In C++, the `byte` type is typically represented using the `std::byte` enumerated type available in the `<cstddef>` header, which provides a way to explicitly handle byte-sized data without being tied to a specific integer type.
Here's a code snippet demonstrating its usage:
#include <cstddef>
#include <iostream>
int main() {
std::byte b = std::byte{0b10101010}; // Initializing a byte with binary value
std::cout << "Byte value: " << std::to_integer<int>(b) << std::endl; // Converting byte to int for output
return 0;
}
Understanding the C++ Byte Data Type
Definition of Byte in C++
In C++, a byte is a data type that typically represents 8 bits of data. This unit is crucial in the realm of programming as it serves as the fundamental building block for data representation. Bytes allow programmers to handle individual pieces of data, especially when working with lower-level functions such as direct memory access, file handling, and network communication.
Importantly, a byte is often represented using an unsigned char data type in C++. While a byte can hold values from 0 to 255 when defined as an unsigned type, in certain contexts, it may also be considered as signed, allowing it to hold values from -128 to 127 using the signed variant.
Characteristics of the C++ Byte Data Type
- Size: A byte always consists of 8 bits, which allows for the storage of 256 unique values.
- Range: When using unsigned char, the range is from 0 to 255, while for signed char, the range is from -128 to 127. Understanding how these ranges affect computation and storage is crucial for developers.
How to Declare and Use a Byte in C++
Declaring a Byte Variable
To declare a byte variable, you can use the `unsigned char` keyword. The syntax is straightforward:
unsigned char myByte = 255; // Example declaration
This line initializes a byte variable `myByte` with the maximum possible value of 255.
Setting and Modifying Byte Values
Setting a byte variable is just as simple as declaring it. You can assign a new value directly:
myByte = 100; // Example of modifying a byte value
This line reassigns `myByte` to 100.
Using Byte in Arithmetic Operations
While you can perform arithmetic operations with byte types, it is essential to be cautious. If the operations result in a value outside the allowable range for a byte, an overflow may occur. Here’s an example of adding two byte values:
unsigned char a = 100;
unsigned char b = 50;
unsigned char sum = a + b; // Summing byte values
However, due to the limited range of `unsigned char`, if `a` and `b` values exceeded 255, it would wrap around, which can lead to unexpected results. Therefore, always check your logic when performing operations on byte types.
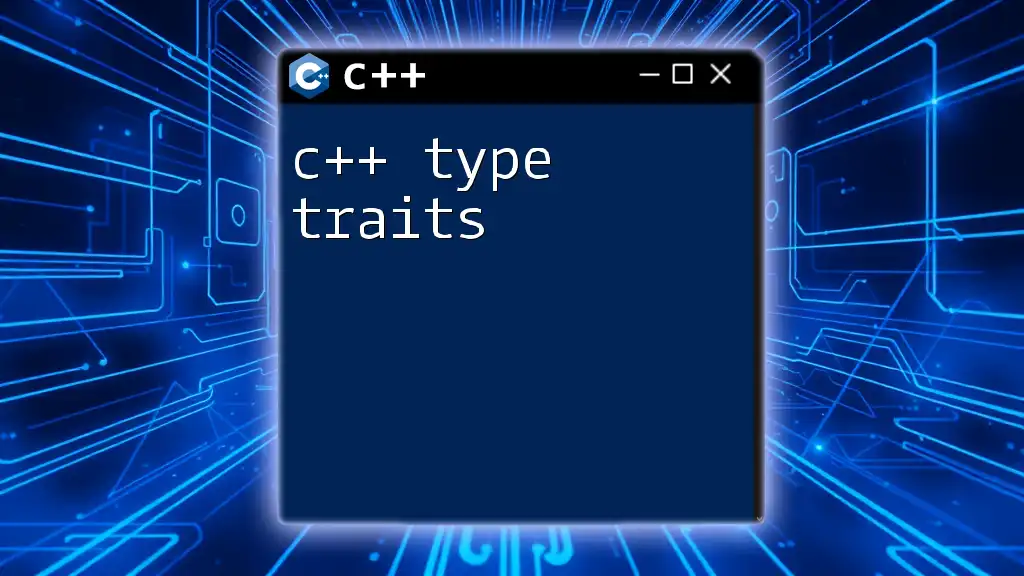
Byte Data Types in C++ Standard Library
Using std::byte (C++17 and onwards)
With the advent of C++17, a new byte type was introduced: `std::byte`. This type provides type safety that traditional byte implementations lack. To use `std::byte`, include the header `<cstddef>`. Here’s how to declare and initialize `std::byte`:
#include <cstddef>
std::byte myStandardByte = std::byte{0b1111'1111};
Benefits of Using std::byte Over Unsigned Char
Utilizing `std::byte` over `unsigned char` offers several advantages:
- Type Safety: `std::byte` does not allow implicit conversions to integral types, which significantly reduces errors in operations.
- Clearer Semantics: When you use `std::byte`, it is clear to other programmers that you are dealing with raw byte data, enhancing code readability.
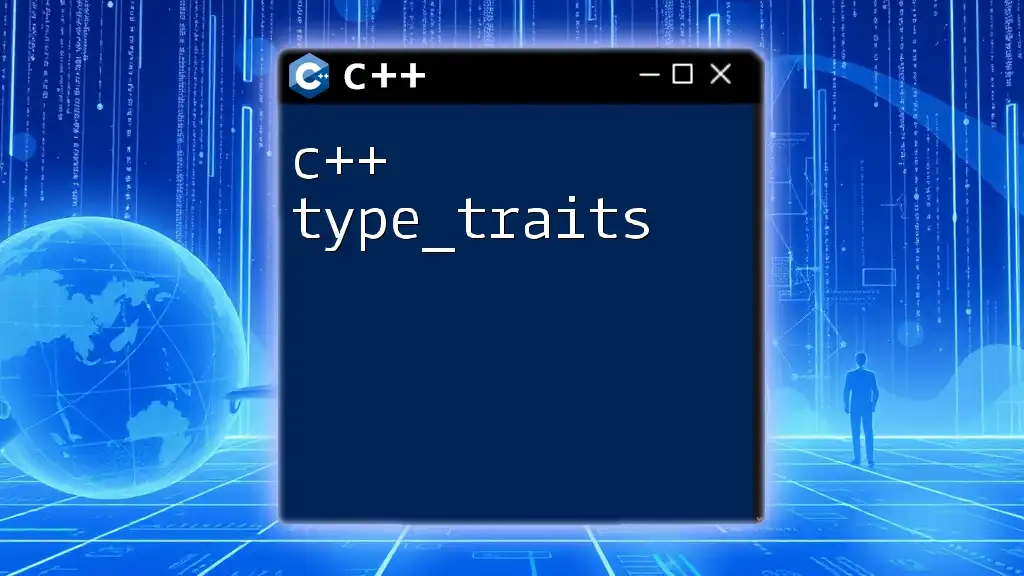
Practical Applications of C++ Byte Data Type
Storing Binary Data
Bytes are instrumental when you need to handle binary data. For instance, if you are implementing functionalities that require storing multiple bytes, such as buffers or arrays:
std::vector<std::byte> buffer(10); // A buffer of 10 bytes
This line declares a vector filled with 10 elements of type `std::byte`. Vectors provide dynamic size capabilities, making them ideal for buffering data.
File Handling and Byte Manipulation
Bytes are heavily utilized in file operations, especially when manipulating binary files. Here’s a simple example that illustrates reading and writing byte data:
#include <fstream>
#include <vector>
int main() {
std::vector<std::byte> dataToWrite = {std::byte{0x1}, std::byte{0x2}, std::byte{0x3}};
std::ofstream outFile("example.bin", std::ios::binary);
outFile.write(reinterpret_cast<const char*>(dataToWrite.data()), dataToWrite.size());
outFile.close();
// Reading back the data
std::vector<std::byte> dataRead(3);
std::ifstream inFile("example.bin", std::ios::binary);
inFile.read(reinterpret_cast<char*>(dataRead.data()), dataRead.size());
inFile.close();
}
In this code, we first write a vector of bytes to a binary file and then read it back. The use of `reinterpret_cast` allows us to treat the `std::byte` data as raw bytes, which is essential for file operations.
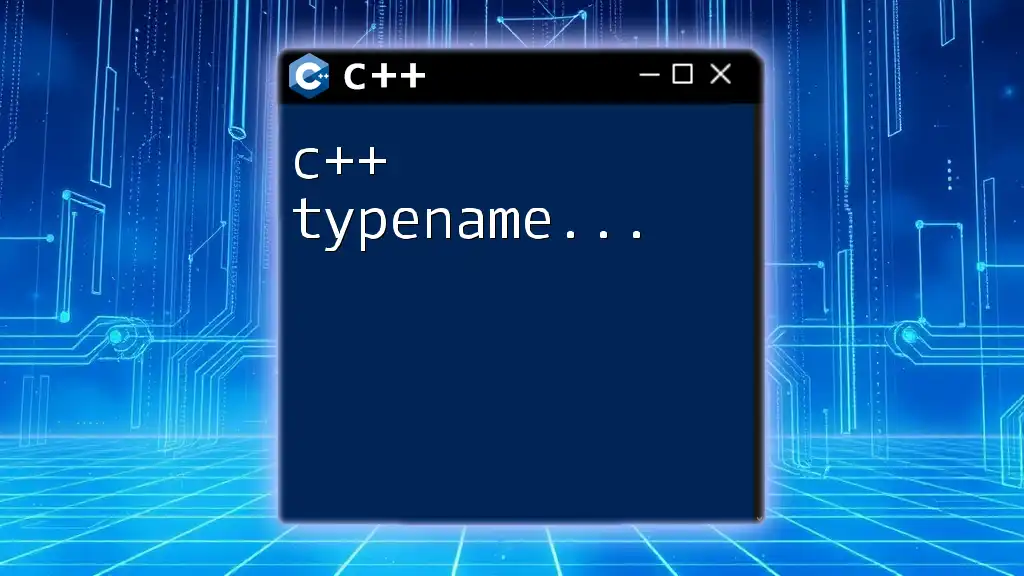
Common Pitfalls and Best Practices
Understanding Limits of Byte Types
One common issue is the overflow that occurs if a byte exceeds its storage capacity. For example:
unsigned char value = 255;
value++; // Watch out for overflow
This would lead to `value` wrapping around to 0, potentially causing logical errors in your application. Always validate inputs and safeguard against unexpected inputs.
Using Bytes in Modern C++
Emphasizing the importance of using `std::byte` is crucial in modern C++ programming. By choosing `std::byte`, you leverage type safety and clearly convey the intent of handling raw byte data. This practice not only makes the code safer but also enhances maintainability.
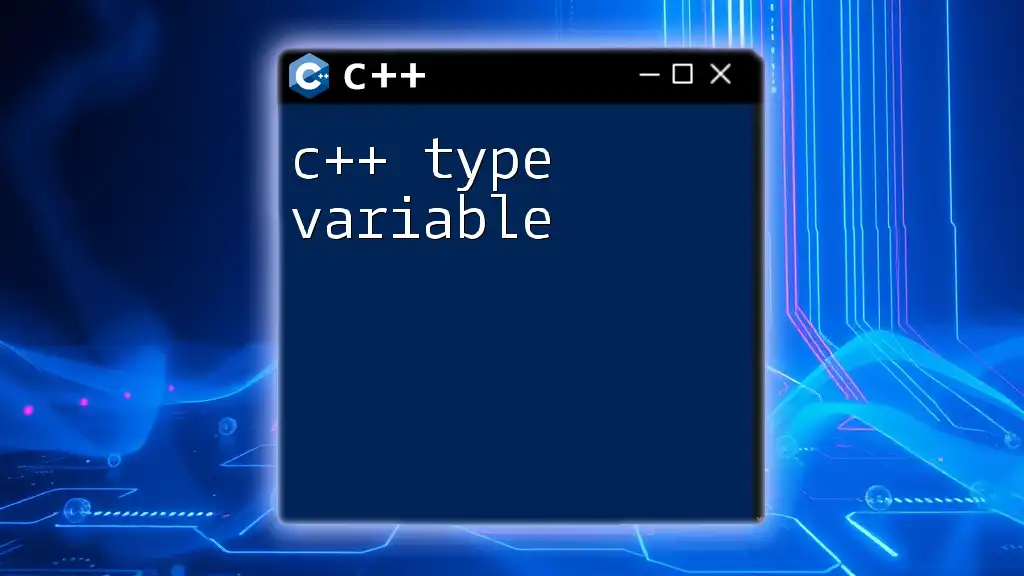
Conclusion
The C++ type byte plays a vital role in low-level programming. Understanding its characteristics, usage, and potential pitfalls can significantly enhance your C++ programming skills. Experimenting with bytes in various scenarios will deepen your understanding and facilitate more efficient data handling in your software projects.