In C++, `nullptr` is a keyword that represents a null pointer, providing a type-safe way to indicate that a pointer does not point to any valid memory location.
Here's a code snippet demonstrating its usage:
#include <iostream>
int main() {
int* ptr = nullptr; // Initialize a pointer to null
if (ptr == nullptr) {
std::cout << "Pointer is null." << std::endl;
}
return 0;
}
Understanding Null in C++
What is Null?
In programming, null is a special value that represents the absence of an object or a value. It indicates that a pointer does not point to any valid data. A null pointer holds a specific value that means it is not referencing any actual memory location. This is crucial in programming to avoid accessing invalid memory locations, which could lead to crashes or undefined behavior.
The Different Types of Null in C++
Null Pointer
A null pointer is a pointer that has been initialized to point to nowhere. In C++, this is a fundamental concept, as it helps to ensure that pointers are either pointing to a valid resource or explicitly indicate that they are not.
To declare a null pointer, you can use the `nullptr` keyword introduced in C++11, which is the preferred way to indicate that a pointer is not pointing to any memory location:
int *ptr = nullptr; // A null pointer in C++
By convention, the pointer is set to `nullptr`, clarifying its intent and making the code more readable than using other numeric representations like `0` or `NULL`.
Null References
Unlike pointers, references in C++ cannot be null. Once a reference is initialized, it must always refer to a valid object. This might seem limiting, but it ensures that references provide a level of safety, avoiding the risk of dereferencing an uninitialized pointer.
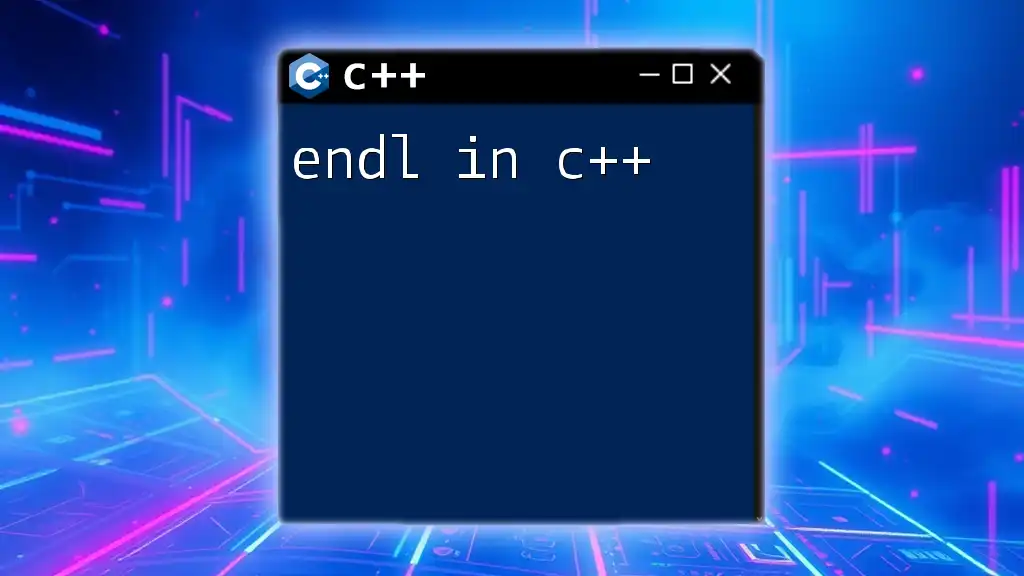
C++ Null: Using nullptr
Introduction to nullptr
The `nullptr` keyword is a type-safe null pointer constant introduced in C++11. It is a better alternative to the traditional `NULL` and `0` because it provides an unambiguous way to represent a null pointer in code.
Using `nullptr` enhances code quality by eliminating potential errors during function overloading because it will not match integer overloads, which could happen if `NULL` or `0` was used.
Example Usage of nullptr
You can easily declare and initialize pointers with `nullptr`, which clearly indicates that they do not point to valid data. For example:
int *ptr = nullptr; // Preferred way to initialize a null pointer
if (ptr == nullptr) {
std::cout << "Pointer is null" << std::endl;
}
This promotes good coding practices by reminding developers to handle potential null cases explicitly.
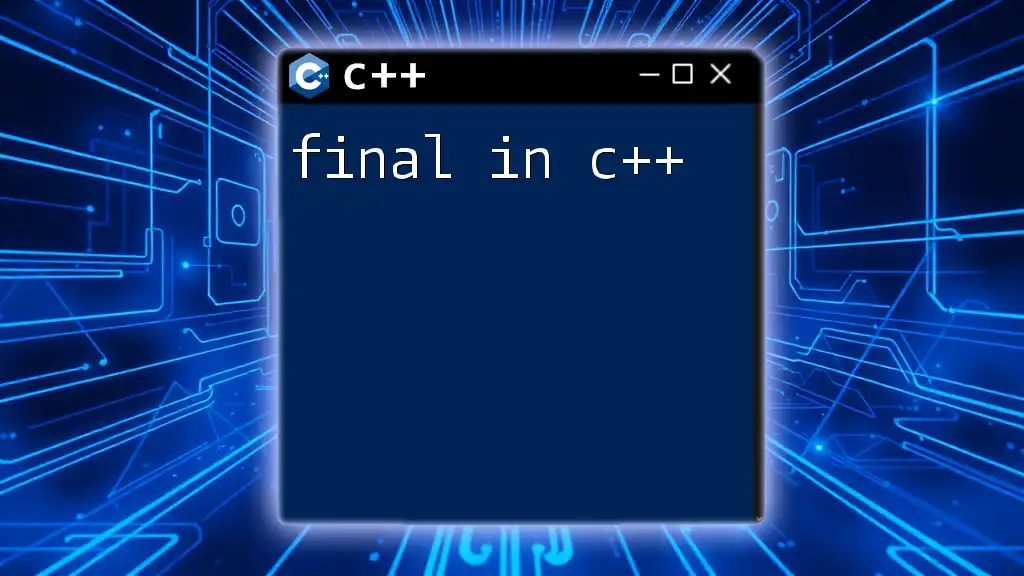
Testing for Null in C++
Checking for Null Pointers
To maintain safe pointer usage, it is essential to check if a pointer is null before attempting to dereference it. Common practices to test for null pointers involve using simple conditional statements. For instance:
if (ptr) {
std::cout << "Pointer is valid!" << std::endl;
} else {
std::cout << "Pointer is null!" << std::endl;
}
This approach helps prevent dereferencing invalid pointers and thus avoiding crashes or undefined behaviors in your application.
Best Practices for Handling Null
- Always initialize pointers to null: This practice ensures that pointers do not contain garbage data that could lead to unforeseen errors.
- Check for null before usage: Implement checks to confirm that pointers are not null before accessing the associated value or resource. This habit greatly enhances code safety and reliability.
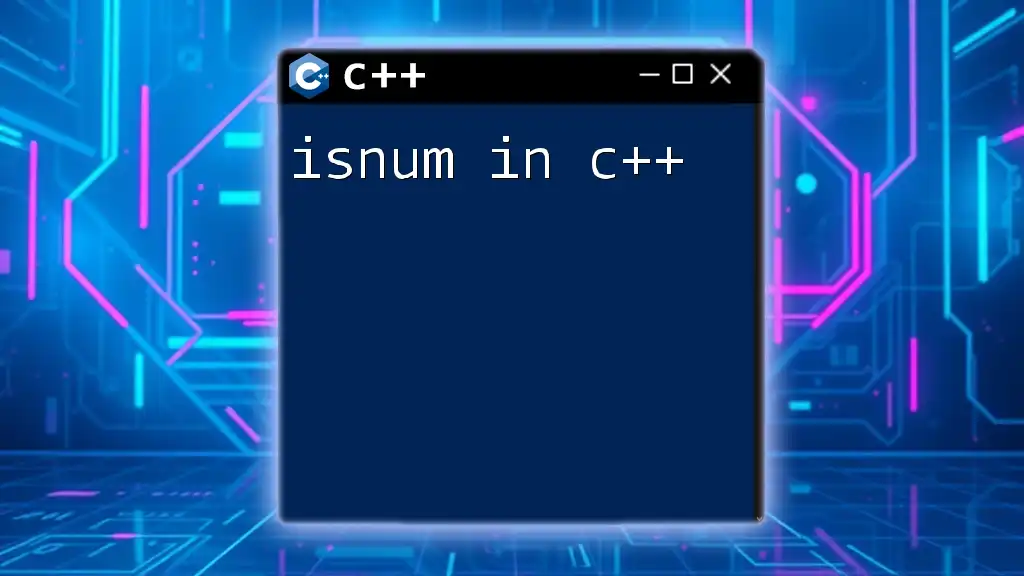
Common Mistakes with Null in C++
Dereferencing Null Pointers
One of the most prevalent mistakes is dereferencing null pointers. This can result in a program crash or undefined behavior, which can be extremely difficult to debug. An example of this might look like:
// Dangerous dereference
std::cout << *ptr; // Undefined behavior
Failing to check whether `ptr` is null before this dereference can lead to disastrous results. It's essential to always validate pointer values prior to dereferencing them.
Misusing NULL, 0, and nullptr
Many developers still confuse `NULL`, `0`, and `nullptr`, which can lead to subtle bugs in the code. For instance, `NULL` is essentially defined as `0`, which could mistakenly be interpreted as an integer in certain contexts. By using `nullptr`, you can distinctly indicate to other developers and compilers that you are working with a pointer.
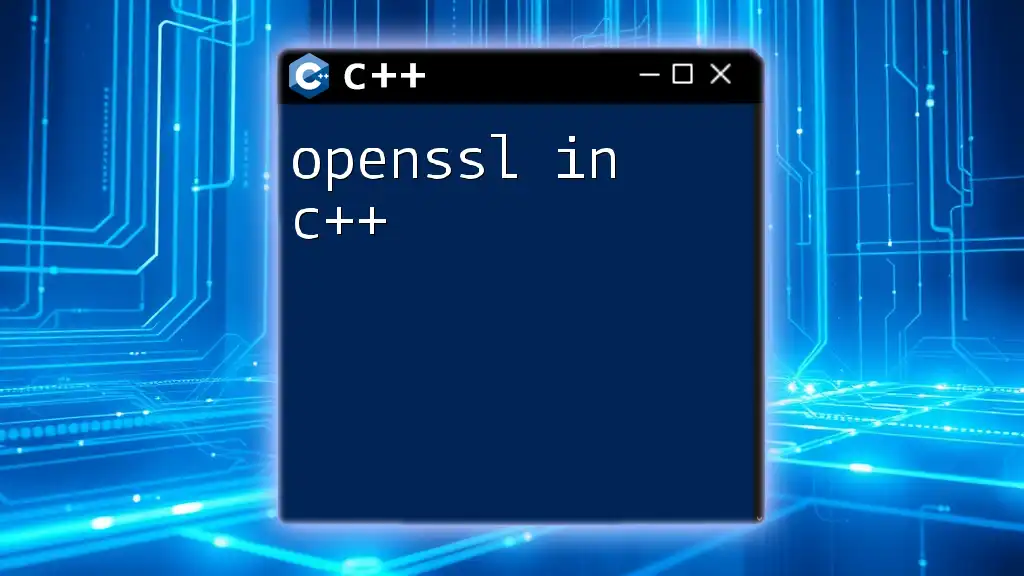
Alternative to Null: Using Smart Pointers
What are Smart Pointers?
Smart pointers are objects that manage the lifetime of a dynamic resource, effectively providing automatic memory management. Using smart pointers is highly recommended over raw pointers, as they help prevent memory leaks and dangling pointers.
Types of Smart Pointers in C++
std::unique_ptr
A `std::unique_ptr` is a smart pointer that retains ownership of an object through a pointer and ensures that there is only one unique pointer to that object at any time. This prevents memory leaks and dangling pointers:
std::unique_ptr<int> ptr = std::make_unique<int>(5); // Creating a unique pointer
std::shared_ptr
`std::shared_ptr` is another type of smart pointer that allows multiple pointers to share ownership of an object. While it is flexible, it can introduce overhead due to reference counting:
std::shared_ptr<int> ptr = std::make_shared<int>(10); // Creating a shared pointer
These smart pointers substantially improve resource management in C++ programs, and understanding when and how to utilize them is crucial for modern C++ development.
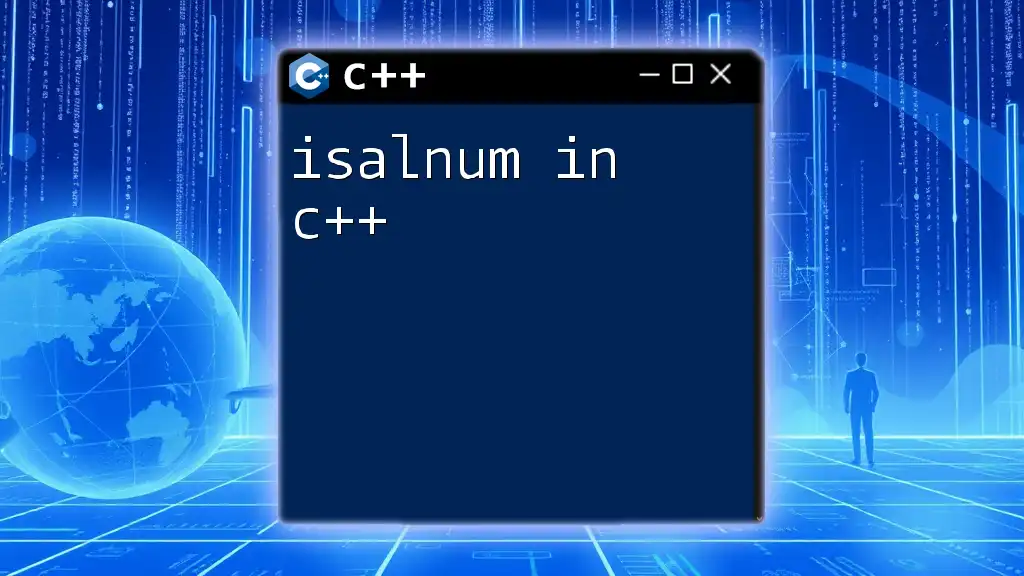
Conclusion
Understanding the role of null in C++ is fundamental for developing robust and error-free applications. By adopting best practices around null pointers, utilizing `nullptr`, and embracing smart pointers, developers can write cleaner, safer code. Making these considerations a part of your programming toolkit will translate to better software solutions and fewer debugging headaches in your C++ projects.
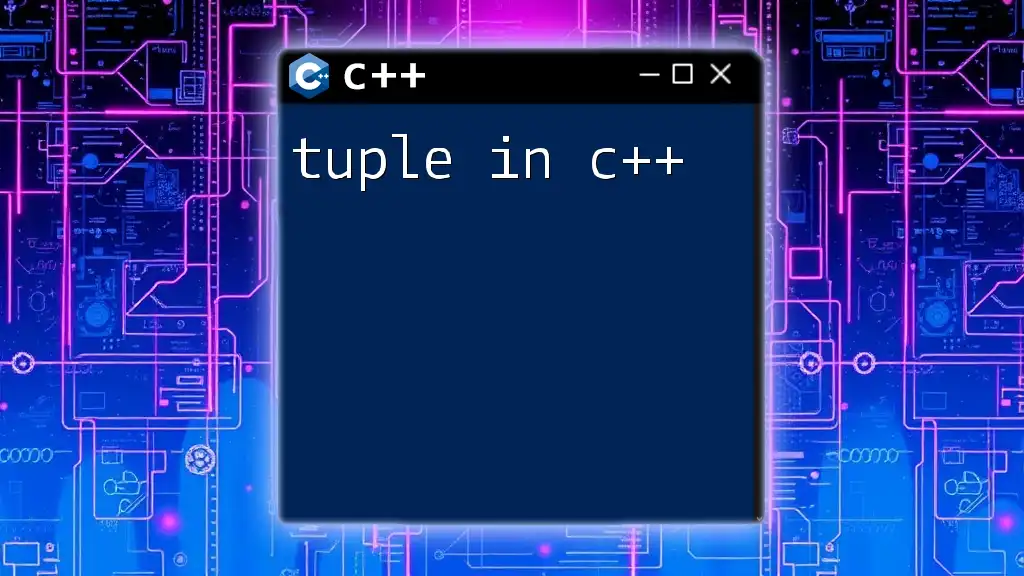
Additional Resources
To dive deeper into the concepts discussed, consider exploring the following resources:
- C++ Primer by Stanley B. Lippman
- Effective C++ by Scott Meyers
- Online C++ tutorials and documentation
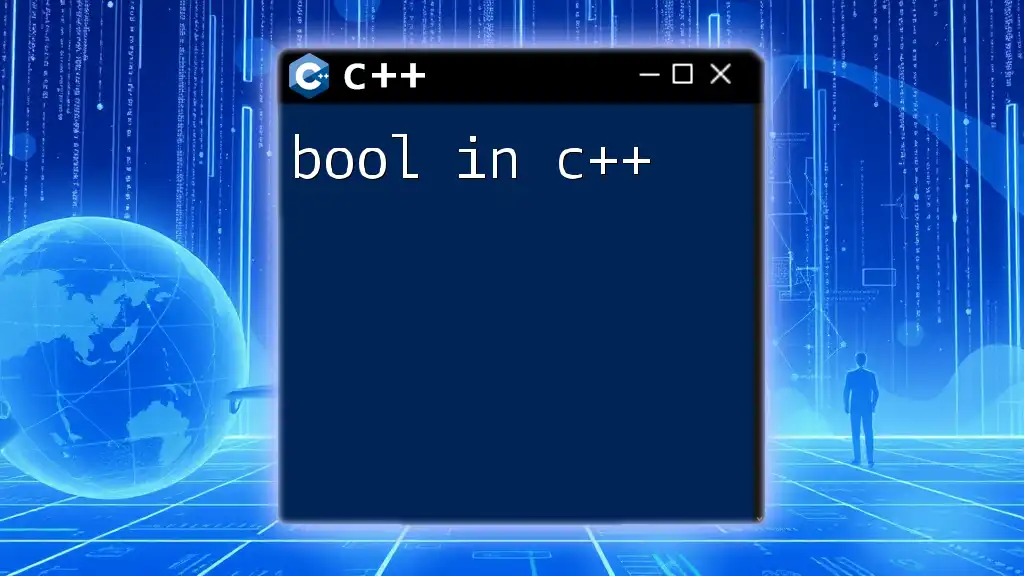
FAQs about Null in C++
What is a null pointer in C++?
A null pointer is a pointer that is not pointing to any valid memory location. In C++, it is often initialized with `nullptr`.
How should I check for null in C++?
You can check if a pointer is null by using an `if` statement. For example, `if (ptr) { /* Valid pointer / } else { / Null pointer */ }`.
Why is nullptr preferred over NULL in C++?
`nullptr` provides type safety, ensuring that it is treated solely as a pointer type, while `NULL` can sometimes be ambiguous as it is defined as `0`.
Can a reference be null in C++?
No, references in C++ cannot be null. Once initialized, a reference must always refer to a valid object.