In C++, the `vector` class from the Standard Template Library (STL) allows you to create dynamic arrays that can resize automatically when elements are added or removed, and it is included by adding `#include <vector>` at the top of your code.
Here's a simple code snippet demonstrating how to include and use a vector:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
What is a Vector in C++?
Definition of a Vector
In C++, a vector is a part of the Standard Template Library (STL) that represents a dynamic array capable of resizing itself when an element is added or removed. Unlike traditional arrays, vectors can grow and shrink in size, which makes them a preferred choice for many applications where the number of elements is not known in advance or may change over time.
Benefits of Using Vectors
Vectors offer numerous advantages over static arrays:
- Dynamic Size: Vectors automatically manage memory, enabling you to add or remove elements without manual intervention.
- Rich Member Functions: With built-in methods, vectors simplify complex operations such as insertion, deletion, and sorting.
- Range Checking: Vector access methods provide bounds checking, reducing the risk of out-of-bounds errors compared to raw arrays.
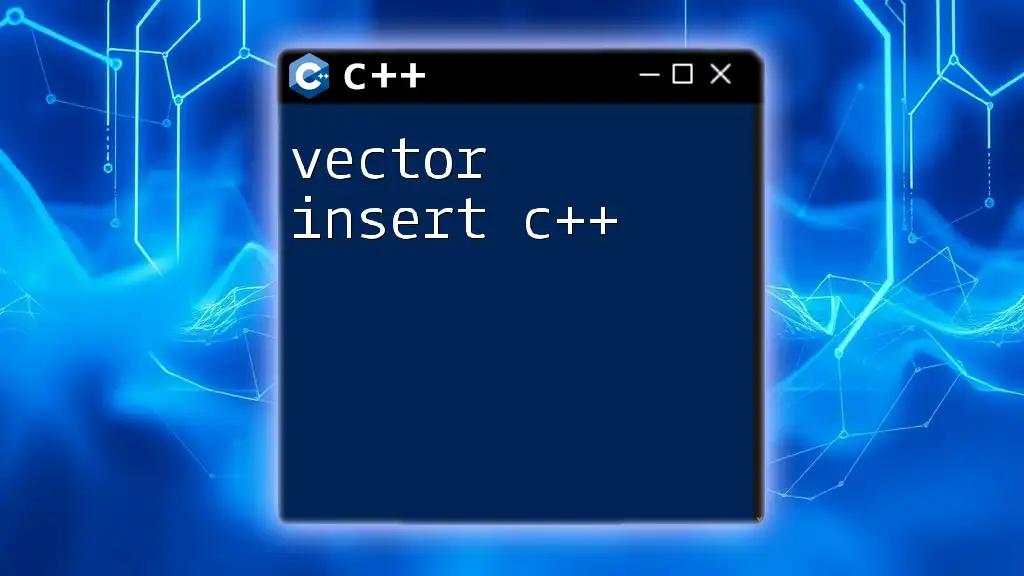
How to Use Vectors in C++
Including the Vector Header
To use vectors in your C++ programs, you need to include the vector library. This is performed using the following include statement:
#include <vector>
This line of code should be placed at the top of your source file, enabling you to leverage all the functionalities provided by the vector class.
Initializing a Vector
Creating a Vector
Initializing a vector in C++ can be done in several ways. Here are examples of the different initialization methods:
std::vector<int> vec1; // Creates an empty vector
std::vector<int> vec2(5); // Creates a vector of size 5 with default values (0)
std::vector<int> vec3{1, 2, 3, 4, 5}; // Creates and initializes a vector with specified values
Each of these methods allows you to declare vectors to suit your needs, whether you want an empty vector or a pre-sized one filled with default values.
Reserve Capacity
Managing memory efficiently is essential in performance-critical applications. You can reserve capacity to avoid multiple allocations when adding elements:
std::vector<int> vec;
vec.reserve(10); // Reserves space for 10 elements
This method preallocates memory, thus minimizing the overhead of reallocating when the vector grows.
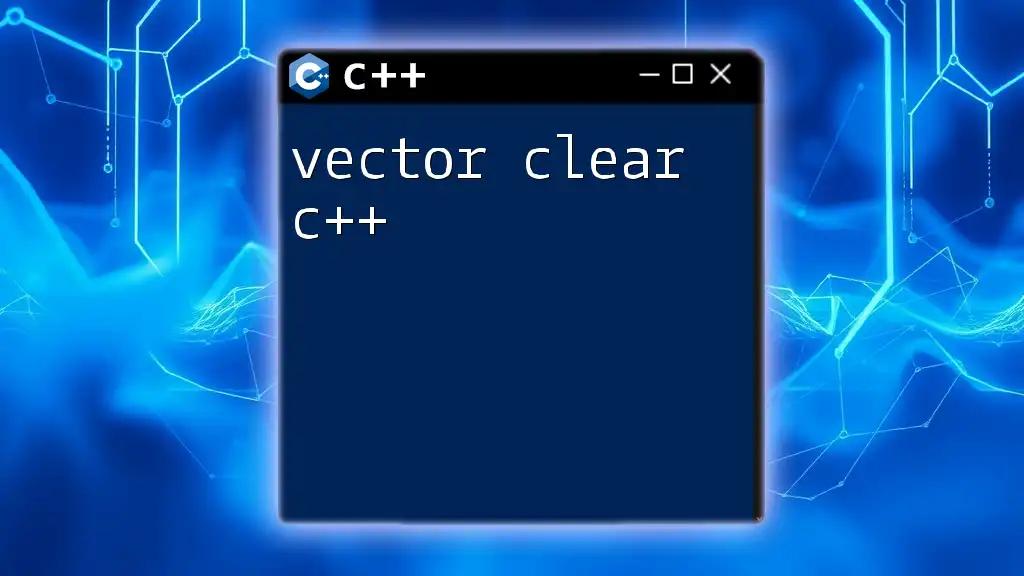
Common Operations on Vectors
Adding Elements
Vectors provide various methods to add elements:
- `push_back()`: Adds an element to the end of the vector.
- `emplace_back()`: Constructs an element in place at the end, allowing for better performance.
- `insert()`: Inserts an element at a specific position.
Here are examples for each:
vec.push_back(6); // Adds '6' to the end of the vector
vec.emplace_back(7); // Constructs '7' in place at the end
vec.insert(vec.begin() + 1, 8); // Inserts '8' at index 1
These methods offer flexibility in managing the vector's contents.
Accessing Elements
You can access vector elements using index-based access or iterators. Both methods are effective:
int firstElement = vec[0]; // Index-based access
for (auto it = vec.begin(); it != vec.end(); ++it) {
std::cout << *it << " "; // Iterator access, prints all elements
}
Using iterators provides a way to traverse the vector without concerns about invalid indexes.
Removing Elements
Removing elements from vectors can be accomplished with:
- `pop_back()`: Removes the last element.
- `erase()`: Removes an element at a specified position or range.
- `clear()`: Empties the entire vector.
Code snippets demonstrating these methods include:
vec.pop_back(); // Removes the last element from the vector
vec.erase(vec.begin() + 1); // Removes the element at index 1
vec.clear(); // Removes all elements from the vector
These operations provide robust options for managing vector contents.
Checking Size and Capacity
Understanding the size and capacity of a vector is crucial for performance optimization. You can use the `size()` method to get the number of elements and `capacity()` to check the allocated size:
std::cout << "Size: " << vec.size() << ", Capacity: " << vec.capacity();
Using these methods can help you make informed decisions when managing vector storage.
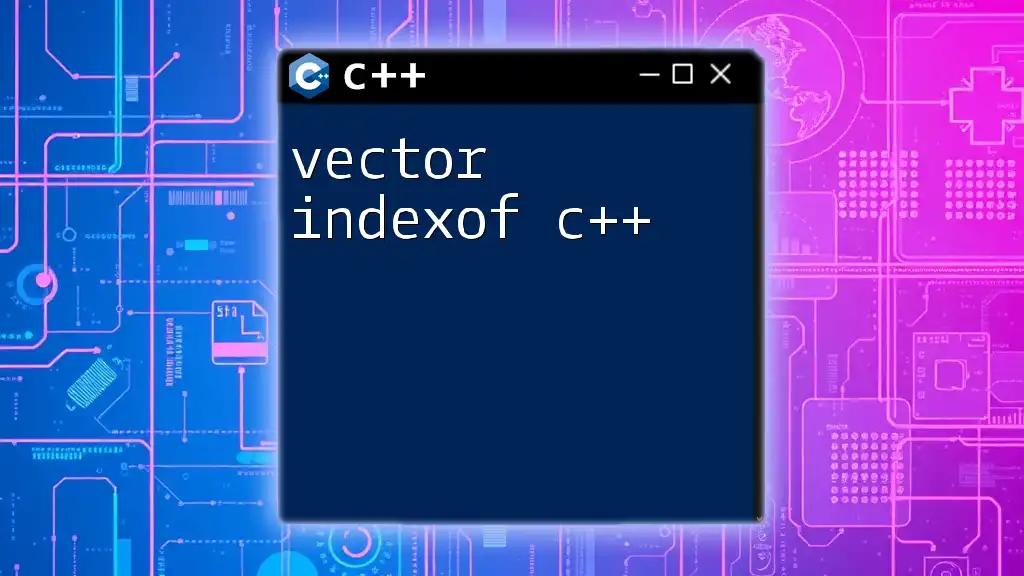
Important Functions and Attributes of Vectors
Functions Specific to Vectors
Vectors in C++ provide several specialized member functions:
- `front()`: Returns the first element.
- `back()`: Returns the last element.
- `data()`: Returns a pointer to the underlying array.
Here are examples for each function:
int first = vec.front(); // Retrieves the first element
int last = vec.back(); // Retrieves the last element
int* array = vec.data(); // Allows access to the underlying array
These functions enable straightforward access to the most critical elements within the vector.
Attributes of Vector
Beyond functions, vectors also offer essential attributes:
- `empty()`: Returns `true` if the vector has no elements.
- `shrink_to_fit()`: Resizes the vector to fit its contents, potentially freeing unused memory.
- `swap()`: Exchanges the contents of two vectors.
Example uses of these attributes include:
if(vec.empty()) { std::cout << "Vector is empty"; }
vec.shrink_to_fit(); // Reduces memory usage to fit the size of the vector
These attributes contribute to efficient memory usage and can optimize performance across applications.
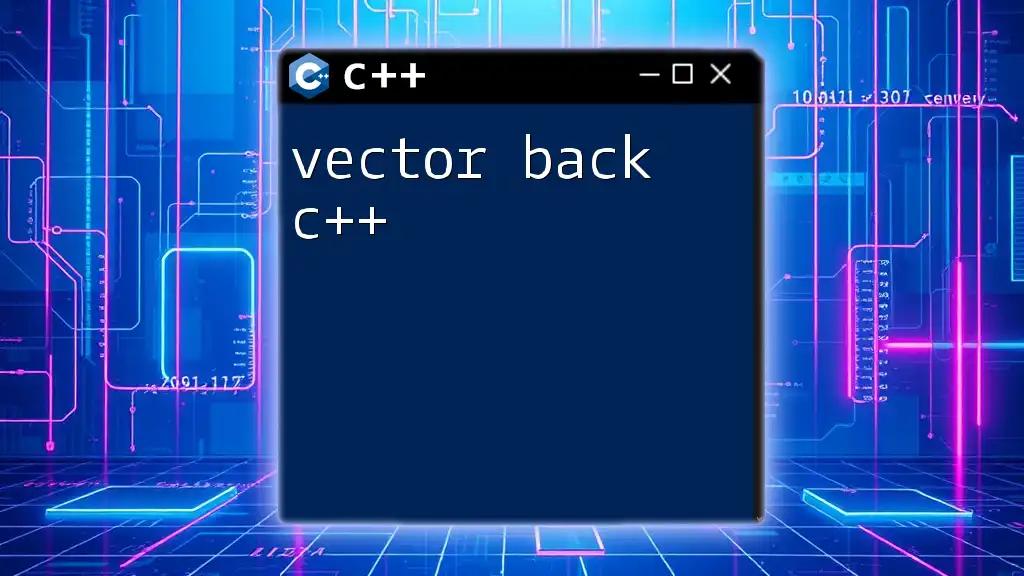
Conclusion
Understanding the vector include c++ and mastering vector functionalities are essential skills for any C++ programmer. Vectors simplify complex data management tasks, providing flexibility and robustness in handling dynamic collections.
Practicing with these operations is crucial, as they integrate seamlessly into various coding scenarios. Embrace the power of vectors in your C++ projects, and remember that learning is a continuous journey.
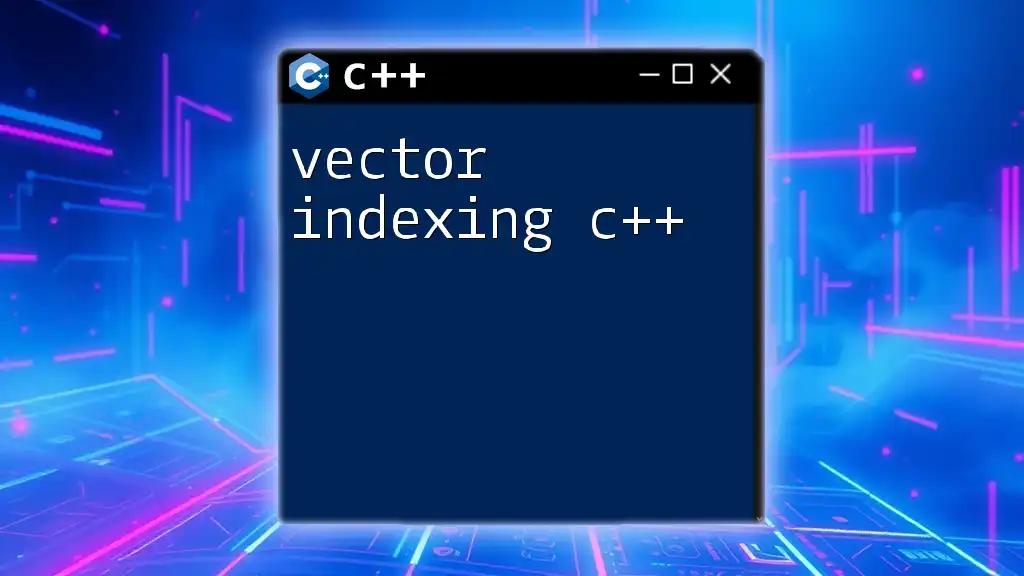
Call to Action
If you found this guide useful, consider subscribing for more tutorials covering various C++ topics. Also, follow our company on social media for constant updates, tips, and insights into mastering C++. Happy coding!