In C++, the `this` pointer refers to the current instance of a class and is used to access class members within its member functions.
Here’s a simple example demonstrating its usage:
#include <iostream>
using namespace std;
class MyClass {
public:
int value;
MyClass(int value) {
this->value = value; // Using 'this' to distinguish class member from parameter
}
void display() {
cout << "Value: " << this->value << endl; // Accessing the member variable using 'this'
}
};
int main() {
MyClass obj(10);
obj.display();
return 0;
}
Understanding the "this" Pointer
What is the "this" Pointer?
The "this" pointer is a special type of pointer available within non-static member functions of a class in C++. It points to the object for which the member function was called and allows access to the member variables and methods of the current object. The significance of "this" becomes paramount when there is a need to distinguish between member variables and parameters, particularly when they share the same name.
Characteristics of "this"
- Type: The type of "this" pointer is a constant pointer to the class type. This means it cannot be reassigned to point to a different object.
- Initialization: The "this" pointer is automatically initialized by the compiler when a member function is called. You don't need to declare it.
- Scope: "this" can be accessed only in the context of non-static member functions; it cannot be used within static functions as there is no instance associated with static members.
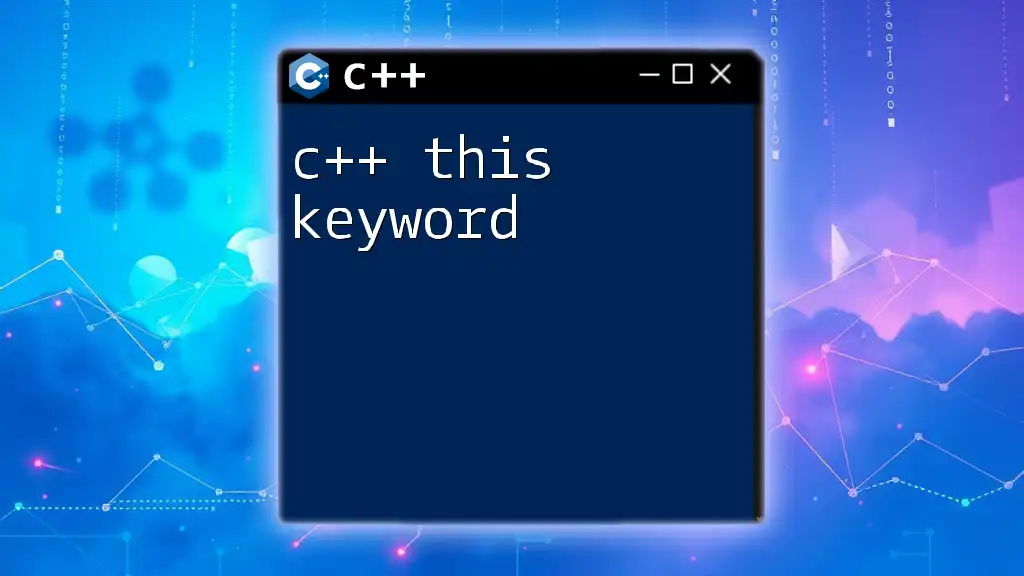
Using the "this" Pointer
Accessing Member Variables
One of the most common uses of "this" is to differentiate member variables from parameters, particularly in constructors. For instance, when a constructor parameter has the same name as a member variable, using "this" allows you to specify that the variable being referenced belongs to the current object.
Example: Basic Usage of "this"
class Example {
private:
int value;
public:
Example(int value) {
this->value = value; // Using 'this' to clarify that we are assigning to the member variable 'value'
}
};
In this example, `this->value` explicitly refers to the member variable `value`, resolving any ambiguity with the parameter.
Method Chaining with "this"
Method chaining is a technique that allows multiple method calls to be linked together in a single statement. This is made possible using "this" to return the current object from a method.
Example: Method Chaining
class Chain {
private:
int value;
public:
Chain& setValue(int value) {
this->value = value; // Setting the member variable
return *this; // Returning the current object for chaining
}
};
With this setup, you can chain multiple calls like this:
Chain obj;
obj.setValue(5).setValue(10); // Resulting in chained calls
Here, `setValue` method returns a reference to the current object, allowing us to call `setValue` consecutively.
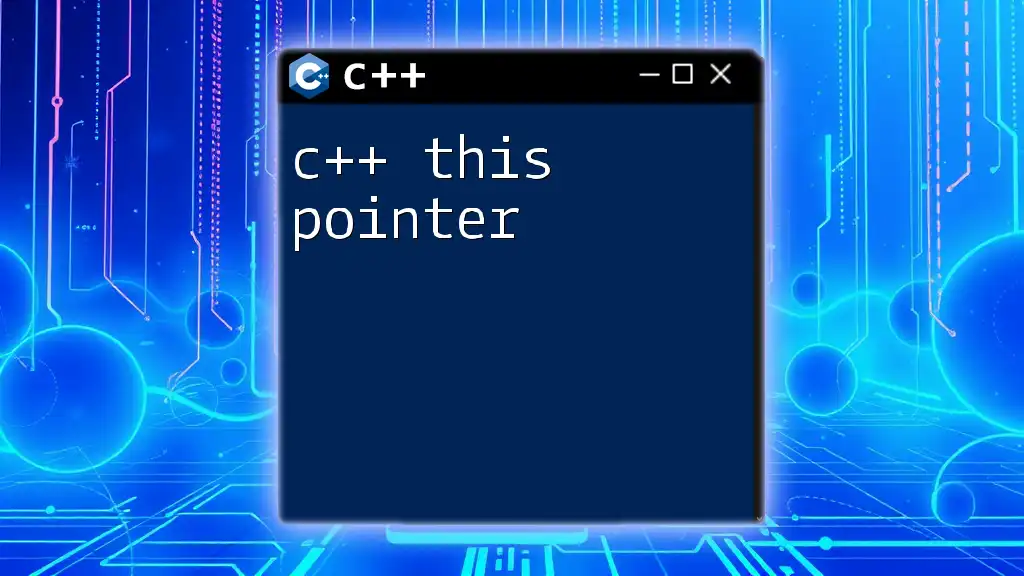
The Role of "this" in Operator Overloading
Implementing Operator Overloading
In C++, operator overloading allows you to define custom behavior for standard operators (like +, -, etc.) when they are applied to user-defined types. The "this" pointer is critical in these functions to access the left-hand operand of the operator.
Example: Operator Overloading for Addition
class Vector {
private:
int x, y;
public:
Vector(int x, int y) : x(x), y(y) {}
Vector operator+(const Vector& v) {
return Vector(this->x + v.x, this->y + v.y); // Accessing members of the current object using 'this'
}
};
In this example, the `operator+` method creates a new `Vector` object that represents the sum of the current object's coordinates and those of the argument.
The Importance of "this" in Comparison Operators
When overloading comparison operators, "this" is used to refer to the object being compared, allowing for a straightforward comparison.
Example: Comparison Operators
class Point {
private:
int x, y;
public:
bool operator==(const Point& p) {
return (this->x == p.x && this->y == p.y); // Using 'this' to access the current object's members
}
};
In this case, the `operator==` function checks for equality between two `Point` objects by accessing their x and y coordinates.
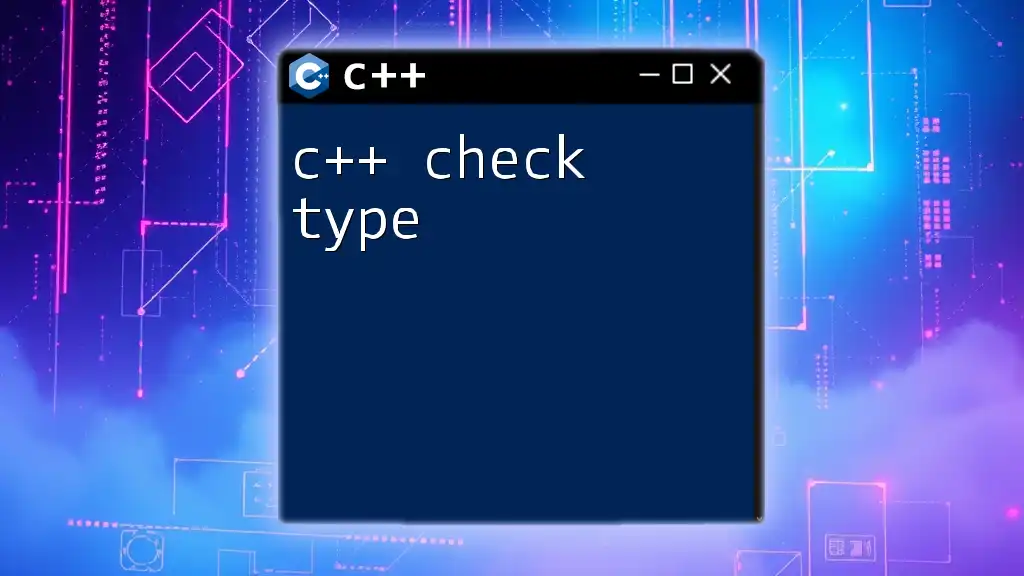
"this" in Virtual Functions
Understanding Polymorphism
Polymorphism is a core principle of object-oriented programming that allows methods to do different things based on the object it is acting upon. In C++, the "this" pointer is especially helpful in virtual functions, allowing derived classes to access their specific implementations.
Example of "this" with Virtual Functions
class Base {
public:
virtual void show() {
std::cout << "Base class" << std::endl;
}
void call() {
this->show(); // Using 'this' to invoke the correct version of show()
}
};
class Derived : public Base {
public:
void show() override {
std::cout << "Derived class" << std::endl;
}
};
Derived d;
d.call(); // Outputs: Derived class
In this example, calling `call()` will invoke the `show()` method of the `Derived` class because of the polymorphic behavior linked with "this".
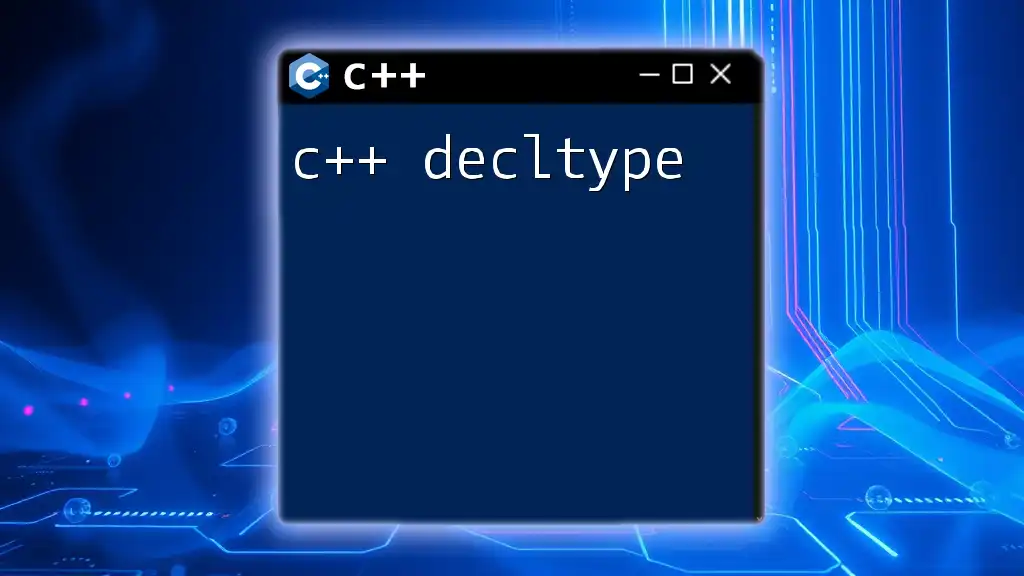
Best Practices for Using "this"
When to Use "this"
Use the "this" pointer in situations where:
- There is potential ambiguity between member variables and parameters.
- You are implementing method chaining.
- In operator overloading to unambiguously refer to the object’s member variables.
Performance Considerations
Using "this" generally does not affect performance significantly as it's optimized by the compiler. However, maintaining code readability and clarity is paramount. Ensure you use "this" when necessary but do not overuse it to the point of cluttering your code.
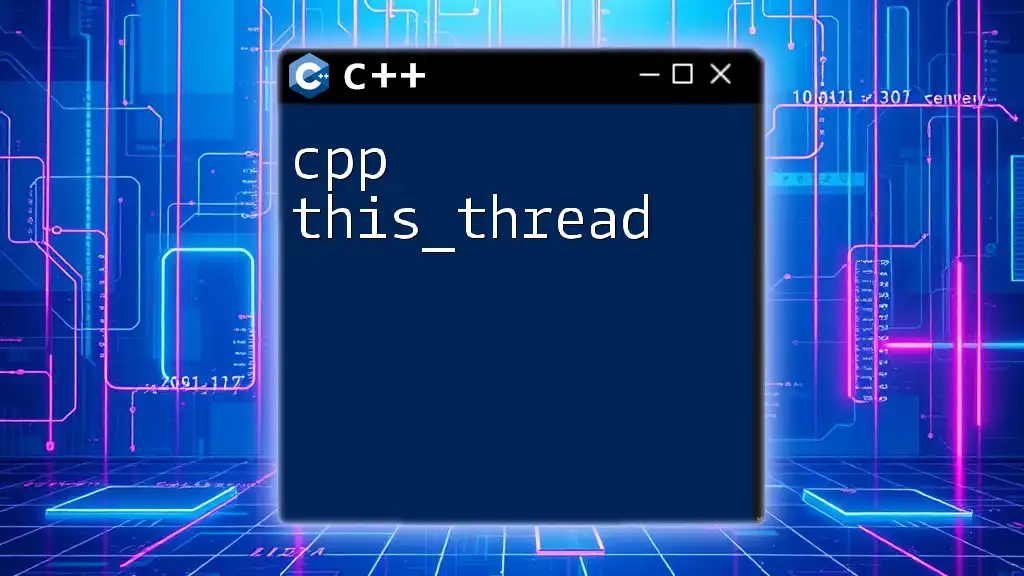
Common Mistakes Involving "this"
Forgetting to Use "this" in Complex Classes
A common mistake developers face is forgetting to use "this" in constructors or functions where member variables may conflict with local scope variables. This can lead to unexpected results and bugs.
Misunderstanding "this" in Static Functions
Since static functions do not belong to any instance, "this" cannot be accessed within them.
Example of Static Function
class Example {
public:
static void staticFunc() {
// 'this' is not accessible here
}
};
This limitation underlines the importance of understanding the context in which "this" can be used.
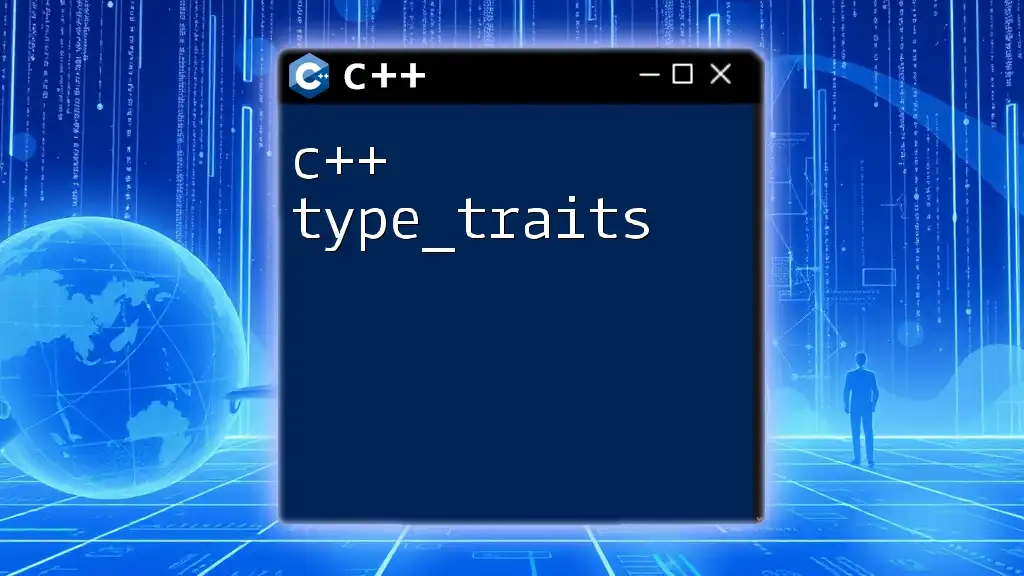
Conclusion
The "this" pointer is an integral part of C++ programming, particularly in the context of classes and object-oriented design. It serves to provide clarity and functionality by enabling access to the current object's members, facilitating method chaining, enhancing operator overloads, and supporting polymorphism in derived classes. As you work with C++, understanding and appropriately using "this" will undoubtedly enhance your programming proficiency. Practice implementing these concepts in your projects, and embrace the power of C++!
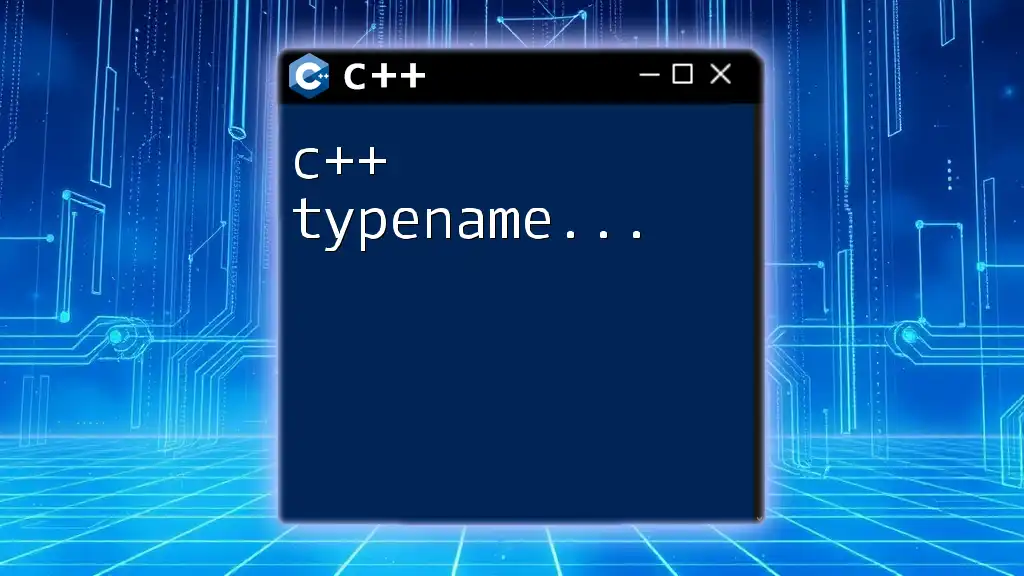
Further Reading and Resources
For those looking to deepen their understanding of object-oriented programming in C++, consider exploring additional resources including books focused on advanced C++ concepts, online tutorials, and courses that emphasize practical coding techniques. Knowing how to effectively utilize "this" will strengthen your coding skills and improve your overall development experience.