In C++, the `isnum` function is not a standard function, but you can check if a string represents a numeric value using `std::all_of` along with `std::isdigit` from the `<cctype>` library.
Here's a code snippet to demonstrate this:
#include <iostream>
#include <string>
#include <cctype>
#include <algorithm>
bool isnum(const std::string& str) {
return !str.empty() && std::all_of(str.begin(), str.end(), ::isdigit);
}
int main() {
std::string input = "12345";
if (isnum(input)) {
std::cout << input << " is a number." << std::endl;
} else {
std::cout << input << " is not a number." << std::endl;
}
return 0;
}
What is isnum in C++?
The `isnum` function in C++ is an essential tool for validating whether a given input is numeric. This validation is crucial in programming as it ensures that the data entered by users meets the expected format, thus preventing errors and unintended behavior in applications. By using `isnum`, programmers can efficiently filter and manage user inputs, making their programs more robust and user-friendly.
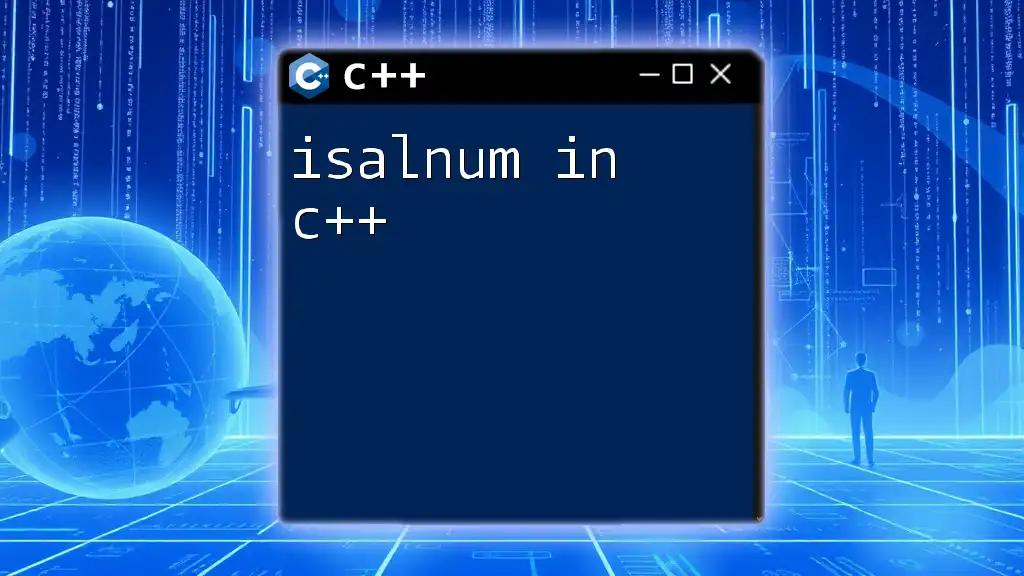
The Relationship Between `isnum` and C++ Data Types
Understanding Data Types in C++
C++ is a statically typed programming language, meaning that every variable must be explicitly defined with a specific data type before it can be used. Basic data types in C++ include:
- `int`: Represents integer values.
- `float`: Represents single-precision floating-point numbers.
- `double`: Represents double-precision floating-point numbers.
Choosing the correct data type is critical for efficient memory usage and performance. When processing user input, it’s crucial to be confident that the data can be successfully converted into the specified type.
How `isnum` Fits In
The primary role of `isnum` is to assess whether a given value is numeric. This function aids in distinguishing between numeric and non-numeric input, which is especially beneficial when accepting user inputs. By implementing `isnum`, programmers can significantly reduce the chances of runtime errors caused by unexpected input values.

Using `isnum` in Practice
Syntax and Basic Usage
The syntax of the `isnum` function is straightforward. Below is a simple implementation of `isnum`, designed to validate string inputs for numeric values.
#include <iostream>
#include <string>
bool isnum(const std::string& str) {
for (char const &c : str) {
if (std::isdigit(c) == 0) return false;
}
return true;
}
int main() {
std::string input;
std::cout << "Enter a number: ";
std::cin >> input;
if (isnum(input)) {
std::cout << "Valid number!" << std::endl;
} else {
std::cout << "Invalid input. Please enter a number." << std::endl;
}
return 0;
}
In the code above, `isnum` iterates through each character in the input string and checks if it represents a digit. If all characters are digits, it returns true; otherwise, it returns false.
Common Scenarios for Using `isnum`
- User Input Validation: An application that requires numeric inputs, such as calculators or mathematical computation tools, must validate input to ensure the user enters only numbers.
- Input Validation in Web Applications: For C++ backend services, ensuring data integrity from user inputs before processing or storage is essential.
- Error Handling: By validating input beforehand, programmers can handle potential exceptions and errors early in the processing phase, making the application more stable.
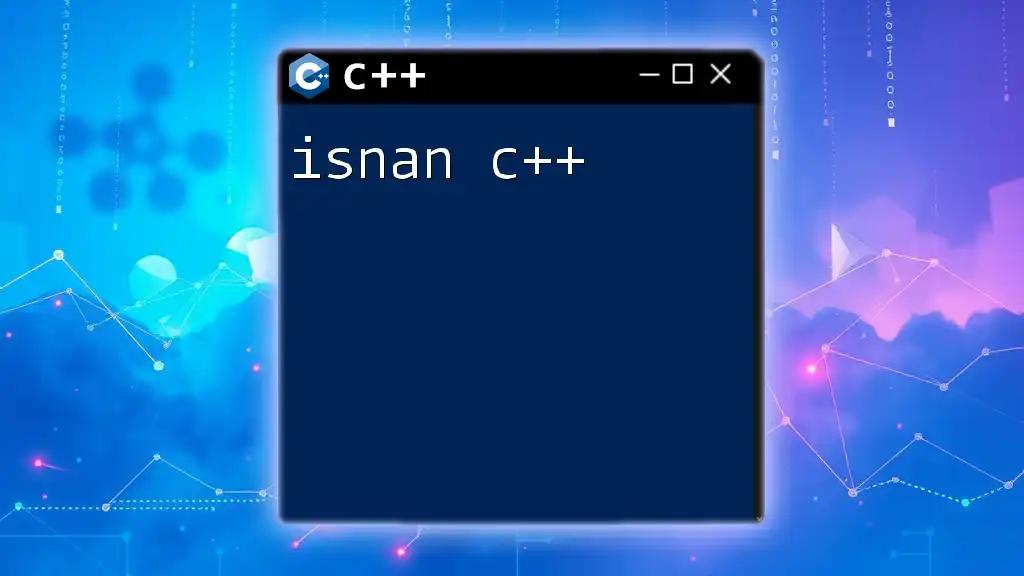
Advanced Usage of `isnum`
Modifying `isnum` for Different Data Types
To further enhance the functionality of `isnum`, it can be modified to handle floating-point numbers. This requires allowing a decimal point in the input. Here is how the enhanced `isnum` might look:
bool isnum(const std::string& str) {
bool decimalPoint = false;
for (char const &c : str) {
if (c == '.') {
if (decimalPoint) return false; // only one decimal point is allowed
decimalPoint = true;
} else if (!std::isdigit(c)) {
return false;
}
}
return true;
}
This updated version provides flexibility to check for both integers and floating-point numbers, making it more versatile for general usage.
Handling Edge Cases and Special Characters
It is important to consider various input cases, such as:
- Negative Numbers: Modify `isnum` to recognize the negative sign (-) at the beginning of the input.
- Empty Strings: Ensure the function returns false for empty inputs, which would not constitute a valid number.
For example, an enhanced version of `isnum` could be created as follows:
bool isnum(const std::string& str) {
if (str.empty()) return false;
bool decimalPoint = false;
bool negativeSign = false;
size_t startIndex = 0;
if (str[0] == '-') {
negativeSign = true;
startIndex = 1; // Start checking after the negative sign
}
for (size_t i = startIndex; i < str.length(); i++) {
char const &c = str[i];
if (c == '.') {
if (decimalPoint) return false; // only one decimal point allowed
decimalPoint = true;
} else if (!std::isdigit(c)) {
return false;
}
}
return true;
}

Best Practices for Input Validation
Importance of Robust Input Handling
Input validation is a crucial part of programming. Neglecting this aspect can lead to severe system failures, data corruption, or security vulnerabilities. By ensuring that inputs are validated, you safeguard your applications from unexpected behaviors.
Tips for Implementing `isnum`
- Modular Code: Keep your `isnum` function modular that allows for easy swapping and testing in different applications.
- Reuse: Implement the function across multiple projects to promote uniformity in input handling.
- Error Messages: Provide clear and concise feedback to users when their input fails validation, helping guide them toward correcting their mistakes.

Conclusion
In summary, understanding how to use `isnum in C++` is an invaluable skill for any programmer. It serves as a powerful mechanism for validating numeric input and helps maintain robustness in applications. By practicing the implementation of `isnum`, developers can ensure their applications can handle user inputs effectively and provide a better overall user experience.
To expand your knowledge further, explore additional resources and documentation to improve your proficiency in C++ programming.