The `<cctype>` library in C++ provides functions for character classification and conversion, enabling operations like checking if a character is a digit or converting a character to uppercase.
Here's an example code snippet demonstrating its usage:
#include <iostream>
#include <cctype>
int main() {
char ch = 'a';
if (isalpha(ch)) {
std::cout << ch << " is an alphabet character." << std::endl;
std::cout << "Uppercase: " << (char)toupper(ch) << std::endl;
}
return 0;
}
What is #include cctype in C++?
To utilize the features provided by the `cctype` library, you must include it in your C++ program by using the following directive:
#include <cctype>
This inclusion should be at the beginning of your program, typically after including other standard libraries. The `#include cctype` directive informs the compiler that you will be using functions defined in the `<cctype>` header file, which is part of the C++ Standard Library.
Header files are essential in C++ as they contain declarations for functions, macros, and types, allowing you to utilize functionality without having to redefine it.
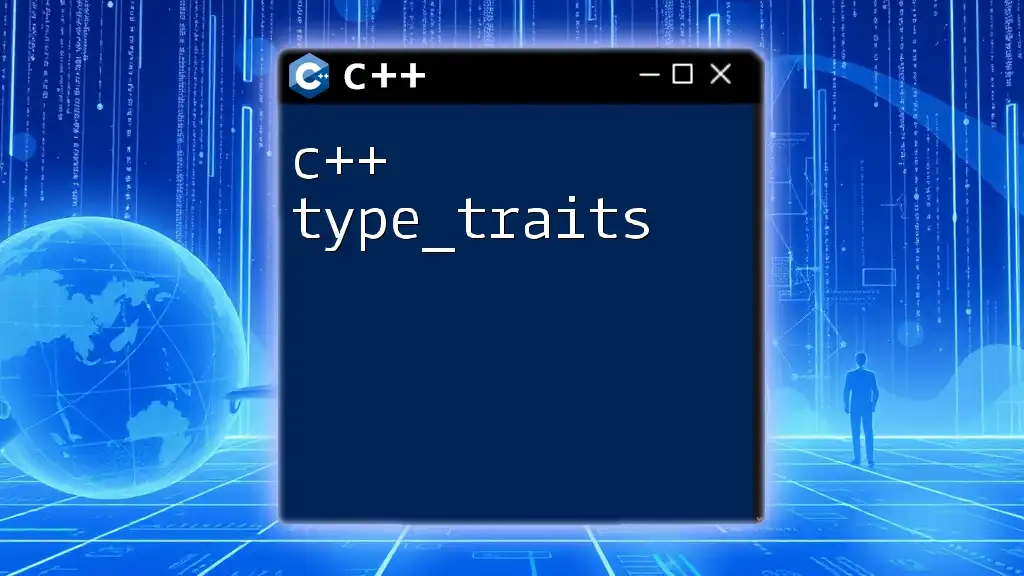
Overview of cctype in C++
The `cctype` library provides a suite of functions designed for handling character data. These functions are primarily used for character classification and conversion, making it easy to perform operations such as determining if a character is a letter, digit, or whitespace. Understanding and utilizing these functions is crucial for tasks that involve text processing and validation.
Character Classification Functions
Character classification involves determining the type of a character. The functions provided in `cctype` allow you to check if a character falls into specific categories.
isalpha: Checking for Alphabetic Characters
The `isalpha` function checks if a character is an alphabet letter (a-z, A-Z). It returns a non-zero value if the character is alphabetic; otherwise, it returns zero.
Example:
char ch = 'A';
if (isalpha(ch)) {
std::cout << ch << " is an alphabetic character." << std::endl;
} else {
std::cout << ch << " is not an alphabetic character." << std::endl;
}
In this example, since 'A' is an alphabetic character, the output will confirm this.
isdigit: Checking for Digits
The `isdigit` function checks if a character is a digit (0-9). Like `isalpha`, it returns a non-zero value for digits and zero otherwise.
Example:
char ch = '5';
if (isdigit(ch)) {
std::cout << ch << " is a digit." << std::endl;
} else {
std::cout << ch << " is not a digit." << std::endl;
}
This code will output that '5' is indeed a digit.
isalnum: Checking for Alphanumeric Characters
The `isalnum` function determines whether a character is either an alphabetic or a numeric character.
Example:
char ch = 'G';
if (isalnum(ch)) {
std::cout << ch << " is an alphanumeric character." << std::endl;
}
Here, 'G' qualifies as an alphanumeric character.
isspace: Checking for Whitespace Characters
The `isspace` function checks for whitespace characters such as spaces, tabs, and newlines.
Example:
char ch = ' ';
if (isspace(ch)) {
std::cout << "It's a whitespace character." << std::endl;
}
This outputs that the character is, in fact, a whitespace.
islower: Checking for Lowercase Letters
Use `islower` to check if a character is a lowercase letter.
Example:
char ch = 'd';
if (islower(ch)) {
std::cout << ch << " is a lowercase letter." << std::endl;
}
The output will confirm that 'd' is lowercase.
isupper: Checking for Uppercase Letters
Similarly, `isupper` checks if a character is an uppercase letter.
Example:
char ch = 'B';
if (isupper(ch)) {
std::cout << ch << " is an uppercase letter." << std::endl;
}
The program will output that 'B' is indeed uppercase.
ispunct: Checking for Punctuation Characters
The `ispunct` function checks if a character is a punctuation mark.
Example:
char ch = '!';
if (ispunct(ch)) {
std::cout << ch << " is a punctuation character." << std::endl;
}
In this instance, the output indicates that '!' is a punctuation character.
Character Conversion Functions
Character conversion functions allow you to change the case of characters.
tolower: Converting Characters to Lowercase
The `tolower` function converts a specified character to lowercase.
Example:
char ch = 'F';
char lowerCh = tolower(ch);
std::cout << "Lowercase of " << ch << " is " << lowerCh << std::endl;
This code converts 'F' to 'f'.
toupper: Converting Characters to Uppercase
The `toupper` function converts a specified character to uppercase.
Example:
char ch = 'g';
char upperCh = toupper(ch);
std::cout << "Uppercase of " << ch << " is " << upperCh << std::endl;
This will take 'g' and convert it to 'G'.
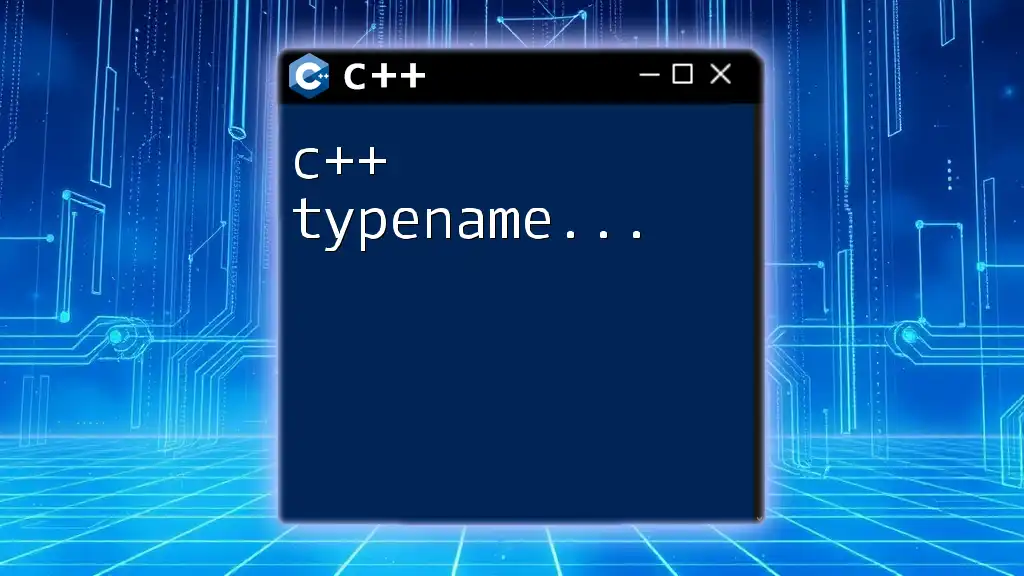
Using cctype Functions in Your C++ Programs
When you integrate `cctype` functions into your C++ programs, it can significantly streamline tasks related to character processing. Here’s a small example that demonstrates how various `cctype` functions can work together:
#include <iostream>
#include <cctype>
#include <string>
int main() {
std::string input = "Hello, World! 123";
for (char ch : input) {
if (isalpha(ch)) {
std::cout << ch << " is alphabetic." << std::endl;
} else if (isdigit(ch)) {
std::cout << ch << " is a digit." << std::endl;
} else if (isspace(ch)) {
std::cout << "'" << ch << "' is a whitespace character." << std::endl;
} else if (ispunct(ch)) {
std::cout << ch << " is a punctuation character." << std::endl;
}
}
return 0;
}
In this example, the program iterates over each character in the string "Hello, World! 123" and classifies them using the functions from `cctype`. The output will convey the types of characters present in the input string.
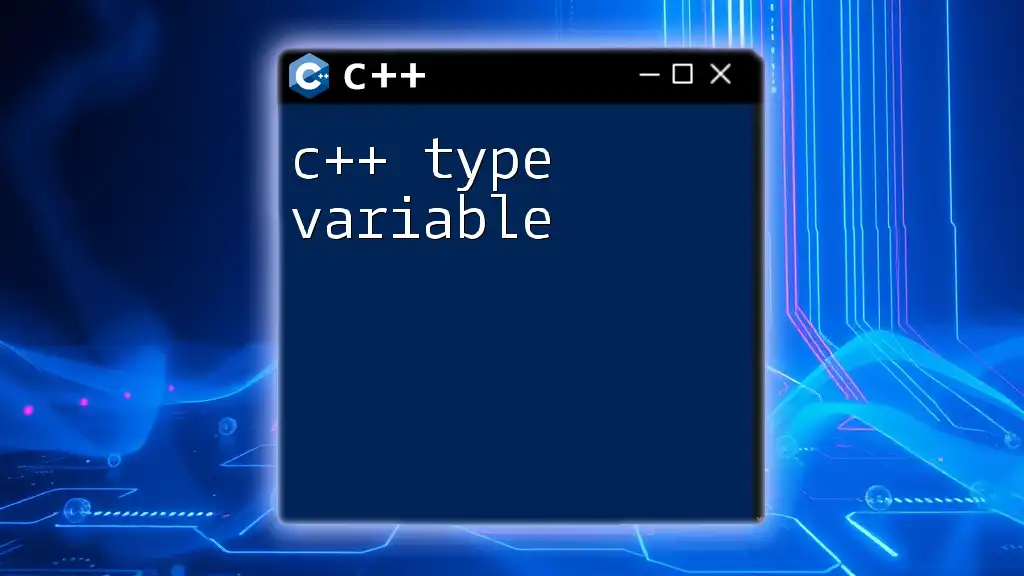
Best Practices for Using cctype in C++
To ensure effective use of `cctype` functions, consider the following best practices:
- Always include the `<cctype>` header in your programs that utilize these functions to avoid compilation errors.
- Combine classification and conversion functions for powerful character manipulation.
- Avoid passing non-character data types to these functions, as this may lead to undefined behavior.
- Do not assume that the `cctype` functions will handle all character sets; they are primarily designed for ASCII characters.
Common mistakes include confusing case for classification or using conversion functions without prior verification of the character type. Always validate before converting to ensure the operations yield the desired results.
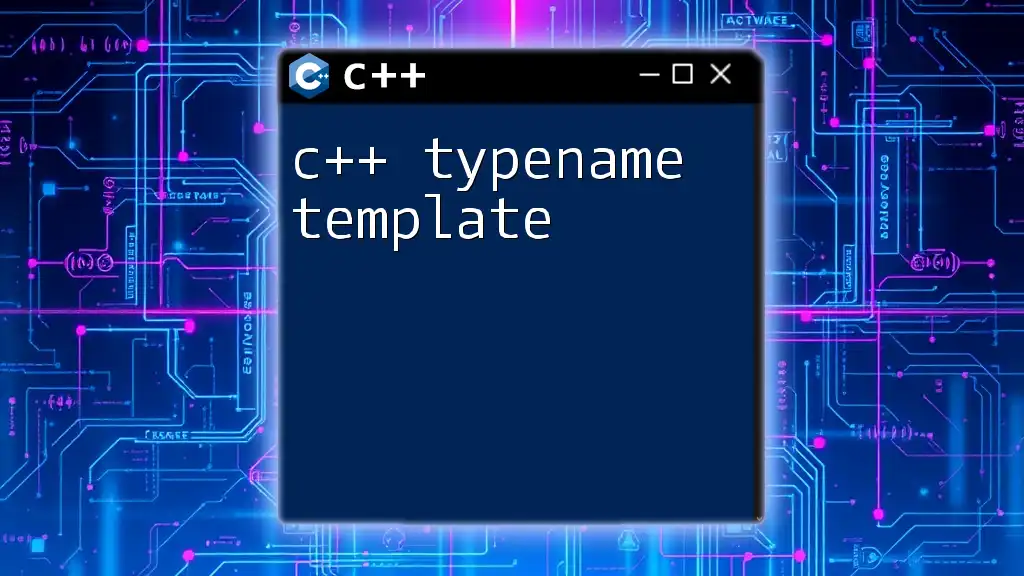
Conclusion
In the realm of C++ programming, the `cctype` library stands out as a vital tool for character handling. From character classification to transformation, mastering these functions facilitates efficient and effective data processing.
Encouraging readers to explore the extensive functionalities of `cctype` can lead to improved text handling in their programs. Practice with diverse examples will help reinforce understanding and skill in utilizing the `cctype` library effectively.
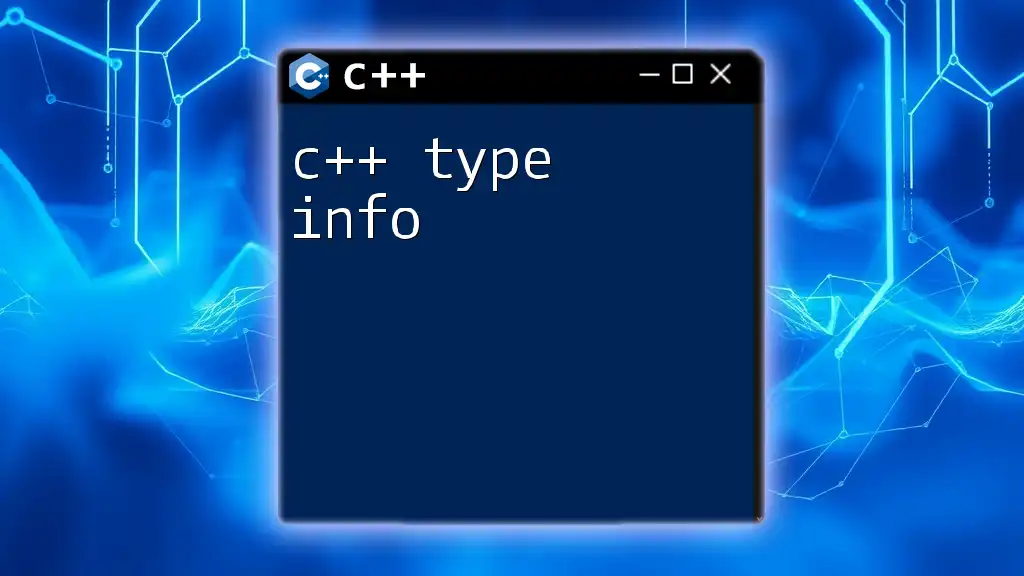
Additional Resources
For further learning, readers can explore comprehensive C++ programming resources, online tutorials, and the official documentation for C++ libraries. Engaging with community forums will also enhance understanding and application of advanced C++ concepts related to character handling and manipulation.