A tuple in C++ is a fixed-size collection of elements of different types, allowing you to group multiple values into a single object.
#include <iostream>
#include <tuple>
int main() {
std::tuple<int, std::string, double> myTuple(1, "Hello", 3.14);
std::cout << std::get<0>(myTuple) << ", " << std::get<1>(myTuple) << ", " << std::get<2>(myTuple) << std::endl;
return 0;
}
What is a Tuple?
A tuple in C++ is a fixed-size collection of heterogeneous values, meaning it can hold multiple data types in a single construct. This feature makes tuples extremely useful for grouping related data without needing to define a separate structure or class.
Tuples are often used in situations where a function needs to return multiple values, or when you want to combine related data types into a cohesive unit. Compared to other data structures such as arrays and structs, tuples provide a more flexible way to manage different types of data without enforcing uniformity.
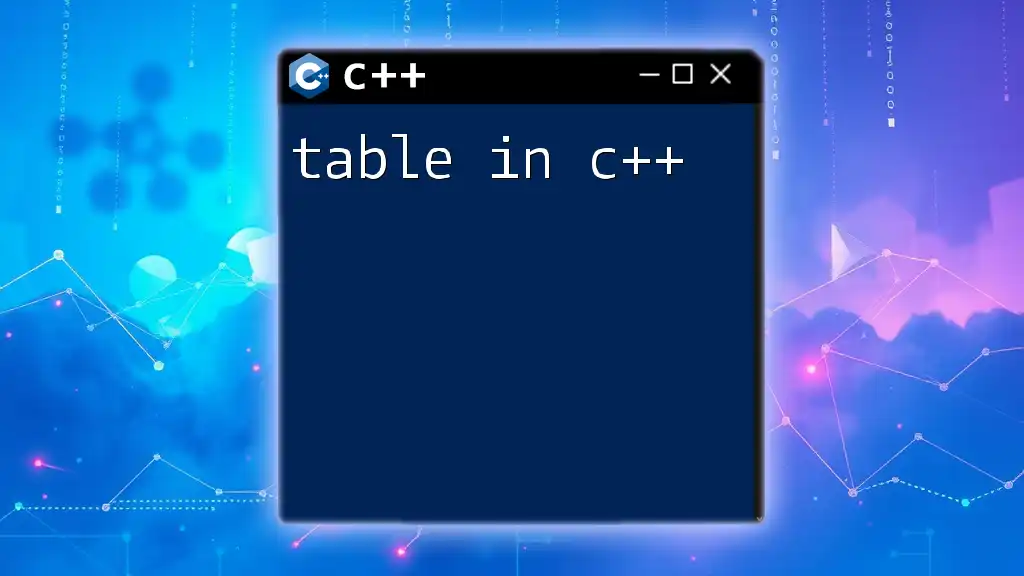
Understanding Tuples in C++
Characteristics of Tuples
-
Heterogeneous Data Types: Unlike arrays, which only hold items of the same data type, a tuple can hold various types, such as integers, doubles, strings, and more.
-
Fixed Size: Once defined, the size of a tuple is constant; you cannot add or remove elements.
-
Immutability: The elements of a tuple cannot be modified directly once set. This characteristic supports data integrity but may require workarounds for cases where data modification is necessary.
Adding Tuple Support in C++
To begin using tuples in your C++ programs, you must include the `<tuple>` header file. Adding this line at the top of your source file enables tuple functionalities:
#include <tuple>
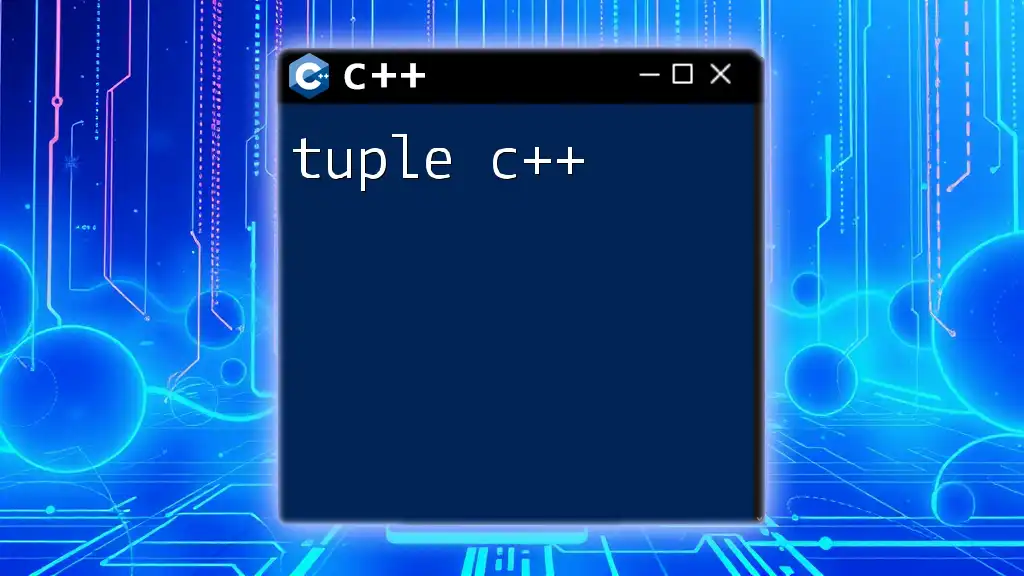
Creating and Initializing Tuples in C++
Declaring Tuples
Declaring a tuple in C++ is straightforward. You can specify the types of values it will hold in angle brackets. Here’s an example of declaring a tuple that can hold an integer, a double, and a string:
std::tuple<int, double, std::string> myTuple;
Initializing Tuples
You can initialize a tuple in a couple of ways, one of which is using `std::make_tuple`. This function automatically deduces the types based on the values you provide. Here’s how you can initialize a tuple with different data types:
auto myTuple = std::make_tuple(1, 3.14, "Hello");
This line creates a tuple containing an integer, a double, and a string. The compiler infers the types, making it convenient and efficient.
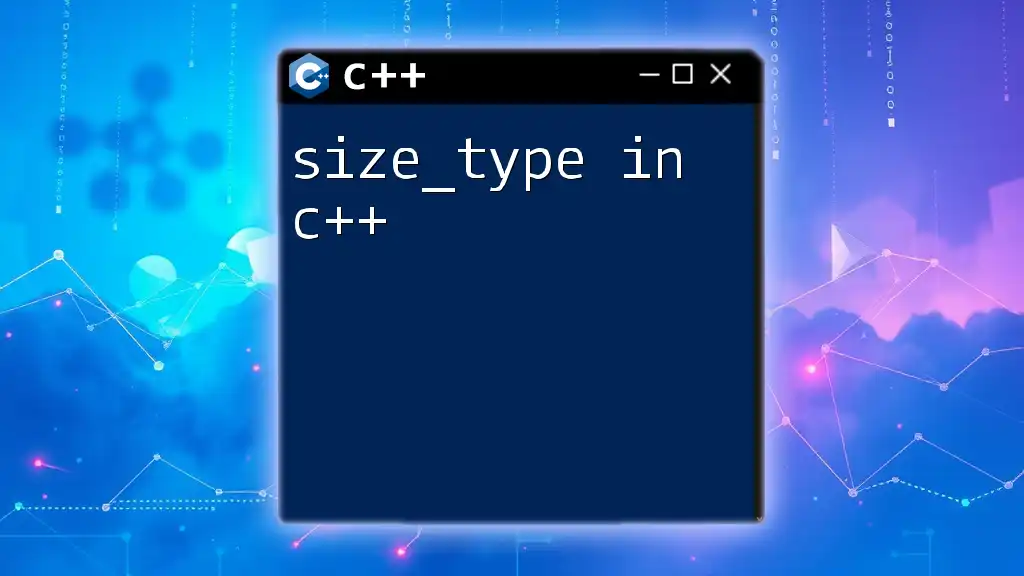
Accessing Tuple Elements
Using `std::get` to Access Elements
To access a tuple element, you use the `std::get` function along with the zero-based index of the element. Here's an example that demonstrates how to retrieve specific elements from a tuple:
#include <iostream>
#include <tuple>
std::tuple<int, double, std::string> myTuple = std::make_tuple(1, 3.14, "Hello");
int myInt = std::get<0>(myTuple); // Accessing the first element
double myDouble = std::get<1>(myTuple); // Accessing the second element
std::string myString = std::get<2>(myTuple); // Accessing the third element
std::cout << myInt << ", " << myDouble << ", " << myString << std::endl;
This example illustrates how easy it is to access the contents of a tuple using their indices.
Structured Bindings (C++17 and later)
Starting with C++17, you can use structured bindings to easily unpack the contents of a tuple into separate variables. This feature enhances code readability. Here’s how it works:
int myInt;
double myDouble;
std::string myString;
std::tie(myInt, myDouble, myString) = myTuple; // Destructuring
std::cout << myInt << ", " << myDouble << ", " << myString << std::endl;
With structured bindings, declaring individual variables becomes cleaner and intuitive.
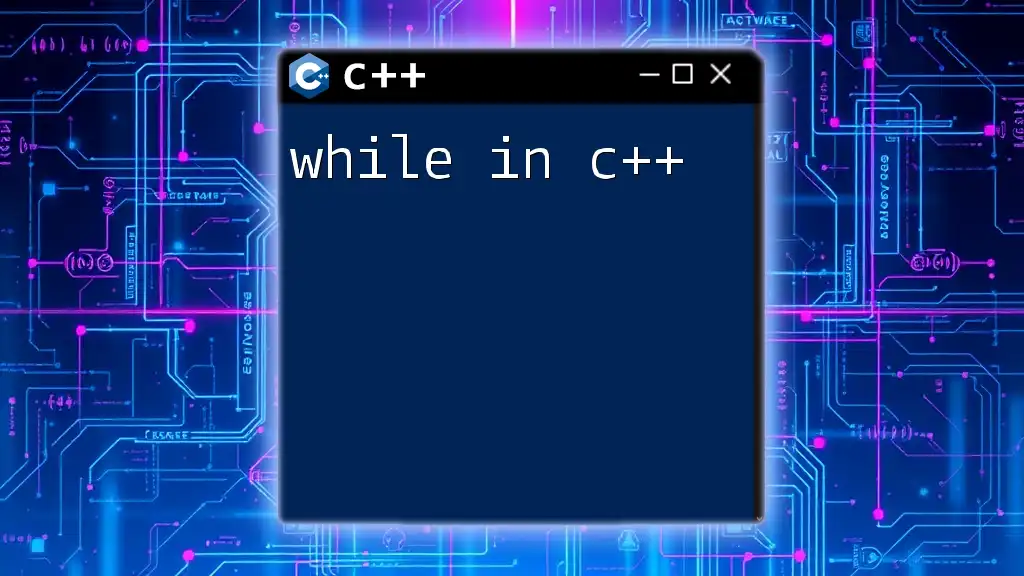
Modifying Tuples
As mentioned earlier, tuples in C++ are immutable. Once you assign values to them, you cannot change those values directly. This immutability enforces a disciplined programming approach; however, if you need to modify a tuple, you can create a new tuple instead. For example:
auto newTuple = std::make_tuple(2, 4.14, "World"); // Create a new tuple with new values
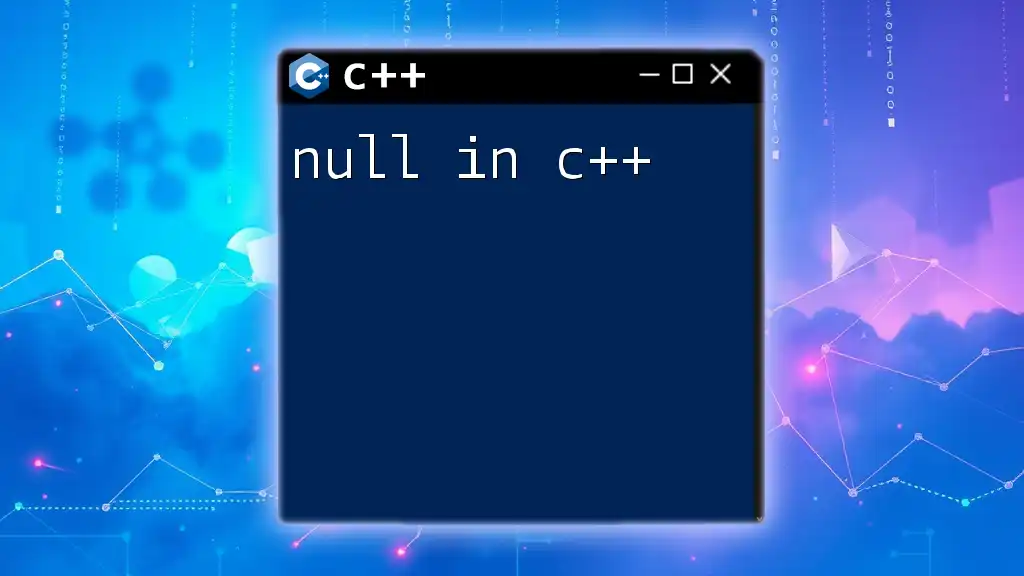
Tuple Operations
Comparing Tuples
C++ allows you to compare tuples for equality. You can use == to check if two tuples are the same, provided they have the same elements in the same order. Here’s an example:
std::tuple<int, double> tupleA = std::make_tuple(1, 3.14);
std::tuple<int, double> tupleB = std::make_tuple(1, 3.14);
if (tupleA == tupleB) {
std::cout << "Tuples are equal!" << std::endl;
}
Swapping Tuples
You can use the `std::swap` function to exchange the contents of two tuples. This comes in handy for sorting or managing collections of data. Here’s a code snippet demonstrating this capability:
std::tuple<int, double> tupleA = std::make_tuple(1, 3.14);
std::tuple<int, double> tupleB = std::make_tuple(2, 6.28);
std::swap(tupleA, tupleB); // Swaps the values of tupleA and tupleB
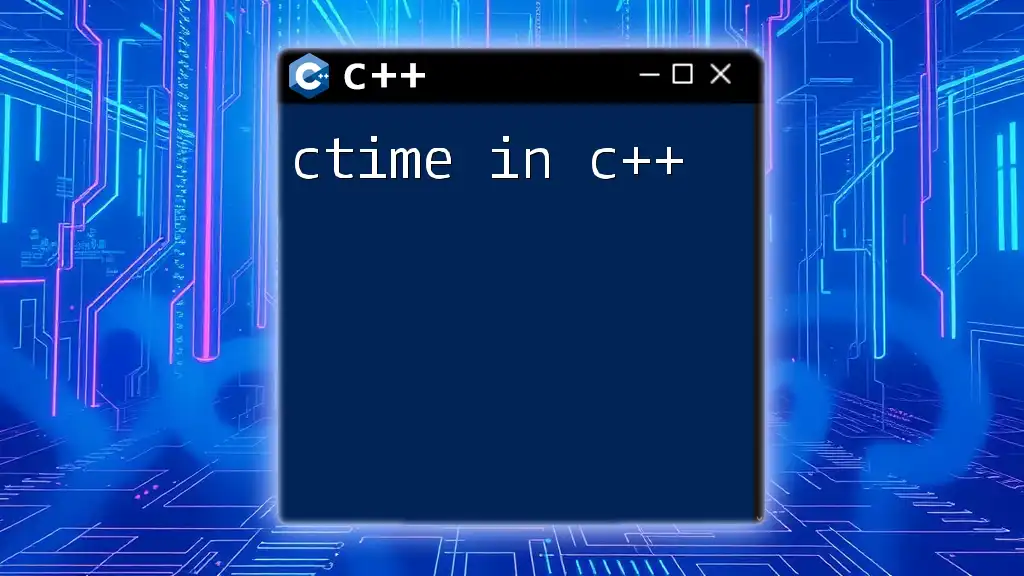
Use Cases for Tuples in C++
Storing Multiple Return Values from Functions
Tuples shine in scenarios where a function needs to return multiple values. Instead of employing multiple output parameters, you can encapsulate them in a tuple. Here’s how to achieve this:
std::tuple<int, double> computeValues() {
return std::make_tuple(1, 3.14);
}
You can then retrieve the result as follows:
auto [value1, value2] = computeValues();
std::cout << value1 << ", " << value2 << std::endl;
Using Tuples for Data Grouping
Tuples allow you to group related data together, making your code cleaner. For instance, when representing a point in a 2D space, you might use a tuple like this:
std::tuple<double, double> point = std::make_tuple(10.5, 20.5);
This simple representation avoids creating an entire class or structure solely for holding coordinates.
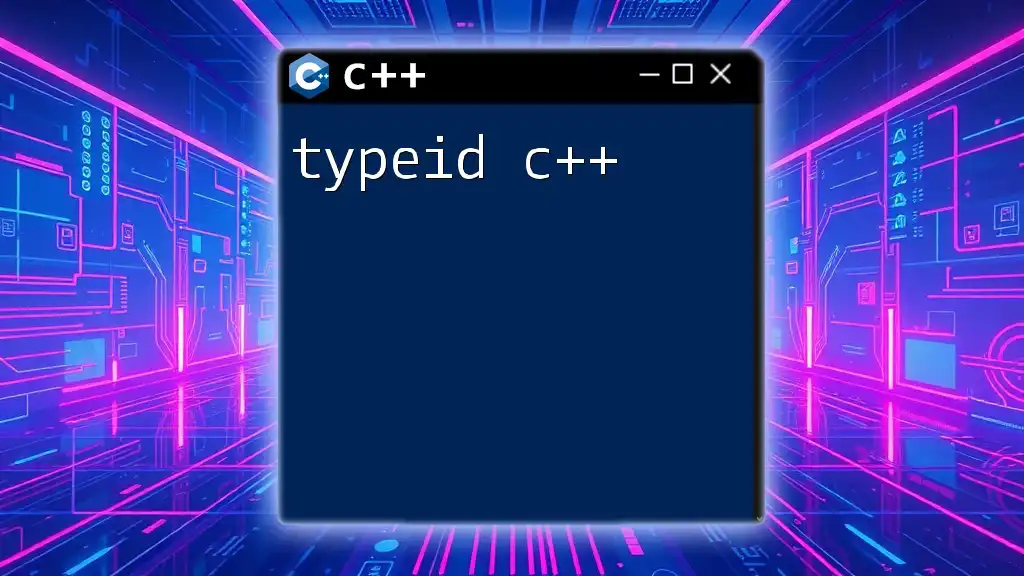
Advanced Tuple Features
Tuple Size and Type
You can retrieve the size of a tuple using `std::tuple_size`. Additionally, `std::tuple_element` allows you to extract the type of a specific element. Here’s an example demonstrating both functionalities:
std::tuple<int, double, std::string> myTuple;
std::cout << "Tuple Size: " << std::tuple_size<decltype(myTuple)>::value << std::endl;
using SecondType = std::tuple_element<1, decltype(myTuple)>::type;
Nested Tuples
Tuples can also contain other tuples, allowing you to create complex data structures. Here’s an example of a nested tuple:
auto nestedTuple = std::make_tuple(std::make_tuple(1, "Inner"), std::make_tuple(2, "Outer"));
To access the elements of the nested tuple, you simply chain the `std::get` function:
auto inner = std::get<0>(nestedTuple);
std::cout << std::get<1>(inner) << std::endl; // Accessing inner tuple's second element
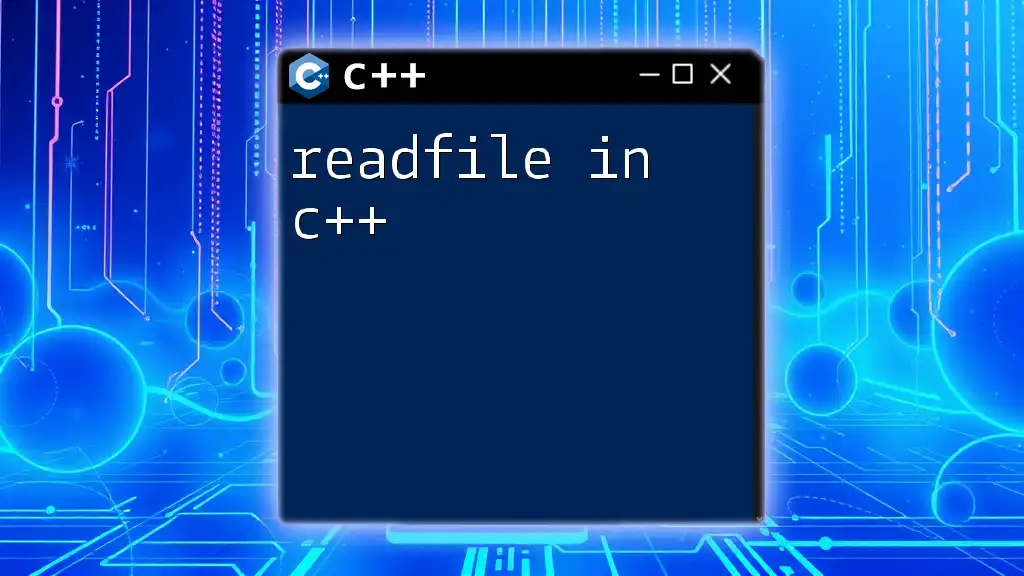
Best Practices for Using Tuples in C++
When to Use and When to Avoid
Using tuples is advantageous when you need a lightweight way to group a handful of related but differently typed items without the overhead of defining a new class or struct. However, if you find yourself frequently needing to modify the values, manage larger sets of data, or if the data types are too numerous or complex, consider using structs or classes instead.
Performance Considerations
Tuples generally provide efficient management of small collections of data. However, keep in mind that they may come with some overhead in terms of both memory and speed when compared to simpler data structures like arrays, especially if not leveraged correctly.
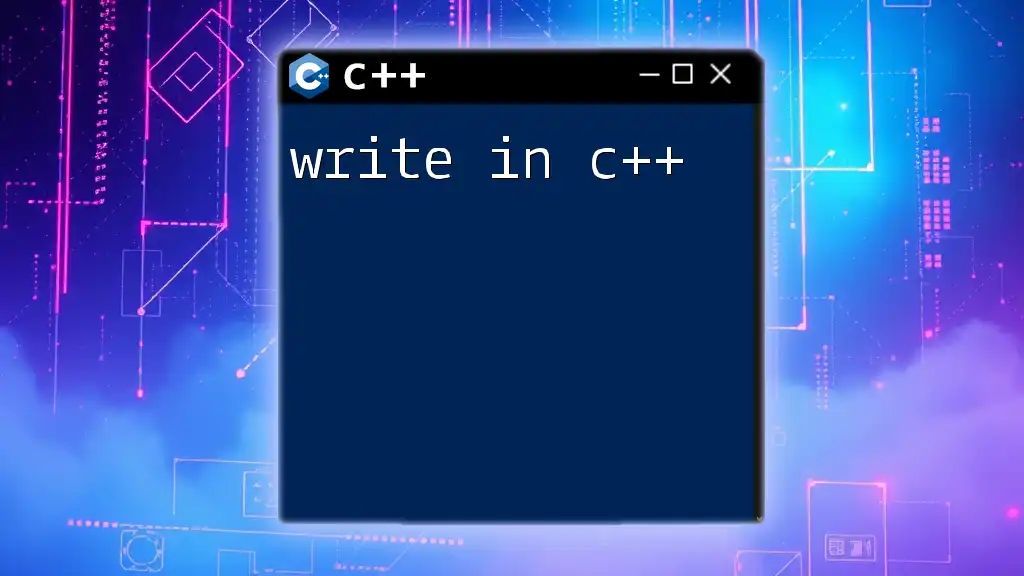
Conclusion
This exploration of tuples in C++ has unveiled their significant benefits, including the ability to group heterogeneous data conveniently and return multiple values from functions. The syntax is simple, and the functionality is versatile, making tuples a valuable addition to every C++ programmer's toolkit.
Take the time to experiment with tuples in your own projects, and enjoy the enhanced clarity and organization they can bring.
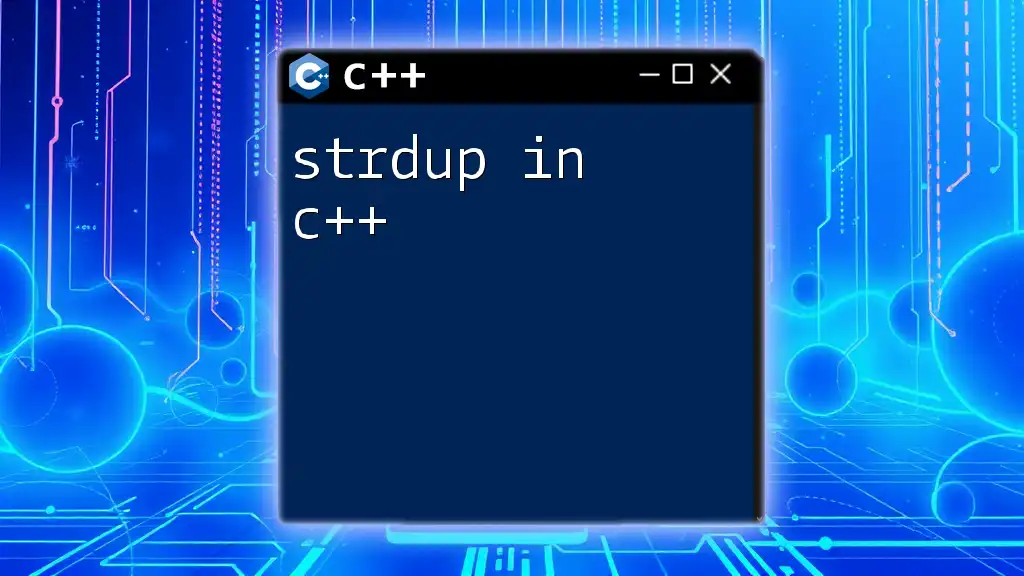
Additional Resources
For those eager to learn more about tuples and delve deeper into C++, refer to the official C++ documentation, tutorials, and coding challenges. Familiarizing yourself with these resources will enhance your understanding and skill in leveraging tuples effectively.
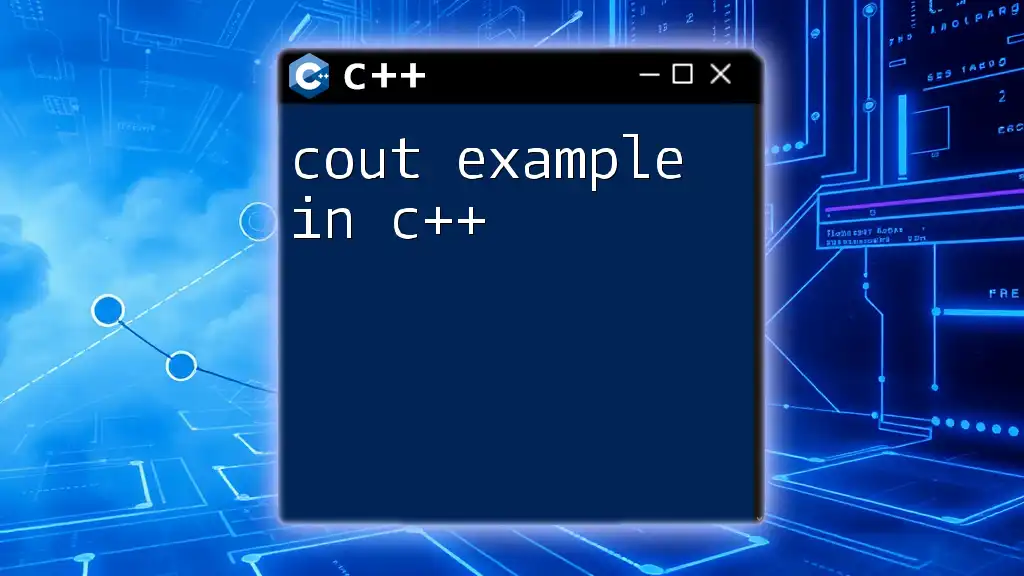
FAQs about Tuples in C++
-
Can a tuple hold different data types?
Yes, tuples can hold multiple and varied data types, making them versatile. -
Are tuples mutable?
No, tuples are immutable in C++; you cannot change their elements after creation. -
When should I use a tuple instead of a struct?
Use tuples when you need to group a few related items of different data types quickly. If the data structure requires frequent modification or involves many related fields, a struct may be more appropriate.