The `is_numeric` function in C++ is used to determine if a given character or string can be classified as a numeric value (i.e., a number).
Here's a code snippet to illustrate its usage:
#include <iostream>
#include <cctype>
bool is_numeric(char c) {
return std::isdigit(c);
}
int main() {
char ch = '5';
if (is_numeric(ch)) {
std::cout << ch << " is a numeric character." << std::endl;
} else {
std::cout << ch << " is not a numeric character." << std::endl;
}
return 0;
}
Understanding the Basics of Numeric Types in C++
What are Numeric Types?
In C++, numeric types are data types used to represent numbers. These types are broadly categorized into integral types, which include integers, and floating-point types, which encompass decimal numbers. Common numeric types in C++ include:
- `int`: Represents integer values.
- `float`: Represents single-precision floating-point numbers.
- `double`: Represents double-precision floating-point numbers.
- `char`: Although primarily used for characters, it can also store small integer values.
Hierarchy of Numeric Types
C++ provides a hierarchy where certain numeric types can represent larger or more precise values than others. For example, a `double` can hold a wider range of values compared to a `float`. Additionally, type casting is crucial in C++ to convert one numeric type to another when needed, particularly in operations involving mixed types.
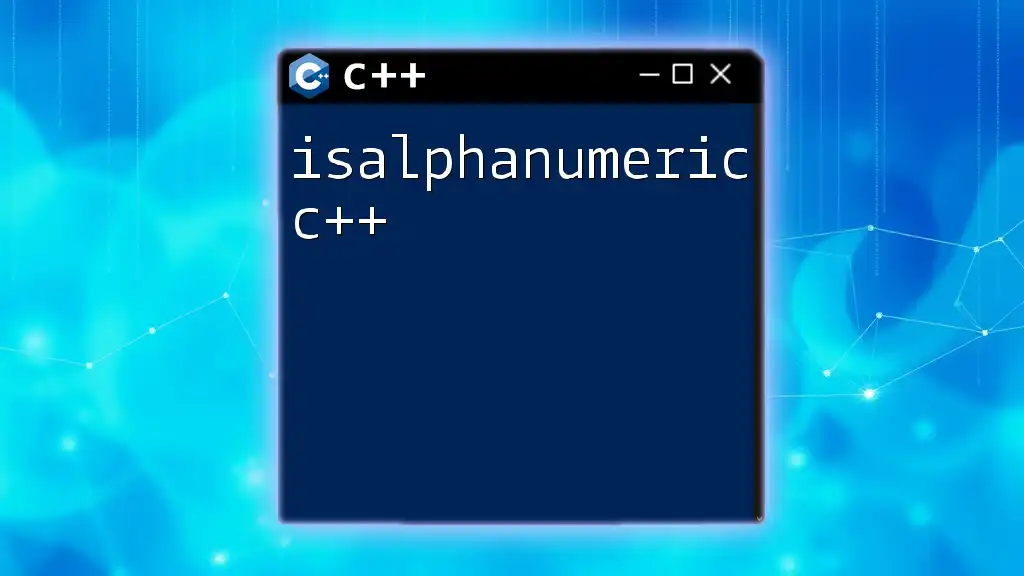
Introduction to `is_numeric`
What is `is_numeric`?
The term `is_numeric` refers to a function or method that checks whether a given value or string can be classified as numeric. In C++, this function is crucial for validating user input, processing data, and ensuring that applications handle only valid numeric values.
Why Use `is_numeric`?
Employing an `is_numeric` function has several significant benefits:
- Input Validation: It helps to validate user input, preventing errors and exceptions during data processing.
- Error Prevention: Mismanagement of data types can lead to runtime errors; `is_numeric` helps circumvent this issue.
- Real-World Applications: Whether in finance, scientific computing, or data analysis, ensuring correctness in numeric processing is crucial.
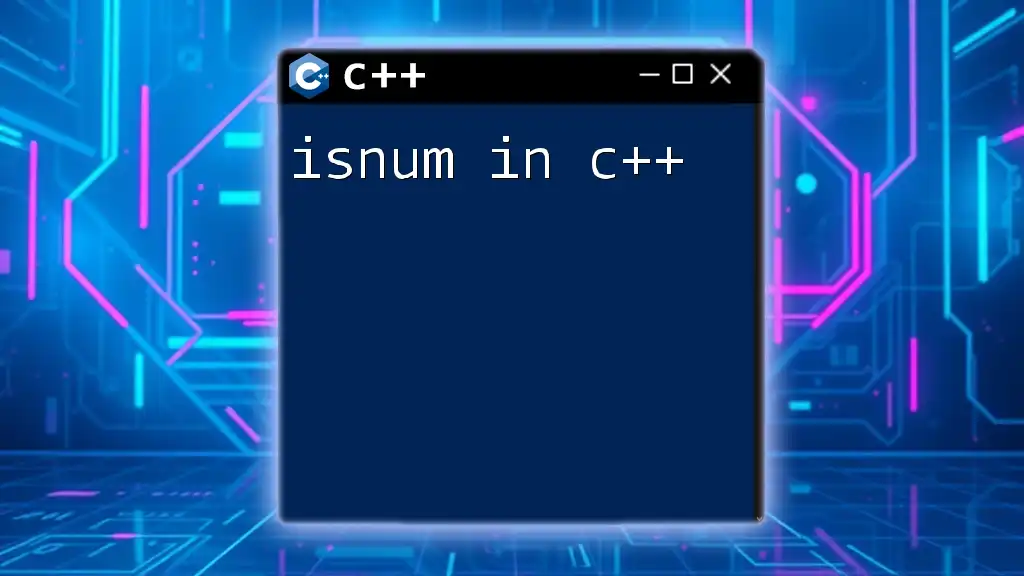
How to Use `is_numeric`
Basic Syntax of `is_numeric`
The basic structure of an `is_numeric` function in C++ assesses whether all characters in a string are numeric digits. It generally returns a boolean value: `true` for numeric strings and `false` otherwise.
Common Use Cases
`is_numeric` is particularly useful in situations where validation is necessary:
- When reading user input in forms.
- During data parsing from files or external sources.
- In mathematical calculations that require number input.
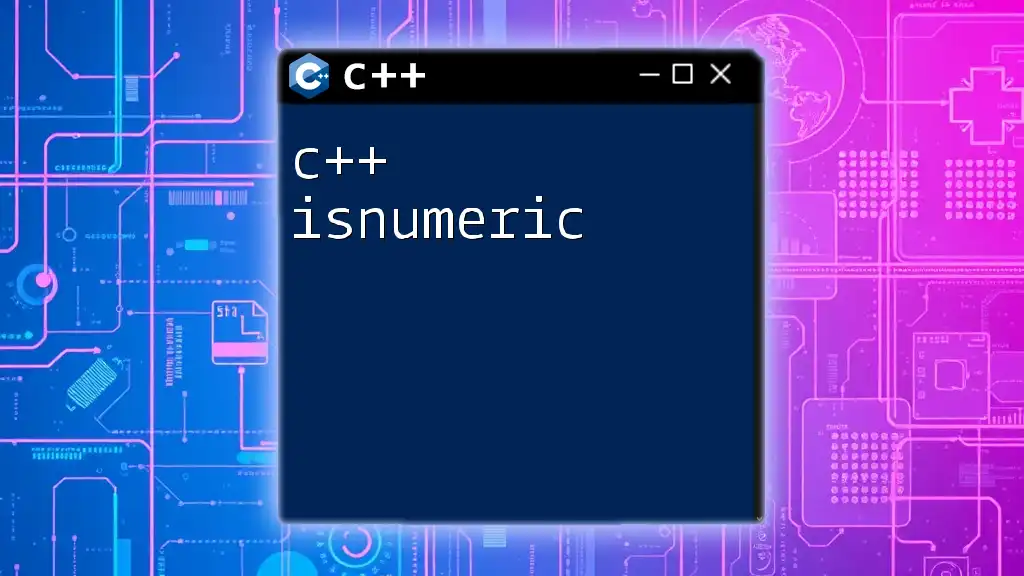
Code Snippets and Examples
Basic Example of `is_numeric`
Here's a simple implementation of an `is_numeric` function:
#include <iostream>
#include <string>
#include <cctype>
bool is_numeric(const std::string& str) {
for (char const &c : str) {
if (std::isdigit(c) == 0) return false;
}
return true;
}
int main() {
std::string input = "12345";
if(is_numeric(input)) {
std::cout << input << " is a numeric string." << std::endl;
} else {
std::cout << input << " is not a numeric string." << std::endl;
}
return 0;
}
Explanation of Code
In this example, the `is_numeric` function iterates over each character in the input string. The `std::isdigit()` function checks if each character is a digit (0-9). If any character fails this check, the function immediately returns `false`. If all characters pass, the function returns `true`, confirming that the input string is numeric.
Handling Edge Cases
Numeric Strings with Special Characters
Numeric inputs can sometimes include characters such as decimal points or negative signs. It is essential to ensure that our `is_numeric` function can handle these cases. For instance:
std::string input = "123.45"; // This should typically return false for a strict numeric check.
Negative Numbers and Signs
Permitting negative numbers also requires slight modifications to our function. Here’s how:
bool is_numeric_extended(const std::string& str) {
bool decimal_found = false;
for (size_t i = 0; i < str.length(); ++i) {
char c = str[i];
if (c == '-' && i == 0) continue; // Allow negative sign only at the start
if (c == '.') {
if (decimal_found) return false; // Only one decimal point is allowed
decimal_found = true;
continue;
}
if (!std::isdigit(c)) return false; // Not a digit
}
return true;
}
In this extended version, the function keeps track of whether it has encountered a decimal point. It allows a negative sign only at the start of the string and ensures that the decimal point appears only once.
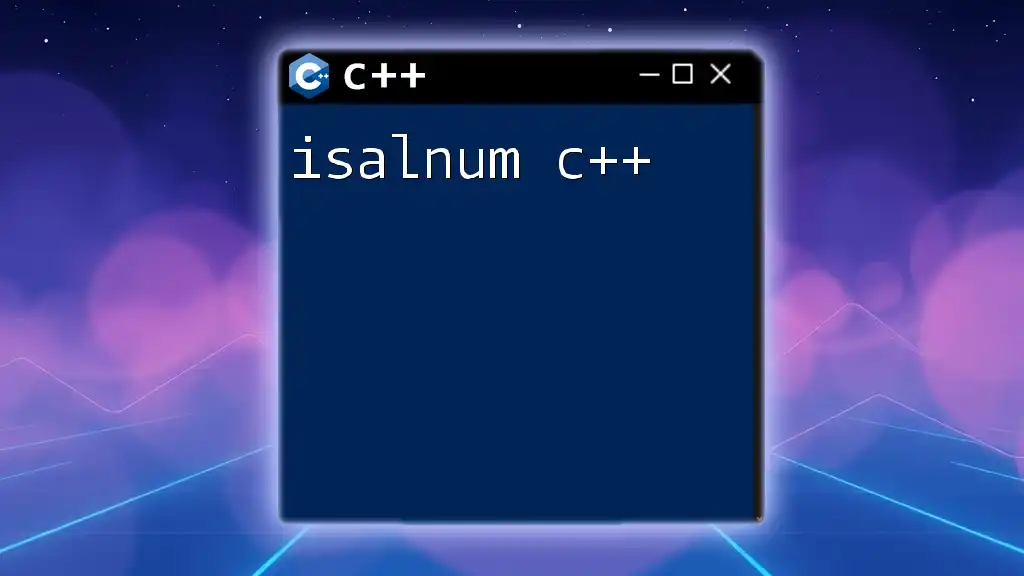
Performance Considerations
Efficiency of `is_numeric`
The time complexity of the `is_numeric` function is O(n), where n is the length of the string. Each character must be checked individually, making this a linear-time operation. When considering performance, it's crucial to benchmark the function against various string lengths to ensure it meets the desired speed for larger inputs.
Memory Usage
In terms of memory efficiency, the function uses minimal additional space since it only requires a few variables—an ideal approach for applications needing real-time validation.
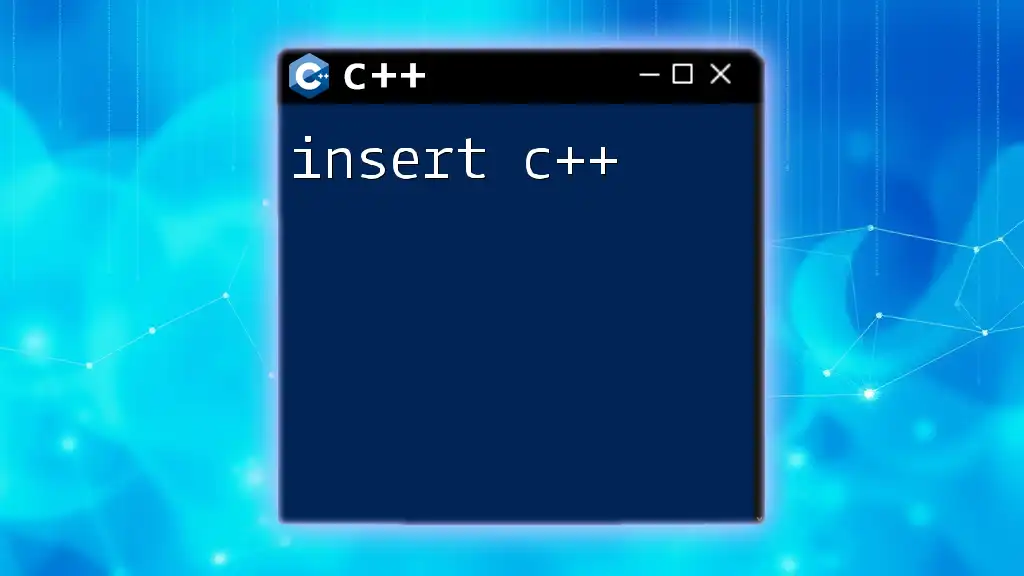
Conclusion
The `is_numeric` function is a powerful tool for ensuring data validity in C++. By leveraging this function, programmers can prevent errors, validate user input, and handle data safely. As you implement `is_numeric` in your projects, consider expanding its capabilities to handle various numeric formats, ensuring your applications are robust and user-friendly.
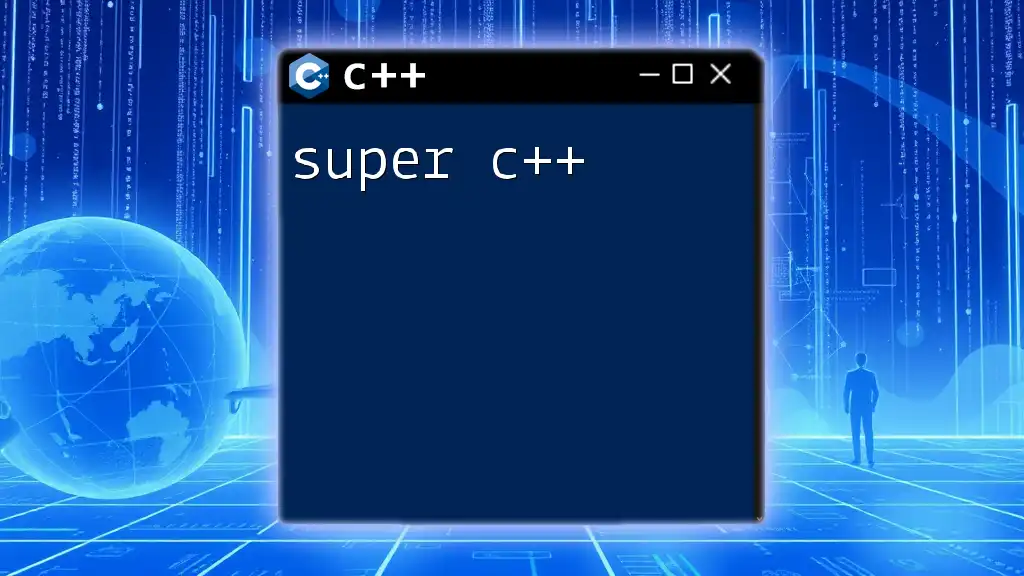
Related Resources
For those interested in diving deeper into numeric types and data validation in C++, additional resources may include reading on numeric type hierarchies, exploring C++ STL documentation, or looking into various tutorials on string manipulation techniques. These materials can enhance your understanding and application of `is_numeric` in diverse programming contexts.