C++ input validation ensures that user inputs meet specified criteria, preventing errors and enhancing program robustness.
#include <iostream>
#include <limits>
int main() {
int number;
std::cout << "Enter a positive integer: ";
while (!(std::cin >> number) || number <= 0) {
std::cin.clear(); // clear the error flag
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n'); // discard invalid input
std::cout << "Invalid input. Please enter a positive integer: ";
}
std::cout << "You entered: " << number << std::endl;
return 0;
}
Understanding C++ Input Validation
Input validation is a critical process that ensures the user-provided data adheres to the expected format and type. In programming, particularly in C++, validating input is essential for preventing potential errors that might occur due to unexpected user behavior. Without proper validation, applications may crash, produce incorrect results, or become susceptible to security vulnerabilities.
In C++, validating user input involves checking the types and formats of input to ensure they conform to the expected parameters before proceeding with further processing. By prioritizing input validation, developers create more robust and secure applications.
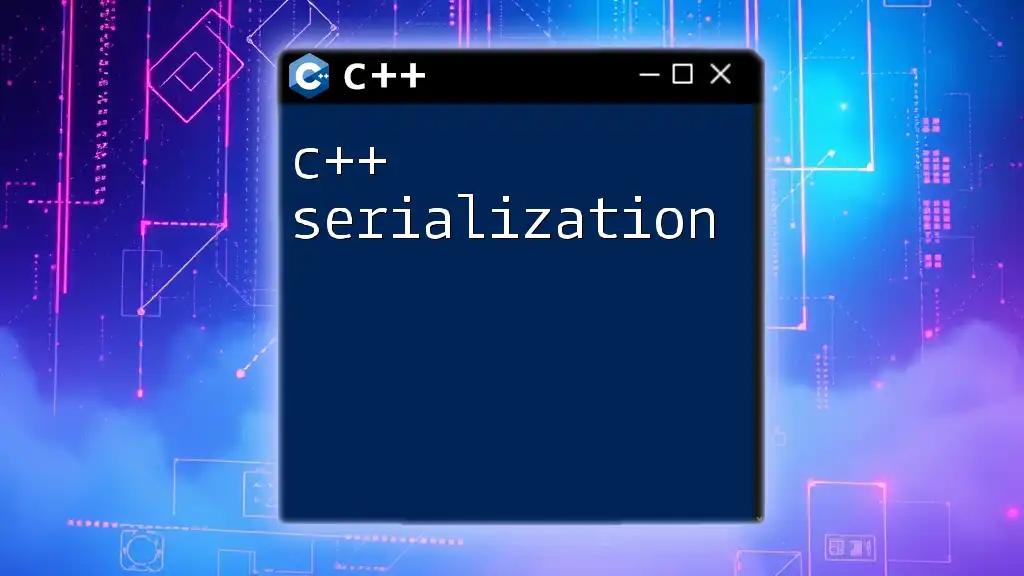
Understanding the Basics of C++
Before delving into input validation, it is important to understand a few key aspects of C++. C++ is a powerful programming language that provides numerous features, including strong type checking and exception handling. These features can be utilized to validate input effectively.
Brief Overview of C++
C++ supports various data types, including integers, floats, and characters, and each data type has corresponding operations. Input in C++ is typically handled using the `cin` object, which reads data from the standard input, usually the keyboard. Here’s a simple example to demonstrate basic input retrieval:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
cout << "You entered: " << number << endl;
return 0;
}
In the above code, the program prompts the user to enter a number, captures the input, and displays it. However, this simple implementation lacks input validation and may fail if the user enters an invalid input type.

Methods for C++ Input Validation
Basic Input Validation Techniques
Using `cin` for Input
To effectively validate input in C++, developers can rely on the `cin` object while simultaneously employing specific techniques. One of the most straightforward methods involves employing `cin` to read input while checking for errors.
Checking Input Types
C++ inherently checks for type safety, meaning the type of variable must be consistent with the type of the input being stored. For example, if the user attempts to input a character when an integer is expected, `cin` will fail. To enhance this, programmers can check for input types using the `std::cin.fail()` method, as demonstrated in the code snippet below:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
if (cin.fail()) {
cout << "Invalid input! Please enter an integer." << endl;
cin.clear(); // Clear the error flag
cin.ignore(numeric_limits<streamsize>::max(), '\n'); // Discard the invalid input
} else {
cout << "You entered: " << number << endl;
}
return 0;
}
In this snippet, if the user inputs an invalid type, the program clears the error flag and ignores the remaining invalid input, allowing for another attempt. This method promotes a better user experience by handling errors smoothly.
Advanced Input Validation Techniques
Function-Based Input Validation
Modularizing your code through functions dedicated to validation can enhance code readability and reusability. By wrapping input validation logic into a function, you effectively separate concerns. Here's an example:
#include <iostream>
using namespace std;
bool validateInput(int &input) {
cout << "Enter a number: ";
cin >> input;
if (cin.fail()) {
cin.clear();
cin.ignore(numeric_limits<streamsize>::max(), '\n'); // Ignore the leftover invalid input
return false; // Return false if the input is invalid
}
return true; // Return true if the input is valid
}
int main() {
int number;
while (!validateInput(number)) {
cout << "Please try again." << endl;
}
cout << "You entered: " << number << endl;
return 0;
}
When utilizing a function for validation, the code becomes more organized, as input requests and checks can be centralized. This structure allows easier maintenance and debugging.
Ranged Input Validation
Validating that input is within a specified range is another crucial aspect of input validation. This is particularly relevant for numeric inputs where certain constraints exist. The following sample code ensures that the user inputted number falls between 1 and 10:
#include <iostream>
using namespace std;
bool validateRange(int input, int min, int max) {
return input >= min && input <= max;
}
int main() {
int number;
do {
cout << "Enter a number between 1 and 10: ";
cin >> number;
} while (!validateRange(number, 1, 10));
cout << "You entered a valid number: " << number << endl;
return 0;
}
In this example, if the user inputs a number outside the defined range, they are prompted to enter a valid number until they comply with the boundaries.
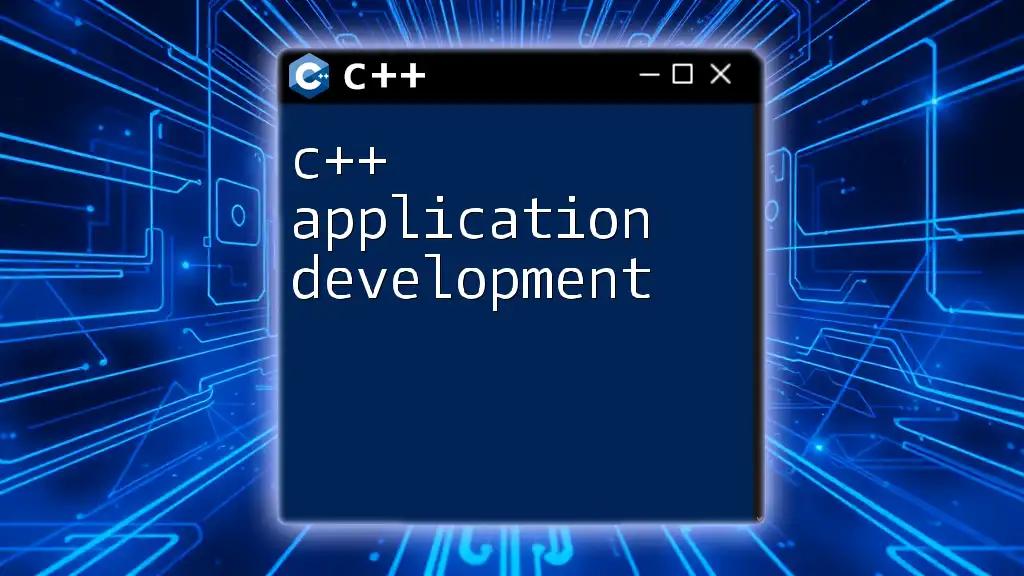
Handling Edge Cases
Dealing with Undefined Input
When working with user input, it is imperative to address undefined or unexpected input types gracefully. Implementing suggestions for user feedback can significantly improve the user experience. Feedback should be clear and informative to guide the user effectively.
Techniques for Robust Input Validation
C++ allows the use of Regular Expressions (regex) to facilitate complex input validation scenarios, like email address validation. The following example demonstrates how to utilize regex for this purpose:
#include <iostream>
#include <regex>
using namespace std;
bool isValidEmail(const string &email) {
regex emailPattern(R"((\w+)(\.{0,1}\w*)*@\w+(\.\w+)+)");
return regex_match(email, emailPattern);
}
int main() {
string email;
cout << "Enter your email: ";
cin >> email;
if (isValidEmail(email)) {
cout << "Valid email!" << endl;
} else {
cout << "Invalid email!" << endl;
}
return 0;
}
In the email validation example, the regex pattern checks the format of the provided email address. The program returns feedback to the user based on whether the input matches the expected format.
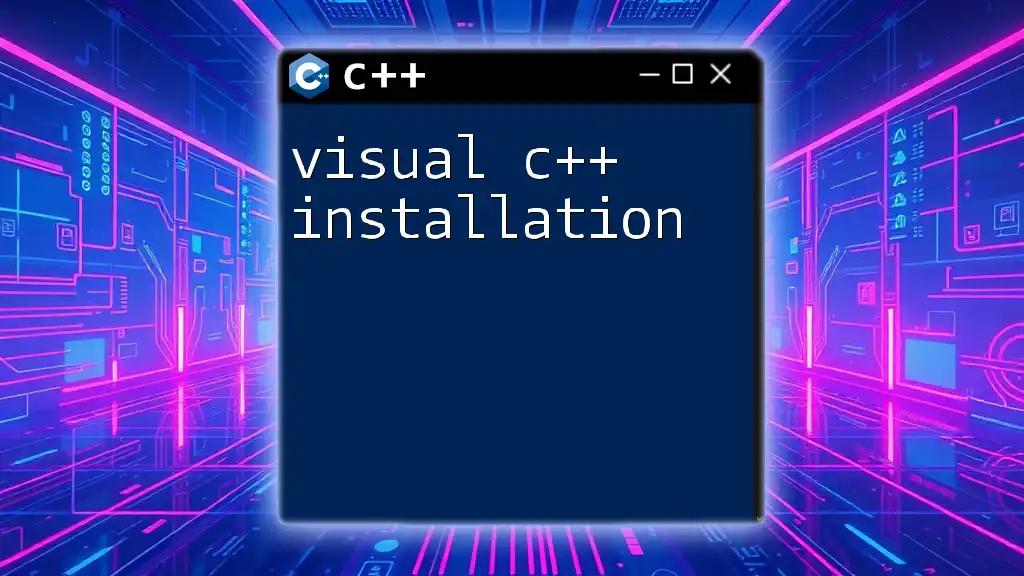
Best Practices for Input Validation in C++
Ensuring User-Friendly Prompts
Clear and concise prompts are vital for effective input validation. Providing obvious instructions can prevent user errors and ensure the data collected is as expected. Consider avoiding technical jargon and using simple language to communicate the input requirements.
Structuring Your Code for Clear Input Validation
To maintain a well-organized codebase, it is essential to adopt practices such as the Single Responsibility Principle. Each function or module in your code should have a single purpose, making it easier to read and maintain. By applying this principle, your project’s validation logic will remain consistent and scalable.
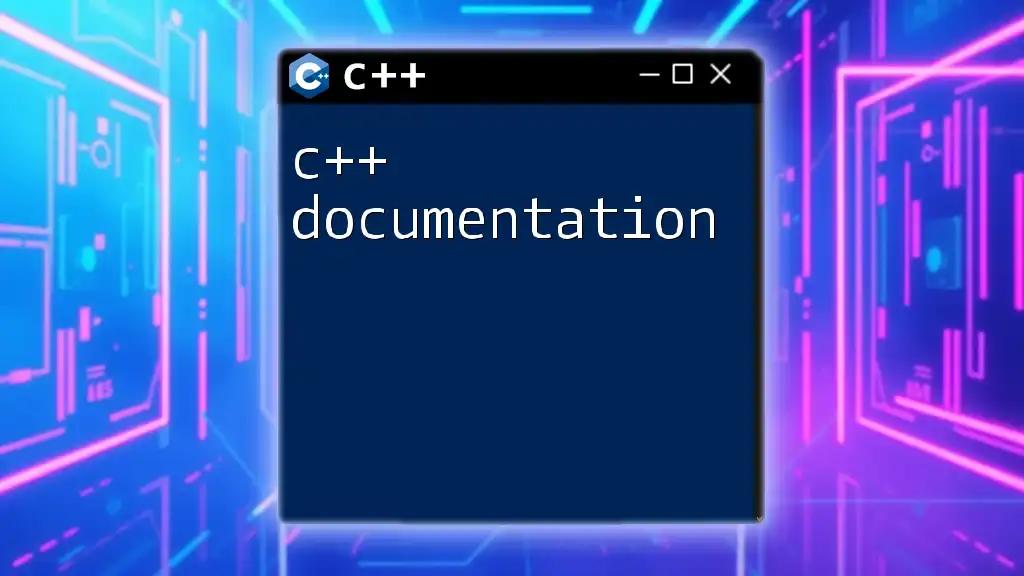
Conclusion
C++ input validation is a fundamental aspect of robust programming that enhances security and user experience. By applying techniques such as type checking, function-based validation, range checks, and regex pattern matching, developers can effectively manage user inputs. By emphasizing input validation, programmers contribute to a more resilient application that can withstand user errors and protect against potential vulnerabilities.