The `.begin()` function in C++ is used to obtain an iterator pointing to the first element of a container, such as a vector or a list.
Here’s a code snippet demonstrating its use with a `std::vector`:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
auto it = numbers.begin(); // Getting an iterator to the first element
std::cout << *it << std::endl; // Output: 1
return 0;
}
Understanding the Basics of C++ Containers
In C++, containers are essential structures that store and manage data efficiently. Each container has its unique properties and use cases. Some of the most common containers include:
- Vector: A dynamic array that can grow or shrink in size.
- List: A doubly linked list that allows for efficient insertions and deletions.
- Deque: A double-ended queue that supports fast insertions and deletions at both ends.
- Set: A collection of unique elements, automatically sorted.
- Map: A collection of key-value pairs that allows for fast retrieval of values based on keys.
Why Use Iterators?
Iterators are a powerful feature in C++ that provide an interface for traversing the elements of a container. They act similarly to pointers, allowing you to iterate through the data structures. The main benefits of using iterators are:
- Consistency: Iterators provide a unified way to access different container types.
- Efficiency: With iterators, you can traverse containers without needing to expose the underlying data structure directly.
- Flexibility: They allow for algorithms that work with any iterable collection, enhancing code reuse.
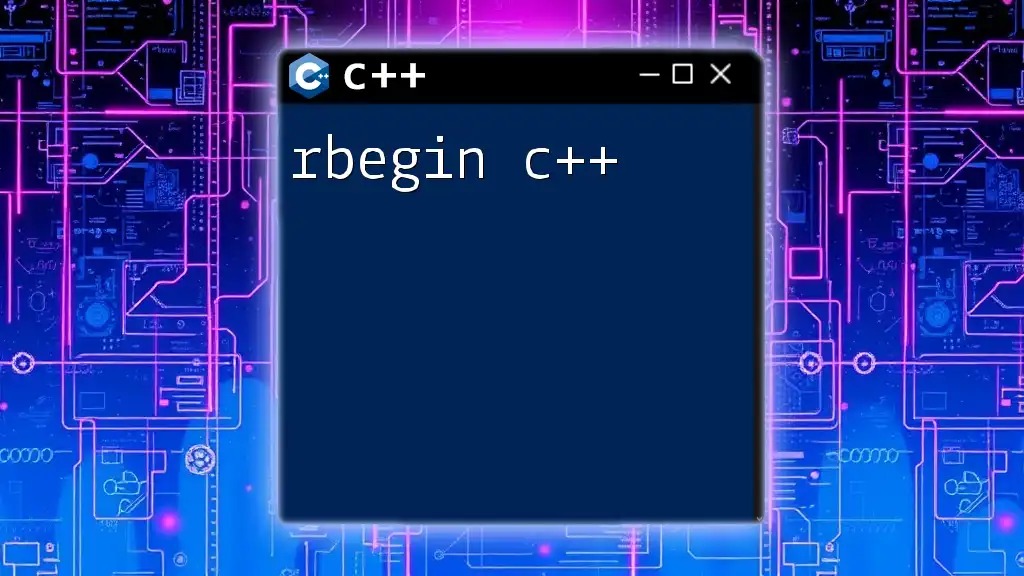
The .begin() Function in C++
What Does .begin() Do?
The `.begin()` function is an integral part of C++'s container manipulation. It returns an iterator that points to the first element of the container. If the container is empty, the behavior will depend on the specific container type, but dereferencing the returned iterator will lead to undefined behavior.
Syntax of .begin()
container.begin();
This straightforward syntax enables access to the first element in various containers, making it a critical function in C++ programming.
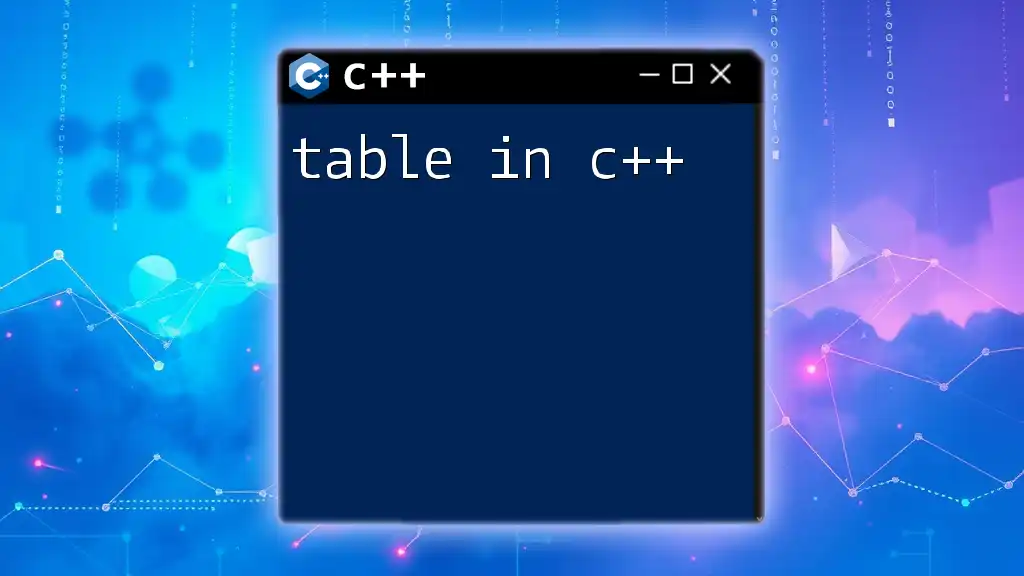
How to Use .begin() with Different Containers
Using .begin() with Vectors
Vectors are one of the most commonly used containers due to their dynamic sizing capabilities. Here’s how to use `.begin()` with a vector:
Example of .begin() with a Vector:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
auto it = numbers.begin();
std::cout << *it << std::endl; // Output: 1
return 0;
}
In this example, `.begin()` returns an iterator pointing to the first element of the vector, and dereferencing it gives us the value `1`. This capability is particularly useful when traversing through larger datasets.
Using .begin() with Lists
Lists in C++ allow for easy insertion and removal of elements from anywhere in the sequence. Using `.begin()` with a list works in a similar fashion:
Example of .begin() with a List:
#include <iostream>
#include <list>
int main() {
std::list<std::string> fruits = {"Apple", "Banana", "Cherry"};
auto it = fruits.begin();
std::cout << *it << std::endl; // Output: Apple
return 0;
}
Here, `.begin()` returns an iterator to the first element of the list, producing `Apple`. Lists are advantageous when you require frequent insertions or deletions.
Using .begin() with Other Containers
Deque
A deque is a versatile container, which allows adding or removing elements from both the front and back efficiently.
Example of .begin() with a Deque:
#include <iostream>
#include <deque>
int main() {
std::deque<double> numbers = {1.5, 2.5, 3.5};
auto it = numbers.begin();
std::cout << *it << std::endl; // Output: 1.5
return 0;
}
In this case, using `.begin()` gives us the first element `1.5`.
Set
A set stores unique elements in a sorted manner. When using `.begin()`, it can retrieve the smallest element.
Example of .begin() with a Set:
#include <iostream>
#include <set>
int main() {
std::set<int> uniqueNumbers = {3, 1, 4};
auto it = uniqueNumbers.begin();
std::cout << *it << std::endl; // Output: 1
return 0;
}
Here, `.begin()` provides access to the smallest number in the set, which is `1`.
Map
Maps are collections of key-value pairs that offer fast retrieval using keys. Using `.begin()` with maps returns an iterator to the first key-value pair.
Example of .begin() with a Map:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> age = {{"Alice", 30}, {"Bob", 25}};
auto it = age.begin();
std::cout << it->first << ": " << it->second << std::endl; // Output: Alice: 30
return 0;
}
In this example, the first element returned by `.begin()` is the pair `"Alice": 30`.
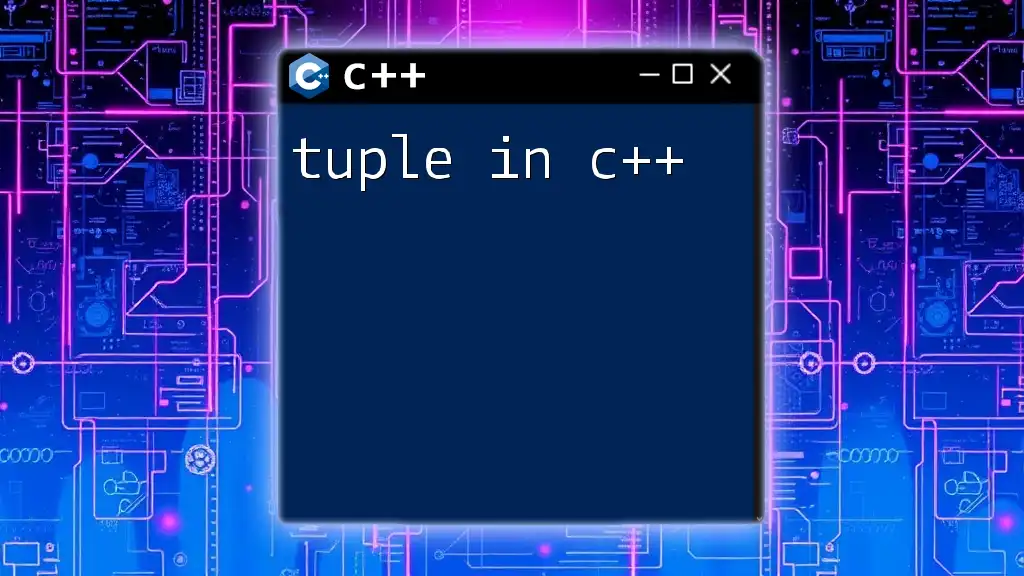
Navigating Through Containers with .begin()
Incrementing the Iterator
Once you obtain an iterator using `.begin()`, you can increment it to navigate through the elements of the container.
Example: Iterating through a Vector:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3};
for (auto it = numbers.begin(); it != numbers.end(); ++it) {
std::cout << *it << " "; // Output: 1 2 3
}
return 0;
}
In this loop, the iterator `it` starts at the beginning of the vector and increments until it reaches the end, allowing us to print each element.
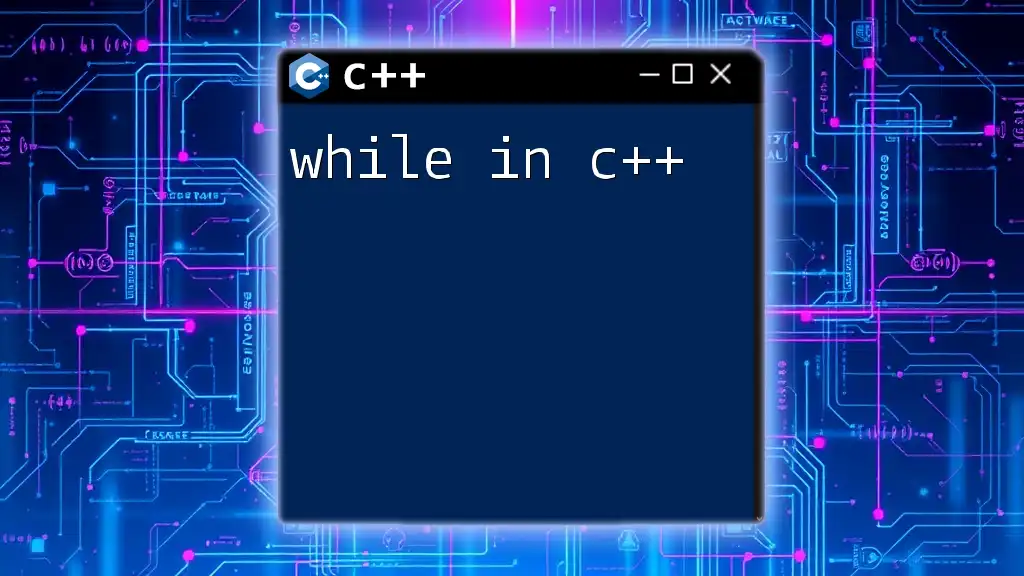
Common Mistakes and Troubleshooting
Using .begin() on Empty Containers
One common mistake is using `.begin()` on an empty container. If you try to dereference an iterator from an empty container, you will encounter undefined behavior. It is crucial to check if the container is empty before dereferencing the iterator:
if (!container.empty()) {
auto it = container.begin();
std::cout << *it;
}
Forgetting to Include Necessary Headers
Another frequent issue arises from not including the required headers for the containers you are using. Always ensure that you import the proper header files, such as:
#include <vector> // for vectors
#include <list> // for lists
#include <deque> // for deques
#include <set> // for sets
#include <map> // for maps
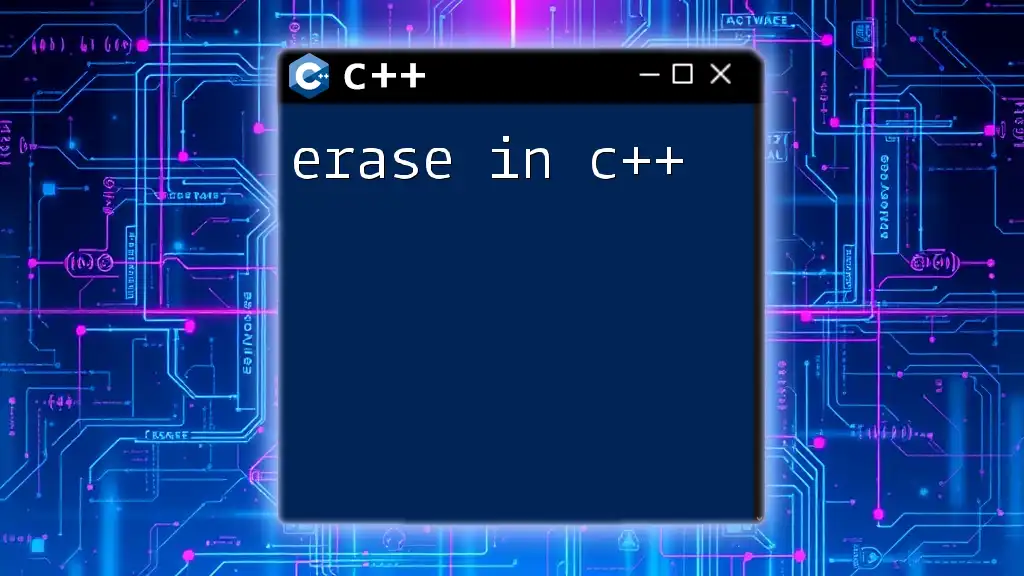
Conclusion
The `.begin()` function in C++ is a cornerstone for working with various containers. It simplifies data access and enhances the flexibility of your code. Understanding how to efficiently utilize `.begin()` with different containers will empower you as a developer to manage data effectively. Practice using `.begin()` across various containers to solidify your understanding and improve your programming skills.