Dijkstra's algorithm is a graph search algorithm that finds the shortest path from a starting vertex to all other vertices in a weighted graph, using C++ for implementation.
Here’s a simple C++ code snippet demonstrating Dijkstra's algorithm:
#include <iostream>
#include <vector>
#include <queue>
#include <utility>
#include <limits>
using namespace std;
void dijkstra(int start, const vector<vector<pair<int, int>>>& graph) {
vector<int> dist(graph.size(), numeric_limits<int>::max());
dist[start] = 0;
priority_queue<pair<int, int>, vector<pair<int, int>>, greater<pair<int, int>>> pq;
pq.push({0, start});
while (!pq.empty()) {
int currentDist = pq.top().first;
int currentNode = pq.top().second;
pq.pop();
if (currentDist > dist[currentNode]) continue;
for (const auto& neighbor : graph[currentNode]) {
int nextNode = neighbor.first;
int weight = neighbor.second;
if (dist[currentNode] + weight < dist[nextNode]) {
dist[nextNode] = dist[currentNode] + weight;
pq.push({dist[nextNode], nextNode});
}
}
}
for (int i = 0; i < dist.size(); i++) {
cout << "Distance from node " << start << " to node " << i << " is " << dist[i] << endl;
}
}
Understanding Dijkstra's Algorithm in C++
What is Dijkstra's Algorithm?
Dijkstra's Algorithm is a classic algorithm used for finding the shortest path in a graph, which may represent, for example, road networks. Named after its inventor Edsger W. Dijkstra, this algorithm is essential in various applications including GPS navigation and network routing. It finds the shortest path from a starting node to all other nodes in a weighted graph with non-negative edges.
Key Concepts
Understanding Dijkstra’s algorithm requires some basic knowledge of graph theory. A graph consists of vertices (or nodes) and edges (connections between nodes). The shortest path problem seeks to determine the minimum distance from a source node to other nodes in a graph.
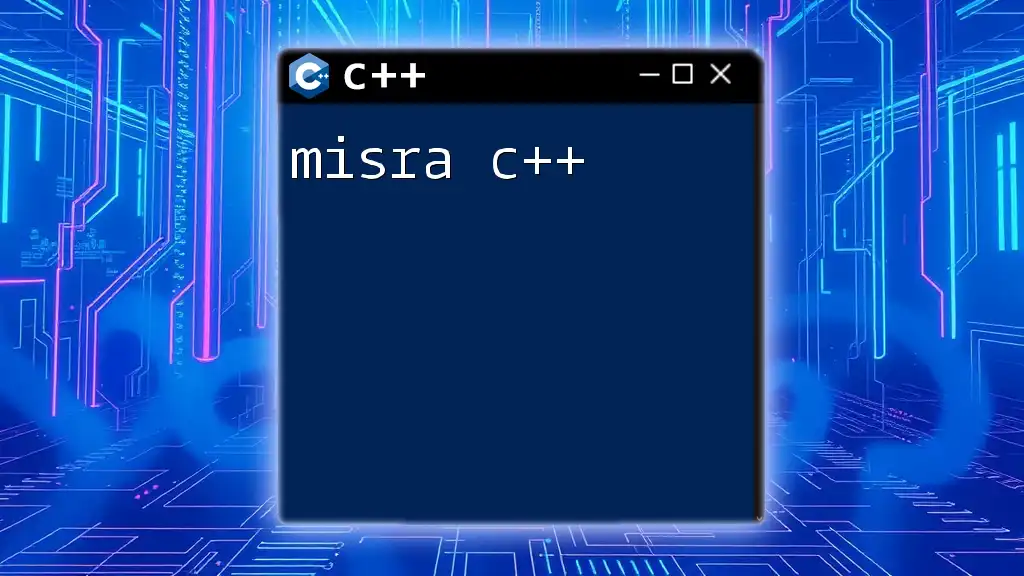
How Dijkstra’s Algorithm Works
The algorithm operates on the principle of progressively exploring the closest node that hasn’t been visited yet. The main steps include:
- Initialization: Set the initial distance to the source node as 0 and to all other nodes as infinity.
- Priority Queue: Utilize a priority queue to select the unvisited node with the smallest tentative distance.
- Relaxing edges: For the current node, examine its neighbors and calculate their tentative distances. If a shorter path is found, update the distance.
Complexity Analysis
Understanding the time and space complexity of Dijkstra's algorithm is crucial for performance analysis:
- Time Complexity: The time complexity primarily depends on the data structure used for the priority queue. Using a binary heap results in \(O(E \log V)\), where \(E\) is the number of edges and \(V\) is the number of vertices.
- Space Complexity: The space complexity is \(O(V)\) for storing distances and the priority queue.
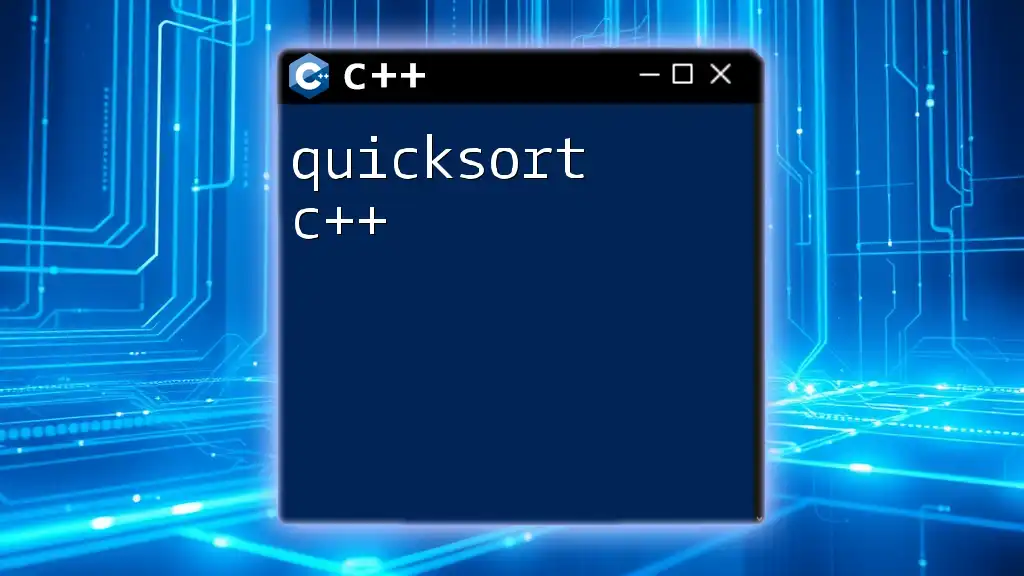
Implementing Dijkstra's Algorithm in C++
Setting Up Your C++ Environment
Before diving into coding Dijkstra's algorithm, it's essential to have the right C++ environment. You can use any IDE such as Visual Studio, Code::Blocks, or even a simple text editor coupled with a command line compiler.
Basic Structure of a C++ Program
C++ programs typically consist of headers, namespace declarations, function definitions, and execution control with the `main` function:
#include <iostream>
// Include necessary headers
using namespace std;
int main() {
// Code goes here
return 0;
}
Creating a Graph Representation
Dijkstra's algorithm requires an effective representation of a graph. You can choose between an adjacency matrix or an adjacency list. An adjacency list is more space-efficient for sparse graphs.
Here’s how to set up a graph using an adjacency list:
#include <iostream>
#include <vector>
#include <utility>
using namespace std;
class Graph {
public:
int V; // Number of vertices
vector<pair<int, int>> *adj; // Pointer to an array of adjacency lists
Graph(int V) {
this->V = V;
adj = new vector<pair<int, int>>[V]; // Initialize adjacency list
}
void addEdge(int u, int v, int w) {
adj[u].push_back(make_pair(v, w)); // Add edge (u, v) with weight w
adj[v].push_back(make_pair(u, w)); // For undirected graph
}
};
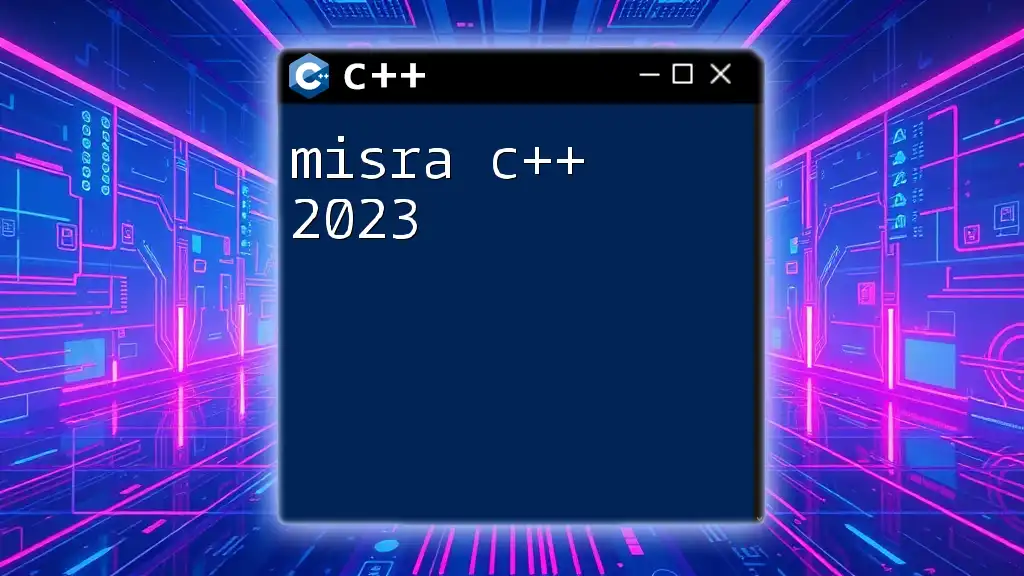
Coding Dijkstra's Algorithm in C++
Step-by-step Coding Guide
Now, let's implement Dijkstra's algorithm in C++. The essential components are initializing distances, using a priority queue, and updating distances.
Complete C++ Code Example
Here’s a full implementation of Dijkstra’s algorithm:
#include <iostream>
#include <vector>
#include <queue>
#include <limits>
using namespace std;
class Graph {
public:
int V;
vector<pair<int, int>> *adj;
Graph(int V) {
this->V = V;
adj = new vector<pair<int, int>>[V];
}
void addEdge(int u, int v, int w) {
adj[u].push_back(make_pair(v, w));
adj[v].push_back(make_pair(u, w));
}
void dijkstra(int src) {
priority_queue<pair<int, int>, vector<pair<int, int>>, greater<pair<int, int>>> pq;
vector<int> dist(V, numeric_limits<int>::max());
pq.push(make_pair(0, src));
dist[src] = 0;
while (!pq.empty()) {
int u = pq.top().second;
pq.pop();
for (auto it : adj[u]) {
int v = it.first;
int weight = it.second;
if (dist[u] + weight < dist[v]) {
dist[v] = dist[u] + weight;
pq.push(make_pair(dist[v], v));
}
}
}
// Print distances
for (int i = 0; i < V; i++) {
cout << "Distance from source to vertex " << i << " is " << dist[i] << endl;
}
}
};
int main() {
Graph g(5); // Create a graph with 5 vertices
g.addEdge(0, 1, 10);
g.addEdge(0, 4, 5);
g.addEdge(1, 2, 1);
g.addEdge(1, 4, 2);
g.addEdge(2, 3, 4);
g.addEdge(3, 0, 7);
g.addEdge(4, 1, 3);
g.addEdge(4, 2, 9);
g.addEdge(4, 3, 2);
g.dijkstra(0); // Call Dijkstra's algorithm from the source vertex 0
return 0;
}
The program begins by initializing the graph and adding edges between the vertices along with their weights. The `dijkstra` method implements the algorithm logic, utilizing a priority queue to determine the shortest distances efficiently. After calculating the shortest paths, it prints the distances to all vertices from the source.
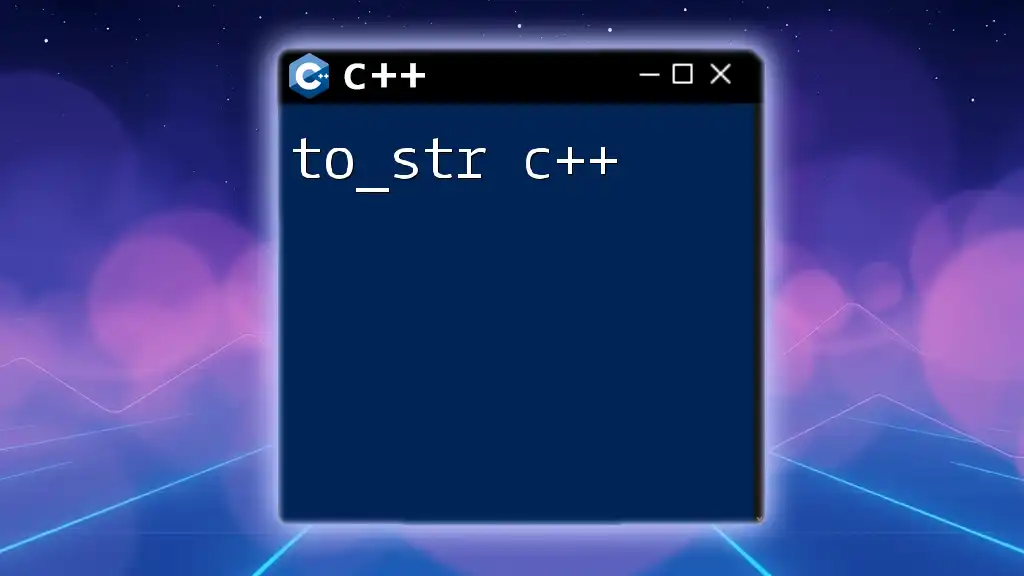
Use Cases and Practical Applications
Dijkstra’s Algorithm has numerous real-world applications. For instance, in networking, it is often used for routing protocols like OSPF (Open Shortest Path First) that determine the shortest paths for data packets. In GPS systems, Dijkstra's Algorithm aids in finding the fastest routes to quantify travel times based on current locations and destinations.
Case Study: Implementing Dijkstra’s Algorithm in a Project
Consider a scenario where a logistics company needs to optimize delivery routes for its fleet. By utilizing Dijkstra's algorithm, the company can determine the quickest path between various distribution centers, leading to reduced fuel costs and faster delivery times. Implementing the algorithm allowed the company to strategically plan its routes, leading to efficiency gains.
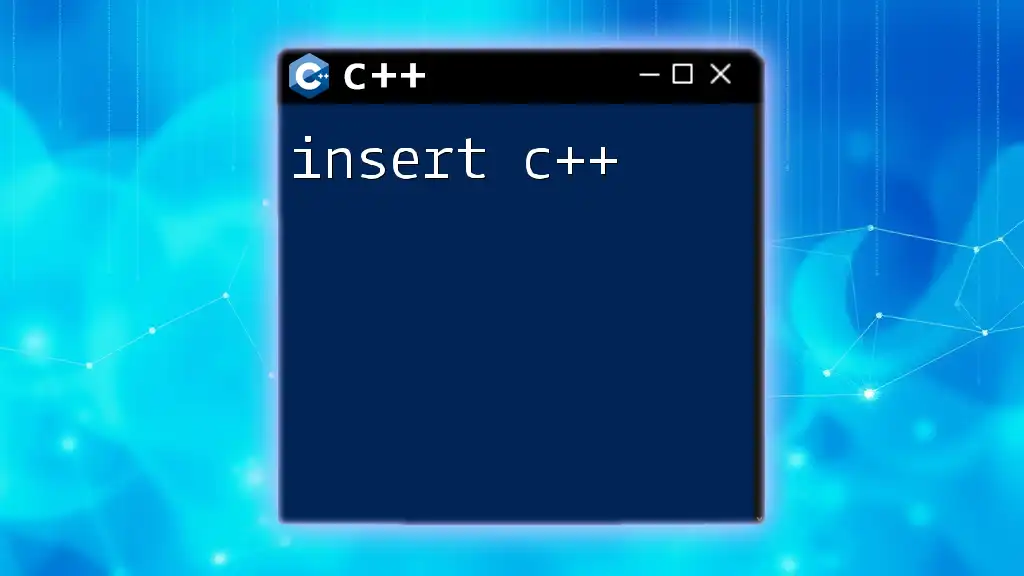
Troubleshooting Common Issues
When implementing Dijkstra's algorithm, you might encounter several challenges. Debugging Errors are common, such as infinite loops or incorrect distance calculations. One effective debugging technique in C++ includes printing the graph structure before and after adding edges.
Optimization Techniques
Dijkstra's algorithm can become inefficient for very large graphs. Using advanced data structures like Fibonacci heaps can help improve its efficiency significantly, leading to better performance in terms of both time and space.
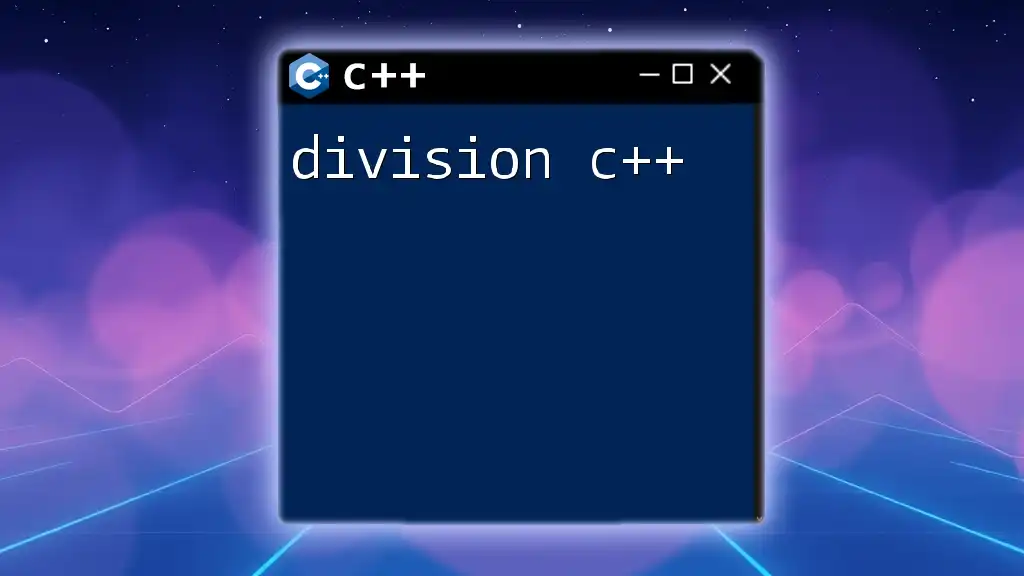
Conclusion
Dijkstra’s Algorithm is a fundamental concept in computer science, especially in the realm of graph theory. By implementing this algorithm in C++, you can unlock powerful capabilities for solving real-world problems involving shortest path calculations. Experiment with the code examples provided, modify them to fit your needs, and practice with various graph structures to enhance your understanding of Dijkstra in C++.
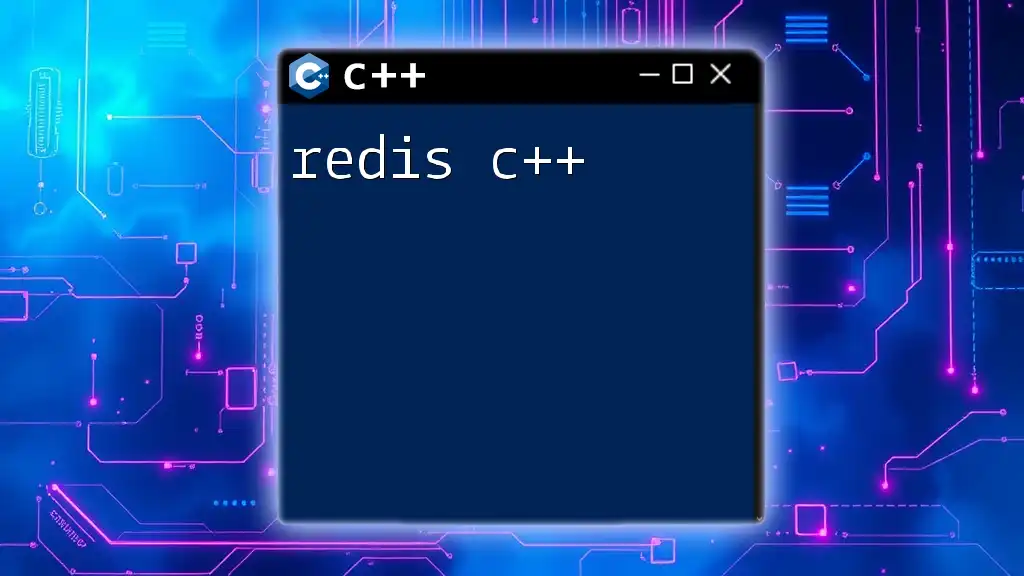
Additional Resources
To deepen your understanding of Dijkstra's algorithm and its applications, consider exploring additional literature and online courses. Engaging with programming communities through forums will also provide valuable insights and support as you venture into the fascinating realm of algorithms.
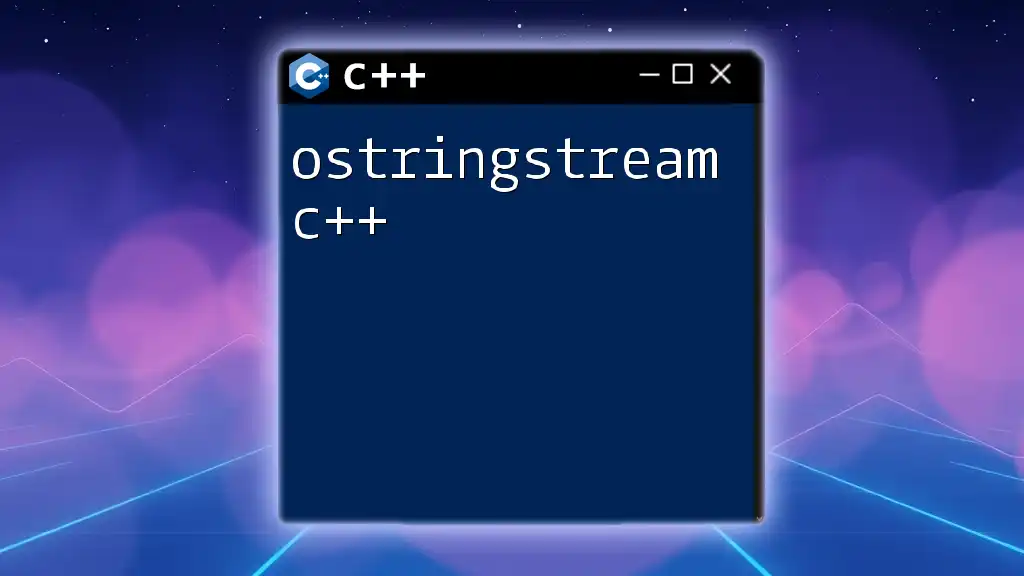
FAQs on Dijkstra's Algorithm in C++
What are the limitations of Dijkstra's Algorithm?
While highly effective, Dijkstra's algorithm cannot handle graphs with negative weight edges because it relies on the premise that once a vertex is visited, its shortest path value is finalized.
How does Dijkstra's Algorithm compare to other pathfinding algorithms?
Compared to algorithms like Bellman-Ford, which can handle negative weights, Dijkstra’s algorithm is more efficient for graphs with non-negative weights due to its greedy approach.
Can Dijkstra's Algorithm handle negative weight edges?
No, Dijkstra's algorithm cannot properly manage negative edge weights. If negative weights are present, consider using the Bellman-Ford algorithm instead.