The `stof` function in C++ is used to convert a string to a floating-point number (specifically `float`), making it useful for parsing numerical data from text.
Here’s a code snippet demonstrating its usage:
#include <iostream>
#include <string>
int main() {
std::string strNumber = "3.14";
float number = std::stof(strNumber);
std::cout << "The floating-point number is: " << number << std::endl;
return 0;
}
What is the C++ stof Function?
The stof function is a part of the C++ Standard Library that allows developers to convert a string representation of a number into a floating-point value (specifically `float`). It is a highly useful function when you need to deal with numeric data stored as text, which is common in user input, configuration files, and other data sources.
In essence, `stof` is a straightforward and effective way to bridge the gap between string formats and numerical calculations.
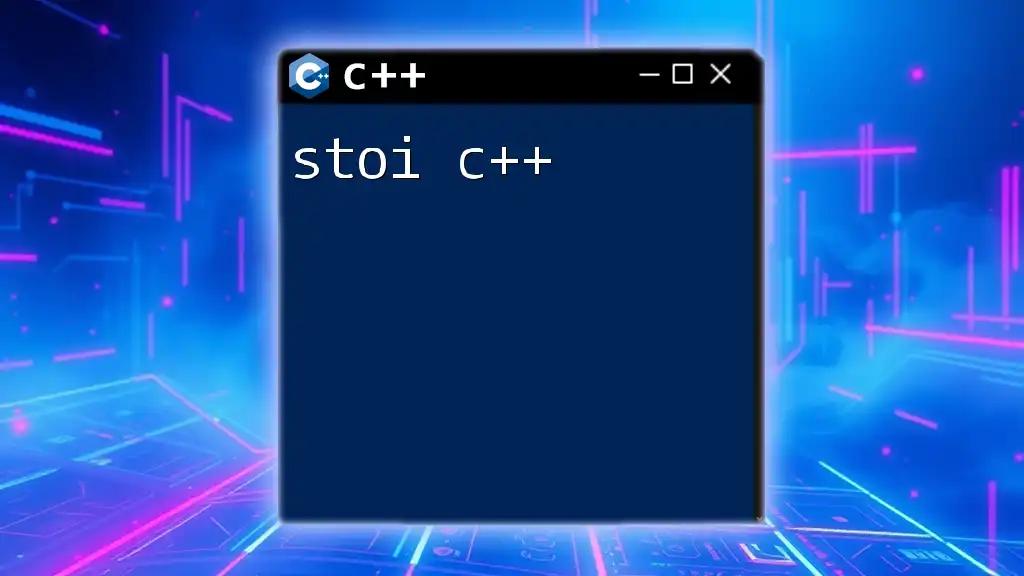
Syntax of the stof Function
The basic syntax of the `stof` function is as follows:
float stof(const std::string& str, std::size_t* idx = 0);
Parameters Explained
-
str: This is the string to be converted. It must represent a valid floating-point number (e.g., "3.14", "0.001", etc.).
-
idx: This is an optional parameter. If provided, it will point to the first character of the string that is not part of the number. This can be useful for parsing or extracting additional information from the string after the numeric value has been obtained.
Return Value
The function returns a `float`, which is the converted numerical value from the provided string. If the conversion fails or an error occurs, it throws exceptions that will be discussed later.
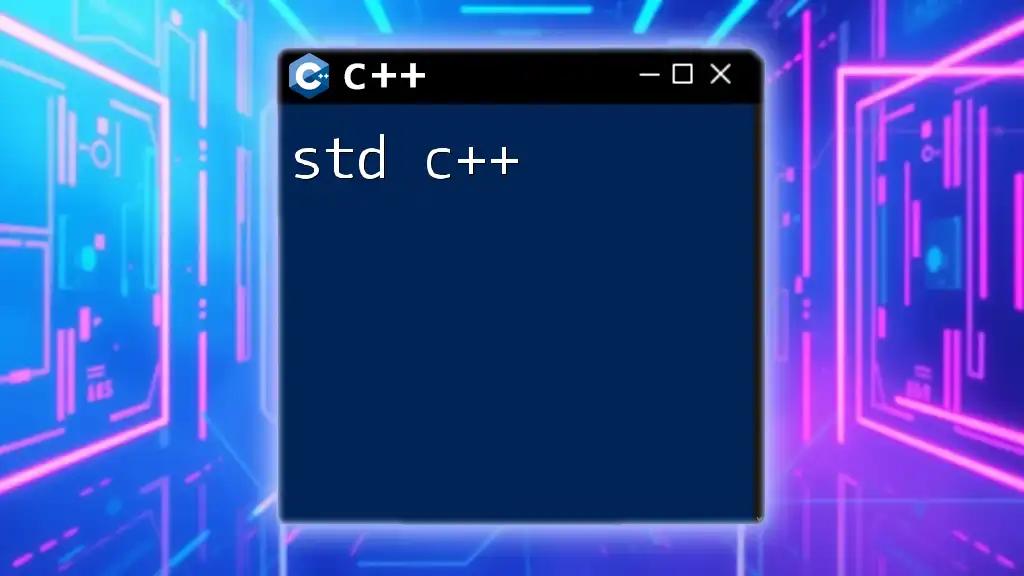
When to Use stof in C++
The stof c++ function is incredibly versatile and can be utilized in several scenarios:
-
Reading User Input: When collecting data from users, inputs are typically in string format. Using `stof`, you can easily convert that input into a floating-point number for calculations.
-
Parsing Data from Files: When reading from files, especially in configurations or data logs, numeric values are often represented as strings. `stof` simplifies the extraction of these values.
-
Performing Calculations: If you're working with expressions or formulas that come in string format, converting them into floats using `stof` allows you to perform arithmetic operations seamlessly.
Comparison with Similar Functions
It's important to understand how `stof` stacks up against similar functions:
-
stof vs. atoi: The `atoi` function converts strings to integers, whereas `stof` is specifically designed for floating-point numbers. If you need decimal precision, `stof` is the clear choice.
-
stof vs. strtof: While `strtof` is also used for converting strings to floats, it is a C-style string function. `stof` is more modern, part of C++'s richer set of string handling functions, and provides better integration with C++ string types.
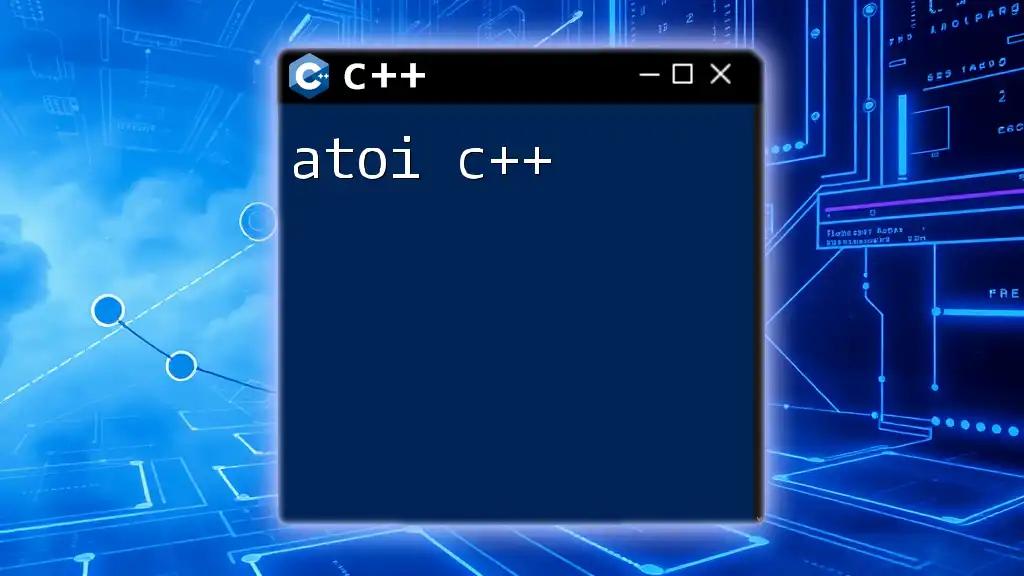
Code Examples Using stof
Basic Conversion Example
To convert a simple string representation of a number into a float, you can use the following code:
#include <iostream>
#include <string>
int main() {
std::string numStr = "3.14";
float num = std::stof(numStr);
std::cout << "Converted float: " << num << std::endl;
return 0;
}
In this code snippet, the string `"3.14"` is successfully converted to a floating-point number, and the output would be:
Converted float: 3.14
Handling Invalid Input
One of the key aspects of working with `stof` is handling potential invalid input. Here's how you can manage exceptions:
#include <iostream>
#include <string>
int main() {
std::string invalidNum = "abc";
try {
float num = std::stof(invalidNum);
} catch (const std::invalid_argument& e) {
std::cout << "Invalid argument: " << e.what() << std::endl;
}
return 0;
}
In this snippet, attempting to convert a non-numeric string results in an exception being caught, preventing the program from crashing and providing useful feedback regarding the error.
Using the idx Parameter
The `idx` parameter can be very handy when you're dealing with strings that contain additional characters after the float value. Here's an example:
#include <iostream>
#include <string>
int main() {
std::string strNum = "3.14abc";
std::size_t pos;
float num = std::stof(strNum, &pos);
std::cout << "Converted float: " << num << ", next character position: " << pos << std::endl;
return 0;
}
In this case, the output will not only show the converted float but also the position of the first unprocessed character, which can be useful for further parsing.
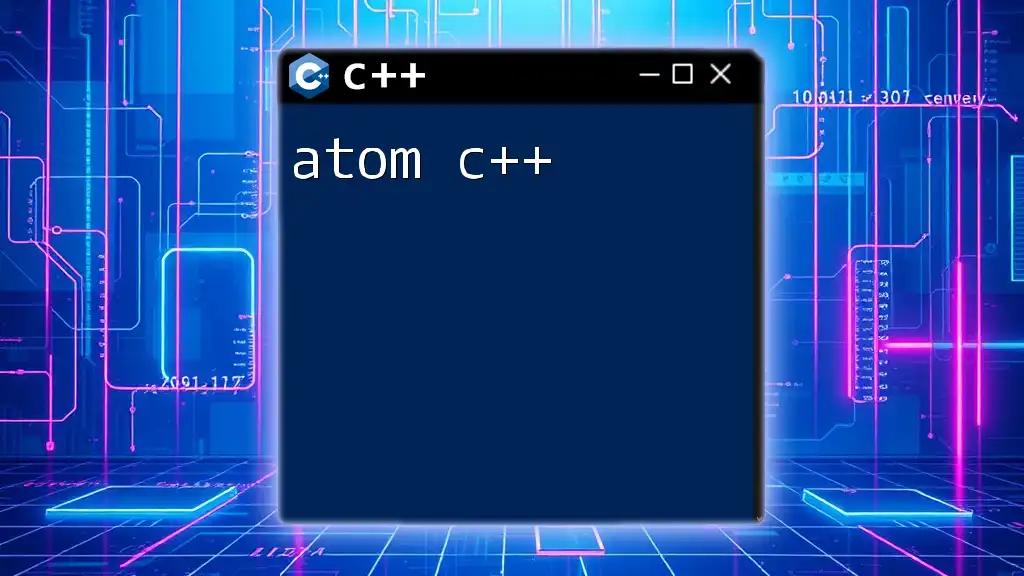
Possible Errors with stof
While `stof` is powerful, it can lead to errors that require careful handling. The most common exceptions include:
-
std::invalid_argument: This occurs when the string does not contain a valid representation of a floating-point number (e.g., trying to convert "abc").
-
std::out_of_range: This happens when the converted number is too large or too small to fit in a float. It's essential to always consider the range of input values you might encounter.
To safeguard your application, you should implement robust exception handling whenever using stof c++.
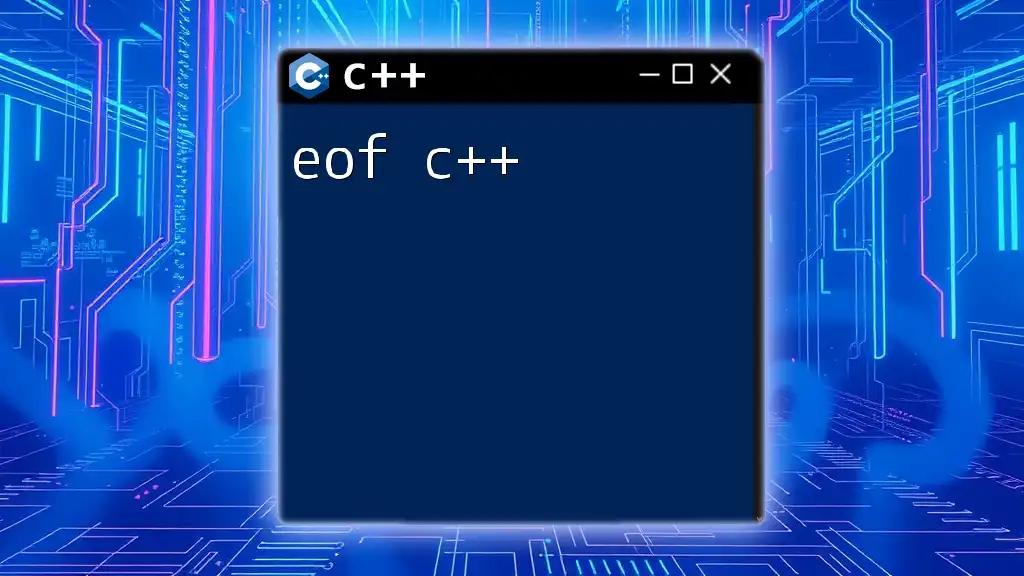
Best Practices for Using stof
When employing `stof`, consider the following best practices:
-
Validation: Always validate the input before passing it to `stof`. Ensure that the string actually can represent a float.
-
Exception Handling: Wrap your `stof` calls in try-catch blocks to gracefully handle any conversion errors.
-
Performance Considerations: If you're dealing with large datasets, analyze whether frequent conversions are needed. Batch processing or validating beforehand may enhance performance.
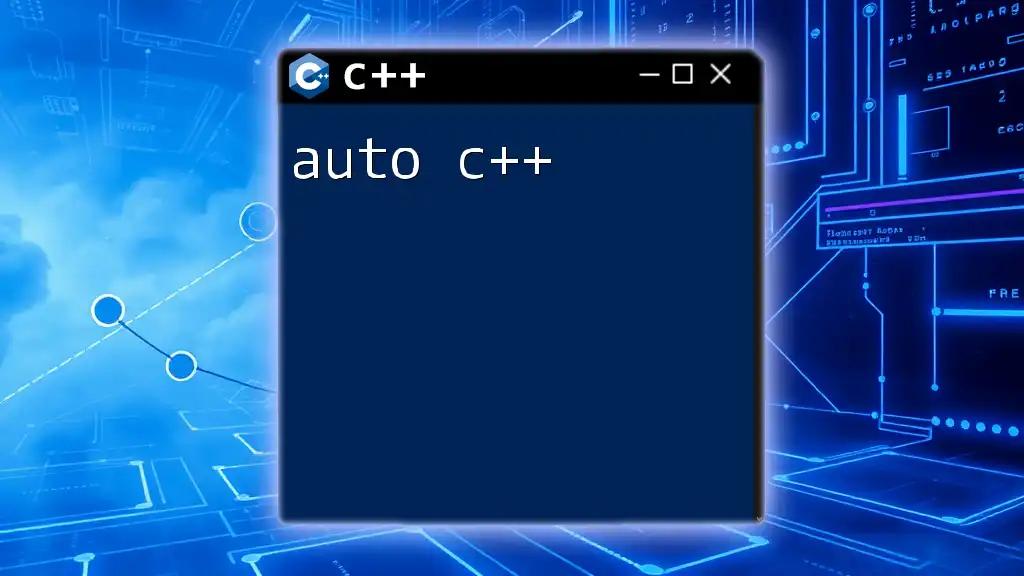
Conclusion
Understanding the stof c++ function is vital for any developer dealing with string to float conversions in C++. By grasping its syntax, potential errors, and best usage practices, you can significantly improve your data handling capabilities in C++ programming.
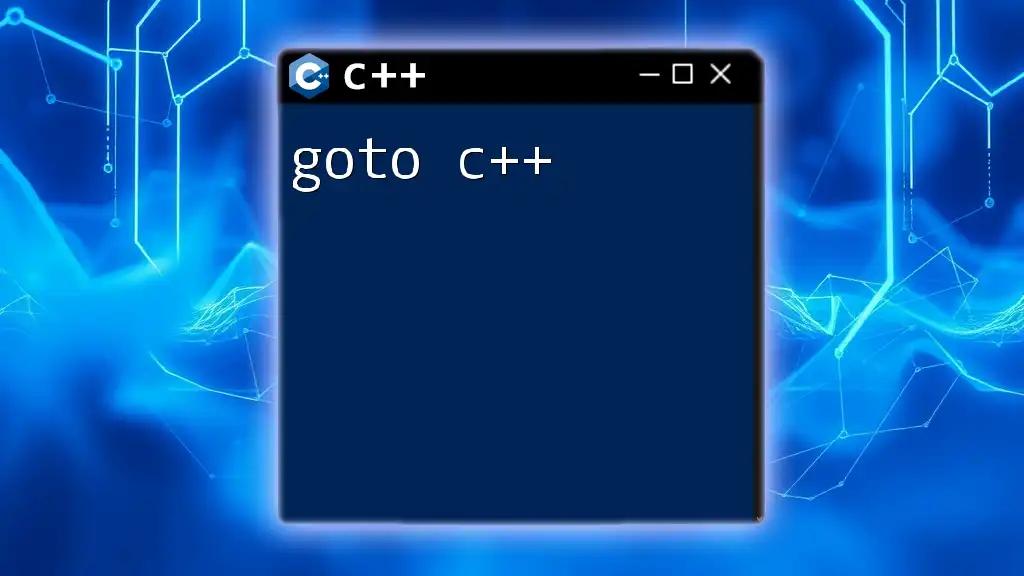
Additional Resources
For further exploration, check out the official C++ documentation, recommended textbooks, and online tutorials that delve deeper into the C++ language's features. Participating in community forums can also facilitate knowledge sharing and skill enhancement.

Call to Action
Try implementing the examples in this guide with different inputs, and remember to share your thoughts and experiences. Explore our related courses to deepen your expertise in C++ commands and techniques!