Bit manipulation in C++ involves using bitwise operators to perform operations on individual bits of binary numbers for efficient data processing and representation. Here’s a simple example that demonstrates flipping a specific bit using the XOR operator:
#include <iostream>
int main() {
int number = 5; // Binary: 0101
int bit_to_flip = 1; // We want to flip the 1st bit (0-indexed)
number ^= (1 << bit_to_flip); // Flip the bit
std::cout << "Result after flipping: " << number << std::endl; // Output: 7 (Binary: 0111)
return 0;
}
Introduction to Bit Manipulation
Understanding Bits and Bytes
In computing, bits are the most fundamental unit of data, represented as either 0 or 1. A group of 8 bits forms a byte, which can represent a variety of data types. Understanding how bits and bytes work is crucial, as even high-level programming languages like C++ inevitably translate data into binary for processing by a computer.
The essence of bit manipulation lies in the control of these bits. By leveraging low-level operations on binary data, programmers can efficiently solve complex problems, optimize performance, and reduce memory usage.
Why Use Bit Manipulation in C++?
Bit manipulation in C++ provides several advantages:
- Performance Benefits: Operations on bits are generally faster than arithmetic operations due to lower computational demands.
- Space Efficiency: Storing flag states and boolean variables within a single byte or integer saves memory.
- Common Applications: Bit manipulation is pivotal in algorithms, graphics, data compression, and cryptography, making it a valuable skill for every C++ programmer.
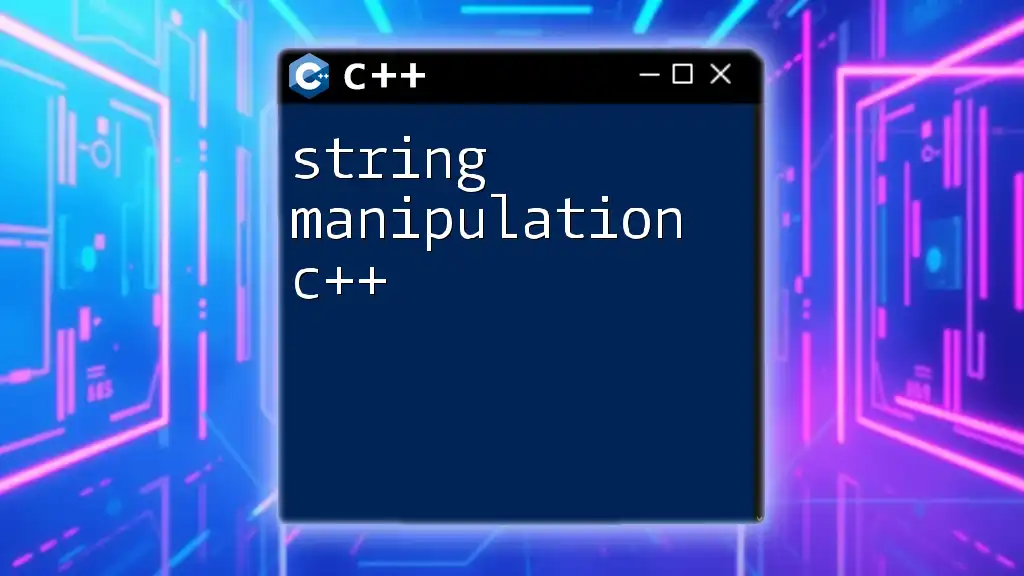
Basic Bit Manipulation Operations
Bitwise Operators Overview
C++ provides a set of operators that allow you to manipulate bits directly. The primary bitwise operators include:
- AND (`&`): Compares each bit of two integers and returns a new integer with bits set only where both bits are 1.
- OR (`|`): Sets bits to 1 if at least one of the corresponding bits is 1.
- XOR (`^`): Sets bits to 1 if the corresponding bits are different.
- NOT (`~`): Inverts all bits (0s become 1s and vice versa).
- Shift Left (`<<`): Moves bits to the left, effectively multiplying the number by powers of two.
- Shift Right (`>>`): Moves bits to the right, effectively dividing the number by powers of two.
Examples of Basic Bitwise Operations
Bitwise AND
The bitwise AND operator is commonly used for masking bits. For instance:
int a = 12; // Binary: 1100
int b = 10; // Binary: 1010
int result = a & b; // Binary: 1000 => Decimal: 8
Here, the result of `a & b` is 8 because only the third bit (from the right) is set in both numbers.
Bitwise OR
The OR operator allows you to set specific bits:
int a = 12; // Binary: 1100
int b = 10; // Binary: 1010
int result = a | b; // Binary: 1110 => Decimal: 14
The OR operation results in 14 since it sets the bits where either `a` or `b` has a 1.
Bitwise XOR
The XOR operator is often used to toggle bits:
int a = 12; // Binary: 1100
int b = 10; // Binary: 1010
int result = a ^ b; // Binary: 0110 => Decimal: 6
The result is 6 as bits toggle only where the bits of `a` and `b` differ.
Bitwise NOT
Inverting bits can be accomplished with NOT:
int a = 12; // Binary: 0000 1100
int result = ~a; // Binary: 1111 0011 => Decimal: -13 (two's complement)
The result is -13, showcasing how the NOT operator flips every bit.
Left Shift and Right Shift
Shifting bits can multiply or divide numbers by two:
int a = 5; // Binary: 0101
int leftShift = a << 1; // Binary: 1010 => Decimal: 10
int rightShift = a >> 1; // Binary: 0010 => Decimal: 2
Here, left-shifting `5` results in `10`, while right-shifting gives `2`.
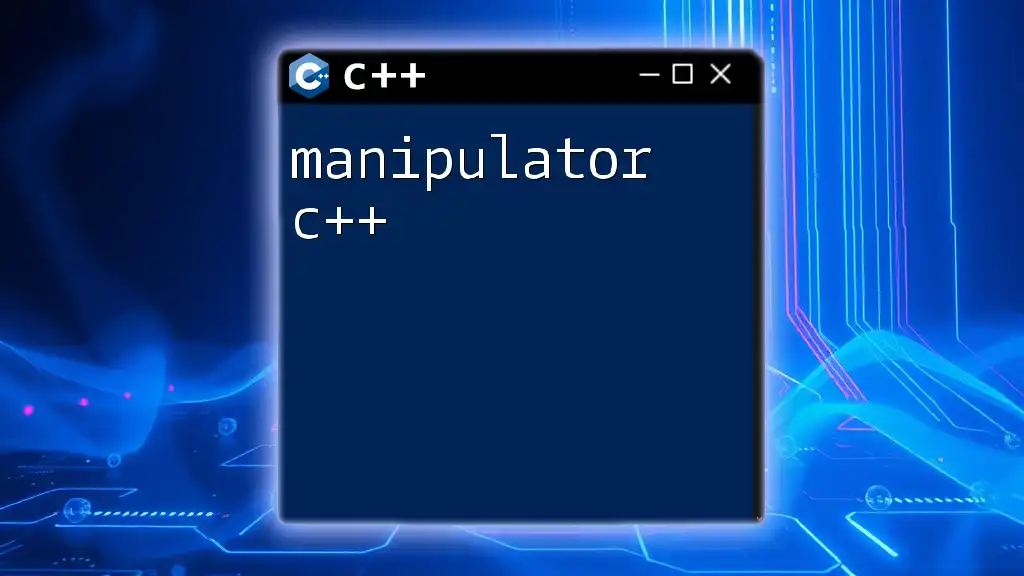
Advanced Bit Manipulation Techniques
Swapping Bits
Swapping Two Variables
You can swap two variables without using a temporary variable via XOR:
int a = 5; // Binary: 0101
int b = 3; // Binary: 0011
a = a ^ b; // Step 1: a = 0101 ^ 0011 => 0110
b = a ^ b; // Step 2: b = 0110 ^ 0011 => 0101 (b is now 5)
a = a ^ b; // Step 3: a = 0110 ^ 0101 => 0011 (a is now 3)
This method is elegant and eliminates the need for temporary storage.
Counting Set Bits
Knowing how many bits are set (i.e., equal to 1) is vital in various applications. Here are two methods:
Method 1: Loop Method
int countSetBits(int n) {
int count = 0;
while (n) {
count += n & 1; // Increment count if the last bit is 1
n >>= 1; // Right shift n
}
return count;
}
Method 2: Brian Kernighan's Algorithm
This algorithm is more efficient:
int countSetBits(int n) {
int count = 0;
while (n) {
n &= (n - 1); // Removes the least significant bit set
count++;
}
return count;
}
This approach continues until no bits are left set.
Checking If a Number is a Power of Two
A number is a power of two if it has exactly one bit set. You can check this condition with:
bool isPowerOfTwo(int n) {
return (n > 0) && ((n & (n - 1)) == 0);
}
This concise method utilizes bitwise operations to check for a single set bit.
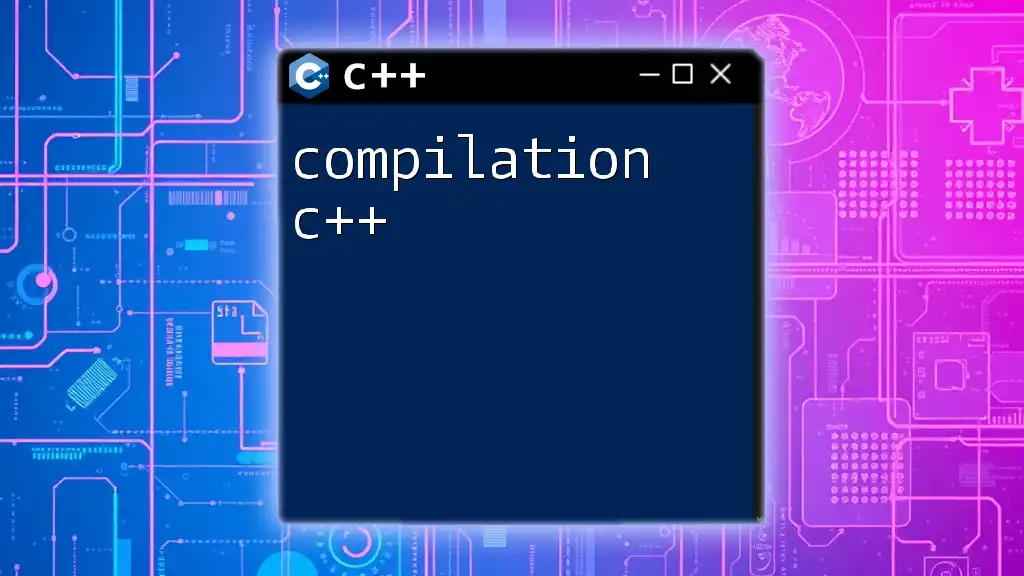
Common Bit Manipulation Patterns
Single Bit Manipulation
Checking a Bit
To check whether a specific bit position (say, 3) is set, use:
if (n & (1 << 3)) {
// Bit at position 3 is set
}
This code snippet shifts `1` left by `3` positions and checks if the result intersects with `n`.
Setting a Bit
To set the 3rd bit of a number:
n |= (1 << 3); // Now the 3rd bit of n is set to 1
This effectively modifies `n`, ensuring the designated bit is turned on.
Clearing a Bit
To clear (set to 0) the 3rd bit:
n &= ~(1 << 3); // Clears the 3rd bit
This snippet first generates a mask that has a `0` at the specified position and then uses AND to set it to `0`.
Multiple Bit Manipulation
Extracting Bits
To get a range of bits from an integer, you can use bit masking:
int extractBits(int n, int start, int count) {
return (n >> start) & ((1 << count) - 1);
}
This shifts the number right and applies a mask to extract the desired bits.
Reversing Bits
For reversing the bits of an integer, the following code snippet does the trick:
unsigned int reverseBits(unsigned int n) {
unsigned int result = 0;
for (int i = 0; i < 32; i++) {
result <<= 1; // Shift result left by 1
result |= (n & 1); // Add the least-significant bit of n to result
n >>= 1; // Shift n right by 1
}
return result;
}
This example iterates through each bit to construct the reversed integer.
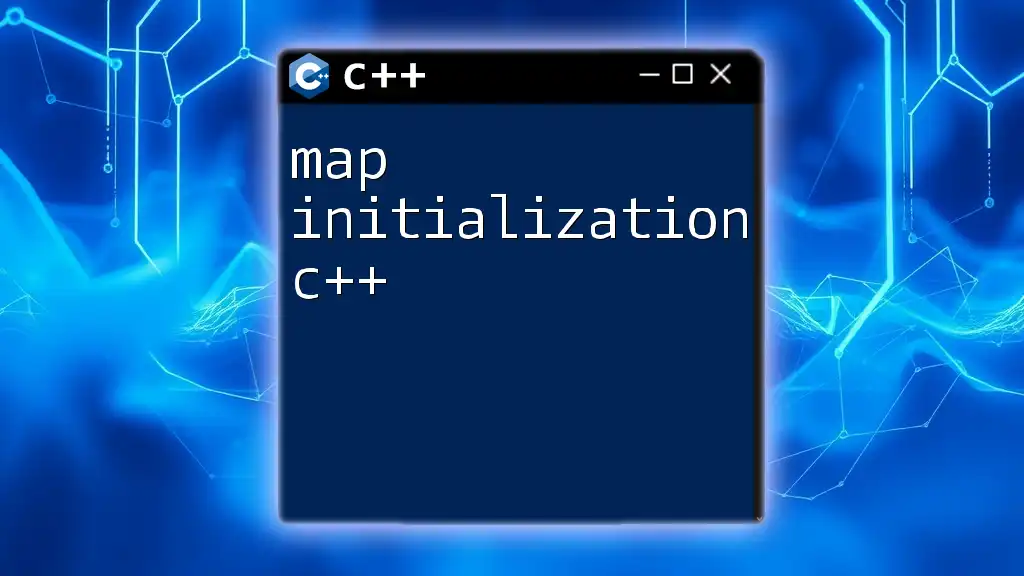
Practical Applications of Bit Manipulation
Graphics and Image Processing
Bit manipulation is crucial in graphics programming, where you can manipulate pixel data stored in integers. By manipulating bits, you can quickly adjust colors, transparency levels, and other properties of pixels—ensuring fast rendering without heavy overhead.
Cryptography
In the realm of cryptography, bit manipulation is used extensively. Operations such as XOR are foundational for creating algorithms that ensure data confidentiality and integrity. For instance, XOR can be used to create stream ciphers where data is combined with a key to produce ciphertext.
Game Development
Bit manipulation is frequently applied in game development for state management. By packing multiple boolean flags into a single integer, developers can efficiently manage a wide range of properties without consuming additional memory.
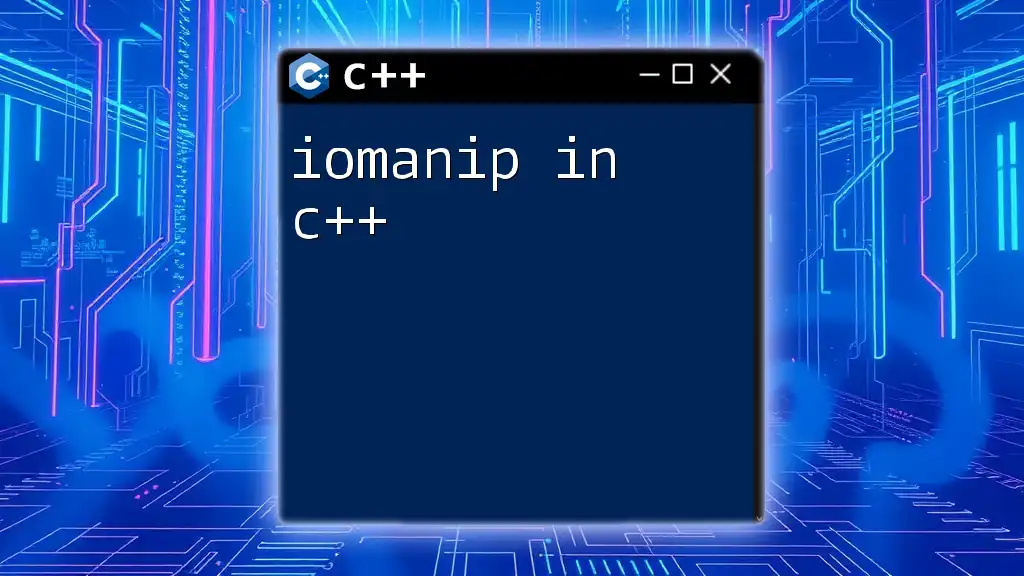
Best Practices and Common Mistakes
Efficient Use of Bit Manipulation
When utilizing bit manipulation, aim for clarity and maintainability. While bit manipulation can enhance performance, overly complex operations can lead to confusion. Keep your code readable and document your intentions clearly.
Debugging Bit Manipulation Code
Debugging bit manipulation can be tricky due to the low-level nature of the operations. Utilize simple debug statements to print intermediate values of variables after bitwise operations to track behavior effectively. Familiarize yourself with tools that can visualize binary representations for easier debugging.
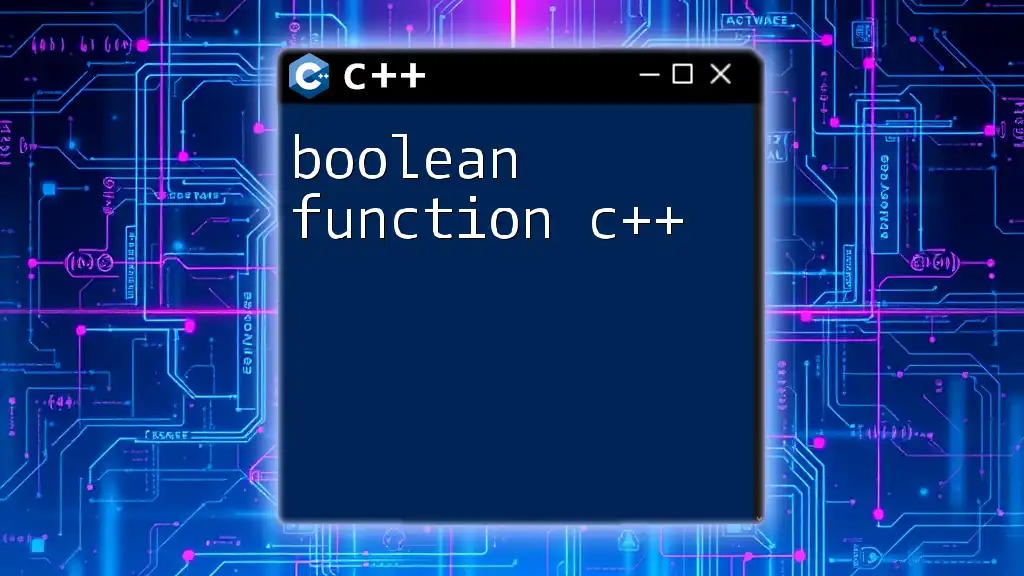
Conclusion
Mastering bit manipulation in C++ is a powerful tool that enhances your programming arsenal. From simple tasks like checking bits to complex applications in graphics and cryptography, understanding how to manipulate bits efficiently can set you apart as a developer. Embrace these techniques and integrate them into your coding habits for improved performance and lower memory usage.
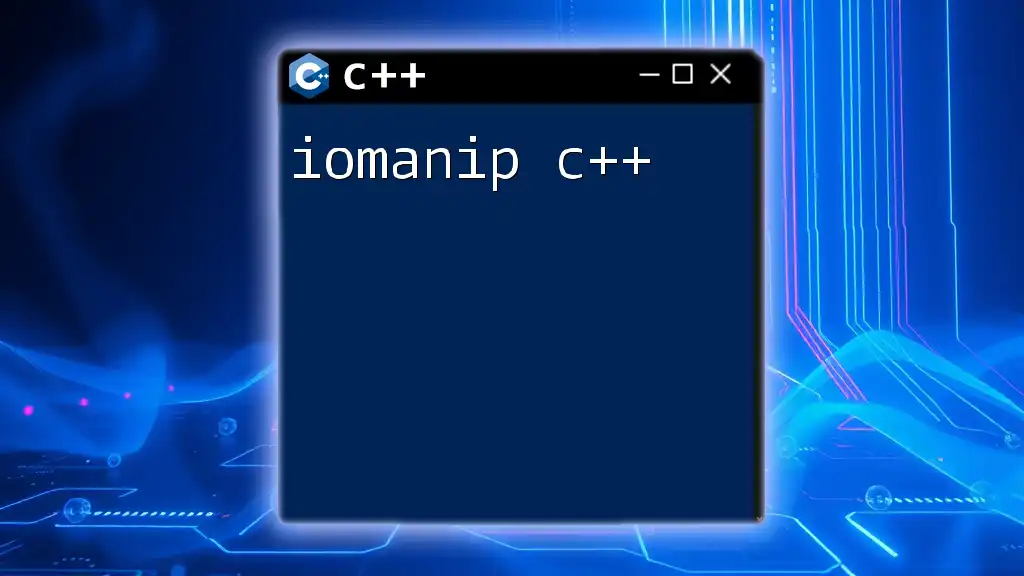
Additional Resources
Recommended Online Tutorials and Courses
Investigate platforms like Codecademy, Coursera, and Udemy for courses focused on C++ and algorithms related to bit manipulation.
Books on Algorithms and C++
Books like "Introduction to Algorithms" by Cormen et al. and "The C++ Programming Language" by Bjarne Stroustrup are excellent resources for deeper dives into efficient programming practices, including bit manipulation.
Community and Forums for Learning
Join developer communities on platforms like Stack Overflow, Reddit, and GitHub to engage in discussions, ask questions, and share your learning journey on bit manipulation in C++.