The `.vimrc` file is a configuration file for Vim that allows you to set up custom commands and features to enhance your C++ coding experience, such as auto-indentation and syntax highlighting.
Here’s an example of a simple `.vimrc` configuration for C++ development:
set nocompatible " Use Vim defaults instead of 100% vi compatibility
filetype indent on " Enable file type detection and indentation
syntax on " Enable syntax highlighting
set tabstop=4 " Number of spaces a <Tab> counts for
set shiftwidth=4 " Number of spaces to use for each step of (auto)indent
set expandtab " Use spaces instead of tabs
set smartindent " Smart indentation for C++
This configuration helps to create a more efficient environment for writing and editing C++ code in Vim.
Understanding `.vimrc`
What is `.vimrc`?
The `.vimrc` file is a configuration file for Vim, a highly customizable text editor that is favored by many programmers. This file allows you to specify various settings and behaviors for Vim, tailoring it to fit your workflow seamlessly. By default, the `.vimrc` file is located in your home directory (`~/.vimrc`), and if it doesn’t exist, you can easily create one to start customizing your Vim environment.
Purpose of `.vimrc`
The primary purpose of the `.vimrc` file is to customize how Vim works, allowing you to create a personalized coding experience that enhances productivity. For C++ development, using a well-tuned `.vimrc` ensures smoother code writing, debugging, and compilation, which can significantly improve your overall workflow.
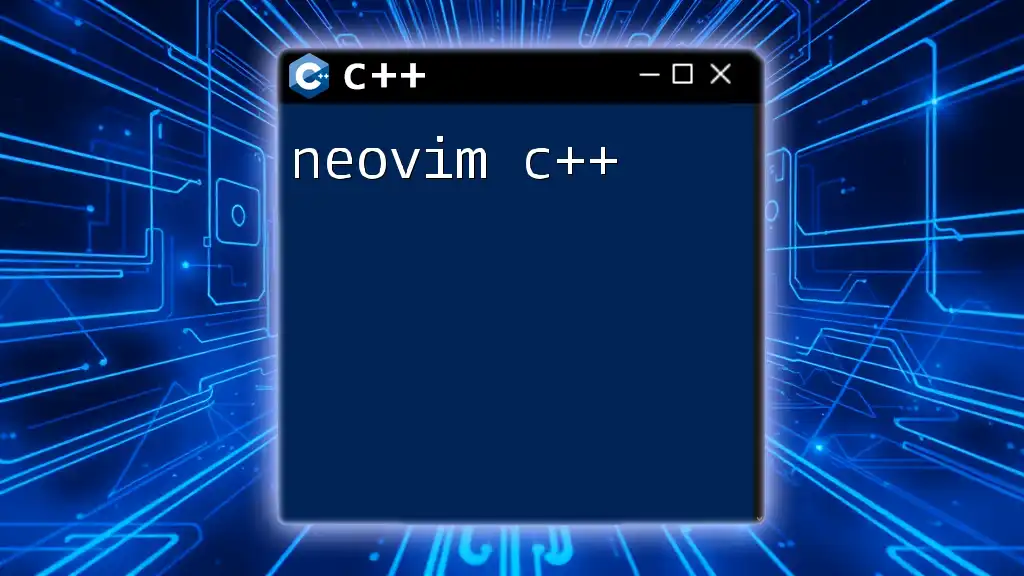
Basic Configuration for C++ in `.vimrc`
Essential Vim Settings for C++
Configuring some essential Vim settings can make a world of difference when working with C++. Here are a couple of fundamental settings worth implementing:
- Syntax Highlighting: Enabling syntax highlighting is critical as it helps you visually distinguish between different elements of your code, such as keywords, comments, and strings. Enable it in your `.vimrc`:
syntax on
- File Type Detection: File type detection ensures that Vim recognizes the type of file you are editing and provides the appropriate syntax highlighting and indent settings. You can enable file type detection using:
filetype plugin indent on
Optimizing Indentation
Indentation is essential in C++, as it improves code readability and maintains a consistent style. Vim allows you to customize indentation settings effectively:
- Spaces vs. Tabs: Decide whether to use spaces or tabs for indentation. Most C++ developers prefer spaces for consistency. Add these lines to your `.vimrc` to set the tab length and enable spaces:
set tabstop=4 " Number of spaces that a <Tab> counts for
set shiftwidth=4 " Number of spaces to use for each step of (auto)indent
set expandtab " Use spaces instead of tabs
By doing this, when you press the `<Tab>` key, it will insert spaces instead of a tab character, giving you more control over the spacing in your code.
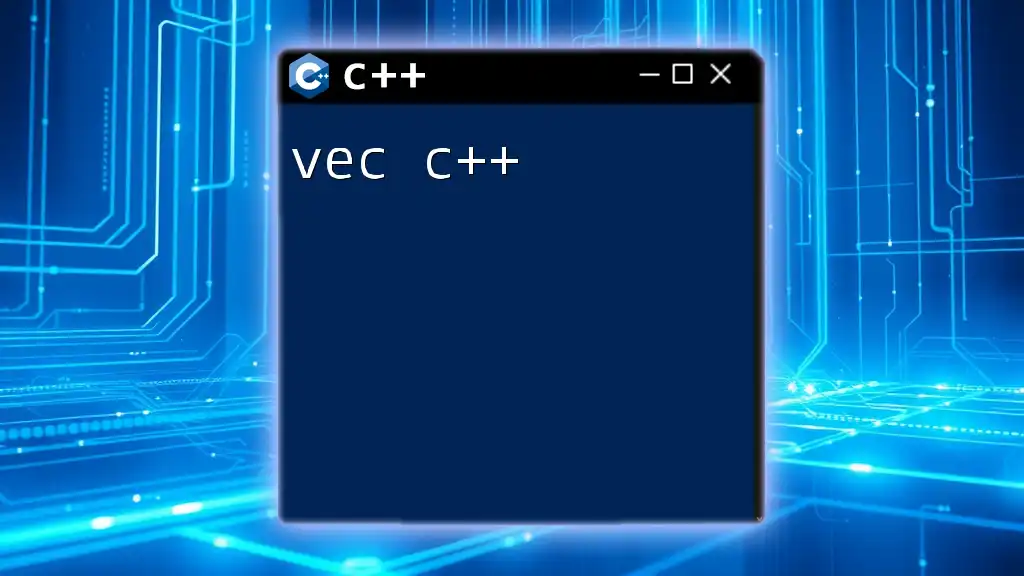
Advanced Customization Options
Code Completion
To enhance your coding efficiency, implementing code completion is invaluable. C++ development can benefit immensely from suggestions and autocompletions that can speed up the coding process. You can improve this with various plugins:
One popular choice is YouCompleteMe. Here’s a basic setup snippet for enabling it in your `.vimrc`:
let g:ycm_auto_trigger = 1
let g:ycm_complete_in_comments_and_strings = 1
Syntax Checking
Real-time error detection is crucial for catching mistakes early in the development process. Integrating a language server like `clangd` can provide syntax checking in real time. Add this line to your `.vimrc`:
autocmd FileType cpp setlocal omnifunc=v:lua.vim.lsp.omnifunc
This configuration enables `clangd` to analyze your code and provide errors/suggestions, ensuring that you write cleaner code with fewer mistakes.
Custom Shortcuts for C++
Defining custom key mappings can greatly enhance your coding speed and efficiency in C++. By creating shortcuts for frequently used commands, you can minimize hand movement and keep your workflow uninterrupted. Consider the following mappings:
nnoremap <Leader>cc :!g++ % -o %< && ./%<CR> " Compile and run C++ file
nnoremap <Leader>r :!./%<CR> " Run the compiled binary
The above shortcuts allow you to compile and run your current C++ file with just a couple of keystrokes, significantly speeding up the testing process.
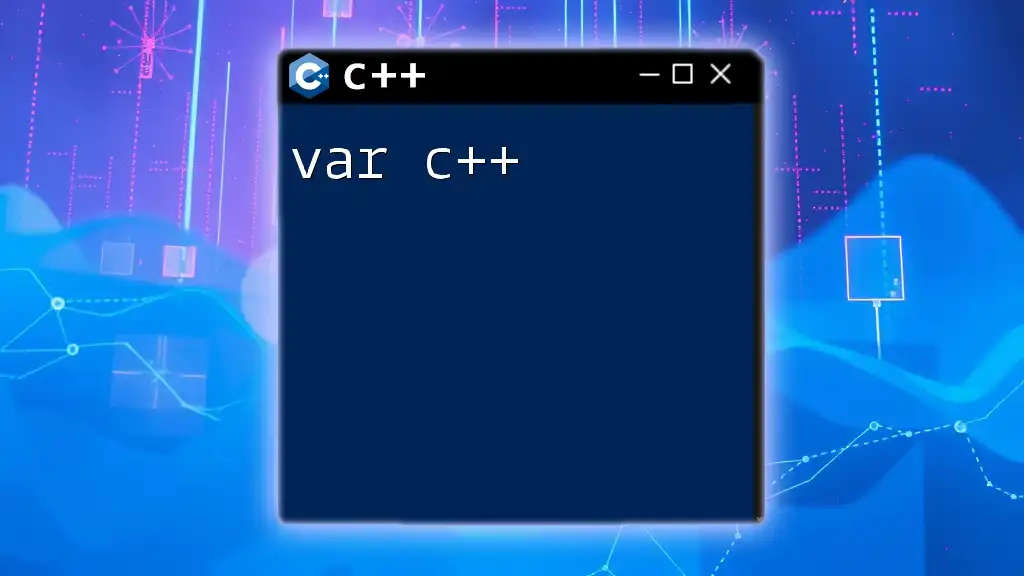
Themed Configuration
Look and Feel
A visually appealing editor can make for a more enjoyable coding experience. Choosing a colorscheme that enhances readability can help reduce eye strain over long coding sessions.
You might set your colorscheme with the following line in your `.vimrc`:
colorscheme gruvbox " Replace with your preferred colorscheme
Fonts and User Interface
Adjusting the font and user interface contributes significantly to readability and overall comfort during work sessions. To set a preferred font, you might consider:
set guifont=Monaco:h12 " Adjust font name and size
This configuration ensures that the text is not only clear but also easy on the eyes.
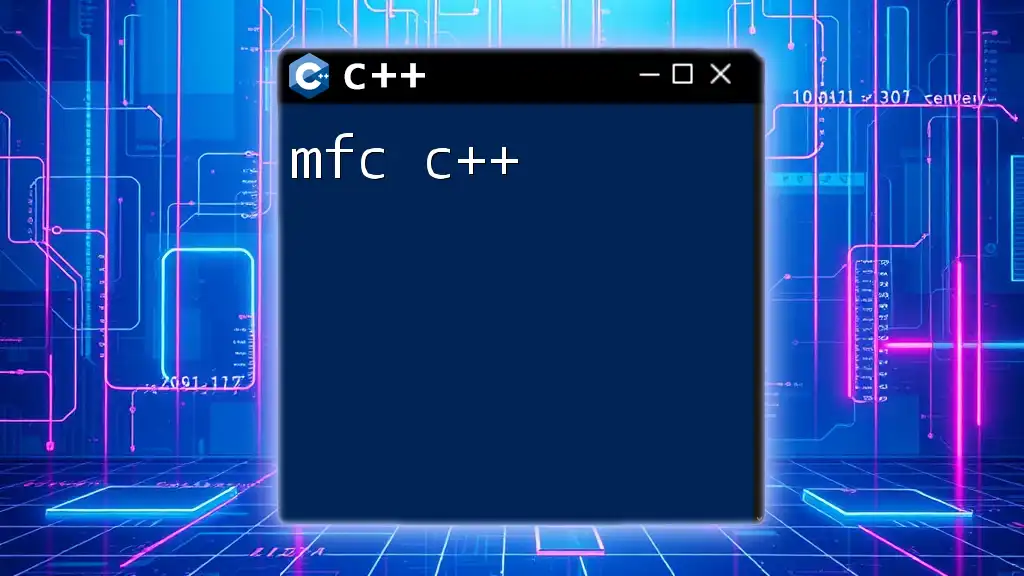
Plugin Recommendations for C++ Development
Popular Plugins
Enhancing your development environment with the right plugins can increase your productivity. Here are some recommended plugins:
- nerdtree: This file explorer allows for quick navigation through your project’s directory structure, making it easier to manage files.
- vim-gutentags: An automatic tag generation tool that enables better code navigation and browsing.
- vim-fugitive: Provides Git integration, making it simple to manage version control without leaving the editor.
Setting Up Plugins
Using a plugin manager, such as vim-plug, streamlines the process of installing and managing plugins. Here’s a basic installation snippet for your `.vimrc`:
call plug#begin('~/.vim/plugged')
Plug 'scrooloose/nerdtree'
call plug#end()
Simply place your desired plugins between the `plug#begin` and `plug#end` calls to include them in your Vim setup.
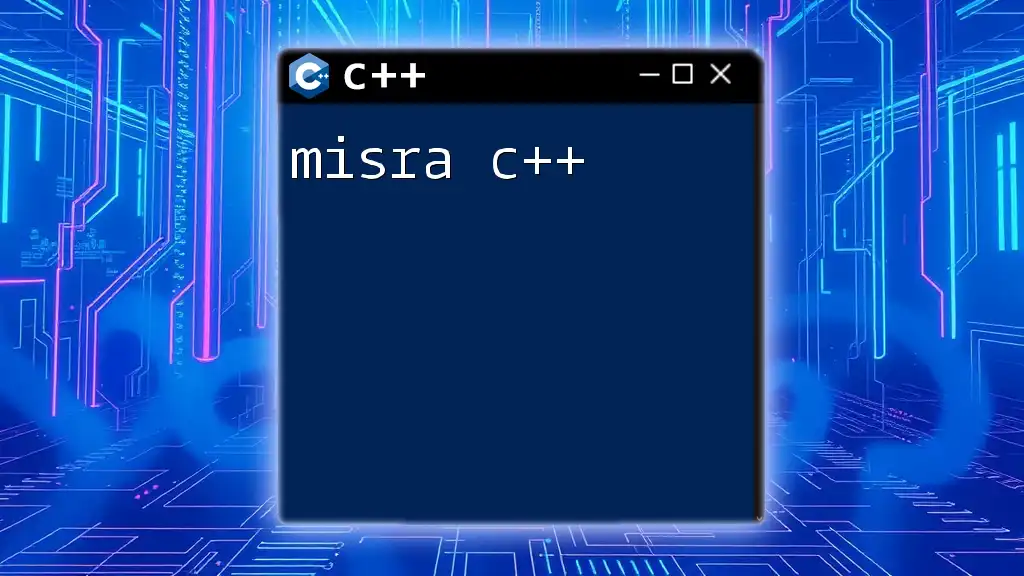
Troubleshooting Common Issues
Configuration Conflicts
Sometimes, conflicts in your `.vimrc` settings can lead to frustrating experiences. If you find that certain settings are not working as intended, it’s essential to identify and troubleshoot these issues.
You can start by commenting out sections of your `.vimrc` file to isolate problematic settings, or even run Vim in a minimal configuration mode (using `vim -u NONE`) to see if the problem persists without your custom configurations.
Performance Tips
Streamlining your `.vimrc` can also help enhance performance. Begin by removing redundant settings or any outdated plugins that may no longer serve a purpose. You can also consider lazy-loading your plugins to reduce the startup time of Vim.
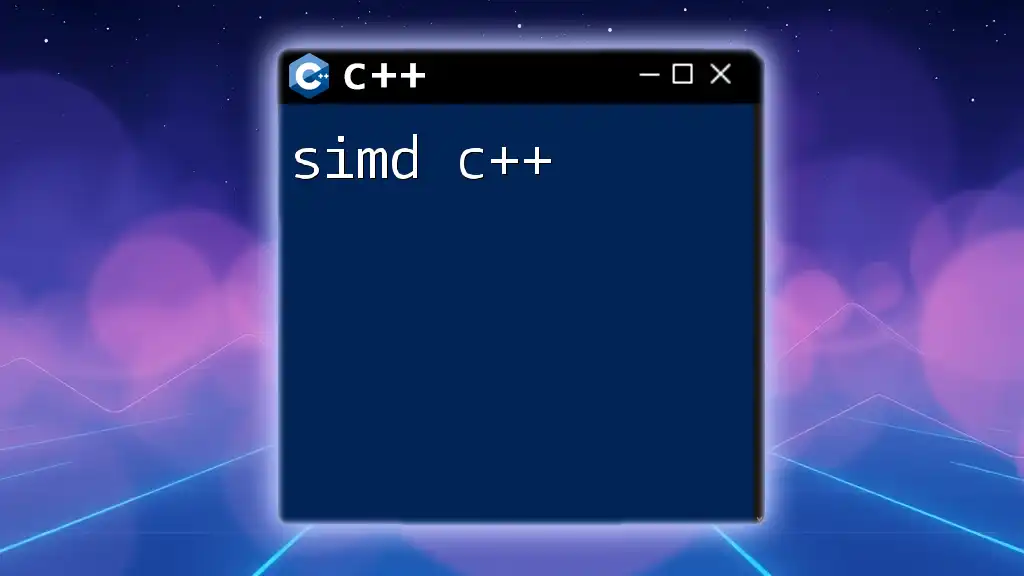
Conclusion
A well-configured `vimrc` is essential for maximizing your C++ development efficiency. By customizing Vim with the settings and tools tailored to your coding needs, you foster a more productive and pleasant coding environment. As you continue to work and grow as a developer, don’t be afraid to adjust your `.vimrc` to better suit your evolving workflow.
Encourage engagement with the community around Vim and C++—sharing insights, configurations, and experiences is key to mastering this powerful editor. Happy coding!
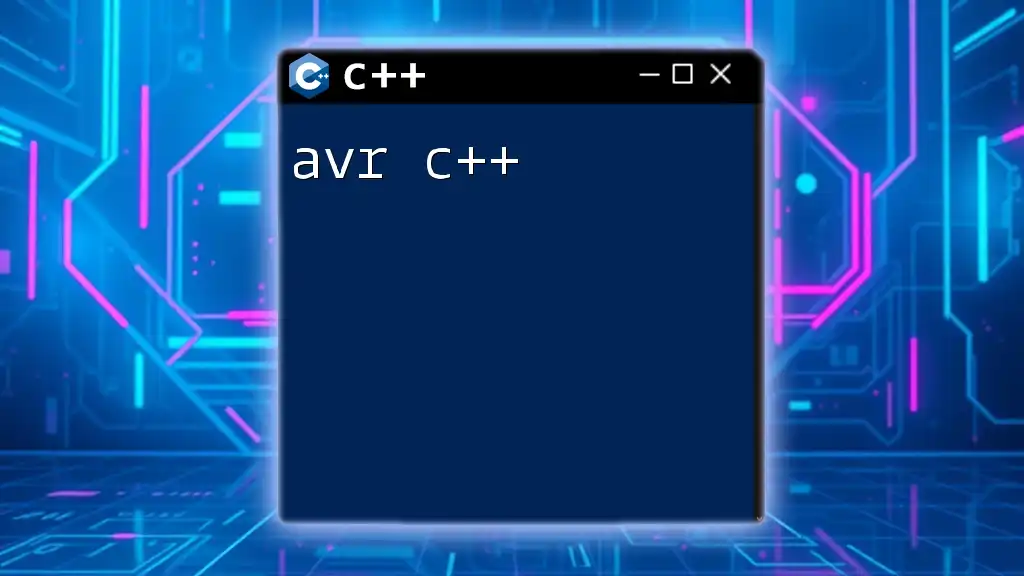
Further Resources
For those interested in diving deeper into Vim and C++, consider exploring resources like Vim documentation, C++ best practices, and vibrant online forums where fellow developers share their wisdom and expertise.