In C++, a template is a blueprint for creating generic classes or functions that allow you to work with any data type, enhancing code reusability and type safety.
Here’s a simple example of a function template that swaps two values:
#include <iostream>
using namespace std;
template<typename T>
void swapValues(T &a, T &b) {
T temp = a;
a = b;
b = temp;
}
int main() {
int x = 5, y = 10;
swapValues(x, y);
cout << "x: " << x << ", y: " << y << endl; // x: 10, y: 5
return 0;
}
What is a Template in C++?
A template in C++ is a powerful feature that allows developers to write generic and reusable code. By defining functions or classes with templates, you can operate with any data type without rewriting code for each type. This leads to more maintainable and less error-prone code. The essential benefits of using templates include:
- Code Generalization: Templates allow you to create a single function or class that works with different types, making your code more abstract and flexible.
- Type Safety: Templates ensure that the type checking is done at compile-time, which helps catch errors early in the development process.
- Reduction of Code Duplication: Instead of writing multiple versions of a function/class for different data types, you can define one version that works for all.
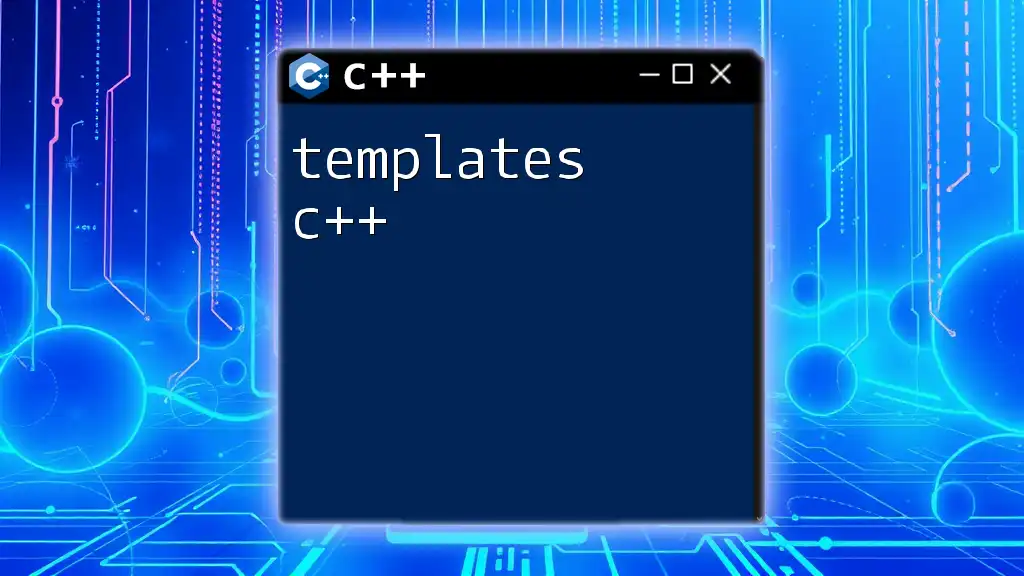
Types of Templates in C++
Function Templates
A function template defines a blueprint for creating functions that can take parameters of different data types. The syntax to declare a function template starts with the `template` keyword followed by template parameters within angle brackets.
Here's an example of a simple function template:
template <typename T>
T add(T a, T b) {
return a + b;
}
In this example, `T` is a placeholder for any data type. When you call `add`, the compiler generates the appropriate function based on the types of arguments you provide.
Usage Example:
int main() {
std::cout << add(5, 3); // Outputs: 8
std::cout << add(5.5, 3.2); // Outputs: 8.7
}
This demonstrates how a single function template can handle both integers and floating-point numbers seamlessly.
Class Templates
Class templates extend the concept of function templates to classes, allowing you to create classes that can handle different data types.
Here’s a basic example of a class template:
template <typename T>
class Box {
private:
T data;
public:
Box(T d) : data(d) {}
T getData() { return data; }
};
In this example, `Box` is a class that can contain a value of any type defined at the point of instantiation.
Usage Example:
int main() {
Box<int> intBox(10);
Box<std::string> strBox("Hello");
std::cout << intBox.getData(); // Outputs: 10
std::cout << strBox.getData(); // Outputs: Hello
}
This flexibility demonstrates how class templates help reduce redundancy across data types.
Variadic Templates
Variadic templates allow you to define templates that accept a variable number of template parameters. This can simplify function definitions that need to process varying types and counts of arguments.
For example, this function can take any number of arguments:
template <typename... Args>
void print(Args... args) {
(std::cout << ... << args) << '\n';
}
The above function uses a fold expression, a feature introduced in C++17, to print all arguments passed to it.
Usage Example:
int main() {
print(1, 2, 3); // Outputs: 123
print("Hello", " ", "world!"); // Outputs: Hello world!
}
Variadic templates add significant flexibility in handling function arguments and enhance the function’s usability.
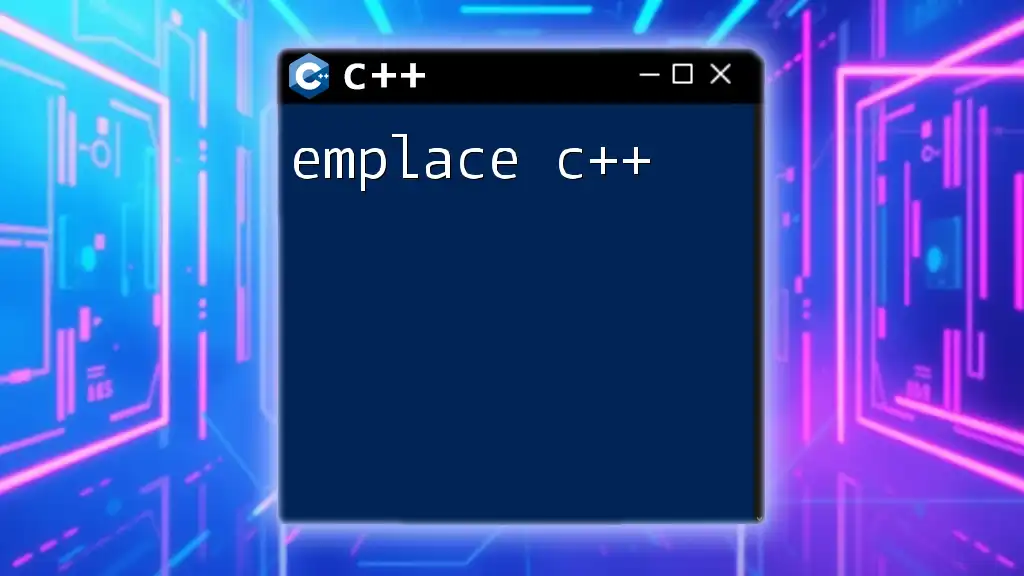
How to Use Templates in C++
Template Declaration
The syntax for declaring templates, both function and class templates, requires the `template` keyword followed by template parameters. Best practices include using clear names for template types and maintaining a consistent structure within your code to enhance readability and understanding.
Template Specialization
Template specialization allows you to define specific implementations for specific types. This custom behavior for particular types is essential when you need different functionality for different data types.
Here is an example of template specialization for a specific data type:
template <>
class Box<int> {
private:
int data;
public:
Box(int d) : data(d) {}
int getData() { return data * 2; } // special behavior for int
};
In this case, the `Box` class is specialized for the `int` type, which alters the method `getData()` to return double the stored value. This approach gives you the ability to customize the functionality as needed.
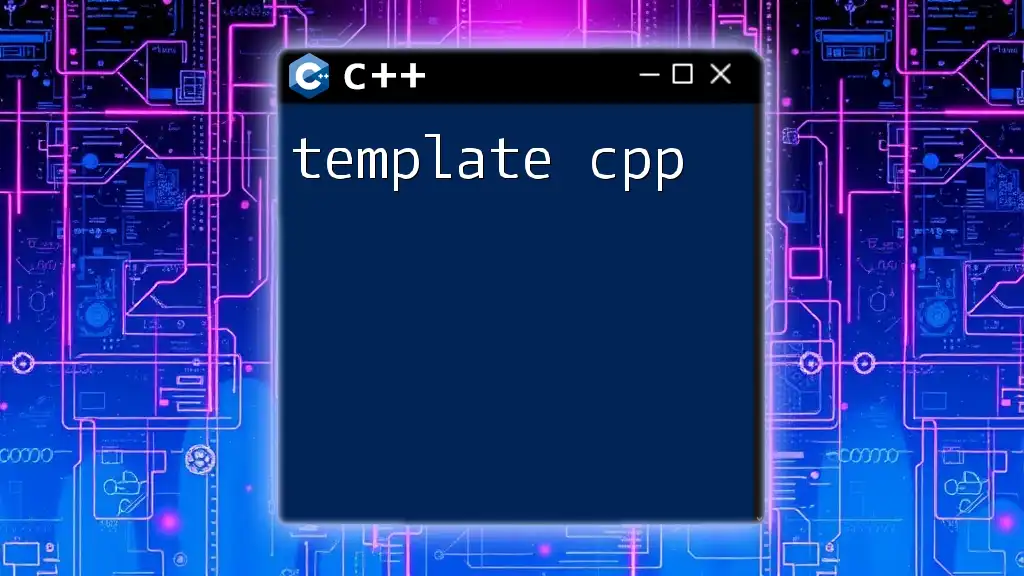
Common Use Cases for C++ Templates
Templates facilitate generic programming, which is a paradigm aimed at abstracting and generalizing solutions through code reusability. Here are a few common scenarios:
- Data Structures: Utilizing templates to create generalized data structures like linked lists, stacks, or queues, helping to keep your codebase clean and reusable.
- Algorithms: Many operations, such as sorting and searching, can be implemented generically with templates, allowing them to work with types like integers, strings, or custom objects without rewriting code.
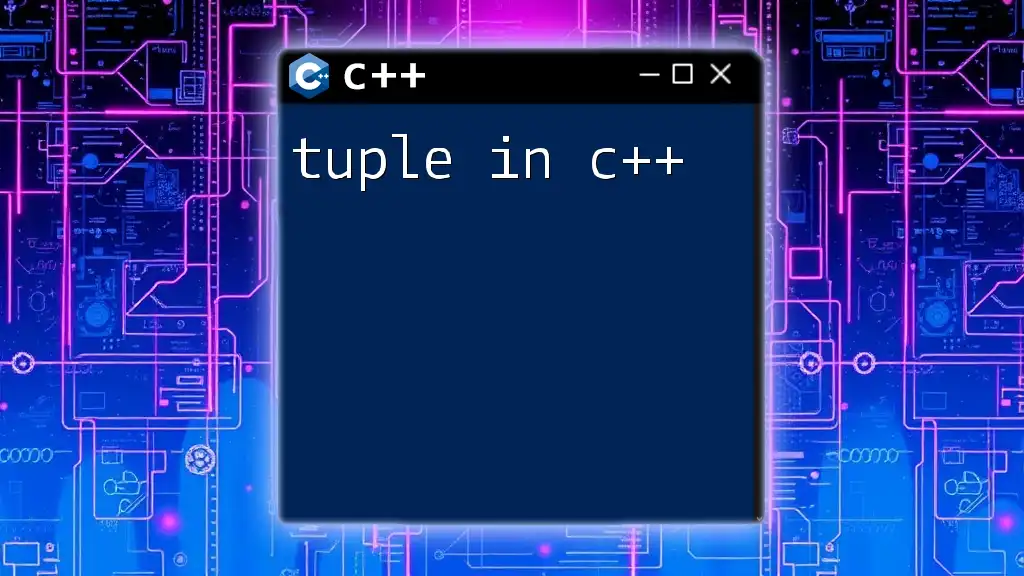
Limitations and Pitfalls of Using Templates
Complexity
One of the primary drawbacks of using templates in C++ is the added complexity they can introduce. Understanding and debugging code that heavily uses templates can be challenging for both novice and experienced programmers.
Compilation Time
Templates can lead to increased compilation times because the compiler generates new code for each instance of a template with a different type. This can slow down build times, particularly in large projects.
Error Messages
Debugging template errors can be daunting. When a template generates an error, the messages can be obscure or overly complex, making it difficult to pinpoint the issue. Familiarity with template mechanisms and error messages can help mitigate this problem.
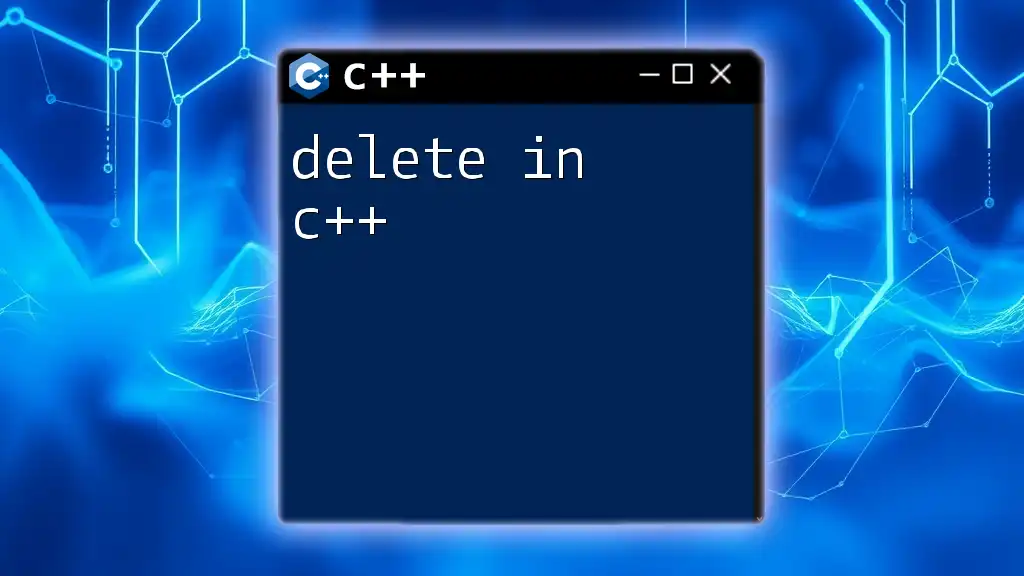
Conclusion
In summary, templates are a vital feature of C++ that enhance code reusability, type safety, and generalization. Whether you are working with function templates, class templates, or even variadic templates, understanding these concepts allows you to create more maintainable and efficient code. By practicing and applying templates in your projects, you can significantly improve your programming skills and code quality.
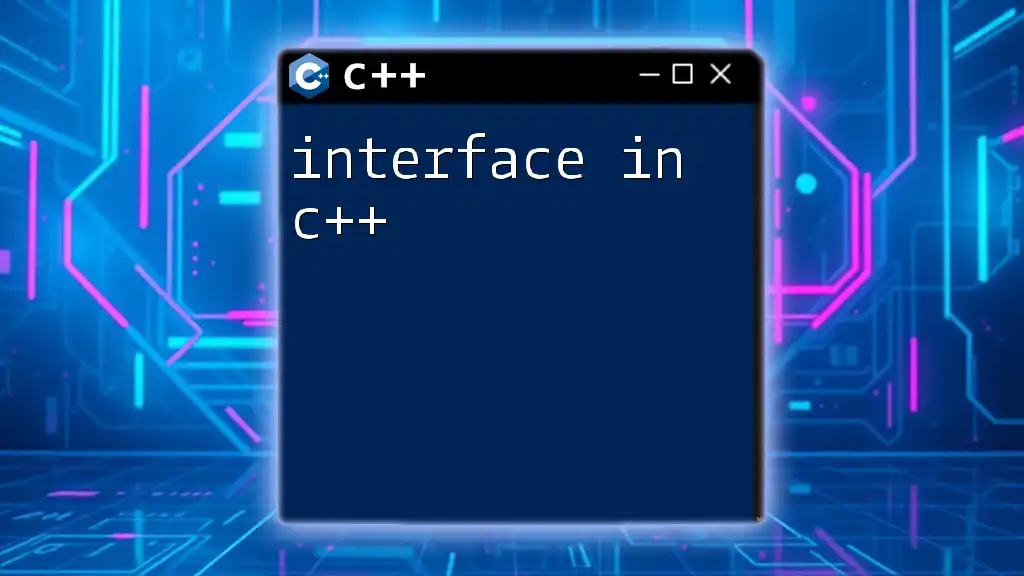
Additional References
For a deeper understanding of templates in C++, consult resources such as the official C++ documentation, online tutorials, and specialized books on advanced C++ programming. Engaging in practical coding exercises with templates will solidify your understanding and application of this powerful feature.