In C++, a vector of pairs allows you to store multiple pairs of values efficiently, providing a convenient way to manage related data items.
#include <iostream>
#include <vector>
#include <utility> // required for std::pair
int main() {
std::vector<std::pair<int, std::string>> vec = { {1, "one"}, {2, "two"}, {3, "three"} };
for (const auto& p : vec) {
std::cout << p.first << ": " << p.second << std::endl;
}
return 0;
}
What are Vectors in C++?
Definition of Vectors
In C++, vectors are dynamic arrays that can grow and shrink in size as needed. They are part of the standard template library (STL) and offer a range of functionalities that make them one of the most versatile data structures in C++. Unlike static arrays, which have a fixed size defined at compile-time, vectors manage memory automatically, providing flexibility and ease of use.
Basic Operations on Vectors
Vectors support various operations, making them an attractive choice for many programming scenarios:
-
Adding Elements: Using the `push_back()` method, you can easily append elements to the end of a vector.
-
Accessing Elements: Elements can be accessed directly using an index like `vec[0]`, akin to array usage.
-
Removing Elements: Use `pop_back()` to remove the last element or the `erase()` method to remove a specific element.
Here’s a simple example that demonstrates these fundamental operations:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec;
vec.push_back(10); // Adding an element
vec.push_back(20); // Adding another element
std::cout << "First element: " << vec[0] << std::endl; // Accessing an element
vec.pop_back(); // Removing the last element
std::cout << "New size after pop_back: " << vec.size() << std::endl; // Output the size
return 0;
}

What are Pairs in C++?
Definition of Pairs
A pair in C++ is a simple data structure provided by the standard template library that allows you to store two related data items together. Each pair consists of two elements, which can be of the same or different data types. The first element is accessed using `.first` and the second using `.second`.
Importance and Use-Cases for Pairs
Pairs are noteworthy for their capability to simplify the representation of complex data. They are particularly useful when you need to store two values that are intrinsically linked:
-
Associative data handling: Pairs can be used to represent key-value associations instead of creating separate structures.
-
Returning multiple values: Functions can use pairs to communicate more than one result succinctly.
Here’s a brief example illustrating the use of pairs:
#include <iostream>
#include <utility> // for std::pair
int main() {
std::pair<int, std::string> myPair(1, "One");
std::cout << "Key: " << myPair.first << ", Value: " << myPair.second << std::endl;
return 0;
}
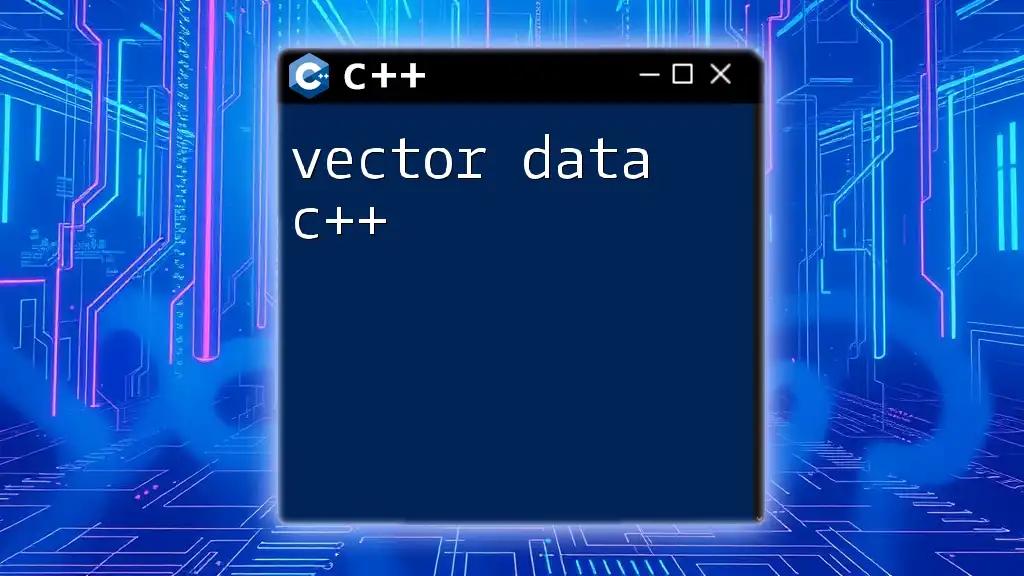
Combining Vectors and Pairs
Using Pairs with Vectors
When combining vectors and pairs, you can utilize the strengths of both data structures. Storing pairs in a vector allows you to manage pairs of related data flexibly while benefiting from dynamic sizing features of vectors.
Example Code Snippet
Here's an example that shows how to store pairs within a vector:
#include <iostream>
#include <vector>
#include <utility> // for std::pair
int main() {
std::vector<std::pair<int, std::string>> vecPairs;
vecPairs.push_back(std::make_pair(1, "First"));
vecPairs.push_back(std::make_pair(2, "Second"));
for (const auto& p : vecPairs) {
std::cout << p.first << ": " << p.second << std::endl;
}
return 0;
}
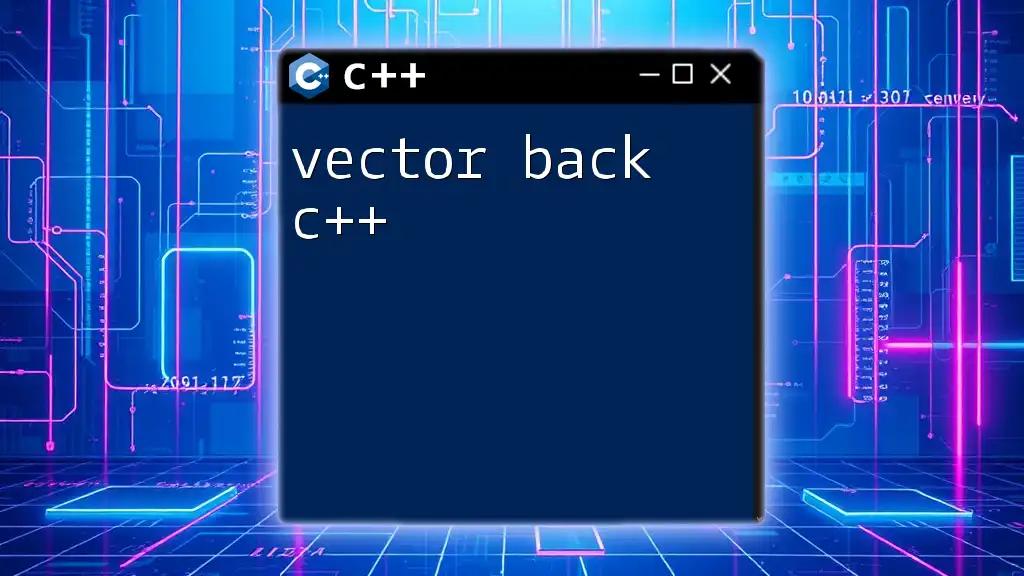
Working with Vector of Pairs
Accessing Elements
Accessing elements in a vector of pairs is straightforward. You can use indexed access just like with a regular vector, but you'll be dealing with pairs instead of single values. It’s also recommended to use const references in the iteration to prevent unnecessary copying.
Modifying Elements
You can directly modify specific elements within pairs stored in a vector. Accessing a pair allows you to set its `.first` or `.second` directly.
Here’s a code snippet demonstrating how to modify elements in a vector of pairs:
#include <iostream>
#include <vector>
#include <utility>
int main() {
std::vector<std::pair<int, std::string>> vecPairs = {
{1, "One"}, {2, "Two"}, {3, "Three"}
};
vecPairs[1].second = "Two Updated"; // Modifying a pair's second element
for (const auto& p : vecPairs) {
std::cout << p.first << ": " << p.second << std::endl;
}
return 0;
}
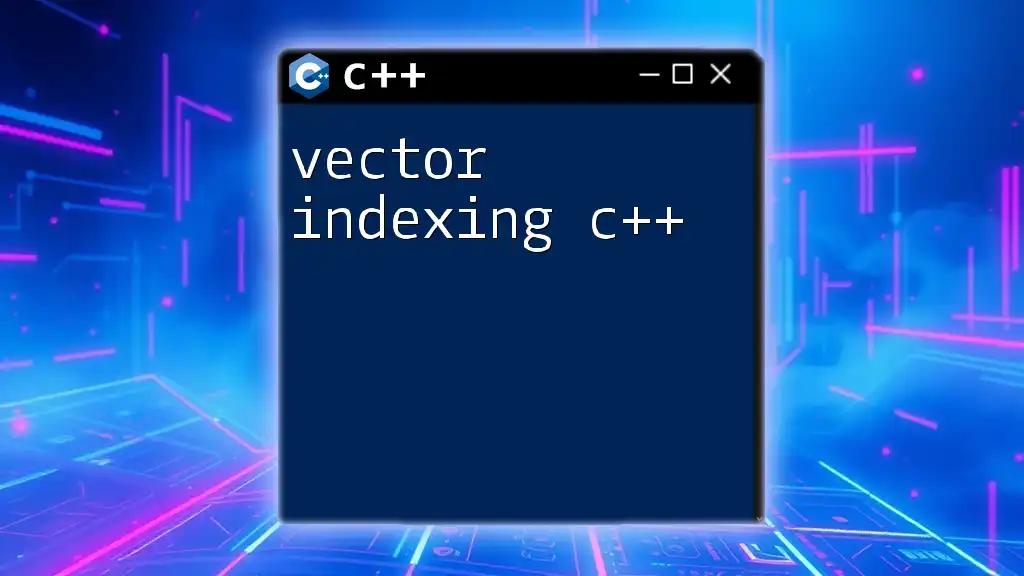
Advantages of Using Pair Vectors
Enhanced Data Organization
Using vectors to store pairs allows you to logically group data together. This grouping enhances data organization and makes your code easier to understand and maintain.
Simplified Code Logic
Using pair vectors can minimize the complexity of your code. Instead of creating custom data structures for simple associations, pair vectors consolidate related data, leading to cleaner and more readable code.
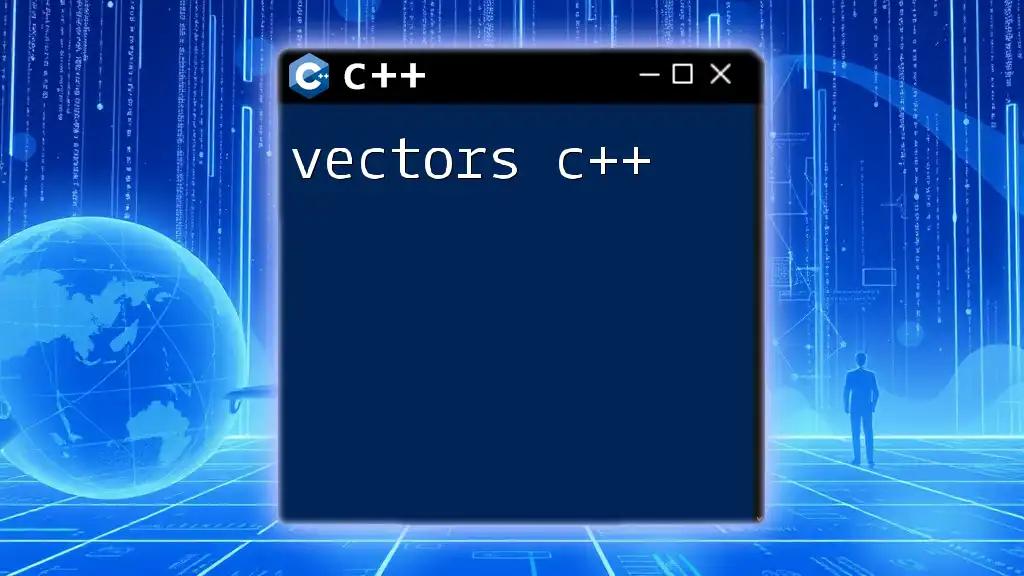
Common Use-Cases for Vector Pairs
Storing Key-Value Pairs
Vector pairs are often used for handling key-value data, such as in associative containers like maps or dictionaries. This simplifies operations wherein both key and value need to be processed together.
Pairing Values with Indices
Another prevalent use-case is in scenarios such as inventory systems or game item management, where items are paired with their indexes or identifiers for easier tracking.
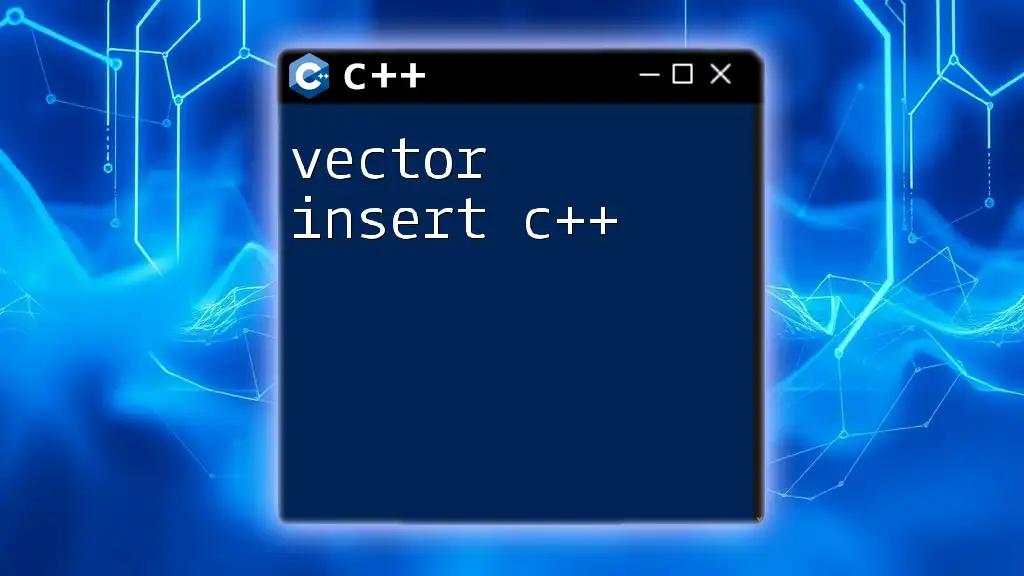
Conclusion
By understanding the vector pair in C++, you can efficiently manage related data while leveraging the benefits that both vectors and pairs bring to your applications. This powerful combination promotes cleaner code and good data organization practices. Embrace these structures in your coding practice to enhance your programming capabilities.

Additional Resources
For more learning, consider exploring the official C++ documentation and engaging in online platforms where you can hone your skills through practice.

FAQs
What is the difference between a pair and a struct?
While both pairs and structs allow grouping of data, pairs are limited to exactly two elements and are typically used for simple associations. In contrast, structs can contain multiple members of different types and are more flexible for complex data structures.
Can I use vectors of pairs with custom data types?
Yes, vectors can store pairs of any types, including custom data types. Ensure that your custom types are compatible with the STL and provide necessary constructors and operators.
How do I sort a vector of pairs?
You can sort a vector of pairs using the `std::sort()` function, specifying a custom comparator to define the sorting logic based on either the first or second element of the pairs, or even both, depending on your requirement.