C++ traits are templates that provide a way to query and define properties of types, allowing for more generic programming and type manipulation.
#include <iostream>
#include <type_traits>
template<typename T>
struct TypeTraits {
static constexpr bool is_pointer = std::is_pointer<T>::value;
static constexpr bool is_integral = std::is_integral<T>::value;
};
int main() {
std::cout << "Is int a pointer? " << TypeTraits<int*>::is_pointer << "\n"; // Outputs: 1
std::cout << "Is int an integral? " << TypeTraits<int>::is_integral << "\n"; // Outputs: 1
return 0;
}
Introduction to C++ Traits
C++ traits refer to a powerful concept within the C++ programming language that pertains to type traits, which provide compile-time information about types. They come into play primarily in the realm of template metaprogramming, a technique that allows developers to make the compiler perform computations based on types at compile-time instead of run-time.
Utilizing C++ traits enhances code reusability and allows for type-checking during compilation, which can reduce runtime errors and improve performance. By utilizing different types of traits, C++ developers can build more robust and flexible applications.
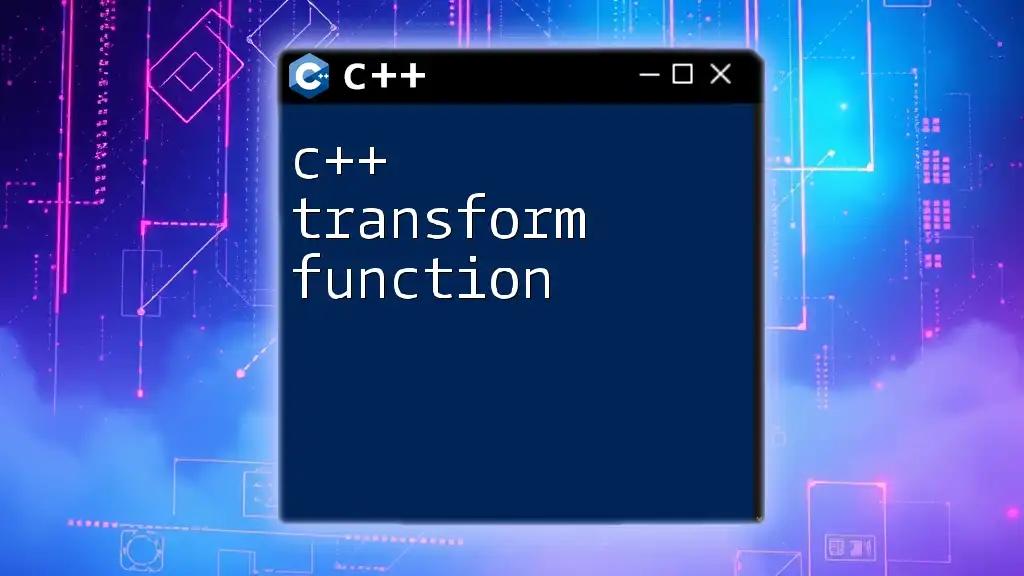
Understanding Type Traits
Type traits are essentially template structures that allow programmers to shine a light on the properties of types involved in their program. They help identify whether a type has particular characteristics or behaviors, which can be crucial for making decisions in templates.
Key Benefits of Type Traits
- Improved performance: Traits allow aspects of types to be known at compile time, which can be optimized away during code generation, leading to faster executables.
- Code clarity and maintainability: By providing a clear way to express constraints on types, traits help others understand how a code interface is expected to behave.
- Reducing template bloat: By providing better specialization techniques, we reduce the number of template instantiations, leading to smaller compiled code.

Central C++ Trait Classes
std::is_integral
The trait `std::is_integral` is an essential type trait in the C++ Standard Library that allows you to determine whether a type is an integral type (such as `int`, `char`, or `long`).
Example Usage:
#include <type_traits>
#include <iostream>
int main() {
std::cout << std::boolalpha;
std::cout << "Is int an integral type? " << std::is_integral<int>::value << std::endl; // true
std::cout << "Is float an integral type? " << std::is_integral<float>::value << std::endl; // false
return 0;
}
In this code snippet, while `int` returns `true`, `float` produces `false`, demonstrating how `std::is_integral` can facilitate type verification.
std::is_floating_point
Similarly, `std::is_floating_point` offers a way to check if a type is a floating-point type.
Illustrated Example:
#include <type_traits>
#include <iostream>
int main() {
std::cout << std::boolalpha;
std::cout << "Is double a floating point? " << std::is_floating_point<double>::value << std::endl; // true
return 0;
}
In this example, the code confirms that `double` is indeed a floating-point type.
std::is_same
The trait `std::is_same` allows for the comparison of two types to determine if they are identical.
Example:
#include <type_traits>
#include <iostream>
int main() {
std::cout << std::boolalpha;
std::cout << "Does int match with float? " << std::is_same<int, float>::value << std::endl; // false
std::cout << "Does int match with int? " << std::is_same<int, int>::value << std::endl; // true
return 0;
}
This trait is particularly useful in template specialization, allowing for more adaptable code paths based on exact type matches.
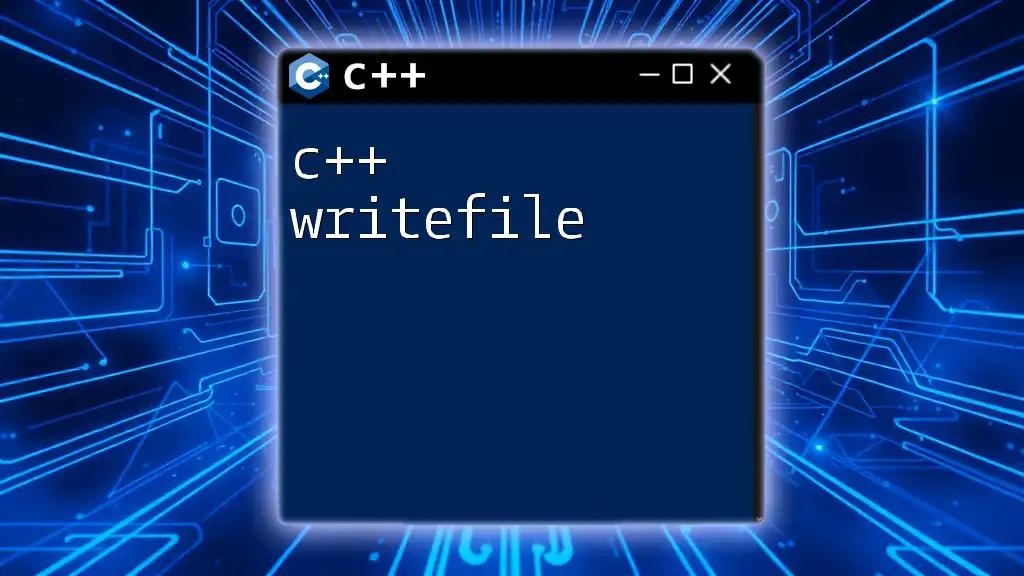
Creating Custom Traits
Introduction to Custom Traits
While the standard library provides a variety of useful traits, there are scenarios where you may need to create your own. Custom traits can help encapsulate specific type behaviors or properties that are not covered by the built-in options, enhancing flexibility in your programming.
Example of Custom Traits
Here is how you can implement a simple custom trait that checks if a type is a pointer.
#include <type_traits>
#include <iostream>
template <typename T>
struct is_pointer {
static const bool value = false; // Base case for non-pointer types
};
template <typename T>
struct is_pointer<T*> {
static const bool value = true; // Specialization for pointer types
};
int main() {
std::cout << std::boolalpha;
std::cout << "Is int* a pointer type? " << is_pointer<int*>::value << std::endl; // true
std::cout << "Is int a pointer type? " << is_pointer<int>::value << std::endl; // false
return 0;
}
In this example, the `is_pointer` trait correctly identifies pointers. The base case defaults to `false`, while the specialization provides a `true` result when evaluating pointer types.

Advanced Usage of Traits
Combining Traits with SFINAE
SFINAE, or Substitution Failure Is Not An Error, is a crucial concept that allows you to create more powerful and flexible template functions. By using SFINAE, you can selectively enable or disable function overloads based on type traits. This technique can improve the quality of your APIs.
Example Code:
#include <type_traits>
#include <iostream>
template <typename T>
typename std::enable_if<std::is_integral<T>::value, void>::type
func(T t) {
std::cout << "Function for integral types.\n";
}
template <typename T>
typename std::enable_if<!std::is_integral<T>::value, void>::type
func(T t) {
std::cout << "Function for non-integral types.\n";
}
int main() {
func(10); // Calls integral version
func(10.5); // Calls non-integral version
return 0;
}
In this example, C++ traits help determine which overload of `func` will be invoked based on whether the argument is integral or non-integral.
Type Traits in STL Algorithms
Stl algorithms extensively utilize C++ traits to ensure type safety and optimize performance. For instance, template functions like `std::sort` will typically require that the types being sorted can be compared, which is validated internally using traits.
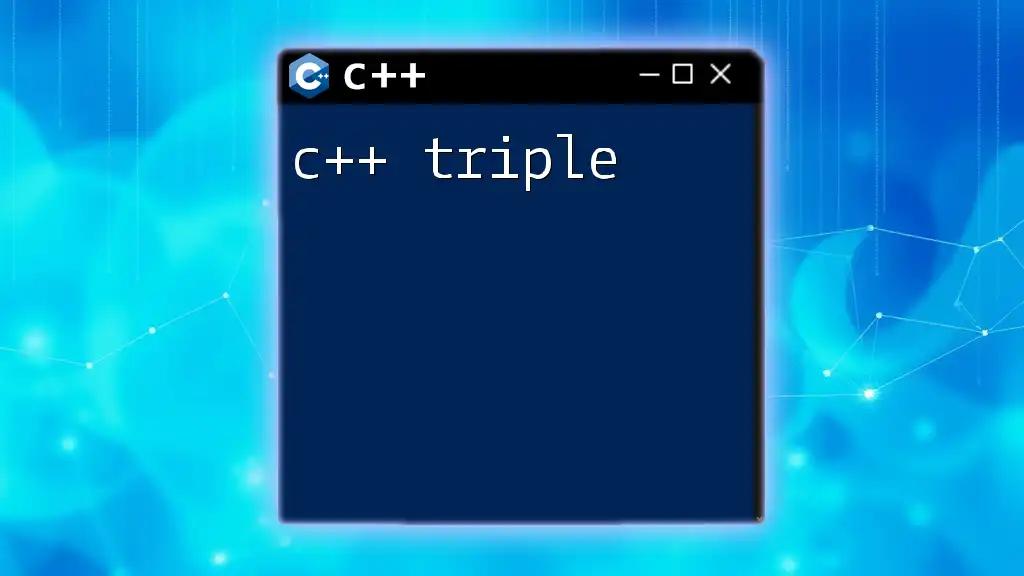
Conclusion
In summary, C++ traits serve as a powerful tool for any developer looking to leverage the capabilities of template metaprogramming. They provide crucial compile-time information about types, leading to improved code performance, clarity, and reusability. Implementing and utilizing type traits effectively can significantly enhance your C++ programming skills, leading to more maintainable and efficient code. For those looking to delve deeper into the subject, numerous resources, including books and online courses, are available for further learning and exploration.