In C++, `DWORD` is a data type defined in Windows programming as an unsigned 32-bit integer, commonly used for various system-level programming tasks.
#include <windows.h>
#include <iostream>
int main() {
DWORD myNumber = 4294967295; // Maximum value for a DWORD
std::cout << "The DWORD value is: " << myNumber << std::endl;
return 0;
}
What is a Dword?
A DWORD (Double Word) is a data type that represents a 32-bit unsigned integer in C++. The term originates from computer architecture, where a "word" refers to the natural data size that a processor can handle in one operation. In the context of DWORD, it essentially doubles that size, leading to a total of 32 bits or 4 bytes.
Key Characteristics of DWORD:
- Size: As a 32-bit unsigned integer, DWORD can represent values ranging from 0 to 4,294,967,295.
- Memory Alignment: Being a fixed-width type ensures consistency and efficiency in memory access.
- Usage Context: Commonly found in Windows APIs and various low-level system programming scenarios.
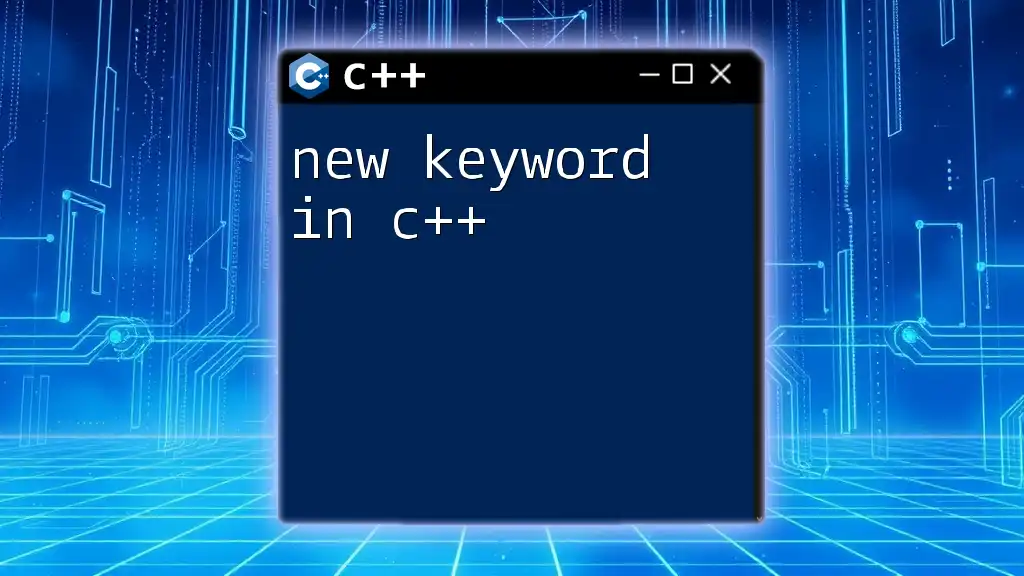
Understanding Data Types in C++
Basic Data Types
C++ features several fundamental data types, including:
- `int`: Represents integers, typically 4 bytes.
- `char`: Represents characters, typically 1 byte.
- `float`: Represents floating-point numbers, typically 4 bytes.
Extended Data Types
Extended data types, such as DWORD, provide additional flexibility and are essential for specialized programming tasks. These data types often come into play when interfacing with operating system-level features, hardware, or components that require precise data manipulation.
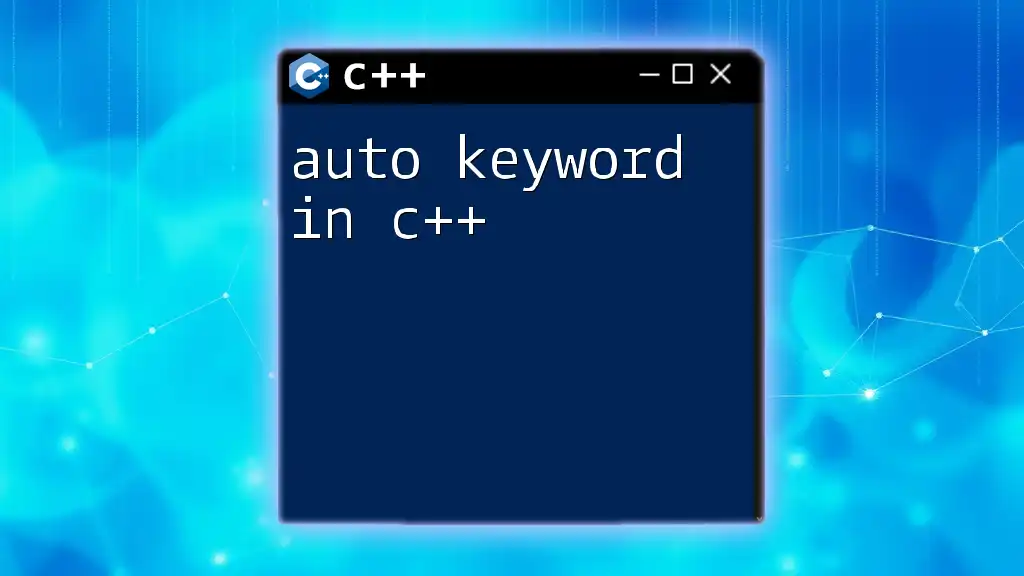
The DWORD Data Type
Defining DWORD in C++
In C++, DWORD is generally defined in the Windows.h header file, which is a vital part of the Windows API. You can define a DWORD simply as follows:
#include <windows.h> // Include header for DWORD
DWORD myVariable; // Declare a DWORD variable
Memory Representation
The DWORD data type occupies 4 bytes in memory. When you assign values to a DWORD, it stores them as a 32-bit unsigned integer. Understanding how memory is allocated for DWORD is crucial for optimizing performance and resource management in your applications.
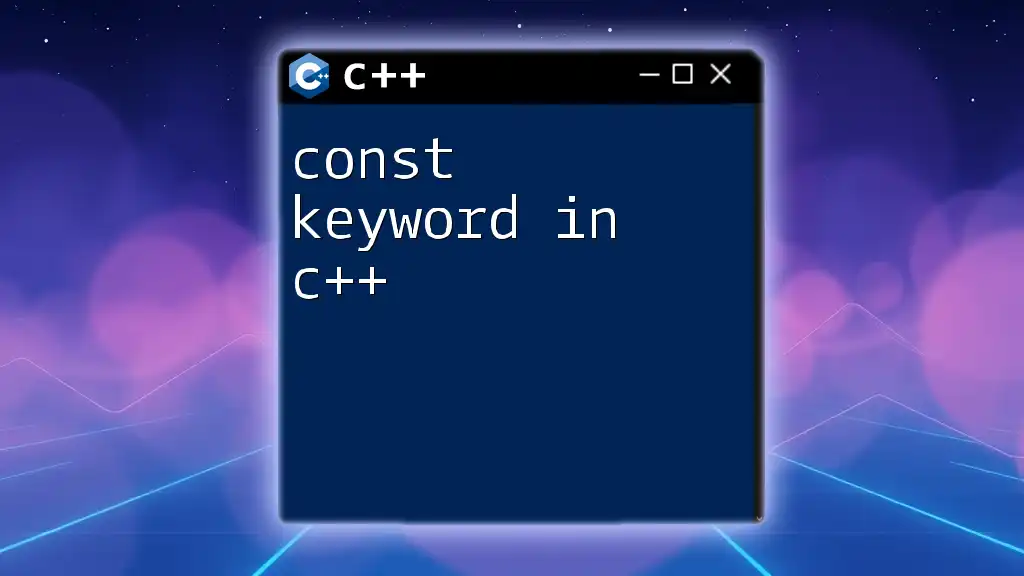
Using DWORD in C++
Declaring a DWORD Variable
To declare a DWORD variable, include the Windows header and use the following syntax:
#include <windows.h> // Include header for DWORD
DWORD myVariable = 100; // Declaration and initialization
Assigning and Manipulating DWORD Values
Manipulating DWORD values is straightforward due to its familiarity as an integer type. The following example illustrates some basic arithmetic operations:
DWORD a = 10;
DWORD b = 20;
DWORD sum = a + b; // Simple addition
This example shows how easy it is to perform operations with DWORD, demonstrating its utility in arithmetic and calculations.
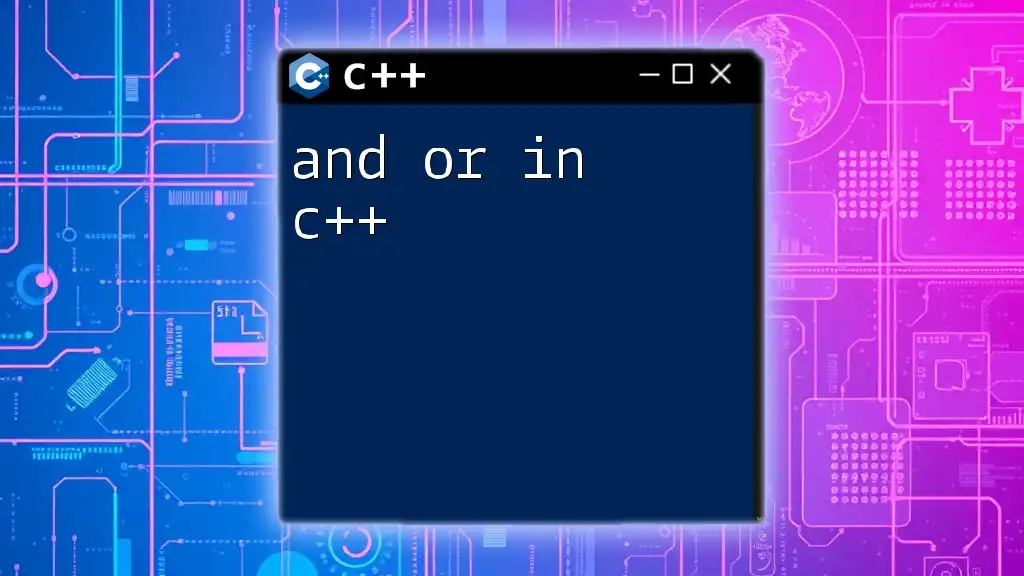
Common Use Cases for DWORD in C++
Working with APIs and Libraries
A significant area where DWORD is frequently used is within the Windows API. For instance, DWORD may be employed to retrieve process identifiers. Here’s an example:
#include <windows.h>
DWORD processId;
GetProcessId(&processId); // Retrieve the current process ID
This demonstrates how DWORD is essential for system-level programming, particularly when working with APIs.
Timers and Delays
Incorporating DWORD for time management is another practical use case. You can set up timing intervals like so:
#include <windows.h>
DWORD waitTime = 1000; // Wait for 1000 milliseconds
Sleep(waitTime); // Pause execution
This is particularly useful while optimizing program flow, especially in multi-threaded applications.
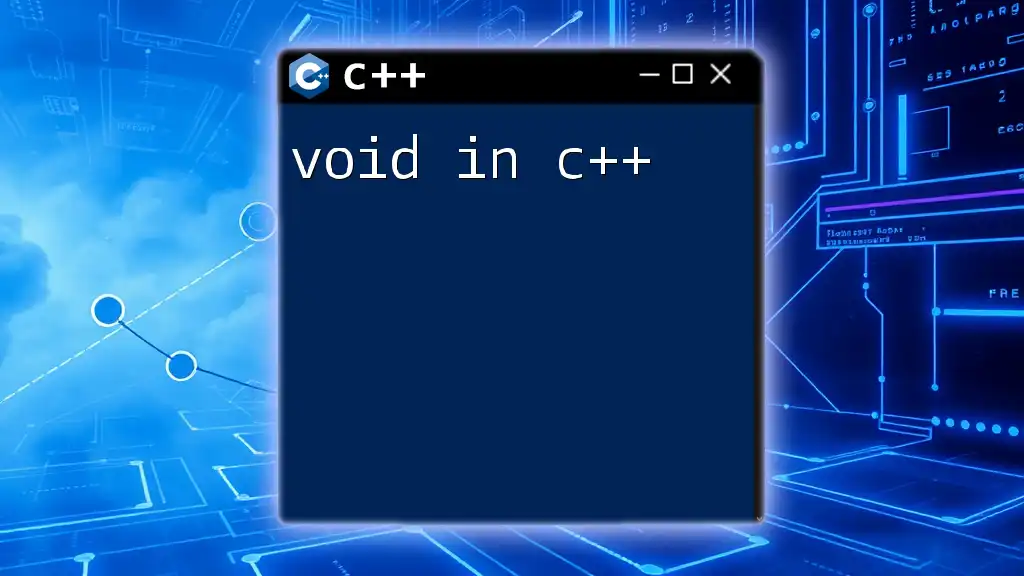
Error Handling with DWORD
Understanding Return Types
DWORD is often utilized in functions that return error codes. This makes it easier to check for errors during function calls. Consider this example:
DWORD result = SomeFunction();
if (result != NO_ERROR) {
// Handle error
}
Using DWORD for error management helps standardize how you handle system calls and API requests, making your code cleaner and more efficient.
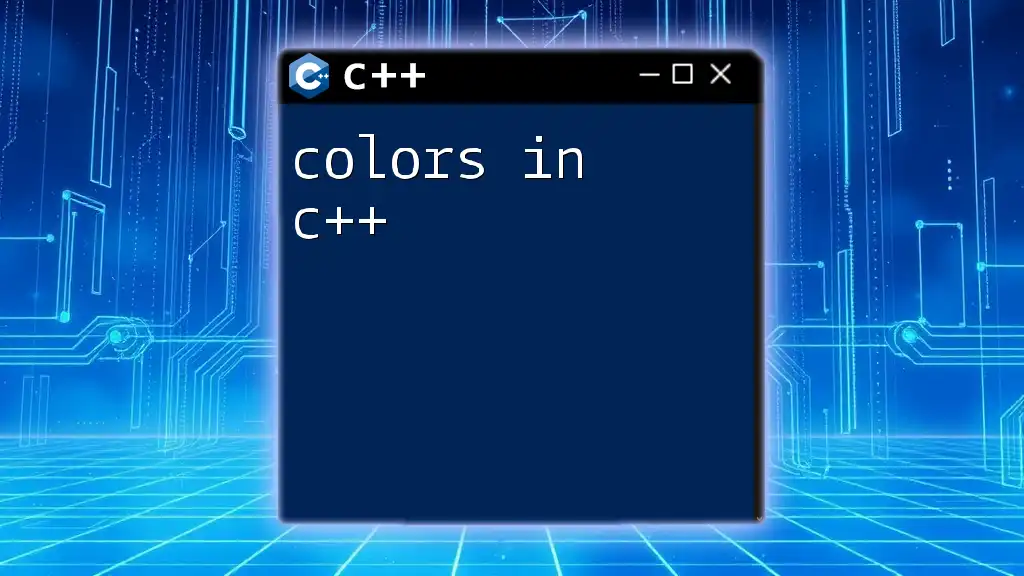
Advantages of Using DWORD
Performance Benefits
The DWORD type can optimize performance in specific programming contexts. Being a fixed-size type allows compilers and processors to manage data more efficiently, resulting in potentially faster execution times.
Compatibility with Various Functions
DWORD’s compatibility with various library functions enhances its versatility. It seamlessly integrates with numerous Windows API functions, as its definition is widely accepted across system architecture and libraries.
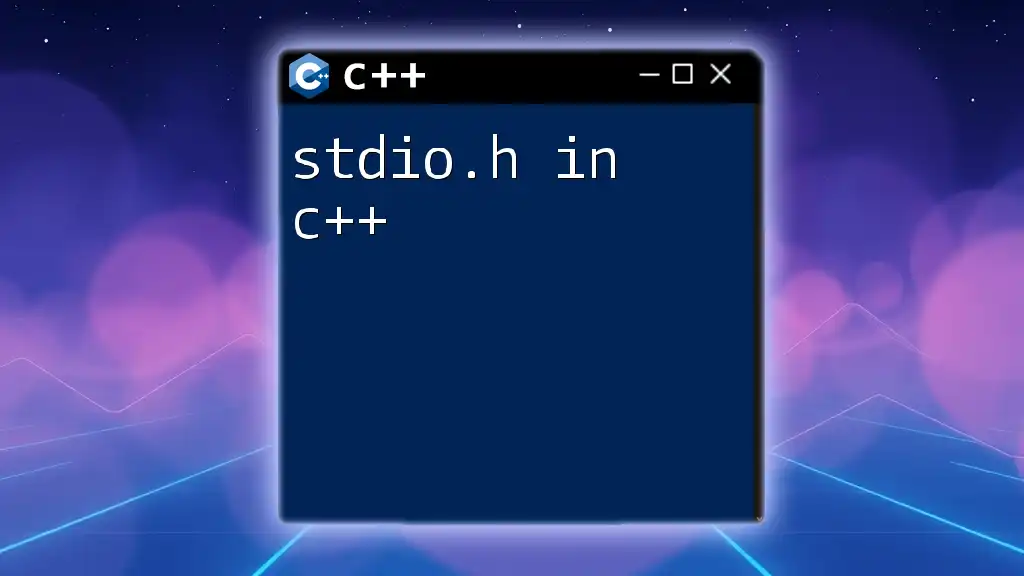
Frequently Asked Questions about DWORD in C++
Is DWORD a Built-in Type?
While DWORD is not a built-in type in the strictest sense, it is defined as a typedef in the Windows.h header file. Thus, it behaves very much like built-in primitive types within a C++ program.
Differences Between DWORD and Other Integer Types
DWORD differs from `int` and `long` primarily in size and the representation of values. While `int` can hold signed integers, DWORD exclusively holds unsigned values. Understanding these differences is key to writing robust and optimized C++ code.
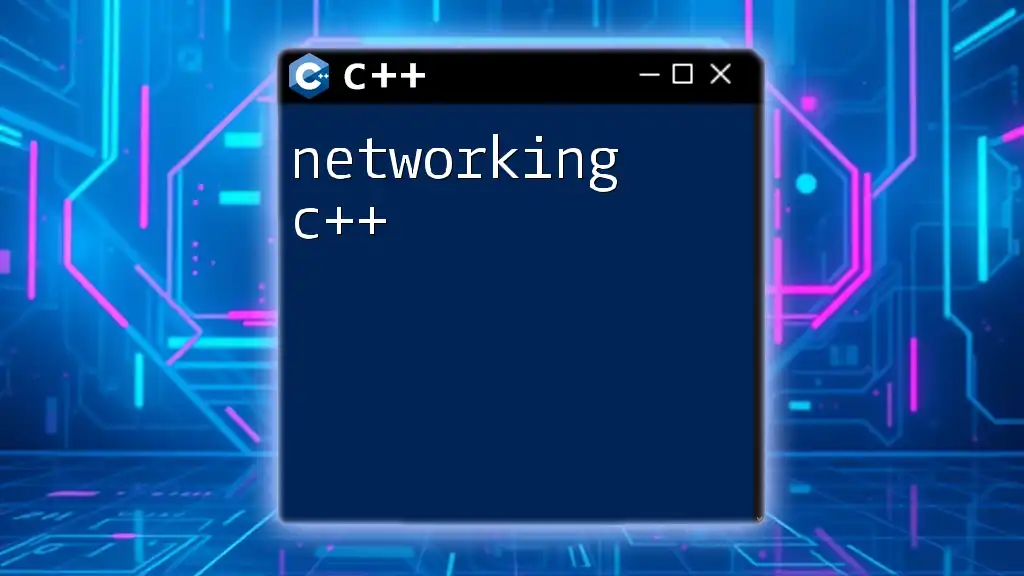
Conclusion
In summary, understanding dword in C++ is vital for developers working with systems programming or any application interfacing with Windows APIs. The DWORD data type is invaluable for manipulating large integers, performing efficient calculations, and managing error handling in a clean and robust manner. As you continue your journey in C++, consider exploring more advanced topics that leverage the flexibility and power of types like DWORD.