The `<iomanip>` library in C++ is used to manipulate the output format of data, allowing you to set options like width, precision, and alignment for better readability.
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::setw(10) << std::left << "Name"
<< std::setw(10) << std::right << "Score" << std::endl;
std::cout << std::setw(10) << std::left << "Alice"
<< std::setw(10) << std::right << 95 << std::endl;
return 0;
}
What is iomanip in C++?
`iomanip` (input/output manipulators) is a header file in C++ that provides a collection of functions and objects to control the formatting of input and output using streams. By adjusting how data is presented, `iomanip` enables programmers to create outputs that are not only functional but also visually appealing. This is particularly significant in applications where readability and clarity are paramount, such as displaying financial information, scientific data, or tables.
Historical Context
Initially introduced as part of the C++ standard library, `iomanip` has evolved alongside the language itself. Its functionality addresses a common need in programming: the efficient and structured formatting of data to enhance user experience.
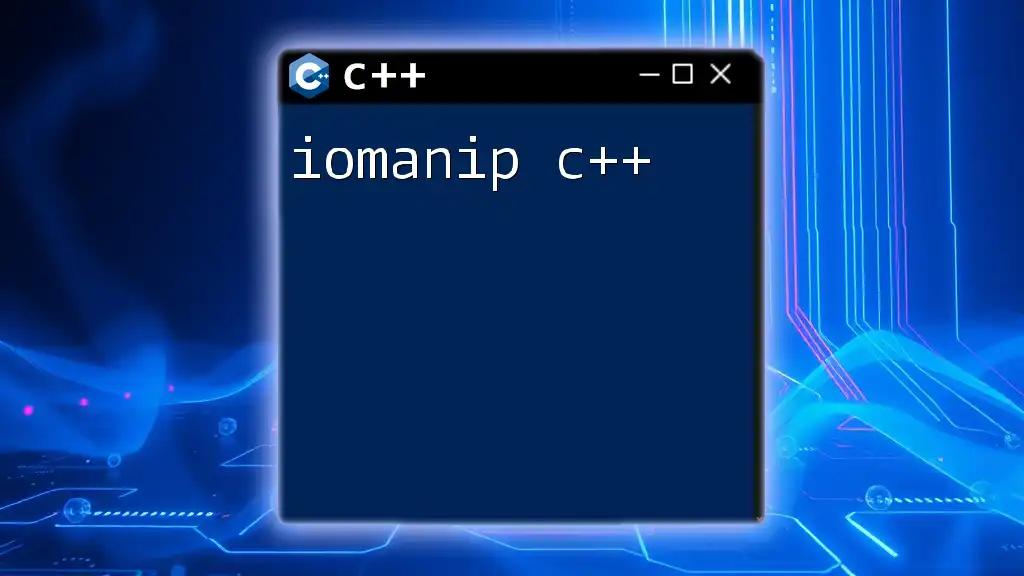
Common Uses of iomanip
Using `iomanip` is essential for developers who seek to display data in an organized and easily interpretable manner. Here are a few scenarios where `iomanip` shines:
- Financial Applications: Precise presentation of currency values.
- Scientific Computing: Clear display of numbers with significant figures.
- Tabular Data: Well-formatted tables for enhanced readability.
As a rule of thumb, if your output can benefit from better alignment, precision, or aesthetics, consider using `iomanip`.
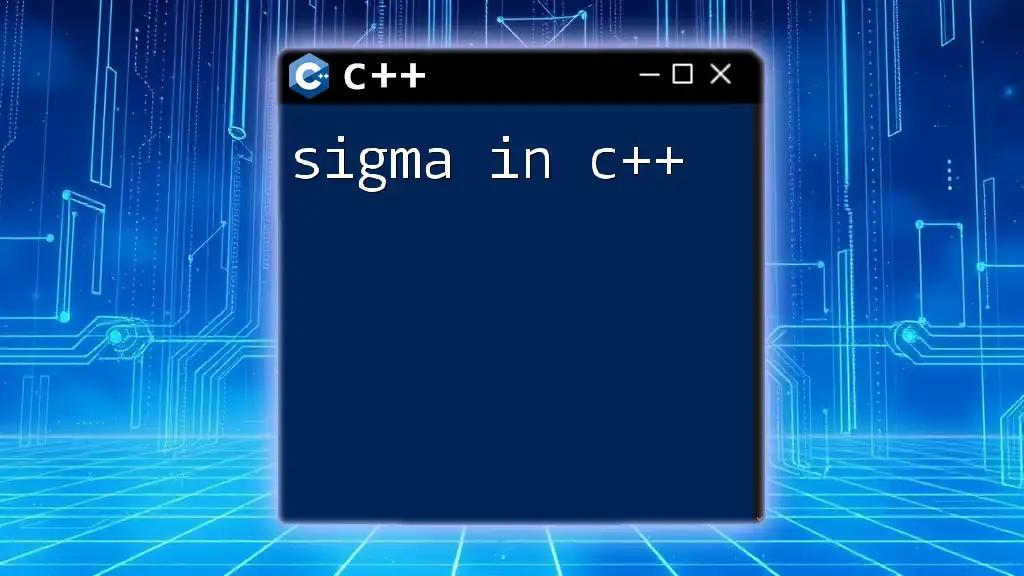
Key Functions in iomanip
Setting Precision
The function `std::setprecision` allows you to specify the number of digits displayed for floating-point numbers. This is particularly useful in situations requiring accuracy.
Code Example:
#include <iostream>
#include <iomanip>
int main() {
double pi = 3.141592653589793;
std::cout << std::setprecision(5) << pi << std::endl; // Output: 3.1416
return 0;
}
Explanation: The output rounds the value of π to four decimal places, demonstrating how you can control precision easily. This is especially critical in scientific applications where precise calculations matter.
Setting Width
Using `std::setw` allows you to define the width of the output field. This ensures that outputs are aligned properly, creating an organized structure.
Code Example:
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::setw(10) << "Hello" << std::endl; // Output: " Hello"
return 0;
}
Explanation: In this example, the keyword "Hello" is right-aligned within a width of 10 characters. Properly setting widths helps to build clean, tabular-like visuals.
Filling Characters
The `std::setfill` function lets you define a specific character to fill empty spaces in the output. This can be handy when formatting numbers or text neatly within given dimensions.
Code Example:
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::setfill('*') << std::setw(10) << 42 << std::endl; // Output: "*******42"
return 0;
}
Explanation: Here, the output fills idle spaces with asterisks, showcasing how to use fill characters effectively. This is useful when you want to visually highlight specific outputs.
Number Format
The manipulators `std::fixed` and `std::scientific` determine how floating-point numbers are displayed.
Code Example for std::fixed:
#include <iostream>
#include <iomanip>
int main() {
double num = 1234.56789;
std::cout << std::fixed << std::setprecision(2) << num << std::endl; // Output: 1234.57
return 0;
}
Explanation: This example uses `std::fixed`, which formats the number to two decimal points. This is essential in scenarios like financial reporting.
Code Example for std::scientific:
#include <iostream>
#include <iomanip>
int main() {
double num = 1234.56789;
std::cout << std::scientific << num << std::endl; // Output: 1.234568e+03
return 0;
}
Explanation: The output is transformed into scientific notation, which is incredibly useful for dealing with very large or small numbers, ensuring clarity for the viewer.
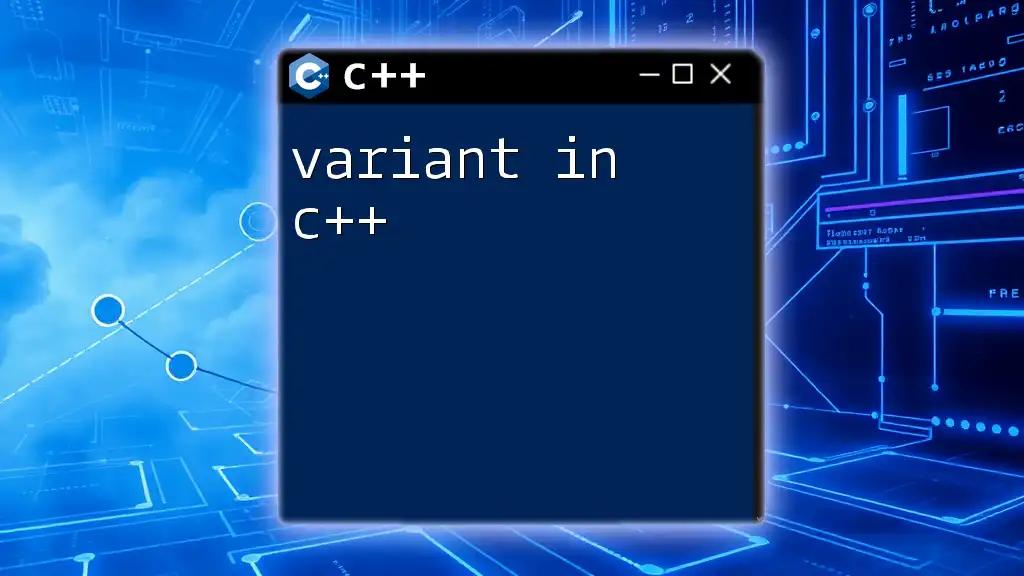
Advanced Formatting Techniques
One of the unique strengths of `iomanip` is the ability to combine multiple manipulators for more comprehensive formatting.
Code Example:
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::setfill('0') << std::setw(5) << 42 << std::endl; // Output: "00042"
std::cout << std::setprecision(3) << std::fixed << 3.14159 << std::endl; // Output: "3.142"
return 0;
}
Explanation: This example shows the combination of zero-filling and setting precision, leading to flexible and appealing outputs. Knowing how to merge different manipulators allows developers to craft outputs that meet various presentation requirements seamlessly.
Manipulating Input Streams
While `iomanip` is predominantly used for output stream formatting, it can also be effectively utilized with input streams. For example, you can control how numbers are read into your program, ensuring they reflect the same formatting conventions expected.
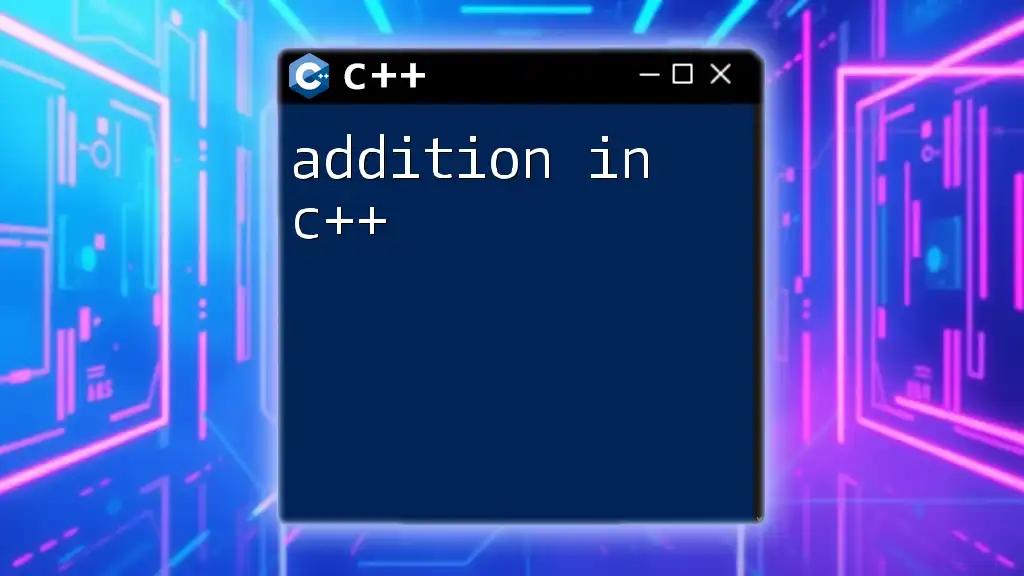
Practical Examples
Creating a structured table of values provides a compelling demonstration of the powers of `iomanip`. Organizing data into rows and columns enhances readability and professional appearance.
Code Example:
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::left << std::setw(10) << "Item" << std::setw(10) << "Price" << std::endl;
std::cout << std::setw(10) << "Apple" << std::setw(10) << "$0.99" << std::endl;
std::cout << std::setw(10) << "Banana" << std::setw(10) << "$0.50" << std::endl;
return 0;
}
Explanation: In the table above, the headers and items are neatly aligned, demonstrating how `iomanip` can create well-structured displays. In practical applications, this makes it easier for users to digest information quickly, leading to a better user experience.
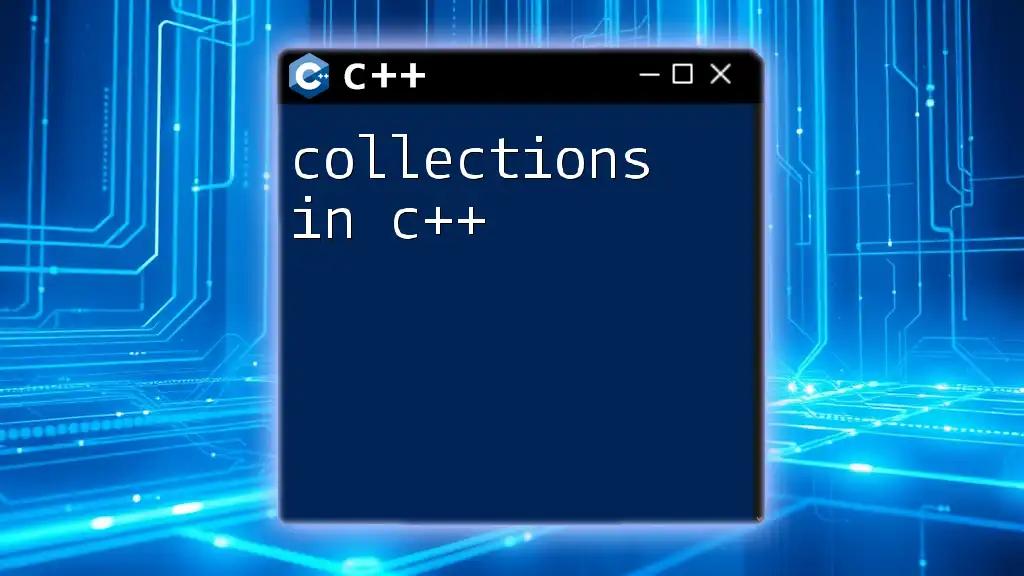
Conclusion
Throughout this guide, we have explored the significant functions and capabilities of `iomanip` in C++. By applying these tools, developers can manipulate the formatting of output streams, enhancing the clarity and presentation of their data.
We encourage you to experiment with `iomanip` in your projects, as hands-on experience will significantly deepen your understanding.
With these newly gained insights, you're well-equipped to elevate your C++ programming skills. Consider diving deeper into the topic with additional resources or by practicing with more complex datasets. Happy coding!