The `islower` function in C++ is used to check if a given character is a lowercase letter, returning a non-zero value if true and zero otherwise. Here’s a simple code snippet demonstrating its usage:
#include <iostream>
#include <cctype>
int main() {
char c = 'a';
if (islower(c)) {
std::cout << c << " is a lowercase letter." << std::endl;
} else {
std::cout << c << " is not a lowercase letter." << std::endl;
}
return 0;
}
Understanding the Basics of `islower`
`islower` is a built-in function in C++ that is used to check whether a given character is a lowercase letter. It plays a crucial role in many programming scenarios, especially in validating character data, formatting text, and processing user input.
What Does `islower` Do?
The `islower` function determines if the provided character falls within the range of lowercase letters ('a' to 'z'). If the character is lowercase, the function returns true; otherwise, it returns false.
The Syntax of `islower`
The function is defined as follows:
#include <cctype>
bool islower(int ch);
This syntax indicates that the function is included in the `<cctype>` header file, which contains various character classification functions.

How to Use `islower` in Your Code
Including the Header File
Before using the `islower` function, it is essential to include the `<cctype>` header file at the beginning of your program. This inclusion allows access to the various character classification functions provided by C++.
#include <cctype>
Basic Usage of `islower`
Here's how you can use `islower` to check if a single character is lowercase:
#include <cctype>
#include <iostream>
int main() {
char ch = 'a';
if (islower(ch)) {
std::cout << ch << " is a lowercase letter." << std::endl;
} else {
std::cout << ch << " is not a lowercase letter." << std::endl;
}
return 0;
}
In this example, the program checks if `ch` is a lowercase letter. The output will notify the user whether the character passed the test.
Checking a Character Array or String
To check if characters within a string are lowercase, you can loop through each character using the `islower` function. Here’s an example:
#include <cctype>
#include <iostream>
#include <string>
int main() {
std::string str = "Hello World!";
for (char ch : str) {
if (islower(ch)) {
std::cout << ch << " is a lowercase letter." << std::endl;
}
}
return 0;
}
This code iterates through each character in the string `str`, and for each character, it checks if it is lowercase. The output will display each lowercase character found in the string.
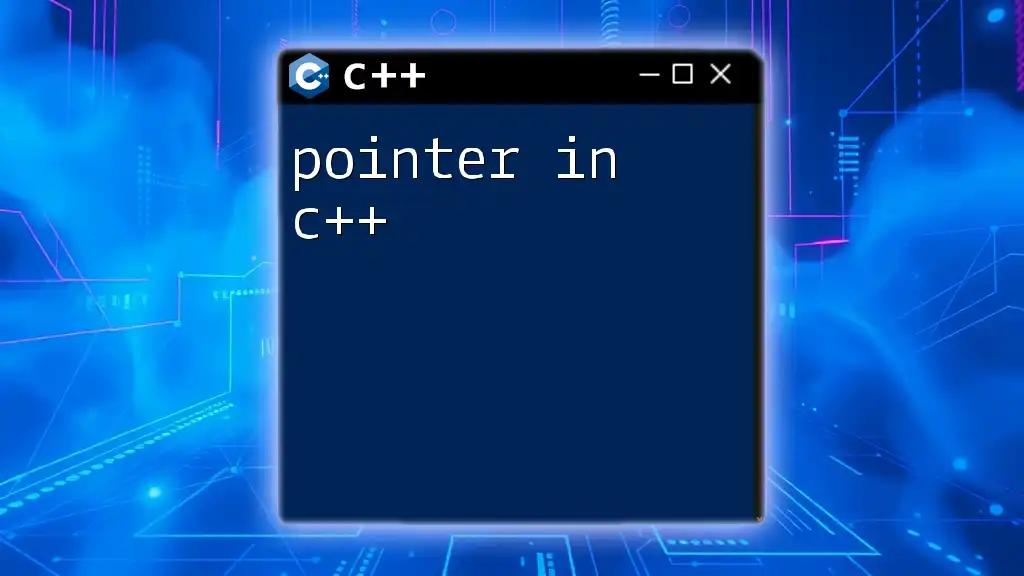
Common Use Cases for `islower`
Input Validation
Validating user input is crucial to ensure data integrity. For instance, when accepting usernames that must contain at least one lowercase letter, `islower` can be employed to validate the input efficiently.
Formatting Text
In text formatting applications, you might want to convert strings to a particular case or emphasize certain letters based on their case. You can use `islower` to identify lowercase letters and apply specific formatting accordingly.
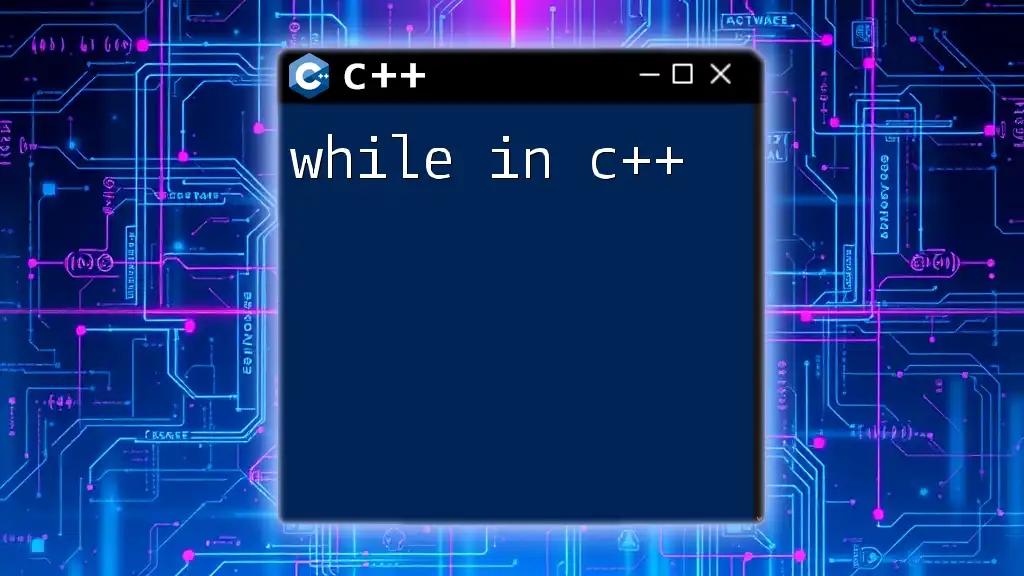
Common Errors and Troubleshooting
Unintended Behavior
When using `islower`, it's important to be aware of how it behaves with special characters. For instance:
#include <cctype>
#include <iostream>
int main() {
char ch = '@'; // Special character
if (islower(ch)) {
std::cout << ch << " is a lowercase letter." << std::endl;
} else {
std::cout << ch << " is not a lowercase letter." << std::endl;
}
return 0;
}
In this case, the output will confirm that the special character `@` is not a lowercase letter. This illustrates that `islower` does not handle non-alphabetic characters.
Handling Non-Character Inputs
If you try to input non-character values, such as numeric digits, `islower` will also return false. It's crucial to ensure that inputs are within character ranges if you aim to use this function effectively.
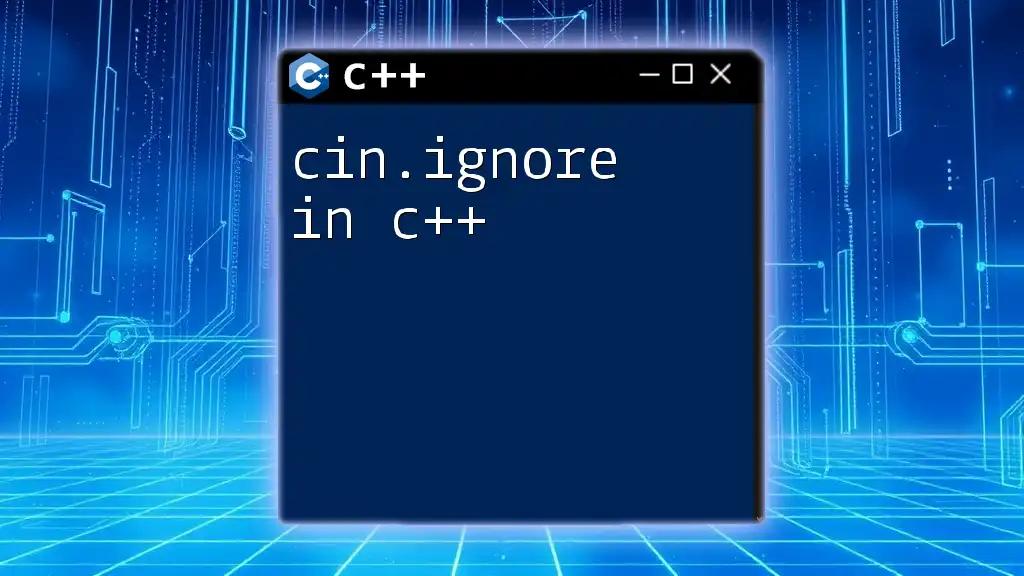
Performance Considerations
Efficiency of `islower`
The `islower` function is quite efficient. It operates directly on character types and is optimized for speed. However, it's important to compare its performance with other string handling methods in specific applications, as using `islower` may be a more straightforward approach than creating custom functions for such checks.
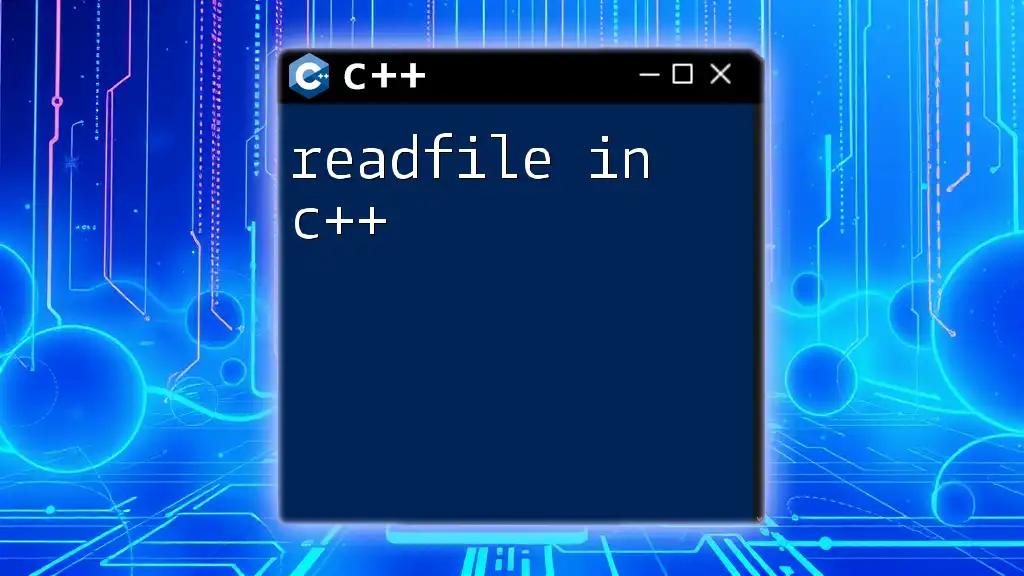
Conclusion
In summary, the `islower` function is an essential tool in C++ for character classification. It provides a simple yet effective way to check the case of characters, making it valuable for various applications such as input validation and text formatting. By mastering functions like `islower`, you can enhance your programming skills and improve the quality of your code.

Additional Resources
References for Further Reading
To explore more about character classification functions, refer to the C++ documentation available on official sites like cppreference.com or other reputable programming resources.
Related Functions
There are other useful character classification functions in C++, such as `isupper` for checking uppercase letters, `isdigit` for numeric digits, and many others. Exploring these functions will further enhance your understanding of character handling in C++.