The `getline` function in C++ is used to read an entire line from a file into a string variable, while handling spaces and special characters seamlessly.
Here’s a code snippet demonstrating its usage:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream infile("example.txt");
std::string line;
if (infile.is_open()) {
while (std::getline(infile, line)) {
std::cout << line << std::endl;
}
infile.close();
}
return 0;
}
Understanding `getline` in C++
What is `getline`?
`getline` is a standard function in C++ that is used to read an entire line from an input stream. Unlike the regular `cin` function, which reads input until a space is reached, `getline` allows reading until a newline character is found, making it particularly useful for reading strings that may contain whitespace.
Why Use `getline` in C++?
Using `getline` provides several advantages over other input methods. It is particularly beneficial when:
- You need to read lines of text, especially from files.
- Whitespace characters within the line are relevant to the data you're processing.
- You are dealing with lines of unknown or varying lengths, as `getline` dynamically allocates the necessary space for the input.
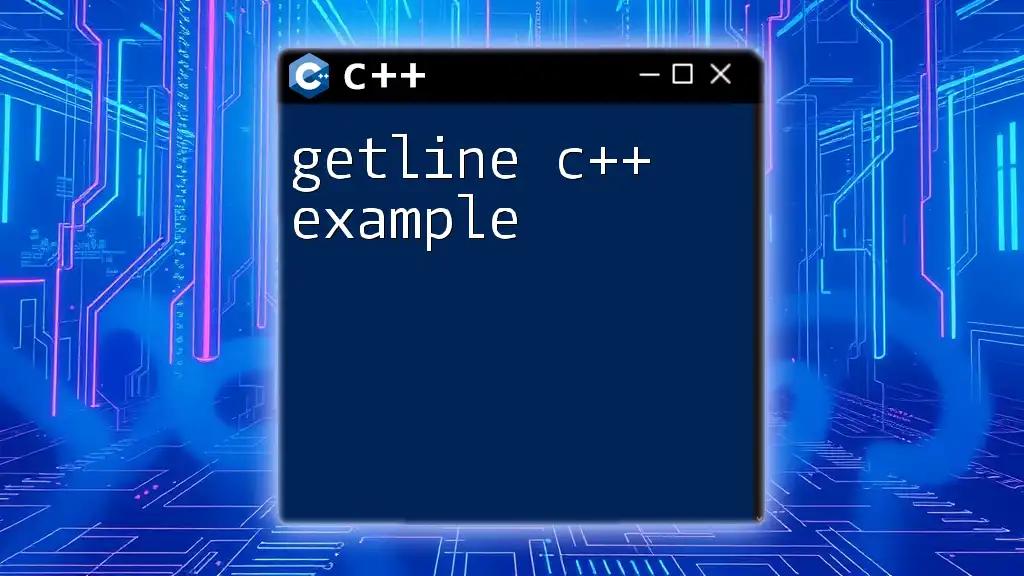
Setting Up Your Development Environment
Required Tools and Compilers
Before starting with `getline`, ensure you have a suitable development environment in place. For C++, you will typically need:
- A C++ compiler such as GCC, Clang, or MSVC.
- An integrated development environment (IDE) or code editor such as Visual Studio Code, Code::Blocks, or CLion.
Creating a Sample File
To effectively demonstrate how to use `file getline c++`, create a sample text file. You may name it `example.txt` and include some lines of text like:
Hello, World!
Welcome to C++ programming.
This is a file reader example.
Enjoy learning!
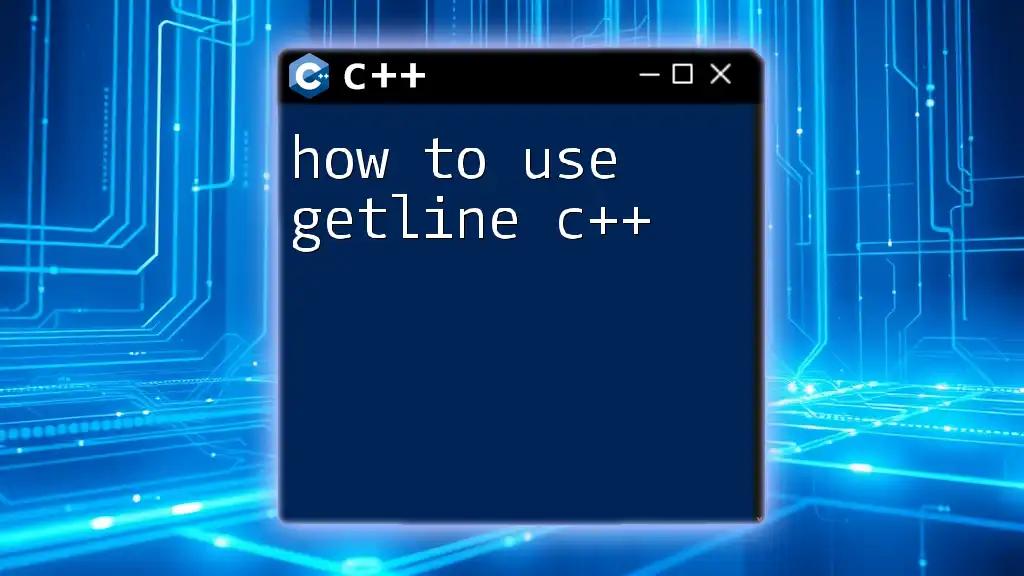
Using `getline` to Read From a File
Opening a File Stream
To read from a file, you first need to open a file stream. In C++, you can achieve this using the `std::ifstream` class from the `<fstream>` library. Here’s how to do it:
#include <fstream>
#include <iostream>
#include <string>
int main() {
std::ifstream infile("example.txt");
// Check if the file was opened successfully
if (!infile) {
std::cerr << "Error opening file!" << std::endl;
return 1;
}
return 0;
}
In this snippet, we attempt to open `example.txt`. If the file doesn't exist or cannot be opened, an error message is printed.
Reading Lines from a File
Once the file is opened successfully, you can use `getline` to read the lines. The syntax for using `getline` is straightforward:
std::getline(input_stream, string_variable);
Example: Basic Usage of `getline` with a File
Here is a full example demonstrating how to read lines from a file using `getline`:
#include <fstream>
#include <iostream>
#include <string>
int main() {
std::ifstream infile("example.txt");
std::string line;
while (getline(infile, line)) {
std::cout << line << std::endl; // Output each line
}
infile.close();
return 0;
}
In this example, the program loops through each line of the file and prints it to the console. Each iteration calls `getline`, which reads a new line until it reaches the end of the file.
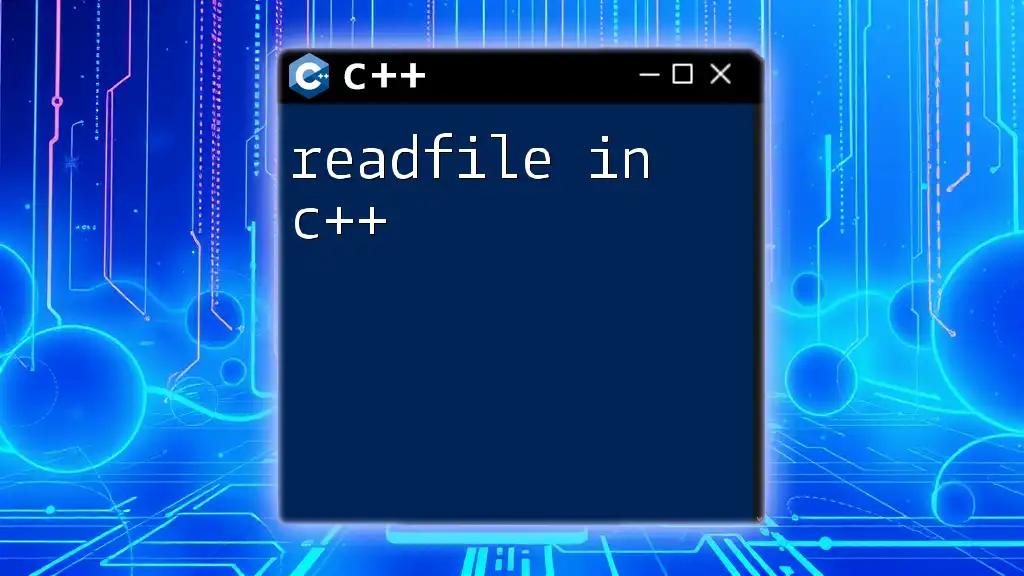
Handling Different Data Formats
Reading Lines with Variable Content
When working with text files, it's common to encounter lines of different lengths. `getline` handles this seamlessly, allowing you to read lines without needing to worry about their size.
Dealing with Empty Lines and Whitespace
Sometimes, your file may contain empty lines or lines with only whitespace. You can easily manage these cases by checking the content of the line after reading it.
if (getline(infile, line) && !line.empty()) {
std::cout << "Line: " << line << std::endl; // Process non-empty lines only
}
This approach ensures that you only work with useful lines, avoiding unnecessary processing of empty data.
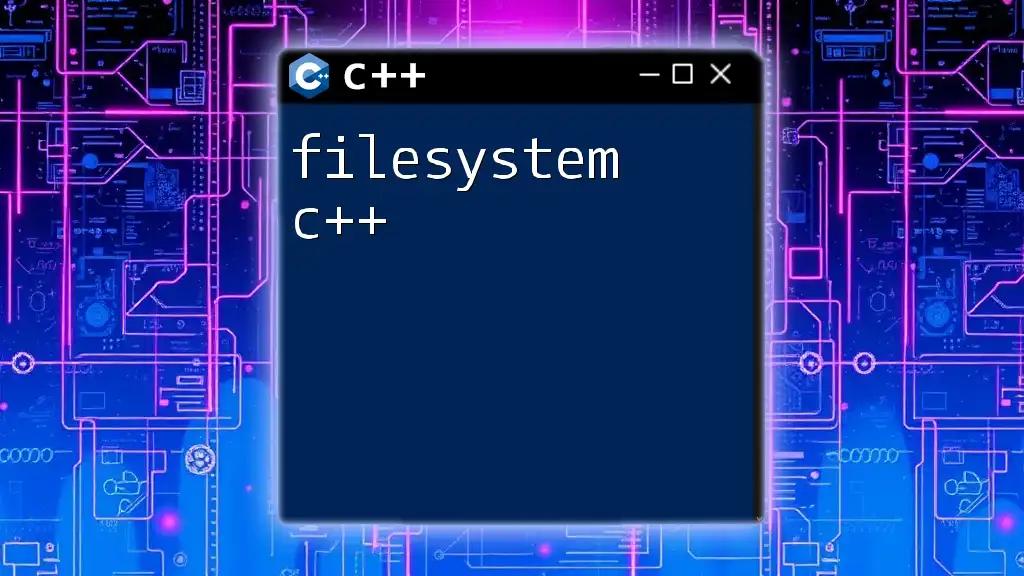
Error Handling and File Validation
Checking for File Open Errors
It's crucial to handle errors gracefully when opening files. Always verify if the file was opened correctly. This avoids runtime errors and unexpected behavior. Use `if (!infile)` as demonstrated in previous examples to check for successful openings.
Handling Reading Errors
In addition to file opening errors, you may encounter issues while reading. Using returned values from `getline` is a practice that helps in error handling:
if (!getline(infile, line)) {
std::cerr << "Error reading the line!" << std::endl;
}
This checks whether reading the line was successful, allowing for robust code execution.
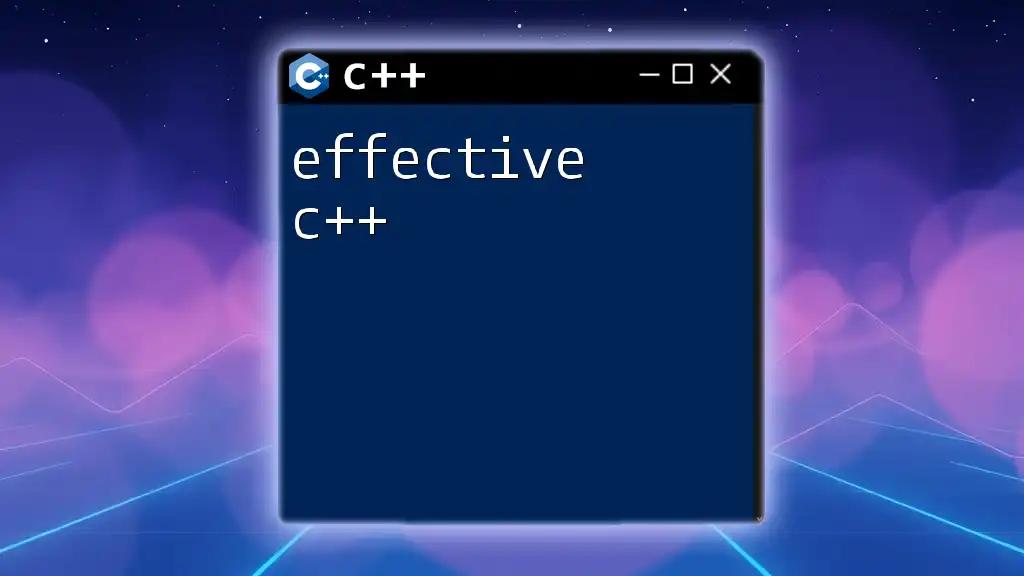
Advanced Usage of `getline`
Reading into Different Data Types
Often, you may need to convert the content read from the file to different data types (e.g., integers or doubles). This can be performed after reading the line.
std::string line;
while (getline(infile, line)) {
int number = std::stoi(line); // Convert string to integer
std::cout << "Number: " << number << std::endl;
}
In this example, each line is parsed as an integer, demonstrating how to manipulate the data after reading.
Custom Delimiters in `getline`
By default, `getline` reads until a newline character. However, you can specify custom delimiters, which is useful when reading formatted data such as CSV files.
std::ifstream infile("data.csv");
std::string line;
while (getline(infile, line, ',')) { // Custom delimiter
std::cout << line << std::endl; // Process each segment separated by comma
}
This allows the reading of each value in a line separated by commas, expanding the versatility of `getline`.
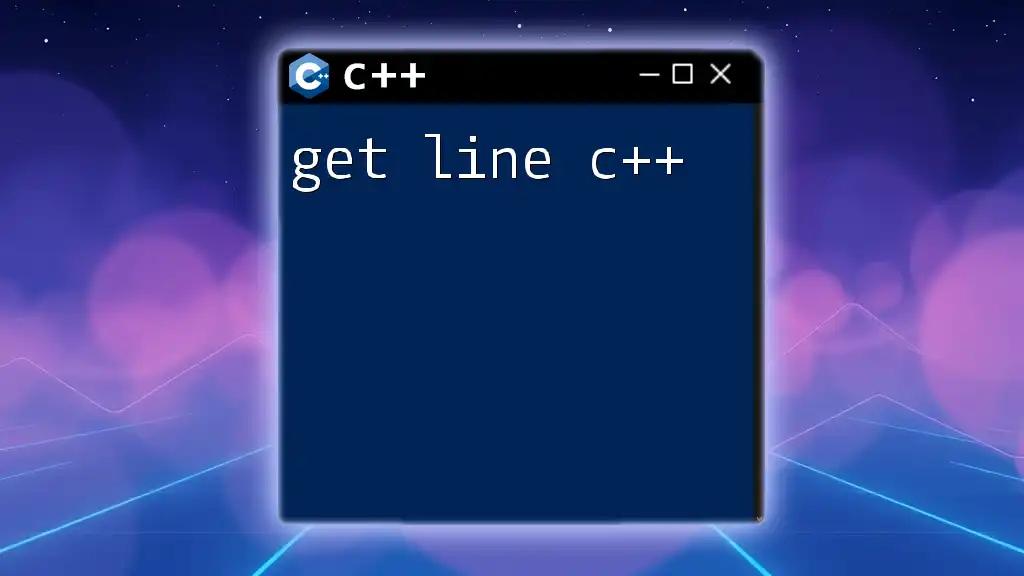
Practical Applications
Common Use Cases for `getline` with Files
`getline` has numerous practical applications, including:
- Reading configuration files.
- Parsing data files containing logs.
- Processing user input from large text files.
Reading Configuration Files
One practical application is reading configuration settings from a file where each line might represent a key-value pair. You can easily split and process these values for use in your applications.
while (getline(infile, line)) {
size_t pos = line.find('=');
if (pos != std::string::npos) {
std::string key = line.substr(0, pos);
std::string value = line.substr(pos + 1);
std::cout << "Key: " << key << ", Value: " << value << std::endl;
}
}
In this snippet, you parse each line to extract keys and values, facilitating dynamic configuration handling.
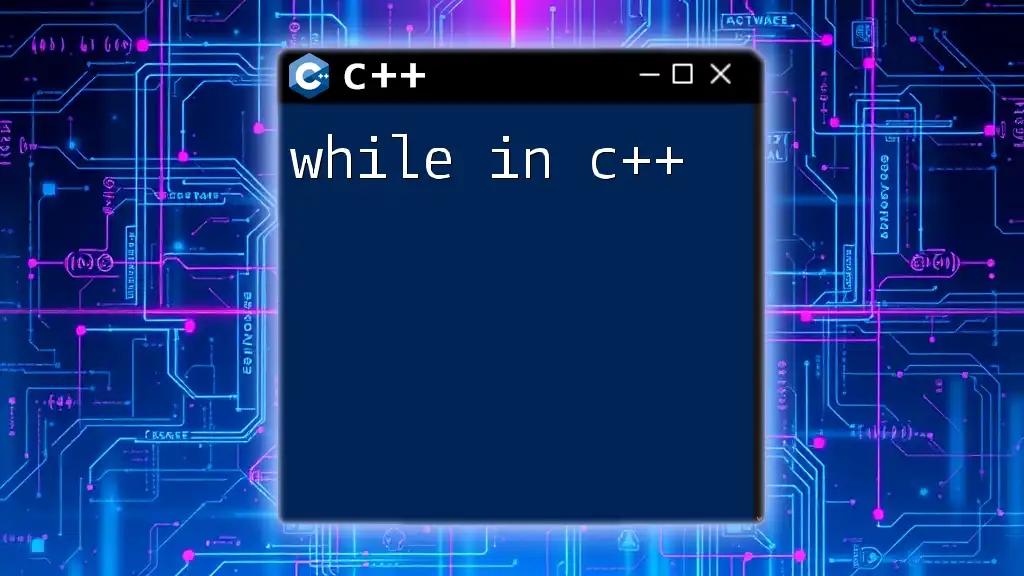
Conclusion
Recap of Key Takeaways
In summary, using `file getline c++` provides a powerful and flexible way to handle text input from files in C++. By mastering this function, you can efficiently read lines, manage variable content, and perform necessary conversions—all while ensuring robust error handling.
Next Steps in Learning C++
To build on your knowledge, consider exploring more advanced topics such as file manipulation, working with other input/output streams, or delving into data structures that can enhance your file processing capabilities.
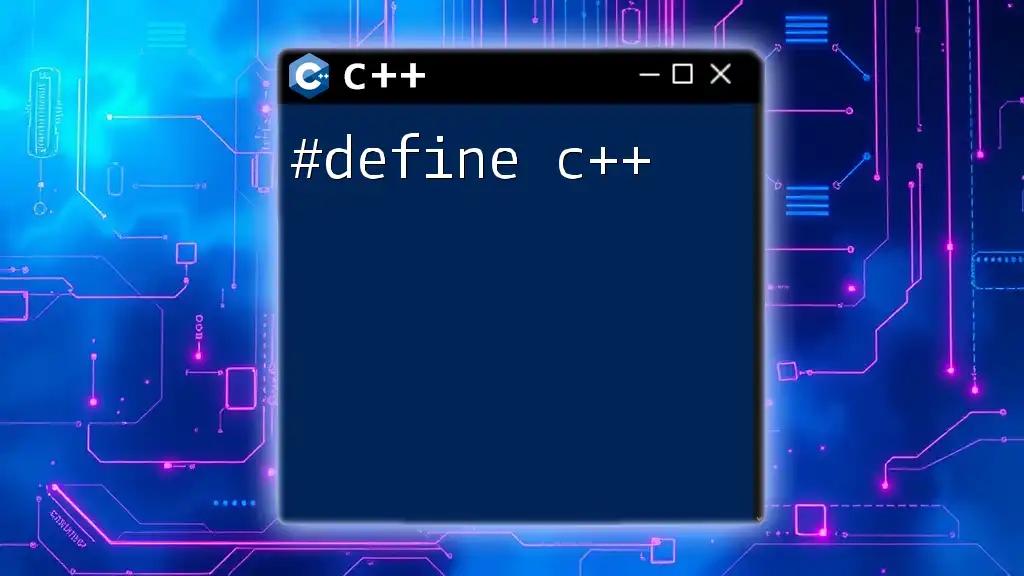
Additional Resources
For further exploration, check out the official C++ documentation on file handling and `getline`. Websites like Stack Overflow and C++ forums can provide additional insights and community support as you continue your learning journey.