A Makefile for C++ is a simple script that helps automate the build process, allowing you to compile your C++ programs efficiently with defined rules and dependencies. Here’s a basic template:
# Makefile template for C++ project
# Compiler
CXX = g++
# Compiler flags
CXXFLAGS = -Wall -g
# Source and executable files
SRC = main.cpp utils.cpp
TARGET = my_program
# Build executable
$(TARGET): $(SRC)
$(CXX) $(CXXFLAGS) $(SRC) -o $(TARGET)
# Clean up generated files
clean:
rm -f $(TARGET)
What is a Makefile?
A Makefile is a special file that defines a set of directives used with the make build automation tool. It is primarily utilized in programming, particularly in C and C++ development, to manage the building and compiling of projects. Rather than having to type long commands to compile each source file, a Makefile allows developers to automate the compilation process, making it efficient and reproducible.
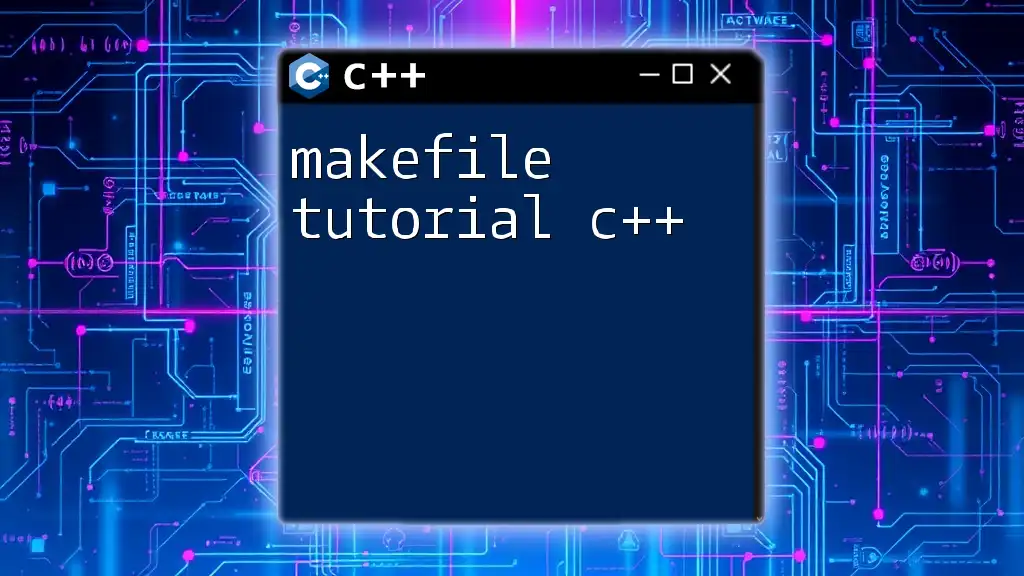
Benefits of Using Makefiles
Using Makefiles presents various advantages, particularly in enhancing the software development workflow:
-
Automation of Build Processes: By defining build rules in a Makefile, developers can compile their projects simply by running the command `make`, which saves time and minimizes manual errors.
-
Streamlining Project Management: Makefiles help to organize and streamline the building and linking stages of software projects. This organizational aspect becomes crucial as projects grow in size and complexity.
-
Handling Dependencies Efficiently: Makefiles automatically track dependencies between files. If you modify one file, only the files that depend on it will be recompiled, which greatly speeds up the development process.
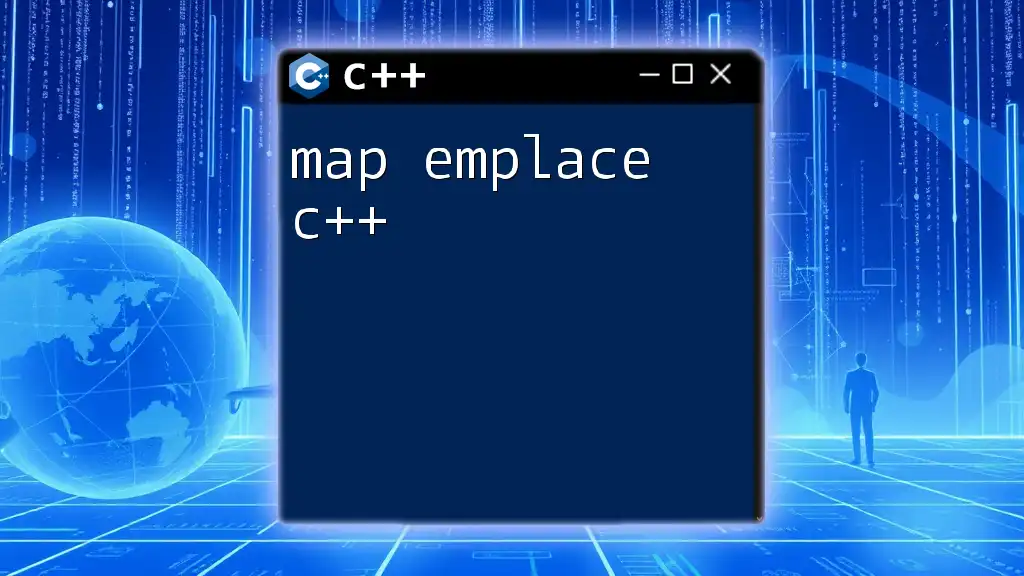
Basic Structure of a Makefile
A Makefile consists of three primary components: targets, prerequisites, and recipes. Understanding these components is essential for creating effective Makefiles.
Components of a Makefile
-
Targets: These define the output files or labels that represent specific tasks in the build process. The first target is often the default action.
-
Prerequisites: These are the input files that the target depends on. If any prerequisite files change, the target will be rebuilt.
-
Recipes: This is the command to execute that builds the target, often consisting of compiler commands.
Key Terms Explained
To clarify the use of Makefiles, here are some key terms:
- Target: The specific file or action to be generated or executed.
- Prerequisite: The source files or component files needed to create the target.
- Recipe: The commands run to create or update the target from its prerequisites.
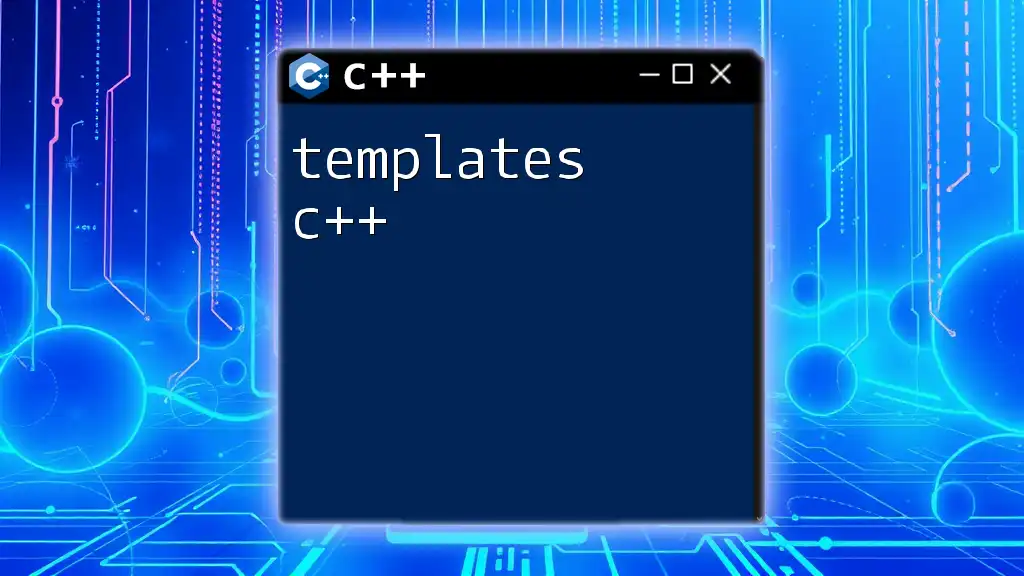
Creating a Simple C++ Makefile
Creating a Makefile doesn't have to be daunting. Here's a simple step-by-step guide to write a basic Makefile for a C++ project.
Example Code Snippet: Basic Makefile Structure
# Simple Makefile Example
CC = g++
CFLAGS = -Wall -g
all: my_program
my_program: main.o utils.o
$(CC) -o my_program main.o utils.o
main.o: main.cpp
$(CC) $(CFLAGS) -c main.cpp
utils.o: utils.cpp
$(CC) $(CFLAGS) -c utils.cpp
clean:
rm -f *.o my_program
In this example, the Makefile specifies the compiler (g++), the flags for warnings and debugging information, and defines the target `my_program`. The rules specify how to compile `.o` files from their corresponding `.cpp` files. The `clean` target is a conventional target that helps to remove generated files, ensuring a clean state for fresh builds.
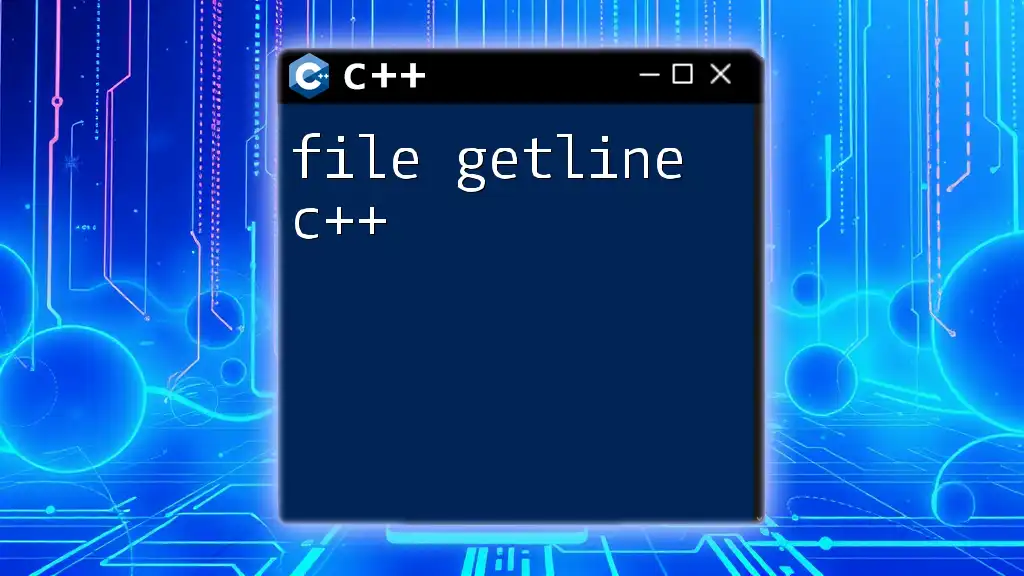
Advanced Makefile Features
As you become familiar with basic Makefiles, you may want to explore advanced features that can provide greater control and efficiency.
Variable Definitions
Using variables within your Makefile allows for cleaner and more maintainable code. You can define variables for compiler options, source files, or other repeated tasks.
Example: Using Variables for Compiler Options
# Advanced Makefile Example
CC = g++
CFLAGS = -Wall -Wextra -O2
SOURCES = main.cpp module1.cpp module2.cpp
OBJECTS = $(SOURCES:.cpp=.o)
TARGET = my_advanced_program
all: $(TARGET)
$(TARGET): $(OBJECTS)
$(CC) -o $@ $^
%.o: %.cpp
$(CC) $(CFLAGS) -c $<
clean:
rm -f $(OBJECTS) $(TARGET)
Phony Targets
Phony targets are targets that do not represent files. They help improve build efficiency and clarity. A common use for phony targets is the `clean` target, as it doesn't produce an output file but provides useful functionality.
.PHONY: all clean
By defining `all` and `clean` as phony, we prevent make from getting confused by files with the same name.
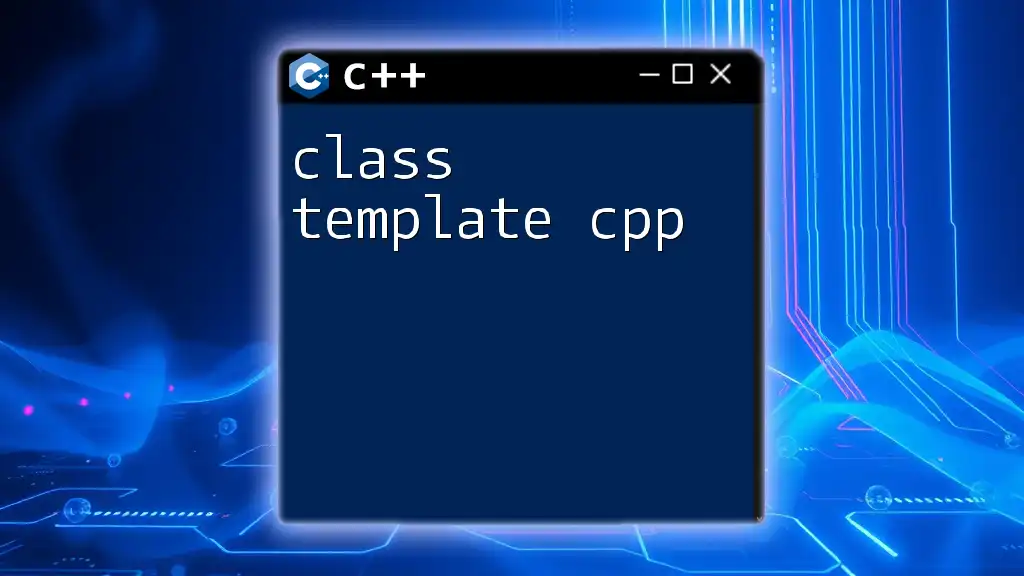
Example C++ Makefile for a Complex Project
When handling larger projects, it’s vital to understand how to structure your Makefile effectively. A multi-file C++ project will often involve numerous source and header files.
Structure of a Multi-file C++ Project
An effective directory layout is key for maintaining clarity, particularly in larger projects. It’s common to organize files into directories such as `src/` for source files, `include/` for header files, and `bin/` for binaries.
Makefile Example C++ for a Multi-file Project
# Example C++ Makefile for a Complex Project
CC = g++
CFLAGS = -Wall -O2
SOURCES = src/main.cpp src/module1.cpp src/module2.cpp
OBJECTS = $(SOURCES:.cpp=.o)
TARGET = bin/my_complex_program
all: $(TARGET)
$(TARGET): $(OBJECTS)
$(CC) -o $@ $^
src/%.o: src/%.cpp
$(CC) $(CFLAGS) -c $< -o $@
clean:
rm -f $(OBJECTS) $(TARGET)
In this example, the Makefile references source files located in a `src/` directory and outputs the final binary to a `bin/` directory. This separation enhances organization and clarity.
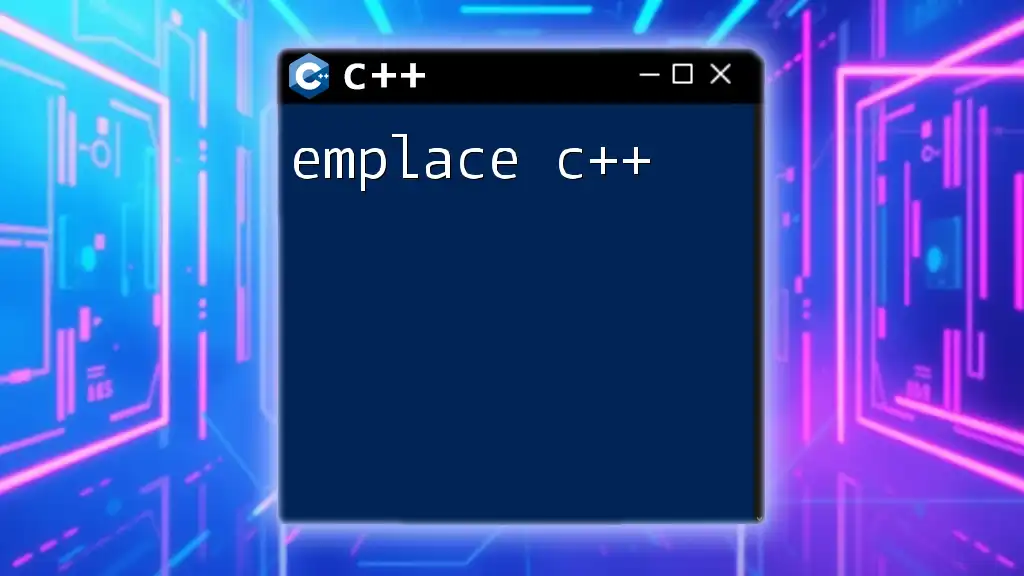
Tips for Writing Effective Makefiles
Creating an effective Makefile requires attention to detail and best practices:
Modularity and Reusability
A great practice in building Makefiles is to create modular rules and targets that can be reused across various projects. This not only saves time but also encourages a cleaner build configuration.
Commenting and Documentation
Documenting your Makefile comprehensively is essential. Comments help in guiding others (or yourself in the future) through the purpose and functionality of various sections. Proper documentation significantly enhances maintainability.
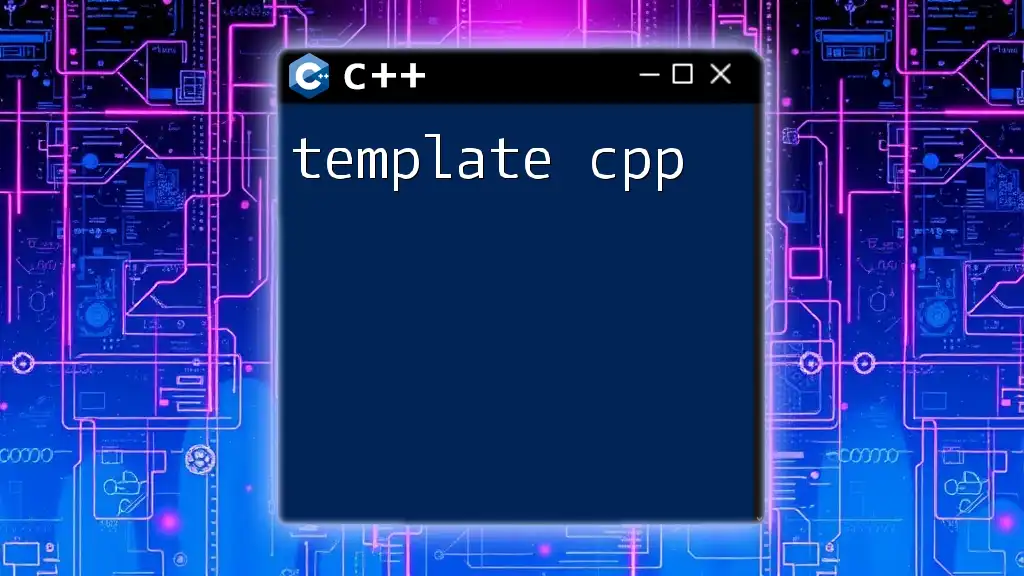
Troubleshooting Common Makefile Issues
Even seasoned developers encounter errors while using Makefiles. Here are common scenarios and how to deal with them:
Debugging Compilation Errors
When compilation errors occur, analyzing the error messages provided by the Make process is crucial. It will guide you towards the problematic files or dependencies.
Common Makefile Pitfalls
It’s easy to forget dependencies, resulting in parts of your project not recompiling as expected. Always ensure that all source files are accounted for in the appropriate rules. Additionally, misunderstandings of phony targets may lead to unintended behaviors; clarity in your target definitions is essential.
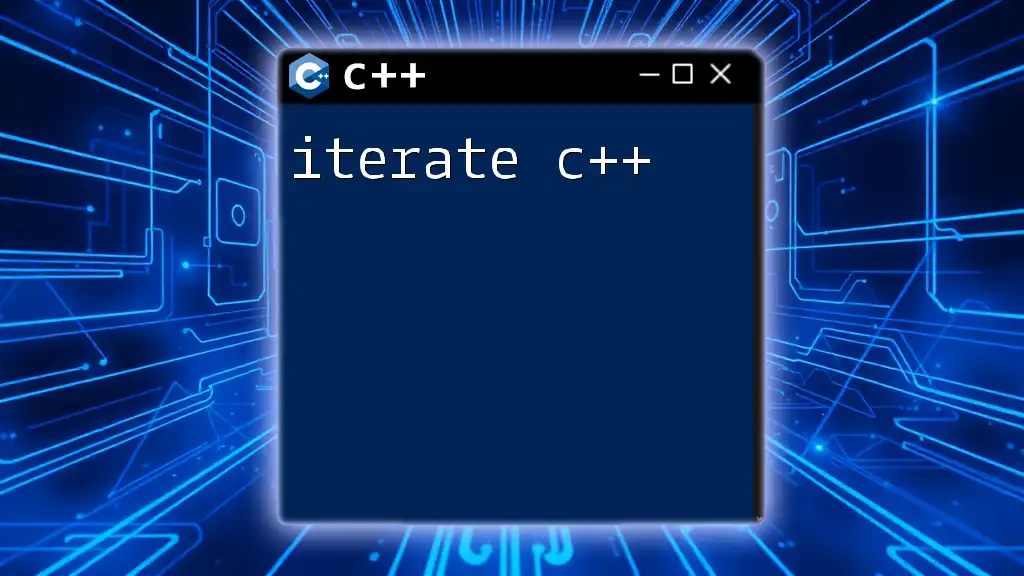
Conclusion
Makefiles are indispensable tools in C++ development. By mastering the art of creating an effective Makefile template, you can streamline your workflow, manage dependencies effectively, and enhance overall project organization. Implementing best practices and understanding advanced concepts will lead to a more efficient development process.
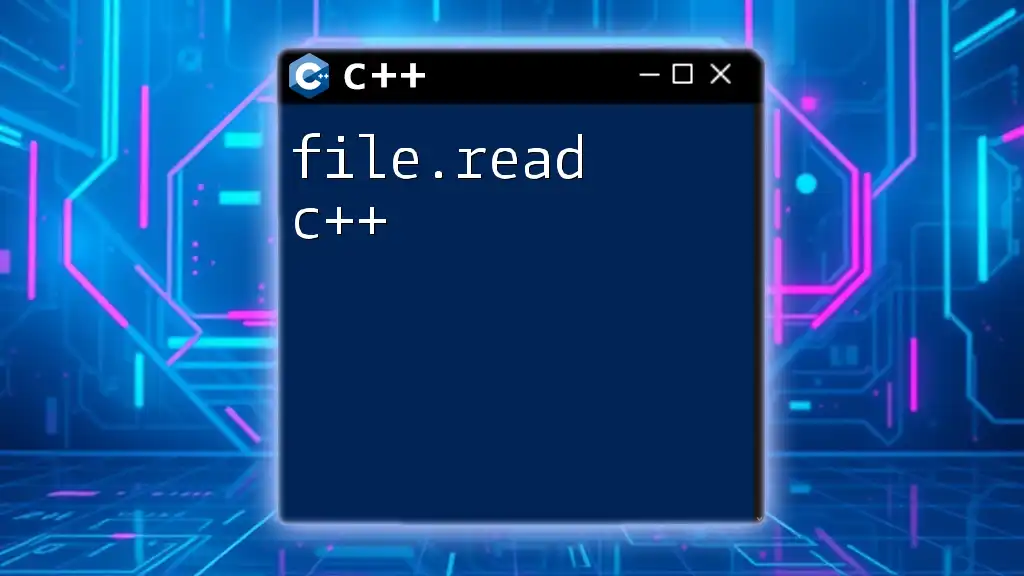
Additional Resources
To further enrich your learning experience in C++ and build automation, some resources to consider include online Makefile generators, community forums, and comprehensive guides available on reputable programming websites. Each of these resources can significantly bolster your understanding of Makefiles and reinforce your programming skills.