The `strptime` function in C++ is used to parse date and time strings into a `tm` structure based on a specified format.
#include <iostream>
#include <ctime>
int main() {
const char* dateTimeStr = "2023-10-01 12:30:45";
struct tm tm;
strptime(dateTimeStr, "%Y-%m-%d %H:%M:%S", &tm);
std::cout << "Parsed time: " << asctime(&tm);
return 0;
}
Understanding Time Representation in C++
In C++, date and time handling is primarily achieved through the `tm` structure, which is defined in the `<ctime>` header. This structure represents calendar time broken down into its components, such as year, month, day, hour, minute, and second. Understanding this representation is crucial for manipulating dates and times effectively.
When working with dates, it's common to interact with various formats, including the ISO 8601 standard (YYYY-MM-DD) and the more human-friendly formats (e.g., MM/DD/YYYY). Knowing these formats helps when parsing or formatting dates using functions like `strptime`.

What is strptime?
`strptime` stands for "string parse time." This function is invaluable in C++ for converting a string representation of date and time into a structured format that can be manipulated programmatically. It helps in translating human-readable date formats into a format that a program can understand.
Originally part of the C standard library, `strptime` has become a crucial component of C++ date-time handling. Some common use cases include reading timestamps from files, converting user input into standardized date formats, and preparing for date arithmetic.
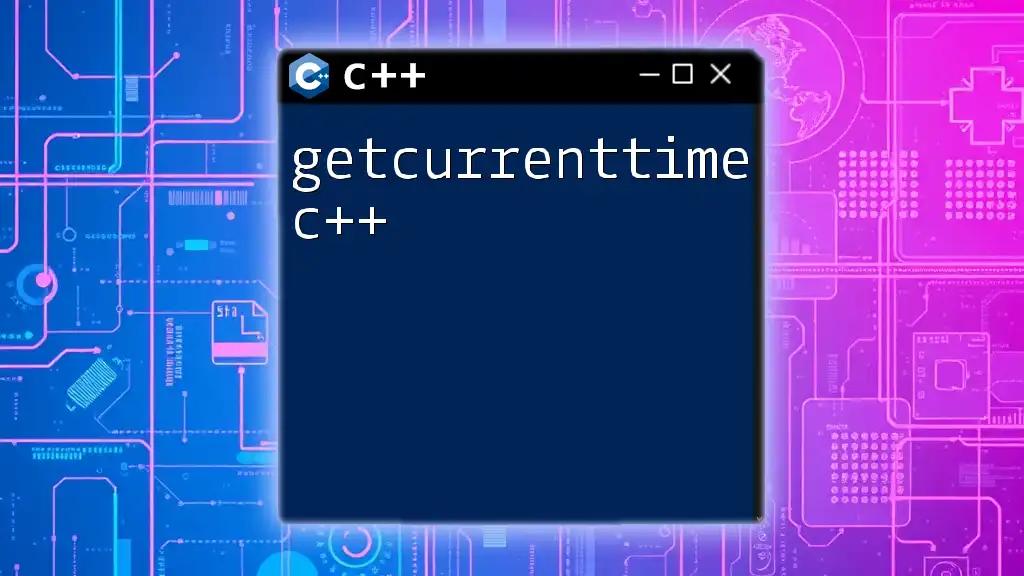
Syntax of strptime
The syntax for the `strptime` function is as follows:
char *strptime(const char *s, const char *fmt, struct tm *tm);
Explanation of Parameters
-
s: This is the input string that you want to parse. It should be formatted according to the formatting string.
-
fmt: The format specifier string that describes the expected format of the date and time. Each part of the string corresponds to a component of the date-time structure.
-
tm: A pointer to a `tm` structure where the parsed date and time will be stored. After execution, this structure will be populated with the parsed values.
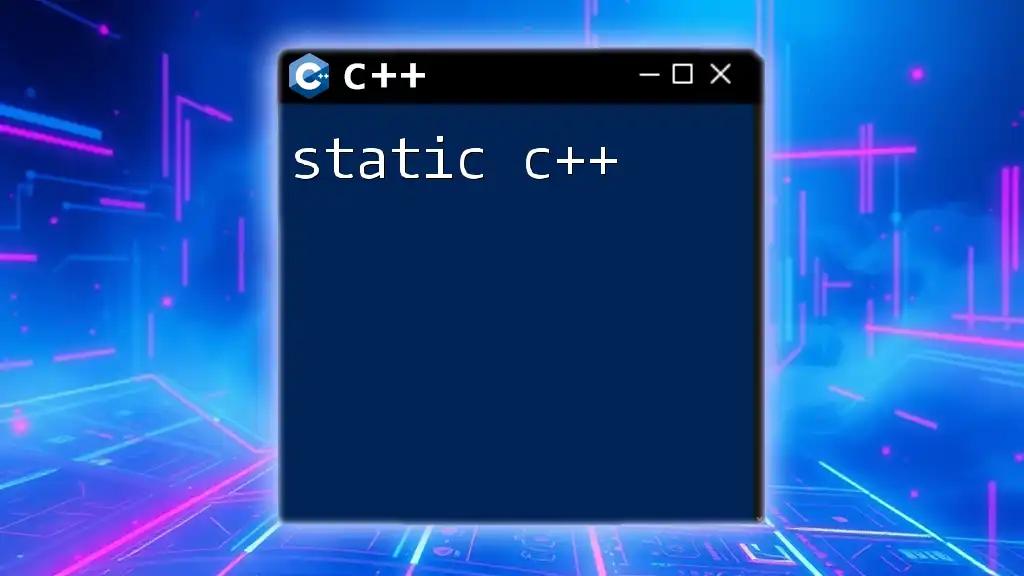
Format Specifiers
Understanding format specifiers is crucial when working with `strptime`. The most commonly used specifiers include:
- %Y: Year with century (e.g., 2023)
- %m: Month as a decimal number (01 - 12)
- %d: Day of the month (01 - 31)
- %H: Hour in 24-hour format (00 - 23)
- %M: Minute (00 - 59)
- %S: Second (00 - 60)
Additionally, there are others such as `%b` for a month's abbreviated name and `%A` for the full name of the day. Depending on your locale settings, some specifiers may behave differently, so it’s essential to configure the locale appropriately for consistent behavior across different systems.

Using strptime: Basic Example
Let’s explore a simple example to illustrate how `strptime` works.
#include <iostream>
#include <iomanip>
#include <ctime>
#include <cstring>
int main() {
const char *dateStr = "2023-10-16 14:30:25";
struct tm tm;
strptime(dateStr, "%Y-%m-%d %H:%M:%S", &tm);
std::cout << "Parsed Date: " << std::put_time(&tm, "%c") << std::endl;
return 0;
}
In this code snippet, we parse the string `dateStr` containing the date and time representation. The specified format (`"%Y-%m-%d %H:%M:%S"`) matches the structure of the input string. After parsing, we utilize `std::put_time` to format the `tm` structure back into a readable string format for output. This demonstrates the effective conversion of data from string format to structured format for further manipulation.
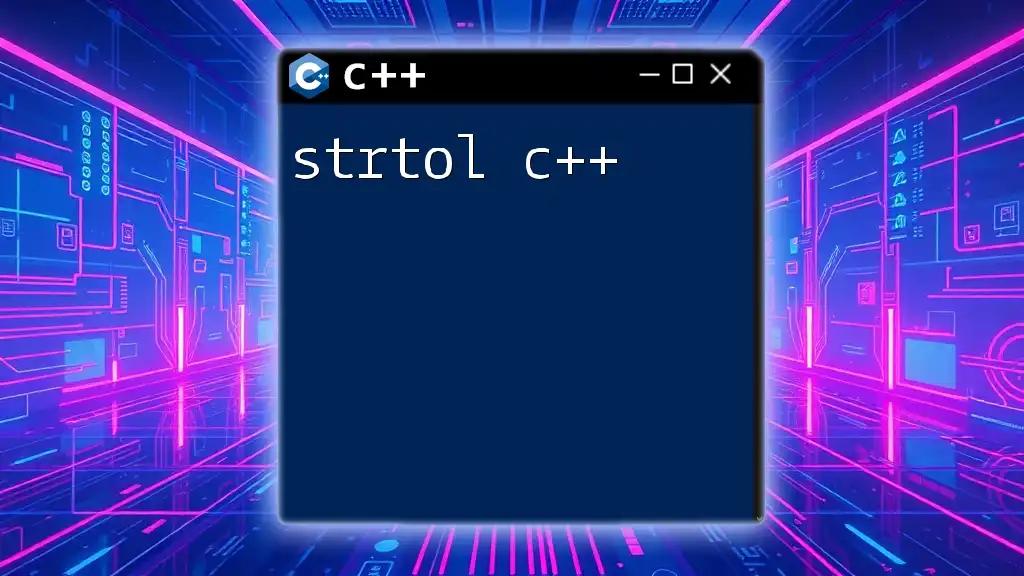
Error Handling with strptime
When using `strptime`, it’s vital to consider error handling. If the parsing fails, `strptime` returns a `nullptr`. This behavior allows you to implement simple checks to verify whether the parsing was successful.
const char *dateStr = "Invalid date string";
struct tm tm;
if (strptime(dateStr, "%Y-%m-%d", &tm) == nullptr) {
std::cerr << "Failed to parse date." << std::endl;
}
In this example, we attempt to parse an invalid date string. The check against `nullptr` enables you to handle errors gracefully, providing feedback to the user or taking corrective actions.
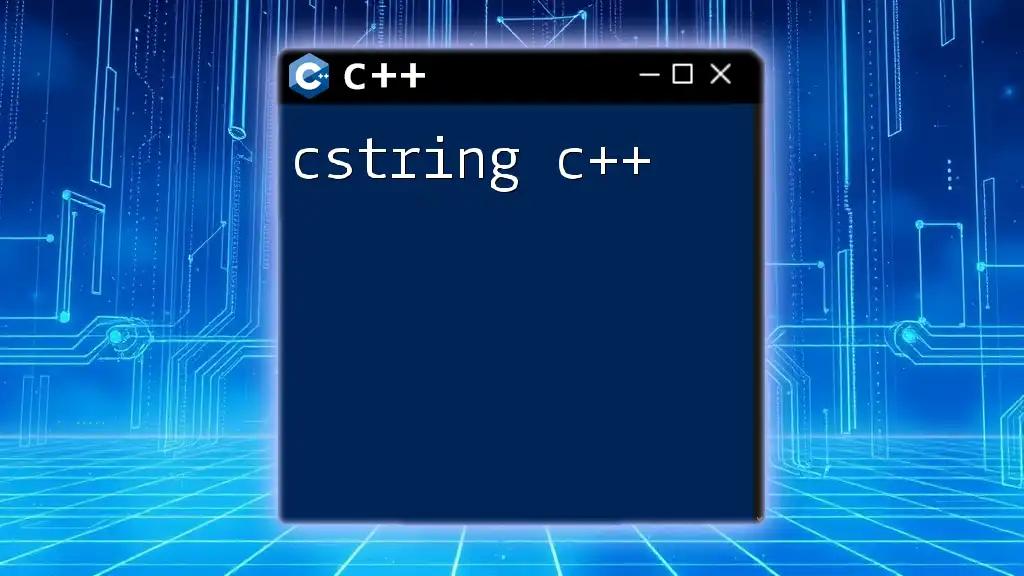
Real-World Applications of strptime
`strptime` shines in various real-world applications. Some notable instances include:
- Data Logging: Parsing timestamps from log files enables easier data analysis and reporting.
- Event Scheduling: User-generated events often require date and time validation, which can be accomplished seamlessly with `strptime`.
For instance, consider a scenario in which you read a log file containing entries with timestamps. By parsing these timestamps using `strptime`, you can easily manipulate them to compute durations, filter logs, or convert them to different timezone formats.
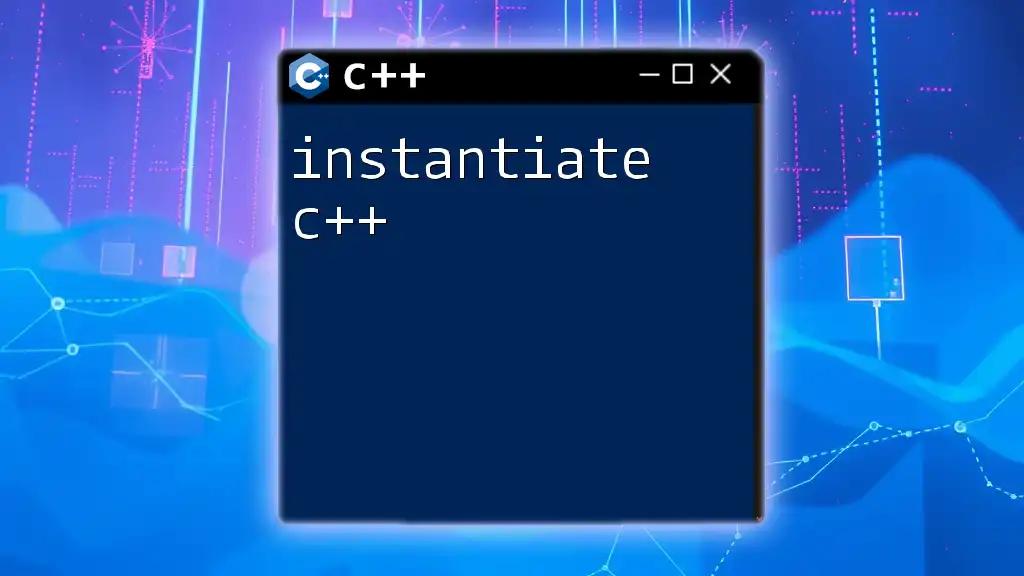
Advanced Usage and Custom Parsing
`strptime` is versatile. You can customize format strings for non-standard or complex date formats. For instance, if you were dealing with cases where the date format is “DD/MM/YYYY” instead of “YYYY-MM-DD”, you can modify the format string accordingly.
Combining multiple `strptime` calls can also help with more intricate parsing tasks. For example, if you receive datetime strings that include both date and time in separate strings, you can first parse the date and then the time, merging the results into one `tm` structure.
Moreover, leveraging C++ libraries such as `<chrono>` can greatly enhance your date-time handling capabilities, allowing you to perform calculations and comparisons directly on time points.
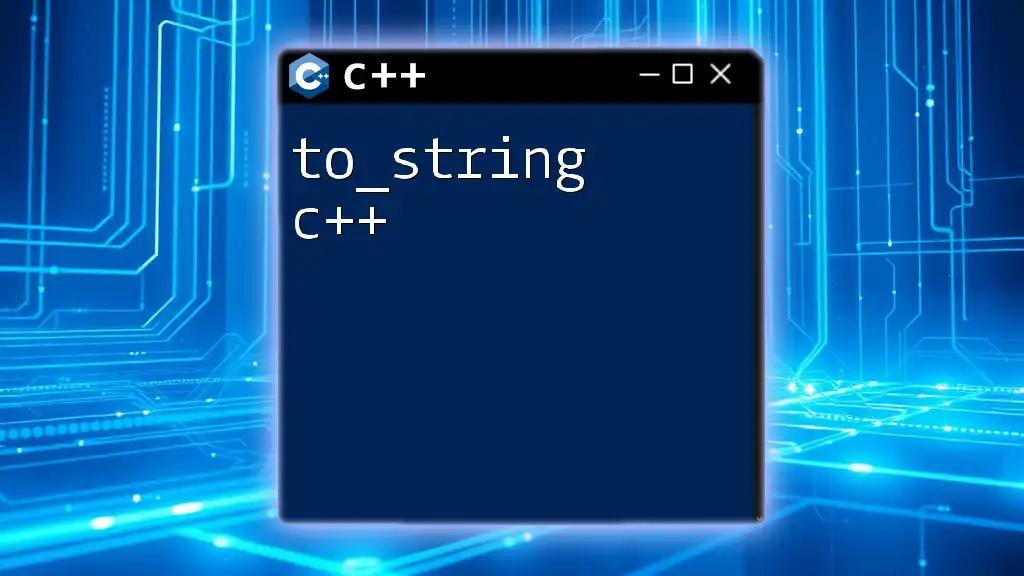
Best Practices
When using `strptime`, consider these best practices:
- Choose the Right Format Specifiers: Ensure the format string matches your input string precisely; inconsistency leads to parsing failures.
- Check Parsing Success: Always check the return value of `strptime` to handle errors before proceeding with any parsed data.
- Performance Considerations: If parsing large datasets or frequent calls are necessary, profile your application to ensure performance is not hindered.
For example, if you’re parsing multiple timestamps in a loop, consider consolidating the parsing process to optimize performance.
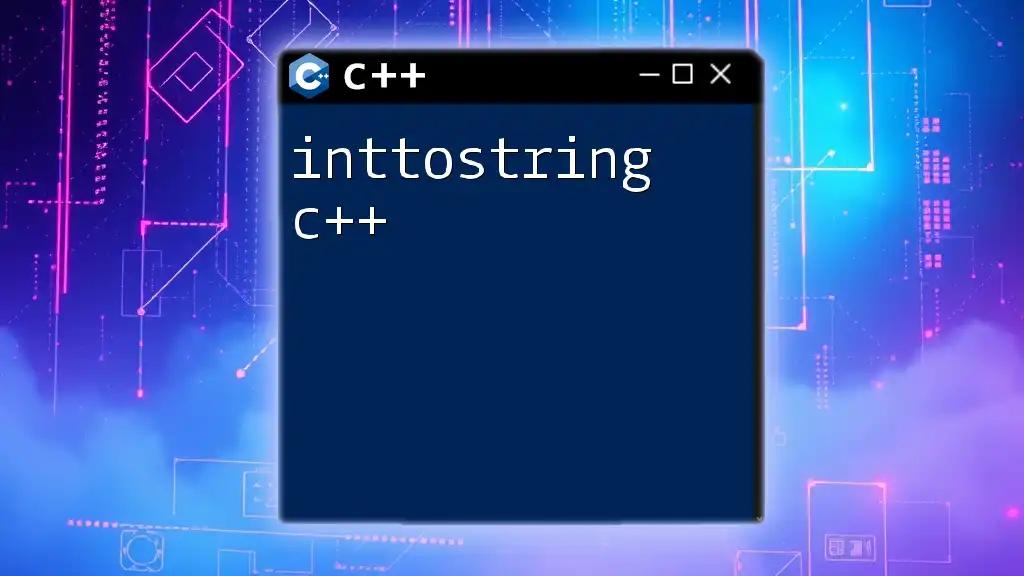
Conclusion
In summary, `strptime` is a powerful function for parsing strings into structured time representations in C++. By mastering its syntax and capabilities, you can efficiently handle various date and time formats for your applications. Whether it's for logging, scheduling, or data analysis, understanding how to utilize `strptime` effectively will significantly enhance your programming skill set. Engage with your community—share experiences, challenges, or insights related to date and time handling.