Redis is an in-memory data structure store that can be easily accessed and manipulated using C++ through a client library like Hiredis.
#include <hiredis/hiredis.h>
int main() {
redisContext *c = redisConnect("127.0.0.1", 6379);
if (c != NULL && c->err) {
printf("Error: %s\n", c->errstr);
// Handle error
}
redisCommand(c, "SET foo bar");
char *value = redisCommand(c, "GET foo")->str;
printf("Value of foo: %s\n", value);
redisFree(c);
return 0;
}
What is Redis?
Redis is an open-source, in-memory data structure store that is often used as a database, cache, and message broker. Known for its high performance and flexibility, Redis supports various data types such as strings, hashes, lists, and sets, making it suitable for a wide range of applications, from simple key-value storage to complex data manipulation tasks.

Why Use C++ with Redis?
Using C++ with Redis harnesses the advantages of a powerful programming language while leveraging Redis’s capabilities. Some key reasons include:
-
Performance: C++ is a compiled language that offers high execution speed and low-latency access, making it ideal for applications requiring rapid data processing.
-
Control over Resources: C++ allows fine-grained control over system resources, which is important in high-performance applications dealing with large datasets and intricate operations.
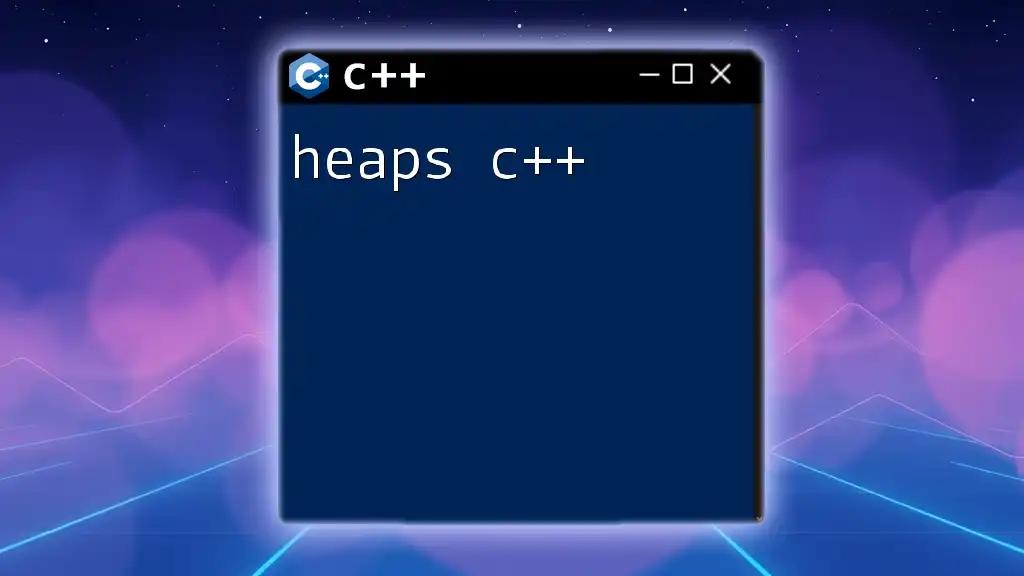
Getting Started with Redis in C++
Setting Up Your Development Environment
Before you start programming with Redis in C++, you need to ensure your development environment is set up correctly.
Required Tools and Libraries:
- Redis Server: You can download Redis from the official Redis website.
- hiredis: This is a minimalistic C client library for Redis that simplifies interaction with Redis from C/C++ code.
How to Install Redis: You can install Redis on your machine following these steps:
- Download the latest stable version of Redis.
- Extract the files and navigate to the Redis directory.
- Compile it using the `make` command.
- Start the Redis server using `src/redis-server`.
Installing the hiredis library: To install hiredis, you can also clone it from GitHub and compile it:
git clone https://github.com/redis/hiredis.git
cd hiredis
make
sudo make install
Connecting to a Redis Server
Connecting to a Redis server is straightforward with hiredis. Below is a small code snippet demonstrating how to do it:
#include <hiredis/hiredis.h>
int main() {
redisContext *c = redisConnect("127.0.0.1", 6379);
if (c == nullptr || c->err) {
if (c) {
printf("Error: %s\n", c->errstr);
redisFree(c);
} else {
printf("Can't allocate redis context\n");
}
return 1;
}
printf("Connected to Redis\n");
redisFree(c);
return 0;
}
In this example, the code attempts to connect to a Redis server running on the localhost. If the connection is successful, a message is printed out. If there is an error, it handles it gracefully by freeing up the allocated resources.
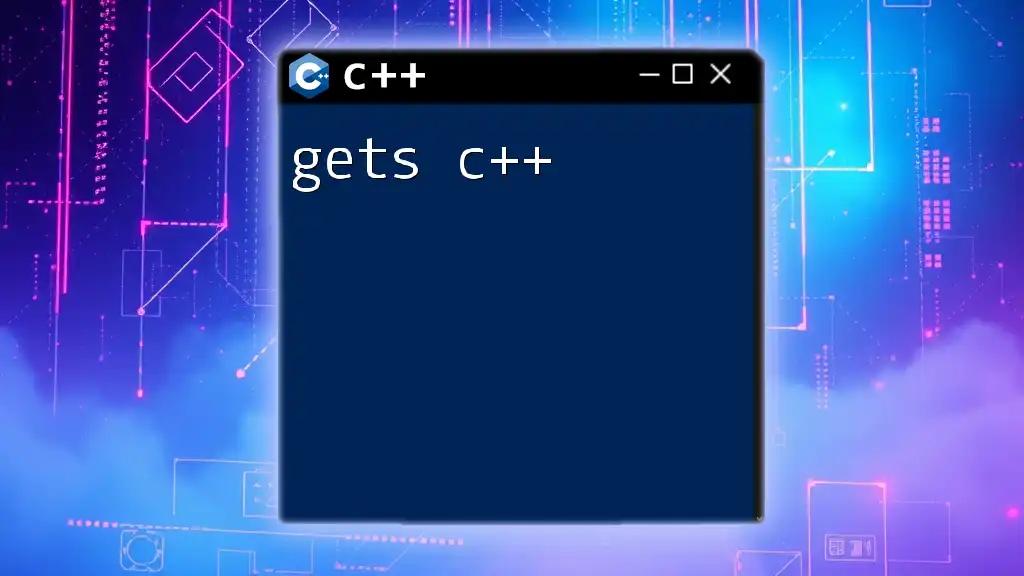
Basic Redis Commands in C++
Setting and Getting Values
Once connected, you can start interacting with Redis using various commands. Here's how to set and retrieve a value:
#include <hiredis/hiredis.h>
int main() {
redisContext *c = redisConnect("127.0.0.1", 6379);
redisCommand(c, "SET key %s", "value");
const char* val = redisCommand(c, "GET key")->str;
printf("Retrieved value: %s\n", val);
redisFree(c); // Clean up
return 0;
}
In this code, the `SET` command stores a string under the key `key`, and the `GET` command retrieves this value. Understanding the command-response pattern is crucial in handling Redis effectively.
Working with Key-Value Pairs
Storing Strings
With Redis, strings are the simplest data type and can be manipulated easily. You can perform operations like incrementing a string:
redisCommand(c, "INCR count");
const char* count = redisCommand(c, "GET count")->str;
printf("Count: %s\n", count);
Storing Lists
Lists in Redis allow you to store sequences of strings. You can push items onto a list and retrieve them:
redisCommand(c, "LPUSH mylist %s", "value1");
redisCommand(c, "LPUSH mylist %s", "value2");
redisCommand(c, "LPUSH mylist %s", "value3");
// Retrieve the list
redisAppendCommand(c, "LRANGE mylist 0 -1");
Storing Hashes
Hashes are perfect for representing objects composed of multiple fields. Below is how you can set and get hash values:
redisCommand(c, "HSET user:1000 name %s", "John Doe");
const char* name = redisCommand(c, "HGET user:1000 name")->str;
printf("User Name: %s\n", name);
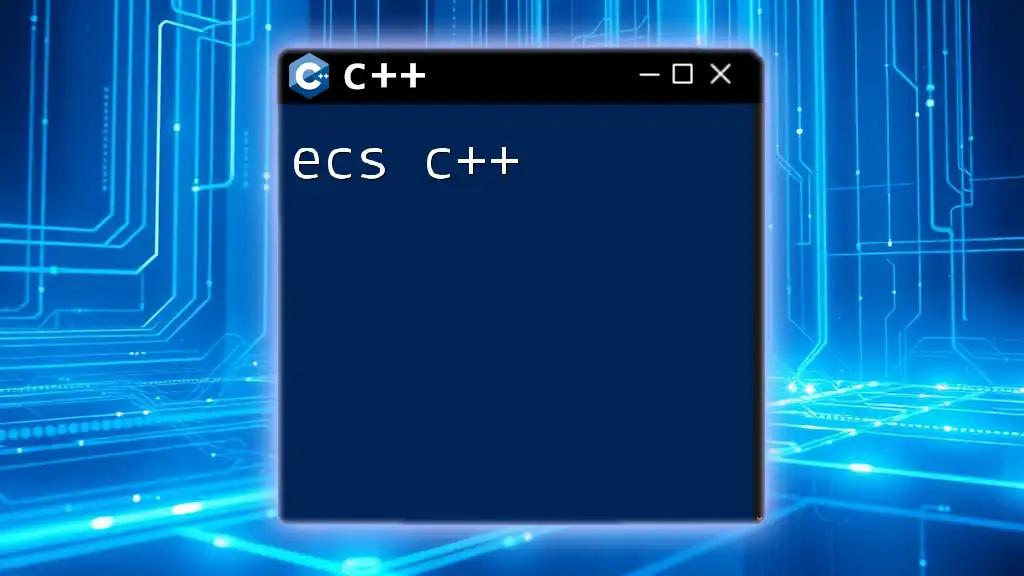
Advanced Redis Features
Transactions and Pipelining
Redis supports transactions through commands like MULTI and EXEC. Transactions are beneficial when you need to ensure all commands execute successfully:
redisAppendCommand(c, "MULTI");
redisAppendCommand(c, "SET key1 %s", "value1");
redisAppendCommand(c, "SET key2 %s", "value2");
redisGetReply(c, nullptr); // Executes transaction
Pub/Sub Messaging
Redis's Pub/Sub feature enables messaging between different parts of a system. Subscribing and publishing messages is seamless:
redisCommand(c, "SUBSCRIBE channel1");
redisCommand(c, "PUBLISH channel1 %s", "Hello Subscribers!");
This is particularly useful in event-driven architectures where components need to react to certain events asynchronously.
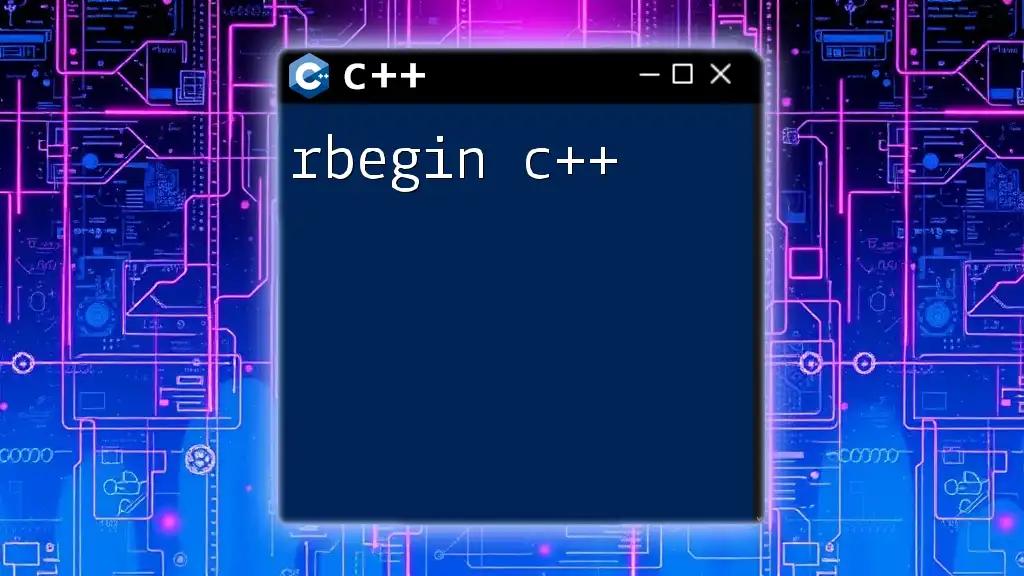
Error Handling and Debugging
Common Issues When Using Redis with C++
When working with Redis in C++, users might encounter issues such as:
- Connection problems: Ensure your Redis server is running and reachable.
- Syntax errors: Monitor the command structure and parameters passed to commands.
- Memory management: With C++, managing memory allocation and deallocation is critical to avoid memory leaks.
Best Practices for Debugging Redis in C++
Debugging in Redis can be facilitated by:
- Logging: Implementing logging at various points in your application helps trace where errors may be happening.
- Using Redis CLI: Testing commands directly in the Redis CLI can help ensure they work before implementing them in code.

Optimizing Performance
Redis Configuration Options
To achieve optimal performance, tweak configurations in `redis.conf`, such as:
- Maxmemory: Adjust this setting according to your available RAM.
- Persistence settings: Analyze your needs for RDB and AOF persistence.
Efficient Use of Memory in C++ with Redis
Managing memory effectively in C++ can significantly impact your application. Utilize smart pointers to avoid memory leaks and ensure efficient memory usage. Always free up resources when they are no longer needed.
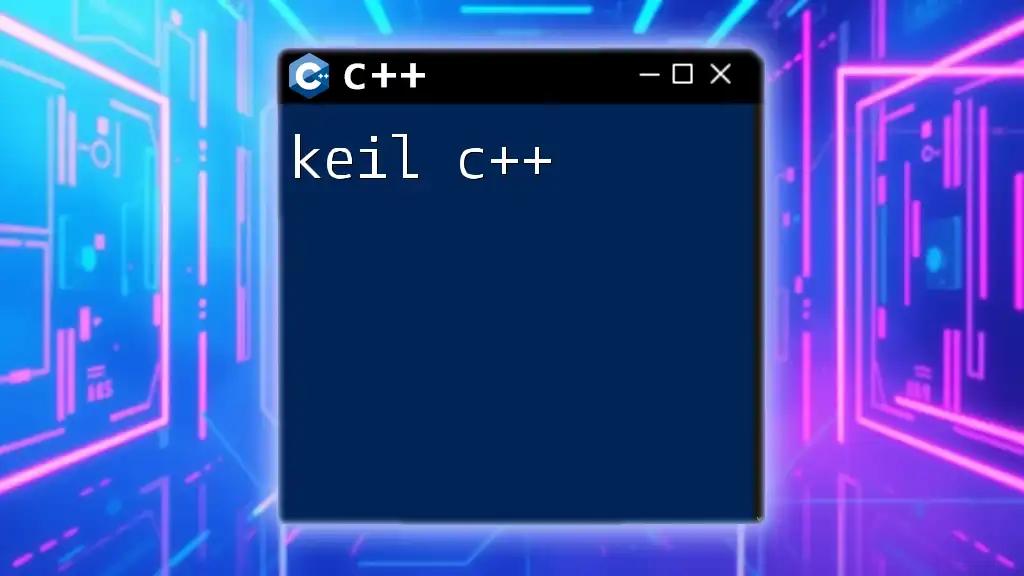
Conclusion
Redis paired with C++ opens doors to developing high-performance applications designed to handle large volumes of data with ease. With this guide, you are equipped to connect, manipulate data, and take advantage of Redis's features efficiently. Experiment with various commands and configurations to discover the full potential of Redis C++ interaction.