In C++, exponential notation allows you to represent floating-point numbers in a more compact form using a base and an exponent, typically denoted as `m * 10^n`, where `m` is the mantissa and `n` is the exponent.
Here's a code snippet demonstrating exponential notation in C++:
#include <iostream>
#include <iomanip>
int main() {
double num = 1.23e4; // Equivalent to 1.23 * 10^4
std::cout << std::fixed << std::setprecision(2) << num << std::endl; // Outputs: 12300.00
return 0;
}
Understanding Exponential Notation
Exponential notation is a way to represent both very large and very small numbers efficiently and concisely. This notation is particularly crucial in programming scenarios where precision and clarity are vital. In C++, exponential notation typically takes the format `mEn`, where `m` is the mantissa, and `n` is the exponent. For instance, the number 5,000 can be represented as `5E3`, indicating \(5 \times 10^3\).
In programming and scientific calculations, understanding how to effectively utilize exponential notation can greatly enhance both the accuracy of your operations and the readability of your code.
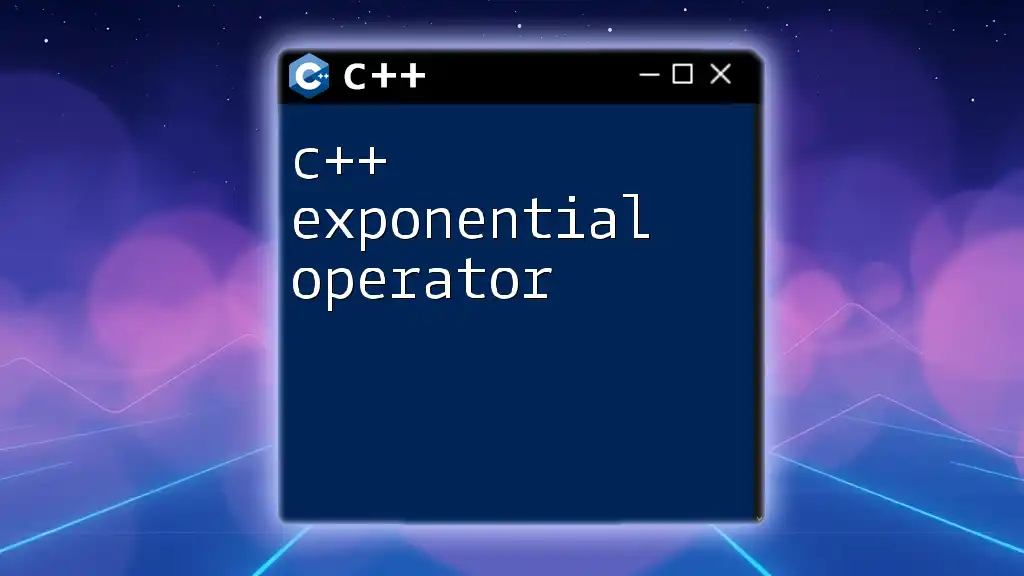
C++ Data Types Supporting Exponential Notation
When working with exponential notation in C++, you will primarily use floating-point data types. These types are special numeric types that provide a way to handle real numbers with decimal points, which is essential for calculations involving exponential values.
Floating-Point Types
C++ includes three main floating-point types: `float`, `double`, and `long double`. Each type offers a different level of precision and range:
- `float`: Typically represents a single-precision 32-bit floating-point value, sufficient for many applications.
- `double`: Generally a double-precision 64-bit floating-point value, offering greater accuracy and a wider range, making it a popular choice for scientific calculations.
- `long double`: Provides even more precision than `double`, but its exact implementation can vary depending on the compiler and system architecture.
Example:
float f = 1.23e4; // Represents 12300.0
double d = 5.67e-3; // Represents 0.00567
In the examples above, `f` holds the value representing 12,300, while `d` accurately represents 0.00567. These types allow for a flexible and efficient way to work with exponential numbers.
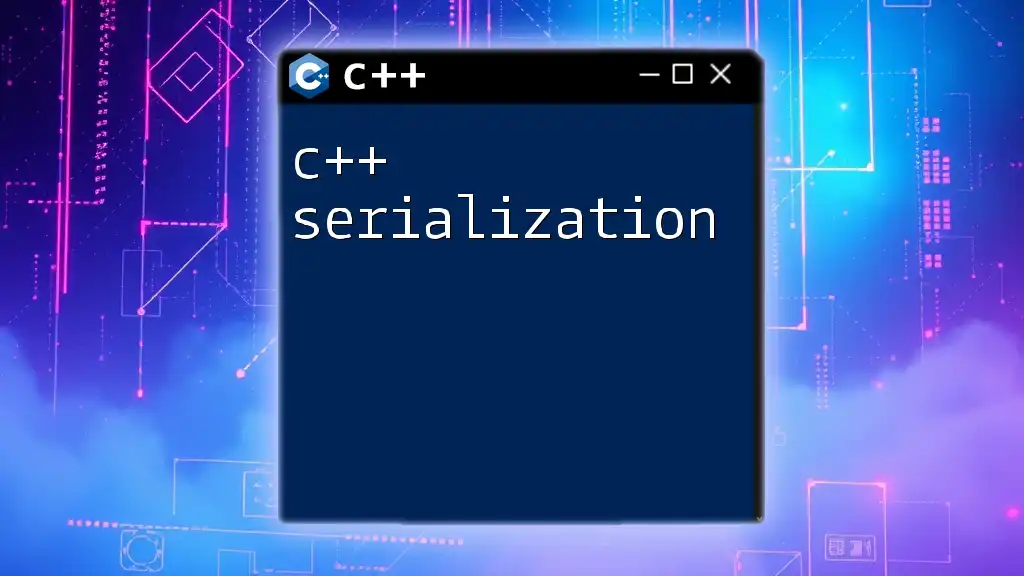
Using Exponential Notation in C++ Output
Displaying numbers in exponential notation is straightforward with the use of C++'s `cout`. By formatting the output correctly, you can present the values in an easily understandable format, particularly when dealing with very large or small numbers.
Formatting options available in C++
To display numbers in scientific or exponential form, C++ provides the `std::scientific` manipulator, which formats floating-point numbers using scientific notation.
Example:
#include <iostream>
#include <iomanip>
int main() {
double num = 123456.789;
std::cout << std::scientific << num << std::endl; // Outputs: 1.234568e+05
return 0;
}
In this snippet, the `std::scientific` manipulator forces `num` to be displayed in scientific notation, making it much easier to handle large numbers at a glance.
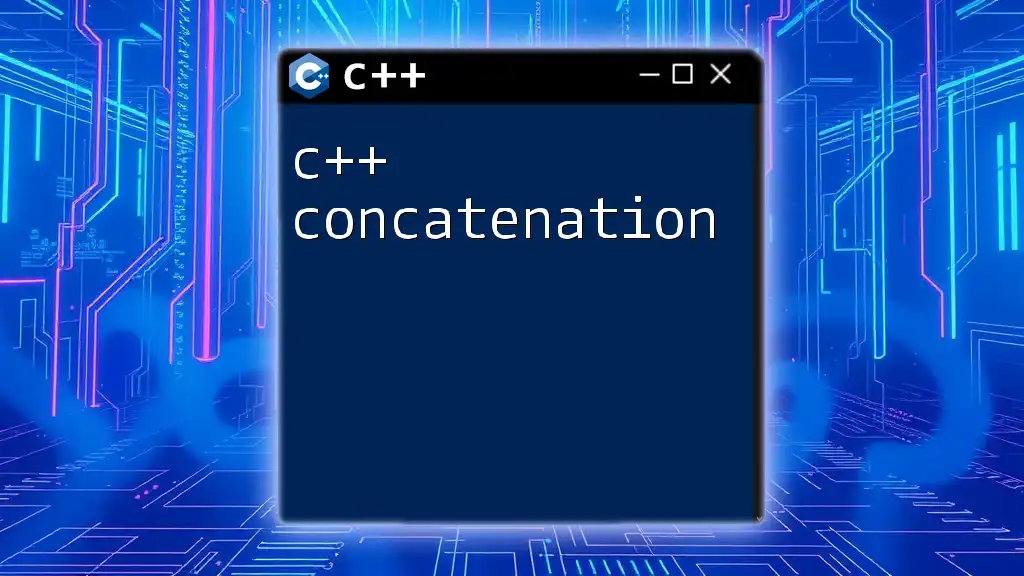
Reading and Converting Exponential Notation
In addition to displaying exponential numbers, C++ also allows you to read them from user input. This can be particularly useful in applications where scientific data may be input in exponential format. The conversion of strings representing exponential values into numeric types can be done easily using functions like `stod` and `stof`.
Example:
#include <iostream>
int main() {
std::string strNumber = "4.5e2"; // Input in exponential form
double number = std::stod(strNumber); // Convert to double
std::cout << number << std::endl; // Outputs: 450
return 0;
}
The code above reads a string representing 450 in exponential form and converts it to a double, showcasing how you can easily handle numerical data in different formats.
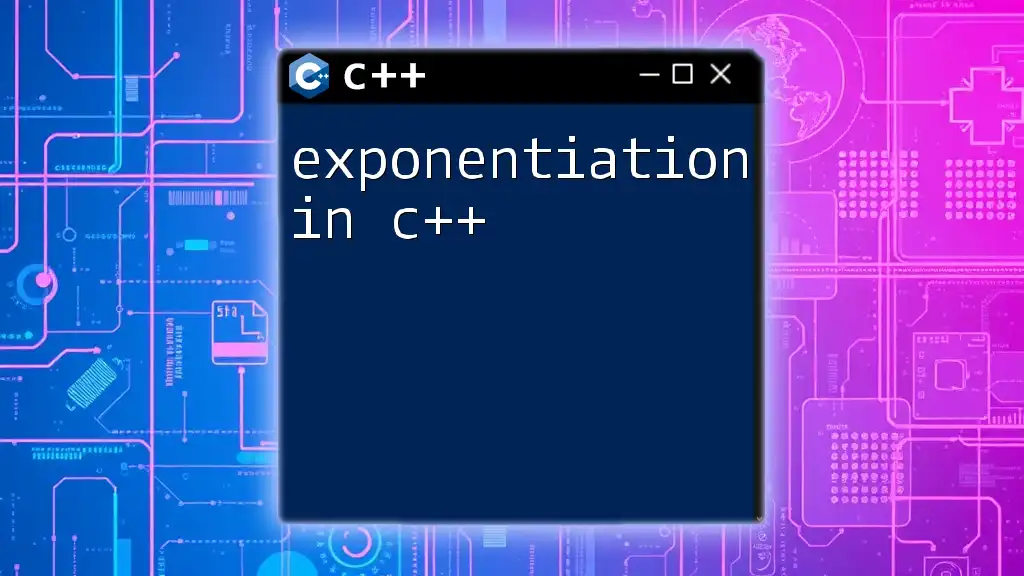
Working with C++ Scientific Notation
C++ supports scientific notation, which is a specific case of exponential notation often used in mathematics and physical sciences. The structure is similar to exponential notation but is specifically geared toward representing values in a scientific context.
When to use scientific notation typically arises in fields such as physics, engineering, and computational sciences, where a significant range of values needs to be expressed compactly.
Example:
#include <iostream>
#include <iomanip>
int main() {
double value = 6.02e23; // Avogadro's number
std::cout << std::scientific << std::setprecision(2) << value << std::endl; // Outputs: 6.02e+23
return 0;
}
Here, the precision is set to two decimal places using `std::setprecision`, making it clear and concise for any scientific calculations involving constants like Avogadro's number.
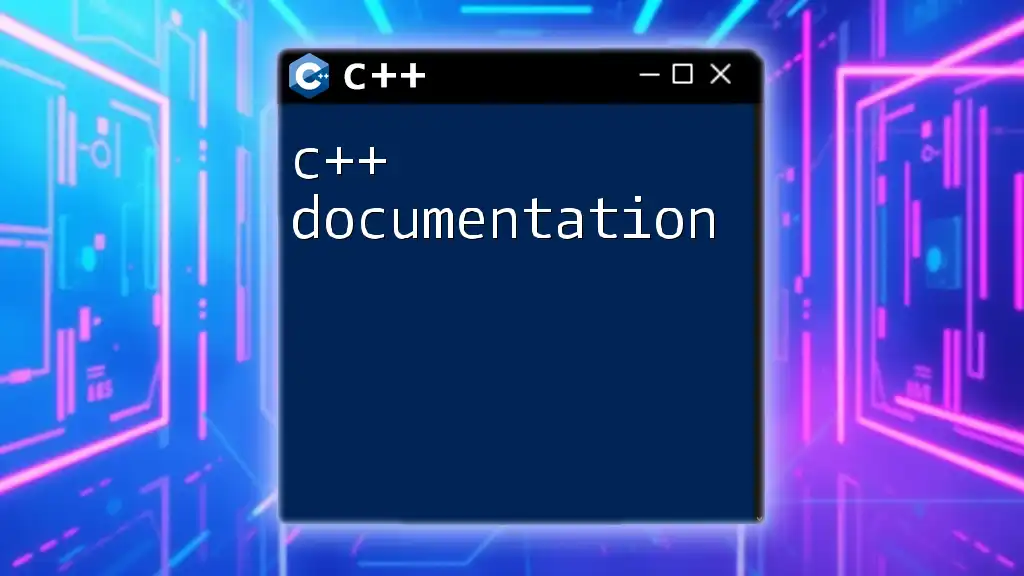
Common Pitfalls and Best Practices
When working with floating-point arithmetic in C++, it’s essential to be aware of its limitations due to precision. Misunderstanding how floating-point numbers are represented in memory can lead to errors, particularly when performing arithmetic operations.
Best practices include:
- Always be aware of precision and rounding issues.
- Handle exceptional cases carefully, such as overflow (exceeding the maximum value) and underflow (approaching zero).
- Use `double` over `float` in critical calculations to minimize potential loss of precision.
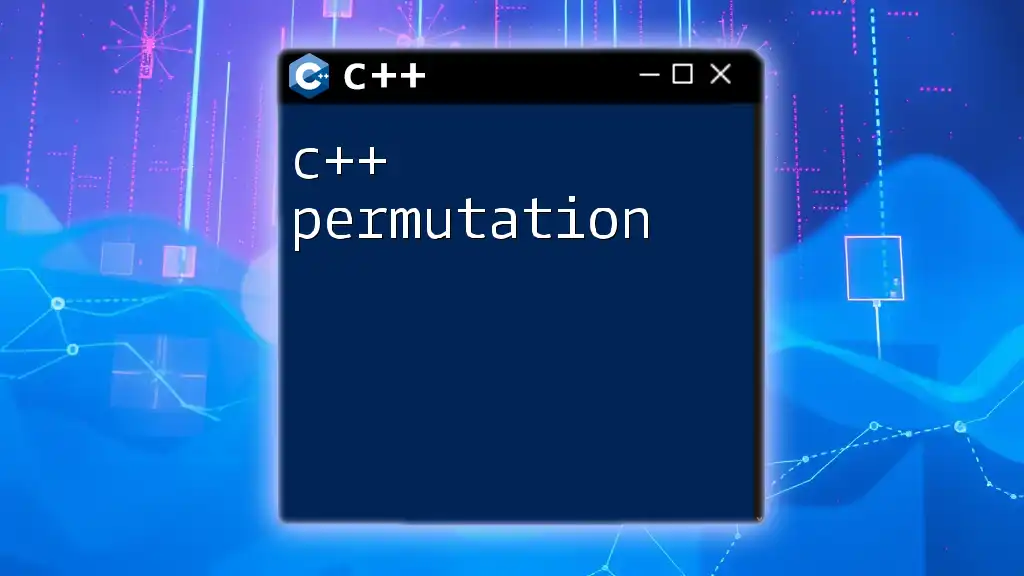
Advanced Applications of Exponential Notation in C++
Exponential notation finds extensive use in scientific calculations, simulations, and algorithms that require handling of large datasets. C++ libraries, such as `<cmath>`, offer numerous functions that facilitate mathematical operations with floating-point numbers, including those in exponential format.
Example:
#include <iostream>
#include <cmath>
int main() {
double a = 1.0e1; // 10
double b = 2.0e2; // 200
double result = pow(a, b); // Exponential increase
std::cout << "Result: " << result << std::endl; // Outputs: Result: 1e+20
return 0;
}
This example demonstrates exponentiation, where `pow` is used to calculate \(10^{200}\), which yields an extremely large number. Handling such calculations correctly is essential for applications ranging from scientific research to financial modeling.
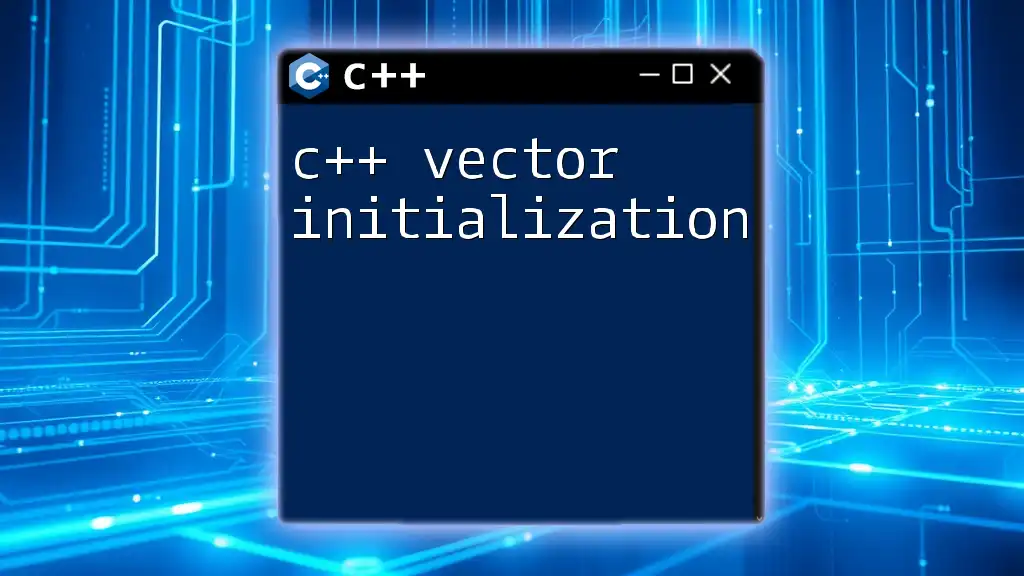
Conclusion
Understanding and effectively using C++ exponential notation is crucial for programming tasks that involve large or small numbers. It opens up opportunities in areas such as scientific programming and data analysis. Knowing how to utilize different data types, display values, and convert them appropriately makes your work more efficient and enhances the readability of your code.
As you explore this topic further, consider diving into advanced applications and best practices. This knowledge not only improves your skills but also enriches your programming repertoire, preparing you for complex challenges ahead.
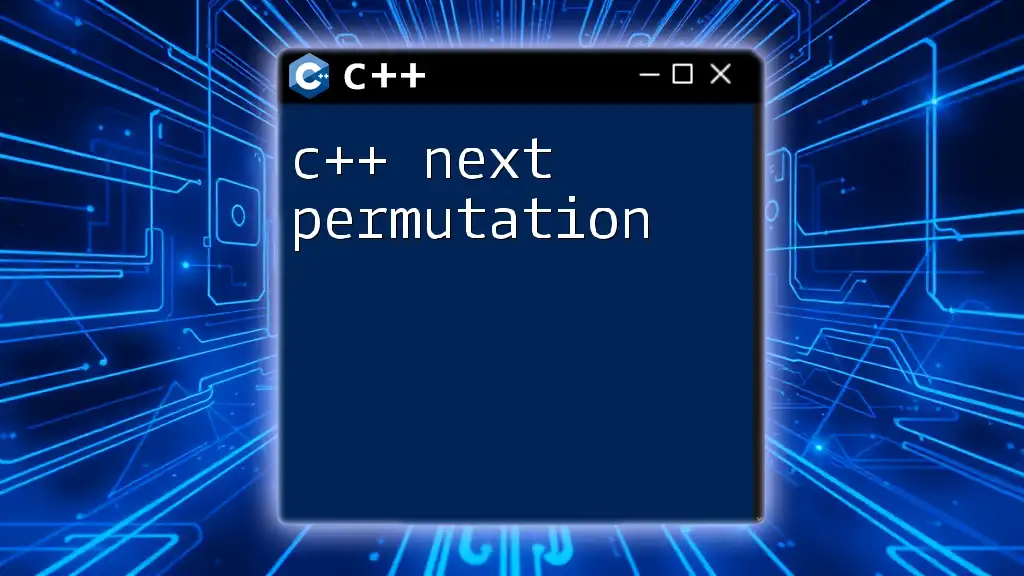
Call to Action
Stay tuned for more tips on mastering C++ and expanding your programming knowledge. Don't forget to subscribe for updates and additional resources related to C++ programming!