In C++, a map can be initialized using an initializer list to create key-value pairs efficiently.
Here’s a code snippet demonstrating map initialization:
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {
{1, "One"},
{2, "Two"},
{3, "Three"}
};
for (const auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
What is a Map in C++?
A map in C++ is a part of the Standard Template Library (STL) that implements a sorted associative container. It stores elements in key-value pairs, where each key is unique. This unique key allows efficient retrieval of values, making maps an essential data structure in many programming scenarios.
Maps differ from other STL containers, such as vectors or lists, in that they are sorted by keys and provide logarithmic time complexity for key-based operations. This key-value association is particularly useful in scenarios where searching for a specific key and accessing its value is required frequently.
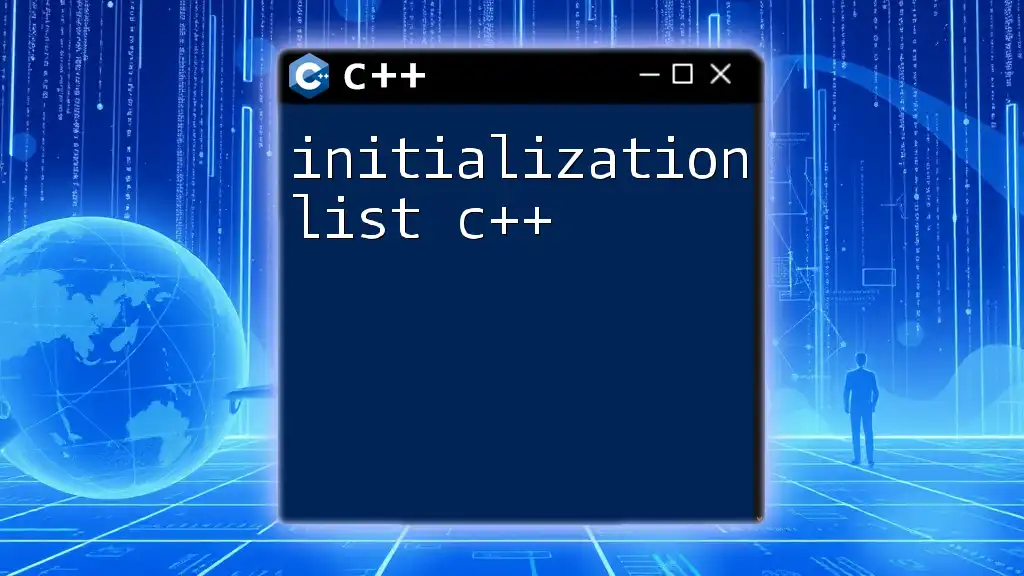
Why Initialize a Map?
Initialization of a map is crucial in C++ programming because it ensures that the map is in a valid state before being used. An uninitialized map can lead to undefined behavior and result in runtime errors. Different initialization methods provide flexibility depending on the requirements, such as performance considerations, readability, and the need to use complex types.
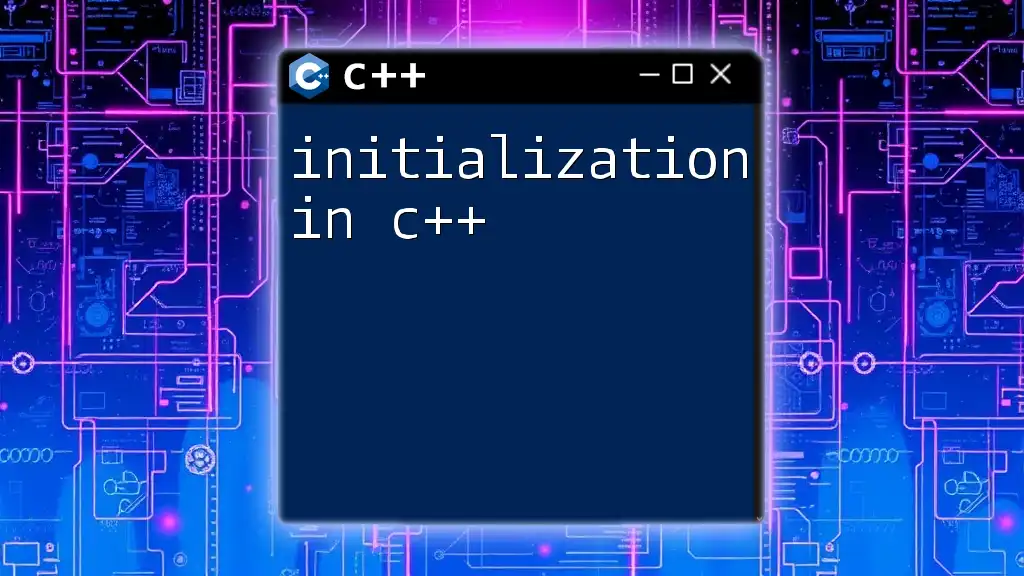
Types of Map Initialization in C++
Default Initialization
When initializing a map without any specific values, it is simply declared as follows:
std::map<int, std::string> myMap;
This statement creates an empty map named `myMap`, which is ready to store integer keys and string values. At this stage, the map contains no elements, and its size is zero. This initialization is useful when the keys and values will be inserted later in the program.
Initializing with Specific Values
To initialize a map with predefined key-value pairs, you can use the following syntax:
std::map<int, std::string> myMap = {{1, "One"}, {2, "Two"}, {3, "Three"}};
This concise syntax allows direct initialization of the map with a set of key-value pairs. Each pair consists of a key (an integer) and its corresponding value (a string). The map is populated immediately upon declaration, providing an efficient way to set up the data structure.
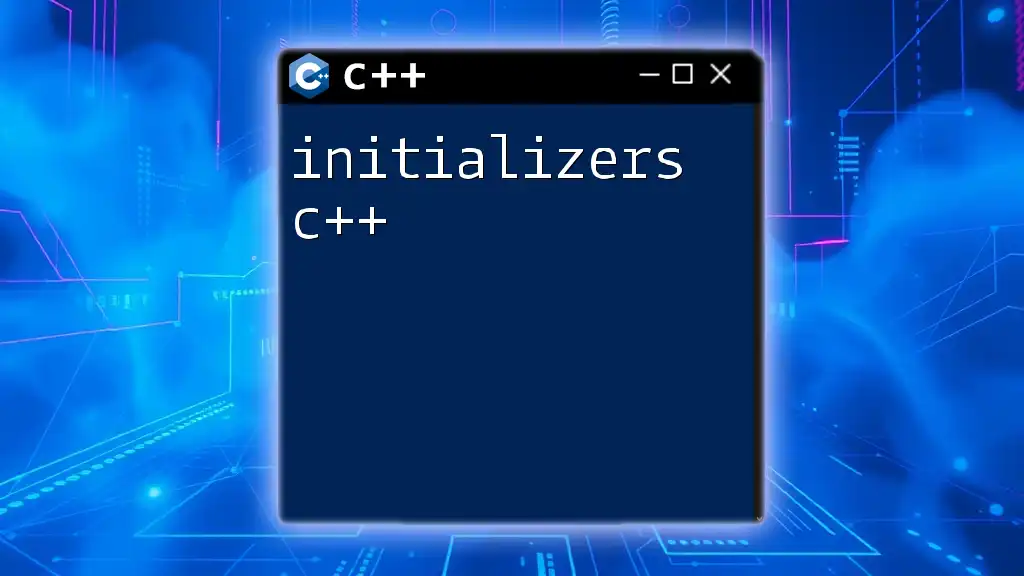
Using C++11 Features for Map Initialization
Using `std::initializer_list`
C++11 introduced the `std::initializer_list`, which simplifies the initialization of containers, including maps. The following code demonstrates this feature:
std::map<int, std::string> myMap{{1, "One"}, {2, "Two"}, {3, "Three"}};
Using `std::initializer_list`, you can initialize the map directly with key-value pairs enclosed in double braces. This method enhances code readability and allows immediate assignment of values during the map's creation.
Using `std::make_pair`
An alternative way to initialize a map involves using the `std::make_pair` function. Consider the following example:
#include <utility>
std::map<int, std::string> myMap = {std::make_pair(1, "One"), std::make_pair(2, "Two")};
The `std::make_pair` function creates pairs of the specified types, making it clear when keys and values of different types are involved. While this method is valid, it is generally more verbose than using the initializer list and might be less preferred for simple key-value pairs.
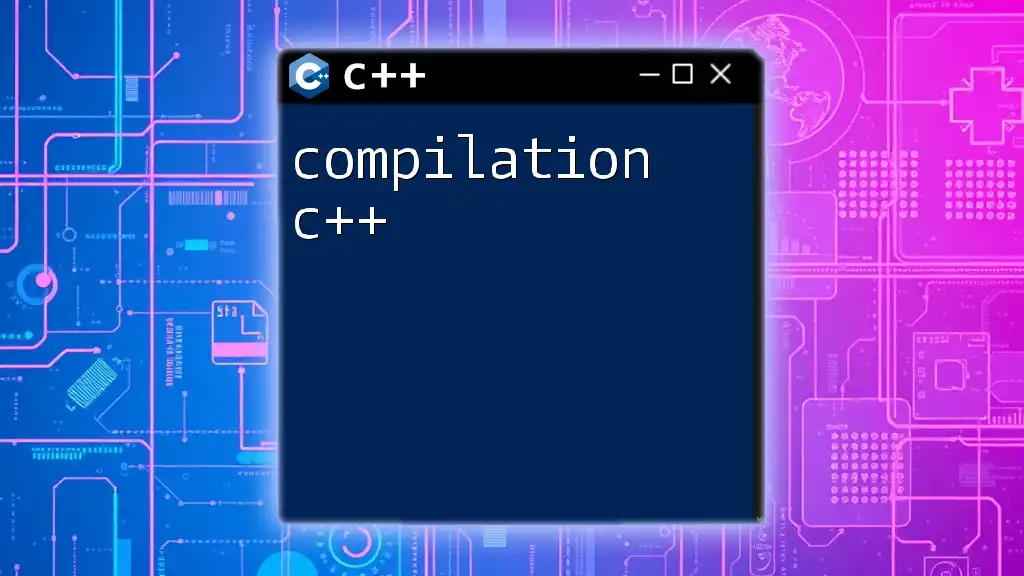
Advanced Map Initialization Techniques
Initializing a Map with Iterators
Maps can also be initialized using iterators, which is especially useful for initializing from other containers, such as vectors. Here’s how you can use this technique:
std::vector<std::pair<int, std::string>> vec{{1, "One"}, {2, "Two"}};
std::map<int, std::string> myMap(vec.begin(), vec.end());
In this example, `vec` is a vector of pairs, and the map is initialized by passing the start and end iterators of the vector to the map's constructor. This method is efficient when transforming one container into another.
Using Constructors for Custom Types
Initializing a map to include custom structs is highly effective for representing homegrown data types. Here’s a structure and its initialization:
struct Person {
int age;
std::string name;
};
std::map<int, Person> people;
This creates a map where each integer key uniquely identifies a `Person`. An example of using this initialization would be:
std::map<int, Person> people = {
{1, {25, "Alice"}},
{2, {30, "Bob"}}
};
In this case, we have a map that effectively uses integers as keys and structs as values. This helps to encapsulate complex types within the map while maintaining the benefits of quick access via unique keys.
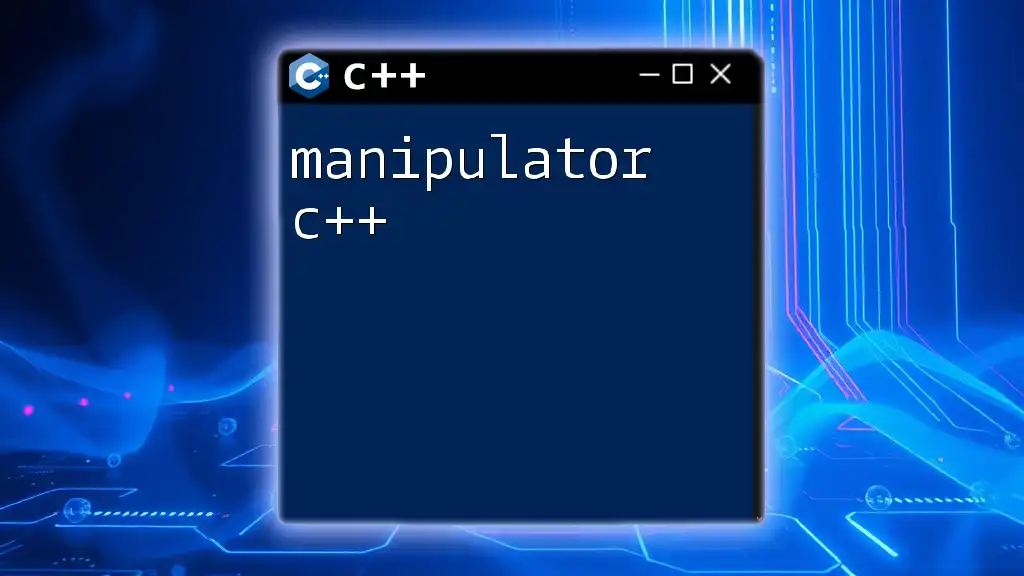
Comparing Different Initialization Methods
Performance Considerations
Performance is a key aspect when choosing an initialization method. While the default initializer can take time if populated later, initializing with a specific value or through iterators can save on performance costs by avoiding multiple insertions. It's crucial to analyze the requirements when deciding the best method.
Code Readability and Maintenance
Using modern C++ features like `std::initializer_list` can greatly enhance code readability. A readable syntax helps maintainability, making it easier for others (or yourself in the future) to understand the code written today.
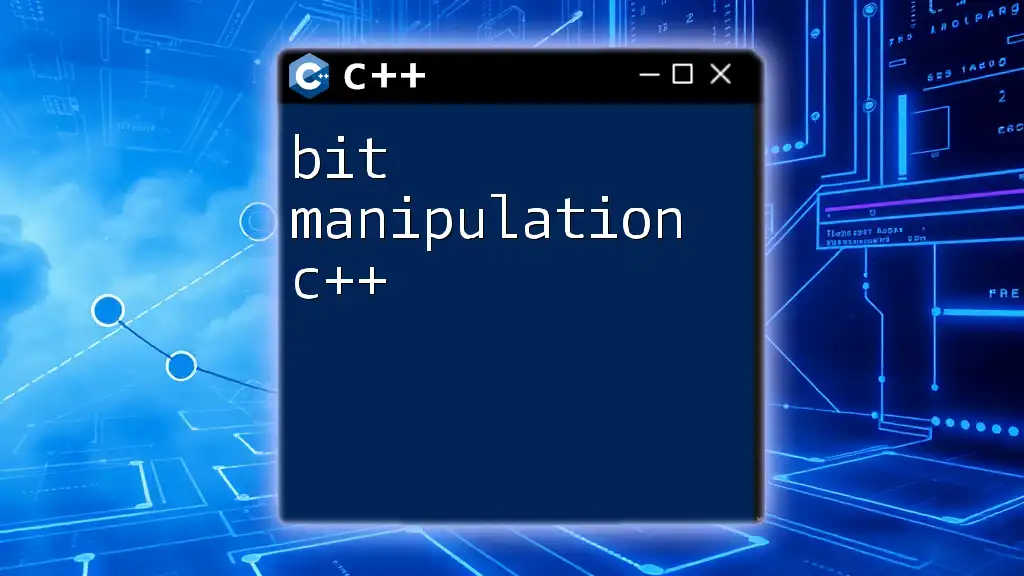
Common Pitfalls in Map Initialization
Forgetting to Include Required Libraries
When working with maps, it’s easy to forget the necessary header files. Ensure you include the required headers, like `<map>` and `<string>`, for successful compilation.
Misunderstanding Key Uniqueness
A map enforces key uniqueness, meaning if you insert a duplicate key, only the latest inserted value will be kept. If your code relies on storing multiple values for the same key, consider using other containers like `std::multimap`.
Handling Memory in Maps
Maps internally manage memory for their elements, but understanding how this works can help you optimize performance. Be mindful of the types you store and watch for unnecessary copies when inserting or updating values.
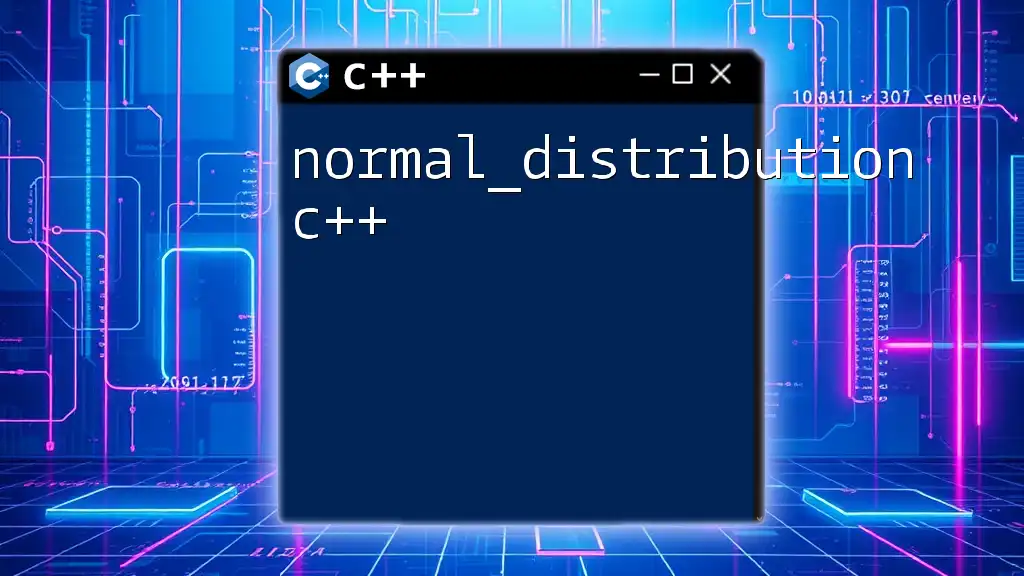
Conclusion
Understanding the intricacies of map initialization in C++ grants developers the ability to utilize this powerful data structure effectively. Whether through default initialization, specific value assignment, or advanced techniques like iterator conversion and custom struct handling, each method has its use cases and best practices. Exploring these avenues not only makes code cleaner and more efficient but also enhances performance and maintainability. Embrace the versatility of maps and experiment with these initialization techniques to see how they best fit your programming needs.
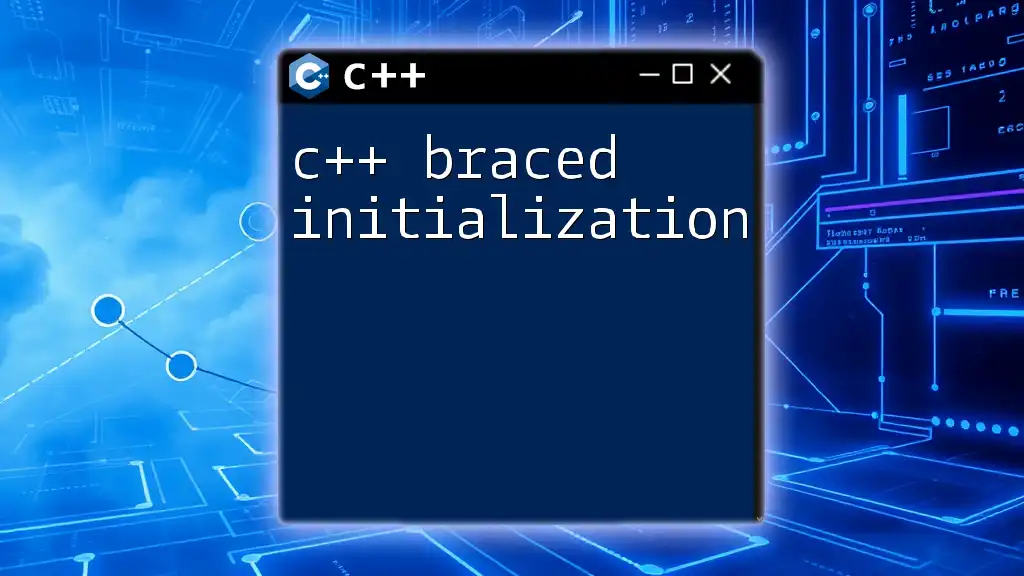
References and Further Reading
For further learning, consider exploring the official C++ documentation on maps, along with recommended tutorials and books tailored to deepen your understanding of STL containers and their usage.