In C++, the error "type name is not allowed" typically occurs when the compiler expects a type name but encounters an invalid or incomplete declaration, often due to syntax errors or misuse of keywords.
Here’s an example that triggers this error:
class MyClass {
int myFunction(); // Correct declaration
int myFunction(); // This causes "type name is not allowed" if this line was incorrectly formatted
};
Understanding the Error Message
What Does "Type Name Is Not Allowed" Mean?
The error message "type name is not allowed" typically indicates issues related to incorrectly declared types in your C++ code. This error arises when the compiler encounters a declaration it does not recognize as valid. Understanding its context is crucial, as this message often points to underlying syntax problems or misuse of reserved keywords.
Why the Error Occurs
This error can occur in several situations, primarily revolving around type name usage. When declaring classes, structs, or enums, certain rules and conventions must be followed. If these conventions are not met—whether through syntax errors, keyword misuse, or improper declarations—the compiler will emit this error to inform you that it cannot process your request correctly.
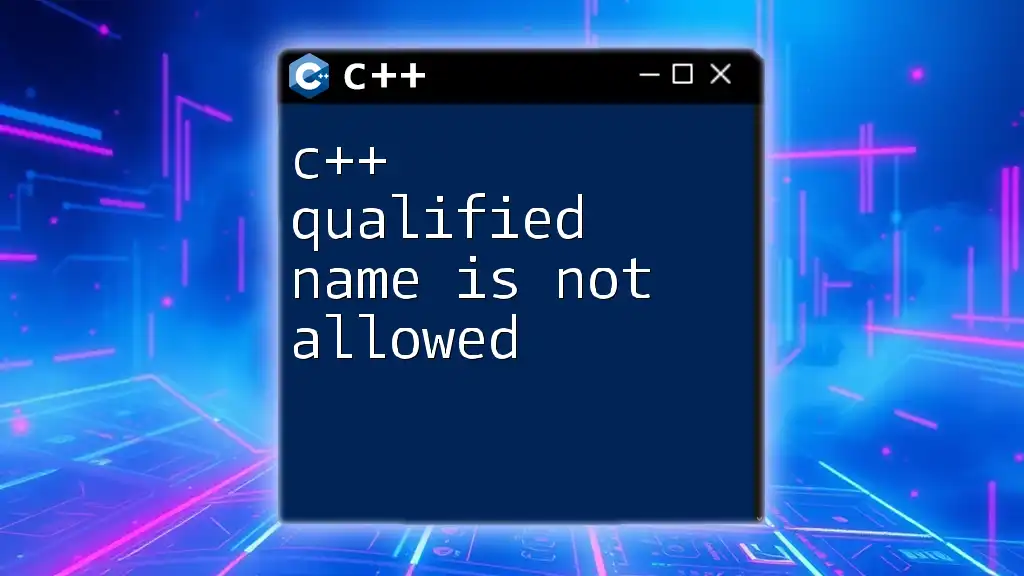
Common Causes and Examples
Misuse of Keywords
C++ has many reserved keywords, and using them as type names can lead to confusion. When you try to declare a class or type using a keyword, the compiler raises the "type name is not allowed" error.
Example: Using a reserved keyword as a type name can result in this error:
class class { // Error: type name is not allowed because 'class' is a keyword.
public:
int value;
};
In this case, the keyword `class` cannot be used as an identifier, and doing so raises a syntax error.
Incorrect Type Definitions
Another frequent cause for the "type name is not allowed" error is missing crucial syntax elements, such as semicolons or misplaced braces. In C++, every type definition must adhere to a specific syntax.
Example: This incorrect structure leads to an error due to a missing semicolon:
struct MyStruct { // Correct
int x;
int y;
} // Note: Must follow with a semicolon before using the struct.
struct YourStruct {
int a;
int b // Error: type name is not allowed due to missing semicolon.
}
The absence of a semicolon after the closing brace of `YourStruct` confuses the compiler, triggering the specified error.
Template Issues
Templates are powerful features in C++, but improper syntax while defining or instantiating templates can also result in the "type name is not allowed" message.
Example: Omitting essential characters in template definitions leads to errors:
template<typename T
class MyClass { // Error: type name is not allowed due to missing '>'.
public:
T value;
};
Here, the missing '>' at the end of the template declaration causes the compiler to misinterpret the code structure, resulting in confusion about the type name.
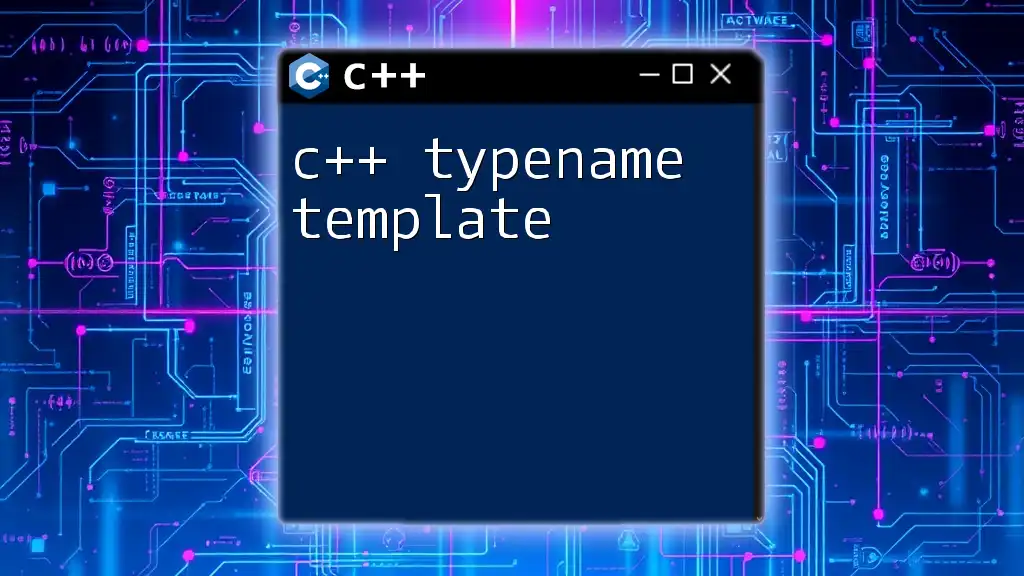
How to Fix the Error
Debugging Techniques
When facing this error, employ systematic debugging techniques. Start by reading the compiler's error messages closely. They often provide crucial hints about where the error originates. Pay attention to nearby lines of code that might be incorrectly formatted or mishandled.
Code Correction Strategies
Using Compiler Error Messages
Compiler messages are your first line of defense. They may indicate the line number and context of the offending code. Reviewing these messages can guide you toward rectifying the issue.
Code Refactoring
A significant key to resolving this error often involves refactoring your code. Take the time to re-evaluate your class and type definitions. Consider renaming conflicting identifiers or restructuring type declarations to comply with C++’s syntax rules.
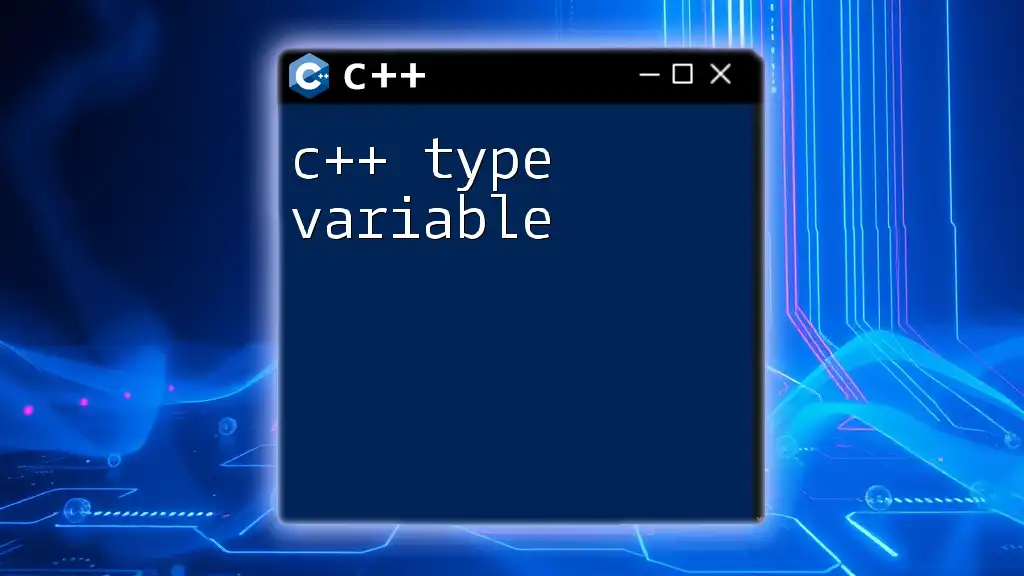
Best Practices to Avoid "Type Name Is Not Allowed"
Naming Conventions
Implementing clear and concise naming conventions helps prevent conflicts with C++ reserved keywords. Avoid using any language keywords as type names, and choose intuitive identifiers that describe the purpose of each type.
Proper Syntax
Paying close attention to syntax is essential. Ensure that every type declaration is correctly formed with all required punctuation. Double-check braces, semicolons, and other critical elements during code reviews or while writing code.
Utilizing IDE Features
Integrated development environments (IDEs) can be incredibly helpful in catching syntax errors early. Many IDEs flag potential errors in real-time, allowing you to correct them before compiling. Make use of these features to minimize errors related to type declarations.
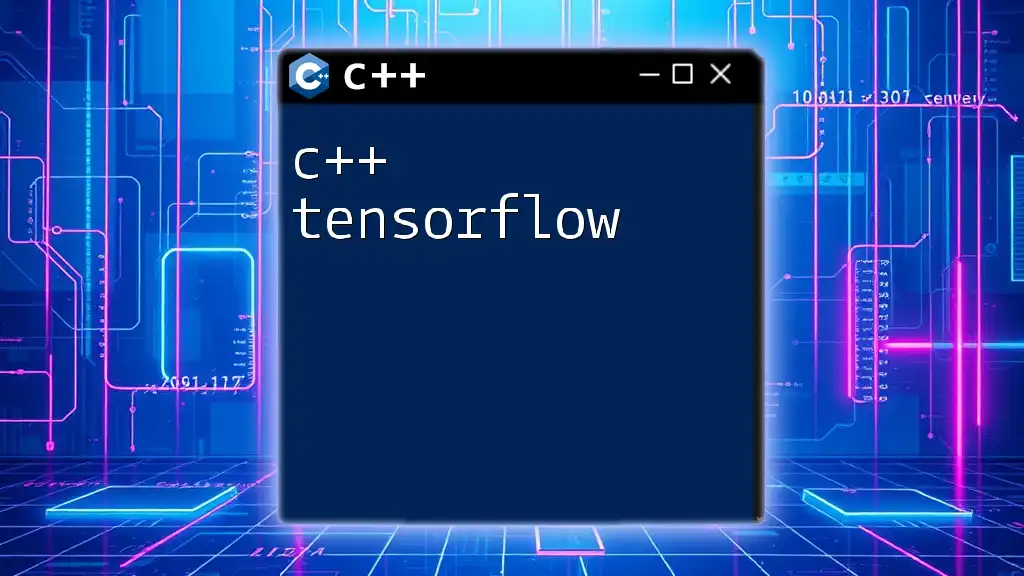
Conclusion
Understanding the "c++ type name is not allowed" error is essential for anyone looking to improve their C++ programming skills. By recognizing the common causes of this error and implementing best practices, you can write clearer, more efficient code. Pay attention to identifiers, maintain proper syntax, and don't hesitate to utilize the debugging tools available to you. Mastering these concepts will significantly enhance your coding experience and effectiveness in C++.
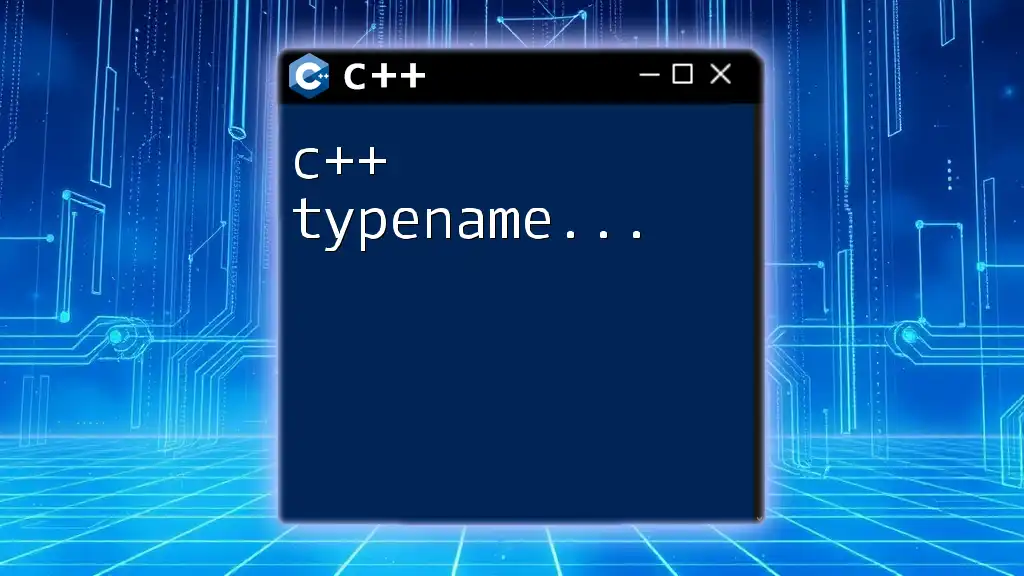
FAQs
What should I do if I encounter this error in legacy code?
When dealing with legacy code encountering this error, review the compiler messages closely. Identify the outdated or incorrect type names used in the code. Refactoring or renaming variables, along with updating syntax to meet modern C++ standards, often resolves the issue while improving code quality.
Are there any tools that can help prevent this error?
Yes, utilizing coding standards tools and linters can help catch many potential issues before they lead to compilation errors. Tools like `CPPcheck`, `Clang-Tidy`, and even integrated features in modern IDEs can provide real-time feedback to prevent such errors from occurring.
Can this error occur in other programming languages?
While similar errors exist in other programming languages, the specific message "type name is not allowed" is unique to C++. However, many languages with reserved keywords will raise errors if you try to use these keywords as identifiers or type names. Understanding how each language handles type declarations is critical for preventing similar issues.