In C++, `bool` is a data type that represents a Boolean value, which can be either `true` or `false`, and is commonly used in control flow statements and logical operations.
Here’s a simple code snippet demonstrating the use of `bool` in C++:
#include <iostream>
using namespace std;
int main() {
bool isRainy = true;
if (isRainy) {
cout << "Don't forget your umbrella!" << endl;
} else {
cout << "Enjoy the sunshine!" << endl;
}
return 0;
}
What is a Bool in C++?
The bool type in C++ is a fundamental data type used to represent binary values, specifically true and false. It is essential for logical operations and control flow within programs. The term boolean originates from mathematician George Boole and is crucial in computer science for decision-making processes.
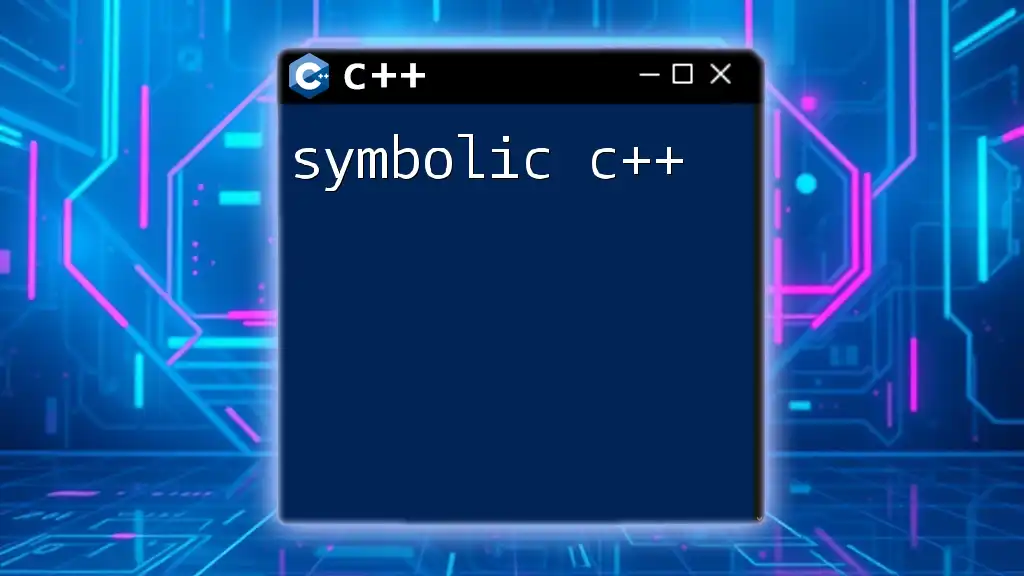
The C++ Bool Type
Characteristics of C++ Bool Type
In C++, the bool type can hold one of two values: true or false. It’s important to note that the bool type typically occupies 1 byte of memory, which is the smallest addressable unit on most systems. This compact size makes bool data types memory-efficient while handling logical operations.
Operations with C++ Bool
Logical operations are fundamental to programming, and the bool type supports operators such as AND (`&&`), OR (`||`), and NOT (`!`). Each of these operators allows you to combine or negate boolean values.
For example, consider the following code snippet demonstrating these logical operations:
bool a = true;
bool b = false;
bool conjunction = a && b; // Logical AND
bool disjunction = a || b; // Logical OR
bool negation = !a; // Logical NOT
- Here, `conjunction` will evaluate to false because both variables must be true for an AND operation to yield true.
- Conversely, `disjunction` will be true as at least one operand is true.
- Meanwhile, `negation` changes the logical state of `a` from true to false.
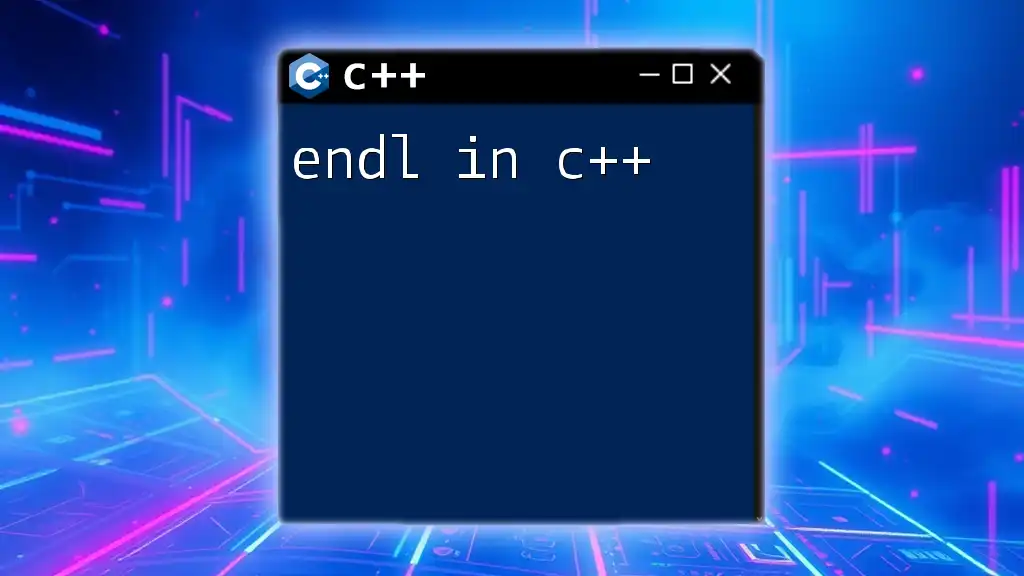
Declaring and Using Boolean in C++
How to Declare a Bool Variable
Declaring a bool variable in C++ is straightforward. The syntax involves using the keyword bool, followed by a variable name and an optional initial value.
For instance:
bool isSunny = true;
In this example, `isSunny` is a boolean variable initialized to true. This capability allows for precise control over program flow based on conditions evaluated throughout the code.
Using C++ Bool in Control Structures
The bool type plays a critical role in control structures such as if-else statements. The value of a bool variable determines which path the program will take.
Consider the following code:
if (isSunny) {
cout << "It's a sunny day!";
} else {
cout << "It's not sunny.";
}
In this case, the output will depend on the state of `isSunny`. If it is true, "It's a sunny day!" will be printed; otherwise, the alternative message is displayed. This structure exemplifies how bool variables leverage dynamic decision-making in programming.
Switching with Boolean Values
While if-else structures are commonly associated with bool, it is important to note that switch-case statements cannot directly utilize boolean values, as they are primarily designed for integral types. Attempting to use a bool in a switch will lead to compile-time errors. For this reason, it is often better to use if-else structures when working with boolean logic.
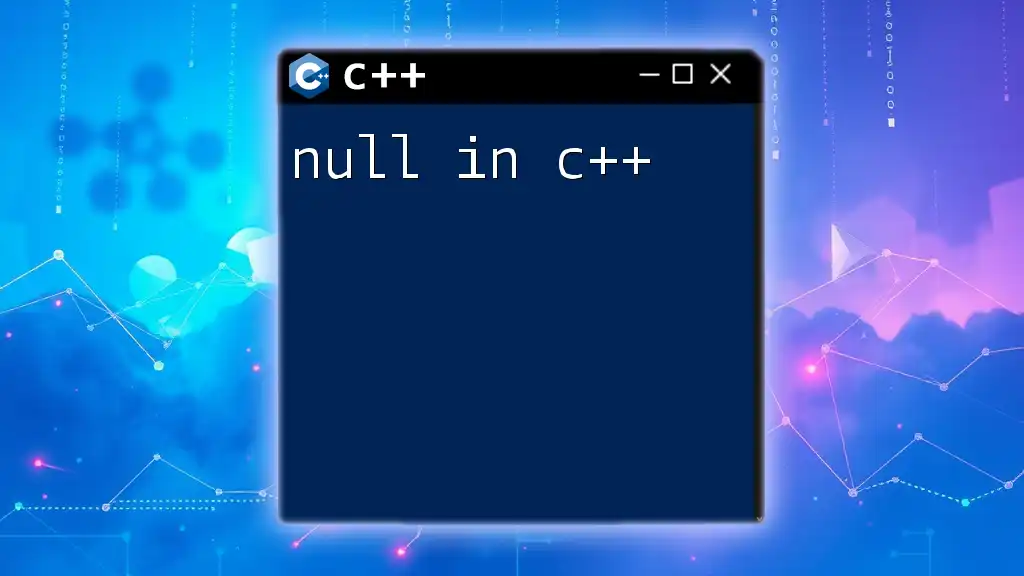
Boolean Expressions in C++
Creating Boolean Expressions
Boolean expressions are constructed using logical operators to compare and combine boolean values. These expressions can evaluate to either true or false based on the operands involved.
For example, you might create a boolean expression that assesses multiple conditions:
bool isRaining = false;
bool isWeekend = true;
bool stayIndoors = isRaining || !isWeekend; // Stay indoors if it's raining or it's not the weekend
Here, the expression `stayIndoors` becomes true if it is either raining or it is not a weekend. Such logical combinations enable robust decision-making capabilities in your programs.
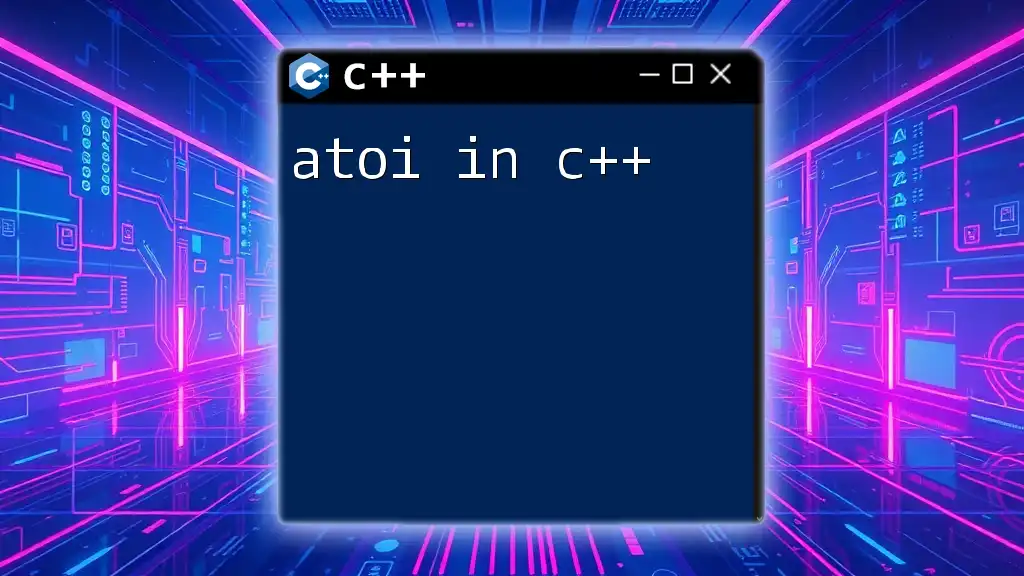
Advantages of Using the C++ Boolean Type
Readability and Maintainability
Using the bool type improves code clarity and comprehension. When you choose descriptive names for boolean variables, such as `isValid` or `isComplete`, readers can easily grasp the intended logic of the code. This practice helps maintain the codebase over time, as it enables future developers to understand conditions quickly.
Memory Efficiency
The compact size of the bool type makes it an excellent choice for applications that require storage of large sets of boolean flags. Where other integral types require multiple bytes, the bool type conservatively uses memory while delivering the necessary functionality.
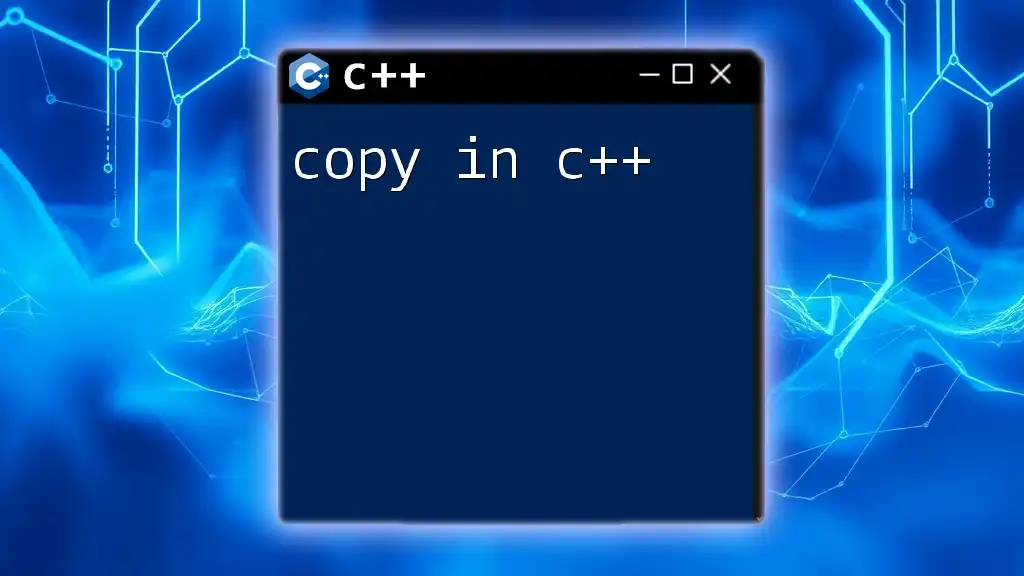
Common Pitfalls with Boolean in C++
Confusing Boolean Values with Integers
Many programmers mistakenly treat boolean variables as if they can be directly assigned numerical values. While true is often represented as 1 and false as 0, this can lead to confusion and bugs in the code.
For instance, consider this line:
int x = true; // This is legal, but may confuse readers
While this code compiles, it can mislead those reading the code into thinking that `x` holds a logical state rather than a numeric representation.
Mixing Boolean with Other Data Types
Combining bool types with other data types can introduce risks. For example, passing a boolean to a function expecting an integer could lead to logical errors. Maintaining type appropriateness helps ensure clearer logic and fewer bugs in your applications.
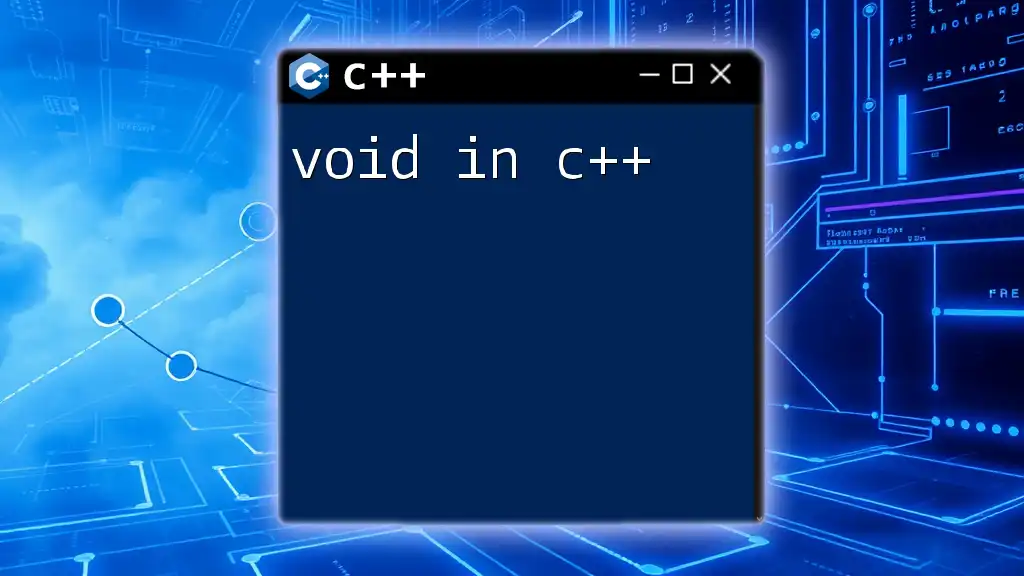
Advanced Usage of Boolean in C++
std::bitset and Bool
For scenarios requiring the storage of multiple boolean values, C++ offers `std::bitset`. This template allows you to manage a sequence of bits efficiently.
Here’s a brief example:
#include <bitset>
std::bitset<8> bits; // 8 boolean values
bits.set(0); // Set first bit to true
In this case, you create a bitset capable of holding eight boolean values, manipulating them with methods to set, reset, and query individual bits.
Custom Boolean Types
When working on large systems, you may find that the built-in bool type is insufficient for specific needs. For such purposes, you can create custom boolean types using classes that can encapsulate additional context or functionalities.
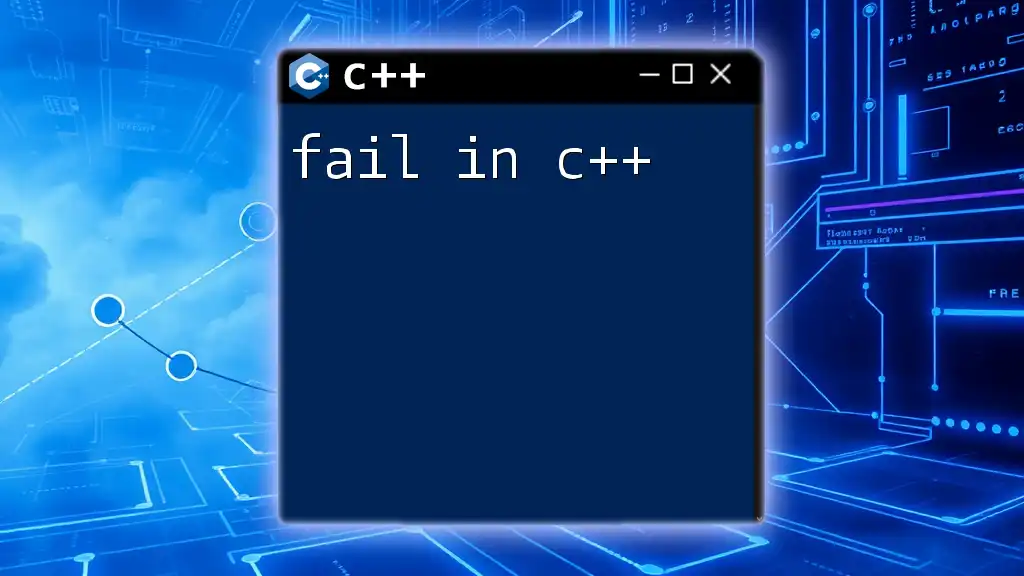
Conclusion
Understanding the bool in C++ is fundamental for effective programming, especially in decision-making processes and logical expressions. By leveraging the properties of the bool type, developers can compose clear, efficient, and maintainable code. Best practices surrounding the usage of boolean variables promise to enhance readability and logical integrity, while advanced techniques like `std::bitset` enable handling complex boolean data efficiently.
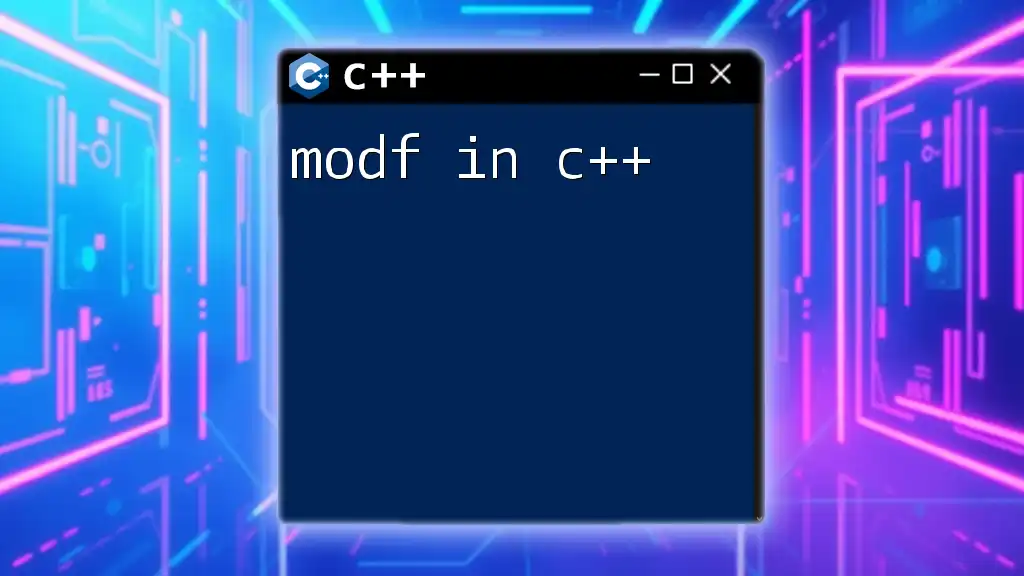
Additional Resources
For further reading on the c++ bool type, consult official C++ documentation, tutorials, and textbooks that delve deeper into boolean logic and control structures. Practicing exercises focused on the usage of bool will solidify your understanding and proficiency in crafting efficient applications.
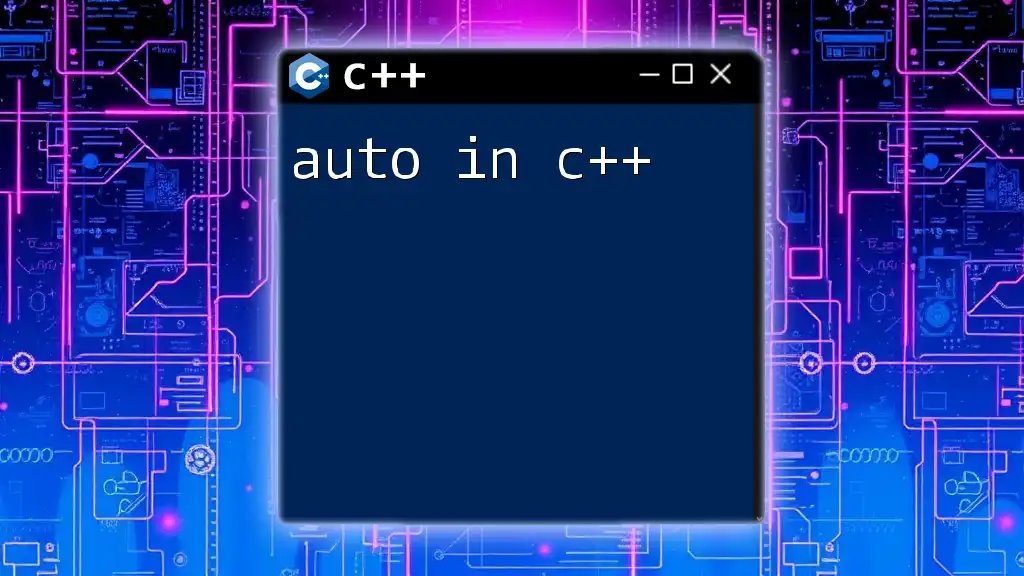
Call to Action
If you're looking to enhance your proficiency in C++, consider joining our courses for quick and concise lessons that will help you master commands like those involving bool in C++.