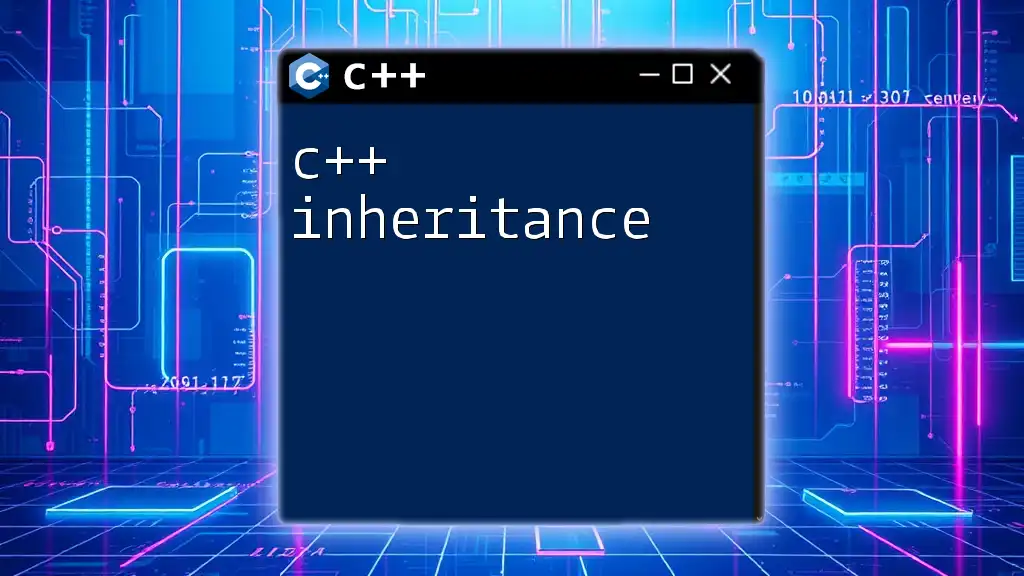
Further Reading
Explore recommended resources for mastering C++, such as books on template programming, online courses, and comprehensive tutorials that cover advanced C++ concepts.
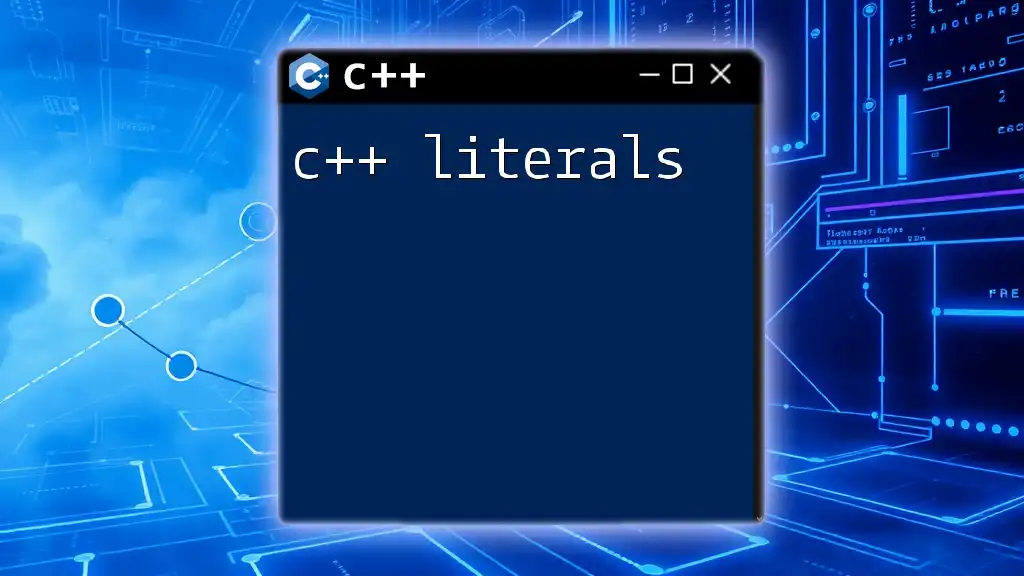
Call to Action
Start implementing type traits in your projects today to enhance type safety and code efficiency. Feel free to reach out for a course on mastering C++ and taking your programming skills to the next level!