In C++, vector indexing allows you to access elements of a vector using their position, with the first element at index 0.
Here’s a simple example demonstrating vector indexing:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {10, 20, 30, 40, 50};
std::cout << "The third element is: " << numbers[2] << std::endl; // Outputs: 30
return 0;
}
Understanding C++ Vector Basics
Definition and Syntax of C++ Vectors
Vectors are a part of the C++ Standard Library and are essentially dynamic arrays that can grow and shrink in size. They allow you to store a sequence of elements of the same type while providing the advantages of automatic memory management.
You can declare and initialize a vector as follows:
#include <iostream>
#include <vector>
std::vector<int> myVector = {1, 2, 3, 4, 5};
This initializes `myVector` with five integers.
Working with Vectors
One of the key features of vectors is their ability to dynamically resize, which means that they can automatically adjust their size when elements are added or removed. The following functions are commonly used to manipulate vectors:
- `push_back()`: Adds an element to the end of the vector.
- `pop_back()`: Removes the last element from the vector.
- `clear()`: Removes all elements from the vector.
Understanding these functions allows you to manage your vector effectively.
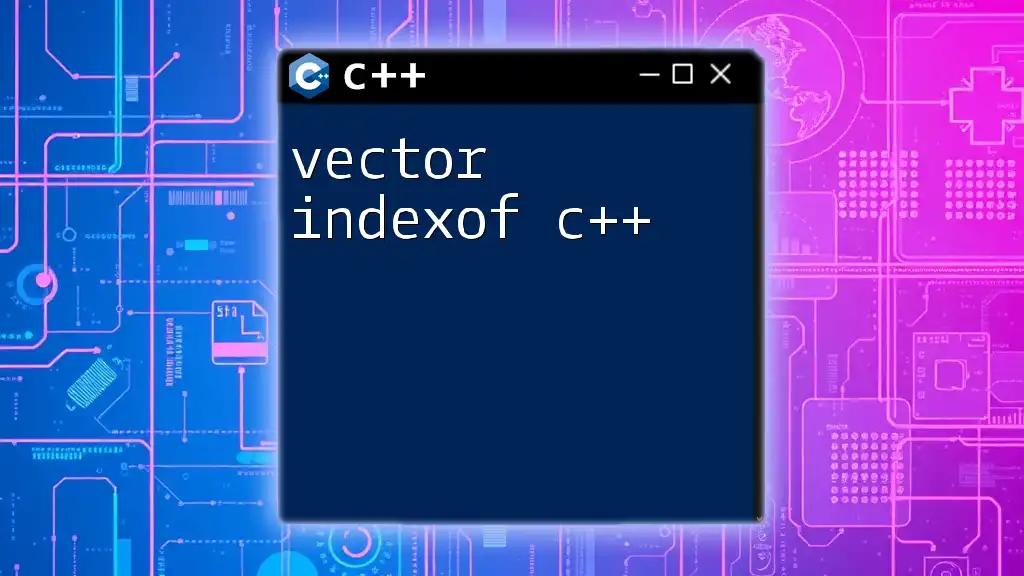
How Indexing Works in C++
What is Vector Indexing in C++?
Vector indexing refers to the method of accessing elements in a vector using their position or index. C++ utilizes zero-based indexing, meaning that the first element is accessed with an index of 0, the second element with an index of 1, and so forth.
Accessing Elements via Index
You can access elements directly via their index using square brackets. For instance:
std::cout << myVector[0]; // Outputs: 1
This retrieves the first element of `myVector`. Understanding this basic operation is crucial for effective vector manipulation.
Using the at() Method for Safe Access
For safer access to elements, especially when you're unsure whether the index falls within the valid range, the `at()` method can be utilized. This method checks for out-of-bounds access and throws an exception if the index is invalid.
std::cout << myVector.at(1); // Outputs: 2
Using `at()` can help prevent runtime errors due to invalid indexing.
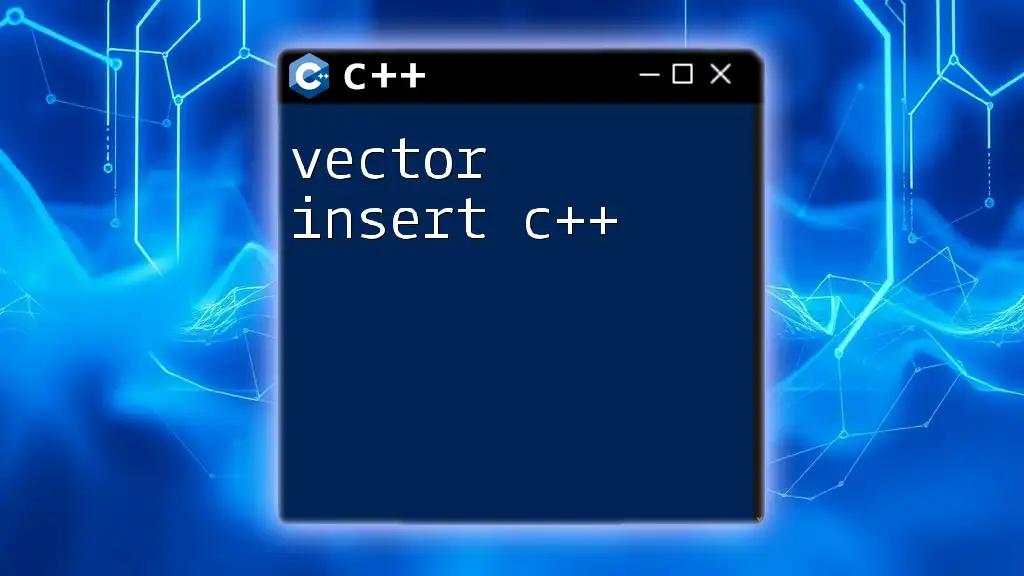
Modifying Elements through Indexing
Changing Values at Specific Indexes
Modifying vector elements is straightforward. You simply assign a new value to a specific index. Here’s how to change the third element:
myVector[2] = 10; // Changes the third element to 10
After executing this line, `myVector` will now contain `{1, 2, 10, 4, 5}`.
Using the at() Method to Modify Values
Just like you can access an element using the `at()` method, you can also modify it in a safe manner. This is particularly helpful in preventing errors when dealing with user input.
myVector.at(3) = 20; // Changes the fourth element to 20
Such practices help ensure the robustness of your code when dealing with unpredictable data.

Size and Capacity
Understanding Vector Size and Capacity
The two terms size and capacity are essential when working with vectors.
-
Size: This refers to the number of elements currently in the vector. It can be accessed using the `size()` method.
-
Capacity: This reflects the total number of elements that the vector can hold before it needs to reallocate memory. You can check the capacity using the `capacity()` method.
Knowing the difference helps in optimizing performance and memory usage.
Impact of Indexing on Size and Capacity
Whenever you add or remove elements from a vector, its capacity may change, which can affect indexing. Avoiding excessive calls to `resize()` will improve performance, especially in loops.
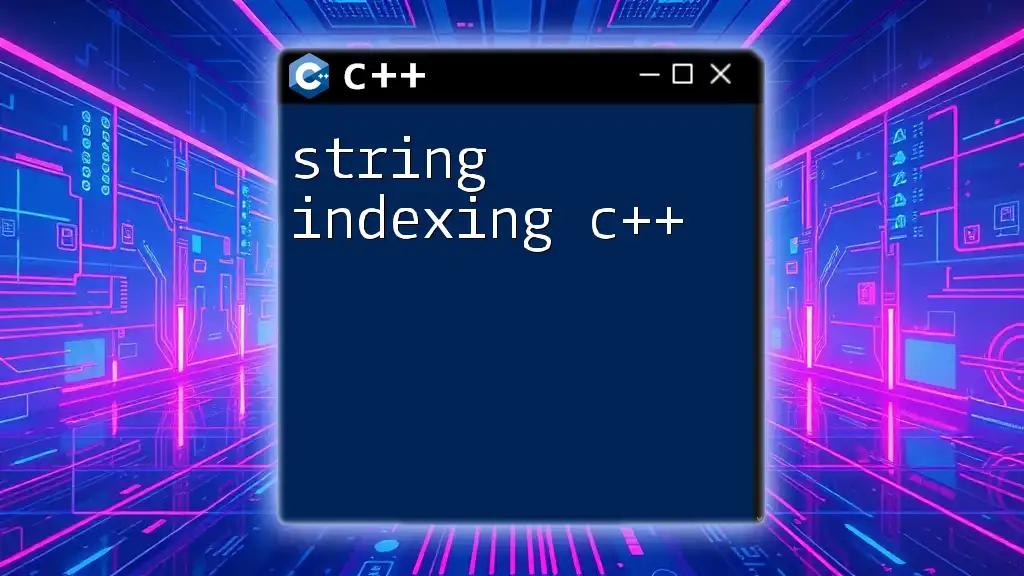
Common Pitfalls of Vector Indexing
Out of Bounds Access
Accessing elements outside the bounds of the vector can lead to undefined behavior. For example:
std::cout << myVector[10]; // Undefined behavior
Such mistakes can crash your program or lead to unexpected results, highlighting the importance of bounds checking.
Using std::vector::size() Safely
Always compare your index against the vector's size before accessing an element. This should be a habit you cultivate to prevent potential errors.

Performance Considerations
Time Complexity of Vector Indexing
C++ vector indexing provides constant time complexity, O(1), for access and modification. This efficiency is one of the primary reasons vectors are a preferred choice when you need quick access to elements.
Comparison with Other Data Structures
When compared to arrays and lists, vectors often offer better performance for dynamic storage needs. Understanding the strengths and weaknesses found in other data structures will help you make better programming decisions.
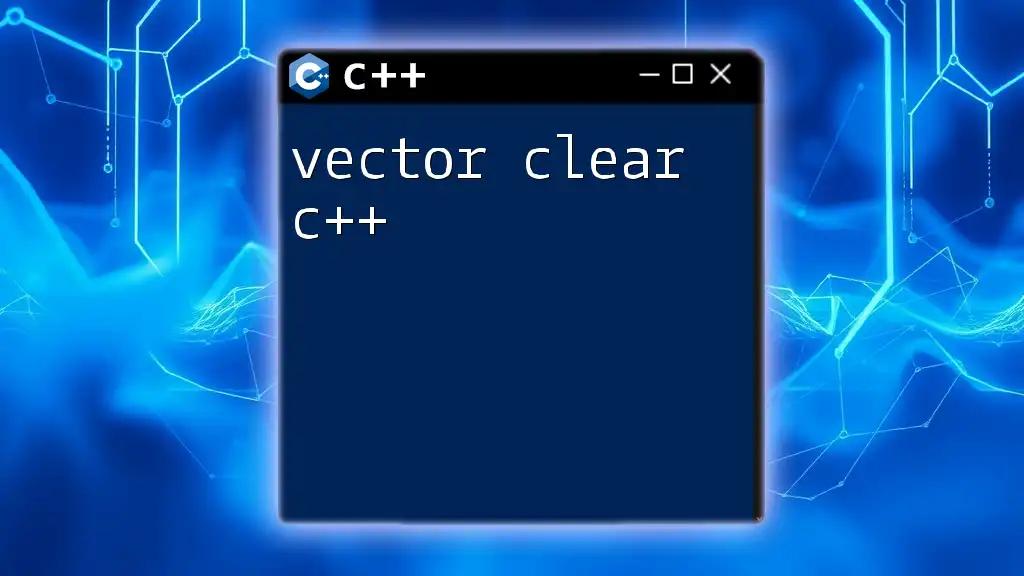
Practical Examples
Use Cases of Indexing in C++ Vectors
Vector indexing opens up several practical applications. For example, you might use a vector of integers to hold scores in a game:
std::vector<int> scores = {10, 20, 30, 40, 50};
for (size_t i = 0; i < scores.size(); ++i) {
std::cout << "Score " << i << ": " << scores[i] << std::endl;
}
This loop iterates over the vector, displaying each score. Practicing these fundamental operations will make you proficient in utilizing vectors.
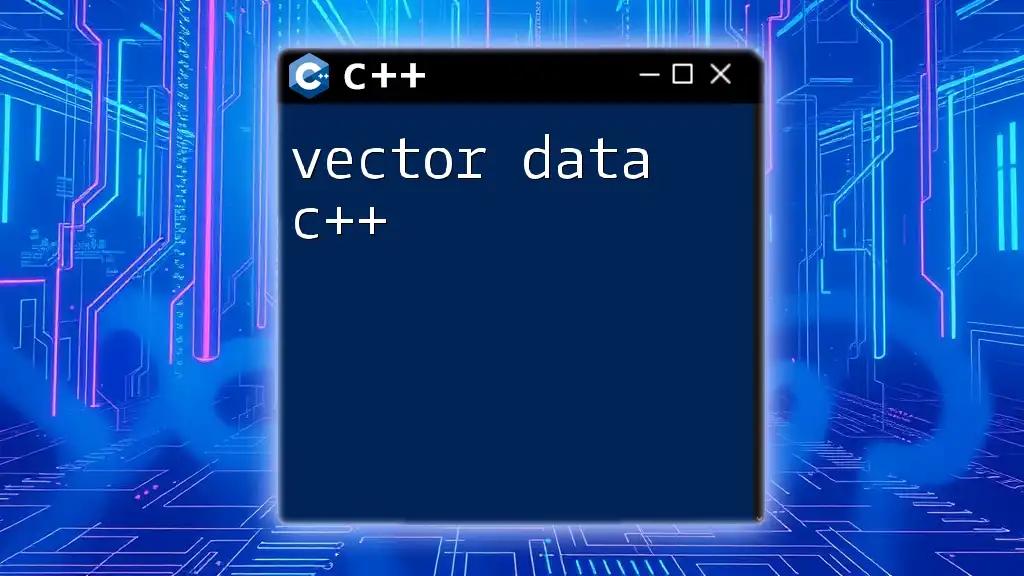
Best Practices for Vector Indexing
When to Use Vectors Over Other Containers
Vectors are an ideal choice when you require dynamic sizing, easy access via indexing, and if you know that you’ll be storing a collection of elements of the same type.
Safety Precautions While Indexing
Always prefer using `at()` for access and modification unless performance is critical and you are confident about bounds. This simple habit can save you a lot of debugging time in the long run.
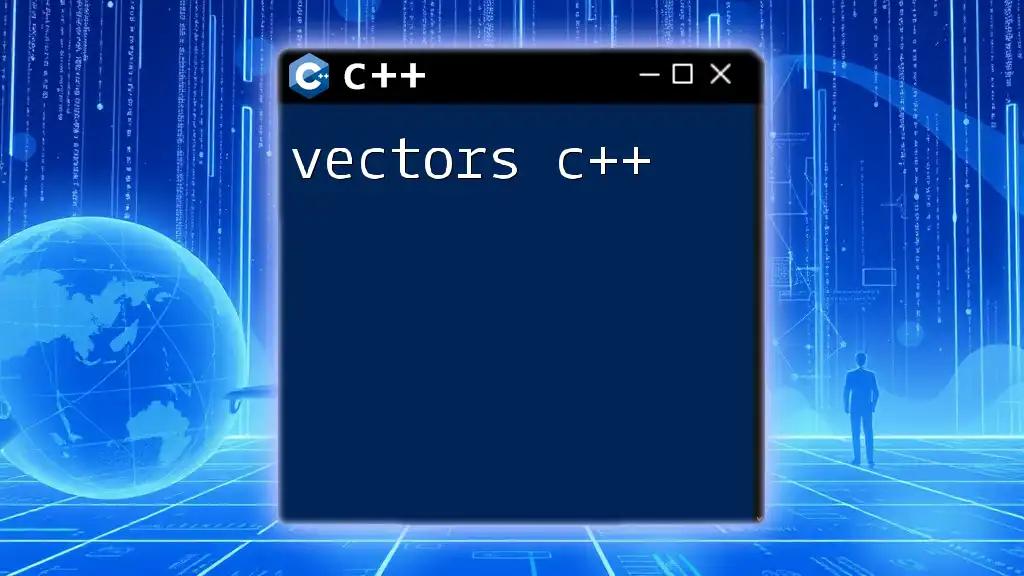
Conclusion
Vector indexing in C++ is a powerful concept that provides programmers with flexibility and efficiency when managing collections of data. Understanding how to properly use indexing will make you more proficient at writing robust C++ code. Make sure to practice these principles to deepen your understanding and improve your programming skills over time.