In C++, you can sort a vector using the `std::sort` function from the `<algorithm>` library, which rearranges the elements in ascending order. Here's a concise example:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {5, 3, 8, 1, 2};
std::sort(vec.begin(), vec.end());
for (int val : vec) std::cout << val << " ";
return 0;
}
Understanding Vectors in C++
What is a Vector?
In C++, a vector is part of the Standard Template Library (STL) and is essentially a dynamic array that can resize itself when elements are added or removed. Vectors have the ability to store a collection of elements, provide flexibility in their size, and offer functions for manipulating stored data.
Advantages of using vectors over arrays include:
- Dynamic size: Vectors can grow and shrink automatically.
- Ease of use: Vectors come with built-in functions for common operations.
- Memory management: Vectors handle their own memory allocation and deallocation, simplifying code for developers.
To declare and initialize a vector, you can do the following:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5}; // Initializing a vector with values
return 0;
}
Basic Operations with Vectors
Vectors support several basic operations that make them powerful data structures. You can add elements using the `push_back()` function, remove elements with `pop_back()`, and access elements using indices.
Here's a code snippet to demonstrate these operations:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers;
// Adding elements
for (int i = 0; i < 5; ++i) {
numbers.push_back(i + 1); // Add 1, 2, 3, 4, 5
}
// Accessing elements
for (int i = 0; i < numbers.size(); ++i) {
std::cout << "Element at index " << i << ": " << numbers[i] << std::endl;
}
// Removing the last element
numbers.pop_back(); // Removes 5
return 0;
}
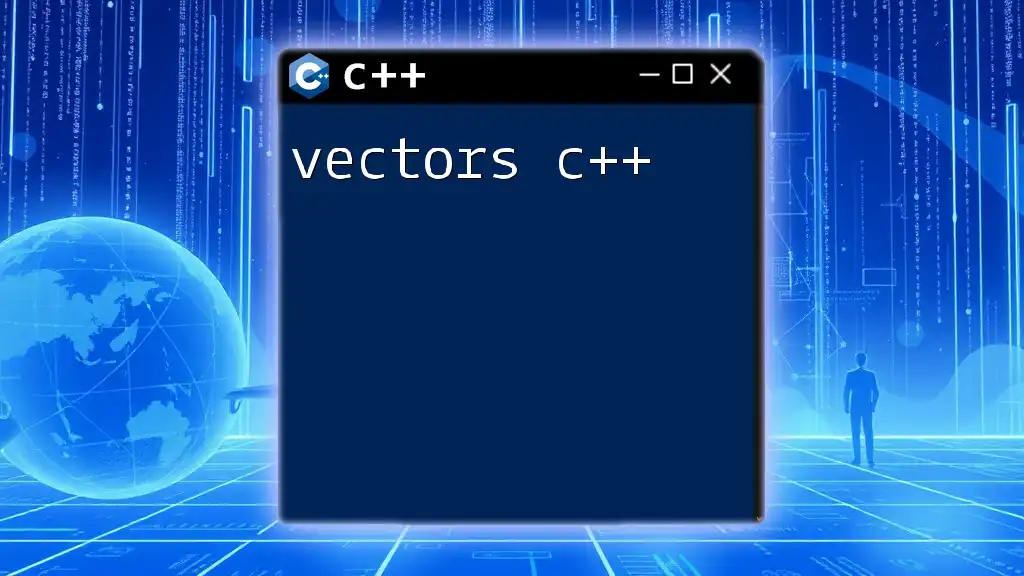
Sorting in C++
What is Sorting?
Sorting involves rearranging elements in a specific order, which is foundational in programming. It enhances data organization and retrieval efficiency. Common sorting applications include preparing data for search operations, data analysis, and managing databases.
Sorting Functions in C++
Introduction to std::sort
C++ provides the `std::sort` function from the `<algorithm>` header, which is a powerful sorting algorithm. It uses an efficient sorting algorithm (typically a variation of quicksort) and can sort any range of elements.
To utilize `std::sort`, ensure you include the header:
#include <algorithm>
Syntax of std::sort
The basic syntax of `std::sort` is:
std::sort(begin_iterator, end_iterator);
This function sorts elements in the range defined by `begin_iterator` and `end_iterator`.
Here's a simple usage example:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {4, 2, 5, 1, 3};
std::sort(numbers.begin(), numbers.end()); // Sort in ascending order
for (int n : numbers) {
std::cout << n << " "; // Output: 1 2 3 4 5
}
return 0;
}
Sorting a Vector
Sorting in Ascending Order
To sort a vector in ascending order, you simply use `std::sort()` as shown above. It's a straightforward method that works efficiently for most datasets.
Sorting in Descending Order
If you wish to sort a vector in descending order, you can provide a custom comparison function or use a lambda function:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {4, 2, 5, 1, 3};
std::sort(numbers.begin(), numbers.end(), std::greater<int>()); // Sort in descending order
for (int n : numbers) {
std::cout << n << " "; // Output: 5 4 3 2 1
}
return 0;
}
Custom Sorting with Comparators
Custom comparators allow you to define your own sorting logic. This is especially useful when dealing with complex data types. For example, consider a structure representing a student:
#include <iostream>
#include <vector>
#include <algorithm>
#include <string>
struct Student {
std::string name;
int score;
};
// Custom comparator to sort by score
bool compareScore(const Student &a, const Student &b) {
return a.score < b.score; // Sort by score
}
int main() {
std::vector<Student> students = {{"Alice", 85}, {"Bob", 95}, {"Charlie", 80}};
std::sort(students.begin(), students.end(), compareScore);
for (const auto &student : students) {
std::cout << student.name << ": " << student.score << std::endl;
}
return 0;
}
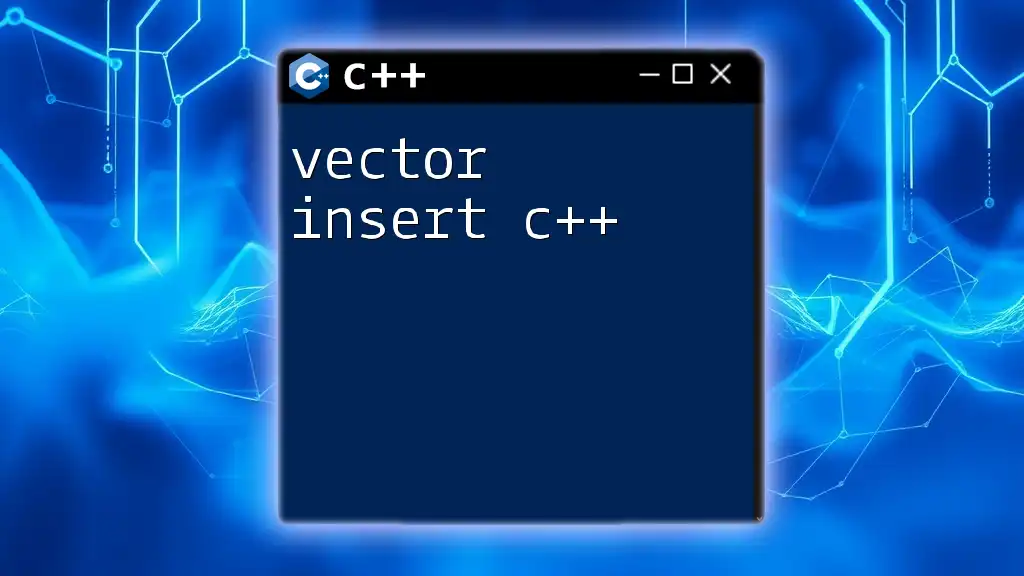
Alternative Sorting Methods
Using std::stable_sort
While `std::sort` performs efficiently, it might not maintain the relative order of equal elements. To achieve stability, you can use `std::stable_sort`, which ensures that elements with equivalent keys retain their original order:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<std::pair<std::string, int>> students = {{"Alice", 80}, {"Bob", 80}, {"Charlie", 90}};
std::stable_sort(students.begin(), students.end(), [](const auto &a, const auto &b) {
return a.second < b.second; // Sorting based on score
});
for (const auto &student : students) {
std::cout << student.first << ": " << student.second << std::endl;
}
return 0;
}
Using std::partial_sort
`std::partial_sort` rearranges elements such that the smallest N elements are sorted while leaving the rest of the elements in an unspecified order. This is useful when you want to find the top N elements without fully sorting a larger dataset:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {8, 1, 6, 3, 9, 4, 5, 7};
std::partial_sort(numbers.begin(), numbers.begin() + 3, numbers.end()); // Sort top 3 elements
for (int n : numbers) {
std::cout << n << " "; // Output: 1 3 4 8 9 6 5 7 (top 3 sorted, rest unknown)
}
return 0;
}
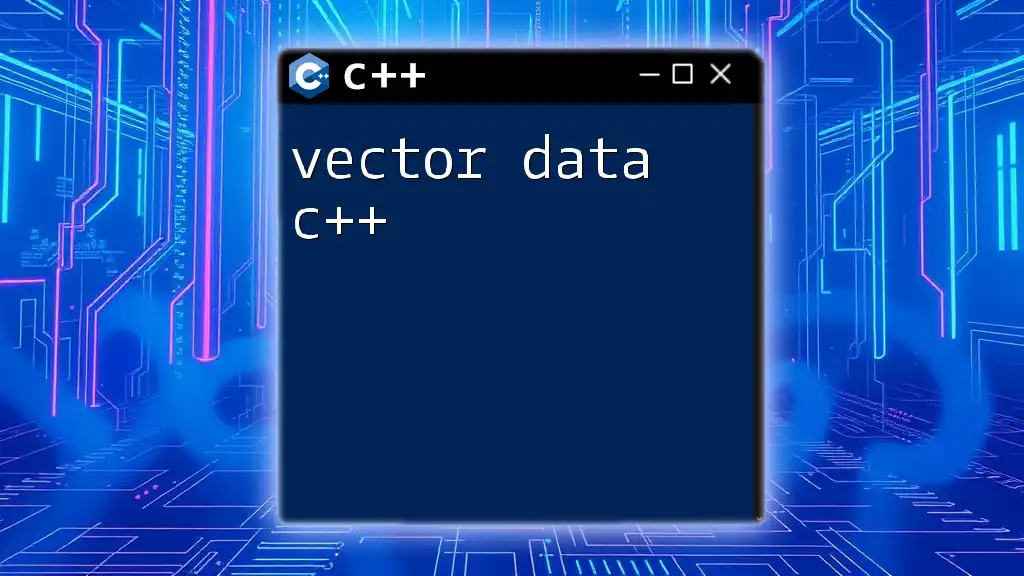
Performance Considerations
Time Complexity of Sorting Algorithms
Understanding the performance of sorting algorithms is crucial. The time complexity for `std::sort` is typically O(n log n) for average and worst cases, which is efficient for most uses. However, in scenarios with an already sorted vector, it can approach O(n).
For `std::stable_sort`, the time complexity is O(n log n), but it may be slower due to additional overhead to maintain stability.
Choosing the Right Sorting Method
When selecting a sorting method, consider the following:
- Size of Dataset: For small datasets, simple methods like `std::sort` are sufficient. For larger datasets, prefer `std::stable_sort`.
- Type of Data: If maintaining relative order among equal elements is essential, use `std::stable_sort`.
- Sorting Criteria: Use custom comparators for complex data types.
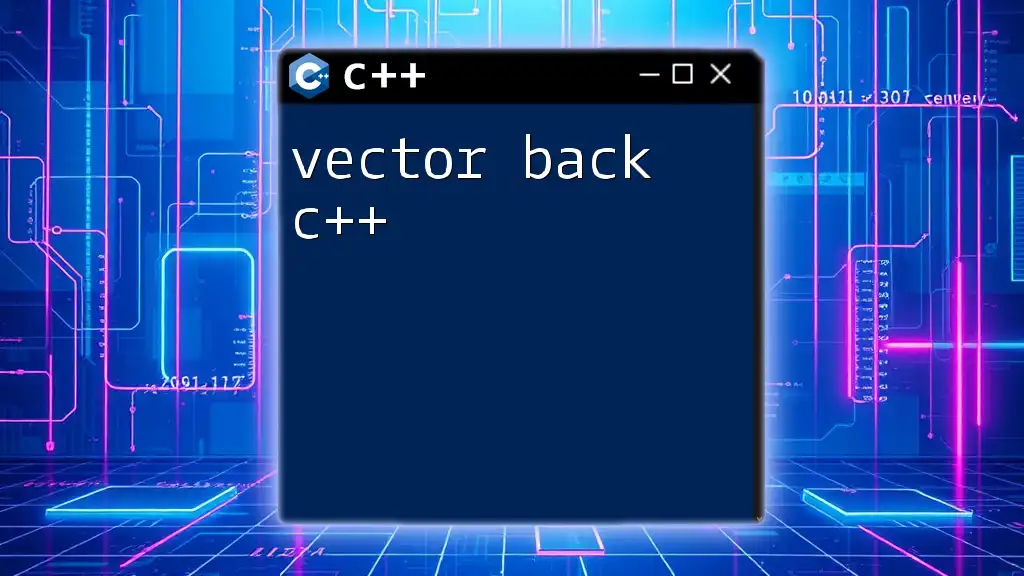
Conclusion
In this guide, we explored the mechanics of sorting vectors in C++ using `std::sort` and alternative sorting methods. Armed with this knowledge, you can efficiently organize your data and streamline your programming tasks. Remember to practice these examples to solidify your understanding of vector sorting techniques in C++.
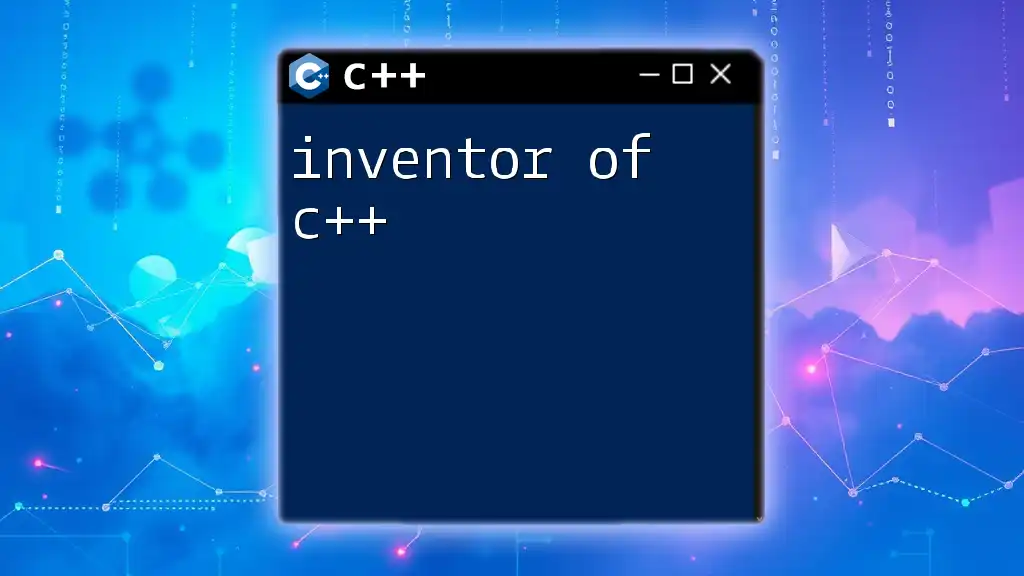
Additional Resources
Recommended Reading
- C++ Official Documentation
- "The C++ Programming Language" by Bjarne Stroustrup
- Online courses on platforms like Coursera and Udemy focusing on C++ and STL.
Practice Exercises
- Sort a vector of strings based on length and alphabetical order.
- Use `std::partial_sort` to find the top 5 highest salaries from a list of employee records.
- Create a custom data structure and implement various sorting methods on it.
If you have further questions, there are numerous forums dedicated to C++ where you can seek help and community support. Happy coding!