In C++, the `static` keyword is used to define variables with a local scope that maintain their value between function calls or to restrict the visibility of a function or variable to the file in which it resides.
Here's a code snippet demonstrating the use of `static`:
#include <iostream>
void counter() {
static int count = 0; // static variable retains its value between calls
count++;
std::cout << "Count: " << count << std::endl;
}
int main() {
counter(); // Output: Count: 1
counter(); // Output: Count: 2
counter(); // Output: Count: 3
return 0;
}
What is the Static Keyword in C++?
The static keyword is a fundamental aspect of C++ that alters the storage duration and visibility of variables and functions. When a variable is defined as static, it retains its value between function calls and is allocated only once in memory for its entire lifetime. This means that a static variable is initialized only once and its lifetime extends throughout the program's execution.
Understanding the implications of the static keyword is crucial when optimizing memory and ensuring the encapsulation of data. It stands in contrast to dynamic storage duration, where variables are allocated and deallocated at runtime, providing less control over memory management.

The Purpose of Static in C++
Using the static keyword brings several benefits:
- Memory Management: Static variables reduce the overhead of memory allocation by ensuring that a single instance of the variable exists throughout the program's execution.
- Data Encapsulation: By restricting the visibility of a static variable or function to its defining file or class, you can protect data and reduce namespace pollution.
- Performance Improvements: Static variables can lead to faster execution times because they reduce the need for repetitive allocations and deallocations during function calls.

Static Variables in C++
Understanding Static Variables
A static variable in C++ is designed to maintain its value across multiple calls to a function. Unlike local variables, which are reinitialized every time a function is called, a static variable is initialized only once. The scope of the static variable can vary depending on whether it is defined inside a function or at the global level.
Usage of Static Variables
Local Static Variables
Local static variables are declared within a function. Their scope is limited to that function, but their lifetime persists throughout the execution of the program.
For example:
void incrementCounter() {
static int counter = 0; // Initialized only once
counter++;
std::cout << "Counter: " << counter << std::endl;
}
In this code, `counter` retains its value between calls to `incrementCounter`. If you call `incrementCounter()` multiple times, it will keep incrementing `counter` without resetting it.
Global Static Variables
Global static variables, on the other hand, are defined outside of all functions and their visibility is restricted to the file they are declared in.
For example:
static int fileScopeCounter = 0; // Only accessible in this file
void increaseFileScopeCounter() {
fileScopeCounter++;
}
Here, `fileScopeCounter` can only be accessed within this source file, which helps to prevent naming conflicts in larger projects where multiple files may define variables with the same name.
Implications of Using Static Variables
It is essential to consider potential pitfalls when using static variables, especially related to multithreading. Since they retain their value across function calls and invocations, it can lead to race conditions if multiple threads try to modify the static variable simultaneously.
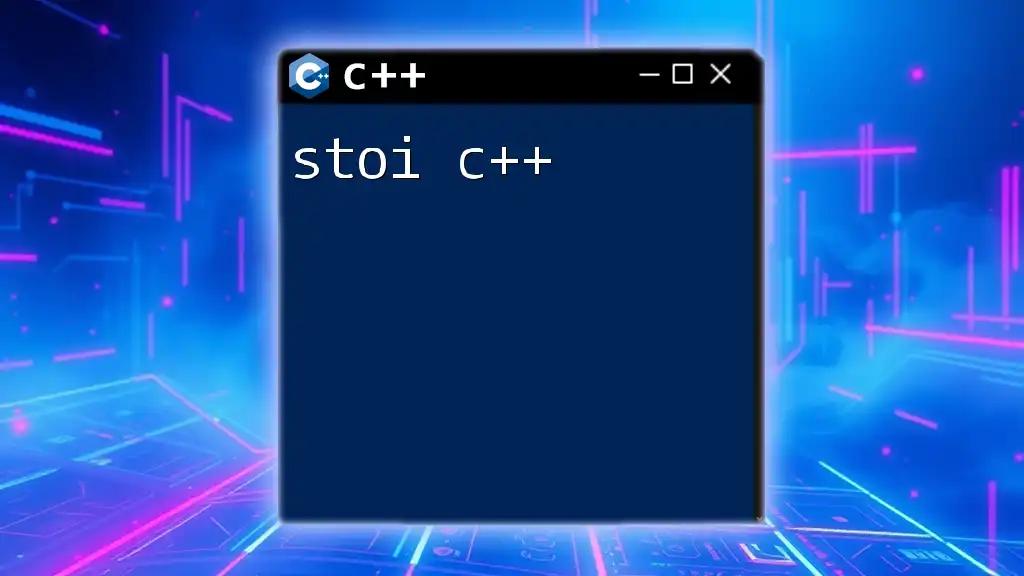
Static Functions in C++
Definition of Static Functions
A static function in C++ is a function that can be invoked without creating an instance of its class. This allows for shared behavior or functionality that does not require an object. Static functions can only access static members (variables or methods) of the class.
Using Static Functions
Class-Scope Static Functions
Static functions are typically defined within a class. For example:
class MathUtils {
public:
static int add(int a, int b) {
return a + b;
}
};
You can call `MathUtils::add(5, 10)` without needing to instantiate the `MathUtils` class. This reduces memory overhead and simplifies usage when the function does not rely on instance data.
Global Static Functions
Global static functions are defined outside classes and are limited in scope to the file they are declared in. This ensures they do not interfere with functions of the same name in other files.
static void privateHelperFunction() {
// This function is not accessible outside this file
}
Benefits of Using Static Functions
Utilizing static functions can lead to several advantages:
- Reduced Memory Overhead: Since static functions do not require an object instance, they occupy less memory and can be called without instantiating the class.
- Encapsulation of Functionality: Keeping a function static limits its visibility and scope, helping to avoid naming collisions.

Static Data Types in C++
What Are Static Data Types?
In C++, a static data type refers to variables or types that are declared as static, meaning they have a lifetime extending beyond the current block of execution. This alters how the data behaves, particularly concerning initialization and memory storage.
Using Static Data Types
Static Integers
For instance, a static integer can retain its value throughout the program lifecycle, which is useful in many scenarios:
static int staticInt = 10; // Remains unchanged until modified explicitly
You can manipulate `staticInt` from various functions as long as they reside in the same file, offering an efficient way to share constant values without exposing them.
Static Arrays
Static arrays are also beneficial for situations where you need a constant set of values. For example:
static int staticArray[5] = {1, 2, 3, 4, 5};
Like static integers, these arrays maintain their values across function calls and provide an efficient, fixed-size data storage mechanism.

Common Use Cases for Static in C++
When to Use Static Variables and Functions
Static variables and functions are especially useful in scenarios that require:
- Shared State Across Function Calls: Use static variables when you need a counter or accumulator that persists across function invocations.
- Utility Functions: Implement static functions to organize functional utilities that do not require object state.
Real-World Example
Consider a logger utility that keeps track of the log messages. This class could implement a static counter to uniquely number logs without requiring instantiation:
class Logger {
private:
static int logCount;
public:
static void logMessage(const std::string& message) {
logCount++;
std::cout << "Log #" << logCount << ": " << message << std::endl;
}
};
int Logger::logCount = 0; // Define static member outside the class
In this example, `Logger::logMessage` can be called from anywhere in your program without needing to create an instance of `Logger`, ensuring data consistency and easy access.
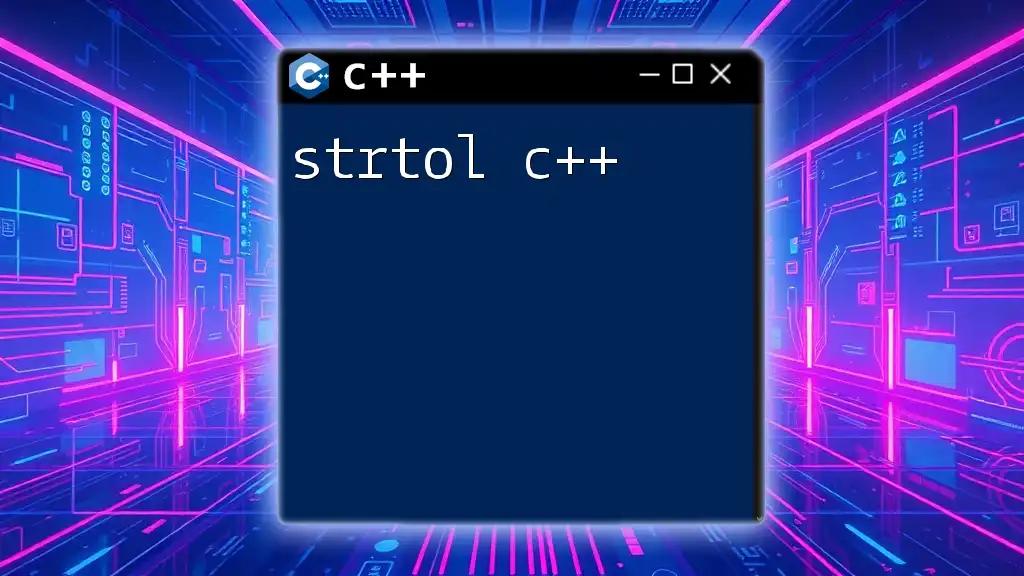
Best Practices When Working with Static in C++
To harness the full potential of static in C++, consider these best practices:
- Limit Usage: Overuse of static can lead to hard-to-track bugs, especially in complex systems. Use them judiciously.
- Be Cautious with Thread Safety: If static variables are accessed in multithreaded environments, ensure proper synchronization to avoid race conditions.
- Group Related Functionality: Design your classes and functions such that all static members serve a unified purpose, enhancing code readability and maintenance.
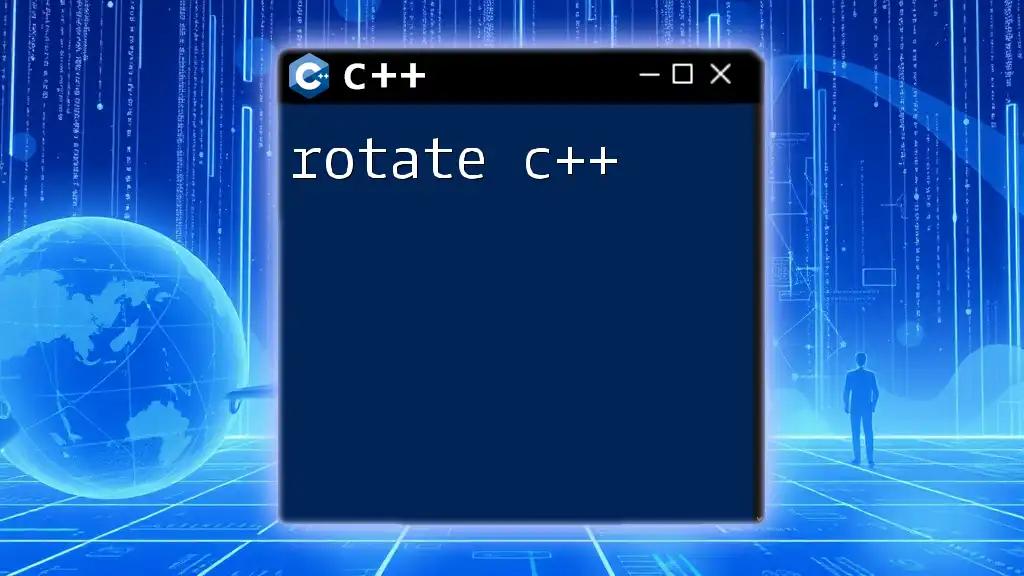
Conclusion
Understanding how to effectively use the static c++ keyword is essential for optimizing memory management, implementing encapsulation, and ensuring efficient function calls. Proficient use of static variables and functions can significantly enhance your coding capabilities while helping you maintain cleaner and more efficient code. By applying the knowledge from this guide, you can confidently incorporate static elements into your C++ programming practice.