The `#define` directive in C++ is used to create symbolic constants or macros, allowing you to define values or code snippets that can be easily reused throughout your program.
#define PI 3.14159
#define SQUARE(x) ((x) * (x))
#include <iostream>
int main() {
std::cout << "Value of PI: " << PI << std::endl;
std::cout << "Square of 5: " << SQUARE(5) << std::endl;
return 0;
}
Understanding Preprocessor Directives
What are Preprocessor Directives?
Preprocessor directives are commands that instruct the compiler to preprocess the code before actual compilation begins. They provide instructions that can alter how the code is treated, enabling developers to maintain flexibility and efficiency in their programming practices.
It's essential to distinguish between preprocessor directives and regular C++ code: while the latter is executed, directives are handled prior to compilation and aren't part of the compiled program.
Why Use Preprocessor Directives?
Using preprocessor directives can lead to significant advantages, including:
- Code Optimization: By removing unnecessary parts of code during compilation, developers can enhance performance.
- Managing Platform-Specific Code: Preprocessor directives allow code to adapt based on the environment it’s compiled in, making it highly versatile.
- Resource Management: Large projects can maintain various pieces of functionality without including every element in every build, reducing bloat and complexity.
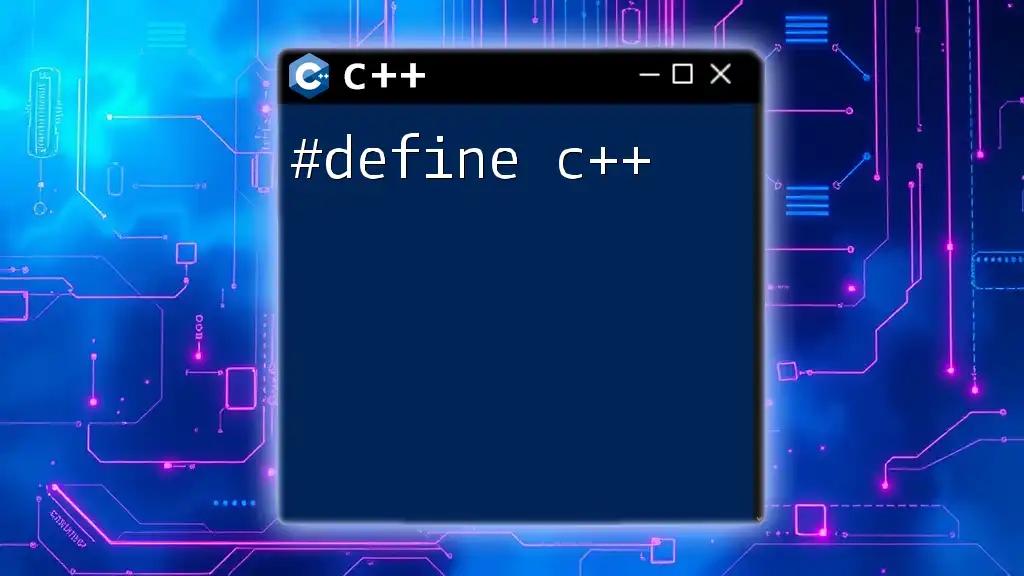
The Basics of the `#define` Directive
What is `#define`?
The `#define` directive is one of the most fundamental preprocessor directives in C++. It serves as a way to define macros—essentially placeholders that can represent constants or expressions throughout your program.
Simple Example of `#define`
Using `#define`, you can create constants that can be reused, improving maintainability. For instance:
#define PI 3.14
Here, `PI` is a macro that will be replaced with `3.14` wherever it appears in the code. This practice not only makes the code clearer but also allows for easy adjustments—change the value in one place, and the change takes effect throughout the program.

Introduction to Conditional Compilation
What is Conditional Compilation?
Conditional compilation allows developers to include or exclude parts of code based on certain conditions. This is especially useful for debugging, enabling or disabling features, or managing platform-specific capabilities without manually changing the code.
Common Use Cases for Conditional Compilation
- Debugging: You can compile debugging information without affecting the release build.
- Feature Toggling: Easily enable or disable features during development or deployment.
- Cross-Platform Development: Include or exclude code for different operating systems or environments.
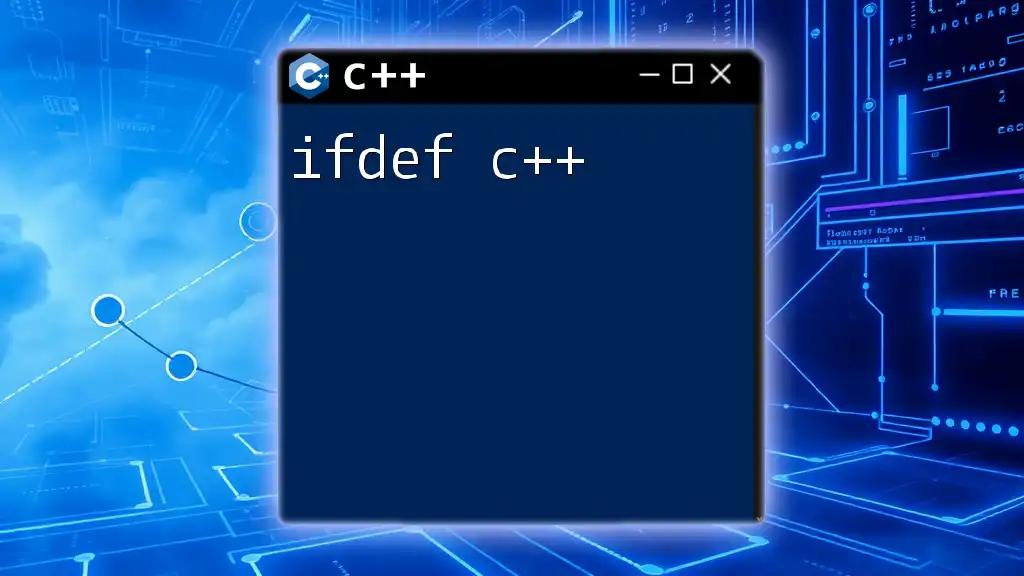
The `#ifdef` and `#ifndef` Directives
What is `#ifdef`?
The `#ifdef` directive allows you to check if a macro has been defined. This is crucial for tailoring code to specific conditions.
Example of `#ifdef`
Consider the following example:
#define DEBUG_MODE
#ifdef DEBUG_MODE
std::cout << "Debug mode is enabled!" << std::endl;
#endif
In this case, if `DEBUG_MODE` is defined, the message "Debug mode is enabled!" will be printed. If not, this portion of code will be ignored, keeping the output clean.
What is `#ifndef`?
The `#ifndef` directive is the opposite of `#ifdef`. It checks whether a macro has not been defined.
Example of `#ifndef`
For example:
#ifndef VERSION
#define VERSION 1.0
#endif
Here, `VERSION` is defined only if it hasn’t been defined previously. This prevents redefinition and ensures that settings are not altered unintentionally.
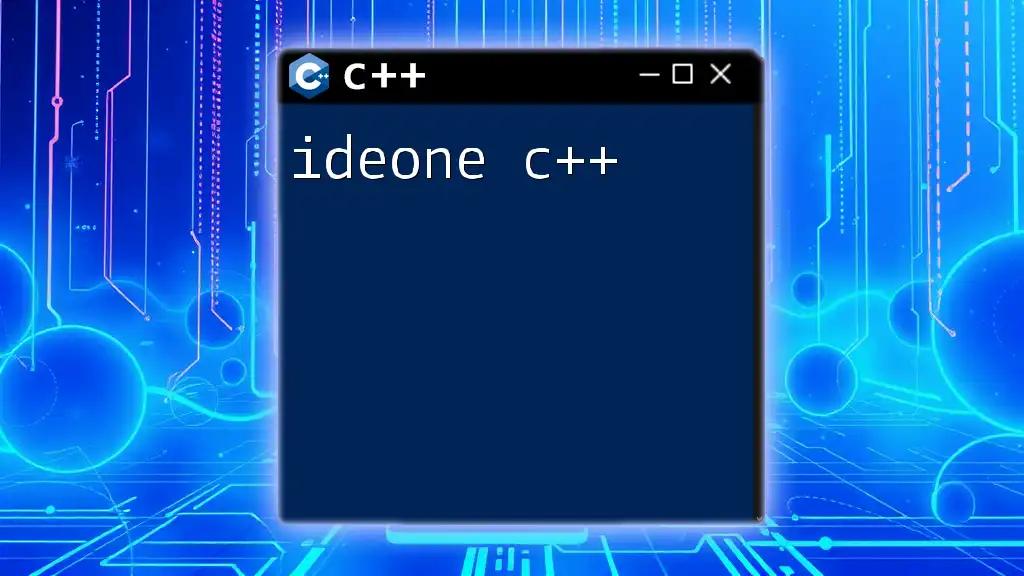
The `#if` and `#elif` Directives
Understanding `#if`
The `#if` directive allows for evaluating constant expressions, introducing flexibility in compiling based on defined values.
Example of `#if`
Consider this snippet:
#define VERSION 2
#if VERSION >= 2
std::cout << "This is version 2 or higher!" << std::endl;
#endif
This code checks the value of `VERSION`. If the condition is met, it compiles the line that follows, demonstrating how different code segments can be conditionally included based on the macro's value.
Conditional Checking with `#elif`
For scenarios requiring multiple conditions, `#elif` comes into play. It acts as an extension of `#if`, allowing for more intricate decision-making.
Example of `#elif`
Here’s an example:
#if VERSION == 1
std::cout << "This is version 1." << std::endl;
#elif VERSION == 2
std::cout << "This is version 2." << std::endl;
#endif
In this case, the output will depend on the defined value of `VERSION`, enabling nuanced control over the compiled code.

Ending Conditional Compilation with `#endif`
What is `#endif`?
The `#endif` directive signifies the end of a conditional compilation block. Failing to properly close these blocks can lead to confusion in your code structure and unexpected behavior during compilation.
Example of `#endif`
Here’s a complete example showing how it ties everything together:
#define FEATURE_ENABLED
#ifdef FEATURE_ENABLED
std::cout << "Feature is enabled." << std::endl;
#endif
In this snippet, the `#endif` marks the conclusion of the `#ifdef` block, ensuring that the conditional compilation is neatly organized.
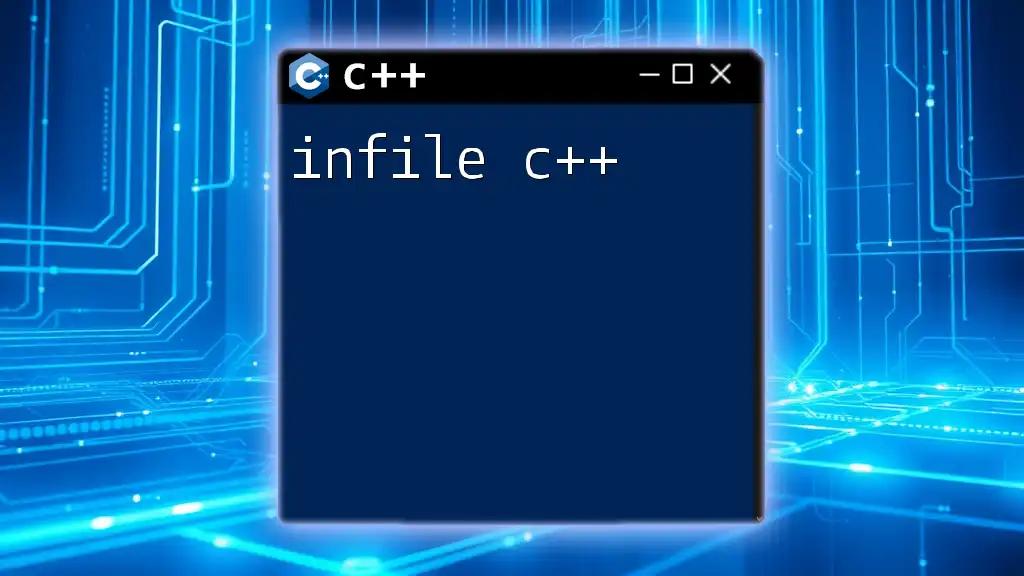
Best Practices for Using Conditional Compilation
Organizing Code Efficiently
To maintain clarity, organize preprocessor directives at the top of your files. Group related directives together, and consider using comments to indicate their purpose. This practice enhances readability and helps other developers understand the reasoning behind your compilation choices.
Avoiding Nested Directives
While nesting preprocessor directives is possible, it can quickly lead to confusion. Aim to limit nested directives in complex blocks, opting instead for clear, linear structures. This encourages maintainability and reduces potential errors.
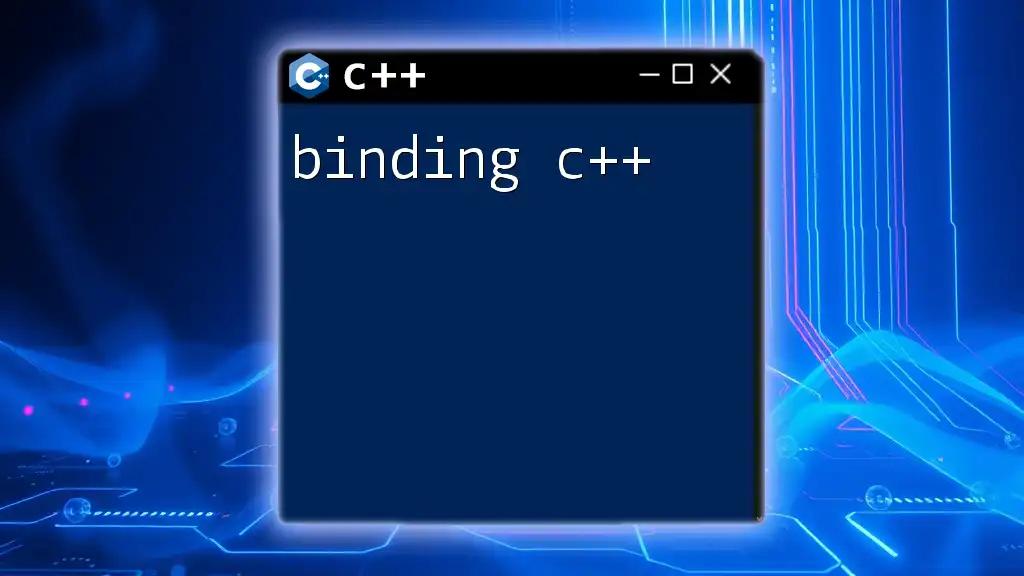
Conclusion
The power of conditional compilation in C++ through directives like `#define`, `#ifdef`, `#ifndef`, `#if`, `#elif`, and `#endif` provides programmers with the flexibility and control necessary for robust application development. Mastering these directives helps create efficient, manageable, and adaptable codebases. Always remember to practice and apply these concepts to solidify your understanding of the functionality and best practices surrounding these preprocessor tools.

Additional Resources
For those eager to delve deeper, consult the official C++ documentation and various online tutorials or courses that offer extensive learning opportunities. Familiarizing yourself with these directives through hands-on practice will ultimately enhance your programming proficiency and confidence.