In C++, the `delete` operator is used to free dynamically allocated memory to prevent memory leaks.
int* ptr = new int; // dynamically allocate memory
*ptr = 42; // assign a value
delete ptr; // free the allocated memory
Understanding the `delete` Operator in C++
The `delete` operator is a fundamental concept in C++ that plays a critical role in memory management. It is used to free up memory that was previously allocated using the `new` operator. In C++, when you allocate memory dynamically, it's your responsibility to release that memory. Failing to do so leads to memory leaks, which can degrade system performance over time.
![Effortless Memory Management: Delete[] in C++ Explained](/images/posts/d/delete-cpp.webp)
What is `delete`?
In the context of C++, the `delete` operator is a way to instruct the compiler that the memory block allocated for an object or an array is no longer needed. This is particularly important in C++ because it relies on manual memory management, unlike languages with automatic garbage collection.
When to Use `delete` in C++
Dynamic Memory Allocation is a common scenario where `delete` is necessary. Whenever you allocate an object or array using the `new` operator, you must use `delete` or `delete[]` to free that memory after you are finished using it.
Basic Syntax of the `delete` Operator
The syntax for using `delete` is straightforward:
delete pointer;
For example, if you dynamically allocate an integer and later want to release that memory, you would do it like this:
int* ptr = new int(42);
delete ptr; // Deleting dynamically allocated integer
This code allocates memory for an integer, assigns the value 42 to it, and then deletes that memory.
Using `delete` with Arrays
When dealing with arrays, it’s crucial to use `delete[]` instead of just `delete`. Using `delete` for an array can lead to undefined behavior and memory corruption. The correct syntax for deleting an array is:
delete[] pointer;
Here’s an example:
int* arr = new int[10];
delete[] arr; // Correct way to delete an array
This will properly deallocate the memory allocated for an array of 10 integers.
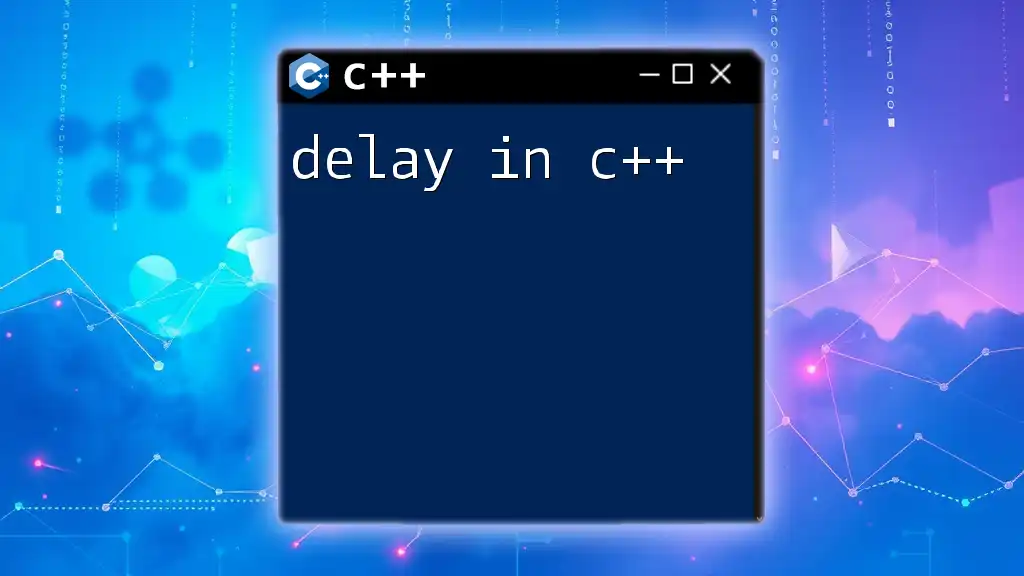
Common Mistakes with `delete`
Double Deletion
One of the most common mistakes involving `delete` is attempting to delete the same pointer more than once, known as double deletion. This not only leads to program crashes but also undefined behavior. Consider this example:
int* ptr = new int(10);
delete ptr;
delete ptr; // Undefined behavior
The first `delete` frees the memory correctly, but the second one attempts to delete memory that has already been freed, causing serious issues.
Not Deleting Allocated Memory
Another common issue is forgetting to delete memory that has been allocated dynamically, leading to memory leaks. If you fail to call `delete` on memory allocated using `new`, that memory remains allocated until the program ends, which can strain your system resources over time. Here’s a simple example:
int* leak = new int(20); // Memory allocated
// No calls to delete leak, leading to a memory leak.
In this case, the memory allocated for `leak` is never freed, resulting in a memory leak.
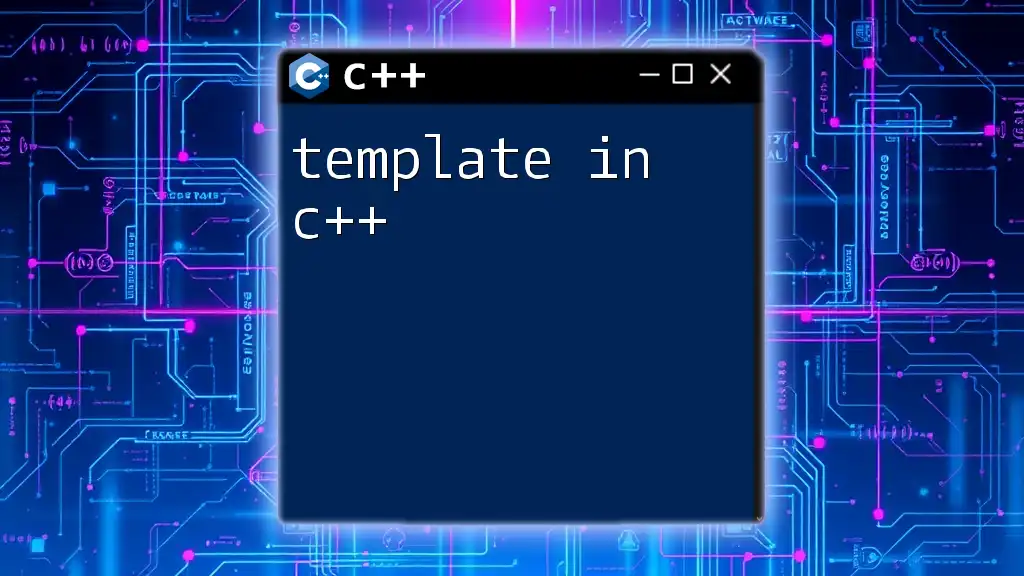
Key Variants of `delete`
`delete` vs. `delete[]`
It is essential to understand the difference between `delete` and `delete[]`. Use `delete` for single objects and `delete[]` for arrays. Using the wrong one can lead to subtle bugs and memory corruption. Here’s a small code snippet illustrating both:
int* single = new int(5);
int* multi = new int[5];
delete single;
delete[] multi; // Correct usage
Smart Pointers as Alternatives
Smart pointers like `std::unique_ptr` and `std::shared_ptr` can help manage memory automatically in C++. They automatically deallocate memory when the smart pointer goes out of scope, thereby reducing the chances of memory leaks and the need to manually call `delete`.
#include <memory>
std::unique_ptr<int> smartPtr(new int(10)); // Automatically managed
Using smart pointers is often considered best practice in modern C++ because they offer automatic resource management without the risks associated with manual memory management.
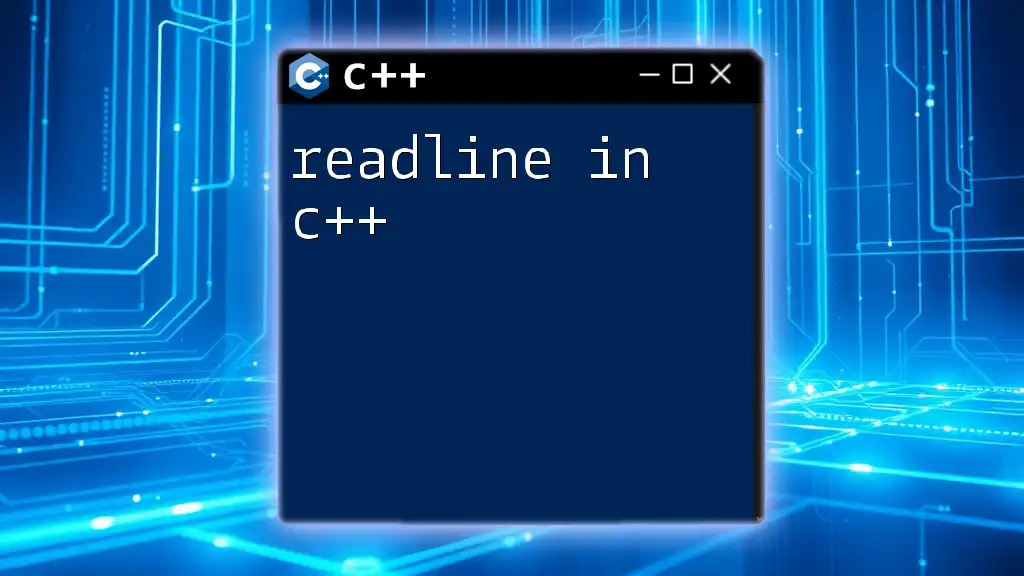
Summary of Best Practices with `delete`
Understanding when and how to safely use the `delete` operator is critical to managing memory in C++. Here are some guiding principles:
- Always pair `new` with `delete` and `new[]` with `delete[]`.
- Avoid double deletion by setting pointers to `nullptr` after deletion.
- Use smart pointers whenever possible to minimize manual memory management.
By adhering to these best practices, you can significantly reduce the risk of memory-related errors in your C++ programs.
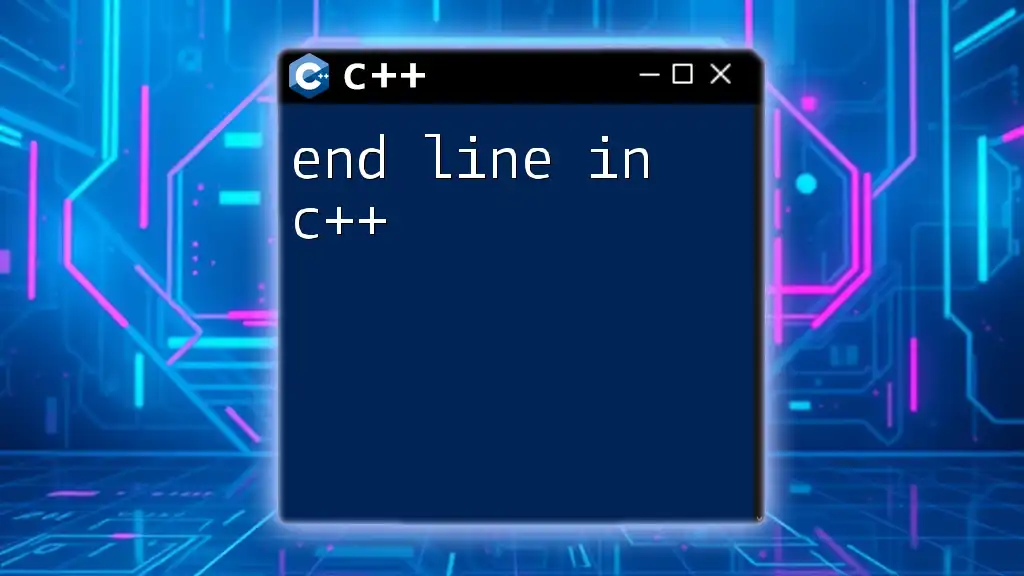
Conclusion
In summary, the `delete` operator is an essential tool for memory management in C++. It allows for the deallocation of memory that is no longer needed, helping to prevent memory leaks and ensure efficient resource management. Understanding the correct usage of `delete` and following best practices will make you a more effective C++ programmer.
Finally, practice is key. Write code, experiment with memory allocation, and get comfortable using `delete` in various scenarios to reinforce your understanding!