OpenSSL in C++ allows developers to implement secure communication protocols and cryptographic operations easily, enabling tasks such as data encryption and certificate management.
Here’s a simple example of how to use OpenSSL to encrypt and decrypt a message in C++:
#include <openssl/aes.h>
#include <openssl/rand.h>
#include <iostream>
#include <string>
void aes_encrypt(const unsigned char* key, const unsigned char* plaintext, unsigned char* ciphertext) {
AES_KEY encryptKey;
AES_set_encrypt_key(key, 128, &encryptKey);
AES_encrypt(plaintext, ciphertext, &encryptKey);
}
void aes_decrypt(const unsigned char* key, const unsigned char* ciphertext, unsigned char* decryptedtext) {
AES_KEY decryptKey;
AES_set_decrypt_key(key, 128, &decryptKey);
AES_decrypt(ciphertext, decryptedtext, &decryptKey);
}
int main() {
unsigned char key[16] = "0123456789abcdef"; // AES key (16 bytes for AES-128)
unsigned char plaintext[16] = "Hello, World!!!"; // Input
unsigned char ciphertext[16]; // Output
unsigned char decryptedtext[16]; // Decrypted output
aes_encrypt(key, plaintext, ciphertext);
aes_decrypt(key, ciphertext, decryptedtext);
std::cout << "Ciphertext: " << ciphertext << "\n";
std::cout << "Decrypted text: " << decryptedtext << "\n";
return 0;
}
Make sure to link against the OpenSSL library when compiling this code!
Understanding Cryptography
What is Cryptography?
Cryptography is the practice of securing communication by converting plain text into an unreadable format known as cipher text. Historically, it has evolved from simple techniques, such as substitution and transposition, to sophisticated algorithms that secure digital communication today. There are two primary types of cryptography:
- Symmetric Cryptography: Utilizes a single key for both encryption and decryption. The key must remain confidential, as anyone with access can decrypt the data.
- Asymmetric Cryptography: Involves a pair of keys, a public key for encryption and a private key for decryption. This addresses the key distribution problem found in symmetric systems.
The Role of OpenSSL in Cryptography
OpenSSL is a robust, full-featured open-source toolkit for implementing the Secure Sockets Layer (SSL) and Transport Layer Security (TLS) protocols, along with numerous cryptographic functions. Its extensive library supports various encryption algorithms, making it critical for developing secure applications in C++. Familiarity with OpenSSL allows developers to implement robust security features, handle certificates, and ensure data integrity.

Setting Up OpenSSL with C++
Installing OpenSSL
To get started with OpenSSL in C++, you need to install the library on your machine. Here’s how to do this on different operating systems:
- Windows: You can use the precompiled binaries available through repositories like slproweb. Alternatively, you may install it through package managers like vcpkg.
- macOS: Use Homebrew to install OpenSSL with the command:
brew install openssl
- Linux: You can typically use your distribution's package manager. For instance, on Ubuntu:
sudo apt update sudo apt install libssl-dev
Integrating OpenSSL with C++ Project
After installation, including OpenSSL in your C++ project is straightforward. Ensure you link the OpenSSL libraries when compiling your project. Below is an example of setting up your C++ project to use OpenSSL:
#include <openssl/ssl.h>
#include <openssl/err.h>
int main() {
SSL_library_init();
OpenSSL_add_all_algorithms();
SSL_load_error_strings();
// Your application logic here
return 0;
}
In this snippet, we initialize the SSL library and register all the algorithms. This setup prepares the OpenSSL functions to be utilized effectively throughout your application.

Basic Operations with OpenSSL in C++
Generating Keys
Keys are fundamental in establishing cryptographic security. OpenSSL simplifies generating key pairs. Here’s how to generate an RSA key pair:
#include <openssl/rsa.h>
#include <openssl/pem.h>
#include <iostream>
void generate_rsa_key() {
RSA* rsa = RSA_generate_key(2048, RSA_F4, nullptr, nullptr);
BIO* bio = BIO_new(BIO_s_mem());
PEM_write_bio_RSAPrivateKey(bio, rsa, nullptr, nullptr, 0, nullptr, nullptr);
// Additional key handling logic here
BIO_free(bio);
RSA_free(rsa);
}
int main() {
generate_rsa_key();
return 0;
}
This code snippet generates a 2048-bit RSA key pair. The `PEM_write_bio_RSAPrivateKey` function writes the private key to a memory BIO. The process of key generation is vital for any secure application and lays the groundwork for the encryption and decryption processes to follow.
Encrypting and Decrypting Data
Symmetric Encryption
Symmetric encryption is crucial for encrypting data quickly. Below is an example of AES (Advanced Encryption Standard) encryption in C++ using OpenSSL:
#include <openssl/aes.h>
#include <cstring>
void encrypt_decrypt_aes(const unsigned char* key, const unsigned char* data, unsigned char* encrypted) {
AES_KEY encryptKey;
AES_set_encrypt_key(key, 128, &encryptKey);
AES_encrypt(data, encrypted, &encryptKey);
}
int main() {
unsigned char key[16] = "0123456789abcdef"; // 128-bit key
unsigned char data[16] = "Hello, OpenSSL!";
unsigned char encrypted[16];
encrypt_decrypt_aes(key, data, encrypted);
// Print or further process the encrypted data
return 0;
}
In this example, we define an encryption function and utilize AES to encrypt a block of data. It’s essential to handle key management and IV (Initialization Vector) securely when using symmetric encryption.
Asymmetric Encryption
Asymmetric encryption like RSA plays a vital role in secure communications, especially when exchanging keys. Here’s a basic example illustrating RSA encryption and decryption:
#include <openssl/rsa.h>
#include <openssl/pem.h>
#include <iostream>
void rsa_encrypt_decrypt(const std::string &message, RSA *public_key, RSA *private_key) {
unsigned char encrypted[256];
unsigned char decrypted[256];
int encrypt_length = RSA_public_encrypt(message.length(), (unsigned char*)message.c_str(), encrypted, public_key, RSA_PKCS1_PADDING);
int decrypt_length = RSA_private_decrypt(encrypt_length, encrypted, decrypted, private_key, RSA_PKCS1_PADDING);
decrypted[decrypt_length] = '\0'; // Null terminate the string
std::cout << "Decrypted message: " << decrypted << std::endl;
}
int main() {
// Assume public_key and private_key are initialized here from a key pair
// Example: RSA* public_key = ...; RSA* private_key = ...;
std::string message = "Secure Message";
// Call your encryption and decryption function using public_key & private_key
return 0;
}
This snippet illustrates how to encrypt and decrypt using RSA in OpenSSL. As with symmetric encryption, managing the keys securely is crucial when using asymmetric encryption.

Working with Certificates
Understanding Certificates
Digital certificates are essential for establishing trust in secure communications. They bind a public key to an identity, ensuring that the data sent across a network is secure and authentic. Certificates are a foundational element of SSL/TLS and online transactions.
Creating and Managing Certificates
You can create a self-signed certificate with OpenSSL as follows:
#include <openssl/x509.h>
#include <openssl/pem.h>
void create_self_signed_cert() {
X509 *x509 = X509_new();
// Set version, serial number, issuer, validity, and subject here
// Bio and key handling code here
BIO_free(bio);
X509_free(x509);
}
int main() {
create_self_signed_cert();
return 0;
}
In this example, a self-signed certificate can be initialized. Populate the necessary fields like version and issuer before using it to secure communications. Managing certificates appropriately is crucial for maintaining SSL/TLS trust.

Practical Applications of OpenSSL in C++
TLS/SSL Communication
Implementing secure communication between a server and a client using TLS/SSL can be accomplished with OpenSSL. Here is a simplified example of an SSL server:
#include <openssl/ssl.h>
#include <openssl/err.h>
void server() {
SSL_CTX* ctx = SSL_CTX_new(SSLv23_server_method());
// Load certificates and set up server logic
SSL* ssl;
int client_sock;
// Accept a client connection and perform SSL handshake
//
// Process secure communication
SSL_CTX_free(ctx);
}
int main() {
server();
return 0;
}
This code initializes an SSL context for a server. The actual implementation requires loading the certificate, managing sockets, and error-checking, but it encapsulates the essential steps.
Verifying Certificates
Certificate verification ensures that the digital certificate presented by a peer is valid. Below is a code example illustrating how to verify a certificate using OpenSSL:
#include <openssl/x509.h>
#include <openssl/x509_vfy.h>
bool verify_certificate(const char *cert_file) {
X509 *cert = nullptr;
FILE *fp = fopen(cert_file, "r");
if (!fp) return false;
cert = PEM_read_X509(fp, NULL, NULL, NULL);
fclose(fp);
// Verify the certificate logic here
X509_free(cert);
return true; // Adjust based on verification result
}
int main() {
verify_certificate("your_cert.pem");
return 0;
}
This code reads a certificate from a PEM file and ensures its authenticity through verification processes.

Best Practices and Security Considerations
Keeping OpenSSL Updated
Using the latest version of OpenSSL is crucial, as updates address vulnerabilities and improve algorithms. Regular updates should be part of your development and deployment process.
Secure Coding Practices
When programming with OpenSSL in C++, it’s vital to:
- Handle errors gracefully: Use error handling functions available in OpenSSL to address issues promptly.
- Safeguard keys and certificates: Never hard-code keys in the source code; consider secure storage solutions for sensitive data.
- Validate all inputs: Ensure data integrity and avoid injection attacks by validating inputs before processing.
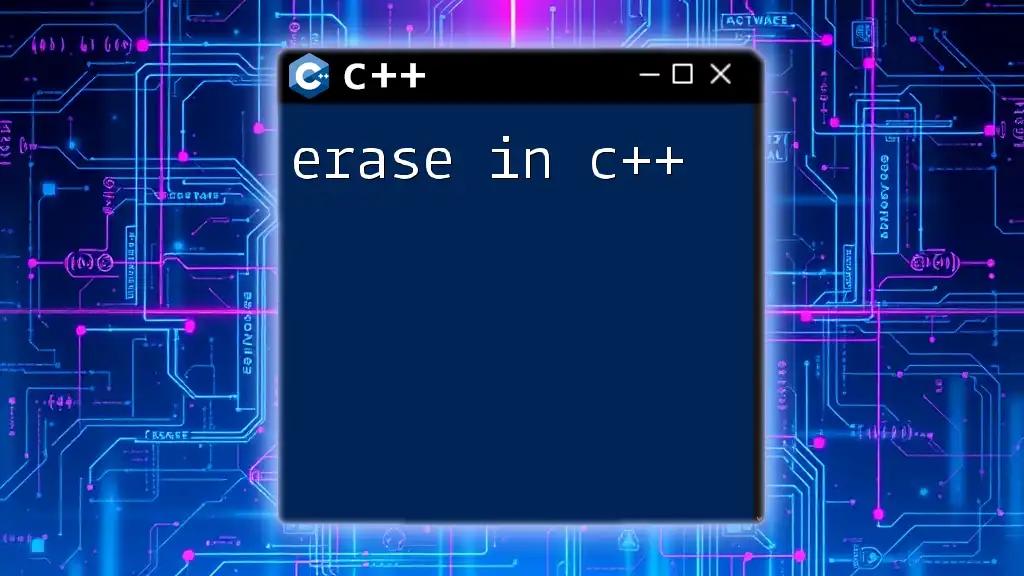
Troubleshooting Common Issues
Compilation Errors
Common compilation errors often arise when linking against the OpenSSL library. Ensure your project settings reference the correct library paths and include directories.
Runtime Errors
OpenSSL may throw runtime exceptions related to memory allocation or operations on uninitialized data. Using debugging tools can help trace these issues effectively.

Conclusion
In this comprehensive guide, we’ve explored the essential features and capabilities of OpenSSL in C++. Through diligent key management, encryption, and secure communication practices, developers can harness the full potential of OpenSSL to secure their applications. As you continue to experiment and learn, consider delving deeper into the myriad of features OpenSSL offers, enhancing both your projects' security posture and your expertise in cryptography.

References and Further Reading
For further exploration, consult the official [OpenSSL Documentation](https://www.openssl.org/docs/) and consider recommended books that delve into cryptography and secure programming practices.